Kain Nobel
Member
Window : Command Icons
Version: 3.5
By: Kain Nobel
Introduction
Easily allows you to put icons in your command windows, without having to go through and edit other scripts.
Features
Screenshots
Script
Instructions
Place Above Main and Below SDK, this script is already set up for Title, Menu and Battle and End Game scenes. Just follow the example of how I set it up and it should be easy enough to understand. I would suggest having this script at the bottom of your scripts, just one up from Main.
FAQ
Compatibility
This script does use SDK, but with some editing you can make it non-SDK. If I have some time I'll make a non-SDK version, but really I expect you to be responsible enough to be able to figure that out on your own :P
Terms and Conditions
Another "Free to use in commercial and non-commercial games" script, but credit is required!
Version: 3.5
By: Kain Nobel
Introduction
Easily allows you to put icons in your command windows, without having to go through and edit other scripts.
Features
- Will put icons in any Window_Command (or Window_HorizCommand)
- No need to edit other scripts to achieve this effect
- Easy to use
Screenshots
The Title...
The Menu...
The Battle (Party Command)...
The Battle (Actor Command)...
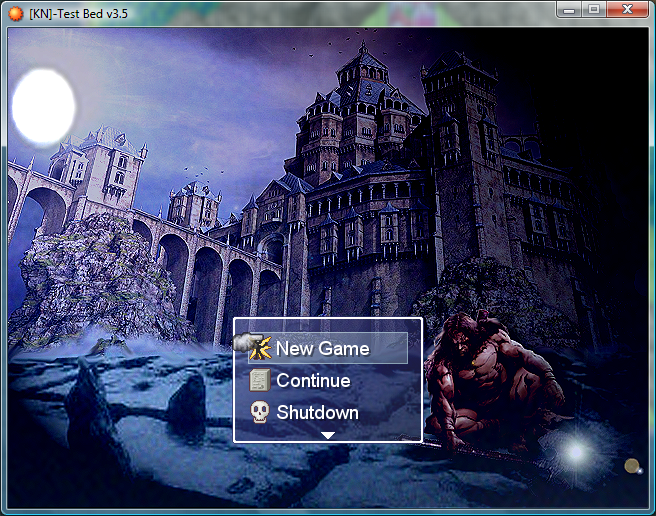
The Menu...
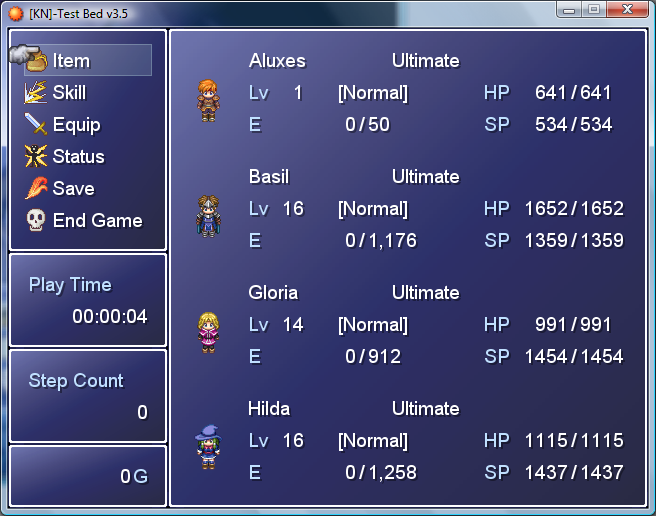
The Battle (Party Command)...
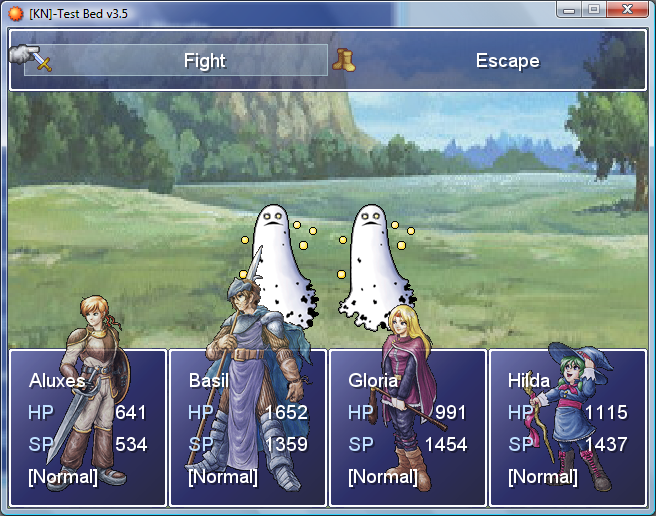
The Battle (Actor Command)...
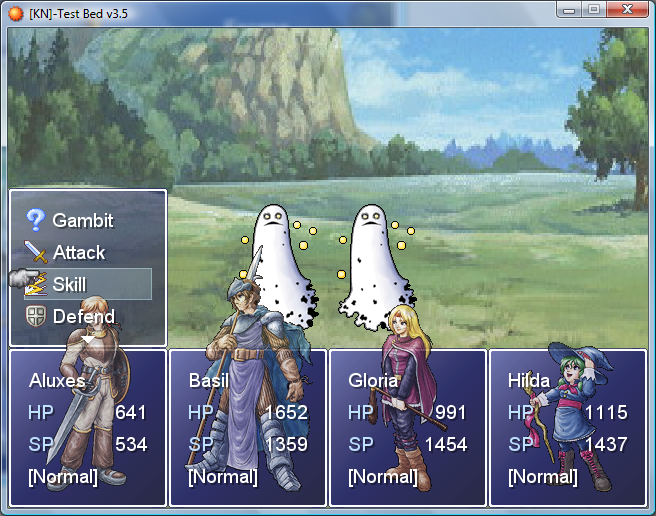
Script
Code:
#===============================================================================
# ** Window : Command Icons
#===============================================================================
#-------------------------------------------------------------------------------
# * SDK Log
#-------------------------------------------------------------------------------
SDK.log('Window.CommandIcons', 'Kain Nobel ©', 3.5, '2009.06.17')
#-------------------------------------------------------------------------------
# * SDK Enabled Test : Begin
#-------------------------------------------------------------------------------
if SDK.enabled?('Window.CommandIcons')
#===============================================================================
# ** Window_Base
#===============================================================================
class Window_Base < Window
#-----------------------------------------------------------------------------
# * Included Modules
#-----------------------------------------------------------------------------
include SDK::Vocab
#-----------------------------------------------------------------------------
# * Icons
#-----------------------------------------------------------------------------
Icons = Hash.new # Don't tamper with
Icons.default = Hash.new # Don't tamper with
Icons.default.default = Hash.new # Don't tamper with
Icons.default.default.default = Hash.new # Don't tamper with
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
# ** Scene_Title : @command_window #
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
s, w = 'Scene_Title', '@command_window'
Icons[s][w][Scene_Title::New_Game] = '050-Skill07'
Icons[s][w][Scene_Title::Continue] = '039-Item08'
Icons[s][w][Scene_Title::Shutdown] = '046-Skill03'
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
# ** Scene_Menu : @command_window #
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
s, w = 'Scene_Menu', '@command_window'
Icons[s][w][Scene_Menu::Item] = '032-Item01'
Icons[s][w][Scene_Menu::Skill] = '044-Skill01'
Icons[s][w][Scene_Menu::Equip] = '001-Weapon01'
Icons[s][w][Scene_Menu::Status] = '050-Skill07'
Icons[s][w][Scene_Menu::Save] = '037-Item06'
Icons[s][w][Scene_Menu::End_Game] = '046-Skill03'
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
# ** Scene_Batte : @actor_command_window #
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
s, w = 'Scene_Battle', '@actor_command_window'
Icons[s][w][Scene_Battle::Attack] = '001-Weapon01'
Icons[s][w][Scene_Battle::Skill] = '044-Skill01'
Icons[s][w][Scene_Battle::Guard] = '009-Shield01'
Icons[s][w][Scene_Battle::Item] = '032-Item01'
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
# ** Scene_Battle : @party_command_window #
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
s, w = 'Scene_Battle', '@party_command_window'
Icons[s][w][Scene_Battle::Fight] = '001-Weapon01'
Icons[s][w][Scene_Battle::Escape] = '020-Accessory05'
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
# ** Scene_End : @command_window
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
s, w = 'Scene_End', '@command_window'
#-----------------------------------------------------------------------------
# * Icon Settings
#-----------------------------------------------------------------------------
def icon_settings
if @icon_settings.nil?
scene_key = "#{$scene.class}"
window_key = get_scene_instance
@icon_settings = Icons[scene_key][window_key]
end
@icon_settings
end
#-----------------------------------------------------------------------------
# * Icon At?
#-----------------------------------------------------------------------------
def icon_at?(index)
settings = icon_settings
settings[@commands[index]]
end
#-----------------------------------------------------------------------------
# * Command Has Icon Key?
#-----------------------------------------------------------------------------
def command_icon_key?(index)
icon_settings.has_key?(@commands[index])
end
#-----------------------------------------------------------------------------
# * Command Has Icon Value?
#-----------------------------------------------------------------------------
def command_icon_value?(index)
icon_settings[@command[index]].is_a?(String)
end
#-----------------------------------------------------------------------------
# * Command Has Icon?
#-----------------------------------------------------------------------------
def command_icon?(index)
icon_settings.has_key?(@commands[index])
end
#-----------------------------------------------------------------------------
# * Command Icon
#-----------------------------------------------------------------------------
def command_icon(index)
RPG::Cache.icon(icon_at?(index))
end
#-----------------------------------------------------------------------------
# * Get Scene Instance
#-----------------------------------------------------------------------------
def get_scene_instance
$scene.instance_variables.each do |object_name|
object = $scene.instance_variable_get(object_name)
return object_name if object == self
end
end
end
#===============================================================================
# ** Window_Command
#===============================================================================
class Window_Command < Window_Selectable
#-----------------------------------------------------------------------------
# * Alias Listings
#-----------------------------------------------------------------------------
alias_method :commandicons_wincommand_drawitem, :draw_item
#-----------------------------------------------------------------------------
# * Draw Item
#-----------------------------------------------------------------------------
def draw_item(index, color)
unless command_icon?(index)
commandicons_wincommand_drawitem(index, color)
return
end
icon = command_icon(index)
op = (color == disabled_color ? 160 : 255)
self.contents.blt(0, 32 * index + 4, icon, icon.rect, op)
self.contents.font.color = color
rect = Rect.new(28, 32 * index, self.contents.width - 28, 32)
self.contents.fill_rect(rect, Color.new(0, 0, 0, 0))
self.contents.draw_text(rect, @commands[index])
end
end
#===============================================================================
# ** Window_HorizCommand
#===============================================================================
class Window_HorizCommand < Window_Selectable
#-----------------------------------------------------------------------------
# * Alias Listings
#-----------------------------------------------------------------------------
alias_method :commandicons_winhcommand_drawitem, :draw_item
#-----------------------------------------------------------------------------
# * Draw Item
#-----------------------------------------------------------------------------
def draw_item(index, color)
unless command_icon?(index)
commandicons_winhcommand_drawitem(index, color)
return
end
icon = command_icon(index)
op = (color == disabled_color ? 160 : 255)
x = index * @c_spacing + 4
self.contents.blt(x, 4, icon, icon.rect, op)
command = commands[index]
x += 28
self.contents.font.color = color
self.contents.draw_text(x, 0, @c_spacing - 8, 32, command, @alignment)
end
end
#-------------------------------------------------------------------------------
# * SDK Enabled Test : End
#-------------------------------------------------------------------------------
end
Code:
#===============================================================================
# ** Window : Command Icons
#===============================================================================
#-------------------------------------------------------------------------------
# * SDK Log
#-------------------------------------------------------------------------------
SDK.log('Window.CommandIcons', 'Kain Nobel ©', 3.5, '2009.06.17')
#-------------------------------------------------------------------------------
# * SDK Enabled Test : Begin
#-------------------------------------------------------------------------------
if SDK.enabled?('Window.CommandIcons')
#===============================================================================
# ** Window_Base
#===============================================================================
class Window_Base < Window
#-----------------------------------------------------------------------------
# * Included Modules
#-----------------------------------------------------------------------------
include SDK::Vocab
#-----------------------------------------------------------------------------
# * Icons
#-----------------------------------------------------------------------------
Icons = Hash.new # Don't tamper with
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
# ** Scene_Title : @command_window #
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
s, w = 'Scene_Title', '@command_window'
Icons[s] ||= Hash.new
Icons[s][w] = Hash.new
Icons[s][w][Scene_Title::New_Game] = '050-Skill07'
Icons[s][w][Scene_Title::Continue] = '039-Item08'
Icons[s][w][Scene_Title::Shutdown] = '046-Skill03'
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
# ** Scene_Menu : @command_window #
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
s, w = 'Scene_Menu', '@command_window'
Icons[s] ||= Hash.new
Icons[s][w] = Hash.new
Icons[s][w][Scene_Menu::Item] = '032-Item01'
Icons[s][w][Scene_Menu::Skill] = '044-Skill01'
Icons[s][w][Scene_Menu::Equip] = '001-Weapon01'
Icons[s][w][Scene_Menu::Status] = '050-Skill07'
Icons[s][w][Scene_Menu::Save] = '037-Item06'
Icons[s][w][Scene_Menu::End_Game] = '046-Skill03'
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
# ** Scene_Batte : @actor_command_window #
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
s, w = 'Scene_Battle', '@actor_command_window'
Icons[s] ||= Hash.new
Icons[s][w] = Hash.new
Icons[s][w][Scene_Battle::Attack] = '001-Weapon01'
Icons[s][w][Scene_Battle::Skill] = '044-Skill01'
Icons[s][w][Scene_Battle::Guard] = '009-Shield01'
Icons[s][w][Scene_Battle::Item] = '032-Item01'
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
# ** Scene_Battle : @party_command_window #
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
s, w = 'Scene_Battle', '@party_command_window'
Icons[s] ||= Hash.new
Icons[s][w] = Hash.new
Icons[s][w][Scene_Battle::Fight] = '001-Weapon01'
Icons[s][w][Scene_Battle::Escape] = '020-Accessory05'
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
# ** Scene_End : @command_window #
#::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::#
s, w = 'Scene_End', '@command_window'
Icons[s] ||= Hash.new
Icons[s][w] = Hash.new
Icons[s][w][Scene_End::To_Title] = '001-Weapon01'
Icons[s][w][Scene_End::Shutdown] = '001-Weapon01'
Icons[s][w][Scene_End::Cancel] = '001-Weapon01'
#-----------------------------------------------------------------------------
# * Update
#-----------------------------------------------------------------------------
alias_method :commandicons_winbase_update, :update
def update
commandicons_winbase_update
if self.respond_to?(:refresh) && self.respond_to?(:commands) && !@icons_set
self.refresh
@icons_set = true
end
end
#-----------------------------------------------------------------------------
# * Icon Settings
#-----------------------------------------------------------------------------
def icon_settings
scene_key = "#{$scene.class}"
window_key = get_scene_instance
if Icons.has_key?(scene_key) && Icons[scene_key].has_key?(window_key)
return Icons[scene_key][window_key]
end
end
#-----------------------------------------------------------------------------
# * Icon At?
#-----------------------------------------------------------------------------
def icon_at?(index)
settings = icon_settings
settings[@commands[index]]
end
#-----------------------------------------------------------------------------
# * Command Icon?
#-----------------------------------------------------------------------------
def command_icon?(index)
return false unless icon_settings.is_a?(Hash)
icons = icon_settings
icons.has_key?(@commands[index]) && icons[@commands[index]].is_a?(String)
end
#-----------------------------------------------------------------------------
# * Command Icon
#-----------------------------------------------------------------------------
def command_icon(index)
RPG::Cache.icon(icon_at?(index))
end
#-----------------------------------------------------------------------------
# * Get Scene Instance
#-----------------------------------------------------------------------------
def get_scene_instance
$scene.instance_variables.each do |object_name|
object = $scene.instance_variable_get(object_name)
next unless object.kind_of?(Window)
next unless object.respond_to?(:commands)
return object_name if object == self
end
end
end
#===============================================================================
# ** Window_Command
#===============================================================================
class Window_Command < Window_Selectable
#-----------------------------------------------------------------------------
# * Alias Listings
#-----------------------------------------------------------------------------
alias_method :commandicons_wincommand_drawitem, :draw_item
#-----------------------------------------------------------------------------
# * Draw Item
#-----------------------------------------------------------------------------
def draw_item(index, color)
unless command_icon?(index)
commandicons_wincommand_drawitem(index, color)
return
end
icon = command_icon(index)
op = (color == disabled_color ? 160 : 255)
self.contents.blt(0, 32 * index + 4, icon, icon.rect, op)
self.contents.font.color = color
rect = Rect.new(28, 32 * index, self.contents.width - 28, 32)
self.contents.fill_rect(rect, Color.new(0, 0, 0, 0))
self.contents.draw_text(rect, @commands[index])
end
end
#===============================================================================
# ** Window_HorizCommand
#===============================================================================
class Window_HorizCommand < Window_Selectable
#-----------------------------------------------------------------------------
# * Alias Listings
#-----------------------------------------------------------------------------
alias_method :commandicons_winhcommand_drawitem, :draw_item
#-----------------------------------------------------------------------------
# * Draw Item
#-----------------------------------------------------------------------------
def draw_item(index, color)
unless command_icon?(index)
commandicons_winhcommand_drawitem(index, color)
return
end
icon = command_icon(index)
op = (color == disabled_color ? 160 : 255)
x = index * @c_spacing + 4
self.contents.blt(x, 4, icon, icon.rect, op)
command = commands[index]
x += 28
self.contents.font.color = color
self.contents.draw_text(x, 0, @c_spacing - 8, 32, command, @alignment)
end
end
#-------------------------------------------------------------------------------
# * SDK Enabled Test : End
#-------------------------------------------------------------------------------
end
Place Above Main and Below SDK, this script is already set up for Title, Menu and Battle and End Game scenes. Just follow the example of how I set it up and it should be easy enough to understand. I would suggest having this script at the bottom of your scripts, just one up from Main.
FAQ
First thing you need to do is set your keys for Scene and Window, where s is the name of the $scene the window will be in, and w is the name of the instance variable which represents the window. Basically, an example would be...
s, w = 'Scene_Menu', '@command_window'
Then, you have to create a new hash within Icons constant for it...
Icons ||= Hash.new # This will create a new hash for the scene, only if it isn't defined
Icons[w] = Hash.new # This will create a new hash for the window
Then you set all your icons like so...
Icons[w]["Command Name"] = "icon filename"
The end result should look like...
...and thats basically how it works...
s, w = 'Scene_Menu', '@command_window'
Then, you have to create a new hash within Icons constant for it...
Icons
Icons
Then you set all your icons like so...
Icons
The end result should look like...
Code:
s, w = 'Scene_Menu', '@command_window'
Icons[s] ||= Hash.new
Icons[s][w] = Hash.new
Icons[s][w]['My Command'] = 'My Icon'
...and thats basically how it works...
Compatibility
This script does use SDK, but with some editing you can make it non-SDK. If I have some time I'll make a non-SDK version, but really I expect you to be responsible enough to be able to figure that out on your own :P
Terms and Conditions
Another "Free to use in commercial and non-commercial games" script, but credit is required!