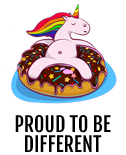
Icedmetal57;271028 said:...
And for Icechamp's question, here's the solution. You may have to scroll a bit, depending on how you're web browser is. Anyway, there's the problem you're having. That 0 as shown in red here, should be a 1 for it to automatically validate the registrants. You'll have to manually validate those users already registered by changing that 0 to a 1 in the database in the users table.
Code:if ((isset($_POST["MM_insert"])) && ($_POST["MM_insert"] == "form1")) { $insertSQL = sprintf("INSERT INTO users (id, login, password, email, reg_dat, validation) VALUES ('', %s, MD5(%s), %s, UNIX_TIMESTAMP(), [color=red]0[/color]);", GetSQLValueString($_POST['login'], "text"), GetSQLValueString($_POST['password'], "text"), GetSQLValueString($_POST['email'], "text"));
Well, I hope that I helped most of you guys and cleared up a few questions as well.
<?php require_once('Connections/Netplay.php'); ?>
<?php
function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "")
{
$theValue = (!get_magic_quotes_gpc()) ? addslashes($theValue) : $theValue;
switch ($theType) {
case "text":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "long":
case "int":
$theValue = ($theValue != "") ? intval($theValue) : "NULL";
break;
case "double":
$theValue = ($theValue != "") ? "'" . doubleval($theValue) . "'" : "NULL";
break;
case "date":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "defined":
$theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue;
break;
}
return $theValue;
}
$editFormAction = $_SERVER['PHP_SELF'];
if (isset($_SERVER['QUERY_STRING'])) {
$editFormAction .= "?" . htmlentities($_SERVER['QUERY_STRING']);
}
if ((isset($_POST["MM_insert"])) && ($_POST["MM_insert"] == "form1")) {
$insertSQL = sprintf("INSERT INTO users (id, login, password, email, reg_dat, validation) VALUES ('', %s, MD5(%s), %s, UNIX_TIMESTAMP(), 0);",
GetSQLValueString($_POST['login'], "text"),
GetSQLValueString($_POST['password'], "text"),
GetSQLValueString($_POST['email'], "text"));
mysql_select_db($database_Netplay, $Netplay);
$Result1 = mysql_query($insertSQL, $Netplay) or die(mysql_error());
$id = mysql_insert_id();
$insertSQL = "INSERT INTO temp_users (id,email,code) VALUES (".$id.",'".$_POST['email']."',MD5('".$_POST['email']."') );";
$Result2 = mysql_query($insertSQL, $Netplay) or die(mysql_error());
$message="Thank you ".$_POST['login']." for your registration on the Netplay Server.\n Please click on this link to confirm your email adress : \n
http://".$_SERVER['PHP_SELF']."/confirm.php?id=".$id."&confirm=".md5($_POST['email'])." \n";
$headers="From: trebor777@servage.net\n";
mail($_POST['email'],"Netplay: Confirm your email",$message,$headers);
$insertGoTo = "success.php";
if (isset($_SERVER['QUERY_STRING'])) {
$insertGoTo .= (strpos($insertGoTo, '?')) ? "&" : "?";
$insertGoTo .= $_SERVER['QUERY_STRING'];
}
header(sprintf("Location: %s", $insertGoTo));
}
mysql_select_db($database_Netplay, $Netplay);
$query_User = "SELECT * FROM users";
$User = mysql_query($query_User, $Netplay) or die(mysql_error());
$row_User = mysql_fetch_assoc($User);
$totalRows_User = mysql_num_rows($User);
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" />
<title>Netplay Registration Page</title>
</head>
<body>
<div align="center">
<p>Netplay Registration Page</p>
<form method="post" name="form1" action="<?php echo $editFormAction; ?>">
<table align="center">
<tr valign="baseline">
<td nowrap align="right">Login:</td>
<td><input type="text" name="login" value="" size="10" maxlength="8">
8 characters maximum</td>
</tr>
<tr valign="baseline">
<td nowrap align="right">Password:</td>
<td><input type="password" name="password" value="" size="10" maxlength="8">
8 characters maximum</td>
</tr>
<tr valign="baseline">
<td nowrap align="right">Email:</td>
<td><input type="text" name="email" value="" size="50" maxlength="255"></td>
</tr>
<tr valign="baseline">
<td nowrap align="right"> </td>
<td><input type="submit" value="Register"></td>
</tr>
</table>
<input type="hidden" name="MM_insert" value="form1">
</form>
<p> </p>
</div>
</body>
</html>
<?php
mysql_free_result($User);
?>
#==============================================================================
# ** Scene_Login
# - The login scene the player is first taken to.
#------------------------------------------------------------------------------
# Muhammet "Mr.Mo" Sivri
# Netplay Plus 2.0
# 1.0
# 02-04-2007
#------------------------------------------------------------------------------
# You can do some edits to this script in Settings.
#==============================================================================
SDK.log('Login', 'Mr.Mo', '2.0', '02-04-07')
# Disable if Network is disabled.
SDK.disable('Login') if !SDK.enabled?('Network')
#-------------------------------------------------------------------------------
# Begin SDK Enabled Check
#-------------------------------------------------------------------------------
if SDK.enabled?('Login')
#-------------------------------------------------------------------------------
class Scene_Login < SDK::Scene_Base
#----------------------------------------------------------------------------
# * Initialize Object
#----------------------------------------------------------------------------
def initialize
# Create Mouse Object
@sprr = 0
$Mouse = Game_Mouse.new
$mouse = $Mouse
$Mouse.visible
# Load database
$data_actors = load_data("Data/Actors.rxdata")
$data_classes = load_data("Data/Classes.rxdata")
$data_skills = load_data("Data/Skills.rxdata")
$data_items = load_data("Data/Items.rxdata")
$data_weapons = load_data("Data/Weapons.rxdata")
$data_armors = load_data("Data/Armors.rxdata")
$data_enemies = load_data("Data/Enemies.rxdata")
$data_troops = load_data("Data/Troops.rxdata")
$data_states = load_data("Data/States.rxdata")
$data_animations = load_data("Data/Animations.rxdata")
$data_tilesets = load_data("Data/Tilesets.rxdata")
$data_common_events = load_data("Data/CommonEvents.rxdata")
$data_system = load_data("Data/System.rxdata")
# Change Animation Size
for an in $data_animations
next if an == nil
frames = an.frames
for i in 0...frames.size
for j in 0...frames[i].cell_max
frames[i].cell_data[j, 1] /= $settings.animation_divide
frames[i].cell_data[j, 2] /= $settings.animation_divide
frames[i].cell_data[j, 3] /= $settings.animation_divide
end
end
end
# Data creation
$game_system = Game_System.new
$game_temp = Game_Temp.new
$game_netplay = Game_Netplay.new
# Network Initialization
Network.initialize
# Count
@count = $settings.server_check_time
@first = true
end
#--------------------------------------------------------------------------
# * Main Window
#--------------------------------------------------------------------------
def main_window
# Create Login
@login_window = Window_Login.new
# Create file if in Debug and it doesn't exist.
if $DEBUG and !FileTest.exist?("Settings.rxdata")
v = [false," "," "]
save_data(v,"Settings.rxdata")
end
# Get Data
set = load_data("Settings.rxdata")
@login_window.username.text = set[1].to_s if set[0]
@login_window.password.text = set[2].to_s if set[0]
# If the data is not remembered
if @login_window.username.text != ""
@login_window.rememberme.check
# Else if it is
else
@login_window.rememberme.uncheck
end
# Create Status window
@status_window = Window_LoginStatus.new
# Create Server Selection
create_server if $settings.display_server
end
#--------------------------------------------------------------------------
# * Main Processing : Transition
#--------------------------------------------------------------------------
def main_transition
Graphics.transition
# Create News
create_news if $settings.display_news
@first = false
end
#--------------------------------------------------------------------------
# * Main Sprite
#--------------------------------------------------------------------------
def main_sprite
# Background Media
@viewport1 = Viewport.new(0, 0, 640, 480)
@sprite2 = Sprite.new
@sprite2.z = 9999
@sprite2.bitmap = RPG::Cache.picture('snowfall_2')
@sprite = Plane.new(@viewport1)
@sprite.bitmap = RPG::Cache.title($data_system.title_name)
end
#--------------------------------------------------------------------------
# * Main Audio
#--------------------------------------------------------------------------
def main_audio
$game_system.bgm_play($data_system.title_bgm)
end
#--------------------------------------------------------------------------
# * Main Processing : Ending
#--------------------------------------------------------------------------
def main_end
$game_system.bgm_stop
end
#--------------------------------------------------------------------------
# * Create Server
#--------------------------------------------------------------------------
def create_server
ostatus = @status_window.status
@status_window.status = "Reseting server list. Please wait..."
# Get the servers
servers = {}
$settings.servers.each {|key, value|
# Check if server online or not
Network.test_connection(value[0],value[1])
status = "Online" if !Network.result
status = "Offline" if Network.result
servers[key] = status
# Reset
Network.testreset
# Update Graphics
Graphics.update if $settings.servers.size > 2
}
# Update Graphics
Graphics.update
# Dispose server window
@server_window = Window_Server.new if @server_window == nil
# Create server window
@server_window.refresh(servers)
# Reset Complete
@status_window.status = ostatus
# Create News
create_news if $settings.display_news and !@first
end
#--------------------------------------------------------------------------
# * Create News
#--------------------------------------------------------------------------
def create_news
# Get Selected Server
server = @server_window.server
return if server == nil
# Return if the selected server is offline
return if server[1] == "Offline"
# Connect temporarly
$settings.servers.each {|key, value|
if server[0].to_s == key.to_s
Network.connect(value[0],value[1])
# Get News
if Network.authenficate == "success"
Graphics.update
@news_window = Window_News.new if @news_window == nil
@news_window.get_news
else
print "You do not have the right gamecode or connection timed out."
end
end
}
end
#--------------------------------------------------------------------------
# * Login
#--------------------------------------------------------------------------
def login
return create_server if @server_window.server[1] == "Offline" or !@server_window.freeze
# Get Updates
result = Network.login(@login_window.username.text,@login_window.password.text)
if result == result.to_i.to_s
# Get Updates
Network.check_for_updates
# Get Guilds
Network.get_guilds
# Store Data
store_data
# Go to Character Creator
$scene = Scene_Character.new
else
@status_window.status = result.to_s
end
end
#--------------------------------------------------------------------------
# * Update
#--------------------------------------------------------------------------
def update
@sprite.ox += 1
update_server
end
def update_server
# Update Controls
if Input.trigger?(Input::DOWN) or Input.trigger?(Input::UP) or Input.trigger?(Keys::TAB)
if !@login_window.username.active
# Activate
@login_window.username.active = true
@login_window.password.active = false
elsif !@login_window.password.active
# Activate
@login_window.password.active = true
@login_window.username.active = false
end
end
# Update Count
@count -= 1
@count = 0 if $DEBUG and Input.press?(Input::CTRL)
# If Count is 0
if @count <= 0 and $settings.server_check_time > 0
@count = $settings.server_check_time
# Create Servers
create_server if @server_window.server[1] != "Online"
end
end
#--------------------------------------------------------------------------
# * Store Data
#--------------------------------------------------------------------------
def store_data
if @login_window.rememberme.checked
v = [true,"#{@login_window.username.text}","#{@login_window.password.text}"]
save_data(v,"Settings.rxdata")
else
v = [false," "," "]
save_data(v,"Settings.rxdata")
end
end
end
end