Hiyas again. Currently my brain is dieing on me and I need some help. Ive been working on a script to give my game character one extra stat(like str) and be able to edit it in game. So far though I have the extra stat added with your guys help and im now trying to make the system in debug to edit it. So far though what has complicated matters is I now basically have two copies of the data base. The one that rpgmaker xp's editor uses and my personal one that has my extra stat. Which leaves me to have to figure out how to have it so when the first one is edited I can save the changes to the second file and still have any changes I made to my extra stat untouched. This having two data base files to deal with is the pits. I really wish I could just work with the original to do all this. Ive looked at Sephs virtual Database script but it just doesn't seam to work the way I would hope. Does anyone have any ideas for me?
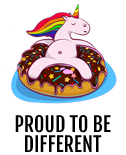