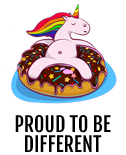
#===============================================================================
# ** Shop : Do Not Sell Certain Goods
#===============================================================================
#-------------------------------------------------------------------------------
# * SDK Log
#-------------------------------------------------------------------------------
if Object.const_defined?(:SDK)
SDK.log('Shop.DoNotSellCertainGoods', , 'Kain Nobel ©', 3.5, '2009.06.17')
end
#===============================================================================
# ** Game_Temp
#===============================================================================
class Game_Temp
#-----------------------------------------------------------------------------
# * Place IDs of permanently unsellable Items here
#-----------------------------------------------------------------------------
Unsellable_Items = [1]
#-----------------------------------------------------------------------------
# * Place IDs of permanently unsellable Armors here
#-----------------------------------------------------------------------------
Unsellable_Armors = [1]
#-----------------------------------------------------------------------------
# * Place IDs of permanently unsellable Weapons here
#-----------------------------------------------------------------------------
Unsellable_Weapons = [1]
#-----------------------------------------------------------------------------
# * Public Instance Variables
#-----------------------------------------------------------------------------
attr_accessor :nosell_items
attr_accessor :nosell_armors
attr_accessor :nosell_weapons
attr_accessor :cant_sell_items
attr_accessor :cant_sell_armors
attr_accessor :cant_sell_weapons
#-----------------------------------------------------------------------------
# * Alias Listings
#-----------------------------------------------------------------------------
alias_method :nosell_gmtemp_initialize, :initialize
#-----------------------------------------------------------------------------
# * Object Initialization
#-----------------------------------------------------------------------------
def initialize
nosell_gmtemp_initialize
reset_unsellables
end
#-----------------------------------------------------------------------------
# * Reset Unsellables
#-----------------------------------------------------------------------------
def reset_unsellables
@nosell_items = Unsellable_Items.dup
@nosell_armors = Unsellable_Armors.dup
@nosell_weapons = Unsellable_Weapons.dup
@cant_sell_items = false
@cant_sell_armors = false
@cant_sell_weapons = false
end
end
#===============================================================================
# ** Game_Party
#===============================================================================
class Game_Party
#-----------------------------------------------------------------------------
# * Public Instance Variables
#-----------------------------------------------------------------------------
attr_reader :show_unsellables
#-----------------------------------------------------------------------------
# * Show Unsellables = (boolean)
#-----------------------------------------------------------------------------
def show_unsellables=(boolean)
@show_unsellables = (boolean == true)
case boolean
when true then reset_unsellables(false)
when false then store_unsellables
end
end
#-----------------------------------------------------------------------------
# * Store Unsellables
#-----------------------------------------------------------------------------
def store_unsellables
@stored_i = Hash.new
@stored_a = Hash.new
@stored_w = Hash.new
@items.each do |id, value|
unless $game_temp.cant_sell_items
next unless $game_temp.nosell_items.include?(id)
end
@stored_i[id] = $data_items[id].price
$data_items[id].price = 0
end
@armors.each do |id, value|
unless $game_temp.cant_sell_armors
next unless $game_temp.nosell_armors.include?(id)
end
@stored_a[id] = $data_armors[id].price
$data_armors[id].price = 0
end
@weapons.each do |id, value|
unless $game_temp.cant_sell_weapons
next unless $game_temp.nosell_weapons.include?(id)
end
@stored_w[id] = $data_weapons[id].price
$data_weapons[id].price = 0
end
end
#-----------------------------------------------------------------------------
# * Reset Unsellables
#-----------------------------------------------------------------------------
def reset_unsellables(reset_temp = false)
$game_temp.reset_unsellables if reset_temp
@stored_i.each {|id, value| $data_items[id].price = value}
@stored_a.each {|id, value| $data_armors[id].price = value}
@stored_w.each {|id, value| $data_weapons[id].price = value}
@stored_i = @stored_a = @stored_w = nil
end
end
#===============================================================================
# ** Interpreter
#===============================================================================
class Interpreter
#-----------------------------------------------------------------------------
# * Can't Sell Items
#-----------------------------------------------------------------------------
def cant_sell_items
$game_temp.cant_sell_items = true
end
#-----------------------------------------------------------------------------
# * Can't Sell Armors
#-----------------------------------------------------------------------------
def cant_sell_armors
$game_temp.cant_sell_armors = true
end
#-----------------------------------------------------------------------------
# * Can't Sell Weapons
#-----------------------------------------------------------------------------
def cant_sell_weapons
$game_temp.cant_sell_weapons = true
end
#-----------------------------------------------------------------------------
# * No Sell Items
#-----------------------------------------------------------------------------
def nsI(*item_ids)
item_ids.each do |id|
next if $game_temp.nosell_items.include?(id)
$game_temp.nosell_items << id
end
end
#-----------------------------------------------------------------------------
# * No Sell Armors
#-----------------------------------------------------------------------------
def nsA(*armor_ids)
armor_ids.each do |id|
next if $game_temp.nosell_armors.include?(id)
$game_temp.nosell_armors << id
end
end
#-----------------------------------------------------------------------------
# * No Sell Weapons
#-----------------------------------------------------------------------------
def nsW(*weapon_ids)
weapon_ids.each do |id|
next if $game_temp.nosell_weapons.include?(id)
$game_temp.nosell_weapons << id
end
end
end
#===============================================================================
# ** Scene_Shop
#===============================================================================
class Scene_Shop
#-----------------------------------------------------------------------------
# * Alias Listings
#-----------------------------------------------------------------------------
alias_method :nosell_snshop_main, :main
alias_method :nosell_snshop_updatebuy, :update_buy
alias_method :nosell_snshop_updatesell, :update_sell
#-----------------------------------------------------------------------------
# * Main
#-----------------------------------------------------------------------------
def main
$game_party.store_unsellables
nosell_snshop_main
$game_party.reset_unsellables(true)
end
#-----------------------------------------------------------------------------
# * Update Buy
#-----------------------------------------------------------------------------
def update_buy
unless $game_party.show_unsellables
$game_party.show_unsellables = true
@buy_window.refresh
return
end
nosell_snshop_updatebuy
end
#-----------------------------------------------------------------------------
# * Update Sell
#-----------------------------------------------------------------------------
def update_sell
if $game_party.show_unsellables
$game_party.show_unsellables = false
@sell_window.refresh
return
end
nosell_snshop_updatesell
end
end
$game_temp.nosell_items += [1, 2, 3, 4, 5, ...]
$game_temp.nosell_armors += [1, 2, 3, 4, 5, ...]
$game_temp.nosell_weapons += [1, 2, 3, 4, 5, ...]
# nsI : Stands for "No Sell Items"
nsI(1, 2, 3, 4, 5, ...)
# nsA : Stands for "No Sell Armors"
nsA(1, 2, 3, 4, 5, ...)
# nsW : Stands for "No Sell Weapons"
nsW(1, 2, 3, 4, 5, ...)
cant_sell_items
cant_sell_armors
cant_sell_weapons