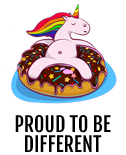
class Window_Item
alias_method :seph_keyitemssimple_wnitm_init, :initialize
def initialize
seph_keyitemssimple_wnitm_init
if $scene.is_a?(Scene_Item)
self.y += 64
self.height -= 64
self.active = false
end
end
#--------------------------------------------------------------------------
# * Filter Element Set
#--------------------------------------------------------------------------
def filter_element_set(element_tag = 20, call_refresh = false)
refresh if call_refresh
@data.each do |item|
if item.is_a?(RPG::Item) || item.is_a?(RPG::Weapon)
@data.delete(item) unless item.element_set.include?(element_tag)
elsif item.is_a?(RPG::Armor)
@data.delete(item) unless item.guard_element_set.include?(element_tag)
end
end
# If item count is not 0, make a bit map and draw all items
@item_max = @data.size
if @item_max > 0
self.contents = Bitmap.new(width - 32, row_max * 32)
for i in 0...@item_max
draw_item(i)
end
end
end
end
#==============================================================================
# ** Window_HorizCommand
#------------------------------------------------------------------------------
# This window deals with general command choices. (Horizontal)
#==============================================================================
class Window_HorizCommand < Window_Selectable
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_reader :commands
attr_accessor :c_spacing
attr_accessor :alignment
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize(width, commands, c_spacing = (width - 32) / commands.size)
# Compute window height from command quantity
super(0, 0, width, 64)
@commands = commands
@item_max = commands.size
@column_max = @item_max
@c_spacing = c_spacing
@alignment = 1
self.contents = Bitmap.new(@item_max * @c_spacing, height - 32)
refresh
self.index = 0
end
#--------------------------------------------------------------------------
# * Command
#--------------------------------------------------------------------------
def command(index = self.index)
return @commands[index]
end
#--------------------------------------------------------------------------
# * Commands
#--------------------------------------------------------------------------
def commands=(commands)
# Return if Commands Are Same
return if @commands == commands
# Reset Commands
@commands = commands
# Resets Item Max
item_max = @item_max
@item_max = @commands.size
@column_max = @item_max
# If Item Max Changes
unless item_max == @item_max
# Deletes Existing Contents (If Exist)
unless self.contents.nil?
self.contents.dispose
self.contents = nil
end
# Recreates Contents
self.contents = Bitmap.new(@item_max * @c_spacing, height - 32)
end
# Refresh Window
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
for i in 0...@item_max
draw_item(i, normal_color)
end
end
#--------------------------------------------------------------------------
# * Draw Item
#--------------------------------------------------------------------------
def draw_item(index, color)
command = commands[index]
x = index * @c_spacing + 4
self.contents.font.color = color
self.contents.draw_text(x, 0, @c_spacing - 8, 32, command, @alignment)
end
#--------------------------------------------------------------------------
# * Disable Item
#--------------------------------------------------------------------------
def disable_item(index)
draw_item(index, disabled_color)
end
#--------------------------------------------------------------------------
# * Cursor Rectangle Update
#--------------------------------------------------------------------------
def update_cursor_rect
if @index < 0
self.cursor_rect.empty
else
self.cursor_rect.set(@c_spacing * @index, 0, @c_spacing, 32)
end
end
end
class Scene_Item
alias_method :seph_keyitemssimple_scnitm_main, :main
alias_method :seph_keyitemssimple_scnitm_update, :update
def main
@item_kind_select = Window_HorizCommand.new(640, ['Items', 'Key Items'])
@item_kind_select.y = 64
seph_keyitemssimple_scnitm_main
@item_kind_select.dispose
end
def update
if @item_kind_select.active
@item_kind_select.update
if Input.trigger?(Input::B)
$game_system.se_play($data_system.cancel_se)
$scene = Scene_Menu.new
return
end
if Input.trigger?(Input::C)
$game_system.se_play($data_system.decison_se)
@item_kind_select.active = false
@item_window.active = true
end
if Input.repeat?(Input::RIGHT) || Input.repeat?(Input::LEFT)
if @item_kind_select.index == 0
@item_window.refresh
else
@item_window.filter_element_set
end
end
return
end
seph_keyitemssimple_scnitm_update
end
end
class Window_Item
alias_method :seph_keyitemssimple_wnitm_init, :initialize
def initialize
seph_keyitemssimple_wnitm_init
if $scene.is_a?(Scene_Item)
self.y += 64
self.height -= 64
self.active = false
end
end
#--------------------------------------------------------------------------
# * Filter Element Set
#--------------------------------------------------------------------------
def filter_element_set(element_tag = 17, call_refresh = false)
refresh if call_refresh
redata = []
@data.each do |item|
if item.is_a?(RPG::Item) || item.is_a?(RPG::Weapon)
redata << item if item.element_set.include?(element_tag)
elsif item.is_a?(RPG::Armor)
redata << item if item.guard_element_set.include?(element_tag)
end
end
@data = redata
# If item count is not 0, make a bit map and draw all items
@item_max = @data.size
if @item_max > 0
self.contents = Bitmap.new(width - 32, row_max * 32)
for i in 0...@item_max
draw_item(i)
end
end
end
end
class Scene_Item
alias_method :seph_keyitemssimple_scnitm_main, :main
alias_method :seph_keyitemssimple_scnitm_update, :update
def main
@item_kind_select = Window_HorizCommand.new(640, ['Items', 'Key Items'])
@item_kind_select.y = 64
seph_keyitemssimple_scnitm_main
@item_kind_select.dispose
end
def update
if @item_kind_select.active
@item_kind_select.update
if Input.trigger?(Input::B)
$game_system.se_play($data_system.cancel_se)
$scene = Scene_Menu.new
return
end
if Input.trigger?(Input::C)
$game_system.se_play($data_system.decision_se)
@item_kind_select.active = false
@item_window.active = true
end
if Input.repeat?(Input::RIGHT) || Input.repeat?(Input::LEFT)
if @item_kind_select.index == 0
@item_window.refresh
else
@item_window.filter_element_set
end
end
return
end
seph_keyitemssimple_scnitm_update
end
end
#==============================================================================
# ** Window_HorizCommand
#------------------------------------------------------------------------------
# This window deals with general command choices. (Horizontal)
#==============================================================================
class Window_HorizCommand < Window_Selectable
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_reader :commands
attr_accessor :c_spacing
attr_accessor :alignment
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize(width, commands, c_spacing = (width - 32) / commands.size)
# Compute window height from command quantity
super(0, 0, width, 64)
@commands = commands
@item_max = commands.size
@column_max = @item_max
@c_spacing = c_spacing
@alignment = 1
self.contents = Bitmap.new(@item_max * @c_spacing, height - 32)
refresh
self.index = 0
end
#--------------------------------------------------------------------------
# * Command
#--------------------------------------------------------------------------
def command(index = self.index)
return @commands[index]
end
#--------------------------------------------------------------------------
# * Commands
#--------------------------------------------------------------------------
def commands=(commands)
# Return if Commands Are Same
return if @commands == commands
# Reset Commands
@commands = commands
# Resets Item Max
item_max = @item_max
@item_max = @commands.size
@column_max = @item_max
# If Item Max Changes
unless item_max == @item_max
# Deletes Existing Contents (If Exist)
unless self.contents.nil?
self.contents.dispose
self.contents = nil
end
# Recreates Contents
self.contents = Bitmap.new(@item_max * @c_spacing, height - 32)
end
# Refresh Window
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
for i in 0...@item_max
draw_item(i, normal_color)
end
end
#--------------------------------------------------------------------------
# * Draw Item
#--------------------------------------------------------------------------
def draw_item(index, color)
command = commands[index]
x = index * @c_spacing + 4
self.contents.font.color = color
self.contents.draw_text(x, 0, @c_spacing - 8, 32, command, @alignment)
end
#--------------------------------------------------------------------------
# * Disable Item
#--------------------------------------------------------------------------
def disable_item(index)
draw_item(index, disabled_color)
end
#--------------------------------------------------------------------------
# * Cursor Rectangle Update
#--------------------------------------------------------------------------
def update_cursor_rect
if @index < 0
self.cursor_rect.empty
else
self.cursor_rect.set(@c_spacing * @index, 0, @c_spacing, 32)
end
end
end
class Window_Item
alias_method :seph_keyitemssimple_wnitm_init, :initialize
def initialize
seph_keyitemssimple_wnitm_init
if $scene.is_a?(Scene_Item)
self.y += 64
self.height -= 64
self.active = false
end
end
#--------------------------------------------------------------------------
# * Filter Element Set
#--------------------------------------------------------------------------
def filter_element_set(element_tag = 17, call_refresh = false)
refresh if call_refresh
redata = []
@data.each do |item|
if item.is_a?(RPG::Item) || item.is_a?(RPG::Weapon)
redata << item if item.element_set.include?(element_tag)
elsif item.is_a?(RPG::Armor)
redata << item if item.guard_element_set.include?(element_tag)
end
end
@data = redata
# If item count is not 0, make a bit map and draw all items
@item_max = @data.size
if @item_max > 0
self.contents = Bitmap.new(width - 32, row_max * 32)
for i in 0...@item_max
draw_item(i)
end
end
end
#--------------------------------------------------------------------------
# * Reverse Filter Element Set
#--------------------------------------------------------------------------
def reverse_filter_element_set(element_tag = 17, call_refresh = false)
refresh if call_refresh
redata = []
@data.each do |item|
if item.is_a?(RPG::Item) || item.is_a?(RPG::Weapon)
redata << item unless item.element_set.include?(element_tag)
elsif item.is_a?(RPG::Armor)
redata << item unless item.guard_element_set.include?(element_tag)
end
end
@data = redata
# If item count is not 0, make a bit map and draw all items
@item_max = @data.size
if @item_max > 0
self.contents = Bitmap.new(width - 32, row_max * 32)
for i in 0...@item_max
draw_item(i)
end
end
end
end
class Scene_Item
alias_method :seph_keyitemssimple_scnitm_main, :main
alias_method :seph_keyitemssimple_scnitm_update, :update
def main
@item_kind_select = Window_HorizCommand.new(640, ['Items', 'Key Items'])
@item_kind_select.y = 64
seph_keyitemssimple_scnitm_main
@item_kind_select.dispose
end
def update
if @item_kind_select.active
@item_kind_select.update
if Input.trigger?(Input::B)
$game_system.se_play($data_system.cancel_se)
$scene = Scene_Menu.new
return
end
if Input.trigger?(Input::C)
$game_system.se_play($data_system.decision_se)
@item_kind_select.active = false
@item_window.active = true
end
if Input.repeat?(Input::RIGHT) || Input.repeat?(Input::LEFT)
if @item_kind_select.index == 0
@item_window.reverse_filter_element_set(17, true)
else
@item_window.filter_element_set(17, true)
end
end
return
end
seph_keyitemssimple_scnitm_update
end
end