ZericCallavis
Member
So, I'm trying to make a few changes to the battle system. Nothing really too fancy, just some basic changes.
What I'm having trouble with right now, is setting up the cursor rectangle to work with this layout:
I've already got it to the default position on the Attack Icon.
Here is the code I'm working with (note: its not complete, I know I'm missing an Input: UP, but I wanted to get left and right to work first before I started with up and down.
Here is what happens when I hit the right arrow key:
I've tried changing the @index multiplier, and the constant that's added or subtracted to it, but to no avail.
Also, I am curious as to if the format of the code is right, considering I made a copy of the cursor rectangle script I was using from my Window_Target script and just pasted it into Window_BattleCommand. I'm curious because of the $game_party.actor.size in the script. I don't think it should be there, but I am unsure of what to replace it with.
If you need the dimensions of the pictures being used for calculation purposes, the are 48 x 48 pixels. The inner square of the picture is fitted perfectly by a 36 x 36 pixel rectangle. There is a 50 pixel difference on the x and y axis for each icon.
I appreciate any help that anyone can provide. Thank you.
EDITTED: I'm pretty sure $game_party.actor.size should be replaced with @item_max, since @item_max in this case = commands.size. Or am I wrong?
EDIT NUMBER 2:
While trying to figure this out, I've begun looking up ruby coding and what not, and I'm trying to understand how this if/else statements work. From what I understand....
@index > 0 ? @index -= 1 : @index = (@item_max - 1)
? refers to a boolean expression. This returns either a true or false. If the boolean expression is true, the part before the colon is used, and if the boolean expression is false, the the part after the colon is used? Am I close?
What I'm having trouble with right now, is setting up the cursor rectangle to work with this layout:
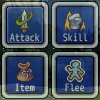
I've already got it to the default position on the Attack Icon.
Here is the code I'm working with (note: its not complete, I know I'm missing an Input: UP, but I wanted to get left and right to work first before I started with up and down.
Code:
 def update_cursor_rect
   self.cursor_rect.set(@index * 60 + 10, 10, 36, 36)
  if Input.trigger?(Input::LEFT)
   @index > 0 ? @index -= 1 : @index = ($game_party.actors.size - 1)
   $game_system.se_play($data_system.cursor_se)
   self.cursor_rect.set(@index * 60 - 10, 10, 36, 36)
  elsif Input.trigger?(Input::RIGHT)
   @index < ($game_party.actors.size - 1) ? @index += 1 : @index = 0
   $game_system.se_play($data_system.cursor_se)
   self.cursor_rect.set(@index * 60 - 10, 10, 32, 32)
  elsif Input.trigger?(Input::DOWN)
   @index < ($game_party.actors.size - 1) ? @index += 1 : @index = 0
   $game_system.se_play($data_system.cursor_se)
   self.cursor_rect.set(@index * 60 - 10, 10, 32, 32)
  end
 end
Here is what happens when I hit the right arrow key:
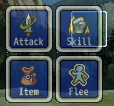
I've tried changing the @index multiplier, and the constant that's added or subtracted to it, but to no avail.
Also, I am curious as to if the format of the code is right, considering I made a copy of the cursor rectangle script I was using from my Window_Target script and just pasted it into Window_BattleCommand. I'm curious because of the $game_party.actor.size in the script. I don't think it should be there, but I am unsure of what to replace it with.
If you need the dimensions of the pictures being used for calculation purposes, the are 48 x 48 pixels. The inner square of the picture is fitted perfectly by a 36 x 36 pixel rectangle. There is a 50 pixel difference on the x and y axis for each icon.
I appreciate any help that anyone can provide. Thank you.
EDITTED: I'm pretty sure $game_party.actor.size should be replaced with @item_max, since @item_max in this case = commands.size. Or am I wrong?
EDIT NUMBER 2:
While trying to figure this out, I've begun looking up ruby coding and what not, and I'm trying to understand how this if/else statements work. From what I understand....
@index > 0 ? @index -= 1 : @index = (@item_max - 1)
? refers to a boolean expression. This returns either a true or false. If the boolean expression is true, the part before the colon is used, and if the boolean expression is false, the the part after the colon is used? Am I close?