silver wind
Member
This is a fun little script I made for spicing up events
Try the demo, it's fun
:D
screenshots:
Features:Try the demo, it's fun
:D
screenshots:
spawn bunnies:
shooting fireballs
replace bunnies with blood stains :
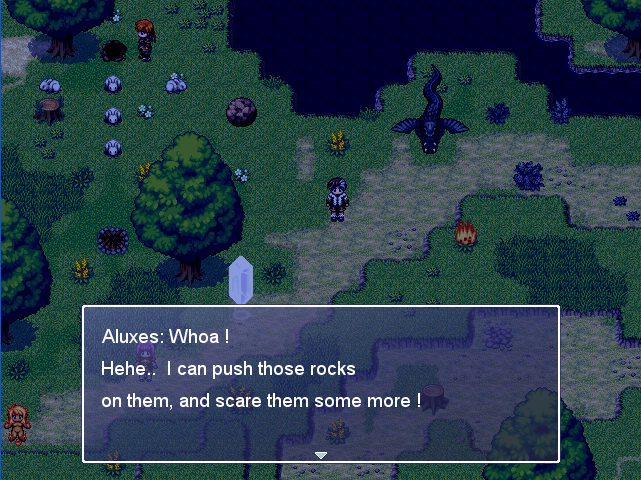
shooting fireballs

replace bunnies with blood stains :
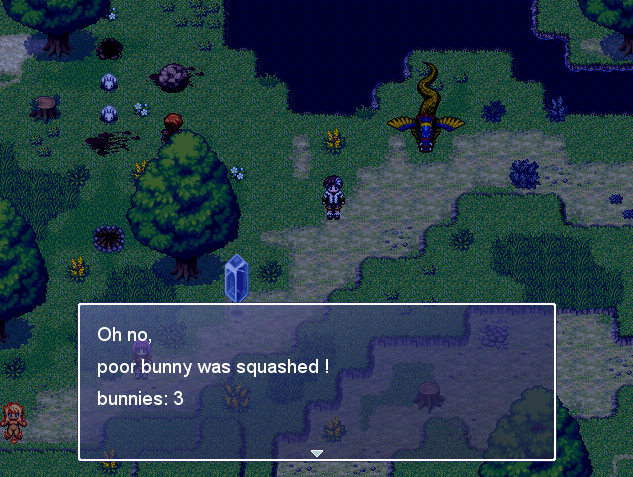
You'll need to place this dll in the project folder: uabm.dll
Place each script in a new page, my script goes below Additional bitmap methods.
Advanced event commands :
[rgss]#----------------------------------------------------#
# Advanced event commands #
# #
# made by: Silver Wind #
# v1.1 #
#----------------------------------------------------#
# Instructions: #
# -Use one of the following lines inside a #
# Conditional Branch (in the script box). #
# #
# event_is_at?(x,y) #
# taken_tile?(x,y) #
# #
# - To check if 2 events touch, use: #
# event_touch?(event1,event2) #
# #
# -To create a new event, put this code in a #
# script box. It can be used for bullets, etc. #
# #
# method 1 : #
# clone_event(event, x, y, name) #
# - event: name or id of an existing event. #
# - name: optional. can be left out. #
# #
# method 2 : #
# spawn_event(sprite, x, y, name, through) * #
# move_route_copy(from,to) ** #
# #
# * name, through, x ,y are all optional. #
# by deafult, through=false and name=EV00X #
# ** Set the move route of your event. #
# from & to can be the event name or the id. #
#----------------------------------------------------#
class Game_Character
# flag: has a collision occured?
def collision( flag, x, y )
return if (self == $game_player)
@last_collision = @collision
if not flag
@collision = nil
else
# collision: true
@collision = [x,y]
end
end
def collision?(x,y)
return (@collision == [x,y] or @last_collision == [x,y] )
end
def any_collision?
# @last_collision gives RMXP more time
# to detect the collision: (the time it takes the event to move 2 tiles.)
return (@collision != nil or @last_collision != nil )
end
def passable?(x, y, d)
if self.is_a?(Game_Event) and self.erased
return false
end
# Get new coordinates
new_x = x + (d == 6 ? 1 : d == 4 ? -1 : 0)
new_y = y + (d == 2 ? 1 : d == 8 ? -1 : 0)
# If coordinates are outside of map
unless $game_map.valid?(new_x, new_y)
# impassable
# edit: collision with map borders
collision(true, new_x, new_y)
return false
end
# If through is ON
if @through
# edit: event is on Through. check collision.
# collistion with tiles
tile_colide = ! $game_map.passable?(new_x, new_y, 10 - d)
# collistion with events
for event in $game_map.events.values
if event.x == new_x and event.y == new_y
event_colide = true
end
break if event_colide
end
colide = (event_colide or tile_colide)
collision(colide, new_x, new_y)
# passable
return true
end
# If unable to leave first move tile in designated direction
unless $game_map.passable?(x, y, d, self)
# edit: NOT a collision. the event is on a non-passable tile
# impassable
return false
end
# If unable to enter move tile in designated direction
unless $game_map.passable?(new_x, new_y, 10 - d)
# impassable
# edit: collision with something
collision(true, new_x, new_y)
return false
end
# Loop all events
for event in $game_map.events.values
# If event coordinates are consistent with move destination
if event.x == new_x and event.y == new_y
# new - collision will ignore events Through flag
# need testing
collision(true, new_x, new_y)
# If through is OFF
unless event.through
# If self is event
if self != $game_player
# impassable
return false
end
# With self as the player and partner graphic as character
if event.character_name != ""
# impassable
return false
end
end
end
end
# If player coordinates are consistent with move destination
if $game_player.x == new_x and $game_player.y == new_y
# If through is OFF
unless $game_player.through
# If your own graphic is the character
if @character_name != ""
# impassable
return false
end
end
end
# passable
collision(false, new_x, new_y)
return true
end
end
class Game_Event < Game_Character
attr_reader :erased
attr_reader :character_name
attr_reader :event
attr_reader :direction
attr_reader :move_frequency
# un used. doesn't seem to work
def moving_to?(x, y)
d = @direction
# Get new coordinates
new_x = @x + (d == 6 ? 1 : d == 4 ? -1 : 0)
new_y = @y + (d == 2 ? 1 : d == 8 ? -1 : 0)
return (new_x == x and new_y == y and not moving?)
end
end
class Game_Temp
attr_accessor :new_events
end
class Game_Character
attr_reader :move_route
end
class Game_Event
def name
return @event.name
end
def get_speed
return @move_speed
end
def set_speed(s)
@move_speed = s
update
end
def set_frequency(f)
@move_frequency = f
update
end
end
class Spriteset_Map
def add_sprite(event_id)
if event_id.is_a?(Array)
event_id.each {|id| add_sprite(id) }
return
end
id = event_id
sprite = Sprite_Character.new(@viewport1, $game_map.events[id])
@character_sprites.push(sprite)
end
alias new_event_update update
def update
event_ids = $game_temp.new_events
unless event_ids.nil?
add_sprite(event_ids)
$game_temp.new_events = nil
end
new_event_update
end
end
class Game_Map
attr_reader :map
alias eventing_initialize initialize
def initialize
eventing_initialize
@groups = Hash.new
end
def occupied_tile?(x,y)
return false if @map.events.keys.size ==0
for i in @map.events.keys
e = @events
if (e.x == x) and (e.y==y)
return true
end
end
# check tiles
return !passable?(x, y, 0)
end
def new_event(sprite, x=0, y=0, name=nil, through=false, group=nil)
ids = @map.events.keys
event_id = events_id_max
# was event_id = (ids.size==0) ? 1 : ((ids.max) +1)
newx = x
newy = y
# if the tile is taken, select an empty nearby tile.
while (occupied_tile?(newx,newy))
# advance non equally, to get tiles like: (1,15)
(rand(1) ==0) ? newx += 1 : newy += 1
end
new_event = RPG::Event.new(newx,newy)
new_event.id = event_id
new_event.name = name.nil? ? "EV00" + event_id.to_s : name
# set the graphic of the event
new_event.pages[0].graphic.character_name = sprite
new_event.pages[0].through = through
l = @map.events.keys.length
@map.events.keys[l]=event_id
@map.events[event_id]=new_event
game_event = Game_Event.new(@map_id, new_event)
@events[event_id] = game_event
add_to_group(event_id, group) if not group.nil?
# This data is used in Spriteset_Map to add the new sprite.
if $game_temp.new_events.nil?
$game_temp.new_events = [event_id]
else
$game_temp.new_events.push(event_id)
end
return event_id
end
#
#-------------------------------------------------------#
# Event Groups #
# if you have a bunch of projectiles flying around #
# and you need to check if any of them hits an event, #
# group them under the same name. #
#-------------------------------------------------------#
def add_to_group(event_id, group)
@groups[group] = [] if (@groups[group]).nil?
@groups[group].push(event_id)
end
#
def get_group(name)
return @groups(ids.max) +1)
end
# ----------------------------------------------#
# these 3 methods are defined again in #
# Additional bitmap methods #
# ----------------------------------------------#
def get_event(key)
if key.is_a?(String)
return get_event_by_name(key)
else
return get_event_by_id(key)
end
end
def get_event_by_name(name)
for i in @events.keys
ev = @events
return ev if ev.name == name
end
#p "Error: No such event #{name}"
return nil
end
def get_event_by_id(i)
for k in @events.keys
ev = @events[k]
return ev if ev.id== i
end
#p "Error: No such event #{name}"
return nil
end
# -------------------------------------------------- #
# ** event_touch? #
# more like event collide. will only return true #
# if one event is moving towards the other event. #
# -------------------------------------------------- #
def event_touch?(event1, event2)
e1 = get_event(event1)
e2 = get_event(event2)
return false if e1.nil? or e2.nil?
# known bug: when creatng an event, erasing it,
# and creating new one with the same name, event.erased = true
return false if e1.erased or e2.erased
return ( collision?(event1, event2) )
end
#
def collision?(event1, event2)
e1 = get_event(event1)
e2 = get_event(event2)
return true if e1.collision?(e2.x, e2.y)
return true if e2.collision?(e1.x, e1.y)
return false
end
end
# ------------------------------------------------------------------ #
class Interpreter
attr_reader :event_id
def get_character(parameter)
# Branch by parameter
case parameter
when -1 # player
return $game_player
when 0 # this event
events = $game_map.events
return events == nil ? nil : events[@event_id]
else # specific event
events = $game_map.events
return events == nil ? nil : get_event(parameter) #events[parameter]
end
end
def get_event(key)
if key.is_a?(String)
return get_event_by_name(key)
else
return get_event_by_id(key)
end
end
def get_event_by_name(name)
events = $game_map.events
for i in events.keys
ev = events
return ev if ev.name == name
end
# Error: No such event
return nil
end
def get_event_by_id(i)
events = $game_map.events
for k in events.keys
ev = events[k]
return ev if ev.id== i
end
# Error: No such event
return nil
end
def erase_event(event)
e = get_character(event)
e.erase
end
def collision?(event1, event2)
e1 = get_character(event1)
e2 = get_character(event2)
return true if e1.collision?(e2.x, e2.y)
return true if e2.collision?(e1.x, e1.y)
return false
end
# did the event colide with ANYTHING.
# including trees, and any map event (through is ignored).
def colide?
e1 = get_character(0)
return e1.any_collision?
end
# -------------------------------------------------- #
# ** event_touch? #
# event 1,2 : event name or id #
# more like event collide. will only return true #
# if one event is moving towards the other event. #
# -------------------------------------------------- #
def event_touch?(event2, event1=nil)
if event1.nil?
# use the calling event
event1 = get_character(0).id
end
e1 = get_character(event1)
e2 = get_character(event2)
return false if e1.nil? or e2.nil?
# known bug: when creatng an event, erasing it,
# and creating new one with the same name, event.erased = true
return false if e1.erased or e2.erased
return ( collision?(event1, event2) )
end
# from, to : event name or id
def move_route_copy(from, to)
events = $game_map.events
if from.is_a?(String)
from = get_event_by_name(from)
from = from.id
end
if to.is_a?(String)
event = get_event_by_name(to)
else
event = events[to]
end
move = events[from].move_route
event.force_move_route(move)
speed = events[from].get_speed
event.set_speed(speed)
freq = events[from].move_frequency
event.set_frequency(freq)
end
def taken_tile?(x,y)
player = get_character(-1)
return true if (player.x==x) and (player.y==y)
return $game_map.occupied_tile?(x,y)
end
def below?(event)
x = get_character(0).x
ev = get_character(event)
return event_at?(x, ev.y+1)
end
def above?(event)
x = get_character(0).x
ev = get_character(event)
return event_at?(x, ev.y-1)
end
def left_of?(event)
y = get_character(0).y
ev = get_character(event)
return event_at?(ev.x-1, y)
end
def right_of?(event)
y = get_character(0).y
ev = get_character(event)
return event_at?(ev.x+1, y)
end
def spawn_event(sprite, x ,y, name, through)
$game_map.new_event(sprite, x, y, name, through)
end
def clone_event(event, x=0, y=0, name=nil, group=nil)
$game_map.clone_event(event, x, y, name, group)
end
def event_at?(x,y)
e = get_character(0)
return (e.x==x and e.y==y)
end
def group_collison?(group)
event1 = get_character(0).id
return $game_map.group_collison?(event1, group)
end
def swap(event1,event2)
e = get_character(event1) #$game_map.events
d = e.direction
@parameters = [event1, 2, event2, d]
# set event location
command_202
end
# The 1st event will be erased
def replace(event1, event2)
swap(event1,event2)
erase_event(event1)
end
end
# ------------------------------------------------------------------ #
# this part was below Additional bitmap methods, but it seems to work here too.
#==============================================================================
# * Edit by Silver Wind
#==============================================================================
class Game_Event
def name
return @event.name
end
end
#-------------------------------------------------------------------------#
class Game_Map
def get_event(key)
if key.is_a?(String)
return get_event_by_name(key)
else
return get_event_by_id(key)
end
end
def get_event_by_name(name)
for i in @events.keys
ev = @events
return ev if ev.name == name
end
# Error: No such event
return nil
end
def get_event_by_id(i)
for k in @events.keys
ev = @events[k]
return ev if ev.id== i
end
# Error: No such event
return nil
end
end
#-------------------------------------------------------------------------#
class Scene_Map
def bitmap_effect(event, action, amount=nil)
ev = $game_map.get_event(event)
sprite = @spriteset.get_sprite(ev)
return if ev.nil?
case (action)
when 'brighten'
sprite.brighten_by(amount)
when 'darken'
sprite.darken_by(amount)
else
eval ("sprite.#{action}")
end
<span style="color:#000080; font-style:italic;">=begin
<span style="color:#000080; font-style:italic;"> when 'invert'
<span style="color:#000080; font-style:italic;"> sprite.invert
<span style="color:#000080; font-style:italic;"> when 'grayscale'
<span style="color:#000080; font-style:italic;"> sprite.grayscale
<span style="color:#000080; font-style:italic;"> when 'hyper_contrast'
<span style="color:#000080; font-style:italic;"> sprite.hyper_contrast
<span style="color:#000080; font-style:italic;"> when 'monochrome'
<span style="color:#000080; font-style:italic;"> sprite.monochrome
<span style="color:#000080; font-style:italic;"> when 'emboss'
<span style="color:#000080; font-style:italic;"> sprite.emboss
<span style="color:#000080; font-style:italic;"> when 'sepia'
<span style="color:#000080; font-style:italic;"> sprite.sepia
<span style="color:#000080; font-style:italic;"> when 'sharpen'
<span style="color:#000080; font-style:italic;"> sprite.sharpen
<span style="color:#000080; font-style:italic;"> when 'soften'
<span style="color:#000080; font-style:italic;"> sprite.soften
<span style="color:#000080; font-style:italic;"> end
<span style="color:#000080; font-style:italic;">=end
end
end
#-------------------------------------------------------------------------#
class Spriteset_Map
def get_sprite(event)
for sprite in @character_sprites
ev = sprite.character
# if it's the same Game_Event object.
if ev.object_id == event.object_id
target_sprite = sprite
end
end
return target_sprite
end
end
#-------------------------------------------------------------------------#
class Sprite_Character < RPG::Sprite
def brighten_by(x)
self.bitmap.brighten(x)
end
def darken_by(x)
self.bitmap.darken(x)
end
def invert
self.bitmap.invert
end
def grayscale
self.bitmap.grayscale
end
def hyper_contrast
self.bitmap.hyper_contrast
end
def monochrome
self.bitmap.monochrome
end
def emboss
self.bitmap.emboss
end
def sepia
self.bitmap.sepia
end
def sharpen
self.bitmap.sharpen
end
def soften
self.bitmap.soften
end
def update
super
# If tile ID, file name, or hue are different from current ones
if @tile_id != @character.tile_id or
@character_name != @character.character_name or
@character_hue != @character.character_hue
# Remember tile ID, file name, and hue
@tile_id = @character.tile_id
@character_name = @character.character_name
@character_hue = @character.character_hue
# If tile ID value is valid
if @tile_id >= 384
#----------------------------- edit -----------------------------#
# clone the bitmap before using it for an event.
bitmap = RPG::Cache.tile($game_map.tileset_name,
@tile_id, @character.character_hue)
self.bitmap = bitmap.clone
#----------------------------------------------------------------#
self.src_rect.set(0, 0, 32, 32)
self.ox = 16
self.oy = 32
# If tile ID value is invalid
else
#----------------------------- edit -----------------------------#
# clone the bitmap before using it for an event.
bitmap = RPG::Cache.character(@character.character_name,
@character.character_hue)
self.bitmap = bitmap.clone
#----------------------------------------------------------------#
@cw = bitmap.width / 4
@ch = bitmap.height / 4
self.ox = @cw / 2
self.oy = @ch
end
end
# Set visible situation
self.visible = (not @character.transparent)
# If graphic is character
if @tile_id == 0
# Set rectangular transfer
sx = @character.pattern * @cw
sy = (@character.direction - 2) / 2 * @ch
self.src_rect.set(sx, sy, @cw, @ch)
end
# Set sprite coordinates
self.x = @character.screen_x
self.y = @character.screen_y
self.z = @character.screen_z(@ch)
# Set opacity level, blend method, and bush depth
self.opacity = @character.opacity
self.blend_type = @character.blend_type
self.bush_depth = @character.bush_depth
# Animation
if @character.animation_id != 0
animation = $data_animations[@character.animation_id]
animation(animation, true)
@character.animation_id = 0
end
end
end
#-------------------------------------------------------------------------#
class Interpreter
def brighten(amount,event=nil)
#return unless $scene.is_a?(Scene_Map)
event = get_character(0).id if event.nil?
$scene.bitmap_effect(event,'brighten',amount)
end
def darken(amount,event=nil)
event = get_character(0).id if event.nil?
$scene.bitmap_effect(event,'darken',amount)
end
def invert(event)
$scene.bitmap_effect(event,'invert')
end
def grayscale(event)
$scene.bitmap_effect(event,'grayscale')
end
def bitmap_effect(action,event=nil,amount=nil)
event = get_character(0).id if event.nil?
$scene.bitmap_effect(event,action,amount)
end
end
[/rgss]
Additional Bitmap Methods 0.9
(it has its own thread here, posted here for convenience)
[rgss]#==============================================================================
class Bitmap
#------------------------------------------------------------------------------
#Make sure you have uabm.dll in your project folder.
#You can get it from (assuming link is not dead):
#http://www.box.net/shared/9m54d8hkzu
#------------------------------------------------------------------------------
Brighten = Win32API.new("uabm.dll", "Brighten", "li", "v")
Darken = Win32API.new("uabm.dll", "Darken", "li", "v")
Invert = Win32API.new("uabm.dll", "Invert", "l", "v")
Grayscale = Win32API.new("uabm.dll", "Grayscale", "l", "v")
Hcontrast = Win32API.new("uabm.dll", "Hcontrast", "l", "v")
Monochrome = Win32API.new("uabm.dll", "Monochrome", "l", "v")
Emboss = Win32API.new("uabm.dll", "Emboss", "l", "v")
Sepia = Win32API.new("uabm.dll", "Sepia", "l", "v")
Sharpen = Win32API.new("uabm.dll", "Sharpen", "l", "v")
Soften = Win32API.new("uabm.dll", "Soften", "l", "v")
#--------------------------------------------------------------------------
# * Brighten (0...255)
#--------------------------------------------------------------------------
def brighten(amount)
Brighten.call(self.__id__, amount)
end
#--------------------------------------------------------------------------
# * Darken (0...255)
#--------------------------------------------------------------------------
def darken(amount)
Darken.call(self.__id__, amount)
end
#--------------------------------------------------------------------------
# * Invert
#--------------------------------------------------------------------------
def invert
Invert.call(self.__id__)
end
#--------------------------------------------------------------------------
# * Grayscale
#--------------------------------------------------------------------------
def grayscale
Grayscale.call(self.__id__)
end
#--------------------------------------------------------------------------
# * Hyper Contrast
#--------------------------------------------------------------------------
def hyper_contrast
Hcontrast.call(self.__id__)
end
#--------------------------------------------------------------------------
# * Monochrome
#--------------------------------------------------------------------------
def monochrome
Monochrome.call(self.__id__)
end
#--------------------------------------------------------------------------
# * Emboss {buggy}
#--------------------------------------------------------------------------
def emboss
Emboss.call(self.__id__)
end
#--------------------------------------------------------------------------
# * Sepia
#--------------------------------------------------------------------------
def sepia
Sepia.call(self.__id__)
end
#--------------------------------------------------------------------------
# * Sharpen {will update with numeric value options}
#--------------------------------------------------------------------------
def sharpen
Sharpen.call(self.__id__)
end
#--------------------------------------------------------------------------
# * Soften {will update with numeric value options}
#--------------------------------------------------------------------------
def soften
Soften.call(self.__id__)
end
end
[/rgss]">
Place each script in a new page, my script goes below Additional bitmap methods.
Advanced event commands :
[rgss]#----------------------------------------------------#
# Advanced event commands #
# #
# made by: Silver Wind #
# v1.1 #
#----------------------------------------------------#
# Instructions: #
# -Use one of the following lines inside a #
# Conditional Branch (in the script box). #
# #
# event_is_at?(x,y) #
# taken_tile?(x,y) #
# #
# - To check if 2 events touch, use: #
# event_touch?(event1,event2) #
# #
# -To create a new event, put this code in a #
# script box. It can be used for bullets, etc. #
# #
# method 1 : #
# clone_event(event, x, y, name) #
# - event: name or id of an existing event. #
# - name: optional. can be left out. #
# #
# method 2 : #
# spawn_event(sprite, x, y, name, through) * #
# move_route_copy(from,to) ** #
# #
# * name, through, x ,y are all optional. #
# by deafult, through=false and name=EV00X #
# ** Set the move route of your event. #
# from & to can be the event name or the id. #
#----------------------------------------------------#
class Game_Character
# flag: has a collision occured?
def collision( flag, x, y )
return if (self == $game_player)
@last_collision = @collision
if not flag
@collision = nil
else
# collision: true
@collision = [x,y]
end
end
def collision?(x,y)
return (@collision == [x,y] or @last_collision == [x,y] )
end
def any_collision?
# @last_collision gives RMXP more time
# to detect the collision: (the time it takes the event to move 2 tiles.)
return (@collision != nil or @last_collision != nil )
end
def passable?(x, y, d)
if self.is_a?(Game_Event) and self.erased
return false
end
# Get new coordinates
new_x = x + (d == 6 ? 1 : d == 4 ? -1 : 0)
new_y = y + (d == 2 ? 1 : d == 8 ? -1 : 0)
# If coordinates are outside of map
unless $game_map.valid?(new_x, new_y)
# impassable
# edit: collision with map borders
collision(true, new_x, new_y)
return false
end
# If through is ON
if @through
# edit: event is on Through. check collision.
# collistion with tiles
tile_colide = ! $game_map.passable?(new_x, new_y, 10 - d)
# collistion with events
for event in $game_map.events.values
if event.x == new_x and event.y == new_y
event_colide = true
end
break if event_colide
end
colide = (event_colide or tile_colide)
collision(colide, new_x, new_y)
# passable
return true
end
# If unable to leave first move tile in designated direction
unless $game_map.passable?(x, y, d, self)
# edit: NOT a collision. the event is on a non-passable tile
# impassable
return false
end
# If unable to enter move tile in designated direction
unless $game_map.passable?(new_x, new_y, 10 - d)
# impassable
# edit: collision with something
collision(true, new_x, new_y)
return false
end
# Loop all events
for event in $game_map.events.values
# If event coordinates are consistent with move destination
if event.x == new_x and event.y == new_y
# new - collision will ignore events Through flag
# need testing
collision(true, new_x, new_y)
# If through is OFF
unless event.through
# If self is event
if self != $game_player
# impassable
return false
end
# With self as the player and partner graphic as character
if event.character_name != ""
# impassable
return false
end
end
end
end
# If player coordinates are consistent with move destination
if $game_player.x == new_x and $game_player.y == new_y
# If through is OFF
unless $game_player.through
# If your own graphic is the character
if @character_name != ""
# impassable
return false
end
end
end
# passable
collision(false, new_x, new_y)
return true
end
end
class Game_Event < Game_Character
attr_reader :erased
attr_reader :character_name
attr_reader :event
attr_reader :direction
attr_reader :move_frequency
# un used. doesn't seem to work
def moving_to?(x, y)
d = @direction
# Get new coordinates
new_x = @x + (d == 6 ? 1 : d == 4 ? -1 : 0)
new_y = @y + (d == 2 ? 1 : d == 8 ? -1 : 0)
return (new_x == x and new_y == y and not moving?)
end
end
class Game_Temp
attr_accessor :new_events
end
class Game_Character
attr_reader :move_route
end
class Game_Event
def name
return @event.name
end
def get_speed
return @move_speed
end
def set_speed(s)
@move_speed = s
update
end
def set_frequency(f)
@move_frequency = f
update
end
end
class Spriteset_Map
def add_sprite(event_id)
if event_id.is_a?(Array)
event_id.each {|id| add_sprite(id) }
return
end
id = event_id
sprite = Sprite_Character.new(@viewport1, $game_map.events[id])
@character_sprites.push(sprite)
end
alias new_event_update update
def update
event_ids = $game_temp.new_events
unless event_ids.nil?
add_sprite(event_ids)
$game_temp.new_events = nil
end
new_event_update
end
end
class Game_Map
attr_reader :map
alias eventing_initialize initialize
def initialize
eventing_initialize
@groups = Hash.new
end
def occupied_tile?(x,y)
return false if @map.events.keys.size ==0
for i in @map.events.keys
e = @events
if (e.x == x) and (e.y==y)
return true
end
end
# check tiles
return !passable?(x, y, 0)
end
def new_event(sprite, x=0, y=0, name=nil, through=false, group=nil)
ids = @map.events.keys
event_id = events_id_max
# was event_id = (ids.size==0) ? 1 : ((ids.max) +1)
newx = x
newy = y
# if the tile is taken, select an empty nearby tile.
while (occupied_tile?(newx,newy))
# advance non equally, to get tiles like: (1,15)
(rand(1) ==0) ? newx += 1 : newy += 1
end
new_event = RPG::Event.new(newx,newy)
new_event.id = event_id
new_event.name = name.nil? ? "EV00" + event_id.to_s : name
# set the graphic of the event
new_event.pages[0].graphic.character_name = sprite
new_event.pages[0].through = through
l = @map.events.keys.length
@map.events.keys[l]=event_id
@map.events[event_id]=new_event
game_event = Game_Event.new(@map_id, new_event)
@events[event_id] = game_event
add_to_group(event_id, group) if not group.nil?
# This data is used in Spriteset_Map to add the new sprite.
if $game_temp.new_events.nil?
$game_temp.new_events = [event_id]
else
$game_temp.new_events.push(event_id)
end
return event_id
end
#
#-------------------------------------------------------#
# Event Groups #
# if you have a bunch of projectiles flying around #
# and you need to check if any of them hits an event, #
# group them under the same name. #
#-------------------------------------------------------#
def add_to_group(event_id, group)
@groups[group] = [] if (@groups[group]).nil?
@groups[group].push(event_id)
end
#
def get_group(name)
return @groups(ids.max) +1)
end
# ----------------------------------------------#
# these 3 methods are defined again in #
# Additional bitmap methods #
# ----------------------------------------------#
def get_event(key)
if key.is_a?(String)
return get_event_by_name(key)
else
return get_event_by_id(key)
end
end
def get_event_by_name(name)
for i in @events.keys
ev = @events
return ev if ev.name == name
end
#p "Error: No such event #{name}"
return nil
end
def get_event_by_id(i)
for k in @events.keys
ev = @events[k]
return ev if ev.id== i
end
#p "Error: No such event #{name}"
return nil
end
# -------------------------------------------------- #
# ** event_touch? #
# more like event collide. will only return true #
# if one event is moving towards the other event. #
# -------------------------------------------------- #
def event_touch?(event1, event2)
e1 = get_event(event1)
e2 = get_event(event2)
return false if e1.nil? or e2.nil?
# known bug: when creatng an event, erasing it,
# and creating new one with the same name, event.erased = true
return false if e1.erased or e2.erased
return ( collision?(event1, event2) )
end
#
def collision?(event1, event2)
e1 = get_event(event1)
e2 = get_event(event2)
return true if e1.collision?(e2.x, e2.y)
return true if e2.collision?(e1.x, e1.y)
return false
end
end
# ------------------------------------------------------------------ #
class Interpreter
attr_reader :event_id
def get_character(parameter)
# Branch by parameter
case parameter
when -1 # player
return $game_player
when 0 # this event
events = $game_map.events
return events == nil ? nil : events[@event_id]
else # specific event
events = $game_map.events
return events == nil ? nil : get_event(parameter) #events[parameter]
end
end
def get_event(key)
if key.is_a?(String)
return get_event_by_name(key)
else
return get_event_by_id(key)
end
end
def get_event_by_name(name)
events = $game_map.events
for i in events.keys
ev = events
return ev if ev.name == name
end
# Error: No such event
return nil
end
def get_event_by_id(i)
events = $game_map.events
for k in events.keys
ev = events[k]
return ev if ev.id== i
end
# Error: No such event
return nil
end
def erase_event(event)
e = get_character(event)
e.erase
end
def collision?(event1, event2)
e1 = get_character(event1)
e2 = get_character(event2)
return true if e1.collision?(e2.x, e2.y)
return true if e2.collision?(e1.x, e1.y)
return false
end
# did the event colide with ANYTHING.
# including trees, and any map event (through is ignored).
def colide?
e1 = get_character(0)
return e1.any_collision?
end
# -------------------------------------------------- #
# ** event_touch? #
# event 1,2 : event name or id #
# more like event collide. will only return true #
# if one event is moving towards the other event. #
# -------------------------------------------------- #
def event_touch?(event2, event1=nil)
if event1.nil?
# use the calling event
event1 = get_character(0).id
end
e1 = get_character(event1)
e2 = get_character(event2)
return false if e1.nil? or e2.nil?
# known bug: when creatng an event, erasing it,
# and creating new one with the same name, event.erased = true
return false if e1.erased or e2.erased
return ( collision?(event1, event2) )
end
# from, to : event name or id
def move_route_copy(from, to)
events = $game_map.events
if from.is_a?(String)
from = get_event_by_name(from)
from = from.id
end
if to.is_a?(String)
event = get_event_by_name(to)
else
event = events[to]
end
move = events[from].move_route
event.force_move_route(move)
speed = events[from].get_speed
event.set_speed(speed)
freq = events[from].move_frequency
event.set_frequency(freq)
end
def taken_tile?(x,y)
player = get_character(-1)
return true if (player.x==x) and (player.y==y)
return $game_map.occupied_tile?(x,y)
end
def below?(event)
x = get_character(0).x
ev = get_character(event)
return event_at?(x, ev.y+1)
end
def above?(event)
x = get_character(0).x
ev = get_character(event)
return event_at?(x, ev.y-1)
end
def left_of?(event)
y = get_character(0).y
ev = get_character(event)
return event_at?(ev.x-1, y)
end
def right_of?(event)
y = get_character(0).y
ev = get_character(event)
return event_at?(ev.x+1, y)
end
def spawn_event(sprite, x ,y, name, through)
$game_map.new_event(sprite, x, y, name, through)
end
def clone_event(event, x=0, y=0, name=nil, group=nil)
$game_map.clone_event(event, x, y, name, group)
end
def event_at?(x,y)
e = get_character(0)
return (e.x==x and e.y==y)
end
def group_collison?(group)
event1 = get_character(0).id
return $game_map.group_collison?(event1, group)
end
def swap(event1,event2)
e = get_character(event1) #$game_map.events
d = e.direction
@parameters = [event1, 2, event2, d]
# set event location
command_202
end
# The 1st event will be erased
def replace(event1, event2)
swap(event1,event2)
erase_event(event1)
end
end
# ------------------------------------------------------------------ #
# this part was below Additional bitmap methods, but it seems to work here too.
#==============================================================================
# * Edit by Silver Wind
#==============================================================================
class Game_Event
def name
return @event.name
end
end
#-------------------------------------------------------------------------#
class Game_Map
def get_event(key)
if key.is_a?(String)
return get_event_by_name(key)
else
return get_event_by_id(key)
end
end
def get_event_by_name(name)
for i in @events.keys
ev = @events
return ev if ev.name == name
end
# Error: No such event
return nil
end
def get_event_by_id(i)
for k in @events.keys
ev = @events[k]
return ev if ev.id== i
end
# Error: No such event
return nil
end
end
#-------------------------------------------------------------------------#
class Scene_Map
def bitmap_effect(event, action, amount=nil)
ev = $game_map.get_event(event)
sprite = @spriteset.get_sprite(ev)
return if ev.nil?
case (action)
when 'brighten'
sprite.brighten_by(amount)
when 'darken'
sprite.darken_by(amount)
else
eval ("sprite.#{action}")
end
<span style="color:#000080; font-style:italic;">=begin
<span style="color:#000080; font-style:italic;"> when 'invert'
<span style="color:#000080; font-style:italic;"> sprite.invert
<span style="color:#000080; font-style:italic;"> when 'grayscale'
<span style="color:#000080; font-style:italic;"> sprite.grayscale
<span style="color:#000080; font-style:italic;"> when 'hyper_contrast'
<span style="color:#000080; font-style:italic;"> sprite.hyper_contrast
<span style="color:#000080; font-style:italic;"> when 'monochrome'
<span style="color:#000080; font-style:italic;"> sprite.monochrome
<span style="color:#000080; font-style:italic;"> when 'emboss'
<span style="color:#000080; font-style:italic;"> sprite.emboss
<span style="color:#000080; font-style:italic;"> when 'sepia'
<span style="color:#000080; font-style:italic;"> sprite.sepia
<span style="color:#000080; font-style:italic;"> when 'sharpen'
<span style="color:#000080; font-style:italic;"> sprite.sharpen
<span style="color:#000080; font-style:italic;"> when 'soften'
<span style="color:#000080; font-style:italic;"> sprite.soften
<span style="color:#000080; font-style:italic;"> end
<span style="color:#000080; font-style:italic;">=end
end
end
#-------------------------------------------------------------------------#
class Spriteset_Map
def get_sprite(event)
for sprite in @character_sprites
ev = sprite.character
# if it's the same Game_Event object.
if ev.object_id == event.object_id
target_sprite = sprite
end
end
return target_sprite
end
end
#-------------------------------------------------------------------------#
class Sprite_Character < RPG::Sprite
def brighten_by(x)
self.bitmap.brighten(x)
end
def darken_by(x)
self.bitmap.darken(x)
end
def invert
self.bitmap.invert
end
def grayscale
self.bitmap.grayscale
end
def hyper_contrast
self.bitmap.hyper_contrast
end
def monochrome
self.bitmap.monochrome
end
def emboss
self.bitmap.emboss
end
def sepia
self.bitmap.sepia
end
def sharpen
self.bitmap.sharpen
end
def soften
self.bitmap.soften
end
def update
super
# If tile ID, file name, or hue are different from current ones
if @tile_id != @character.tile_id or
@character_name != @character.character_name or
@character_hue != @character.character_hue
# Remember tile ID, file name, and hue
@tile_id = @character.tile_id
@character_name = @character.character_name
@character_hue = @character.character_hue
# If tile ID value is valid
if @tile_id >= 384
#----------------------------- edit -----------------------------#
# clone the bitmap before using it for an event.
bitmap = RPG::Cache.tile($game_map.tileset_name,
@tile_id, @character.character_hue)
self.bitmap = bitmap.clone
#----------------------------------------------------------------#
self.src_rect.set(0, 0, 32, 32)
self.ox = 16
self.oy = 32
# If tile ID value is invalid
else
#----------------------------- edit -----------------------------#
# clone the bitmap before using it for an event.
bitmap = RPG::Cache.character(@character.character_name,
@character.character_hue)
self.bitmap = bitmap.clone
#----------------------------------------------------------------#
@cw = bitmap.width / 4
@ch = bitmap.height / 4
self.ox = @cw / 2
self.oy = @ch
end
end
# Set visible situation
self.visible = (not @character.transparent)
# If graphic is character
if @tile_id == 0
# Set rectangular transfer
sx = @character.pattern * @cw
sy = (@character.direction - 2) / 2 * @ch
self.src_rect.set(sx, sy, @cw, @ch)
end
# Set sprite coordinates
self.x = @character.screen_x
self.y = @character.screen_y
self.z = @character.screen_z(@ch)
# Set opacity level, blend method, and bush depth
self.opacity = @character.opacity
self.blend_type = @character.blend_type
self.bush_depth = @character.bush_depth
# Animation
if @character.animation_id != 0
animation = $data_animations[@character.animation_id]
animation(animation, true)
@character.animation_id = 0
end
end
end
#-------------------------------------------------------------------------#
class Interpreter
def brighten(amount,event=nil)
#return unless $scene.is_a?(Scene_Map)
event = get_character(0).id if event.nil?
$scene.bitmap_effect(event,'brighten',amount)
end
def darken(amount,event=nil)
event = get_character(0).id if event.nil?
$scene.bitmap_effect(event,'darken',amount)
end
def invert(event)
$scene.bitmap_effect(event,'invert')
end
def grayscale(event)
$scene.bitmap_effect(event,'grayscale')
end
def bitmap_effect(action,event=nil,amount=nil)
event = get_character(0).id if event.nil?
$scene.bitmap_effect(event,action,amount)
end
end
[/rgss]
Additional Bitmap Methods 0.9
(it has its own thread here, posted here for convenience)
[rgss]#==============================================================================
class Bitmap
#------------------------------------------------------------------------------
#Make sure you have uabm.dll in your project folder.
#You can get it from (assuming link is not dead):
#http://www.box.net/shared/9m54d8hkzu
#------------------------------------------------------------------------------
Brighten = Win32API.new("uabm.dll", "Brighten", "li", "v")
Darken = Win32API.new("uabm.dll", "Darken", "li", "v")
Invert = Win32API.new("uabm.dll", "Invert", "l", "v")
Grayscale = Win32API.new("uabm.dll", "Grayscale", "l", "v")
Hcontrast = Win32API.new("uabm.dll", "Hcontrast", "l", "v")
Monochrome = Win32API.new("uabm.dll", "Monochrome", "l", "v")
Emboss = Win32API.new("uabm.dll", "Emboss", "l", "v")
Sepia = Win32API.new("uabm.dll", "Sepia", "l", "v")
Sharpen = Win32API.new("uabm.dll", "Sharpen", "l", "v")
Soften = Win32API.new("uabm.dll", "Soften", "l", "v")
#--------------------------------------------------------------------------
# * Brighten (0...255)
#--------------------------------------------------------------------------
def brighten(amount)
Brighten.call(self.__id__, amount)
end
#--------------------------------------------------------------------------
# * Darken (0...255)
#--------------------------------------------------------------------------
def darken(amount)
Darken.call(self.__id__, amount)
end
#--------------------------------------------------------------------------
# * Invert
#--------------------------------------------------------------------------
def invert
Invert.call(self.__id__)
end
#--------------------------------------------------------------------------
# * Grayscale
#--------------------------------------------------------------------------
def grayscale
Grayscale.call(self.__id__)
end
#--------------------------------------------------------------------------
# * Hyper Contrast
#--------------------------------------------------------------------------
def hyper_contrast
Hcontrast.call(self.__id__)
end
#--------------------------------------------------------------------------
# * Monochrome
#--------------------------------------------------------------------------
def monochrome
Monochrome.call(self.__id__)
end
#--------------------------------------------------------------------------
# * Emboss {buggy}
#--------------------------------------------------------------------------
def emboss
Emboss.call(self.__id__)
end
#--------------------------------------------------------------------------
# * Sepia
#--------------------------------------------------------------------------
def sepia
Sepia.call(self.__id__)
end
#--------------------------------------------------------------------------
# * Sharpen {will update with numeric value options}
#--------------------------------------------------------------------------
def sharpen
Sharpen.call(self.__id__)
end
#--------------------------------------------------------------------------
# * Soften {will update with numeric value options}
#--------------------------------------------------------------------------
def soften
Soften.call(self.__id__)
end
end
[/rgss]">
- Replacing an event with a blood graphic:
[rgss]blood_stain = clone_event("blood")
replace( e, blood_stain )
[/rgss]
replace 'e' with the event's name or id.
'blood' is a name of an existing event on the map.
if you don't want to have a blood event already on the map,
you can use:
[rgss]blood_stain = spawn_event(sprite, x ,y, name, through)
replace( e, blood_stain )
[/rgss]
- check collision with any events in the 'fireballs' group:
first, clone a fireball and group your it under 'fireballs':
clone_event("fireball_event",x,y,name,'fireballs')
in a map event, in cond. branch, use
group_collision?("fireballs")
- check if event is above/below another event:
[rgss]above?(e)
below?(e)
left_of?(e)
right_of?(e)
[/rgss]
replace e with event's name / id number.
- Bitmap methods:
[rgss]brighten(e, x)
darken(e, x)
invert(e)
grayscale(e)
[/rgss]
replace x with a number (1-255, I believe)
[rgss]blood_stain = clone_event("blood")
replace( e, blood_stain )
[/rgss]
replace 'e' with the event's name or id.
'blood' is a name of an existing event on the map.
if you don't want to have a blood event already on the map,
you can use:
[rgss]blood_stain = spawn_event(sprite, x ,y, name, through)
replace( e, blood_stain )
[/rgss]
- check collision with any events in the 'fireballs' group:
first, clone a fireball and group your it under 'fireballs':
clone_event("fireball_event",x,y,name,'fireballs')
in a map event, in cond. branch, use
group_collision?("fireballs")
- check if event is above/below another event:
[rgss]above?(e)
below?(e)
left_of?(e)
right_of?(e)
[/rgss]
replace e with event's name / id number.
- Bitmap methods:
[rgss]brighten(e, x)
darken(e, x)
invert(e)
grayscale(e)
[/rgss]
replace x with a number (1-255, I believe)
- Includes Additional Bitmap Methods by Untra & Glitchfinder
- Invert /grayscale / lighten/ darken a sprite
- Spawn new events
- Clone events
- Detect event touch event (even if set to Through)
- Organize events in groups
- Detect when collision occurs with any event in the group.
- Check event's x,y easily
- Detect if event is above/below/right/left of another event
- Detect when events collide with anything on the map, including tiles.
- Detect when events hit the map borders.
- Handle the spawned events using script:
- erase event
- swap with another event
I plan on adding new features like a case statement using comments, and an option to use items on a map event.
Tell me if have an idea for other cool features.
Enjoy.
If you use this, credit Untra & Glitchfinder.">