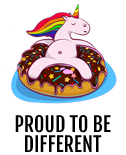
class Scene_A
def main
...
Graphics.transition(100)
loop do
...
end
Graphics.freeze
...
end
...
end
class Scene_B
def main
...
Graphics.transition(100)
loop do
...
end
Graphics.freeze
...
end
...
end
class Scene_A
...
Graphics.transition(1000)
...
end
class Scene_B
...
Graphics.transition(2)
...
end
#==============================================================================
# ** Scene_RubyLogo
#------------------------------------------------------------------------------
# This class makes a Ruby splash screen.
#==============================================================================
class Scene_RubyIntro
#--------------------------------------------------------------------------
# * Main Processing
#--------------------------------------------------------------------------
def main
# If battle test
if $BTEST
battle_test
return
end
# Load database
$data_actors = load_data("Data/Actors.rxdata")
$data_classes = load_data("Data/Classes.rxdata")
$data_skills = load_data("Data/Skills.rxdata")
$data_items = load_data("Data/Items.rxdata")
$data_weapons = load_data("Data/Weapons.rxdata")
$data_armors = load_data("Data/Armors.rxdata")
$data_enemies = load_data("Data/Enemies.rxdata")
$data_troops = load_data("Data/Troops.rxdata")
$data_states = load_data("Data/States.rxdata")
$data_animations = load_data("Data/Animations.rxdata")
$data_tilesets = load_data("Data/Tilesets.rxdata")
$data_common_events = load_data("Data/CommonEvents.rxdata")
$data_system = load_data("Data/System.rxdata")
# Make system object
$game_system = Game_System.new
# Make title graphic
@sprite = Sprite.new
@sprite.bitmap = RPG::Cache.picture("Ruby Logo")
# If enabled, make @continue_enabled true; if disabled, make it false
@continue_enabled = false
for i in 0..3
if FileTest.exist?("Save#{i+1}.rxdata")
@continue_enabled = true
end
end
# Play title BGM
$game_system.bgm_play($data_system.title_bgm)
# Stop playing ME and BGS
Audio.me_stop
Audio.bgs_stop
# Execute transition
Graphics.transition(100)
# Main loop
loop do
# Update game screen
Graphics.update
# Update input information
Input.update
# Frame update
update
# Abort loop if screen is changed
if $scene != self
break
end
end
# Prepare for transition
Graphics.freeze
# Dispose of command window
# Dispose of title graphic
@sprite.bitmap.dispose
@sprite.dispose
end
#--------------------------------------------------------------------------
# * Frame Update
#--------------------------------------------------------------------------
def update
# Update command window
# If C button was pressed
if Input.trigger?(Input::C)
# Branch by command window cursor position
Graphics.transition(100)
$scene = Scene_Title.new
end
if Input.trigger?(Input::B)
# Branch by command window cursor position
Graphics.transition(100)
$scene = Scene_Title.new
end
end
def battle_test
# Load database (for battle test)
$data_actors = load_data("Data/BT_Actors.rxdata")
$data_classes = load_data("Data/BT_Classes.rxdata")
$data_skills = load_data("Data/BT_Skills.rxdata")
$data_items = load_data("Data/BT_Items.rxdata")
$data_weapons = load_data("Data/BT_Weapons.rxdata")
$data_armors = load_data("Data/BT_Armors.rxdata")
$data_enemies = load_data("Data/BT_Enemies.rxdata")
$data_troops = load_data("Data/BT_Troops.rxdata")
$data_states = load_data("Data/BT_States.rxdata")
$data_animations = load_data("Data/BT_Animations.rxdata")
$data_tilesets = load_data("Data/BT_Tilesets.rxdata")
$data_common_events = load_data("Data/BT_CommonEvents.rxdata")
$data_system = load_data("Data/BT_System.rxdata")
# Reset frame count for measuring play time
Graphics.frame_count = 0
# Make each game object
$game_temp = Game_Temp.new
$game_system = Game_System.new
$game_switches = Game_Switches.new
$game_variables = Game_Variables.new
$game_self_switches = Game_SelfSwitches.new
$game_screen = Game_Screen.new
$game_actors = Game_Actors.new
$game_party = Game_Party.new
$game_troop = Game_Troop.new
$game_map = Game_Map.new
$game_player = Game_Player.new
# Set up party for battle test
$game_party.setup_battle_test_members
# Set troop ID, can escape flag, and battleback
$game_temp.battle_troop_id = $data_system.test_troop_id
$game_temp.battle_can_escape = true
$game_map.battleback_name = $data_system.battleback_name
# Play battle start SE
$game_system.se_play($data_system.battle_start_se)
# Play battle BGM
$game_system.bgm_play($game_system.battle_bgm)
# Switch to battle screen
$scene = Scene_Battle.new
end
end
@sprite = Sprite.new
@sprite.bitmap = RPG::Cache.picture("Ruby Logo")
[highlight] # If enabled, make @continue_enabled true; if disabled, make it false
@continue_enabled = false
for i in 0..3
if FileTest.exist?("Save#{i+1}.rxdata")
@continue_enabled = true
end
end[/highlight]
# Play title BGM
$game_system.bgm_play($data_system.title_bgm)
begin
Graphics.freeze
[highlight] $scene = Scene_RubyIntro.new
while $scene != nil
$scene.main
end
Graphics.transition(100)
Graphics.freeze[/highlight]
$scene = Scene_Title.new
while $scene != nil
$scene.main
end
Graphics.transition(20)
rescue Errno::ENOENT
filename = $!.message.sub("No such file or directory - ", "")
print("File #{filename} was not found.")
end