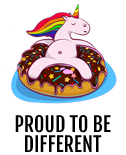
Amy":x9ip67wh said:Because fuck I'm never gonna makegame
Good. Good. Good. Good. Good.HiPoyion":l34z8lrq said:Then again we're doing computer science so we're thinking of games as a computer science problem and not a story telling one.
int mousePosX;
int mousePosY;
Object touchObject = null; // Initially nothing is being touched by the mouse
void OnUpdate() {
if ( IsMouseDown() == true && touchObject == null ) {
// Record starting mouse position
mousePosX = GetMouseX();
mousePosY = GetMouseY();
touchObject = HitTest( mousePosX, mousePosY ); // What are we touching?
} else if ( touchObject != null ) {
// We are touching something since last frame
if ( IsMouseDown() == false ) { // Touching something, but the mouse is no longer down
int releaseVelocityX = GetMouseX() - mousePosX;
int releaseVelocityY = GetMouseY() - mousePosY;
if ( VectorLength( releaseVelocityX, releaseVelocityY ) > <span style="color: #0000dd;">10 ) { // Release velocity above 10px/frame
touchObject.OnSwipe( releaseVelocityX, releaseVelocityY ); // Released at a high velocity is probably a swipe
} else {
touchObject.OnClick(); // Released at a low velocity is probably a click
}
touchObject = null; // Let go of whatever we were touching
} else { // MouseDown == true
touchObject.OnMove( GetMouseX() - mousePosX, GetMouseY() - mousePosY ); // Haven't let go of object, so move it by the vector
// Record new mouse position for this frame
mousePosX = GetMouseX();
mousePosY = GetMouseY();
}
}
}
Xilef":2ydmgtfg said:Keep at it potion it's these kinds of things that teach you more than anything else. Swipe gesture is pretty tough to code, I think the first time I did it was in my placement job - just need to break it down to its components.
Pseudo code for a shitty object drag, click and fling detection system when you only have information for the mouse x/y and if it is pressed or not. This is frame time dependant (which is bad).
C++:int mousePosX; int mousePosY; Object touchObject = null; // Initially nothing is being touched by the mouse void OnUpdate() { if ( IsMouseDown() == true && touchObject == null ) { // Record starting mouse position mousePosX = GetMouseX(); mousePosY = GetMouseY(); touchObject = HitTest( mousePosX, mousePosY ); // What are we touching? } else if ( touchObject != null ) { // We are touching something since last frame if ( IsMouseDown() == false ) { // Touching something, but the mouse is no longer down int releaseVelocityX = GetMouseX() - mousePosX; int releaseVelocityY = GetMouseY() - mousePosY; if ( VectorLength( releaseVelocityX, releaseVelocityY ) > <span style="color: #0000dd;">10 ) { // Release velocity above 10px/frame touchObject.OnSwipe( releaseVelocityX, releaseVelocityY ); // Released at a high velocity is probably a swipe } else { touchObject.OnClick(); // Released at a low velocity is probably a click } touchObject = null; // Let go of whatever we were touching } else { // MouseDown == true touchObject.OnMove( GetMouseX() - mousePosX, GetMouseY() - mousePosY ); // Haven't let go of object, so move it by the vector // Record new mouse position for this frame mousePosX = GetMouseX(); mousePosY = GetMouseY(); } } }
def event_resolve(events_list, sprites):
moving = False
if events_list[0]:
mouse_click_position = mouse_tracking.get_pixel_at_click() # store players click location to compare to the release location
while not events_list[1]: # We then wait for the player to release the mouse button
events_list = event_loop.get_events(events_list)
if events_list[1]:
mouse_release_position = mouse_tracking.get_pixel_at_lift() # stores players release location for comparison
direction = mouse_tracking.get_player_facing_direction(mouse_click_position, mouse_release_position) # Compares the values to give the player a direction to move
moving = True # We consider the player to be moving with the intention of stopping any input during that time.
return direction, moving
if events_list[2]:
terminate()
EVT_MOUSE_DOWN = 0
EVT_MOUSE_UP = 1
EVT_QUIT = 2
moving = False # This needs to be held outside of the event_resolve logic
def event_resolve(events_list, sprites):
if events_listEVT_MOUSE_DOWN]:
if not moving: # Logically this can be combined with the if statement
moving = True
mouse_click_position = mouse_tracking.get_pixel_at_click()
elif events_listEVT_MOUSE_UP]:
if moving: # Logically this should always be true (can't lift the mouse if it wasn't already down)
moving = False
mouse_release_position = mouse_tracking.get_pixel_at_lift()
direction = mouse_tracking.get_player_facing_direction(mouse_click_position, mouse_release_position)
# At this point direction = swipe movement vector
return direction, True
elif events_listEVT_QUIT]:
terminate()