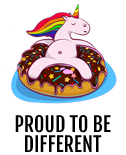
#=============================================================
# 1-Scene Custom Menu System
#=============================================================
# LegACy
# Version 1.13
# 7.09.06
#=============================================================
# This script is a further development of Hydrolic's CMS
# request. I enhance it toward every aspect of a menu system
# so now it all operates in one scene full of animation.
# There's an animated sprite and element wheel features.
# There's also different category for items implemented.
# Now there's enhanced equipment features as well as faceset
# features. Don't forget the icon command feature, too!
#
# To put items into different catagory, simply apply
# attributes to them, you can apply more than 1 attributes
# to each item. In default, the attributes are :
# :: 17 > Recovery items
# :: 18 > Weaponry
# :: 19 > Armor
# :: 20 > Accessories
# :: 21 > Key Items
# :: 22 > Miscellanous Items
#
# For attributes customization, look at lines 340 - 345.
# For animated sprite, turn @animate to true value.
# For face graphic instead of charset, turn @potrait true
# at lines 461 & 1259, then make sure each character has a
# potrait. For Aluxes, the picture will be named 'Potrait_1'.
# If you want the potrait to be class-based however, turn
# @class value to true, under @potrait. The picture will be
# named 'Class_1' for every actor with fighter (ID 1) class.
# Don't forget to put the icons I provided in the icon folder.
#
# Special thanks to Hydrolic for the idea, Diego for the
# element wheel, and SephirotSpawn for sprite animation.
#=============================================================
#==============================================================================
# ** Bitmap
#==============================================================================
class Bitmap
def draw_line(start_x, start_y, end_x, end_y, start_color, width = 1, end_color = start_color)
distance = (start_x - end_x).abs + (start_y - end_y).abs
if end_color == start_color
for i in 1..distance
x = (start_x + 1.0 * (end_x - start_x) * i / distance).to_i
y = (start_y + 1.0 * (end_y - start_y) * i / distance).to_i
if width == 1
self.set_pixel(x, y, start_color)
else
self.fill_rect(x, y, width, width, start_color)
end
end
else
for i in 1..distance
x = (start_x + 1.0 * (end_x - start_x) * i / distance).to_i
y = (start_y + 1.0 * (end_y - start_y) * i / distance).to_i
r = start_color.red * (distance-i)/distance + end_color.red * i/distance
g = start_color.green * (distance-i)/distance + end_color.green * i/distance
b = start_color.blue * (distance-i)/distance + end_color.blue * i/distance
a = start_color.alpha * (distance-i)/distance + end_color.alpha * i/distance
if width == 1
self.set_pixel(x, y, Color.new(r, g, b, a))
else
self.fill_rect(x, y, width, width, Color.new(r, g, b, a))
end
end
end
end
end
#==============================================================================
# ** Game_Actor
#------------------------------------------------------------------------------
# This class handles the actor. It's used within the Game_Actors class
# ($game_actors) and refers to the Game_Party class ($game_party).
#==============================================================================
class Game_Actor < Game_Battler
#--------------------------------------------------------------------------
# * Get Current Experience Points
#--------------------------------------------------------------------------
def now_exp
return @exp - @exp_list[@level]
end
#--------------------------------------------------------------------------
# * Get Needed Experience Points
#--------------------------------------------------------------------------
def next_exp
return @exp_list[@level+1] > 0 ? @exp_list[@level+1] - @exp_list[@level] : 0
end
end
#==============================================================================
# ** Game_Map
#------------------------------------------------------------------------------
# This class handles the map. It includes scrolling and passable determining
# functions. Refer to "$game_map" for the instance of this class.
#==============================================================================
class Game_Map
#--------------------------------------------------------------------------
# * Get Map Name
#--------------------------------------------------------------------------
def name
load_data("Data/MapInfos.rxdata")[@map_id].name
end
end
#==============================================================================
# ** Window_Base
#------------------------------------------------------------------------------
# This class is for all in-game windows.
#==============================================================================
class Window_Base < Window
FONT_SIZE = 16
NUMBER_OF_ELEMENTS = 8
ELEMENT_ORDER = [1,2,3,4,5,6, 7, 8]
GRAPH_SCALINE_COLOR = Color.new(255, 255, 255, 128)
GRAPH_SCALINE_COLOR_SHADOW = Color.new( 0, 0, 0, 192)
GRAPH_LINE_COLOR = Color.new(255, 255, 64, 255)
GRAPH_LINE_COLOR_MINUS = Color.new( 64, 255, 255, 255)
GRAPH_LINE_COLOR_PLUS = Color.new(255, 64, 64, 255)
def draw_actor_element_radar_graph(actor, x, y, radius = 43)
cx = x + radius + FONT_SIZE + 48
cy = y + radius + FONT_SIZE + 32
for loop_i in 0..NUMBER_OF_ELEMENTS
if loop_i == 0
else
@pre_x = @now_x
@pre_y = @now_y
@pre_ex = @now_ex
@pre_ey = @now_ey
@color1 = @color2
end
if loop_i == NUMBER_OF_ELEMENTS
eo = ELEMENT_ORDER[0]
else
eo = ELEMENT_ORDER[loop_i]
end
er = actor.element_rate(eo)
estr = $data_system.elements[eo]
@color2 = er < 0 ? GRAPH_LINE_COLOR_MINUS : er > 100 ? GRAPH_LINE_COLOR_PLUS : GRAPH_LINE_COLOR
er = er.abs
th = Math::PI * (0.5 - 2.0 * loop_i / NUMBER_OF_ELEMENTS)
@now_x = cx + (radius * Math.cos(th)).floor
@now_y = cy - (radius * Math.sin(th)).floor
@now_wx = cx - 6 + ((radius + FONT_SIZE * 3 / 2) * Math.cos(th)).floor - FONT_SIZE
@now_wy = cy - ((radius + FONT_SIZE * 1 / 2) * Math.sin(th)).floor - FONT_SIZE/2
@now_vx = cx + ((radius + FONT_SIZE * 8 / 2) * Math.cos(th)).floor - FONT_SIZE
@now_vy = cy - ((radius + FONT_SIZE * 3 / 2) * Math.sin(th)).floor - FONT_SIZE/2
@now_ex = cx + (er*radius/100 * Math.cos(th)).floor
@now_ey = cy - (er*radius/100 * Math.sin(th)).floor
if loop_i == 0
@pre_x = @now_x
@pre_y = @now_y
@pre_ex = @now_ex
@pre_ey = @now_ey
@color1 = @color2
else
end
next if loop_i == 0
self.contents.draw_line(cx+1,cy+1, @now_x+1,@now_y+1, GRAPH_SCALINE_COLOR_SHADOW)
self.contents.draw_line(@pre_x+1,@pre_y+1, @now_x+1,@now_y+1, GRAPH_SCALINE_COLOR_SHADOW)
self.contents.draw_line(cx,cy, @now_x,@now_y, GRAPH_SCALINE_COLOR)
self.contents.draw_line(@pre_x,@pre_y, @now_x,@now_y, GRAPH_SCALINE_COLOR)
self.contents.draw_line(@pre_ex,@pre_ey, @now_ex,@now_ey, @color1, 2, @color2)
self.contents.font.color = system_color
self.contents.draw_text(@now_wx,@now_wy, FONT_SIZE*3.1, FONT_SIZE, estr, 1)
self.contents.font.color = Color.new(255,255,255,128)
self.contents.draw_text(@now_vx,@now_vy, FONT_SIZE*2, FONT_SIZE, er.to_s + "%", 2)
self.contents.font.color = normal_color
end
end
#--------------------------------------------------------------------------
# Draw Stat Bar
# actor : actor
# x : bar x-coordinate
# y : bar y-coordinate
# stat : stat to be displayed
#--------------------------------------------------------------------------
def draw_bar(actor, x, y, stat, width = 156, height = 7)
bar_color = Color.new(0, 0, 0, 255)
end_color = Color.new(255, 0, 0, 255)
max = 10
case stat
when "hp"
bar_color = Color.new(150, 0, 0, 255)
end_color = Color.new(255, 255, 60, 255)
min = actor.hp
max = actor.maxhp
when "sp"
bar_color = Color.new(0, 0, 155, 255)
end_color = Color.new(255, 255, 255, 255)
min = actor.sp
max = actor.maxsp
when "exp"
bar_color = Color.new(0, 155, 0, 255)
end_color = Color.new(255, 255, 255, 255)
unless actor.level == $data_actors[actor.id].final_level
min = actor.now_exp
max = actor.next_exp
else
min = 1
max = 1
end
end
# Draw Border
for i in 0..height
self.contents.fill_rect(x + i, y + height - i, width + 1, 1,
Color.new(50, 50, 50, 255))
end
# Draw Background
for i in 1..(height - 1)
r = 100 * (height - i) / height + 0 * i / height
g = 100 * (height - i) / height + 0 * i / height
b = 100 * (height - i) / height + 0 * i / height
a = 255 * (height - i) / height + 255 * i / height
self.contents.fill_rect(x + i, y + height - i, width, 1,
Color.new(r, b, g, a))
end
# Draws Bar
for i in 1..( (min.to_f / max.to_f) * width - 1)
for j in 1..(height - 1)
r = bar_color.red * (width - i) / width + end_color.red * i / width
g = bar_color.green * (width - i) / width + end_color.green * i / width
b = bar_color.blue * (width - i) / width + end_color.blue * i / width
a = bar_color.alpha * (width - i) / width + end_color.alpha * i / width
self.contents.fill_rect(x + i + j, y + height - j, 1, 1,
Color.new(r, g, b, a))
end
end
case stat
when "hp"
draw_actor_hp(actor, x - 1, y - 18)
when "sp"
draw_actor_sp(actor, x - 1, y - 18)
when "exp"
draw_actor_exp(actor, x - 1, y - 18)
end
end
#--------------------------------------------------------------------------
# * Draw Sprite
#--------------------------------------------------------------------------
def draw_sprite(x, y, name, hue, frame)
bitmap = RPG::Cache.character(name, hue)
cw = bitmap.width / 4
ch = bitmap.height / 4
# Current Animation Slide
case frame
when 0 ;b = 0
when 1 ;b = cw
when 2 ;b = cw * 2
when 3 ;b = cw * 3
end
# Bitmap Rectange
src_rect = Rect.new(b, 0, cw, ch)
# Draws Bitmap
self.contents.blt(x - cw / 2, y - ch, bitmap, src_rect)
end
#--------------------------------------------------------------------------
# * Get Upgrade Text Color
#--------------------------------------------------------------------------
def up_color
return Color.new(74, 210, 74)
end
#--------------------------------------------------------------------------
# * Get Downgrade Text Color
#--------------------------------------------------------------------------
def down_color
return Color.new(170, 170, 170)
end
#--------------------------------------------------------------------------
# * Draw Potrait
# actor : actor
# x : draw spot x-coordinate
# y : draw spot y-coordinate
#--------------------------------------------------------------------------
def draw_actor_potrait(actor, x, y, classpotrait = false, width = 96, height = 96)
classpotrait ? bitmap = RPG::Cache.picture('Class_' + actor.class_id.to_s) :
bitmap = RPG::Cache.picture('Potrait_' + actor.id.to_s)
src_rect = Rect.new(0, 0, width, height)
self.contents.blt(x, y, bitmap, src_rect)
end
#--------------------------------------------------------------------------
# * Draw parameter
# actor : actor
# x : draw spot x-coordinate
# y : draw spot y-coordinate
# type : parameter type
#------------------------------------------------------------------------
def draw_actor_parameter(actor, x, y, type, width = 120)
case type
when 0
parameter_name = $data_system.words.atk
parameter_value = actor.atk
when 1
parameter_name = $data_system.words.pdef
parameter_value = actor.pdef
when 2
parameter_name = $data_system.words.mdef
parameter_value = actor.mdef
when 3
parameter_name = $data_system.words.str
parameter_value = actor.str
when 4
parameter_name = $data_system.words.dex
parameter_value = actor.dex
when 5
parameter_name = $data_system.words.agi
parameter_value = actor.agi
when 6
parameter_name = $data_system.words.int
parameter_value = actor.int
when 7
parameter_name = "Evasion"
parameter_value = actor.eva
end
self.contents.font.color = system_color
self.contents.draw_text(x, y, 120, 32, parameter_name)
self.contents.font.color = normal_color
self.contents.draw_text(x + width, y, 36, 32, parameter_value.to_s, 2)
end
end
#==============================================================================
# ** Scene_Title
#------------------------------------------------------------------------------
# This class performs title screen processing.
#==============================================================================
class Scene_Title
#--------------------------------------------------------------------------
# * Alias Listings
#--------------------------------------------------------------------------
alias legacy_CMS_scenetitle_main main
#--------------------------------------------------------------------------
# * Main Processing
#--------------------------------------------------------------------------
def main
$recov = 17
$weapon = 18
$armor = 19
$accessory = 20
$key = 21
$misc = 22
legacy_CMS_scenetitle_main
end
end
#==============================================================================
# ** Window_NewCommand
#------------------------------------------------------------------------------
# This window deals with general command choices.
#==============================================================================
class Window_NewCommand < Window_Selectable
#--------------------------------------------------------------------------
# * Object Initialization
# width : window width
# commands : command text string array
#--------------------------------------------------------------------------
def initialize(width, commands, icon = 'none')
# Compute window height from command quantity
super(0, 0, width, commands.size / 3 * 32 + 32)
@item_max = commands.size
@commands = commands
@icon = icon
@column_max = 3
self.contents = Bitmap.new(width - 32, @item_max/3 * 32)
refresh
self.index = 0
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
for i in 0...@item_max
draw_item(i, normal_color)
end
end
#--------------------------------------------------------------------------
# * Draw Item
# index : item number
# color : text color
#--------------------------------------------------------------------------
def draw_item(index, color)
self.contents.font.color = color
rect = Rect.new((109 * (index / 2)), 32 * (index % 2), self.width / @column_max - 16, 32)
self.contents.fill_rect(rect, Color.new(0, 0, 0, 0))
unless @icon == 'none'
bitmap = RPG::Cache.icon(@icon + index.to_s)
self.contents.blt((106 * (index / 2)), 32 * (index % 2) + 4, bitmap, Rect.new(0, 0, 24, 24))
end
self.contents.draw_text(rect, @commands[index])
end
#--------------------------------------------------------------------------
# * Disable Item
# index : item number
#--------------------------------------------------------------------------
def disable_item(index)
draw_item(index, disabled_color)
end
end
#==============================================================================
# ** Window_NewMenuStatus
#------------------------------------------------------------------------------
# This window displays party member status on the menu screen.
#==============================================================================
class Window_NewMenuStatus < Window_Base
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
$game_party.actors.size != 4 ? i = 14 : i = 0
$game_party.actors.size == 1 ? i = 24 : i = i
super(0, 0, 480, ($game_party.actors.size * 84) + i)
self.contents = Bitmap.new(width - 32, height - 32)
refresh
self.active = false
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
for i in 0...$game_party.actors.size
x = 4
y = (i * 76) - 6
actor = $game_party.actors[i]
self.contents.font.size = 19
self.contents.font.bold = true
draw_actor_class(actor, x, y - 1)
draw_actor_state(actor, x + 160, y - 1)
self.contents.font.size = 15
draw_actor_parameter(actor, x, y + 14, 0)
draw_actor_parameter(actor, x, y + 29, 1)
draw_actor_parameter(actor, x, y + 44, 2)
draw_actor_parameter(actor, x, y + 59, 3)
draw_actor_parameter(actor, x + 240, y + 14, 4)
draw_actor_parameter(actor, x + 240, y + 29, 5)
draw_actor_parameter(actor, x + 240, y + 44, 6)
draw_bar(actor, x + 240, y + 75, 'exp')
end
end
end
#==============================================================================
# ** Window_Actor
#------------------------------------------------------------------------------
# This window displays party member status on the menu screen.
#==============================================================================
class Window_Actor < Window_Selectable
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
$game_party.actors.size != 4 ? i = 14 : i = 0
$game_party.actors.size == 1 ? i = 24 : i = i
super(0, 0, 160, ($game_party.actors.size * 84) + i)
self.contents = Bitmap.new(width - 32, height - 32)
@frame = 0
@potrait = false
@class = false
refresh
self.active = false
self.index = -1
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
@item_max = $game_party.actors.size
for i in 0...$game_party.actors.size
x = 4
y = (i * 77) - 12
actor = $game_party.actors[i]
self.contents.font.size = 17
self.contents.font.bold = true
@potrait ? draw_actor_potrait(actor, x, y - 1, @class) : draw_sprite(x + 20,
y + 57, actor.character_name, actor.character_hue, @frame)
draw_actor_name(actor, x + 52, y + 6)
draw_actor_level(actor, x + 52, y + 24)
draw_bar(actor, x - 3, y + 60, 'hp', 120)
draw_bar(actor, x - 3, y + 75, 'sp', 120)
end
end
#--------------------------------------------------------------------------
# * Cursor Rectangle Update
#--------------------------------------------------------------------------
def update_cursor_rect
if @index < 0
self.cursor_rect.empty
else
self.cursor_rect.set(-4, (@index * 77) - 2, self.width - 24, 77)
end
end
#--------------------------------------------------------------------------
# Frame Update
#--------------------------------------------------------------------------
def frame_update
@frame == 3 ? @frame = 0 : @frame += 1
refresh
end
end
#==============================================================================
# ** Window_Stat
#------------------------------------------------------------------------------
# This window displays play time on the menu screen.
#==============================================================================
class Window_Stat < Window_Base
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
super(0, 0, 320, 96)
self.contents = Bitmap.new(width - 32, height - 32)
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
self.contents.font.color = system_color
self.contents.font.size = 18
self.contents.font.bold = true
self.contents.draw_text(4, -4, 120, 32, "Play Time")
cx = contents.text_size($data_system.words.gold).width
self.contents.draw_text(4, 18, 120, 32, "Step Count")
self.contents.draw_text(4, 38, cx, 32, $data_system.words.gold, 2)
@total_sec = Graphics.frame_count / Graphics.frame_rate
hour = @total_sec / 60 / 60
min = @total_sec / 60 % 60
sec = @total_sec % 60
text = sprintf("%02d:%02d:%02d", hour, min, sec)
self.contents.font.color = normal_color
self.contents.draw_text(144, -4, 120, 32, text, 2)
self.contents.draw_text(144, 18, 120, 32, $game_party.steps.to_s, 2)
self.contents.draw_text(144, 40, 120, 32, $game_party.gold.to_s, 2)
end
#--------------------------------------------------------------------------
# * Frame Update
#--------------------------------------------------------------------------
def update
super
if Graphics.frame_count / Graphics.frame_rate != @total_sec
refresh
end
end
end
#==============================================================================
# ** Window_Location
#------------------------------------------------------------------------------
# This window displays current map name.
#==============================================================================
class Window_Location < Window_Base
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
super(0, 0, 640, 48)
self.contents = Bitmap.new(width - 32, height - 32)
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
self.contents.font.color = system_color
self.contents.font.bold = true
self.contents.font.size = 20
self.contents.draw_text(4, -4, 120, 24, "Location")
self.contents.font.color = normal_color
self.contents.draw_text(170, -4, 400, 24, $game_map.name.to_s, 2)
end
end
#==============================================================================
# ** Window_NewHelp
#------------------------------------------------------------------------------
# This window shows skill and item explanations along with actor status.
#==============================================================================
class Window_NewHelp < Window_Base
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
super(0, 0, 640, 48)
self.contents = Bitmap.new(width - 32, height - 32)
end
#--------------------------------------------------------------------------
# * Set Text
# text : text string displayed in window
# align : alignment (0..flush left, 1..center, 2..flush right)
#--------------------------------------------------------------------------
def set_text(text, align = 0)
self.contents.font.bold = true
self.contents.font.size = 20
# If at least one part of text and alignment differ from last time
if text != @text or align != @align
# Redraw text
self.contents.clear
self.contents.font.color = normal_color
self.contents.draw_text(4, -4, self.width - 40, 24, text, align)
@text = text
@align = align
@actor = nil
end
self.visible = true
end
end
#==============================================================================
# ** Window_NewItem
#------------------------------------------------------------------------------
# This window displays items in possession on the item and battle screens.
#==============================================================================
class Window_NewItem < Window_Selectable
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
super(0, 96, 480, 336)
@column_max = 2
@attribute = $recov
refresh
self.index = 0
self.active = false
end
#--------------------------------------------------------------------------
# * Get Item
#--------------------------------------------------------------------------
def item
return @data[self.index]
end
#--------------------------------------------------------------------------
# * Updates Window With New Item Type
# attribute : new item type
#--------------------------------------------------------------------------
def update_item(attribute)
@attribute = attribute
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
if self.contents != nil
self.contents.dispose
self.contents = nil
end
@data = []
# Add item
for i in 1...$data_items.size
if $game_party.item_number(i) > 0 and
$data_items[i].element_set.include?(@attribute)
@data.push($data_items[i])
end
end
# Also add weapons and armors
for i in 1...$data_weapons.size
if $game_party.weapon_number(i) > 0 and
$data_weapons[i].element_set.include?(@attribute)
@data.push($data_weapons[i])
end
end
for i in 1...$data_armors.size
if $game_party.armor_number(i) > 0 and
$data_armors[i].guard_element_set.include?(@attribute)
@data.push($data_armors[i])
end
end
# If item count is not 0, make a bit map and draw all items
@item_max = @data.size
if @item_max > 0
self.contents = Bitmap.new(width - 32, row_max * 32)
for i in 0...@item_max
draw_item(i)
end
end
end
#--------------------------------------------------------------------------
# * Draw Item
# index : item number
#--------------------------------------------------------------------------
def draw_item(index)
item = @data[index]
case item
when RPG::Item
number = $game_party.item_number(item.id)
when RPG::Weapon
number = $game_party.weapon_number(item.id)
when RPG::Armor
number = $game_party.armor_number(item.id)
end
if item.is_a?(RPG::Item) and
$game_party.item_can_use?(item.id)
self.contents.font.color = normal_color
else
self.contents.font.color = disabled_color
end
x = 4 + index % 2 * (208 + 32)
y = index / 2 * 32
rect = Rect.new(x, y, self.width / @column_max - 32, 32)
self.contents.fill_rect(rect, Color.new(0, 0, 0, 0))
bitmap = RPG::Cache.icon(item.icon_name)
opacity = self.contents.font.color == normal_color ? 255 : 128
self.contents.blt(x, y + 4, bitmap, Rect.new(0, 0, 24, 24), opacity)
self.contents.draw_text(x + 28, y, 132, 32, item.name, 0)
self.contents.draw_text(x + 160, y, 16, 32, ":", 1)
self.contents.draw_text(x + 176, y, 24, 32, number.to_s, 2)
end
#--------------------------------------------------------------------------
# * Help Text Update
#--------------------------------------------------------------------------
def update_help
@help_window.set_text(self.item == nil ? "" : self.item.description)
end
end
#==============================================================================
# ** Window_NewSkill
#------------------------------------------------------------------------------
# This window displays usable skills on the skill screen.
#==============================================================================
class Window_NewSkill < Window_Selectable
#--------------------------------------------------------------------------
# * Object Initialization
# actor : actor
#--------------------------------------------------------------------------
def initialize(actor)
super(0, 96, 480, 336)
@actor = actor
@column_max = 2
refresh
self.index = 0
self.active = false
end
#--------------------------------------------------------------------------
# * Acquiring Skill
#--------------------------------------------------------------------------
def skill
return @data[self.index]
end
#--------------------------------------------------------------------------
# * Updates Window With New Actor
# actor : new actor
#--------------------------------------------------------------------------
def update_actor(actor)
@actor = actor
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
if self.contents != nil
self.contents.dispose
self.contents = nil
end
@data = []
for i in [email=0...@actor.skills.size]0...@actor.skills.size[/email]
skill = $data_skills[@actor.skills[i]]
if skill != nil
@data.push(skill)
end
end
# If item count is not 0, make a bit map and draw all items
@item_max = @data.size
if @item_max > 0
self.contents = Bitmap.new(width - 32, row_max * 32)
for i in 0...@item_max
draw_item(i)
end
end
end
#--------------------------------------------------------------------------
# * Draw Item
# index : item number
#--------------------------------------------------------------------------
def draw_item(index)
skill = @data[index]
if @actor.skill_can_use?(skill.id)
self.contents.font.color = normal_color
else
self.contents.font.color = disabled_color
end
x = 4 + index % 2 * (208 + 32)
y = index / 2 * 32
rect = Rect.new(x, y, self.width / @column_max - 32, 32)
self.contents.fill_rect(rect, Color.new(0, 0, 0, 0))
bitmap = RPG::Cache.icon(skill.icon_name)
opacity = self.contents.font.color == normal_color ? 255 : 128
self.contents.blt(x, y + 4, bitmap, Rect.new(0, 0, 24, 24), opacity)
self.contents.draw_text(x + 28, y, 124, 32, skill.name, 0)
self.contents.draw_text(x + 152 , y, 48, 32, skill.sp_cost.to_s, 2)
end
#--------------------------------------------------------------------------
# * Help Text Update
#--------------------------------------------------------------------------
def update_help
@help_window.set_text(self.skill == nil ? "" : self.skill.description)
end
end
#==============================================================================
# ** Window_EquipStat
#------------------------------------------------------------------------------
# This window displays actor parameter changes on the equipment screen.
#==============================================================================
class Window_EquipStat < Window_Base
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_accessor :changes
attr_accessor :mode
#--------------------------------------------------------------------------
# * Object Initialization
# actor : actor
#--------------------------------------------------------------------------
def initialize(actor)
super(0, 96, 240, 336)
self.contents = Bitmap.new(width - 32, height - 32)
@actor = actor
@changes = [0, 0, 0, 0, 0, 0, 0, 0]
@mode = 0
@elem_text = ""
@stat_text = ""
refresh
end
#--------------------------------------------------------------------------
# * Updates Window With New Actor
# actor : new actor
#--------------------------------------------------------------------------
def update_actor(actor)
@actor = actor
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
self.contents.font.size = 18
self.contents.font.bold = true
for i in 0..7
draw_actor_parameter(@actor, 16, (i * 20) - 8, i, 96)
end
self.contents.font.color = system_color
oldelem_text = ""
oldstat_text = ""
if @mode == 0
item = $data_weapons[@actor.weapon_id]
if item != nil
more = false
for i in item.element_set
next if i == $recov || i == $weapon || i == $armor ||
i == $accesory || i == $key || i == $misc
oldelem_text += ", " if more
oldelem_text += $data_system.elements[i].to_s
more = true
end
more = false
for i in item.plus_state_set
oldstat_text += ", " if more
oldstat_text += $data_states[i].name
more = true
end
else
oldelem_text = ""
oldstat_text = ""
end
else
item = $data_armors[eval("@actor.armor#{mode}_id")]
if item != nil
more = false
for i in item.guard_element_set
next if i == $recov || i == $weapon || i == $armor ||
i == $accesory || i == $key || i == $misc
oldelem_text += ", " if more
oldelem_text += $data_system.elements[i].to_s
more = true
end
more = false
for i in item.guard_state_set
oldstat_text += ", " if more
oldstat_text += $data_states[i].name
more = true
end
else
oldelem_text = ""
oldstat_text = ""
end
end
if @new_atk != nil
self.contents.font.color = system_color
self.contents.draw_text(152, -8, 32, 32, "»»", 1)
if @changes[0] == 0
self.contents.font.color = normal_color
elsif @changes[0] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, -8, 32, 32, @new_atk.to_s, 2)
end
if @new_pdef != nil
self.contents.font.color = system_color
self.contents.draw_text(152, 12, 32, 32, "»»", 1)
if @changes[1] == 0
self.contents.font.color = normal_color
elsif @changes[1] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, 12, 32, 32, @new_pdef.to_s, 2)
end
if @new_mdef != nil
self.contents.font.color = system_color
self.contents.draw_text(152, 32, 32, 32, "»»", 1)
if @changes[2] == 0
self.contents.font.color = normal_color
elsif @changes[2] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, 32, 32, 32, @new_mdef.to_s, 2)
end
if @new_str != nil
self.contents.font.color = system_color
self.contents.draw_text(152, 52, 32, 32, "»»", 1)
if @changes[3] == 0
self.contents.font.color = normal_color
elsif @changes[3] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, 52, 32, 32, @new_str.to_s, 2)
end
if @new_dex != nil
self.contents.font.color = system_color
self.contents.draw_text(152, 72, 32, 32, "»»", 1)
if @changes[4] == 0
self.contents.font.color = normal_color
elsif @changes[4] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, 72, 32, 32, @new_dex.to_s, 2)
end
if @new_agi != nil
self.contents.font.color = system_color
self.contents.draw_text(152, 92, 32, 32, "»»", 1)
if @changes[5] == 0
self.contents.font.color = normal_color
elsif @changes[5] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, 92, 32, 32, @new_agi.to_s, 2)
end
if @new_int != nil
self.contents.font.color = system_color
self.contents.draw_text(152, 112, 32, 32, "»»", 1)
if @changes[6] == 0
self.contents.font.color = normal_color
elsif @changes[6] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, 112, 32, 32, @new_int.to_s, 2)
end
if @new_eva != nil
self.contents.font.color = system_color
self.contents.draw_text(152, 132, 32, 32, "»»", 1)
if @changes[7] == 0
self.contents.font.color = normal_color
elsif @changes[7] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, 132, 32, 32, @new_eva.to_s, 2)
end
if @mode == 0
unless @actor.weapon_id == 0 or @actor.weapon_id == nil
# If this actor DOES have weapons equipped, sets up data
level = $game_weapons[@actor.weapon_id].weapon_level
rank = $game_weapons[@actor.weapon_id].weapon_class
exp = $game_weapons[@actor.weapon_id].exp
n_exp = $game_weapons[@actor.weapon_id].next_exp
break_amount = $game_weapons[@actor.weapon_id].break_amount
broken = $game_weapons[@actor.weapon_id].weapon_broke? == true ? "Yes" : "No"
# So, we're now going to make the text
self.contents.font.color = system_color
self.contents.draw_text(4, 160, self.width - 32, 32, "Weapon Level")
self.contents.draw_text(4, 180, self.width - 32, 32, "Weapon Rank")
self.contents.draw_text(4, 200, self.width - 32, 32, "EXP")
self.contents.draw_text(4, 220, self.width - 32, 32, "Next Level")
self.contents.draw_text(4, 240, self.width - 32, 32, "Break %")
self.contents.draw_text(4, 260, self.width - 32, 32, "Broken?")
# Text done, the data is now shown in the proper line
self.contents.font.color = normal_color
self.contents.draw_text(129, 160, self.width - 32, 32, level.to_s)
self.contents.draw_text(129, 180, self.width - 32, 32, rank.to_s)
self.contents.draw_text(129, 200, self.width - 32, 32, exp.to_s)
self.contents.draw_text(129, 220, self.width - 32, 32, n_exp.to_s)
self.contents.draw_text(129, 240, self.width - 32, 32, break_amount.to_s)
self.contents.draw_text(129, 260, self.width - 32, 32, broken)
else
# If there are no weapons equipped, show this message
# Otherwise it would crash!
self.contents.font.color = system_color
self.contents.draw_text(4, 258, self.width - 32, 32, "No Weapon!")
end
end
end
#--------------------------------------------------------------------------
# * Set parameters after changing equipment
# new_atk : attack power after changing equipment
# new_pdef : physical defense after changing equipment
# new_mdef : magic defense after changing equipment
# new_str : strength after changing equipment
# new_dex : dexterity after changing equipment
# new_agi : agility after changing equipment
# new_int : inteligence after changing equipment
# new_eva : evasion after changing equipment
#--------------------------------------------------------------------------
def set_new_parameters(new_atk, new_pdef, new_mdef, new_str, new_dex,
new_agi, new_int, new_eva, elem_text, stat_text)
flag = false
if new_atk != @new_atk || new_pdef != @new_pdef || new_str != @new_str ||
new_mdef != @new_mdef || new_dex != @new_dex || new_agi != @new_agi ||
new_eva != @new_eva || elem_text != @elem_text || stat_text != @stat_text
flag = true
end
@new_atk = new_atk
@new_pdef = new_pdef
@new_mdef = new_mdef
@new_str = new_str
@new_dex = new_dex
@new_agi = new_agi
@new_int = new_int
@new_eva = new_eva
@elem_text = elem_text
@stat_text = stat_text
if flag
refresh
end
end
end
#==============================================================================
# ** Window_Equipment
#------------------------------------------------------------------------------
# This window displays items the actor is currently equipped with on the
# equipment screen.
#==============================================================================
class Window_Equipment < Window_Selectable
#--------------------------------------------------------------------------
# * Object Initialization
# actor : actor
#--------------------------------------------------------------------------
def initialize(actor)
super(0, 96, 240, 176)
self.contents = Bitmap.new(width - 32, height - 32)
@actor = actor
refresh
self.index = 0
self.active = false
end
#--------------------------------------------------------------------------
# * Updates Window With New Actor
# actor : new actor
#--------------------------------------------------------------------------
def update_actor(actor)
@actor = actor
refresh
end
#--------------------------------------------------------------------------
# * Item Acquisition
#--------------------------------------------------------------------------
def item
return @data[self.index]
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
@data = []
@data.push($data_weapons[@actor.weapon_id])
@data.push($data_armors[@actor.armor1_id])
@data.push($data_armors[@actor.armor2_id])
@data.push($data_armors[@actor.armor3_id])
@data.push($data_armors[@actor.armor4_id])
@item_max = @data.size
self.contents.font.color = system_color
self.contents.font.size = 19
self.contents.font.bold = true
self.contents.draw_text(0, 28 * 0, 76, 32, $data_system.words.weapon)
self.contents.draw_text(0, 28 * 1, 76, 32, $data_system.words.armor1)
self.contents.draw_text(0, 28 * 2, 76, 32, $data_system.words.armor2)
self.contents.draw_text(0, 28 * 3, 76, 32, $data_system.words.armor3)
self.contents.draw_text(0, 28 * 4, 76, 32, $data_system.words.armor4)
self.contents.font.bold = false
for i in 0..4
draw_item_name(@data[i], 76, 28 * i)
end
end
#--------------------------------------------------------------------------
# * Update Cursor Rectangle
#--------------------------------------------------------------------------
def update_cursor_rect
# Calculate cursor width
cursor_width = self.width / @column_max - 24
# Calculate cursor coordinates
x = @index % @column_max * (cursor_width + 24)
y = @index * 28
# Update cursor rectangle
self.cursor_rect.set(x - 4, y, cursor_width, 32)
end
#--------------------------------------------------------------------------
# * Help Text Update
#--------------------------------------------------------------------------
def update_help
@help_window.set_text(self.item == nil ? "" : self.item.description)
end
end
#==============================================================================
# ** Window_EquipmentItem
#------------------------------------------------------------------------------
# This window displays choices when opting to change equipment on the
# equipment screen.
#==============================================================================
class Window_EquipmentItem < Window_Selectable
#--------------------------------------------------------------------------
# * Object Initialization
# actor : actor
# equip_type : equip region (0-3)
#--------------------------------------------------------------------------
def initialize(actor, equip_type)
super(0, 272, 240, 160)
@actor = actor
@equip_type = equip_type
refresh
self.active = false
self.index = -1
end
#--------------------------------------------------------------------------
# * Updates Window With New Actor
# actor : new actor
#--------------------------------------------------------------------------
def update_actor(actor)
@actor = actor
refresh
end
#--------------------------------------------------------------------------
# * Updates Window With New Equipment Type
# equip_type : new teyp of equipment
#--------------------------------------------------------------------------
def update_equipment(equip_type)
@equip_type = equip_type
refresh
end
#--------------------------------------------------------------------------
# * Item Acquisition
#--------------------------------------------------------------------------
def item
if self.index == 0
return @data[@item_max - 1]
else
return @data[self.index - 1]
end
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
if self.contents != nil
self.contents.dispose
self.contents = nil
end
@data = []
# Add equippable weapons
if @equip_type == 0
weapon_set = $data_classes[@actor.class_id].weapon_set
for i in 1...$data_weapons.size
if $game_party.weapon_number(i) > 0 and weapon_set.include?(i)
@data.push($data_weapons[i])
end
end
end
# Add equippable armor
if @equip_type != 0
armor_set = $data_classes[@actor.class_id].armor_set
for i in 1...$data_armors.size
if $game_party.armor_number(i) > 0 and armor_set.include?(i)
if $data_armors[i].kind == @equip_type-1
@data.push($data_armors[i])
end
end
end
end
# Add blank page
@data.push(nil)
# Make a bit map and draw all items
@item_max = @data.size
self.contents = Bitmap.new(width - 32, row_max * 32)
for i in 0...@item_max - 1
draw_item(i)
end
self.contents.draw_text(4, 0, 204, 32, 'Unequip', 0)
end
#--------------------------------------------------------------------------
# * Draw Item
# index : item number
#--------------------------------------------------------------------------
def draw_item(index)
item = @data[index]
x = 4
y = (index + 1) * 32
case item
when RPG::Weapon
number = $game_party.weapon_number(item.id)
when RPG::Armor
number = $game_party.armor_number(item.id)
end
bitmap = RPG::Cache.icon(item.icon_name)
self.contents.blt(x, y + 4, bitmap, Rect.new(0, 0, 24, 24))
self.contents.font.color = normal_color
self.contents.font.size = 20
self.contents.font.bold = true
self.contents.draw_text(x + 28, y, 132, 32, item.name, 0)
self.contents.draw_text(x + 160, y, 12, 32, ":", 1)
self.contents.draw_text(x + 176, y, 24, 32, number.to_s, 2)
end
#--------------------------------------------------------------------------
# * Help Text Update
#--------------------------------------------------------------------------
def update_help
@help_window.set_text(self.item == nil ? 'Unequip the current equipment.' :
self.item.description)
end
end
#==============================================================================
# ** Window_Status
#------------------------------------------------------------------------------
# This window displays full status specs on the status screen.
#==============================================================================
class Window_NewStatus < Window_Base
def initialize(actor)
super(0, 96, 640, 336)
self.contents = Bitmap.new(width - 32, height - 32)
@actor = actor
@potrait = false
@class = false
refresh
self.active = false
end
#--------------------------------------------------------------------------
# * Updates Window With New Actor
# actor : new actor
#--------------------------------------------------------------------------
def update_actor(actor)
@actor = actor
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
self.contents.font.size = 22
self.contents.font.bold = false
draw_actor_name(@actor, 12, 0)
if @potrait
draw_actor_potrait(@actor, 12, 40, @class)
draw_bar(@actor, 120, 60, 'hp', 200)
draw_bar(@actor, 120, 92, 'sp', 200)
draw_bar(@actor, 120, 124, 'exp', 200)
else
draw_actor_graphic(@actor, 40, 120)
draw_bar(@actor, 96, 60, 'hp', 200)
draw_bar(@actor, 96, 92, 'sp', 200)
draw_bar(@actor, 96, 124, 'exp', 200)
end
draw_actor_class(@actor, 262, 0)
draw_actor_level(@actor, 184, 0)
draw_actor_state(@actor, 96, 0)
self.contents.font.size = 17
self.contents.font.bold = true
for i in 0..7
draw_actor_parameter(@actor, 386, (i * 20) - 6, i)
end
self.contents.font.color = system_color
self.contents.font.size = 16
draw_actor_element_radar_graph(@actor, 48, 136)
self.contents.font.size = 18
self.contents.font.color = system_color
self.contents.draw_text(380, 156, 96, 32, "Equipment")
self.contents.draw_text(300, 180, 96, 32, "Weapon")
self.contents.draw_text(300, 204, 96, 32, "Shield")
self.contents.draw_text(300, 228, 96, 32, "Helmet")
self.contents.draw_text(300, 252, 96, 32, "Armor")
self.contents.draw_text(300, 276, 96, 32, "Accessory")
equip = $data_weapons[@actor.weapon_id]
if @actor.equippable?(equip)
draw_item_name($data_weapons[@actor.weapon_id], 406, 180)
else
self.contents.font.color = knockout_color
self.contents.draw_text(406, 180, 192, 32, "Nothing equipped")
end
equip1 = $data_armors[@actor.armor1_id]
if @actor.equippable?(equip1)
draw_item_name($data_armors[@actor.armor1_id], 406, 204)
else
self.contents.font.color = crisis_color
self.contents.draw_text(406, 204, 192, 32, "Nothing equipped")
end
equip2 = $data_armors[@actor.armor2_id]
if @actor.equippable?(equip2)
draw_item_name($data_armors[@actor.armor2_id], 406, 228)
else
self.contents.font.color = crisis_color
self.contents.draw_text(406, 228, 192, 32, "Nothing equipped")
end
equip3 = $data_armors[@actor.armor3_id]
if @actor.equippable?(equip3)
draw_item_name($data_armors[@actor.armor3_id], 406, 252)
else
self.contents.font.color = crisis_color
self.contents.draw_text(406, 252, 192, 32, "Nothing equipped")
end
equip4 = $data_armors[@actor.armor4_id]
if @actor.equippable?(equip4)
draw_item_name($data_armors[@actor.armor4_id], 406, 276)
else
self.contents.font.color = crisis_color
self.contents.draw_text(406, 276, 192, 32, "Nothing equipped")
end
end
def dummy
self.contents.font.color = system_color
self.contents.draw_text(320, 112, 96, 32, $data_system.words.weapon)
self.contents.draw_text(320, 176, 96, 32, $data_system.words.armor1)
self.contents.draw_text(320, 240, 96, 32, $data_system.words.armor2)
self.contents.draw_text(320, 304, 96, 32, $data_system.words.armor3)
self.contents.draw_text(320, 368, 96, 32, $data_system.words.armor4)
draw_item_name($data_weapons[@actor.weapon_id], 320 + 24, 144)
draw_item_name($data_armors[@actor.armor1_id], 320 + 24, 208)
draw_item_name($data_armors[@actor.armor2_id], 320 + 24, 272)
draw_item_name($data_armors[@actor.armor3_id], 320 + 24, 336)
draw_item_name($data_armors[@actor.armor4_id], 320 + 24, 400)
end
end
#==============================================================================
# ** Scene_Menu
#------------------------------------------------------------------------------
# This class performs menu screen processing.
#==============================================================================
class Scene_Menu
#--------------------------------------------------------------------------
# * Object Initialization
# menu_index : command cursor's initial position
#--------------------------------------------------------------------------
def initialize(menu_index = 0)
@menu_index = menu_index
@update_frame = 0
@animate = false # True if you want the sprite to be animated.
@extraequip = true # True if you want to have optimize and unequip all command.
@targetactive = false
@exit = false
@actor = $game_party.actors[0]
end
#--------------------------------------------------------------------------
# * Main Processing
#--------------------------------------------------------------------------
def main
# Make command window
s1 = ' ' + $data_system.words.item
s2 = ' ' + $data_system.words.skill
s3 = ' ' + $data_system.words.equip
s4 = ' ' + 'Status'
s5 = ' ' + 'Save'
s6 = ' ' + 'Exit'
t1 = ' ' + 'Recov.'
t2 = ' ' + 'Weaponry'
t3 = 'Armor'
t4 = 'Accessory'
t5 = 'Quest'
t6 = 'Misc.'
u1 = 'To Title'
u2 = 'Quit'
v1 = 'Optimize'
v2 = 'Unequip All'
@command_window = Window_NewCommand.new(320, [s1, s2, s3, s4, s5, s6], 'menu')
@command_window.y = -96
@command_window.index = @menu_index
# If number of party members is 0
if $game_party.actors.size == 0
# Disable items, skills, equipment, and status
@command_window.disable_item(0)
@command_window.disable_item(1)
@command_window.disable_item(2)
@command_window.disable_item(3)
end
# If save is forbidden
if $game_system.save_disabled
# Disable save
@command_window.disable_item(4)
end
# Make stat window
@stat_window = Window_Stat.new
@stat_window.x = 320
@stat_window.y = -96
# Make status window
@status_window = Window_NewMenuStatus.new
@status_window.x = 640
@status_window.y = 96
#@status_window.z = 110
@location_window = Window_Location.new
@location_window.y = 480
@actor_window = Window_Actor.new
@actor_window.x = -160
@actor_window.y = 96
@itemcommand_window = Window_NewCommand.new(320, [t1, t2, t3, t4, t5, t6])
@itemcommand_window.x = -320
@itemcommand_window.active = false
@help_window = Window_NewHelp.new
@help_window.x = -640
@help_window.y = 432
@item_window = Window_NewItem.new
@item_window.x = -480
@item_window.help_window = @help_window
@skill_window = Window_NewSkill.new(@actor)
@skill_window.x = -480
@skill_window.help_window = @help_window
@equipstat_window = Window_EquipStat.new(@actor)
@equipstat_window.x = -480
@equip_window = Window_Equipment.new(@actor)
@equip_window.x = -240
@equip_window.help_window = @help_window
@equipitem_window = Window_EquipmentItem.new(@actor, 0)
@equipitem_window.x = -240
@equipitem_window.help_window = @help_window
@equipenhanced_window = Window_Command.new(160, [v1, v2])
@equipenhanced_window.x = -160
@equipenhanced_window.active = false
@playerstatus_window = Window_NewStatus.new(@actor)
@playerstatus_window.x = -640
@end_window = Window_Command.new(120, [u1, u2])
@end_window.x = 640
@end_window.active = false
@spriteset = Spriteset_Map.new
@windows = [@command_window, @stat_window, @status_window,
@location_window, @actor_window, @itemcommand_window,@help_window,
@item_window, @skill_window, @equipstat_window, @equip_window,
@equipitem_window, @equipenhanced_window, @playerstatus_window,
@end_window]
@windows.each {|i| i.opacity = 200}
# Execute transition
Graphics.transition
# Main loop
loop do
# Update game screen
Graphics.update
# Update input information
Input.update
# Frame update
update
# Abort loop if screen is changed
if $scene != self
break
end
end
# Prepare for transition
Graphics.freeze
# Dispose of windows
@spriteset.dispose
@windows.each {|i| i.dispose}
end
#--------------------------------------------------------------------------
# * Frame Update
#--------------------------------------------------------------------------
def update
# Update windows
@windows.each {|i| i.update}
animate
menu_update
if @animate
@update_frame += 1
if @update_frame == 3
@update_frame = 0
@actor_window.frame_update
end
end
end
#--------------------------------------------------------------------------
# * Animating windows.
#--------------------------------------------------------------------------
def animate
if @command_window.active && @skill_window.x == -480
@command_window.y += 6 if @command_window.y < 0
@stat_window.y += 6 if @stat_window.y < 0
@actor_window.x += 10 if @actor_window.x < 0
@status_window.x -= 30 if @status_window.x > 160
@location_window.y -= 3 if @location_window.y > 432
elsif @exit == true
@command_window.y -= 6 if @command_window.y > -96
@stat_window.y -= 6 if @stat_window.y > -96
@actor_window.x -= 10 if @actor_window.x > -160
@status_window.x += 30 if @status_window.x < 640
@location_window.y += 3 if @location_window.y < 480
$scene = Scene_Map.new if @location_window.y == 480
end
if @itemcommand_window.active
if @itemcommand_window.x < 0
@stat_window.x += 40
@command_window.x += 40
@itemcommand_window.x += 40
end
else
if @itemcommand_window.x > -320 && @command_window.active
@stat_window.x -= 40
@command_window.x -= 40
@itemcommand_window.x -= 40
end
end
if @item_window.active
if @item_window.x < 0
@location_window.x += 40
@help_window.x += 40
@status_window.x += 30
@actor_window.x += 30
@item_window.x += 30
end
elsif @targetactive != true
if @item_window.x > -480 && @command_window.index == 0
@help_window.x -= 40
@location_window.x -= 40
@item_window.x -= 30
@actor_window.x -= 30
@status_window.x -= 30
end
end
if @skill_window.active
if @skill_window.x < 0
@location_window.x += 40
@help_window.x += 40
@status_windo
#=============================================================
# 1-Scene Custom Menu System
#=============================================================
# LegACy
# Version 1.13
# 7.09.06
#=============================================================
# This script is a further development of Hydrolic's CMS
# request. I enhance it toward every aspect of a menu system
# so now it all operates in one scene full of animation.
# There's an animated sprite and element wheel features.
# There's also different category for items implemented.
# Now there's enhanced equipment features as well as faceset
# features. Don't forget the icon command feature, too!
#
# To put items into different catagory, simply apply
# attributes to them, you can apply more than 1 attributes
# to each item. In default, the attributes are :
# :: 17 > Recovery items
# :: 18 > Weaponry
# :: 19 > Armor
# :: 20 > Accessories
# :: 21 > Key Items
# :: 22 > Miscellanous Items
#
# For attributes customization, look at lines 340 - 345.
# For animated sprite, turn @animate to true value.
# For face graphic instead of charset, turn @potrait true
# at lines 461 & 1259, then make sure each character has a
# potrait. For Aluxes, the picture will be named 'Potrait_1'.
# If you want the potrait to be class-based however, turn
# @class value to true, under @potrait. The picture will be
# named 'Class_1' for every actor with fighter (ID 1) class.
# Don't forget to put the icons I provided in the icon folder.
#
# Special thanks to Hydrolic for the idea, Diego for the
# element wheel, and SephirotSpawn for sprite animation.
#=============================================================
#==============================================================================
# ** Bitmap
#==============================================================================
class Bitmap
def draw_line(start_x, start_y, end_x, end_y, start_color, width = 1, end_color = start_color)
distance = (start_x - end_x).abs + (start_y - end_y).abs
if end_color == start_color
for i in 1..distance
x = (start_x + 1.0 * (end_x - start_x) * i / distance).to_i
y = (start_y + 1.0 * (end_y - start_y) * i / distance).to_i
if width == 1
self.set_pixel(x, y, start_color)
else
self.fill_rect(x, y, width, width, start_color)
end
end
else
for i in 1..distance
x = (start_x + 1.0 * (end_x - start_x) * i / distance).to_i
y = (start_y + 1.0 * (end_y - start_y) * i / distance).to_i
r = start_color.red * (distance-i)/distance + end_color.red * i/distance
g = start_color.green * (distance-i)/distance + end_color.green * i/distance
b = start_color.blue * (distance-i)/distance + end_color.blue * i/distance
a = start_color.alpha * (distance-i)/distance + end_color.alpha * i/distance
if width == 1
self.set_pixel(x, y, Color.new(r, g, b, a))
else
self.fill_rect(x, y, width, width, Color.new(r, g, b, a))
end
end
end
end
end
#==============================================================================
# ** Game_Actor
#------------------------------------------------------------------------------
# This class handles the actor. It's used within the Game_Actors class
# ($game_actors) and refers to the Game_Party class ($game_party).
#==============================================================================
class Game_Actor < Game_Battler
#--------------------------------------------------------------------------
# * Get Current Experience Points
#--------------------------------------------------------------------------
def now_exp
return @exp - @exp_list[@level]
end
#--------------------------------------------------------------------------
# * Get Needed Experience Points
#--------------------------------------------------------------------------
def next_exp
return @exp_list[@level+1] > 0 ? @exp_list[@level+1] - @exp_list[@level] : 0
end
end
#==============================================================================
# ** Game_Map
#------------------------------------------------------------------------------
# This class handles the map. It includes scrolling and passable determining
# functions. Refer to "$game_map" for the instance of this class.
#==============================================================================
class Game_Map
#--------------------------------------------------------------------------
# * Get Map Name
#--------------------------------------------------------------------------
def name
load_data("Data/MapInfos.rxdata")[@map_id].name
end
end
#==============================================================================
# ** Window_Base
#------------------------------------------------------------------------------
# This class is for all in-game windows.
#==============================================================================
class Window_Base < Window
FONT_SIZE = 16
NUMBER_OF_ELEMENTS = 8
ELEMENT_ORDER = [1,2,3,4,5,6, 7, 8]
GRAPH_SCALINE_COLOR = Color.new(255, 255, 255, 128)
GRAPH_SCALINE_COLOR_SHADOW = Color.new( 0, 0, 0, 192)
GRAPH_LINE_COLOR = Color.new(255, 255, 64, 255)
GRAPH_LINE_COLOR_MINUS = Color.new( 64, 255, 255, 255)
GRAPH_LINE_COLOR_PLUS = Color.new(255, 64, 64, 255)
def draw_actor_element_radar_graph(actor, x, y, radius = 43)
cx = x + radius + FONT_SIZE + 48
cy = y + radius + FONT_SIZE + 32
for loop_i in 0..NUMBER_OF_ELEMENTS
if loop_i == 0
else
@pre_x = @now_x
@pre_y = @now_y
@pre_ex = @now_ex
@pre_ey = @now_ey
@color1 = @color2
end
if loop_i == NUMBER_OF_ELEMENTS
eo = ELEMENT_ORDER[0]
else
eo = ELEMENT_ORDER[loop_i]
end
er = actor.element_rate(eo)
estr = $data_system.elements[eo]
@color2 = er < 0 ? GRAPH_LINE_COLOR_MINUS : er > 100 ? GRAPH_LINE_COLOR_PLUS : GRAPH_LINE_COLOR
er = er.abs
th = Math::PI * (0.5 - 2.0 * loop_i / NUMBER_OF_ELEMENTS)
@now_x = cx + (radius * Math.cos(th)).floor
@now_y = cy - (radius * Math.sin(th)).floor
@now_wx = cx - 6 + ((radius + FONT_SIZE * 3 / 2) * Math.cos(th)).floor - FONT_SIZE
@now_wy = cy - ((radius + FONT_SIZE * 1 / 2) * Math.sin(th)).floor - FONT_SIZE/2
@now_vx = cx + ((radius + FONT_SIZE * 8 / 2) * Math.cos(th)).floor - FONT_SIZE
@now_vy = cy - ((radius + FONT_SIZE * 3 / 2) * Math.sin(th)).floor - FONT_SIZE/2
@now_ex = cx + (er*radius/100 * Math.cos(th)).floor
@now_ey = cy - (er*radius/100 * Math.sin(th)).floor
if loop_i == 0
@pre_x = @now_x
@pre_y = @now_y
@pre_ex = @now_ex
@pre_ey = @now_ey
@color1 = @color2
else
end
next if loop_i == 0
self.contents.draw_line(cx+1,cy+1, @now_x+1,@now_y+1, GRAPH_SCALINE_COLOR_SHADOW)
self.contents.draw_line(@pre_x+1,@pre_y+1, @now_x+1,@now_y+1, GRAPH_SCALINE_COLOR_SHADOW)
self.contents.draw_line(cx,cy, @now_x,@now_y, GRAPH_SCALINE_COLOR)
self.contents.draw_line(@pre_x,@pre_y, @now_x,@now_y, GRAPH_SCALINE_COLOR)
self.contents.draw_line(@pre_ex,@pre_ey, @now_ex,@now_ey, @color1, 2, @color2)
self.contents.font.color = system_color
self.contents.draw_text(@now_wx,@now_wy, FONT_SIZE*3.1, FONT_SIZE, estr, 1)
self.contents.font.color = Color.new(255,255,255,128)
self.contents.draw_text(@now_vx,@now_vy, FONT_SIZE*2, FONT_SIZE, er.to_s + "%", 2)
self.contents.font.color = normal_color
end
end
#--------------------------------------------------------------------------
# Draw Stat Bar
# actor : actor
# x : bar x-coordinate
# y : bar y-coordinate
# stat : stat to be displayed
#--------------------------------------------------------------------------
def draw_bar(actor, x, y, stat, width = 156, height = 7)
bar_color = Color.new(0, 0, 0, 255)
end_color = Color.new(255, 0, 0, 255)
max = 10
case stat
when "hp"
bar_color = Color.new(150, 0, 0, 255)
end_color = Color.new(255, 255, 60, 255)
min = actor.hp
max = actor.maxhp
when "sp"
bar_color = Color.new(0, 0, 155, 255)
end_color = Color.new(255, 255, 255, 255)
min = actor.sp
max = actor.maxsp
when "exp"
bar_color = Color.new(0, 155, 0, 255)
end_color = Color.new(255, 255, 255, 255)
unless actor.level == $data_actors[actor.id].final_level
min = actor.now_exp
max = actor.next_exp
else
min = 1
max = 1
end
end
# Draw Border
for i in 0..height
self.contents.fill_rect(x + i, y + height - i, width + 1, 1,
Color.new(50, 50, 50, 255))
end
# Draw Background
for i in 1..(height - 1)
r = 100 * (height - i) / height + 0 * i / height
g = 100 * (height - i) / height + 0 * i / height
b = 100 * (height - i) / height + 0 * i / height
a = 255 * (height - i) / height + 255 * i / height
self.contents.fill_rect(x + i, y + height - i, width, 1,
Color.new(r, b, g, a))
end
# Draws Bar
for i in 1..( (min.to_f / max.to_f) * width - 1)
for j in 1..(height - 1)
r = bar_color.red * (width - i) / width + end_color.red * i / width
g = bar_color.green * (width - i) / width + end_color.green * i / width
b = bar_color.blue * (width - i) / width + end_color.blue * i / width
a = bar_color.alpha * (width - i) / width + end_color.alpha * i / width
self.contents.fill_rect(x + i + j, y + height - j, 1, 1,
Color.new(r, g, b, a))
end
end
case stat
when "hp"
draw_actor_hp(actor, x - 1, y - 18)
when "sp"
draw_actor_sp(actor, x - 1, y - 18)
when "exp"
draw_actor_exp(actor, x - 1, y - 18)
end
end
#--------------------------------------------------------------------------
# * Draw Sprite
#--------------------------------------------------------------------------
def draw_sprite(x, y, name, hue, frame)
bitmap = RPG::Cache.character(name, hue)
cw = bitmap.width / 4
ch = bitmap.height / 4
# Current Animation Slide
case frame
when 0 ;b = 0
when 1 ;b = cw
when 2 ;b = cw * 2
when 3 ;b = cw * 3
end
# Bitmap Rectange
src_rect = Rect.new(b, 0, cw, ch)
# Draws Bitmap
self.contents.blt(x - cw / 2, y - ch, bitmap, src_rect)
end
#--------------------------------------------------------------------------
# * Get Upgrade Text Color
#--------------------------------------------------------------------------
def up_color
return Color.new(74, 210, 74)
end
#--------------------------------------------------------------------------
# * Get Downgrade Text Color
#--------------------------------------------------------------------------
def down_color
return Color.new(170, 170, 170)
end
#--------------------------------------------------------------------------
# * Draw Potrait
# actor : actor
# x : draw spot x-coordinate
# y : draw spot y-coordinate
#--------------------------------------------------------------------------
def draw_actor_potrait(actor, x, y, classpotrait = false, width = 96, height = 96)
classpotrait ? bitmap = RPG::Cache.picture('Class_' + actor.class_id.to_s) :
bitmap = RPG::Cache.picture('Potrait_' + actor.id.to_s)
src_rect = Rect.new(0, 0, width, height)
self.contents.blt(x, y, bitmap, src_rect)
end
#--------------------------------------------------------------------------
# * Draw parameter
# actor : actor
# x : draw spot x-coordinate
# y : draw spot y-coordinate
# type : parameter type
#------------------------------------------------------------------------
def draw_actor_parameter(actor, x, y, type, width = 120)
case type
when 0
parameter_name = $data_system.words.atk
parameter_value = actor.atk
when 1
parameter_name = $data_system.words.pdef
parameter_value = actor.pdef
when 2
parameter_name = $data_system.words.mdef
parameter_value = actor.mdef
when 3
parameter_name = $data_system.words.str
parameter_value = actor.str
when 4
parameter_name = $data_system.words.dex
parameter_value = actor.dex
when 5
parameter_name = $data_system.words.agi
parameter_value = actor.agi
when 6
parameter_name = $data_system.words.int
parameter_value = actor.int
when 7
parameter_name = "Evasion"
parameter_value = actor.eva
end
self.contents.font.color = system_color
self.contents.draw_text(x, y, 120, 32, parameter_name)
self.contents.font.color = normal_color
self.contents.draw_text(x + width, y, 36, 32, parameter_value.to_s, 2)
end
end
#==============================================================================
# ** Scene_Title
#------------------------------------------------------------------------------
# This class performs title screen processing.
#==============================================================================
class Scene_Title
#--------------------------------------------------------------------------
# * Alias Listings
#--------------------------------------------------------------------------
alias legacy_CMS_scenetitle_main main
#--------------------------------------------------------------------------
# * Main Processing
#--------------------------------------------------------------------------
def main
$recov = 17
$weapon = 18
$armor = 19
$accessory = 20
$key = 21
$misc = 22
legacy_CMS_scenetitle_main
end
end
#==============================================================================
# ** Window_NewCommand
#------------------------------------------------------------------------------
# This window deals with general command choices.
#==============================================================================
class Window_NewCommand < Window_Selectable
#--------------------------------------------------------------------------
# * Object Initialization
# width : window width
# commands : command text string array
#--------------------------------------------------------------------------
def initialize(width, commands, icon = 'none')
# Compute window height from command quantity
super(0, 0, width, commands.size / 3 * 32 + 32)
@item_max = commands.size
@commands = commands
@icon = icon
@column_max = 3
self.contents = Bitmap.new(width - 32, @item_max/3 * 32)
refresh
self.index = 0
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
for i in 0...@item_max
draw_item(i, normal_color)
end
end
#--------------------------------------------------------------------------
# * Draw Item
# index : item number
# color : text color
#--------------------------------------------------------------------------
def draw_item(index, color)
self.contents.font.color = color
rect = Rect.new((109 * (index / 2)), 32 * (index % 2), self.width / @column_max - 16, 32)
self.contents.fill_rect(rect, Color.new(0, 0, 0, 0))
unless @icon == 'none'
bitmap = RPG::Cache.icon(@icon + index.to_s)
self.contents.blt((106 * (index / 2)), 32 * (index % 2) + 4, bitmap, Rect.new(0, 0, 24, 24))
end
self.contents.draw_text(rect, @commands[index])
end
#--------------------------------------------------------------------------
# * Disable Item
# index : item number
#--------------------------------------------------------------------------
def disable_item(index)
draw_item(index, disabled_color)
end
end
#==============================================================================
# ** Window_NewMenuStatus
#------------------------------------------------------------------------------
# This window displays party member status on the menu screen.
#==============================================================================
class Window_NewMenuStatus < Window_Base
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
$game_party.actors.size != 4 ? i = 14 : i = 0
$game_party.actors.size == 1 ? i = 24 : i = i
super(0, 0, 480, ($game_party.actors.size * 84) + i)
self.contents = Bitmap.new(width - 32, height - 32)
refresh
self.active = false
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
for i in 0...$game_party.actors.size
x = 4
y = (i * 76) - 6
actor = $game_party.actors[i]
self.contents.font.size = 15
self.contents.font.bold = true
draw_actor_class(actor, x, y - 1)
draw_actor_state(actor, x + 160, y - 1)
self.contents.font.size = 15
draw_actor_parameter(actor, x, y + 14, 0)
draw_actor_parameter(actor, x, y + 29, 1)
draw_actor_parameter(actor, x, y + 44, 2)
draw_actor_parameter(actor, x, y + 59, 3)
draw_actor_parameter(actor, x + 240, y + 14, 4)
draw_actor_parameter(actor, x + 240, y + 29, 5)
draw_actor_parameter(actor, x + 240, y + 44, 6)
draw_bar(actor, x + 240, y + 75, 'exp')
end
end
end
#==============================================================================
# ** Window_Actor
#------------------------------------------------------------------------------
# This window displays party member status on the menu screen.
#==============================================================================
class Window_Actor < Window_Selectable
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
$game_party.actors.size != 4 ? i = 14 : i = 0
$game_party.actors.size == 1 ? i = 24 : i = i
super(0, 0, 160, ($game_party.actors.size * 84) + i)
self.contents = Bitmap.new(width - 32, height - 32)
@frame = 0
@potrait = false
@class = false
refresh
self.active = false
self.index = -1
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
@item_max = $game_party.actors.size
for i in 0...$game_party.actors.size
x = 4
y = (i * 77) - 12
actor = $game_party.actors[i]
self.contents.font.size = 17
self.contents.font.bold = true
@potrait ? draw_actor_potrait(actor, x, y - 1, @class) : draw_sprite(x + 20,
y + 57, actor.character_name, actor.character_hue, @frame)
draw_actor_name(actor, x + 52, y + 6)
draw_actor_level(actor, x + 52, y + 24)
draw_bar(actor, x - 3, y + 60, 'hp', 120)
draw_bar(actor, x - 3, y + 75, 'sp', 120)
end
end
#--------------------------------------------------------------------------
# * Cursor Rectangle Update
#--------------------------------------------------------------------------
def update_cursor_rect
if @index < 0
self.cursor_rect.empty
else
self.cursor_rect.set(-4, (@index * 77) - 2, self.width - 24, 77)
end
end
#--------------------------------------------------------------------------
# Frame Update
#--------------------------------------------------------------------------
def frame_update
@frame == 3 ? @frame = 0 : @frame += 1
refresh
end
end
#==============================================================================
# ** Window_Stat
#------------------------------------------------------------------------------
# This window displays play time on the menu screen.
#==============================================================================
class Window_Stat < Window_Base
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
super(0, 0, 320, 96)
self.contents = Bitmap.new(width - 32, height - 32)
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
self.contents.font.color = system_color
self.contents.font.size = 18
self.contents.font.bold = true
self.contents.draw_text(4, -4, 120, 32, "Play Time")
cx = contents.text_size($data_system.words.gold).width
self.contents.draw_text(4, 18, 120, 32, "Step Count")
self.contents.draw_text(4, 38, cx, 32, $data_system.words.gold, 2)
@total_sec = Graphics.frame_count / Graphics.frame_rate
hour = @total_sec / 60 / 60
min = @total_sec / 60 % 60
sec = @total_sec % 60
text = sprintf("%02d:%02d:%02d", hour, min, sec)
self.contents.font.color = normal_color
self.contents.draw_text(144, -4, 120, 32, text, 2)
self.contents.draw_text(144, 18, 120, 32, $game_party.steps.to_s, 2)
self.contents.draw_text(144, 40, 120, 32, $game_party.gold.to_s, 2)
end
#--------------------------------------------------------------------------
# * Frame Update
#--------------------------------------------------------------------------
def update
super
if Graphics.frame_count / Graphics.frame_rate != @total_sec
refresh
end
end
end
#==============================================================================
# ** Window_Location
#------------------------------------------------------------------------------
# This window displays current map name.
#==============================================================================
class Window_Location < Window_Base
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
super(0, 0, 640, 48)
self.contents = Bitmap.new(width - 32, height - 32)
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
self.contents.font.color = system_color
self.contents.font.bold = true
self.contents.font.size = 20
self.contents.draw_text(4, -4, 120, 24, "Location")
self.contents.font.color = normal_color
self.contents.draw_text(170, -4, 400, 24, $game_map.name.to_s, 2)
end
end
#==============================================================================
# ** Window_NewHelp
#------------------------------------------------------------------------------
# This window shows skill and item explanations along with actor status.
#==============================================================================
class Window_NewHelp < Window_Base
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
super(0, 0, 640, 48)
self.contents = Bitmap.new(width - 32, height - 32)
end
#--------------------------------------------------------------------------
# * Set Text
# text : text string displayed in window
# align : alignment (0..flush left, 1..center, 2..flush right)
#--------------------------------------------------------------------------
def set_text(text, align = 0)
self.contents.font.bold = true
self.contents.font.size = 20
# If at least one part of text and alignment differ from last time
if text != @text or align != @align
# Redraw text
self.contents.clear
self.contents.font.color = normal_color
self.contents.draw_text(4, -4, self.width - 40, 24, text, align)
@text = text
@align = align
@actor = nil
end
self.visible = true
end
end
#==============================================================================
# ** Window_NewItem
#------------------------------------------------------------------------------
# This window displays items in possession on the item and battle screens.
#==============================================================================
class Window_NewItem < Window_Selectable
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
super(0, 96, 480, 336)
@column_max = 2
@attribute = $recov
refresh
self.index = 0
self.active = false
end
#--------------------------------------------------------------------------
# * Get Item
#--------------------------------------------------------------------------
def item
return @data[self.index]
end
#--------------------------------------------------------------------------
# * Updates Window With New Item Type
# attribute : new item type
#--------------------------------------------------------------------------
def update_item(attribute)
@attribute = attribute
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
if self.contents != nil
self.contents.dispose
self.contents = nil
end
@data = []
# Add item
for i in 1...$data_items.size
if $game_party.item_number(i) > 0 and
$data_items[i].element_set.include?(@attribute)
@data.push($data_items[i])
end
end
# Also add weapons and armors
for i in 1...$data_weapons.size
if $game_party.weapon_number(i) > 0 and
$data_weapons[i].element_set.include?(@attribute)
@data.push($data_weapons[i])
end
end
for i in 1...$data_armors.size
if $game_party.armor_number(i) > 0 and
$data_armors[i].guard_element_set.include?(@attribute)
@data.push($data_armors[i])
end
end
# If item count is not 0, make a bit map and draw all items
@item_max = @data.size
if @item_max > 0
self.contents = Bitmap.new(width - 32, row_max * 32)
for i in 0...@item_max
draw_item(i)
end
end
end
#--------------------------------------------------------------------------
# * Draw Item
# index : item number
#--------------------------------------------------------------------------
def draw_item(index)
item = @data[index]
case item
when RPG::Item
number = $game_party.item_number(item.id)
when RPG::Weapon
number = $game_party.weapon_number(item.id)
when RPG::Armor
number = $game_party.armor_number(item.id)
end
if item.is_a?(RPG::Item) and
$game_party.item_can_use?(item.id)
self.contents.font.color = normal_color
else
self.contents.font.color = disabled_color
end
x = 4 + index % 2 * (208 + 32)
y = index / 2 * 32
rect = Rect.new(x, y, self.width / @column_max - 32, 32)
self.contents.fill_rect(rect, Color.new(0, 0, 0, 0))
bitmap = RPG::Cache.icon(item.icon_name)
opacity = self.contents.font.color == normal_color ? 255 : 128
self.contents.blt(x, y + 4, bitmap, Rect.new(0, 0, 24, 24), opacity)
self.contents.draw_text(x + 28, y, 132, 32, item.name, 0)
self.contents.draw_text(x + 160, y, 16, 32, ":", 1)
self.contents.draw_text(x + 176, y, 24, 32, number.to_s, 2)
end
#--------------------------------------------------------------------------
# * Help Text Update
#--------------------------------------------------------------------------
def update_help
@help_window.set_text(self.item == nil ? "" : self.item.description)
end
end
#==============================================================================
# ** Window_NewSkill
#------------------------------------------------------------------------------
# This window displays usable skills on the skill screen.
#==============================================================================
class Window_NewSkill < Window_Selectable
#--------------------------------------------------------------------------
# * Object Initialization
# actor : actor
#--------------------------------------------------------------------------
def initialize(actor)
super(0, 96, 480, 336)
@actor = actor
@column_max = 2
refresh
self.index = 0
self.active = false
end
#--------------------------------------------------------------------------
# * Acquiring Skill
#--------------------------------------------------------------------------
def skill
return @data[self.index]
end
#--------------------------------------------------------------------------
# * Updates Window With New Actor
# actor : new actor
#--------------------------------------------------------------------------
def update_actor(actor)
@actor = actor
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
if self.contents != nil
self.contents.dispose
self.contents = nil
end
@data = []
for i in [email=0...@actor.skills.size]0...@actor.skills.size[/email]
skill = $data_skills[@actor.skills[i]]
if skill != nil
@data.push(skill)
end
end
# If item count is not 0, make a bit map and draw all items
@item_max = @data.size
if @item_max > 0
self.contents = Bitmap.new(width - 32, row_max * 32)
for i in 0...@item_max
draw_item(i)
end
end
end
#--------------------------------------------------------------------------
# * Draw Item
# index : item number
#--------------------------------------------------------------------------
def draw_item(index)
skill = @data[index]
if @actor.skill_can_use?(skill.id)
self.contents.font.color = normal_color
else
self.contents.font.color = disabled_color
end
x = 4 + index % 2 * (208 + 32)
y = index / 2 * 32
rect = Rect.new(x, y, self.width / @column_max - 32, 32)
self.contents.fill_rect(rect, Color.new(0, 0, 0, 0))
bitmap = RPG::Cache.icon(skill.icon_name)
opacity = self.contents.font.color == normal_color ? 255 : 128
self.contents.blt(x, y + 4, bitmap, Rect.new(0, 0, 24, 24), opacity)
self.contents.draw_text(x + 28, y, 124, 32, skill.name, 0)
self.contents.draw_text(x + 152 , y, 48, 32, skill.sp_cost.to_s, 2)
end
#--------------------------------------------------------------------------
# * Help Text Update
#--------------------------------------------------------------------------
def update_help
@help_window.set_text(self.skill == nil ? "" : self.skill.description)
end
end
#==============================================================================
# ** Window_EquipStat
#------------------------------------------------------------------------------
# This window displays actor parameter changes on the equipment screen.
#==============================================================================
class Window_EquipStat < Window_Base
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_accessor :changes
attr_accessor :mode
#--------------------------------------------------------------------------
# * Object Initialization
# actor : actor
#--------------------------------------------------------------------------
def initialize(actor)
super(0, 96, 240, 336)
self.contents = Bitmap.new(width - 32, height - 32)
@actor = actor
@changes = [0, 0, 0, 0, 0, 0, 0, 0]
@mode = 0
@elem_text = ""
@stat_text = ""
refresh
end
#--------------------------------------------------------------------------
# * Updates Window With New Actor
# actor : new actor
#--------------------------------------------------------------------------
def update_actor(actor)
@actor = actor
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
self.contents.font.size = 18
self.contents.font.bold = true
for i in 0..7
draw_actor_parameter(@actor, 16, (i * 20) - 8, i, 96)
end
if @new_atk != nil
self.contents.font.color = system_color
self.contents.draw_text(152, -8, 32, 32, "»»", 1)
if @changes[0] == 0
self.contents.font.color = normal_color
elsif @changes[0] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, -8, 32, 32, @new_atk.to_s, 2)
end
if @new_pdef != nil
self.contents.font.color = system_color
self.contents.draw_text(152, 12, 32, 32, "»»", 1)
if @changes[1] == 0
self.contents.font.color = normal_color
elsif @changes[1] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, 12, 32, 32, @new_pdef.to_s, 2)
end
if @new_mdef != nil
self.contents.font.color = system_color
self.contents.draw_text(152, 32, 32, 32, "»»", 1)
if @changes[2] == 0
self.contents.font.color = normal_color
elsif @changes[2] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, 32, 32, 32, @new_mdef.to_s, 2)
end
if @new_str != nil
self.contents.font.color = system_color
self.contents.draw_text(152, 52, 32, 32, "»»", 1)
if @changes[3] == 0
self.contents.font.color = normal_color
elsif @changes[3] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, 52, 32, 32, @new_str.to_s, 2)
end
if @new_dex != nil
self.contents.font.color = system_color
self.contents.draw_text(152, 72, 32, 32, "»»", 1)
if @changes[4] == 0
self.contents.font.color = normal_color
elsif @changes[4] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, 72, 32, 32, @new_dex.to_s, 2)
end
if @new_agi != nil
self.contents.font.color = system_color
self.contents.draw_text(152, 92, 32, 32, "»»", 1)
if @changes[5] == 0
self.contents.font.color = normal_color
elsif @changes[5] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, 92, 32, 32, @new_agi.to_s, 2)
end
if @new_int != nil
self.contents.font.color = system_color
self.contents.draw_text(152, 112, 32, 32, "»»", 1)
if @changes[6] == 0
self.contents.font.color = normal_color
elsif @changes[6] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, 112, 32, 32, @new_int.to_s, 2)
end
if @new_eva != nil
self.contents.font.color = system_color
self.contents.draw_text(152, 132, 32, 32, "»»", 1)
if @changes[7] == 0
self.contents.font.color = normal_color
elsif @changes[7] == -1
self.contents.font.color = down_color
else
self.contents.font.color = up_color
end
self.contents.draw_text(176, 132, 32, 32, @new_eva.to_s, 2)
end
if @mode == 0
unless @actor.weapon_id == 0 or @actor.weapon_id == nil
# If this actor DOES have weapons equipped, sets up data
level = $game_weapons[@actor.weapon_id].weapon_level
rank = $game_weapons[@actor.weapon_id].weapon_class
exp = $game_weapons[@actor.weapon_id].exp
n_exp = $game_weapons[@actor.weapon_id].next_exp
break_amount = $game_weapons[@actor.weapon_id].break_amount
broken = $game_weapons[@actor.weapon_id].weapon_broke? == true ? "Yes" : "No"
# So, we're now going to make the text
self.contents.font.color = system_color
self.contents.draw_text(4, 160, self.width - 32, 32, "Skill Level")
self.contents.draw_text(4, 180, self.width - 32, 32, "Rank")
self.contents.draw_text(4, 200, self.width - 32, 32, "EXP")
self.contents.draw_text(4, 220, self.width - 32, 32, "Next Level")
self.contents.draw_text(4, 240, self.width - 32, 32, "Break %")
self.contents.draw_text(4, 260, self.width - 32, 32, "Broken?")
# Text done, the data is now shown in the proper line
self.contents.font.color = normal_color
self.contents.draw_text(129, 160, self.width - 32, 32, level.to_s)
self.contents.draw_text(129, 180, self.width - 32, 32, rank.to_s)
self.contents.draw_text(129, 200, self.width - 32, 32, exp.to_s)
self.contents.draw_text(129, 220, self.width - 32, 32, n_exp.to_s)
self.contents.draw_text(129, 240, self.width - 32, 32, break_amount.to_s)
self.contents.draw_text(129, 260, self.width - 32, 32, broken)
else
# If there are no weapons equipped, show this message
# Otherwise it would crash!
self.contents.font.color = system_color
self.contents.draw_text(4, 258, self.width - 32, 32, "No Weapon!")
end
end
end
#--------------------------------------------------------------------------
# * Set parameters after changing equipment
# new_atk : attack power after changing equipment
# new_pdef : physical defense after changing equipment
# new_mdef : magic defense after changing equipment
# new_str : strength after changing equipment
# new_dex : dexterity after changing equipment
# new_agi : agility after changing equipment
# new_int : inteligence after changing equipment
# new_eva : evasion after changing equipment
#--------------------------------------------------------------------------
def set_new_parameters(new_atk, new_pdef, new_mdef, new_str, new_dex,
new_agi, new_int, new_eva, elem_text, stat_text)
flag = false
if new_atk != @new_atk || new_pdef != @new_pdef || new_str != @new_str ||
new_mdef != @new_mdef || new_dex != @new_dex || new_agi != @new_agi ||
new_eva != @new_eva || elem_text != @elem_text || stat_text != @stat_text
flag = true
end
@new_atk = new_atk
@new_pdef = new_pdef
@new_mdef = new_mdef
@new_str = new_str
@new_dex = new_dex
@new_agi = new_agi
@new_int = new_int
@new_eva = new_eva
@elem_text = elem_text
@stat_text = stat_text
if flag
refresh
end
end
end
#==============================================================================
# ** Window_Equipment
#------------------------------------------------------------------------------
# This window displays items the actor is currently equipped with on the
# equipment screen.
#==============================================================================
class Window_Equipment < Window_Selectable
#--------------------------------------------------------------------------
# * Object Initialization
# actor : actor
#--------------------------------------------------------------------------
def initialize(actor)
super(0, 96, 240, 176)
self.contents = Bitmap.new(width - 32, height - 32)
@actor = actor
refresh
self.index = 0
self.active = false
end
#--------------------------------------------------------------------------
# * Updates Window With New Actor
# actor : new actor
#--------------------------------------------------------------------------
def update_actor(actor)
@actor = actor
refresh
end
#--------------------------------------------------------------------------
# * Item Acquisition
#--------------------------------------------------------------------------
def item
return @data[self.index]
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
@data = []
@data.push($data_weapons[@actor.weapon_id])
@data.push($data_armors[@actor.armor1_id])
@data.push($data_armors[@actor.armor2_id])
@data.push($data_armors[@actor.armor3_id])
@data.push($data_armors[@actor.armor4_id])
@item_max = @data.size
self.contents.font.color = system_color
self.contents.font.size = 19
self.contents.font.bold = true
self.contents.draw_text(0, 28 * 0, 76, 32, $data_system.words.weapon)
self.contents.draw_text(0, 28 * 1, 76, 32, $data_system.words.armor1)
self.contents.draw_text(0, 28 * 2, 76, 32, $data_system.words.armor2)
self.contents.draw_text(0, 28 * 3, 76, 32, $data_system.words.armor3)
self.contents.draw_text(0, 28 * 4, 76, 32, $data_system.words.armor4)
self.contents.font.bold = false
for i in 0..4
draw_item_name(@data[i], 76, 28 * i)
end
end
#--------------------------------------------------------------------------
# * Update Cursor Rectangle
#--------------------------------------------------------------------------
def update_cursor_rect
# Calculate cursor width
cursor_width = self.width / @column_max - 24
# Calculate cursor coordinates
x = @index % @column_max * (cursor_width + 24)
y = @index * 28
# Update cursor rectangle
self.cursor_rect.set(x - 4, y, cursor_width, 32)
end
#--------------------------------------------------------------------------
# * Help Text Update
#--------------------------------------------------------------------------
def update_help
@help_window.set_text(self.item == nil ? "" : self.item.description)
end
end
#==============================================================================
# ** Window_EquipmentItem
#------------------------------------------------------------------------------
# This window displays choices when opting to change equipment on the
# equipment screen.
#==============================================================================
class Window_EquipmentItem < Window_Selectable
#--------------------------------------------------------------------------
# * Object Initialization
# actor : actor
# equip_type : equip region (0-3)
#--------------------------------------------------------------------------
def initialize(actor, equip_type)
super(0, 272, 240, 160)
@actor = actor
@equip_type = equip_type
refresh
self.active = false
self.index = -1
end
#--------------------------------------------------------------------------
# * Updates Window With New Actor
# actor : new actor
#--------------------------------------------------------------------------
def update_actor(actor)
@actor = actor
refresh
end
#--------------------------------------------------------------------------
# * Updates Window With New Equipment Type
# equip_type : new teyp of equipment
#--------------------------------------------------------------------------
def update_equipment(equip_type)
@equip_type = equip_type
refresh
end
#--------------------------------------------------------------------------
# * Item Acquisition
#--------------------------------------------------------------------------
def item
if self.index == 0
return @data[@item_max - 1]
else
return @data[self.index - 1]
end
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
if self.contents != nil
self.contents.dispose
self.contents = nil
end
@data = []
# Add equippable weapons
if @equip_type == 0
weapon_set = $data_classes[@actor.class_id].weapon_set
for i in 1...$data_weapons.size
if $game_party.weapon_number(i) > 0 and weapon_set.include?(i)
@data.push($data_weapons[i])
end
end
end
# Add equippable armor
if @equip_type != 0
armor_set = $data_classes[@actor.class_id].armor_set
for i in 1...$data_armors.size
if $game_party.armor_number(i) > 0 and armor_set.include?(i)
if $data_armors[i].kind == @equip_type-1
@data.push($data_armors[i])
end
end
end
end
# Add blank page
@data.push(nil)
# Make a bit map and draw all items
@item_max = @data.size
self.contents = Bitmap.new(width - 32, row_max * 32)
for i in 0...@item_max - 1
draw_item(i)
end
self.contents.draw_text(4, 0, 204, 32, 'Unequip', 0)
end
#--------------------------------------------------------------------------
# * Draw Item
# index : item number
#--------------------------------------------------------------------------
def draw_item(index)
item = @data[index]
x = 4
y = (index + 1) * 32
case item
when RPG::Weapon
number = $game_party.weapon_number(item.id)
when RPG::Armor
number = $game_party.armor_number(item.id)
end
bitmap = RPG::Cache.icon(item.icon_name)
self.contents.blt(x, y + 4, bitmap, Rect.new(0, 0, 24, 24))
self.contents.font.color = normal_color
self.contents.font.size = 20
self.contents.font.bold = true
self.contents.draw_text(x + 28, y, 132, 32, item.name, 0)
self.contents.draw_text(x + 160, y, 12, 32, ":", 1)
self.contents.draw_text(x + 176, y, 24, 32, number.to_s, 2)
end
#--------------------------------------------------------------------------
# * Help Text Update
#--------------------------------------------------------------------------
def update_help
@help_window.set_text(self.item == nil ? 'Unequip the current equipment.' :
self.item.description)
end
end
#==============================================================================
# ** Window_Status
#------------------------------------------------------------------------------
# This window displays full status specs on the status screen.
#==============================================================================
class Window_NewStatus < Window_Base
def initialize(actor)
super(0, 96, 640, 336)
self.contents = Bitmap.new(width - 32, height - 32)
@actor = actor
@potrait = false
@class = false
refresh
self.active = false
end
#--------------------------------------------------------------------------
# * Updates Window With New Actor
# actor : new actor
#--------------------------------------------------------------------------
def update_actor(actor)
@actor = actor
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
self.contents.font.size = 16
self.contents.font.bold = false
draw_actor_name(@actor, 12, 0)
if @potrait
draw_actor_potrait(@actor, 12, 40, @class)
draw_bar(@actor, 120, 60, 'hp', 200)
draw_bar(@actor, 120, 92, 'sp', 200)
draw_bar(@actor, 120, 124, 'exp', 200)
else
draw_actor_graphic(@actor, 40, 120)
draw_bar(@actor, 96, 60, 'hp', 200)
draw_bar(@actor, 96, 92, 'sp', 200)
draw_bar(@actor, 96, 124, 'exp', 200)
end
draw_actor_class(@actor, 262, 0)
draw_actor_level(@actor, 184, 0)
draw_actor_state(@actor, 96, 0)
self.contents.font.size = 17
self.contents.font.bold = true
for i in 0..7
draw_actor_parameter(@actor, 386, (i * 20) - 6, i)
end
self.contents.font.color = system_color
self.contents.font.size = 16
draw_actor_element_radar_graph(@actor, 48, 136)
self.contents.font.size = 18
self.contents.font.color = system_color
self.contents.draw_text(380, 156, 96, 32, "Equipment")
self.contents.draw_text(300, 180, 96, 32, "Weapon")
self.contents.draw_text(300, 204, 96, 32, "Shield")
self.contents.draw_text(300, 228, 96, 32, "Helmet")
self.contents.draw_text(300, 252, 96, 32, "Armor")
self.contents.draw_text(300, 276, 96, 32, "Accessory")
equip = $data_weapons[@actor.weapon_id]
if @actor.equippable?(equip)
draw_item_name($data_weapons[@actor.weapon_id], 406, 180)
else
self.contents.font.color = knockout_color
self.contents.draw_text(406, 180, 192, 32, "Nothing equipped")
end
equip1 = $data_armors[@actor.armor1_id]
if @actor.equippable?(equip1)
draw_item_name($data_armors[@actor.armor1_id], 406, 204)
else
self.contents.font.color = crisis_color
self.contents.draw_text(406, 204, 192, 32, "Nothing equipped")
end
equip2 = $data_armors[@actor.armor2_id]
if @actor.equippable?(equip2)
draw_item_name($data_armors[@actor.armor2_id], 406, 228)
else
self.contents.font.color = crisis_color
self.contents.draw_text(406, 228, 192, 32, "Nothing equipped")
end
equip3 = $data_armors[@actor.armor3_id]
if @actor.equippable?(equip3)
draw_item_name($data_armors[@actor.armor3_id], 406, 252)
else
self.contents.font.color = crisis_color
self.contents.draw_text(406, 252, 192, 32, "Nothing equipped")
end
equip4 = $data_armors[@actor.armor4_id]
if @actor.equippable?(equip4)
draw_item_name($data_armors[@actor.armor4_id], 406, 276)
else
self.contents.font.color = crisis_color
self.contents.draw_text(406, 276, 192, 32, "Nothing equipped")
end
end
def dummy
self.contents.font.color = system_color
self.contents.draw_text(320, 112, 96, 32, $data_system.words.weapon)
self.contents.draw_text(320, 176, 96, 32, $data_system.words.armor1)
self.contents.draw_text(320, 240, 96, 32, $data_system.words.armor2)
self.contents.draw_text(320, 304, 96, 32, $data_system.words.armor3)
self.contents.draw_text(320, 368, 96, 32, $data_system.words.armor4)
draw_item_name($data_weapons[@actor.weapon_id], 320 + 24, 144)
draw_item_name($data_armors[@actor.armor1_id], 320 + 24, 208)
draw_item_name($data_armors[@actor.armor2_id], 320 + 24, 272)
draw_item_name($data_armors[@actor.armor3_id], 320 + 24, 336)
draw_item_name($data_armors[@actor.armor4_id], 320 + 24, 400)
end
end
#==============================================================================
# ** Scene_Menu
#------------------------------------------------------------------------------
# This class performs menu screen processing.
#==============================================================================
class Scene_Menu
#--------------------------------------------------------------------------
# * Object Initialization
# menu_index : command cursor's initial position
#--------------------------------------------------------------------------
def initialize(menu_index = 0)
@menu_index = menu_index
@update_frame = 0
@animate = false # True if you want the sprite to be animated.
@extraequip = true # True if you want to have optimize and unequip all command.
@targetactive = false
@exit = false
@actor = $game_party.actors[0]
end
#--------------------------------------------------------------------------
# * Main Processing
#--------------------------------------------------------------------------
def main
# Make command window
s1 = ' ' + $data_system.words.item
s2 = ' ' + $data_system.words.skill
s3 = ' ' + $data_system.words.equip
s4 = ' ' + 'Status'
s5 = ' ' + 'Save'
s6 = ' ' + 'Exit'
t1 = ' ' + 'Recov.'
t2 = ' ' + 'Weaponry'
t3 = 'Armor'
t4 = 'Accessory'
t5 = 'Quest'
t6 = 'Misc.'
u1 = 'To Title'
u2 = 'Quit'
v1 = 'Optimize'
v2 = 'Unequip All'
@command_window = Window_NewCommand.new(320, [s1, s2, s3, s4, s5, s6], 'menu')
@command_window.y = -96
@command_window.index = @menu_index
# If number of party members is 0
if $game_party.actors.size == 0
# Disable items, skills, equipment, and status
@command_window.disable_item(0)
@command_window.disable_item(1)
@command_window.disable_item(2)
@command_window.disable_item(3)
end
# If save is forbidden
if $game_system.save_disabled
# Disable save
@command_window.disable_item(4)
end
# Make stat window
@stat_window = Window_Stat.new
@stat_window.x = 320
@stat_window.y = -96
# Make status window
@status_window = Window_NewMenuStatus.new
@status_window.x = 640
@status_window.y = 96
#@status_window.z = 110
@location_window = Window_Location.new
@location_window.y = 480
@actor_window = Window_Actor.new
@actor_window.x = -160
@actor_window.y = 96
@itemcommand_window = Window_NewCommand.new(320, [t1, t2, t3, t4, t5, t6])
@itemcommand_window.x = -320
@itemcommand_window.active = false
@help_window = Window_NewHelp.new
@help_window.x = -640
@help_window.y = 432
@item_window = Window_NewItem.new
@item_window.x = -480
@item_window.help_window = @help_window
@skill_window = Window_NewSkill.new(@actor)
@skill_window.x = -480
@skill_window.help_window = @help_window
@equipstat_window = Window_EquipStat.new(@actor)
@equipstat_window.x = -480
@equip_window = Window_Equipment.new(@actor)
@equip_window.x = -240
@equip_window.help_window = @help_window
@equipitem_window = Window_EquipmentItem.new(@actor, 0)
@equipitem_window.x = -240
@equipitem_window.help_window = @help_window
@equipenhanced_window = Window_Command.new(160, [v1, v2])
@equipenhanced_window.x = -160
@equipenhanced_window.active = false
@playerstatus_window = Window_NewStatus.new(@actor)
@playerstatus_window.x = -640
@end_window = Window_Command.new(120, [u1, u2])
@end_window.x = 640
@end_window.active = false
@spriteset = Spriteset_Map.new
@windows = [@command_window, @stat_window, @status_window,
@location_window, @actor_window, @itemcommand_window,@help_window,
@item_window, @skill_window, @equipstat_window, @equip_window,
@equipitem_window, @equipenhanced_window, @playerstatus_window,
@end_window]
@windows.each {|i| i.opacity = 200}
# Execute transition
Graphics.transition
# Main loop
loop do
# Update game screen
Graphics.update
# Update input information
Input.update
# Frame update
update
# Abort loop if screen is changed
if $scene != self
break
end
end
# Prepare for transition
Graphics.freeze
# Dispose of windows
@spriteset.dispose
@windows.each {|i| i.dispose}
end
#--------------------------------------------------------------------------
# * Frame Update
#--------------------------------------------------------------------------
def update
# Update windows
@windows.each {|i| i.update}
animate
menu_update
if @animate
@update_frame += 1
if @update_frame == 3
@update_frame = 0
@actor_window.frame_update
end
end
end
#--------------------------------------------------------------------------
# * Animating windows.
#--------------------------------------------------------------------------
def animate
if @command_window.active && @skill_window.x == -480
@command_window.y += 6 if @command_window.y < 0
@stat_window.y += 6 if @stat_window.y < 0
@actor_window.x += 10 if @actor_window.x < 0
@status_window.x -= 30 if @status_window.x > 160
@location_window.y -= 3 if @location_window.y > 432
elsif @exit == true
@command_window.y -= 6 if @command_window.y > -96
@stat_window.y -= 6 if @stat_window.y > -96
@actor_window.x -= 10 if @actor_window.x > -160
@status_window.x += 30 if @status_window.x < 640
@location_window.y += 3 if @location_window.y < 480
$scene = Scene_Map.new if @location_window.y == 480
end
if @itemcommand_window.active
if @itemcommand_window.x < 0
@stat_window.x += 40
@command_window.x += 40
@itemcommand_window.x += 40
end
else
if @itemcommand_window.x > -320 && @command_window.active
@stat_window.x -= 40
@command_window.x -= 40
@itemcommand_window.x -= 40
end
end
if @item_window.active
if @item_window.x < 0
@location_window.x += 40
@help_window.x += 40
@status_window.x += 30
@actor_window.x += 30
@item_window.x += 30
end
elsif @targetactive != true
if @item_window.x > -480 && @command_window.index == 0
@help_window.x -= 40
@location_window.x -= 40
@item_window.x -= 30
@actor_window.x -= 30
@status_window.x -= 30
end
end
if @skill_window.active
if @skill_window.x < 0
@location_window.x += 40
@help_window.x += 40
@status_window.x += 30
@actor_window.x += 30
@skill_window.x += 30
end
elsif @targetactive != true
if @skill_window.x > -480 && @command_window.index == 3
@help_window.x -= 40
@location_window.x -= 40
@skill_window.x -= 30
@actor_window.x -= 30
@status_window.x -= 30
end
end
if @equip_window.active
if @equipstat_window.x < 0
@status_window.x += 48
@actor_window.x += 48
@equip_window.x += 48
@equipitem_window.x += 48
@equipstat_window.x += 48
@location_window.x += 64
@help_window.x += 64
end
elsif ! @equipitem_window.active && ! @equipenhanced_window.active
if @equipstat_window.x > -480 && @command_window.index == 1
@equipstat_window.x -= 48
@equip_window.x -= 48
@equipitem_window.x -= 48
@actor_window.x -= 48
@status_window.x -= 48
@help_window.x -= 64
@location_window.x -= 64
end
end
if @equipenhanced_window.active
if @equipenhanced_window.x < 0
@stat_window.x += 16
@command_window.x += 16
@equipenhanced_window.x += 16
end
else
if @equipenhanced_window.x > -160 && @equip_window.active
@equipenhanced_window.x -= 16
@stat_window.x -= 16
@command_window.x -= 16
end
end
if @playerstatus_window.active
if @playerstatus_window.x < 0
@status_window.x += 40
@actor_window.x += 40
@playerstatus_window.x += 40
end
else
if @playerstatus_window.x > -640 && @command_window.index == 4
@playerstatus_window.x -= 40
@actor_window.x -= 40
@status_window.x -= 40
end
end
if @end_window.active
blt(x, y, src_bitmap, src_rect[, opacity])
Performs a block transfer from the src_bitmap box src_rect (Rect) to the specified bitmap coordinates (x, y).
opacity can be set from 0 to 255.
contents
Refers to the bitmap used for the window's contents.
RPG::Cache
A module that loads each of RPGXP's graphic formats, creates a Bitmap object, and retains it.
RPG::Cache.icon(filename)
Gets an icon graphic.
# We need a window to make this work xD
window = Window_Base.new(0, 0, 64, 64)
window.contents = Bitmap.new(32, 32)
# First of all you only can insert a bitmap inside a bitmap.
# So the first thing we should do is to load the icon
icon = RPG::Cache.icon("001-Weapon01")
# After loading the icon it´s a good practice to prepare all we need
# pass within the blt command
# -> Source Rectangle taken from the icon (in our case will be all the bitmap)
rect = Rect.new(0, 0, icon.width, icon.height)
# -> X and Y Position of the icon inside the window
x = 10
y = 10
# Well opacity is not required and we don´t really need to put it here ^^
# Now, calling the blt!
window.contents.blt(x, y, icon, rect)
# And that´s all ^^ Try to test this script inside a map event.