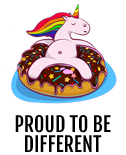
#==============================================================================
# ** Letter by Letter Message Window
#------------------------------------------------------------------------------
# Slipknot (dubealex.com/asylum)
# Version 1.11
# September 8, 2006
#------------------------------------------------------------------------------
# Thanks to:
# - Dubealex, for some of the features.
# - RPG Advocate, for the hexadecimal color.
#==============================================================================
#------------------------------------------------------------------------------
# SDK log
#------------------------------------------------------------------------------
SDK.log('Letter by Letter Message Window', 'Slipknot', '1.11', '08.09.06')
#------------------------------------------------------------------------------
# Begin SDK Enabled Check
#------------------------------------------------------------------------------
if SDK.state('Letter by Letter Message Window')
# Loads the maps' names
$data_map_infos = load_data('Data/MapInfos.rxdata')
#==============================================================================
# ** Game_Message
#------------------------------------------------------------------------------
# This class handles the message data
#==============================================================================
class Game_Message
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_accessor :letter_by_letter, :speed, :can_skip, :height, :font,
:sound_enable, :sound, :path, :face_rect, :fit, :skin, :nb_skin,
:nbyo, :opacity, :shadow, :outline, :pause, :autoclose_frames
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
# Letter by letter mode
@letter_by_letter = true
# Lettter by letter mode's speed
@speed = 1
# If this option is false, the player can't skip the message
@can_skip = true
# Height of each line, used within the fit or above event options
@height = 32
# Always resize the message
@fit = true
# Font for the mesage text
@font = Font.default_name
# Sound enable
@sound_enable = false
# Sound for letter by letter, ['filename', vol]
@sound = ['032-Switch01', 80]
# Folder for the message pictures
@path = 'Graphics/Pictures/'
# Face rect (only the last two numbers are used)
@face_rect = Rect.new(0, 0, 96, 96)
# Skin for the message window, nil = default
@skin = nil
# Skin for the name box, nil = default
@nb_skin = nil
# Name box y offset
@nbyo = 20
# Message window's opacity
@opacity = 160
# Outline text
@outline = false
# Shadow text
@shadow = true
# Show or not the pause graphic
@pause = true
# Frames before the message autoclose
@autoclose_frames = 8
end
end
#==============================================================================
# ** Game_System
#------------------------------------------------------------------------------
# Adds Game Message
#==============================================================================
class Game_System
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_reader :message
#--------------------------------------------------------------------------
# * Alias Listing
#--------------------------------------------------------------------------
alias slipknot_lblms_initialize initialize
#--------------------------------------------------------------------------
# * Load Database
#--------------------------------------------------------------------------
def initialize
slipknot_lblms_initialize
@message = Game_Message.new
end
end
#==============================================================================
# ** Game_Event
#------------------------------------------------------------------------------
# Adds a reader to the Event Name
#==============================================================================
class Game_Event < Game_Character
#--------------------------------------------------------------------------
# * Name
#--------------------------------------------------------------------------
def name
@event.name
end
end
#==============================================================================
# ** Spriteset_Map
#------------------------------------------------------------------------------
# Adds a reader to the Character Sprites
#==============================================================================
class Spriteset_Map
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_reader :character_sprites
end
#==============================================================================
# ** Window_Message
#------------------------------------------------------------------------------
# Rewrites Window_Message
#==============================================================================
class Window_Message < Window_Selectable
#--------------------------------------------------------------------------
# * Alias Listing
#--------------------------------------------------------------------------
alias slipknot_lblms_initialize initialize
alias slipknot_lblms_terminatemessage terminate_message
#--------------------------------------------------------------------------
# * Initialize
#--------------------------------------------------------------------------
def initialize
slipknot_lblms_initialize
@autoclose = -1
end
#--------------------------------------------------------------------------
# * Terminate Message
#--------------------------------------------------------------------------
def terminate_message
slipknot_lblms_terminatemessage
[@name_box, @picture].each do |x|
x.dispose if x && ! x.disposed?
end
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.opacity = $game_system.message_frame == 0 ? 255 : 0
self.back_opacity = system.opacity
unless system.fit
self.width, self.height = 480, 160
skin = system.skin ? system.skin : $game_system.windowskin_name
self.windowskin = RPG::Cache.windowskin(skin)
self.contents = Bitmap.new(448, 128)
else
contents.clear
contents.font.color = normal_color
contents.font.size = Font.default_size
end
contents.font.name = system.font
@x = @y = @wait_count = indent = 0
@fit_size, @sound = false, system.sound_enable
@start_x = 4
@cursor_width = [0, 0, 0, 0]
@x = 8 if $game_temp.choice_start == 0
return if ! (@text = $game_temp.message_text)
@text.gsub!(/\\\\/) { "\000" }
@text.gsub!(/\\[Vv]\[([0-9]+)\]/) { $game_variables[$1.to_i] }
@text.gsub!('\$') { $game_party.gold.to_s }
@text.gsub!(/\\[Nn]\[([0-9]+)\]/) do
$game_actors[$1.to_i] ? $game_actors[$1.to_i].name : ''
end
@text.gsub!(/\\[Nn][Pp]\[([\d+])\]/) do
$game_party.actors[$1.to_i] ? $game_party.actors[$1.to_i].name : ''
end
@text.gsub!(/\\[Cc]lass\[(\d+)\]/) do
$game_actors[$1.to_i] ? $game_actors[$1.to_i].class_name : ''
end
@text.gsub!(/\\[Mm]ap/) { $data_map_infos[$game_map.map_id].name }
gold_set = @text.gsub!(/\\[Gg]/, '')
if @text[/\\[Nn]ame/]
if @text.sub!(/\\[Nn]ame\[(.*?)\]/, '')
name_text = $1
elsif @text.sub!(/\\[Nn]ame/, '')
name_text = $game_map.events[$game_system.map_interpreter.event_id].name
end
end
if @text[/\\[Ff]ace/]
# Left
if @text.sub!(/\\[Ff]ace{(.+?)}/, '')
face, face_name = 1, $1
# Right
elsif @text.sub!(/\\[Ff]ace\[(.+?)\]/, '')
face, face_name = 2, $1
end
end
picture = $1 if @text.sub!(/\\[Pp]ic\[(.+?)\]/, '')
if @text[/\\[Pp]/]
if @text.sub!(/\\[Pp]\[([-1,0-9]+)\]/, '')
event = $1.to_i
elsif @text.gsub!(/\\[Pp]/, '')
event = $game_system.map_interpreter.event_id
end
end
@text.gsub!('\$') { $game_party.gold.to_s }
@text.gsub!(/\\[Cc]\[([0-9A-Fa-f #]+?)\]/) { "\001[#$1]" }
@text.gsub!(/\\[Cc]/) { "\001[0]" }
@text.gsub!(/\\[Ii]con{([IiWwAaSs])}\[(\d+)\]/) { change_icon($1, $2.to_i) }
@text.gsub!(/\\[Ii]con\[(.*?)\]/) { "\002[#$1]" }
@text.gsub!('\!') { "\003" }
@text.gsub!('\.') { "\004" }
@text.gsub!(/\\[Ss]\[([Xx\d]+)\]/) { "\005[#$1]" }
@text.gsub!(/\\[Bb]/) { "\006" }
@text.gsub!(/\\[Ii]/) { "\007" }
@text.gsub!(/\\[Ff]\[(.*?)\]/) { "\010[#$1]" }
@text.gsub!(/\\\%\[(\d+)\]/) { "\011[#$1]" }
@text.gsub!('\%') { "\011" }
if @fit_size = (event || system.fit)
lines_size = [0, 0, 0, 0]
save, lines = @text.clone, 0
while (c = @text.slice!(/./m))
if c == "\n"
lines += 1
break if lines == 4
if lines >= $game_temp.choice_start
lines_size[lines] += 16
end
next
end
lines_size[lines] += eval_text(c, true)
end
end
if face
if @fit_size
mh = system.height
fh = system.face_rect.height
lines = (fh.to_f / mh.to_f).ceil if (lines * mh) < fh
f_x = face == 2 ? 0 : lines_size.max + 16
f_y = (lines * mh) <= fh ? 0 : (lines * mh - fh) / 2
@start_x += system.face_rect.width + 4 if face == 2
indent += system.face_rect.width + 8
else
f_x, f_y = face == 2 ? 16 : 336, 16
@start_x += system.face_rect.width + 36 if face == 2
end
f_bitmap = RPG::Cache.load_bitmap(system.path, face_name)
end
if @fit_size
@text = save
self.height = lines * system.height + 32
self.height += 32 if $game_temp.num_input_variable_id > 0
self.width = lines_size.max + indent + 40
windowskin = system.skin ? system.skin : $game_system.windowskin_name
self.windowskin = RPG::Cache.windowskin(windowskin)
self.contents = Bitmap.new(self.width - 32, self.height - 32)
contents.font.name = system.font
end
contents.blt(f_x, f_y, f_bitmap, system.face_rect) if face
if ! event
h2 = self.height / 2
self.y = $game_temp.in_battle ? 96 - h2 + system.nbyo :
case $game_system.message_position
when 0 then 96 - h2 + system.nbyo
when 1 then 240 - h2
when 2 then 384 - h2
end
self.x = 320 - self.width / 2
else
c = event > 0 ? $game_map.events[event] : $game_player
mx, my = 636 - self.width, 476 - self.height
fx = [[c.screen_x - self.width / 2, 4].max, mx].min
sy = name_text ? system.nbyo + 4 : 4
ch = [$scene.spriteset.character_sprites[event - 1].bitmap.height /
4 + 4, 48].max
fy = [[c.screen_y - (ch + self.height), sy].max, my].min
self.x, self.y = fx, fy
end
if name_text
@name_box = Window_NameBox.new(x, y - system.nbyo, name_text)
@name_box.back.opacity = 0 if $game_system.message_frame == 1
end
if picture
@picture = Sprite.new
@picture.bitmap = RPG::Cache.load_bitmap(system.path, picture)
@picture.x = self.x + self.width - @picture.bitmap.width
@picture.y = self.y - @picture.bitmap.height
end
if gold_set
@gold_window = Window_Gold.new
@gold_window.x = 560 - @gold_window.width
if $game_temp.in_battle
@gold_window.y = 192
else
@gold_window.y = self.y >= 128 ? 32 : 384
end
@gold_window.opacity = self.opacity
@gold_window.back_opacity = self.back_opacity
end
end
#--------------------------------------------------------------------------
# * Evaluate Text
#--------------------------------------------------------------------------
def eval_text(c, read = false)
case c
when "\000"
c = '\\'
when "\001"
@text.sub!(/\[(.*?)\]/, '')
return 0 if read
h, c = $1, $1.to_i
contents.font.color = h.slice!(/./) == '#' ? hex_color(h) : text_color(c)
return
when "\002"
@text.sub!(/\[(.*?)\]/, '')
return 24 if read
y = @fit_size ? system.height * @y + (system.height - 24) / 2 : 32 * @y + 4
contents.blt(@x + @start_x, y, RPG::Cache.icon($1.to_s), Rect.new(0, 0, 24, 24))
@x += 24
return unless @y >= $game_temp.choice_start
@cursor_width[@y] += 24
return
when "\003"
return 0 if read
@stop = true
return
when "\004"
return 0 if read
@wait_count += 10
return
when "\005"
@text.sub!(/\[([x\d]+)\]/, '')
if $1.downcase == 'x'
contents.font.size = Font.default_size
else
contents.font.size = [[$1.to_i, 6].max, system.height].min
end
return 0
when "\006"
contents.font.bold = (! contents.font.bold)
return 0
when "\007"
contents.font.italic = (! contents.font.italic)
return 0
when "\010"
@text.sub!(/\[(.*?)\]/, '')
if $1.downcase == 'x'
contents.font.name = system.font
else
contents.font.name = [$1.to_s, system.font]
end
return 0
when "\011"
@text.sub!(/\[(\d+)\]/, '')
return 0 if read
@autoclose = $1 ? $1.to_i : system.autoclose_frames
return
when "\n"
@y += 1
@x = 0
@x = 8 if @y >= $game_temp.choice_start
return
end
w = contents.text_size(c).width
return w if read
y = @fit_size ? system.height * @y : 32 * @y
if system.outline
color = contents.font.color.dup
contents.font.color.set(0, 0, 0, 255)
contents.draw_text(@x + @start_x + 1, y, w * 2, system.height, c)
contents.draw_text(@x + @start_x, y + 1, w * 2, system.height, c)
contents.draw_text(@x + @start_x - 1, y, w * 2, system.height, c)
contents.draw_text(@x + @start_x, y - 1, w * 2, system.height, c)
contents.font.color = color
contents.draw_text(@x + @start_x, y, w * 2, system.height, c)
elsif system.shadow
color = contents.font.color.dup
contents.font.color.set(0, 0, 0, 192)
contents.draw_text(@x + @start_x + 2, y + 2, w * 2, system.height, c)
contents.font.color = color
contents.draw_text(@x + @start_x, y, w * 2, system.height, c)
else
contents.draw_text(@x + @start_x, y, w * 2, system.height, c)
end
@sound = (system.sound_enable && c != ' ')
@x += w
return if @y < $game_temp.choice_start || @y > 3
@cursor_width[@y] += w
end
#--------------------------------------------------------------------------
# * Finish
#--------------------------------------------------------------------------
def finish
if temp.choice_max > 0
@item_max, self.active, self.index = temp.choice_max, true, 0
end
if temp.num_input_variable_id > 0
digits_max = temp.num_input_digits_max
number = $game_variables[temp.num_input_variable_id]
@input_number_window = Window_InputNumber.new(digits_max)
input_number.number = number
input_number.x = x + 8
input_number.y = y + temp.num_input_start * (@fit_size ? system.height : 32)
end
end
#--------------------------------------------------------------------------
# * Database Icon
#--------------------------------------------------------------------------
def change_icon(option, index)
s = case option.downcase
when 'i' then $data_items[index]
when 'w' then $data_weapons[index]
when 'a' then $data_armors[index]
when 's' then $data_skills[index]
end
return sprintf("\002[%s]%s", s.icon_name, s.name) if s.name
end
#--------------------------------------------------------------------------
# * Hexadecimal Color
#--------------------------------------------------------------------------
def hex_color(string)
return normal_color if string.size != 6
r = g = b = 0
5.times do |i|
s = string.slice!(/./m)
v = hex_convert(s.downcase)
case i
when 0 then r += v * 16
when 1 then r += v
when 2 then g += v * 16
when 3 then g += v
when 4 then b += v * 16
when 5 then b += v
end
end
return Color.new(r, g, b)
end
#--------------------------------------------------------------------------
def hex_convert(c)
return c.to_i if c[/[0-9]/]
case c
when 'a' then 10
when 'b' then 11
when 'c' then 12
when 'd' then 13
when 'e' then 14
when 'f' then 15
end
end
#--------------------------------------------------------------------------
# * Game Message
#--------------------------------------------------------------------------
def system() $game_system.message end
#--------------------------------------------------------------------------
# * Game Temp
#--------------------------------------------------------------------------
def temp() $game_temp end
#--------------------------------------------------------------------------
# * Input Number Window
#--------------------------------------------------------------------------
def input_number() @input_number_window end
#--------------------------------------------------------------------------
# * Frame Update
#--------------------------------------------------------------------------
def update
if @contents_showing
super
if @fade_in
self.contents_opacity += 24
if input_number
input_number.contents_opacity += 24
end
@fade_in = contents_opacity != 255
return
end
if @text
if Input.trigger?(13)
if @stop
self.pause = @stop = false
return
end
@skip = system.can_skip
end
return if @stop
if @wait_count > 0 && ! @skip
@wait_count -= 1
return
end
loop do
if (c = @text.slice!(/./m))
eval_text(c)
if @stop
self.pause = system.pause
return
end
if ! @skip && @sound
Audio.se_play('Audio/SE/' + system.sound[0], system.sound[1])
@sound = false
end
@wait_count += system.speed
else
@text = nil
break
end
break if ! @skip
end
return if @text || @autoclose != -1
finish
return
else
if @autoclose > 0
@autoclose -= 1
return
elsif @autoclose == 0
terminate_message
@autoclose = -1
return
end
end
end
if input_number
input_number.update
if Input.trigger?(13)
$game_system.se_play($data_system.decision_se)
$game_variables[$game_temp.num_input_variable_id] = input_number.number
$game_map.need_refresh = true
input_number.dispose
@input_number_window = nil
terminate_message
end
return
end
if @contents_showing
self.pause = ($game_temp.choice_max == 0) & system.pause
if Input.trigger?(12)
if $game_temp.choice_max > 0 && $game_temp.choice_cancel_type > 0
$game_system.se_play($data_system.cancel_se)
$game_temp.choice_proc.call($game_temp.choice_cancel_type - 1)
terminate_message
end
end
if Input.trigger?(13)
if $game_temp.choice_max > 0
$game_system.se_play($data_system.decision_se)
$game_temp.choice_proc.call(self.index)
end
terminate_message
end
return
end
if ! @fade_out && $game_temp.message_text
@contents_showing = temp.message_window_showing = true
@stop = false
@autoclose = -1
@skip = (! system.letter_by_letter)
reset_window
refresh
@wait_count, self.visible = 0, true
return
end
return if ! visible
@fade_out = true
self.opacity -= 48
if self.opacity == 0
self.visible = @fade_out = false
$game_temp.message_window_showing = false
end
end
#--------------------------------------------------------------------------
# * Updates Cursor Rectangle
#--------------------------------------------------------------------------
def update_cursor_rect
if index >= 0
n = $game_temp.choice_start + @index
y = (@fit_size ? system.height : 32) * n
cursor_rect.set(4 + @start_x, y, @cursor_width.max + 8,
@fit_size ? system.height : 32)
else
cursor_rect.empty
end
end
end
#==============================================================================
# ** Window_NameBox
#------------------------------------------------------------------------------
# This window is used to display the box above the message.
#==============================================================================
class Window_NameBox < Sprite
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_reader :back
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize(x, y, text)
dumb = Bitmap.new(160, 42)
dumb.font.name = system.font
size = dumb.text_size(text).width
dumb.dispose
@back = Window_Base.new(x, y, size + 12, 32)
skin = system.nb_skin ? system.nb_skin : $game_system.windowskin_name
@back.windowskin = RPG::Cache.windowskin(skin)
viewport = Viewport.new(x + 6, y + 5, size, 22)
@back.z = viewport.z = 9999
super(viewport)
self.bitmap = Bitmap.new(size, 22)
bitmap.font.name = system.font
bitmap.draw_text(0, 0, size, 22, text)
end
#--------------------------------------------------------------------------
# * Game Message
#--------------------------------------------------------------------------
def system() $game_system.message end
#--------------------------------------------------------------------------
# * Dispose
#--------------------------------------------------------------------------
def dispose
@back.dispose
@back = nil
super
end
end
#==============================================================================
# ** Interpreter
#------------------------------------------------------------------------------
# Adds a reader to the Event ID and Game Message
#==============================================================================
class Interpreter
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_reader :event_id
#--------------------------------------------------------------------------
# * Game Message
#--------------------------------------------------------------------------
def message
$game_system.message
end
end
#==============================================================================
# ** Scene_Map
#------------------------------------------------------------------------------
# Adds a reader to the Spriteset
#==============================================================================
class Scene_Map
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_reader :spriteset
end
#------------------------------------------------------------------------------
# End SDK Enabled Test
#------------------------------------------------------------------------------
end
#==============================================================================
# ** Multiple Poisons
#------------------------------------------------------------------------------
# Scripted by: Yeyinde
# Version 1.1.0
# February 1 & 2, 2007
#==============================================================================
#--------------------------------------------------------------------------
# * Initialize Constants
#--------------------------------------------------------------------------
SP_POISONS = [] # Add the state ids of SP draining poisons here
BATTLE_DAMAGE = {18 => 11} # Format: state_id => damage_division
BATTLE_DAMAGE.default = 10 # Do not change
MAP_DAMAGE = {18 => 101} # Format: state_id => damage_division
MAP_DAMAGE.default = 100 # Do not change
# Damage correction (To prevent /0 errors)
# DO NOT EDIT!
BATTLE_DAMAGE.each do |state_id, damage|
BATTLE_DAMAGE[state_id] = BATTLE_DAMAGE.default if damage == 0
end
MAP_DAMAGE.each do |state_id, damage|
MAP_DAMAGE[state_id] = MAP_DAMAGE.default if damage == 0
end
#==============================================================================
# ** Game_Battler
#------------------------------------------------------------------------------
# This class deals with battlers. It's used as a superclass for the Game_Actor
# and Game_Enemy classes.
#==============================================================================
class Game_Battler
#--------------------------------------------------------------------------
# * Application of Slip Damage Effects - Overwrite
#--------------------------------------------------------------------------
def slip_damage_effect
# Set damage
self.damage = 0
sp_damage = 0
# Branch per state held
@states.each do |i|
# If the state has slip damage
if $data_states[i].slip_damage
# Add to damage
if SP_POISONS.include?(i)
sp_damage += self.maxsp / BATTLE_DAMAGE[i]
else
self.damage += self.maxhp / BATTLE_DAMAGE[i]
end
end
end
# Dispersion
if self.damage.abs > 0
amp = [self.damage * 15 / 100, 1].max
self.damage += rand(amp+1) + rand(amp+1) - amp
end
if sp_damage.abs > 0
amp = [sp_damage * 15 / 100, 1].max
sp_damage += rand(amp+1) + rand(amp+1) - amp
end
# Subtract damage from HP and SP
self.hp -= self.damage
self.sp -= sp_damage
# End Method
return true
end
end
#==============================================================================
# ** Game_Party
#------------------------------------------------------------------------------
# This class handles the party. It includes information on amount of gold
# and items. Refer to "$game_party" for the instance of this class.
#==============================================================================
class Game_Party
#--------------------------------------------------------------------------
# * Slip Damage Check (for map) - Overwrite
#--------------------------------------------------------------------------
def check_map_slip_damage
# Branch per actor
@actors.each do |actor|
# If actor has more than 0 HP and has a slip damage state
if actor.hp > 0 and actor.slip_damage?
# Set damage
damage = 0
sp_damage = 0
# Branch per state held
actor.states.each do |i|
# If the state has slip damage
if $data_states[i].slip_damage
# Add to damage
if SP_POISONS.include?(i)
sp_damage += actor.maxsp / MAP_DAMAGE[i]
else
damage += actor.maxhp / MAP_DAMAGE[i]
end
end
end
# Take damage
if damage > 0
actor.hp -= [damage, 1].max
elsif damage < 0
actor.hp -= [damage, -1].min
end
# Take SP damage
if sp_damage > 0
actor.sp -= [sp_damage, 1].max
elsif sp_damage < 0
actor.sp -= [sp_damage, -1].min
end
# If the actor has no more HP
if actor.hp == 0
# Play the actor collapse SE
$game_system.se_play($data_system.actor_collapse_se)
end
# Flash the screen to indicate damage
if damage > 0 or sp_damage > 0
$game_screen.start_flash(Color.new(255,0,0,128), 4)
elsif damage < 0 or sp_damage < 0
$game_screen.start_flash(Color.new(0,255,0,128), 4)
end
# Go to game over if the party is all dead
$game_temp.gameover = $game_party.all_dead?
end
end
end
end
#==============================================================================
# 本脚本æ
#==============================================================================
# ** COGWHEEL Plug 'n' Play Menu Bars (based on Syvkal's revisions)
#-------------------------------------------------------------------------------
# by DerVVulfman
# Version 1.1
# 06-28-06
#------------------------------------------------------------------------------
# This is a revision of Cogwheel's famous bargraph system, now set as an inser-
# table script to display bargraphs behind values in the menus.
#
# To prevent conflict with Cogwheel's RTAB system, two key definitions have been
# renamed: "gauge_rect" to "cw_gauge" & "gradation_rect" to "cw_grad_rect."
#
#
# Affected Systems: Main Menu
# Skill Menu
# Status Menu
# Hero Select Menu (for Items & Skills)
# Equipment Menu
# BattleStatus Menu
#
# The system uses a series of CONSTANTS that can be edited here. They control
# the basic gauge colors and the manner the gauge is filled:
# Gauge Border Colors
COG_COLOR1 = Color.new(0, 0, 0, 192) # Outer Border
COG_COLOR2 = Color.new(255, 255, 192, 192) # Inner Border
# Gauge Empty filler
COG_COLOR3 = Color.new(0, 0, 0, 192) # Half of Inner Shading
COG_COLOR4 = Color.new(64, 0, 0, 192) # Half of Inner Shading
# Alignment
COG_ALIGN1 = 1 # Type 1: (0: Left / 1: Center / 2: Right Justify)
COG_ALIGN2 = 2 # Type 2: (0: Upper / 1: Central / 2: Lower)
COG_ALIGN3 = 0 # FILL ALIGNMENT (0: Left Justify / 1: Right Justify)
# Gauge Settings
COG_GRADE1 = 1 # EMPTY gauge (0: Side / 1: Vertical / 2: Slanted)
COG_GRADE2 = 0 # FILLER gauge (0: Side / 1: Vertical / 2: Slanted)
#==============================================================================
# ** Game_Actor
#------------------------------------------------------------------------------
# This class handles the actor. It's used within the Game_Actors class
# ($game_actors) and refers to the Game_Party class ($game_party).
#==============================================================================
class Game_Actor < Game_Battler
#--------------------------------------------------------------------------
# * Get EXP - numeric for calculations
#--------------------------------------------------------------------------
def now_exp
return @exp - @exp_list[@level]
end
#--------------------------------------------------------------------------
# * Get Next Level EXP - numeric for calculations
#--------------------------------------------------------------------------
def next_exp
return @exp_list[@level+1] > 0 ? @exp_list[@level+1] - @exp_list[@level] : 0
end
#--------------------------------------------------------------------------
# * End of Class
#--------------------------------------------------------------------------
end
#==============================================================================
# ** Window_Base
#------------------------------------------------------------------------------
# This class is for all in-game windows.
#==============================================================================
class Window_Base < Window
#--------------------------------------------------------------------------
# * Draw EXP w/ Bars
# actor : actor
# x : draw spot x-coordinate
# y : draw spot y-coordinate
#--------------------------------------------------------------------------
alias draw_actor_exp_original draw_actor_exp
def draw_actor_exp(actor, x, y, width = 204)
if actor.next_exp != 0
rate = actor.now_exp.to_f / actor.next_exp
else
rate = 1
end
# Calculate Bar Gradiation
if actor.next_exp != 0
rate = actor.now_exp.to_f / actor.next_exp
else
rate = 1
end
# Adjust Bar Color based on Gradiation
color1 = Color.new(80 - 24 * rate, 80 * rate, 14 * rate, 192)
color2 = Color.new(100 - 72 * rate, 240 * rate, 62 * rate, 192)
# Calculate Bar Width
if actor.next_exp != 0
exp = width * actor.now_exp / actor.next_exp
else
exp = width
end
# Draw Bar Graph
cw_gauge(x, y + 25, width, 10, exp, color1, color2)
# Call original EXP
draw_actor_exp_original(actor, x, y)
end
#--------------------------------------------------------------------------
# * Draw HP w/ Bars
# actor : actor
# x : draw spot x-coordinate
# y : draw spot y-coordinate
# width : draw spot width
#--------------------------------------------------------------------------
alias draw_actor_hp_original draw_actor_hp
def draw_actor_hp(actor, x, y, width = 144)
# Calculate Bar Gradiation
if actor.maxhp != 0
rate = actor.hp.to_f / actor.maxhp
else
rate = 0
end
# Adjust Bar Color based on Gradiation
color1 = Color.new(216 - 24 * rate, 0 * rate, 0 * rate, 192)
color2 = Color.new(255 * rate, 165 * rate, 0 * rate, 192)
# Calculate Bar Width
if actor.maxhp != 0
hp = width * actor.hp / actor.maxhp
else
hp = 0
end
# Draw Bar Graph
cw_gauge(x, y + 25, width, 10, hp, color1, color2)
# Call original HP
draw_actor_hp_original(actor, x, y, width)
end
#--------------------------------------------------------------------------
# * Draw SP w/ Bars
# actor : actor
# x : draw spot x-coordinate
# y : draw spot y-coordinate
# width : draw spot width
#--------------------------------------------------------------------------
alias draw_actor_sp_original draw_actor_sp
def draw_actor_sp(actor, x, y, width = 144)
# Calculate Bar Gradiation
if actor.maxsp != 0
rate = actor.sp.to_f / actor.maxsp
else
rate = 1
end
# Adjust Bar Color based on Gradiation
color1 = Color.new(14 * rate, 80 - 24 * rate, 80 * rate, 192)
color2 = Color.new(62 * rate, 240 - 72 * rate, 240 * rate, 192)
# Calculate Bar Width
if actor.maxsp != 0
sp = width * actor.sp / actor.maxsp
else
sp = width
end
# Draw Bar Graph
cw_gauge(x + width * 0 / 100, y + 25, width, 10, sp, color1, color2)
# Call original SP
draw_actor_sp_original(actor, x, y, width)
end
#--------------------------------------------------------------------------
# * Draw Parameter w/ Bars
# actor : actor
# x : draw spot x-coordinate
# y : draw spot y-coordinate
# type : parameter type (0-6)
#--------------------------------------------------------------------------
alias draw_actor_parameter_original draw_actor_parameter
def draw_actor_parameter(actor, x, y, type)
# Choose Color & Parameter Type
case type
when 0
e1 = actor.atk
c6 = Color.new(253, 53, 56, 192)
c5 = Color.new(242, 2, 6, 192)
when 1
e1 = actor.pdef
c6 = Color.new(238, 254, 124, 192)
c5 = Color.new(228, 253, 48, 192)
when 2
e1 = actor.mdef
c6 = Color.new(150, 37, 184, 192)
c5 = Color.new(170, 57, 204, 192)
when 3
e1 = actor.str
c6 = Color.new(253, 163, 33, 192)
c5 = Color.new(254, 209, 154, 192)
when 4
e1 = actor.dex
c6 = Color.new(255, 255, 255, 192)
c5 = Color.new(222, 222, 222, 192)
when 5
e1 = actor.agi
c6 = Color.new(124, 254, 155, 192)
c5 = Color.new(33, 253, 86, 192)
when 6
e1 = actor.int
c6 = Color.new(119, 203, 254, 192)
c5 = Color.new(8, 160, 253, 192)
end
# Calculate Bar Gradiation
e2 = 999
if e1.to_f != 0
rate = e1.to_f / e2.to_f
else
rate = 1
end
# Adjust Bar Color based on Gradiation & Parameter Type
for i in 0..7
r = c6.red * rate
g = (c6.green - 10) * rate
b = c6.blue * rate
a = c6.alpha
end
# Calculate Bar Width
width = 168
if e1.to_f != 0
par = width * e1.to_f / e2.to_f
else
par = width
end
# Equipment Calc Fix
case type
when 0
if e1 == 0
par = 0
end
when 1
if e1 == 0
par = 0
end
when 2
if e1 == 0
par = 0
end
end
# Draw Bar Graph
cw_gauge(x , y + 25, width, 7, par, c5, Color.new(r, g, b, a))
# Call Original Parameter
draw_actor_parameter_original(actor, x, y, type)
end
#--------------------------------------------------------------------------
# * Gauge Rectangle (New to Class)
#--------------------------------------------------------------------------
def cw_gauge(x, y, width, height, gauge, color1, color2)
# Use Cogwheel PRESETS
color3 = COG_COLOR1
color4 = COG_COLOR2
color5 = COG_COLOR3
color6 = COG_COLOR4
align1 = COG_ALIGN1
align2 = COG_ALIGN2
align3 = COG_ALIGN3
grade1 = COG_GRADE1
grade2 = COG_GRADE2
# Create Rectangle Width based on gauge max.
rect_width = width
case align1
when 1
x += (rect_width - width) / 2
when 2
x += rect_width - width
end
case align2
when 1
y -= height / 2
when 2
y -= height
end
self.contents.fill_rect(x, y, width, height, color3)
self.contents.fill_rect(x + 1, y + 1, width - 2, height - 2, color4)
if align3 == 0
if grade1 == 2
grade1 = 3
end
if grade2 == 2
grade2 = 3
end
end
if (align3 == 1 and grade1 == 0) or grade1 > 0
color = color5
color5 = color6
color6 = color
end
if (align3 == 1 and grade2 == 0) or grade2 > 0
color = color1
color1 = color2
color2 = color
end
self.contents.cw_grad_rect(x + 2, y + 2, width - 4, height - 4, color5, color6, grade1)
if align3 == 1
x += width - gauge
end
self.contents.cw_grad_rect(x + 2, y + 2, gauge - 4, height - 4, color1, color2, grade2)
end
#--------------------------------------------------------------------------
# * End of Class
#--------------------------------------------------------------------------
end
#==============================================================================
# ** Bitmap
#==============================================================================
class Bitmap
#--------------------------------------------------------------------------
# * Gradation Rectangle
#--------------------------------------------------------------------------
def cw_grad_rect(x, y, width, height, color3, color4, align = 0)
if align == 0
for i in x...x + width
red = color3.red + (color4.red - color3.red) * (i - x) / (width - 1)
green = color3.green +
(color4.green - color3.green) * (i - x) / (width - 1)
blue = color3.blue +
(color4.blue - color3.blue) * (i - x) / (width - 1)
alpha = color3.alpha +
(color4.alpha - color3.alpha) * (i - x) / (width - 1)
color = Color.new(red, green, blue, alpha)
fill_rect(i, y, 1, height, color)
end
elsif align == 1
for i in y...y + height
red = color3.red +
(color4.red - color3.red) * (i - y) / (height - 1)
green = color3.green +
(color4.green - color3.green) * (i - y) / (height - 1)
blue = color3.blue +
(color4.blue - color3.blue) * (i - y) / (height - 1)
alpha = color3.alpha +
(color4.alpha - color3.alpha) * (i - y) / (height - 1)
color = Color.new(red, green, blue, alpha)
fill_rect(x, i, width, 1, color)
end
elsif align == 2
for i in x...x + width
for j in y...y + height
red = color3.red + (color4.red - color3.red) *
((i - x) / (width - 1.0) + (j - y) / (height - 1.0)) / 2
green = color3.green + (color4.green - color3.green) *
((i - x) / (width - 1.0) + (j - y) / (height - 1.0)) / 2
blue = color3.blue + (color4.blue - color3.blue) *
((i - x) / (width - 1.0) + (j - y) / (height - 1.0)) / 2
alpha = color3.alpha + (color4.alpha - color3.alpha) *
((i - x) / (width - 1.0) + (j - y) / (height - 1.0)) / 2
color = Color.new(red, green, blue, alpha)
set_pixel(i, j, color)
end
end
elsif align == 3
for i in x...x + width
for j in y...y + height
red = color3.red + (color4.red - color3.red) *
((x + width - i) / (width - 1.0) + (j - y) / (height - 1.0)) / 2
green = color3.green + (color4.green - color3.green) *
((x + width - i) / (width - 1.0) + (j - y) / (height - 1.0)) / 2
blue = color3.blue + (color4.blue - color3.blue) *
((x + width - i) / (width - 1.0) + (j - y) / (height - 1.0)) / 2
alpha = color3.alpha + (color4.alpha - color3.alpha) *
((x + width - i) / (width - 1.0) + (j - y) / (height - 1.0)) / 2
color = Color.new(red, green, blue, alpha)
set_pixel(i, j, color)
end
end
end
end
#--------------------------------------------------------------------------
# * End of Class
#--------------------------------------------------------------------------
end
#==============================================================================
# ** Dynamic Enemies
#------------------------------------------------------------------------------
# SephirothSpawn
# Version 3.01
# 2007-05-11
# SDK : Version 2.0+, Part 1
#------------------------------------------------------------------------------
# * Version History :
#
# Version 1 ---------------------------------------------------- (2006-08-06)
# Version 2 ---------------------------------------------------- (2006-10-07)
# - Update : Updated to work with Trickster's Curve Generator Module
# Version 3 ---------------------------------------------------- (2007-02-28)
# - Update : Rescripted entire system
# Version 3.01 ------------------------------------------------ (2007-05-11)
# - Bug Fix : Fixed Extremely Minor Base Stat Level Error
#------------------------------------------------------------------------------
# * Requirements :
#
# Method & Class Library 2.1+
#------------------------------------------------------------------------------
# * Description
#
# This script was designed to allow your game to have enemies that develope
# as your actors do. No longer will you encounter the same old boring enemy
# each time. As the developer, you can control the development of each stat
# for each enemy. The script assigns the enemy a semi-random and controled
# level depending on the party's average level. The stats are then pulled
# from a generated curve based of your definitions.
#------------------------------------------------------------------------------
# * Instructions
#
# Place The Script Below the SDK and Above Main.
# To Customize your enemy stats, refer to the customization instructions.
#------------------------------------------------------------------------------
# * Customization
#
# Setting unaffected enemies
# - Unaffected_Enemies = [enemy_id, enemy_id, ...]
#
# Setting enemy stats
# - Stats = {
# enemy_id => { <stat_name> => [ generator_type, *args ], ... }
# , ...
# }
#
# Stat Names : 'maxhp', 'maxsp', 'str', 'dex', 'agi', 'int', 'atk',
# 'pdef', 'mdef', 'eva', 'hit', 'exp', 'gold'
#
# ** Refer to Curve Generator System for type and args specification.
#
# Enemy Min & Max Level
# - Min_Levels = { enemy_id => min_level, ... }
# - Max_Levels = { enemy_id => max_level, ... }
#
# Default Min & Max Level (For all non-defined enemies)
# - Min_Levels.default = min_level
# - Max_Levels.default = max_level
#
# Range For Enemy Levels Off Party Level
# - Level_Ranges = { enemy_id => (min_v..max_v), ... }
#
# Range For Enemy Levels Off Party Level (For all non-defined enemies)
# - Level_Ranges.default = (min_v..max_v)
#--------------------------------------------------------------------------
# * Syntax :
#
# Enemy Level
# - <game_enemy>.level
#
# Dynamic Enemies
# - <game_enemy>.is_dynamic?
#==============================================================================
#------------------------------------------------------------------------------
# * SDK Log Script
#------------------------------------------------------------------------------
SDK.log('Dynamic Enemies', 'SephirothSpawn', 3.01, '2007-05-11')
SDK.check_requirements(2.0, [], {'Method & Class Library' => 2.1})
#------------------------------------------------------------------------------
# * Begin SDK Enable Test
#------------------------------------------------------------------------------
if SDK.enabled?('Dynamic Enemies')
#==============================================================================
# ** Dynamic_Enemies
#==============================================================================
module Dynamic_Enemies
#--------------------------------------------------------------------------
# * Saves Curves
#
# ~ @cache = { <generator_setup> => <curve>, ... }
#--------------------------------------------------------------------------
@cache = {}
#--------------------------------------------------------------------------
# * Unaffected Enemies
#
# ~ Unaffected_Enemies = [enemy_id, enemy_id, ...]
#--------------------------------------------------------------------------
Unaffected_Enemies = []
#--------------------------------------------------------------------------
# * Stats
#
# ~ enemy_id => { <stat_name> => [ generator_type, *args ], ... }
#
# Stat Names : 'maxhp', 'maxsp', 'str', 'dex', 'agi', 'int', 'atk',
# 'pdef', 'mdef', 'eva', 'hit', 'exp', 'gold'
#
# ** Refer to Curve Generator System for type and args specification.
#--------------------------------------------------------------------------
Stats = {
2 => {
'maxhp' => [0, 1, 5, 1, 100],
'maxsp' => [0, 1, 99, 150, 50],
'str' => [0, 1, 99, 50, 5],
'dex' => [0, 1, 99, 50, 10],
'agi' => [0, 1, 99, 50, 10],
'int' => [0, 1, 99, 10, 5]
},
4 => {
'maxhp' => [0, 1, 99, 500, 150],
'maxsp' => [0, 1, 99, 200, 75],
'str' => [0, 1, 99, 50, 10],
'dex' => [0, 1, 99, 50, 10],
'agi' => [0, 1, 99, 50, 10],
'int' => [0, 1, 99, 10, 10]
},
35 => {
'maxhp' => [0, 1, 99, 500, 150],
'maxsp' => [0, 1, 99, 200, 75],
'str' => [0, 1, 99, 50, 10],
'dex' => [0, 1, 99, 50, 10],
'agi' => [0, 1, 99, 50, 10],
'int' => [0, 1, 99, 10, 10]
},
34 => {
'maxhp' => [0, 1, 99, 500, 150],
'maxsp' => [0, 1, 99, 200, 75],
'str' => [0, 1, 99, 50, 10],
'dex' => [0, 1, 99, 50, 10],
'agi' => [0, 1, 99, 50, 10],
'int' => [0, 1, 99, 10, 10]
},
33 => {
'maxhp' => [0, 1, 99, 500, 150],
'maxsp' => [0, 1, 99, 200, 75],
'str' => [0, 1, 99, 50, 10],
'dex' => [0, 1, 99, 50, 10],
'agi' => [0, 1, 99, 50, 10],
'int' => [0, 1, 99, 10, 10]
},
13 => {
'maxhp' => [0, 1, 99, 500, 150],
'maxsp' => [0, 1, 99, 200, 75],
'str' => [0, 1, 99, 50, 10],
'dex' => [0, 1, 99, 50, 10],
'agi' => [0, 1, 99, 50, 10],
'int' => [0, 1, 99, 10, 10]
},
36 => {
'maxhp' => [0, 1, 99, 500, 150],
'maxsp' => [0, 1, 99, 200, 75],
'str' => [0, 1, 99, 50, 10],
'dex' => [0, 1, 99, 50, 10],
'agi' => [0, 1, 99, 50, 10],
'int' => [0, 1, 99, 50, 10]
},
3 => {
'maxhp' => [0, 1, 99, 500, 150],
'maxsp' => [0, 1, 99, 200, 75],
'str' => [0, 1, 99, 50, 10],
'dex' => [0, 1, 99, 50, 10],
'agi' => [0, 1, 99, 50, 10],
'int' => [0, 1, 99, 50, 10]
},
7 => {
'maxhp' => [0, 1, 99, 500, 150],
'maxsp' => [0, 1, 99, 200, 75],
'str' => [0, 1, 99, 50, 10],
'dex' => [0, 1, 99, 50, 10],
'agi' => [0, 1, 99, 50, 10],
'int' => [0, 1, 99, 50, 10]
},
11 => {
'maxhp' => [0, 1, 99, 500, 150],
'maxsp' => [0, 1, 99, 200, 75],
'str' => [0, 1, 99, 50, 10],
'dex' => [0, 1, 99, 50, 10],
'agi' => [0, 1, 99, 50, 10],
'int' => [0, 1, 99, 50, 10]
},
41 => {
'maxhp' => [0, 1, 99, 500, 150],
'maxsp' => [0, 1, 99, 200, 75],
'str' => [0, 1, 99, 50, 10],
'dex' => [0, 1, 99, 50, 10],
'agi' => [0, 1, 99, 50, 10],
'int' => [0, 1, 99, 50, 10]
},
42 => {
'maxhp' => [0, 1, 99, 500, 150],
'maxsp' => [0, 1, 99, 200, 75],
'str' => [0, 1, 99, 50, 10],
'dex' => [0, 1, 99, 50, 10],
'agi' => [0, 1, 99, 50, 10],
'int' => [0, 1, 99, 50, 10]
},
5 => {
'maxhp' => [0, 1, 99, 500, 150],
'maxsp' => [0, 1, 99, 200, 75],
'str' => [0, 1, 99, 50, 10],
'dex' => [0, 1, 99, 50, 10],
'agi' => [0, 1, 99, 50, 10],
'int' => [0, 1, 99, 50, 10]
}
}
#--------------------------------------------------------------------------
# * Defaults : BE CAREFUL WITH ALTERATION
#--------------------------------------------------------------------------
Stats.default = {}
# Default For All Non-Defined Stats
Stats.default.default = [0, 1, 99, 500, 220]
Stats.values.each do |stat_settings|
# Default For All Non-Defined Stats For Defined Enemies
stat_settings.default = [0, 1, 99, 500, 220]
end
#--------------------------------------------------------------------------
# * Level Specifications
#
# Enemy Min & Max Level
# - Min_Levels = { enemy_id => min_level, ... }
# - Max_Levels = { enemy_id => max_level, ... }
#
# Default Min & Max Level (For all non-defined enemies)
# - Min_Levels.default = min_level
# - Max_Levels.default = max_level
#
# Range For Enemy Levels Off Party Level
# - Level_Ranges = { enemy_id => (min_v..max_v), ... }
#
# Range For Enemy Levels Off Party Level (For all non-defined enemies)
# - Level_Ranges.default = (min_v..max_v)
#--------------------------------------------------------------------------
Min_Levels = {}
Max_Levels = {}
Level_Ranges = {}
Min_Levels.default = 1
Max_Levels.default = 99
Level_Ranges.default = (0..0)
#--------------------------------------------------------------------------
# * Get Base Stat
#--------------------------------------------------------------------------
def self.get_base_stat(enemy_id, stat_name, level)
# Gets Curve Data
curve_data = Stats[enemy_id][stat_name]
# Checks For Pre-defined Curve
if @cache.has_key?(curve_data)
return @cache[curve_data][level]
end
# Creates Curve Data
curve = Curve_Generator.generate_curve(*curve_data)
# Save Curve
@cache[curve_data] = curve
# Return Stat
return curve[level]
end
#--------------------------------------------------------------------------
# * Generate Level
#--------------------------------------------------------------------------
def self.generate_level(enemy_id)
# Collect Average Party Level
level = 0
$game_party.actors.each {|a| level += a.level}
level /= $game_party.actors.size
# Cap Level
level = [[level, Min_Levels[enemy_id]].max, Max_Levels[enemy_id]].min
# Modifies Level
level += Level_Ranges[enemy_id].random
# Return Level Data
return level
end
end
#==============================================================================
# ** Game_Enemy
#==============================================================================
class Game_Enemy < Game_Battler
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_reader :level
#--------------------------------------------------------------------------
# * Alias Listings
#--------------------------------------------------------------------------
alias_method :seph_dynenemies_gmenmy_init, :initialize
alias_method :seph_dynenemies_gmenmy_maxhp, :base_maxhp
alias_method :seph_dynenemies_gmenmy_maxsp, :base_maxhp
alias_method :seph_dynenemies_gmenmy_str, :base_str
alias_method :seph_dynenemies_gmenmy_dex, :base_dex
alias_method :seph_dynenemies_gmenmy_agi, :base_agi
alias_method :seph_dynenemies_gmenmy_int, :base_int
alias_method :seph_dynenemies_gmenmy_atk, :base_atk
alias_method :seph_dynenemies_gmenmy_eva, :base_eva
alias_method :seph_dynenemies_gmenmy_pdef, :base_pdef
alias_method :seph_dynenemies_gmenmy_mdef, :base_mdef
alias_method :seph_dynenemies_gmenmy_exp, :exp
alias_method :seph_dynenemies_gmenmy_gold, :gold
#--------------------------------------------------------------------------
# * Is Dynamic
#--------------------------------------------------------------------------
def is_dynamic?(enemy_id = @enemy_id)
return Dynamic_Enemies::Stats.has_key?(enemy_id)
end
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize(troop_id, member_index)
# Gets Enemy ID
enemy_id = $data_troops[troop_id].members[member_index].enemy_id
# Set Level
@level = Dynamic_Enemies.generate_level(enemy_id)
# Setup Dynamic Enemy
setup_dynamic_enemy(enemy_id) if is_dynamic?(enemy_id)
# Original Initialization
seph_dynenemies_gmenmy_init(troop_id, member_index)
end
#--------------------------------------------------------------------------
# * Setup Dynamic Enemy
#--------------------------------------------------------------------------
def setup_dynamic_enemy(enemy_id)
# Set Dynamic Stats
@dyn_base_maxhp = Dynamic_Enemies.get_base_stat(enemy_id, 'hp', @level)
@dyn_base_maxsp = Dynamic_Enemies.get_base_stat(enemy_id, 'sp', @level)
@dyn_base_str = Dynamic_Enemies.get_base_stat(enemy_id, 'str', @level)
@dyn_base_dex = Dynamic_Enemies.get_base_stat(enemy_id, 'dex', @level)
@dyn_base_agi = Dynamic_Enemies.get_base_stat(enemy_id, 'agi', @level)
@dyn_base_int = Dynamic_Enemies.get_base_stat(enemy_id, 'int', @level)
@dyn_base_atk = Dynamic_Enemies.get_base_stat(enemy_id, 'atk', @level)
@dyn_base_eva = Dynamic_Enemies.get_base_stat(enemy_id, 'eva', @level)
@dyn_base_pdef = Dynamic_Enemies.get_base_stat(enemy_id, 'pdef', @level)
@dyn_base_mdef = Dynamic_Enemies.get_base_stat(enemy_id, 'mdef', @level)
@dyn_gold = Dynamic_Enemies.get_base_stat(enemy_id, 'gold', @level)
@dyn_exp = Dynamic_Enemies.get_base_stat(enemy_id, 'exp', @level)
end
#--------------------------------------------------------------------------
# * Get Basic Maximum HP
#--------------------------------------------------------------------------
def base_maxhp
return is_dynamic? ? @dyn_base_maxhp : seph_dynenemies_gmenmy_maxhp
end
#--------------------------------------------------------------------------
# * Get Basic Maximum SP
#--------------------------------------------------------------------------
def base_maxsp
return is_dynamic? ? @dyn_base_maxsp : seph_dynenemies_gmenmy_maxsp
end
#--------------------------------------------------------------------------
# * Get Basic Strength
#--------------------------------------------------------------------------
def base_str
return is_dynamic? ? @dyn_base_str : seph_dynenemies_gmenmy_str
end
#--------------------------------------------------------------------------
# * Get Basic Dexterity
#--------------------------------------------------------------------------
def base_dex
return is_dynamic? ? @dyn_base_dex : seph_dynenemies_gmenmy_dex
end
#--------------------------------------------------------------------------
# * Get Basic Agility
#--------------------------------------------------------------------------
def base_agi
return is_dynamic? ? @dyn_base_agi : seph_dynenemies_gmenmy_agi
end
#--------------------------------------------------------------------------
# * Get Basic Intelligence
#--------------------------------------------------------------------------
def base_int
return is_dynamic? ? @dyn_base_int : seph_dynenemies_gmenmy_int
end
#--------------------------------------------------------------------------
# * Get Basic Attack Power
#--------------------------------------------------------------------------
def base_atk
return is_dynamic? ? @dyn_base_atk : seph_dynenemies_gmenmy_atk
end
#--------------------------------------------------------------------------
# * Get Basic Physical Defense
#--------------------------------------------------------------------------
def base_pdef
return is_dynamic? ? @dyn_base_pdef : seph_dynenemies_gmenmy_pdef
end
#--------------------------------------------------------------------------
# * Get Basic Magic Defense
#--------------------------------------------------------------------------
def base_mdef
return is_dynamic? ? @dyn_base_mdef : seph_dynenemies_gmenmy_mdef
end
#--------------------------------------------------------------------------
# * Get Basic Evasion
#--------------------------------------------------------------------------
def base_eva
return is_dynamic? ? @dyn_base_eva : seph_dynenemies_gmenmy_eva
end
#--------------------------------------------------------------------------
# * Exp
#--------------------------------------------------------------------------
def exp
return is_dynamic? ? @dyn_exp : seph_dynenemies_gmenmy_exp
end
#--------------------------------------------------------------------------
# * Gold
#--------------------------------------------------------------------------
def gold
return is_dynamic? ? @dyn_gold : seph_dynenemies_gmenmy_gold
end
end
#--------------------------------------------------------------------------
# * End SDK Enable Test
#--------------------------------------------------------------------------
end
#==============================================================================
# ** Dynamic Enemies - Quick Database Addition
#------------------------------------------------------------------------------
# SephirothSpawn
# Version 1
# 2007-08-05
# SDK : Version 2.0+, Part I
#------------------------------------------------------------------------------
# * Version History :
#
# Version 1 ---------------------------------------------------- (2006-08-06)
#------------------------------------------------------------------------------
# * Requirements :
#
# Dynamic Enemies System (3.0+)
# Method & Class Library 2.1+
#------------------------------------------------------------------------------
# * Description
#
# This script was designed to allow you to quickly modify your enemy stats
# rather than over-complicating things. It uses the quick point-slope
# formula (base_value + inflation * enemy_level). Here, whatever you input
# in the database will be the base_value. All you have to do is modify the
# inflation values for each stat for each enemy. Any modifications to the
# Stats constant in Dynamic Enemies system will be skipped (if you
# setup for the stats for enemy 1, this will NOT override those settings).
#------------------------------------------------------------------------------
# * Instructions
#
# Place The Script Below the SDK and Above Main.
# To Customize your enemy stats, refer to the customization instructions.
#------------------------------------------------------------------------------
# * Customization
#
# Setting Inflation Values
# - Inflation_Values = { enemy_id => { <stat_name> => inflation, ...}, ... }
#
# Stat Names : 'maxhp', 'maxsp', 'str', 'dex', 'agi', 'int', 'atk',
# 'pdef', 'mdef', 'eva', 'hit', 'exp', 'gold'
#==============================================================================
#------------------------------------------------------------------------------
# * SDK Log Script
#------------------------------------------------------------------------------
SDK.log('Dynamic Enemies - Quick Database Addition', 'SephirothSpawn', 1.0,
'2007-08-05')
SDK.check_requirements(2.0, [], {'Method & Class Library' => 2.1,
'Dynamic Enemies' => 3.0})
#------------------------------------------------------------------------------
# * Begin SDK Enable Test
#------------------------------------------------------------------------------
if SDK.enabled?('Dynamic Enemies - Quick Database Addition')
#==============================================================================
# ** Dynamic_Enemies
#==============================================================================
module Dynamic_Enemies
#--------------------------------------------------------------------------
# * Inflation Values
#
# Setting Inflation Values
# - Inflation_Values = {
# enemy_id => { <stat_name> => inflation, ...}, ...
# }
#
# Simple Modification Instrucitons
#
# 1) Below this line : Inflation_Values = {}, for each of your enemies,
# add:
#
# Inflation_Values[enemy_id] = {}
#
# 2) For each of your enemies stats, add:
#
# Inflation_Values[enemy_id][<stat_name>] = inflation_value
#
# 3) Repeat for all enemies
#
# 4) Setting default for all enemeis, for all stats
#
# Default_Inflation_Values[<stat_name>] = inflation
#
#
# Stat Names : 'maxhp', 'maxsp', 'str', 'dex', 'agi', 'int', 'atk',
# 'pdef', 'mdef', 'eva', 'hit', 'exp', 'gold'
#
# NOTE : I have set hit to have a base value as 100. You can find it down
# in the Do Not Modify section if you really want to modify it. I
# just wouldn't suggest it.
#--------------------------------------------------------------------------
Inflation_Values = {}
#--------------------------------------------------------------------------
# * Default Settings
#--------------------------------------------------------------------------
Default_Inflation_Values = {}
Default_Inflation_Values['maxhp'] = 100
Default_Inflation_Values['maxsp'] = 50
Default_Inflation_Values['str'] = 3
Default_Inflation_Values['dex'] = 3
Default_Inflation_Values['agi'] = 3
Default_Inflation_Values['int'] = 3
Default_Inflation_Values['atk'] = 0
Default_Inflation_Values['pdef'] = 0
Default_Inflation_Values['mdef'] = 0
Default_Inflation_Values['eva'] = 0
Default_Inflation_Values['hit'] = 0
Default_Inflation_Values['exp'] = 1
Default_Inflation_Values['gold'] = 2
#--------------------------------------------------------------------------
# * Do Not Modify
#--------------------------------------------------------------------------
# Loads enemy data
data_enemies = load_data('Data/Enemies.rxdata')
# Create stats array
stats = ['maxhp', 'maxsp', 'str', 'dex', 'agi', 'int', 'atk',
'pdef', 'mdef', 'eva', 'hit', 'exp', 'gold']
# Pass through all enemies
for i in 1...data_enemies.size
# Create enemy stat hash unless already made
Stats[i] = {} unless Stats.has_key?(i)
# Pass Through all stats
for stat in stats
# Next if stat curve already set up
next if Stats[i].has_key?(stat)
# Get Base Value from Database
if stat == 'hit'
base = 100
else
base = eval "data_enemies[i].#{stat}"
end
# Get Inflation Value
if Inflation_Values.has_key?(i) &&
Inflation_Values[i].has_key?(stat)
inflation = Inflation_Values[i][stat]
else
inflation = Default_Inflation_Values[stat]
end
# Set Stat Curve
Stats[i][stat] = [0, Min_Levels[i], Max_Levels[i], base, inflation]
end
end
end
#--------------------------------------------------------------------------
# * End SDK Enable Test
#--------------------------------------------------------------------------
end
#==========================================================================
# ** SG Optional Battle Music
#==========================================================================
# sandgolem
# Version 1
# 24.06.06
#==========================================================================
# Switch number to activate the Even Music
#
#Also note, in Event enabling this switch, change battle win ME to null
Scene_Map::SG_Disable_BattleMusic = 27
#==========================================================================
#
# To check for updates or find more scripts, visit:
# http://www.gamebaker.com/rmxp/scripts/
#
# To use this script, copy it and insert it in a new section above "Main",
# under the default scripts, and the SDK if you're using it.
#
# Have problems? Official topic:
# http://forums.gamebaker.com/showthread.php?t=13
#
#==========================================================================
begin
SDK.log('SG Optional Battle Music', 'sandgolem', 1, '24.06.06')
if SDK.state('SG Optional Battle Music') != true
@sg_nobattlebgm_disabled = true
end
rescue
end
if !@sg_nobattlebgm_disabled
#--------------------------------------------------------------------------
class Game_System
alias sandgolem_nobattlebgm_system_bgm bgm_play
def bgm_play(bgm)
if !$sg_keep_music
sandgolem_nobattlebgm_system_bgm(bgm)
end
end
alias sandgolem_nobattlebgm_system_bgmstop bgm_stop
def bgm_stop
if !$sg_keep_music
sandgolem_nobattlebgm_system_bgmstop
end
end
end
class Scene_Map
alias sandgolem_nobattlebgm_map_callbattle call_battle
def call_battle
if $game_switches[SG_Disable_BattleMusic]
$sg_keep_music = true
end
sandgolem_nobattlebgm_map_callbattle
$sg_keep_music = nil
end
end
#--------------------------------------------------------------------------
end
#==============================================================================
# * Hima's Elemental Reflector v. 1.3
#------------------------------------------------------------------------------
# This script will allow you to set your character or enemies to reflect any element
# you want. You can also create armors or skills that create elemental reflector.
#
# Version History
# ---------------------------------------------------------
# 1.0 - First Released
# 1.1 - Fixed infinite reflect problem
# 1.2 - More features added
# a.) You can now choose to reflect any physical or magical skill.
# b.) Physical reflect will also reflect any basic attack.
# c.) Basic attack with element will also be reflected from elemental reflector.
# 1.3 - Fixed some bugs that occur when you don't put all the ELEMENTS you defined into the database.
# ---------------------------------------------------------
# contact : ninomiya_mako@hotmail.com
#==============================================================================
module HIMA_REFLECT
#--------------------------------------------------------------------------
# - Customize Point -
# ELEMENTS - This is where you create the name of element you want to reflect. This is how it works
# {Name => Reflected Element ID, Name => Reflected Element ID, ...}
# The name can be anything, and ID is ID of the element that this will reflect. You have to
# create a new element that match what you input here.
# Special Elment ID are as follows :
#
# "Physical" -> For any physical skill, with atk_f greater than 0
# "Magic" -> For any magic skill, with int_f (mind_f) greater than 0
#
# WORD - Message that will pop up when reflection occurs.
#--------------------------------------------------------------------------
ELEMENTS = {"Reflect Fire" => 1,"Reflect Ice" => 2,"Reflect Thunder" => 3,"Reflect Water" => 4,"Reflect Earth" => 5,"Reflect Wind" => 6,"Reflect Light" => 7,"Reflect Darkness" => 8,"Deflector" => "Physical","Reflector" => "Magic"}
WORD = "Reflect"
end
module RPG
class Skill
def type_of_skill
if @int_f > 0
return "Magic"
elsif @atk_f > 0
return "Physical"
else
return nil
end
end
end
end
class Game_Battler
attr_accessor :reflect # Reflect Flag
alias hima_reflect_init initialize
def initialize
@reflect = 0 #0 = no reflect, 1 = basic_attack, 2 = skill_effect
hima_reflect_init
end
alias hima_reflect_attack_effect attack_effect
def attack_effect(attacker)
# Check for reflect basic attack
element_to_reflect = HIMA_REFLECT::ELEMENTS.index("Physical")
reflect_id = $data_system.elements.index(element_to_reflect)
if reflect_id != nil
if self.element_reflect(reflect_id) == 1
if attacker.element_reflect(reflect_id) == 1
self.damage = "Block"
self.reflect = 0
else
self.damage = HIMA_REFLECT::WORD
self.reflect = 1
end
return false
end
end
# End of reflect basic attack
# Check for reflect element of basic attack
for q in 0...attacker.element_set.size
element_id = attacker.element_set[q]
element_to_reflect = HIMA_REFLECT::ELEMENTS.index(element_id)
if element_to_reflect != nil
reflect_id = $data_system.elements.index(element_to_reflect)
if self.element_reflect(reflect_id) == 1
if attacker.element_reflect(reflect_id) == 1
self.damage = "Block"
self.reflect = 0
else
self.damage = HIMA_REFLECT::WORD
self.reflect = 1
end
return false
end
end
end #end for
# End of reflect element of basic attack
hima_reflect_attack_effect(attacker)
end
alias hima_reflect_skill_effect skill_effect
def skill_effect(user, skill)
# Reflect physical or magic skill
if skill.type_of_skill != nil
element_id = skill.type_of_skill
element_to_reflect = HIMA_REFLECT::ELEMENTS.index(element_id)
if element_to_reflect != nil
reflect_id = $data_system.elements.index(element_to_reflect)
if reflect_id != nil
if self.element_reflect(reflect_id) == 1
if user.element_reflect(reflect_id) == 1
self.damage = "Block"
self.reflect = 0
else
self.damage = HIMA_REFLECT::WORD
self.reflect = 2
end
return false
end
end
end
end
# Reflect specific element
for q in 0...skill.element_set.size
element_id = skill.element_set[q]
element_to_reflect = HIMA_REFLECT::ELEMENTS.index(element_id)
if element_to_reflect != nil
reflect_id = $data_system.elements.index(element_to_reflect)
if reflect_id != nil
if self.element_reflect(reflect_id) == 1
if user.element_reflect(reflect_id) == 1
self.damage = "Block"
self.reflect = 0
else
self.damage = HIMA_REFLECT::WORD
self.reflect = 2
end
return false
end
end
end
end #end for
hima_reflect_skill_effect(user,skill)
end
def element_reflect(element_id)
if self.is_a?(Game_Actor)
result = $data_classes[self.class_id].element_ranks[element_id]
for i in [@armor1_id, @armor2_id, @armor3_id, @armor4_id]
armor = $data_armors[i]
if armor != nil and armor.guard_element_set.include?(element_id)
result = 1
end
end
for i in @states
if $data_states[i].guard_element_set.include?(element_id)
result = 1
end
end
else
result = $data_enemies[self.enemy_id].element_ranks[element_id]
for i in @states
if $data_states[i].guard_element_set.include?(element_id)
result = 1
end
end
end
# Method end
return result
end
end
class Game_Enemy < Game_Battler
attr_accessor :enemy_id
end
class Scene_Battle
alias hima_update_phase4_step5 update_phase4_step5
def update_phase4_step5
hima_update_phase4_step5
reflect = 0
for target in @target_battlers
if target.reflect != 0
reflect = target.reflect
target.reflect = 0
end
end
if reflect > 0
@target_battlers = []
@target_battlers.push(@active_battler)
case reflect
when 1
@active_battler.attack_effect(@active_battler)
when 2
@active_battler.skill_effect(@active_battler,@skill)
end #case
@phase4_step = 4
else
@phase4_step = 6
end
end
end
$scene = Scene_Party.new(4, [1])
I believe that it is referring to this part of the code:Script 'Dargor's Party Changer v3.3' line 1376: ArgumentError occurred.
comparison of Fixnum with Array failed
if Input.trigger?(Input::C)
member = @members_window.members[@members_window.index]
return if member.locked
if @party_window.members.size < @max_actors[team_index] and
not @party_window.members.include?(member) and
not $game_temp.selected_members.include?(member)
for i in 0...@max_actors[team_index]
actor = @members_window.members[@members_window.index]
next if @party_window.members[i] != nil
next if @party_window.members.include?(actor)
@members_window.selected_members[@members_window.index] = true
@members_window.refresh
@status_window.refresh
@party_window.members[i] = actor
@party_window.refresh
end
return
end
end