Hey y'all
I'm trying to make a speech-bubble type message system work with SBS
The only one I found so far that does pretty much precisely what I wanted (bubble speech + functioning speech in combat) is Wachunga MMW
But I find that it conflicts with Enu/Atoa's SBS
This is the error message I'm getting
Looking for a fix, I found out someone else had a similar issue years ago, but it was left unresolved.
I'd be willing to switch for another message system entirely...
I actually attempted using another one, Custom Message Script by Hodgeelmf
While that one doesn't crash on entering a battle, it does crash when battle text would be displayed.
I thought of maybe figuring a way to temporarily disable it in gameplay
So, deactivate it before a fight that requires speech, using boring-default-speech, and then reactivate it
But my no-scripting-skills self couldn't figure how to do that without breaking anything.
I'm assuming the issues stem from the fact SBS uses non-default spritesets?... I'm not exactly a scripter, so I just stumble in the dark here. I figured maybe someone else could know how to actually fix this.
Is anyone able to help make this work?
Thank you for your patience.
I'm trying to make a speech-bubble type message system work with SBS
The only one I found so far that does pretty much precisely what I wanted (bubble speech + functioning speech in combat) is Wachunga MMW
Code:
#==============================================================================
# ** Multiple Message Windows
#------------------------------------------------------------------------------
# Wachunga
# 1.1
# 2006-11-10
# See [url=https://github.com/wachunga/rmxp-multiple-message-windows]https://github.com/wachunga/rmxp-multip ... ge-windows[/url] for details
#==============================================================================
#==============================================================================
# Settings
#==============================================================================
# filename of message tail used for speech bubbles
# must be in the Graphics/Windowskins/ folder
FILENAME_SPEECH_TAIL = "blue-speech_tail.png"
# note that gradient windowskins aren't ideal for tails
# filenames of message tail and windowskin used for thought bubbles
# must also be in the Graphics/Windowskins/ folder
FILENAME_THOUGHT_TAIL = "white-thought_tail.png"
FILENAME_THOUGHT_WINDOWSKIN = "white-windowskin.png"
# used for message.location
TOP = 8
BOTTOM = 2
LEFT = 4
RIGHT = 6
class Game_Message
# Any of the below can be changed by a Call Script event during gameplay.
# E.g. turn letter-by-letter mode off with: message.letter_by_letter = false
attr_accessor :move_during
attr_accessor :letter_by_letter
attr_accessor :text_speed
attr_accessor :skippable
attr_accessor :resize
attr_accessor :floating
attr_accessor :autocenter
attr_accessor :show_tail
attr_accessor :show_pause
attr_accessor :location
attr_accessor :font_name
attr_accessor :font_size
attr_accessor :font_color
attr_accessor :font_color_thought
def initialize
# whether or not messages appear one letter at a time
@letter_by_letter = true
# note: can also change within a single message with \L
# the default speed at which text appears in letter-by-letter mode
@text_speed = 1
# note: can also change within a single message with \S[n]
# whether or not players can skip to the end of (letter-by-letter) messages
@skippable = true
# whether or not messages are automatically resized based on the message
@resize = true
# whether or not message windows are positioned above
# characters/events by default, i.e. without needing \P every message
# (only works if resize messages enabled -- otherwise would look very odd)
@floating = true
# whether or not to automatically center lines within the message
@autocenter = true
# whether or not event-positioned messages have a tail (for speech bubbles)
# (only works if floating and resized messages enabled -- otherwise would
# look very odd indeed)
@show_tail = true
# whether or not to display "waiting for user input" pause graphic
# (probably want this disabled for speech bubbles)
@show_pause = false
# whether the player is permitted to move while messages are displayed
@move_during = true
# the default location for floating messages (relative to the event)
# note that an off-screen message will be "flipped" automatically
@location = TOP
# name of font to use for text (any TrueType from Windows/Fonts folder)
@font_name = Font.default_name
# note that you can use an array of fonts to specify multiple
# e.g. ['Verdana', 'MS PGothic']
# (if Verdana is not available, MS PGothic will be used instead)
# font size for text (default is 22)
@font_size = Font.default_size
# default font color (same 0-7 as for \c[n])
@font_color = 0
# font color used just for thought bubbles (\@)
@font_color_thought = 1
end
end
#------------------------------------------------------------------------------
class Window_Message < Window_Selectable
def initialize(msgindex)
super(80, 304, 480, 160)
self.contents = Bitmap.new(width - 32, height - 32)
self.visible = false
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
self.z = 9000 + msgindex * 5 # permits messages to overlap legibly
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
@fade_in = false
@fade_out = false
@contents_showing = false
@cursor_width = 0
self.active = false
self.index = -1
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
@msgindex = msgindex
@tail = Sprite.new
@tail.bitmap =
if @msgindex == 0
RPG::Cache.windowskin(FILENAME_SPEECH_TAIL)
else
# don't use cached version or else all tails
# are rotated when multiple are visible at once
Bitmap.new("Graphics/Windowskins/"+FILENAME_SPEECH_TAIL)
end
# keep track of orientation of tail bitmap
if @tail.bitmap.orientation == nil
@tail.bitmap.orientation = 0
end
# make origin the center, not top left corner
@tail.ox = @tail.bitmap.width/2
@tail.oy = @tail.bitmap.height/2
@tail.z = 9999
@tail.visible = false
@windowskin = $game_system.windowskin_name
@font_color = $game_system.message.font_color
@update_text = true
@letter_by_letter = $game_system.message.letter_by_letter
@text_speed = $game_system.message.text_speed
# id of character for speech bubbles
@float_id = nil
# location of box relative to speaker
@location = $game_system.message.location
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
end
def dispose
terminate_message
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
# have to check all windows before claiming that no window is showing
if $game_temp.message_text.compact.empty?
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
$game_temp.message_window_showing = false
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
end
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
if @input_number_window != nil
@input_number_window.dispose
end
super
end
def terminate_message
self.active = false
self.pause = false
self.index = -1
self.contents.clear
# Clear showing flag
@contents_showing = false
# Clear variables related to text, choices, and number input
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
@tail.visible = false
# note that these variables are now indexed arrays
$game_temp.message_text[@msgindex] = nil
# Call message callback
if $game_temp.message_proc[@msgindex] != nil
# make sure no message boxes are displaying
if $game_temp.message_text.compact.empty?
$game_temp.message_proc[@msgindex].call
end
$game_temp.message_proc[@msgindex] = nil
end
@update_text = true
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
$game_temp.choice_start = 99
$game_temp.choice_max = 0
$game_temp.choice_cancel_type = 0
$game_temp.choice_proc = nil
$game_temp.num_input_start = 99
$game_temp.num_input_variable_id = 0
$game_temp.num_input_digits_max = 0
# Open gold window
if @gold_window != nil
@gold_window.dispose
@gold_window = nil
end
end
def refresh
self.contents.clear
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
@x = @y = 0 # now instance variables
@float_id = nil
@location = $game_system.message.location
@windowskin = $game_system.windowskin_name
@font_color = $game_system.message.font_color
@line_widths = nil
@wait_for_input = false
@tail.bitmap =
if @msgindex == 0
RPG::Cache.windowskin(FILENAME_SPEECH_TAIL)
else
Bitmap.new("Graphics/Windowskins/"+FILENAME_SPEECH_TAIL)
end
RPG::Cache.windowskin(FILENAME_SPEECH_TAIL)
@tail.bitmap.orientation = 0 if @tail.bitmap.orientation == nil
@text_speed = $game_system.message.text_speed
@letter_by_letter = $game_system.message.letter_by_letter
@delay = @text_speed
@player_skip = false
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
@cursor_width = 0
# Indent if choice
if $game_temp.choice_start == 0
@x = 8
end
# If waiting for a message to be displayed
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
if $game_temp.message_text[@msgindex] != nil
@text = $game_temp.message_text[@msgindex] # now an instance variable
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
# Control text processing
begin
last_text = @text.clone
@text.gsub!(/\\[Vv][Aa][Rr]\[([0-9]+)\]/) { $game_variables[$1.to_i] }
end until @text == last_text
@text.gsub!(/\\[Nn]\[([0-9]+)\]/) do
$game_actors[$1.to_i] != nil ? $game_actors[$1.to_i].name : ""
end
# Change "\\\\" to "\000" for convenience
@text.gsub!(/\\\\/) { "\000" }
# Change "\\C" to "\001" and "\\G" to "\002"
@text.gsub!(/\\[Cc]\[([0-9]+)\]/) { "\001[#{$1}]" }
@text.gsub!(/\\[Gg]/) { "\002" }
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
@text.gsub!(/\\[Nn]\[[Ee]([0-9]+)\]/) do
$data_enemies[$1.to_i] != nil ? $data_enemies[$1.to_i].name : ""
end
# Change "\\MAP" to map name
@text.gsub!(/\\[Mm][Aa][Pp]/) { $game_map.name }
# Change "\\L" to "\003" (toggle letter-by-letter)
@text.gsub!(/\\[Ll]/) { "\003" }
# Change "\\S" to "\004" (text speed)
@text.gsub!(/\\[Ss]\[([0-9]+)\]/) { "\004[#{$1}]" }
# Change "\\D" to "\005" (delay)
@text.gsub!(/\\[Dd]\[([0-9]+)\]/) { "\005[#{$1}]" }
# Change "\\!" to "\006" (self close)
@text.gsub!(/\\[!]/) { "\006" }
# Change "\\?" to "\007" (wait for user input)
@text.gsub!(/\\[?]/) { "\007" }
# Change "\\B" to "\010" (bold)
@text.gsub!(/\\[Bb]/) { "\010" }
# Change "\\I" to "\011" (italic)
@text.gsub!(/\\[Ii]/) { "\011" }
# Get rid of "\\@" (thought bubble)
if @text.gsub!(/\\[@]/, "") != nil
@windowskin = FILENAME_THOUGHT_WINDOWSKIN
@font_color = $game_system.message.font_color_thought
@tail.bitmap =
if @msgindex == 0
RPG::Cache.windowskin(FILENAME_THOUGHT_TAIL)
else
Bitmap.new("Graphics/Windowskins/"+FILENAME_THOUGHT_TAIL)
end
@tail.bitmap.orientation = 0 if @tail.bitmap.orientation == nil
end
# Get rid of "\\+" (multiple messages)
@text.gsub!(/\\[+]/, "")
# Get rid of "\\^", "\\v", "\\<", "\\>" (relative message location)
if @text.gsub!(/\\\^/, "") != nil
@location = 8
elsif @text.gsub!(/\\[Vv]/, "") != nil
@location = 2
elsif @text.gsub!(/\\[<]/, "") != nil
@location = 4
elsif @text.gsub!(/\\[>]/, "") != nil
@location = 6
end
# Get rid of "\\P" (position window to given character)
if @text.gsub!(/\\[Pp]\[([0-9]+)\]/, "") != nil
@float_id = $1.to_i
elsif @text.gsub!(/\\[Pp]\[([a-zA-Z])\]/, "") != nil and
$game_temp.in_battle
@float_id = $1.downcase
elsif @text.gsub!(/\\[Pp]/, "") != nil or
($game_system.message.floating and $game_system.message.resize) and
!$game_temp.in_battle
@float_id = $game_system.map_interpreter.event_id
end
if $game_system.message.resize or $game_system.message.autocenter
# calculate length of lines
text = @text.clone
temp_bitmap = Bitmap.new(1,1)
temp_bitmap.font.name = $game_system.message.font_name
temp_bitmap.font.size = $game_system.message.font_size
@line_widths = [0,0,0,0]
for i in 0..3
line = text.split(/\n/)[3-i]
if line == nil
next
end
line.gsub!(/[\001-\007](\[\w+\])?/, "")
line.chomp.split(//).each do |c|
case c
when "\000"
c = "\\"
when "\010"
# bold
temp_bitmap.font.bold = !temp_bitmap.font.bold
c = ''
when "\011"
# italics
temp_bitmap.font.italic = !temp_bitmap.font.italic
c = ''
end
@line_widths[3-i] += temp_bitmap.text_size(c).width
end
if (3-i) >= $game_temp.choice_start
# account for indenting
@line_widths[3-i] += 8 unless $game_system.message.autocenter
end
end
if $game_temp.num_input_variable_id > 0
# determine cursor_width as in Window_InputNumber
# (can't get from @input_number_window because it doesn't exist yet)
cursor_width = temp_bitmap.text_size("0").width + 8
# use this width to calculate line width (+8 for indent)
input_number_width = cursor_width*$game_temp.num_input_digits_max
input_number_width += 8 unless $game_system.message.autocenter
@line_widths[$game_temp.num_input_start] = input_number_width
end
temp_bitmap.dispose
end
resize
reposition if @float_id != nil
self.contents.font.name = $game_system.message.font_name
self.contents.font.size = $game_system.message.font_size
self.contents.font.color = text_color(@font_color)
self.windowskin = RPG::Cache.windowskin(@windowskin)
# autocenter first line if enabled
# (subsequent lines are done as "\n" is encountered)
if $game_system.message.autocenter and @text != ""
@x = (self.width-40)/2 - @line_widths[0]/2
end
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
end
end
#--------------------------------------------------------------------------
# * Resize Window
#--------------------------------------------------------------------------
def resize
if !$game_system.message.resize
# reset to defaults
self.width = 480
self.height = 160
self.contents = Bitmap.new(width - 32, height - 32)
self.x = 80 # undo any centering
return
end
max_x = @line_widths.max
max_y = 4
@line_widths.each do |line|
max_y -= 1 if line == 0 and max_y > 1
end
if $game_temp.choice_max > 0
# account for indenting
max_x += 8 unless $game_system.message.autocenter
end
self.width = max_x + 40
self.height = max_y * 32 + 32
self.contents = Bitmap.new(width - 32, height - 32)
self.x = 320 - (self.width/2) # center
end
#--------------------------------------------------------------------------
# * Reposition Window
#--------------------------------------------------------------------------
def reposition
if $game_temp.in_battle
if "abcd".include?(@float_id) # must be between a and d
@float_id = @float_id[0] - 97 # a = 0, b = 1, c = 2, d = 3
return if $scene.spriteset.actor_sprites[@float_id] == nil
sprite = $scene.spriteset.actor_sprites[@float_id]
else
@float_id -= 1 # account for, e.g., player entering 1 for index 0
return if $scene.spriteset.enemy_sprites[@float_id] == nil
sprite = $scene.spriteset.enemy_sprites[@float_id]
end
char_height = sprite.height
char_width = sprite.width
char_x = sprite.x
char_y = sprite.y - char_height/2
else # not in battle...
char = (@float_id == 0 ? $game_player : $game_map.events[@float_id])
if char == nil
# no such character
@float_id = nil
return
end
# close message (and stop event processing) if speaker is off-screen
if char.screen_x <= 0 or char.screen_x >= 640 or
char.screen_y <= 0 or char.screen_y > 480
terminate_message
$game_system.map_interpreter.command_115
return
end
char_height = RPG::Cache.character(char.character_name,0).height / 4
char_width = RPG::Cache.character(char.character_name,0).width / 4
# record coords of character's center
char_x = char.screen_x
char_y = char.screen_y - char_height/2
end
params = [char_height, char_width, char_x, char_y]
# position window and message tail
vars = new_position(params)
x = vars[0]
y = vars[1]
# check if any window locations need to be "flipped"
if @location == 4 and x < 0
# switch to right
@location = 6
vars = new_position(params)
x = vars[0]
if (x + self.width) > 640
# right is no good either...
if y >= 0
# switch to top
@location = 8
vars = new_position(params)
else
# switch to bottom
@location = 2
vars = new_position(params)
end
end
elsif @location == 6 and (x + self.width) > 640
# switch to left
@location = 4
vars = new_position(params)
x = vars[0]
if x < 0
# left is no good either...
if y >= 0
# switch to top
@location = 8
vars = new_position(params)
else
# switch to bottom
@location = 2
vars = new_position(params)
end
end
elsif @location == 8 and y < 0
# switch to bottom
@location = 2
vars = new_position(params)
y = vars[1]
if (y + self.height) > 480
# bottom is no good either...
# note: this will probably never occur given only 3 lines of text
x = vars[0]
if x >= 0
# switch to left
@location = 4
vars = new_position(params)
else
# switch to right
@location = 6
vars = new_position(params)
end
end
elsif @location == 2 and (y + self.height) > 480
# switch to top
@location = 8
vars = new_position(params)
y = vars[1]
if y < 0
# top is no good either...
# note: this will probably never occur given only 3 lines of text
x = vars[0]
if x >= 0
# switch to left
@location = 4
vars = new_position(params)
else
# switch to right
@location = 6
vars = new_position(params)
end
end
end
x = vars[0]
y = vars[1]
tail_x = vars[2]
tail_y = vars[3]
# adjust windows if near edge of screen
if x < 0
x = 0
elsif (x + self.width) > 640
x = 640 - self.width
end
if y < 0
y = 0
elsif (y + self.height) > 480
y = 480 - self.height
elsif $game_temp.in_battle and @location == 2 and (y > (320 - self.height))
# when in battle, prevent enemy messages from overlapping battle status
# (note that it could still happen from actor messages, though)
y = 320 - self.height
tail_y = y
end
# finalize positions
self.x = x
self.y = y
@tail.x = tail_x
@tail.y = tail_y
end
#--------------------------------------------------------------------------
# * Determine New Window Position
#--------------------------------------------------------------------------
def new_position(params)
char_height = params[0]
char_width = params[1]
char_x = params[2]
char_y = params[3]
if @location == 8
# top
x = char_x - self.width/2
y = char_y - char_height/2 - self.height - @tail.bitmap.height/2
@tail.bitmap.rotation(0)
tail_x = x + self.width/2
tail_y = y + self.height
elsif @location == 2
# bottom
x = char_x - self.width/2
y = char_y + char_height/2 + @tail.bitmap.height/2
@tail.bitmap.rotation(180)
tail_x = x + self.width/2
tail_y = y
elsif @location == 4
# left
x = char_x - char_width/2 - self.width - @tail.bitmap.width/2
y = char_y - self.height/2
@tail.bitmap.rotation(270)
tail_x = x + self.width
tail_y = y + self.height/2
elsif @location == 6
# right
x = char_x + char_width/2 + @tail.bitmap.width/2
y = char_y - self.height/2
@tail.bitmap.rotation(90)
tail_x = x
tail_y = y + self.height/2
end
return [x,y,tail_x,tail_y]
end
#--------------------------------------------------------------------------
# * Update Text
#--------------------------------------------------------------------------
def update_text
if @text != nil
# Get 1 text character in c (loop until unable to get text)
while ((c = @text.slice!(/./m)) != nil)
# If \\
if c == "\000"
# Return to original text
c = "\\"
end
# If \C[n]
if c == "\001"
# Change text color
@text.sub!(/\[([0-9]+)\]/, "")
color = $1.to_i
if color >= 0 and color <= 7
self.contents.font.color = text_color(color)
end
# go to next text
next
end
# If \G
if c == "\002"
# Make gold window
if @gold_window == nil
@gold_window = Window_Gold.new
@gold_window.x = 560 - @gold_window.width
if $game_temp.in_battle
@gold_window.y = 192
else
@gold_window.y = self.y >= 128 ? 32 : 384
end
@gold_window.opacity = self.opacity
@gold_window.back_opacity = self.back_opacity
end
# go to next text
next
end
# If \L
if c == "\003"
# toggle letter-by-letter mode
@letter_by_letter = !@letter_by_letter
# go to next text
next
end
# If \S[n]
if c == "\004"
@text.sub!(/\[([0-9]+)\]/, "")
speed = $1.to_i
if speed >= 0
@text_speed = speed
# reset player skip after text speed change
@player_skip = false
end
return
end
# If \D[n]
if c == "\005"
@text.sub!(/\[([0-9]+)\]/, "")
delay = $1.to_i
if delay >= 0
@delay += delay
# reset player skip after delay
@player_skip = false
end
return
end
# If \!
if c == "\006"
# close message and return from method
terminate_message
return
end
# If \?
if c == "\007"
@wait_for_input = true
return
end
if c == "\010"
# bold
self.contents.font.bold = !self.contents.font.bold
end
if c == "\011"
# italics
self.contents.font.italic = !self.contents.font.italic
end
# If new line text
if c == "\n"
# Update cursor width if choice
if @y >= $game_temp.choice_start
width = $game_system.message.autocenter ? @line_widths[@y]+8 : @x
@cursor_width = [@cursor_width, width].max
end
# Add 1 to y
@y += 1
if $game_system.message.autocenter and @text != ""
@x = (self.width-40)/2 - @line_widths[@y]/2
else
@x = 0
# Indent if choice
if @y >= $game_temp.choice_start
@x = 8
end
end
# go to next text
next
end
# Draw text
self.contents.draw_text(4 + @x, 32 * @y, 40, 32, c)
# Add x to drawn text width
@x += self.contents.text_size( c ).width
# add text speed to time to display next character
@delay += @text_speed unless !@letter_by_letter or @player_skip
return if @letter_by_letter and !@player_skip
end
end
# If choice
if $game_temp.choice_max > 0
@item_max = $game_temp.choice_max
self.active = true
self.index = 0
end
# If number input
if $game_temp.num_input_variable_id > 0
digits_max = $game_temp.num_input_digits_max
number = $game_variables[$game_temp.num_input_variable_id]
@input_number_window = Window_InputNumber.new(digits_max)
@input_number_window.number = number
@input_number_window.x =
if $game_system.message.autocenter
offset = (self.width-40)/2-@line_widths[$game_temp.num_input_start]/2
self.x + offset + 4
else
self.x + 8
end
@input_number_window.y = self.y + $game_temp.num_input_start * 32
end
@update_text = false
end
#--------------------------------------------------------------------------
# * Set Window Position and Opacity Level
#--------------------------------------------------------------------------
def reset_window
if $game_temp.in_battle
self.y = 16
else
case $game_system.message_position
when 0 # up
self.y = 16
when 1 # middle
self.y = 160
when 2 # down
self.y = 304
end
end
if $game_system.message_frame == 0
self.opacity = 255
else
self.opacity = 0
end
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
# transparent speech bubbles don't look right, so keep opacity at 255
# self.back_opacity = 160
@tail.opacity = 255
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
end
def update
super
# If fade in
if @fade_in
self.contents_opacity += 24
if @input_number_window != nil
@input_number_window.contents_opacity += 24
end
if self.contents_opacity == 255
@fade_in = false
end
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
# return
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
end
# If inputting number
if @input_number_window != nil
@input_number_window.update
# Confirm
if Input.trigger?(Input::C)
$game_system.se_play($data_system.decision_se)
$game_variables[$game_temp.num_input_variable_id] =
@input_number_window.number
$game_map.need_refresh = true
# Dispose of number input window
@input_number_window.dispose
@input_number_window = nil
terminate_message
end
return
end
# If message is being displayed
if @contents_showing
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
# Confirm or cancel finishes waiting for input or message
if Input.trigger?(Input::C) or Input.trigger?(Input::B)
if @wait_for_input
@wait_for_input = false
self.pause = false
elsif $game_system.message.skippable
@player_skip = true
end
end
if need_reposition?
reposition # update message position for character/screen movement
if @contents_showing == false
# i.e. if char moved off screen
return
end
end
if @update_text and !@wait_for_input
if @delay == 0
update_text
else
@delay -= 1
end
return
end
# If choice isn't being displayed, show pause sign
if !self.pause and ($game_temp.choice_max == 0 or @wait_for_input)
self.pause = true unless !$game_system.message.show_pause
end
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
# Cancel
if Input.trigger?(Input::B)
if $game_temp.choice_max > 0 and $game_temp.choice_cancel_type > 0
$game_system.se_play($data_system.cancel_se)
$game_temp.choice_proc.call($game_temp.choice_cancel_type - 1)
terminate_message
end
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
# personal preference: cancel button should also continue
terminate_message
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
end
# Confirm
if Input.trigger?(Input::C)
if $game_temp.choice_max > 0
$game_system.se_play($data_system.decision_se)
$game_temp.choice_proc.call(self.index)
end
terminate_message
end
return
end
# If display wait message or choice exists when not fading out
if @fade_out == false and $game_temp.message_text[@msgindex] != nil
@contents_showing = true
$game_temp.message_window_showing = true
reset_window
refresh
Graphics.frame_reset
self.visible = true
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
if show_message_tail?
@tail.visible = true
elsif @tail.visible
@tail.visible = false
end
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
self.contents_opacity = 0
if @input_number_window != nil
@input_number_window.contents_opacity = 0
end
@fade_in = true
return
end
# If message which should be displayed is not shown, but window is visible
if self.visible
@fade_out = true
self.opacity -= 48
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
@tail.opacity -= 48 if @tail.opacity > 0
if need_reposition?
reposition # update message position for character/screen movement
end
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
if self.opacity == 0
self.visible = false
@fade_out = false
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
@tail.visible = false if @tail.visible
# have to check all windows before claiming that no window is showing
if $game_temp.message_text.compact.empty?
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
$game_temp.message_window_showing = false
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
end
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
end
return
end
end
#--------------------------------------------------------------------------
# * Repositioning Determination
#--------------------------------------------------------------------------
def need_reposition?
if !$game_temp.in_battle and $game_system.message.floating and
$game_system.message.resize and @float_id != nil
if $game_system.message.move_during and @float_id == 0 and
(($game_player.last_real_x != $game_player.real_x) or
($game_player.last_real_y != $game_player.real_y))
# player with floating message moved
# (note that relying on moving? leads to "jumpy" message boxes)
return true
elsif ($game_map.last_display_y != $game_map.display_y) or
($game_map.last_display_x != $game_map.display_x)
# player movement or scroll event caused the screen to scroll
return true
else
char = $game_map.events[@float_id]
if char != nil and
((char.last_real_x != char.real_x) or
(char.last_real_y != char.real_y))
# character moved
return true
end
end
end
return false
end
#--------------------------------------------------------------------------
# * Show Message Tail Determination
#--------------------------------------------------------------------------
def show_message_tail?
if $game_system.message.show_tail and $game_system.message.floating and
$game_system.message.resize and $game_system.message_frame == 0 and
@float_id != nil
return true
end
return false
end
def update_cursor_rect
if @index >= 0
n = $game_temp.choice_start + @index
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
if $game_system.message.autocenter
x = 4 + (self.width-40)/2 - @cursor_width/2
else
x = 8
end
self.cursor_rect.set(x, n * 32, @cursor_width, 32)
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
else
self.cursor_rect.empty
end
end
end
#------------------------------------------------------------------------------
class Game_Character
attr_reader :last_real_x # last map x-coordinate
attr_reader :last_real_y # last map y-coordinate
alias wachunga_game_char_update update
def update
@last_real_x = @real_x
@last_real_y = @real_y
wachunga_game_char_update
end
end
#------------------------------------------------------------------------------
class Game_Player < Game_Character
alias wachunga_mmw_game_player_update update
def update
# The conditions are changed so the player can move around while messages
# are showing (if move_during is true), but not if user is making a
# choice or inputting a number
# Note that this check overrides the default one (later in the method)
# because it is more general
unless moving? or
@move_route_forcing or
($game_system.map_interpreter.running? and
!$game_temp.message_window_showing) or
($game_temp.message_window_showing and
!$game_system.message.move_during) or
($game_temp.choice_max > 0 or $game_temp.num_input_digits_max > 0)
case Input.dir4
when 2
move_down
when 4
move_left
when 6
move_right
when 8
move_up
end
end
wachunga_mmw_game_player_update
end
end
#------------------------------------------------------------------------------
class Game_Temp
alias wachunga_mmw_game_temp_initialize initialize
def initialize
wachunga_mmw_game_temp_initialize
@message_text = []
@message_proc = []
end
end
#------------------------------------------------------------------------------
class Sprite_Battler < RPG::Sprite
# necessary for positioning messages relative to battlers
attr_reader :height
attr_reader :width
end
#------------------------------------------------------------------------------
class Scene_Battle
# necessary for accessing actor/enemy sprites in battle
attr_reader :spriteset
end
#------------------------------------------------------------------------------
class Spriteset_Battle
# necessary for accessing actor/enemy sprites in battle
attr_reader :actor_sprites
attr_reader :enemy_sprites
end
#------------------------------------------------------------------------------
class Scene_Map
# can't alias these methods unfortunately
# (SDK would help compatibility a little)
def main
# Make sprite set
@spriteset = Spriteset_Map.new
# Make message window
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
@message_window = []
@message_window[0] = Window_Message.new(0)
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
# Transition run
Graphics.transition
# Main loop
loop do
# Update game screen
Graphics.update
# Update input information
Input.update
# Frame update
update
# Abort loop if screen is changed
if $scene != self
break
end
end
# Prepare for transition
Graphics.freeze
# Dispose of sprite set
@spriteset.dispose
# Dispose of message window
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
for mw in @message_window
mw.dispose
end
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
# If switching to title screen
if $scene.is_a?(Scene_Title)
# Fade out screen
Graphics.transition
Graphics.freeze
end
end
def update
# Loop
loop do
# Update map, interpreter, and player order
# (this update order is important for when conditions are fulfilled
# to run any event, and the player isn't provided the opportunity to
# move in an instant)
$game_map.update
$game_system.map_interpreter.update
$game_player.update
# Update system (timer), screen
$game_system.update
$game_screen.update
# Abort loop if player isn't place moving
unless $game_temp.player_transferring
break
end
# Run place move
transfer_player
# Abort loop if transition processing
if $game_temp.transition_processing
break
end
end
# Update sprite set
@spriteset.update
# Update message window
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
for mw in @message_window
mw.update
end
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
# If game over
if $game_temp.gameover
# Switch to game over screen
$scene = Scene_Gameover.new
return
end
# If returning to title screen
if $game_temp.to_title
# Change to title screen
$scene = Scene_Title.new
return
end
# If transition processing
if $game_temp.transition_processing
# Clear transition processing flag
$game_temp.transition_processing = false
# Execute transition
if $game_temp.transition_name == ""
Graphics.transition(20)
else
Graphics.transition(40, "Graphics/Transitions/" +
$game_temp.transition_name)
end
end
# If showing message window
if $game_temp.message_window_showing
return
end
# If encounter list isn't empty, and encounter count is 0
if $game_player.encounter_count == 0 and $game_map.encounter_list != []
# If event is running or encounter is not forbidden
unless $game_system.map_interpreter.running? or
$game_system.encounter_disabled
# Confirm troop
n = rand($game_map.encounter_list.size)
troop_id = $game_map.encounter_list[n]
# If troop is valid
if $data_troops[troop_id] != nil
# Set battle calling flag
$game_temp.battle_calling = true
$game_temp.battle_troop_id = troop_id
$game_temp.battle_can_escape = true
$game_temp.battle_can_lose = false
$game_temp.battle_proc = nil
end
end
end
# If B button was pressed
if Input.trigger?(Input::B)
# If event is running, or menu is not forbidden
unless $game_system.map_interpreter.running? or
$game_system.menu_disabled
# Set menu calling flag or beep flag
$game_temp.menu_calling = true
$game_temp.menu_beep = true
end
end
# If debug mode is ON and F9 key was pressed
if $DEBUG and Input.press?(Input::F9)
# Set debug calling flag
$game_temp.debug_calling = true
end
# If player is not moving
unless $game_player.moving?
# Run calling of each screen
if $game_temp.battle_calling
call_battle
elsif $game_temp.shop_calling
call_shop
elsif $game_temp.name_calling
call_name
elsif $game_temp.menu_calling
call_menu
elsif $game_temp.save_calling
call_save
elsif $game_temp.debug_calling
call_debug
end
end
end
#--------------------------------------------------------------------------
# * New Message Window Addition
#--------------------------------------------------------------------------
def new_message_window(index)
if @message_window[index] != nil
# clear message windows at and after this index
last_index = @message_window.size - 1
last_index.downto(index) do |i|
if @message_window[i] != nil
@message_window[i].dispose
@message_window[i] = nil
end
end
@message_window.compact!
end
@message_window.push(Window_Message.new(index))
end
end
#------------------------------------------------------------------------------
class Scene_Battle
# can't alias these methods unfortunately
# (SDK would help compatibility a little)
def main
# Initialize each kind of temporary battle data
$game_temp.in_battle = true
$game_temp.battle_turn = 0
$game_temp.battle_event_flags.clear
$game_temp.battle_abort = false
$game_temp.battle_main_phase = false
$game_temp.battleback_name = $game_map.battleback_name
$game_temp.forcing_battler = nil
# Initialize battle event interpreter
$game_system.battle_interpreter.setup(nil, 0)
# Prepare troop
@troop_id = $game_temp.battle_troop_id
$game_troop.setup(@troop_id)
# Make actor command window
s1 = $data_system.words.attack
s2 = $data_system.words.skill
s3 = $data_system.words.guard
s4 = $data_system.words.item
@actor_command_window = Window_Command.new(160, [s1, s2, s3, s4])
@actor_command_window.y = 160
@actor_command_window.back_opacity = 160
@actor_command_window.active = false
@actor_command_window.visible = false
# Make other windows
@party_command_window = Window_PartyCommand.new
@help_window = Window_Help.new
@help_window.back_opacity = 160
@help_window.visible = false
@status_window = Window_BattleStatus.new
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
@message_window = []
@message_window[0] = Window_Message.new(0)
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
# Make sprite set
@spriteset = Spriteset_Battle.new
# Initialize wait count
@wait_count = 0
# Execute transition
if $data_system.battle_transition == ""
Graphics.transition(20)
else
Graphics.transition(40, "Graphics/Transitions/" +
$data_system.battle_transition)
end
# Start pre-battle phase
start_phase1
# Main loop
loop do
# Update game screen
Graphics.update
# Update input information
Input.update
# Frame update
update
# Abort loop if screen is changed
if $scene != self
break
end
end
# Refresh map
$game_map.refresh
# Prepare for transition
Graphics.freeze
# Dispose of windows
@actor_command_window.dispose
@party_command_window.dispose
@help_window.dispose
@status_window.dispose
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
for mw in @message_window
mw.dispose
end
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
if @skill_window != nil
@skill_window.dispose
end
if @item_window != nil
@item_window.dispose
end
if @result_window != nil
@result_window.dispose
end
# Dispose of sprite set
@spriteset.dispose
# If switching to title screen
if $scene.is_a?(Scene_Title)
# Fade out screen
Graphics.transition
Graphics.freeze
end
# If switching from battle test to any screen other than game over screen
if $BTEST and not $scene.is_a?(Scene_Gameover)
$scene = nil
end
end
def update
# If battle event is running
if $game_system.battle_interpreter.running?
# Update interpreter
$game_system.battle_interpreter.update
# If a battler which is forcing actions doesn't exist
if $game_temp.forcing_battler == nil
# If battle event has finished running
unless $game_system.battle_interpreter.running?
# Rerun battle event set up if battle continues
unless judge
setup_battle_event
end
end
# If not after battle phase
if @phase != 5
# Refresh status window
@status_window.refresh
end
end
end
# Update system (timer) and screen
$game_system.update
$game_screen.update
# If timer has reached 0
if $game_system.timer_working and $game_system.timer == 0
# Abort battle
$game_temp.battle_abort = true
end
# Update windows
@help_window.update
@party_command_window.update
@actor_command_window.update
@status_window.update
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
for mw in @message_window
mw.update
end
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
# Update sprite set
@spriteset.update
# If transition is processing
if $game_temp.transition_processing
# Clear transition processing flag
$game_temp.transition_processing = false
# Execute transition
if $game_temp.transition_name == ""
Graphics.transition(20)
else
Graphics.transition(40, "Graphics/Transitions/" +
$game_temp.transition_name)
end
end
# If message window is showing
if $game_temp.message_window_showing
return
end
# If effect is showing
if @spriteset.effect?
return
end
# If game over
if $game_temp.gameover
# Switch to game over screen
$scene = Scene_Gameover.new
return
end
# If returning to title screen
if $game_temp.to_title
# Switch to title screen
$scene = Scene_Title.new
return
end
# If battle is aborted
if $game_temp.battle_abort
# Return to BGM used before battle started
$game_system.bgm_play($game_temp.map_bgm)
# Battle ends
battle_end(1)
return
end
# If waiting
if @wait_count > 0
# Decrease wait count
@wait_count -= 1
return
end
# If battler forcing an action doesn't exist,
# and battle event is running
if $game_temp.forcing_battler == nil and
$game_system.battle_interpreter.running?
return
end
# Branch according to phase
case @phase
when 1 # pre-battle phase
update_phase1
when 2 # party command phase
update_phase2
when 3 # actor command phase
update_phase3
when 4 # main phase
update_phase4
when 5 # after battle phase
update_phase5
end
end
#--------------------------------------------------------------------------
# * New Message Window Addition
#--------------------------------------------------------------------------
def new_message_window(index)
if @message_window[index] != nil
# clear message windows at and after this index
last_index = @message_window.size - 1
last_index.downto(index) do |i|
if @message_window[i] != nil
@message_window[i].dispose
@message_window[i] = nil
end
end
@message_window.compact!
end
@message_window.push(Window_Message.new(index))
end
end
#------------------------------------------------------------------------------
class Game_System
attr_reader :message
alias wachunga_mmw_game_system_init initialize
def initialize
wachunga_mmw_game_system_init
@message = Game_Message.new
end
end
#------------------------------------------------------------------------------
class Interpreter
attr_reader :event_id
alias wachunga_mmw_interp_setup setup
def setup(list, event_id)
wachunga_mmw_interp_setup(list, event_id)
# index of window for the message
@msgindex = 0
# whether multiple messages are displaying
@multi_message = false
end
def setup_choices(parameters)
# Set choice item count to choice_max
$game_temp.choice_max = parameters[0].size
# Set choice to message_text
for text in parameters[0]
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
# just add index for array
$game_temp.message_text[@msgindex] += text + "\n"
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
end
# Set cancel processing
$game_temp.choice_cancel_type = parameters[1]
# Set callback
current_indent = @list[@index].indent
$game_temp.choice_proc = Proc.new { |n| @branch[current_indent] = n }
end
#--------------------------------------------------------------------------
# * Show Text
#--------------------------------------------------------------------------
def command_101
# If other text has been set to message_text
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
if $game_temp.message_text[@msgindex] != nil
if @multi_message
@msgindex += 1
$scene.new_message_window(@msgindex)
else
# End
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
return false
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
end
end
@msgindex = 0 if !@multi_message
@multi_message = false
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
# Set message end waiting flag and callback
@message_waiting = true
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
# just adding indexes
$game_temp.message_proc[@msgindex] = Proc.new { @message_waiting = false }
# Set message text on first line
$game_temp.message_text[@msgindex] = @list[@index].parameters[0] + "\n"
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
line_count = 1
# Loop
loop do
# If next event command text is on the second line or after
if @list[@index+1].code == 401
# Add the second line or after to message_text
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
# just adding index
$game_temp.message_text[@msgindex]+=@list[@index+1].parameters[0]+"\n"
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
line_count += 1
# If event command is not on the second line or after
else
# If next event command is show choices
if @list[@index+1].code == 102
# If choices fit on screen
if @list[@index+1].parameters[0].size <= 4 - line_count
# Advance index
@index += 1
# Choices setup
$game_temp.choice_start = line_count
setup_choices(@list[@index].parameters)
end
# If next event command is input number
elsif @list[@index+1].code == 103
# If number input window fits on screen
if line_count < 4
# Advance index
@index += 1
# Number input setup
$game_temp.num_input_start = line_count
$game_temp.num_input_variable_id = @list[@index].parameters[0]
$game_temp.num_input_digits_max = @list[@index].parameters[1]
end
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
# start multimessage if next line is "Show Text" starting with "\\+"
elsif @list[@index+1].code == 101
if @list[@index+1].parameters[0][0..1]=="\\+"
@multi_message = true
@message_waiting = false
end
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
end
# Continue
return true
end
# Advance index
@index += 1
end
end
#--------------------------------------------------------------------------
# * Show Choices
#--------------------------------------------------------------------------
def command_102
# If text has been set to message_text
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
# just adding index
if $game_temp.message_text[@msgindex] != nil
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
# End
return false
end
# Set message end waiting flag and callback
@message_waiting = true
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
# adding more indexes
$game_temp.message_proc[@msgindex] = Proc.new { @message_waiting = false }
# Choices setup
$game_temp.message_text[@msgindex] = ""
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
$game_temp.choice_start = 0
setup_choices(@parameters)
# Continue
return true
end
#--------------------------------------------------------------------------
# * Input Number
#--------------------------------------------------------------------------
def command_103
# If text has been set to message_text
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
# just adding index
if $game_temp.message_text[@msgindex] != nil
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
# End
return false
end
# Set message end waiting flag and callback
@message_waiting = true
#------------------------------------------------------------------------------
# Begin Multiple Message Windows Edit
#------------------------------------------------------------------------------
# adding more indexes
$game_temp.message_proc[@msgindex] = Proc.new { @message_waiting = false }
# Number input setup
$game_temp.message_text[@msgindex] = ""
#------------------------------------------------------------------------------
# End Multiple Message Windows Edit
#------------------------------------------------------------------------------
$game_temp.num_input_start = 0
$game_temp.num_input_variable_id = @parameters[0]
$game_temp.num_input_digits_max = @parameters[1]
# Continue
return true
end
#--------------------------------------------------------------------------
# * Script
#--------------------------------------------------------------------------
# Fix for RMXP bug: call script boxes that return false hang the game
# See, e.g., [url=http://rmxp.org/forums/showthread.php?p=106639]http://rmxp.org/forums/showthread.php?p=106639[/url]
#--------------------------------------------------------------------------
def command_355
# Set first line to script
script = @list[@index].parameters[0] + "\n"
# Loop
loop do
# If next event command is second line of script or after
if @list[@index+1].code == 655
# Add second line or after to script
script += @list[@index+1].parameters[0] + "\n"
# If event command is not second line or after
else
# Abort loop
break
end
# Advance index
@index += 1
end
# Evaluation
result = eval(script)
# If return value is false
if result == false
# End
#------------------------------------------------------------------------------
# Begin Edit
#------------------------------------------------------------------------------
#return false
#------------------------------------------------------------------------------
# End Edit
#------------------------------------------------------------------------------
end
# Continue
return true
end
def message
$game_system.message
end
end
#------------------------------------------------------------------------------
class Game_Map
attr_accessor :last_display_x # last display x-coord * 128
attr_accessor :last_display_y # last display y-coord * 128
alias wachunga_mmw_game_map_update update
def update
@last_display_x = @display_x
@last_display_y = @display_y
wachunga_mmw_game_map_update
end
def name
return load_data('Data/MapInfos.rxdata')[@map_id].name
end
end
#------------------------------------------------------------------------------
class Bitmap
attr_accessor :orientation
#--------------------------------------------------------------------------
# * Rotation Calculation
#--------------------------------------------------------------------------
def rotation(target)
return if not [0, 90, 180, 270].include?(target) # invalid orientation
if @rotation != target
degrees = target - @orientation
if degrees < 0
degrees += 360
end
rotate(degrees)
end
end
#--------------------------------------------------------------------------
# * Rotate Square (Clockwise)
#--------------------------------------------------------------------------
def rotate(degrees = 90)
# method originally by SephirothSpawn
# would just use Sprite.angle but its rotation is buggy
# (see [url=http://www.rmxp.org/forums/showthread.php?t=12044]http://www.rmxp.org/forums/showthread.php?t=12044[/url])
return if not [90, 180, 270].include?(degrees)
copy = self.clone
if degrees == 90
# Passes Through all Pixels on Dummy Bitmap
for i in 0...self.height
for j in 0...self.width
self.set_pixel(width - i - 1, j, copy.get_pixel(j, i))
end
end
elsif degrees == 180
for i in 0...self.height
for j in 0...self.width
self.set_pixel(width - j - 1, height - i - 1, copy.get_pixel(j, i))
end
end
elsif degrees == 270
for i in 0...self.height
for j in 0...self.width
self.set_pixel(i, height - j - 1, copy.get_pixel(j, i))
end
end
end
@orientation = (@orientation + degrees) % 360
end
end
But I find that it conflicts with Enu/Atoa's SBS
Code:
#==============================================================================
# Sideview Battle System Configurations Version 2.2xp
#==============================================================================
# Original Script by:
# Enu ([url=http://rpgex.sakura.ne.jp/home/]http://rpgex.sakura.ne.jp/home/[/url])
# Conversion to XP by:
# Atoa
# Original translation versions by:
# Kylock
# Translation continued by:
# Mr. Bubble
# XP version Translation by:
# cairn
# Special thanks:
# Shu (for translation help)
# Moonlight (for her passionate bug support for this script)
# NightWalker (for his community support for this script)
# XRXS (for the script of damage gravity, which was modified and
# used as the system's base of damage exibition)
# Squall (for the FF styled damage script, which was modified
# and added to the damage exibition system)
# KGC (for the STBreaker script, which was the base for the
# attribute limit system)
# Herena Isaberu (for her support in XP version bug fixes)
# Enu (for making an awesome battle system)
#==============================================================================
#==============================================================================
# ■ module N01
#------------------------------------------------------------------------------
# Sideview Battle System Config
#==============================================================================
module N01
#--------------------------------------------------------------------------
# ● Settings
#--------------------------------------------------------------------------
# Battle member starting positions
# X Y X Y X Y X Y
ACTOR_POSITION = [[230,280],[130,290],[180,200],[220,270]]
# Maximum party members that can fight at the same time.
# Remember to add/remove coordinates in ACTOR_POSITION if you adjust
# the MAX_MEMBER value.
MAX_MEMBER = 4
# Delay time after a battler completes an action in frames.
ACTION_WAIT = 6
# Delay time before enemy collapse (defeat of enemy) animation in frames.
COLLAPSE_WAIT = 6
# Delay before victory is processed in frames.
WIN_WAIT = 30
# Animation ID for any unarmed attack.
NO_WEAPON = 4
# Damage modifications when using two weapons. Values are percentages.
# 1st Wpn, 2nd Wpn
TWO_SWORDS_STYLE = [100,50]
# Auto-Life State: Revivial Animation ID
RESURRECTION = 25
# POP Window indicator words. For no word results, use "".
POP_MISS = "Miss" # Attack missed
POP_EVA = "Evade" # Attack avoided
POP_CRI = "Crit" # Attack scored a critical hit
# Set to false to remove shadow under actors
SHADOW = false
# true: Use actor's walking graphic.
# false: Don't use actor's walking graphic.
# If false, battler file with "_1" is required since walking file is not used.
# "_1" and subsequent files ("_2", "_3", etc.) should be uniform in size.
WALK_ANIME = false
# Number of frames in a battler animation file. (horizontal frames)
ANIME_PATTERN = 4
# Number of types of battler animation file. (vertical frames)
ANIME_KIND = 1
#==============================================================================
# ■ Single Action Engine
#------------------------------------------------------------------------------
# These are utilized by sequenced actions and have no utility alone.
#==============================================================================
# A single-action cannot be used by itself unless it is used as part of a
# sequence.
ANIME = {
#--------------------------------------------------------------------------
# ● Battler Animations
#--------------------------------------------------------------------------
# No. - Battler graphic file used.
# 0: Normal Battler Graphic. In the case of Actors, 0 refers to
# default walking graphic.
# n: "Character Name + _n", where n refers to the file number
# extension. An example file would be "$Ralph_1". These
# files are placed in the Characters folder.
# Use "1" for non-standard battler, like Minkoff's.
#
# Row - Vertical position (row) of cells in battler graphic file. (0~3)
# Speed - Refresh rate of animation. Lower numbers are faster.
# Loop - [0: "Round-Trip" Loop] Example: 1 2 3 2 1 2 3 2 1 ...
# [1: "One-Way" Loop] Example: 1 2 3 1 2 3 1 2 3 ...
# [2: One Loop, no repeat] Example: 1 2 3 .
# Wait - Time, in frames, before animation loops again.
# Does not apply if Loop=2
# Fixed - Defines loop behavior or specific fixed cell display.
# -2: Reverse Loop Animation
# -1: Normal Loop Animation
# 0: No Loop Animation
# 0~3: Fixed cell display. Refers to cell on character sprite
# sheet starting where 0 = left-most cell.
# Z - Set battler's Z priority.
# Shadow - Set true to display battler shadow during animation; false to hide.
# Weapon - Weapon animation to play with battler animation. For no weapon
# animation, use "".
# Action Name No. Row Speed Loop Wait Fixed Z Shadow Weapon
"WAIT" => [ 1, 0, 15, 1, 0, -1, 0, true,"" ],
"WAIT(FIXED)" => [ 1, 0, 10, 1, 0, -1, 0, true,"" ],
"RIGHT(FIXED)" => [ 1, 0, 10, 1, 0, -1, 0, true,"" ],
"DAMAGE" => [ 1, 0, 4, 1, 0, 0, 0, true,"" ],
"ATTACK_FAIL" => [ 1, 0, 10, 1, 0, 1, 0, true,"" ],
"MOVE_TO" => [ 1, 0, 10, 1, 0, -1, 0, true,"" ],
"MOVE_AWAY" => [ 1, 0, 8, 1, 0, -1, 0, true,"" ],
"ABOVE_DISPLAY" => [ 1, 0, 2, 1, 0, -1, 600, true,"" ],
"WPN_SWING_V" => [ 1, 0, 10, 1, 0, -1, 2, true,"VERT_SWING"],
"WPN_SWING_VL" => [ 1, 0, 10, 1, 0, -1, 2, true,"VERT_SWINGL"],
"WPN_SWING_VS" => [ 1, 0, 10, 1, 0, -1, 2, true,"VERT_SWING"],
"WPN_SWING_UNDER" => [ 1, 0, 10, 1, 0, -1, 2, true,"UNDER_SWING"],
"WPN_SWING_OVER" => [ 1, 0, 10, 1, 0, -1, 2, true,"OVER_SWING"],
"WPN_RAISED" => [ 1, 0, 10, 1, 0, -1, 2, true,"RAISED"],
#--------------------------------------------------------------------------
# ● Weapon Animations
#--------------------------------------------------------------------------
# Weapon Animations can only be used with Battler Animations as seen
# and defined above.
#
# Xa - Distance weapon sprite is moved on the X-axis.
# Ya - Distance weapon sprite is moved on the Y-axis. Please note that
# the Y-axis is inverted. This means negative values move up, positive
# values move down.
# Za - If true, weapon sprite is displayed over battler sprite.
# If false, weapon sprite is displayed behind battler sprite.
# A1 - Starting angle of weapon sprite rotation. Negative numbers will
# result in counter-clockwise rotation.
# A2 - Ending angle of weapon sprite rotation. Rotation will stop here.
# Or - Rotation Origin - [0: Center] [1: Upper Left] [2: Upper Right]
# [3:Bottom Left] [4:Bottom Right]
# Inv - Invert - If true, horizontally inverts weapon sprite.
# Xs - X scale - Stretches weapon sprite horizontally by a factor of X.
# Values may be decimals. (0.6, 0.9, etc.)
# Ys - Y scale - Stretches weapon sprite vertically by a factor of Y.
# Values may be decimals. (0.6, 0.9, etc.)
# Xp - X pitch - For adjusting the X axis. This number changes the initial
# X coordinate.
# Yp - Y pitch - For adjusting the Y axis. This number changes the initial
# Y coordinate.
# Weapon2 - If set to true, Two Weapon Style's Weapon 2 sprite will be used
# instead.
# Action Name Xa Ya Za A1 A2 Or Inv Xs Ys Xp Yp Weapon2
"VERT_SWING" => [ 6, 8,false,-135, 45, 4,false, 1, 1, -6, -12,false],
"VERT_SWINGL" => [ 6, 8,false,-135, 45, 4,false, 1, 1, -6, -12, true],
"UNDER_SWING" => [ 6, 8,false, 270, 45, 4,false, 1, 1, -6, -12,false],
"OVER_SWING" => [ 6, 8,false, 45,-100, 4,false, 1, 1, -6, -12,false],
"RAISED" => [ 6, -4,false, 90, -45, 4,false, 1, 1, -6, -12,false],
#--------------------------------------------------------------------------
# ● Battler Movements
#--------------------------------------------------------------------------
# Origin - Defines the origin of movement based on an (x,y) coordinate plane.
# 1 unit = 1 pixel
# [0: Battler's Current Position]
# [1: Battler's Selected Target]
# [2: Screen; (0,0) is at upper-left of screen]
# [3: Battle Start Position]
# X - X-axis pixels from origin.
# Y - Y-axis pixels from origin. Please note that the Y-axis is
# inverted. This means negative values move up, positive values move down.
# Time - Travel time. Larger numbers are slower. The distance is
# divided by one frame of movement.
# Accel - Positive values accelerates frames. Negative values decelerates.
# Jump - Negative values produce a jumping arc. Positive values produce
# a reverse arc. [0: No jump]
# Animation - Battler Animation utilized during movement.
# Origin X Y Time Accel Jump Animation
"NO_MOVE" => [ 0, 0, 0, 1, 0, 0, "WAIT(FIXED)"],
"START_POSITION" => [ 0, 54, 0, 1, 0, 0, "MOVE_TO"],
"BEFORE_MOVE" => [ 3, 32, 0, 18, -1, 0, "MOVE_TO"],
"AFTER_MOVE" => [ 0, -32, 0, 8, -1, 0, "MOVE_TO"],
"4_MAN_ATTACK_1" => [ 2, 564, 186, 12, -1, 0, "MOVE_TO"],
"4_MAN_ATTACK_2" => [ 2, 564, 212, 12, -1, 0, "MOVE_TO"],
"4_MAN_ATTACK_3" => [ 2, 504, 174, 12, -1, 0, "MOVE_TO"],
"4_MAN_ATTACK_4" => [ 2, 504, 254, 12, -1, 0, "MOVE_TO"],
"DEAL_DAMAGE" => [ 0, 32, 0, 4, -1, 0, "DAMAGE"],
"EXTRUDE" => [ 0, 12, 0, 1, 1, 0, "DAMAGE"],
"FLEE_SUCCESS" => [ 0, -300, 0,300, 1, 0, "MOVE_AWAY"],
"FLEE_FAIL" => [ 0, -48, 0, 16, 1, 0, "MOVE_AWAY"],
"VICTORY_JUMP" => [ 0, 0, 0, 20, 0, -5, "MOVE_TO"],
"MOVING_TARGET" => [ 0, 0, 0, 18, -1, 0, "MOVE_TO"],
"MOVING_TARGET_FAST" => [ 0, 0, -12, 8, 0, -2, "MOVE_TO"],
"PREV_MOVING_TARGET" => [ 0, 24, 0, 12, -1, 0, "MOVE_TO"],
"PREV_MOVING_TARGET_FAST" => [ 0, 24, 0, 1, 0, 0, "MOVE_TO"],
"MOVING_TARGET_RIGHT" => [ 0, 96, 32, 16, -1, 0, "MOVE_TO"],
"MOVING_TARGET_LEFT" => [ 0, 96, -32, 16, -1, 0, "MOVE_TO"],
"JUMP_TO" => [ 0, -32, 0, 8, -1, -4, "MOVE_TO"],
"JUMP_AWAY" => [ 0, 32, 0, 8, -1, -4, "MOVE_AWAY"],
"JUMP_TO_TARGET" => [ 0, 12, -12, 12, -1, -6, "MOVE_TO"],
"THROW_ALLY" => [ 0, -24, 0, 16, 0, -2, "MOVE_TO"],
"TRAMPLE" => [ 0, 12, -32, 12, -1, -6, "ABOVE_DISPLAY"],
"PREV_JUMP_ATTACK" => [ 0, -32, 0, 12, -1, -2, "WPN_SWING_V"],
"PREV_STEP_ATTACK" => [ 0, 12, 0, 12, -1, -5, "WPN_SWING_VS"],
"REAR_SWEEP_ATTACK" => [ 0, 12, 0, 16, 0, -3, "WPN_SWING_V"],
"JUMP_FIELD_ATTACK" => [ 0, 0, 0, 16, 0, -5, "WPN_SWING_V"],
"DASH_ATTACK" => [ 0, -96, 0, 16, 2, 0, "WPN_SWING_V"],
"RIGHT_DASH_ATTACK" => [ 0, -96, 32, 16, 2, 0, "WPN_SWING_V"],
"LEFT_DASH_ATTACK" => [ 0, -96, -32, 16, 2, 0, "WPN_SWING_V"],
"RIGHT_DASH_ATTACK2" => [ 0,-128, 48, 16, 2, 0, "WPN_SWING_V"],
"LEFT_DASH_ATTACK2" => [ 0,-128, -48, 16, 2, 0, "WPN_SWING_V"],
#--------------------------------------------------------------------------
# ● Battler Float Animations
#--------------------------------------------------------------------------
# These types of single-actions defines the movement of battlers from their
# own shadows. Please note that it is not possible to move horizontally
# while floating from a shadow.
#
# Type - Always "float".
# A - Starting float height. Negative values move up.
# Positive values move down.
# B - Ending float height. This height is maintained until another action.
# Time - Duration of movement from point A to point B
# Animation - Specifies the Battler Animation to be used.
# Type A B Time Animation
"FLOAT_" => ["float", -22, -20, 2, "WAIT(FIXED)"],
"FLOAT_2" => ["float", -20, -18, 2, "WAIT(FIXED)"],
"FLOAT_3" => ["float", -18, -20, 2, "WAIT(FIXED)"],
"FLOAT_4" => ["float", -20, -22, 2, "WAIT(FIXED)"],
"JUMP_STOP" => ["float", 0, -80, 4, "WAIT(FIXED)"],
"JUMP_LAND" => ["float", -80, 0, 4, "WAIT(FIXED)"],
"LIFT" => ["float", 0, -30, 4, "WAIT(FIXED)"],
#--------------------------------------------------------------------------
# ● Battler Position Reset
#--------------------------------------------------------------------------
# These types of single-actions define when a battler's turn is over and
# will reset the battler back to its starting coordinates. This type of
# single-action is required in all sequences.
#
# Please note that after a sequence has used this type of single-action,
# no more damage can be done by the battler since its turn is over.
#
# Type - Always "reset"
# Time - Time it takes to return to starting coordinates. Movement speed
# fluctuates depending on distance from start coordinates.
# Accel - Positive values accelerate. Negative values decelerate.
# Jump - Negative values produce a jumping arc. Positive values produce
# a reverse arc. [0: No jump]
# Animation - Specifies the Battler Animation to be used.
# Type Time Accel Jump Animation
"COORD_RESET" => ["reset", 16, 0, 0, "MOVE_TO"],
"FLEE_RESET" => ["reset", 16, 0, 0, "MOVE_AWAY"],
#--------------------------------------------------------------------------
# ● Forced Battler Actions
#--------------------------------------------------------------------------
# These types of single-actions allow forced control of other battlers
# that you define.
#
# Type - Specifies action type. "SINGLE" or "SEQUENCE"
#
# Object - The battler that will execute the action defined under Action
# Name. 0 is for selected target, and any other number is a State
# number (1~999), and affects all battlers with the State on them.
# By adding a - (minus sign) followed by a Skill ID number (1~999),
# it will define the actors that know the specified skill, besides
# the original actor.
# If you want to designate an actor by their index ID number,
# add 1000 to their index ID number. If the system cannot designate
# the index number(such as if actor is dead or ran away), it will
# select the nearest one starting from 0. If a response fails,
# the action will be canceled. (Example: Ylva's actor ID is 4. A
# value of 1004 would define Ylva as the Object.)
#
# Reset Type - Specifies method of returning the battler to its original
# location.
# Action Name - Specifies action used. If Type is SINGLE, then Action Name
# must be a single-action function. If Type is SEQUENCE,
# the Action Name must an action sequence name.
# Type Object Reset Type Action Name
"LIGHT_BLOWBACK" => ["SINGLE", 0, "COORD_RESET", "EXTRUDE"],
"RIGHT_TURN" => ["SINGLE", 0, "COORD_RESET", "CLOCKWISE_TURN"],
"DROP_DOWN" => ["SINGLE", 0, "COORD_RESET", "Y_SHRINK"],
"IMPACT_1" => ["SINGLE", 0, "COORD_RESET", "OBJ_TO_SELF"],
"LIFT_ALLY" => ["SINGLE", 0, "", "LIFT"],
"ULRIKA_ATTACK" => ["SEQUENCE", 18, "COORD_RESET", "ULRIKA_ATTACK_1"],
"4_MAN_ATK_1" => ["SEQUENCE", -101, "COORD_RESET", "4_MAN_ATTACK_1"],
"4_MAN_ATK_2" => ["SEQUENCE", -102, "COORD_RESET", "4_MAN_ATTACK_2"],
"4_MAN_ATK_3" => ["SEQUENCE", -103, "COORD_RESET", "4_MAN_ATTACK_3"],
"ALLY_FLING" => ["SEQUENCE", 1000, "COORD_RESET", "THROW"],
#--------------------------------------------------------------------------
# ● Target Modification
#--------------------------------------------------------------------------
# Changes battler's target in battle. Original target will still be stored.
# Current battler is the only battler capable of causing damage.
#
# Type - Always "target"
#
# Object - The battler that will have its target modified. 0 is selected
# target, any other number is a State ID number (1~999), and
# changes all battlers with that state on them to target the new
# designated target.
# If you want to designate an actor by their index ID number,
# add 1000 to their index ID number. If the system cannot designate
# the index number(such as if actor is dead or ran away), it will
# select the nearest one starting from 0. If a response fails,
# the action will be canceled. (Example: Ylva's actor ID is 4. A
# value of 1004 would define Ylva as the Object.)
#
# Target - New Target. [0=Self] [1=Self's Target]
# [2=Self's Target After Modification]
# [3=Reset to Previous Target (if 2 was used)]
# Target Mod name Type Object Target
"REAL_TARGET" => ["target", 0, 0],
"TWO_UNIFIED_TARGETS" => ["target", 18, 1],
"FOUR_UNIFIED_TARGETS" => ["target", 19, 1],
"ALLY_TO_THROW" => ["target", 1000, 2],
"THROW_TARGET" => ["target", 1000, 3],
#--------------------------------------------------------------------------
# ● Skill Linking
#--------------------------------------------------------------------------
# Linking to the next skill will stop any current action. Linking to the
# next skill will also require and consume MP/HP cost of that skill.
#
# Type - Always "der"
# Chance - Chance, in percent, to link to the defined skill ID. (0~100)
# Link - true: actor does not require Skill ID learned to link.
# false: actor requires Skill ID learned.
# Skill ID - ID of the skill that will be linked to.
# Action Name Type Chance Link Skill ID
"LINK_SKILL_91" => ["der", 100, true, 91],
"LINK_SKILL_92" => ["der", 100, true, 92],
#--------------------------------------------------------------------------
# ● Action Conditions
#--------------------------------------------------------------------------
# If the condition is not met, all current actions are canceled.
#
# A: Type - always "nece"
# B: Object - Object that Condition refers to. [0=Self] [1=Target]
# [2=All Enemies] [3=All Allies]
# C: Content - [0=State] [1=Parameter] [2=Switch] [3=Variable] [4=Skill]
#
# D: Condition - This value is determined by the value you set for Content.
# [0] State: State ID
# [1] Parameter: [0=Current HP] [1=Current MP] [2=ATK] [3=DEX] [4=AGI] [5=INT]
# [2] Switch: Game Switch Number
# [3] Variable: Game Variable Number
# [4] Skill: Skill ID
#
# E: Supplement - Supplement for the Condition as defined above.
# [0] State: Amount required. If number is positive, the condition is how
# many have the state, while a negative number are those who
# don't have the state.
# [1] Parameter: If Object is more than one battler, average is used.
# Success if Parameter is greater than value. If Value
# is negative, then success if lower.
# [2] Switch: [true: Switch ON succeeds] [false: Switch OFF succeeds]
# [3] Variable: Game variable value used to determine if condition is met. If
# supplement value is positive, Game Variable must have more
# than the defined amount to succeed. If supplement value has a
# minus symbol (-) attached, Game Variable must have less than
# the defined amount to succeed. (Ex: -250 means the Game
# Variable must have a value less than 250 to succeed.)
# [4] Skill: Required amount of battlers that have the specified skill
# ID learned.
# Type Obj Cont Cond Supplement
# A B C D E
"2_MAN_ATK_COND" => ["nece", 3, 0, 18, 1],
"4_MAN_ATK_COND" => ["nece", 3, 0, 19, 3],
"FLOAT_STATE" => ["nece", 0, 0, 17, 1],
"CAT_STATE" => ["nece", 0, 0, 20, 1],
#--------------------------------------------------------------------------
# ● Battler Rotation
#--------------------------------------------------------------------------
# Rotates battler image. Weapon Animations are not automatically adjusted
# for Battler Rotation like with Invert settings.
#
# Type - always "angle"
# Time - Duration duration of rotation animation in frames.
# Start - Starting angle. 0-360 degrees. Can be negative.
# End - Ending Angle. 0-360 degrees. Can be negative.
# Return - true: End of rotation is the same as end of duration.
# false: Rotation animation as defined.
# Type Time Start End Return
"FALLEN" => ["angle", 1, -90, -90,false],
"CLOCKWISE_TURN" => ["angle", 48, 0,-360,false],
"COUNTERCLOCKWISE_TURN" => ["angle", 6, 0, 360,false],
#--------------------------------------------------------------------------
# ● Battler Zoom
#--------------------------------------------------------------------------
# Stretch and shrink battler sprites with these single-actions.
#
# Type - always "zoom"
# Time - Duration of zoom animation in frames.
# X - X scale - Stretches battler sprite horizontally by a factor of X.
# 1.0 is normal size, 0.5 is half size.
# Y - Y scale - Stretches battler sprite vertically by a factor of Y.
# 1.0 would be normal size, 0.5 would be half size.
# Return - true: End of rotation is the same as end of duration.
# false: Zoom animation as defined.
# Battler zoom is still temporary.
# Type Time X Y Return
"X_SHRINK" => ["zoom", 16, 0.5, 1.0, true],
"Y_SHRINK" => ["zoom", 16, 1.0, 0.5, true],
#--------------------------------------------------------------------------
# ● Damage and Database-Assigned Animations
#--------------------------------------------------------------------------
# These single-actions deal with animations, particularly with those assigned
# in the Database for Weapons, Skills and Items. These are what causes
# any damage/healing/state/etc. application from Weapons, Skills and Items.
#
# A difference between "anime" and "m_a" single-actions is that
# "anime" triggered animations will move with the Object on the screen. The
# Z-axis of animations will always be over battler sprites. If "OBJ_ANIM"
# is added at the beginning of the name, it will be both damage and defined
# animation.
#
# Type - always "anime"
# ID - (-1): Uses assigned animation from game Database.
# (-2): Uses equipped Weapon animation as assigned in the Database.
# (1~999): Database Animation ID.
# Object - [0=Self] [1=Target]
# Invert - If set to true, the animation is inverted horizontally.
# Wait - true: Sequence will not continue until animation is completed.
# false: Sequence will continue regardless of animation length.
# Weapon2 - true: If wielding two weapons, damage and animation will be
# based off Weapon 2.
# Type ID Object Invert Wait Weapon2
"OBJ_ANIM" => ["anime", -1, 1, false,false, false],
"OBJ_ANIM_WEIGHT" => ["anime", -1, 1, false, true, false],
"OBJ_ANIM_WEAPON" => ["anime", -2, 1, false,false, false],
"OBJ_ANIM_L" => ["anime", -1, 1, false,false, true],
"HIT_ANIM" => ["anime", 14, 1, false,false, false],
"KILL_HIT_ANIM" => ["anime", 67, 1, false,false, false],
#--------------------------------------------------------------------------
# ● Movement and Display of Animations
#--------------------------------------------------------------------------
# These single-actions provide motion options for animations used for
# effects such as long-ranged attacks and projectiles. Weapon sprites
# may also substitute animations.
#
# A difference between "m_a" and "anime" single-actions is that "m_a"
# animations will stay where the Object was even if the Object moved.
#
# Type - always "m_a"
# ID - 1~999: Database Animation ID
# 0: No animation displayed.
# Object - Animation's target. [0=Target] [1=Enemy's Area]
# [2=Party's Area] [4=Self]
# Pass - [0: Animation stops when it reaches the Object.]
# [1: Animation passes through the Object and continues.]
# Time - Duration of animation travel time and display. Larger values
# decrease travel speed. Increase this value if the animation
# being played is cut short.
# Arc - Trajectory - Positive values produce a low arc.
# Negative values produce a high arc.
# [0: No Arc]
# Xp - X Pitch - This value adjusts the initial X coordinate of the
# animation. Enemy calculation will be automatically inverted.
# Yp - Y Pitch - This value adjusts the initial X coordinate of the
# animation.
# Start - Defines origin of animation movement.
# [0=Self] [1=Target] [2=No Movement]
# Z-axis - true: Animation will be over the battler sprite.
# false: Animation will be behind battler sprite.
# Weapon - Insert only "Throwing Weapon Rotation" and
# "Throwing Skill Rotation" actions. For no weapon sprite, use "".
# Type ID Object Pass Time Arc Xp Yp Start Z Weapon
"START_MAGIC_ANIM" => ["m_a", 2, 4, 0, 50, 0, 0, 0, 2,false,""],
"OBJ_TO_SELF" => ["m_a", 51, 0, 0, 18, 0, 0, 0, 1,false,""],
"START_WEAPON_THROW"=> ["m_a", 0, 0, 0, 16, -24, 0, 0, 0,false,"WPN_ROTATION"],
"END_WEAPON_THROW" => ["m_a", 0, 0, 0, 16, 24, 0, 0, 1,false,"WPN_ROTATION"],
"STAND_CAST" => ["m_a",100, 1, 0, 50, 0, 0, 0, 2, true,""],
#--------------------------------------------------------------------------
# ● Throwing Weapon Rotation
#--------------------------------------------------------------------------
# These are used to rotate weapon sprites that are "thrown" with Movement of
# Animation single-actions. These must be used while the sprite is in flight.
# You may assign a different weapon graphic to be thrown in this
# configuration script under Throwing Weapon Graphic Settings.
#
# Start - Starting angle in degrees (0-360)
# End - Ending angle in degrees. (0-360)
# Time - Duration, in frames, of a single rotation. Rotation will continue
# until the animation is complete.
# Start Angle Time
"WPN_ROTATION" => [ 0, 360, 8],
#--------------------------------------------------------------------------
# ● Throwing Skill Rotation
#--------------------------------------------------------------------------
# Different from Throwing Weapon Rotation. These single-actions are used to
# rotate weapon sprites that are "thrown" with Movement of Animation single
# actions. These are specifically used with skills. You may assign a different weapon graphic to be
# thrown in this configuration script under Throwing Weapon Graphic Settings.
#
# Start - Starting angle in degrees (0-360)
# End - Ending angle in degrees. (0-360)
# Time - Duration, in frames, of a single rotation. Rotation will continue
# until the animation is complete.
# Type - Always "skill".
# Weapon Action Name Start End Time Type
"WPN_THROW" => [ 0, 360, 8, "skill"],
#--------------------------------------------------------------------------
# ● Status Balloon Animation
#--------------------------------------------------------------------------
# Uses Balloon.png in the System folder.
#
# Type - Always "balloon"
# Row - Determines row from the Balloon.png (0~9)
# Loop - Balloon loop behavior. Balloon disappears when loop is
# complete. [0="One-Way" Loop] [1="Round-Trip" Loop]
# Emote Name Type Row Loop
"STATUS-NORMAL" => ["balloon", 6, 1],
"STATUS-CRITICAL" => ["balloon", 5, 1],
"STATUS-SLEEP" => ["balloon", 9, 1],
#--------------------------------------------------------------------------
# ● Sound Effect Actions
#--------------------------------------------------------------------------
# Type1 - always "sound"
# Type2 - ["se","bgm","bgs"]
# Pitch - Value between 50 and 150.
# Vol - Volume - Value between 0 and 100.
# Filename - Name of the sound to be played.
# Type1 Type2 Pitch Vol Filename
"062-Swing01" => ["sound", "se", 80, 100, "062-Swing01"],
#--------------------------------------------------------------------------
# ● Game Speed Modifier
#--------------------------------------------------------------------------
# Type - always "fps"
# Speed - Speed in Frames Per Second. 40 is normal frame rate.
# Use with care as this function modifies FPS directly and will conversly
# affect any active timers or time systems.
# Type Speed
"FPS_SLOW" => ["fps", 20],
"FPS_NORMAL" => ["fps", 40],
#--------------------------------------------------------------------------
# ● State Granting Effects
#--------------------------------------------------------------------------
# Type - always "sta+"
# Object - [0=Self] [1=Target] [2=All Enemies] [3=All Allies]
# [4=All Allies (excluding user)]
# State ID - State ID to be granted.
# Type Object State ID
"2_MAN_TECH_GRANT" => ["sta+", 0, 18],
"4_MAN_TECH_GRANT" => ["sta+", 0, 19],
"CATFORM_GRANT" => ["sta+", 0, 20],
#--------------------------------------------------------------------------
# ● State Removal Effects
#--------------------------------------------------------------------------
# Type - always "sta-"
# Object - [0=Self] [1=Target] [2=All Enemies] [3=All Allies]
# [4=All Allies (excluding user)]
# State ID - State ID to be removed.
# Type Object State ID
"2_MAN_TECH_REVOKE" => ["sta-", 3, 18],
"4_MAN_TECH_REVOKE" => ["sta-", 3, 19],
#--------------------------------------------------------------------------
# ● Battler Transformation Effects
#--------------------------------------------------------------------------
# Type - always "change"
# Reset - true: Battler sprite reverts back to default file after battle.
# false: Transformation is permanent after battle.
# Filename - Battler graphics file that will be transformed to.
# Type Reset Filename
"TRANSFORM_CAT" => ["change", true,"007-Fighter07"],
"TRANSFORM_CANCEL" => ["change", true,"040-Mage08"],
#--------------------------------------------------------------------------
# ● Cut-In Image Effects
#--------------------------------------------------------------------------
# Only one image can be displayed at a time.
#
# X1 - Image's starting X-coordinate.
# Y1 - Starting Y-coordinate.
# X2 - Ending X-coordinate.
# Y2 - Ending Y-coordinate.
# Time - Length of time from start to end. Higher value is slower.
# Z-axis - true: Image appears over BattleStatus Window.
# false: Image appears behind BattleStatus Window.
# Filename - File name from .Graphics\Pictures folder.
# Type X1 Y1 X2 Y2 Time Z-axis Filename
"CUT_IN_START" => ["pic",-280, 48, 0, 64, 14, false,"016-Thief01"],
"CUT_IN_END" => ["pic", 0, 48, 640, 64, 12, false,"016-Thief01"],
#--------------------------------------------------------------------------
# ● Game Switch Settings
#--------------------------------------------------------------------------
# Type - Always "switch"
# Switch - Switch number from the game database.
# ON/OFF - [true:Switch ON] [false:Switch OFF]
#
# Type Switch ON/OFF
"GAME_SWITCH_1_ON" => ["switch", 1, true],
#--------------------------------------------------------------------------
# ● Game Variable Settings
#--------------------------------------------------------------------------
# Type - Always "variable"
# Var - Variable Number from the game database.
# Oper - [0=Set] [1=Add] [2=Sub] [3=Mul] [4=Div] [5=Mod]
# X - value of the operation.
#
# Type Var Oper X
"GAME_VAR_1_+1" => ["variable", 1, 1, 1],
#--------------------------------------------------------------------------
# ● Script Operation Settings
#--------------------------------------------------------------------------
# Type - Always "script"
#
# Inserts a simple script code into the action sequence. In the sample,
# where it says p=1 can be replaced with any script. Character strings
# and anything beyond functions will not work. (?)
# Type
"TEST_SCRIPT" => ["script", "
p = 1
"],
#--------------------------------------------------------------------------
# ● Special Modifiers - DO NOT CHANGE THESE NAMES
#--------------------------------------------------------------------------
# Clear image - Clears images such as Cut-in graphics.
# Afterimage ON - Activates Afterimage of battler.
# Afterimage OFF - Deactivates Afterimage.
# Invert - Invert animation. Use Invert again in a sequence to cancel
# because "COORD_RESET" does not reset Invert.
# Don't Wait - Any actions after Don't Wait is applied are done instantly.
# Apply "Don't Wait" again in a sequence to trigger off.
# Can Collapse - Triggers collapse of battler when HP is 0.
# Required in every damage sequence.
# Two Wpn Only - The single-action following Two Wpn Only will only execute
# if the actor is wielding two weapons. If the actor is not,
# the single-action will be skipped and will move on to the next.
# One Wpn Only - The single-action following One Wpn Only will only execute
# if the actor is wielding one weapon. If the actor is not,
# the single-action will be skipped and will move on to the next.
# Process Skill - The Return marker for individual processing of a skill.
# Process Skill End - The End marker for individual processing of a skill.
# Start Pos Change - Changes the Start Position to wherever the battler
# currently is on screen.
# Start Pos Return - Returns battler to original Start Position.
# Cancel Action - Trigger the "end" of battler's turn which will cause the
# the next battler's turn to execute.
# This includes the function of Can Collapse, and no
# additional damage can be dealt by the battler after this.
# End - This is used when no action is automatically recognized.
#
# Note: If you wish to understand how Process Skill and Process Skill End
# functions, please examine the "SKILL_ALL" sequence in this Config
# and use the Float All skill provided in the demo to see how it works.
"Clear image" => ["Clear image"],
"Afterimage ON" => ["Afterimage ON"],
"Afterimage OFF" => ["Afterimage OFF"],
"Invert" => ["Invert"],
"Don't Wait" => ["Don't Wait"],
"Can Collapse" => ["Can Collapse"],
"Two Wpn Only" => ["Two Wpn Only"],
"One Wpn Only" => ["One Wpn Only"],
"Process Skill" => ["Process Skill"],
"Process Skill End" => ["Process Skill End"],
"Start Pos Change" => ["Start Pos Change"],
"Start Pos Return" => ["Start Pos Return"],
"Cancel Action" => ["Cancel Action"],
"End" => ["End"]
#--------------------------------------------------------------------------
# ● About Wait
#--------------------------------------------------------------------------
# When there is only a numerical value as a single-action name, it will be
# considered a delay, in frames, before the Action Sequence continues.
# (i.e. "10", "42") Because of this, single-action function names for the
# effects defined above cannot be entirely numerical. Any Battler Animations
# that have been prompted will persist when Waiting.
}
#==============================================================================
# ■ Action Sequence
#------------------------------------------------------------------------------
# Action sequences are made of the single-action functions defined above.
#==============================================================================
# Action Sequences defined here can be used for Actor/Enemy actions below.
# Sequences are processed left to right in order.
ACTION = {
#------------------------------- Basic Actions --------------------------------
"BATTLE_START" => ["START_POSITION","COORD_RESET"],
"WAIT" => ["WAIT"],
"WAIT-CRITICAL" => ["NO_MOVE","WAIT(FIXED)","STATUS-CRITICAL","22"],
"WAIT-NORMAL" => ["NO_MOVE","WAIT(FIXED)","STATUS-NORMAL","22"],
"WAIT-SLEEP" => ["NO_MOVE","WAIT(FIXED)","STATUS-SLEEP","22"],
"WAIT-FLOAT" => ["WAIT(FIXED)","6","FLOAT_","4",
"FLOAT_2","4","FLOAT_3","4",
"FLOAT_4","4"],
"DEAD" => ["FALLEN","ATTACK_FAIL"],
"DAMAGE" => ["DEAL_DAMAGE","COORD_RESET"],
"FLEE" => ["FLEE_SUCCESS"],
"ENEMY_FLEE" => ["FLEE_SUCCESS","COORD_RESET"],
"FLEE_FAIL" => ["FLEE_FAIL","WAIT(FIXED)","8","COORD_RESET"],
"COMMAND_INPUT" => ["BEFORE_MOVE"],
"COMMAND_SELECT" => ["COORD_RESET"],
"GUARD_ATTACK" => ["WAIT(FIXED)","4","FLOAT_STATE","FLOAT_",
"2","FLOAT_2","2","FLOAT_3","2",
"FLOAT_4","2"],
"EVADE_ATTACK" => ["JUMP_AWAY","JUMP_AWAY","WAIT(FIXED)","16",
"COORD_RESET"],
"ENEMY_EVADE_ATTACK" => ["JUMP_AWAY","WAIT(FIXED)","16","COORD_RESET"],
"VICTORY" => ["WAIT(FIXED)","16","RIGHT(FIXED)","VICTORY_JUMP",
"WAIT(FIXED)","Don't Wait","CAT_STATE",
"START_MAGIC_ANIM","TRANSFORM_CANCEL","WAIT(FIXED)","Don't Wait"],
"RESET_POSITION" => ["COORD_RESET"],
#---------------------- "Forced Action" Sequences --------------------------
"ULRIKA_ATTACK_1" => ["2","MOVING_TARGET_LEFT","WAIT(FIXED)",
"START_MAGIC_ANIM","WPN_SWING_UNDER","WPN_RAISED",
"48","RIGHT_DASH_ATTACK","64","FLEE_RESET"],
"4_MAN_ATTACK_1" => ["2","4_MAN_ATTACK_2","WAIT(FIXED)","START_MAGIC_ANIM",
"WPN_SWING_UNDER","WPN_RAISED","90",
"LEFT_DASH_ATTACK","96","FLEE_RESET"],
"4_MAN_ATTACK_2" => ["2","4_MAN_ATTACK_3","WAIT(FIXED)","START_MAGIC_ANIM",
"WPN_SWING_UNDER","WPN_RAISED","60","RIGHT_DASH_ATTACK2","RIGHT_TURN",
"OBJ_ANIM","128","FLEE_RESET"],
"4_MAN_ATTACK_3" => ["2","4_MAN_ATTACK_4","WAIT(FIXED)","START_MAGIC_ANIM",
"WPN_SWING_UNDER","WPN_RAISED","34","LEFT_DASH_ATTACK2","RIGHT_TURN",
"OBJ_ANIM","144","FLEE_RESET"],
"THROW" => ["CLOCKWISE_TURN","4","MOVING_TARGET_FAST","JUMP_AWAY","4",
"WAIT(FIXED)","JUMP_AWAY","WAIT(FIXED)","32"],
#---------------------- Basic Action Oriented ------------------------------
"NORMAL_ATTACK" => ["PREV_MOVING_TARGET","WPN_SWING_V","OBJ_ANIM_WEIGHT",
"12","WPN_SWING_VL","OBJ_ANIM_L","Two Wpn Only","16",
"Can Collapse","FLEE_RESET"],
"ENEMY_UNARMED_ATK" => ["PREV_MOVING_TARGET","WPN_SWING_V","OBJ_ANIM_WEIGHT",
"Can Collapse","FLEE_RESET"],
"SKILL_USE" => ["BEFORE_MOVE","WAIT(FIXED)","START_MAGIC_ANIM",
"WPN_SWING_UNDER","WPN_RAISED","WPN_SWING_V",
"OBJ_ANIM_WEIGHT","Can Collapse","24","COORD_RESET"],
"SKILL_ALL" => ["BEFORE_MOVE","START_MAGIC_ANIM","WPN_SWING_UNDER","WPN_RAISED",
"Process Skill","WPN_SWING_V","OBJ_ANIM","24",
"Process Skill End","Can Collapse","COORD_RESET"],
"ITEM_USE" => ["PREV_MOVING_TARGET","WAIT(FIXED)","24","OBJ_ANIM_WEIGHT",
"Can Collapse","COORD_RESET"],
#------------------------------ Skill Sequences -------------------------------
"MULTI_ATTACK" => ["Afterimage ON","PREV_STEP_ATTACK","WPN_SWING_VL","OBJ_ANIM_WEAPON",
"WAIT(FIXED)","16","OBJ_ANIM_WEAPON","WPN_SWING_UNDER",
"WPN_SWING_OVER","4","JUMP_FIELD_ATTACK","WPN_SWING_VL",
"OBJ_ANIM_WEAPON","WAIT(FIXED)","16","OBJ_ANIM_WEAPON",
"Invert","WPN_SWING_V","WPN_SWING_VL","12","Invert",
"JUMP_FIELD_ATTACK","WPN_SWING_VL","OBJ_ANIM_WEAPON",
"JUMP_AWAY","JUMP_AWAY","WAIT(FIXED)",
"OBJ_ANIM_WEAPON","DASH_ATTACK","WPN_SWING_VL","Can Collapse",
"Afterimage OFF","16","FLEE_RESET"],
"MULTI_ATTACK_RAND" => ["PREV_STEP_ATTACK","WPN_SWING_VL","OBJ_ANIM_WEAPON","WAIT(FIXED)","16",
"PREV_STEP_ATTACK","WPN_SWING_VL","OBJ_ANIM_WEAPON","WAIT(FIXED)","16",
"PREV_STEP_ATTACK","WPN_SWING_VL","OBJ_ANIM_WEAPON","WAIT(FIXED)","16",
"PREV_STEP_ATTACK","WPN_SWING_VL","OBJ_ANIM_WEAPON","Can Collapse","COORD_RESET"],
"RAPID_MULTI_ATTACK" => ["PREV_MOVING_TARGET","WPN_SWING_V","LIGHT_BLOWBACK","OBJ_ANIM_WEAPON",
"PREV_MOVING_TARGET_FAST","WPN_SWING_V","LIGHT_BLOWBACK","WPN_SWING_VL","OBJ_ANIM_WEAPON",
"PREV_MOVING_TARGET_FAST","WPN_SWING_V","LIGHT_BLOWBACK","WPN_SWING_VL","OBJ_ANIM_WEAPON",
"PREV_MOVING_TARGET_FAST","WPN_SWING_V","LIGHT_BLOWBACK","WPN_SWING_VL","OBJ_ANIM_WEAPON",
"PREV_MOVING_TARGET_FAST","WPN_SWING_V","LIGHT_BLOWBACK","WPN_SWING_VL","OBJ_ANIM_WEAPON",
"PREV_MOVING_TARGET_FAST","WPN_SWING_V","LIGHT_BLOWBACK","WPN_SWING_VL","OBJ_ANIM_WEAPON",
"Can Collapse","12","COORD_RESET"],
"2-MAN_ATTACK" => ["2_MAN_ATK_COND","TWO_UNIFIED_TARGETS","ULRIKA_ATTACK",
"MOVING_TARGET_RIGHT","WAIT(FIXED)","START_MAGIC_ANIM","WPN_SWING_UNDER",
"WPN_RAISED","48","KILL_HIT_ANIM","LEFT_DASH_ATTACK","64","OBJ_ANIM",
"Can Collapse","FLEE_RESET","2_MAN_TECH_REVOKE"],
"2-MAN_ATTACK_ASSIST"=> ["2_MAN_TECH_GRANT"],
"4-MAN_ATTACK" => ["4_MAN_ATK_COND","FOUR_UNIFIED_TARGETS","4_MAN_ATK_1",
"4_MAN_ATK_2","4_MAN_ATK_3","4_MAN_ATTACK_1","WAIT(FIXED)",
"START_MAGIC_ANIM","WPN_SWING_UNDER","WPN_RAISED","90",
"KILL_HIT_ANIM","RIGHT_DASH_ATTACK","64","OBJ_ANIM_WEIGHT",
"Can Collapse","FLEE_RESET","4_MAN_TECH_REVOKE"],
"4-MAN_ATTACK_ASSIST"=> ["4_MAN_TECH_GRANT"],
"THROW_WEAPON" => ["BEFORE_MOVE","WPN_SWING_V","062-Swing01","WAIT(FIXED)",
"START_WEAPON_THROW","12","OBJ_ANIM_WEAPON","Can Collapse",
"END_WEAPON_THROW","COORD_RESET"],
"MULTI_SHOCK" => ["JUMP_TO","JUMP_STOP","Process Skill",
"REAL_TARGET","WPN_SWING_V","IMPACT_1","8",
"OBJ_ANIM_WEAPON","Process Skill End","Can Collapse",
"JUMP_LAND","COORD_RESET"],
"SHOCK_WAVE" => ["REAL_TARGET","WPN_SWING_V","IMPACT_1","20",
"OBJ_ANIM_WEIGHT","Can Collapse"],
"SKILL_90_SEQUENCE" => ["PREV_MOVING_TARGET","OBJ_ANIM","WPN_SWING_V",
"16","LINK_SKILL_91","COORD_RESET"],
"SKILL_91_SEQUENCE" => ["FLEE_FAIL","START_MAGIC_ANIM","WPN_SWING_UNDER","WPN_RAISED",
"8","OBJ_ANIM","LINK_SKILL_92","COORD_RESET"],
"CUT_IN" => ["WAIT(FIXED)","START_MAGIC_ANIM","CUT_IN_START",
"75","CUT_IN_END","8","PREV_MOVING_TARGET",
"WPN_SWING_V","OBJ_ANIM_WEIGHT","Can Collapse",
"Clear image","FLEE_RESET"],
"STOMP" => ["JUMP_TO_TARGET","HIT_ANIM","DROP_DOWN","JUMP_AWAY",
"TRAMPLE","HIT_ANIM","DROP_DOWN","JUMP_AWAY",
"TRAMPLE","OBJ_ANIM","DROP_DOWN","JUMP_AWAY",
"JUMP_AWAY","Can Collapse","WAIT(FIXED)","8","FLEE_RESET"],
"ALL_ATTACK_1" => ["BEFORE_MOVE","WAIT(FIXED)","START_MAGIC_ANIM","WPN_SWING_UNDER",
"WPN_RAISED","STAND_CAST","WPN_SWING_V","48",
"OBJ_ANIM_WEIGHT","Can Collapse","COORD_RESET"],
"TRANSFORM_CAT" => ["JUMP_TO","WAIT(FIXED)","START_MAGIC_ANIM","32",
"TRANSFORM_CAT","WAIT(FIXED)","CATFORM_GRANT","32","JUMP_AWAY"],
"THROW_FRIEND" => ["ALLY_TO_THROW","MOVING_TARGET","LIFT_ALLY","4",
"062-Swing01","THROW_TARGET","ALLY_FLING",
"THROW_ALLY","WAIT(FIXED)","OBJ_ANIM","COORD_RESET",
"WAIT(FIXED)","32"],
#-------------------------------------------------------------------------------
"End" => ["End"]}
end
#==============================================================================
# ■ Game_Actor
#------------------------------------------------------------------------------
# Actor Basic Action Settings
#==============================================================================
class Game_Actor < Game_Battler
#--------------------------------------------------------------------------
# ● Actor Unarmed Attack Animation Sequence
#--------------------------------------------------------------------------
# when 1 <- Actor ID number
# return "NORMAL_ATTACK" <- Corresponding action sequence name.
def non_weapon
case @actor_id
when 1 # Actor ID
return "NORMAL_ATTACK"
end
# Default action sequence for all unassigned Actor IDs.
return "NORMAL_ATTACK"
end
#--------------------------------------------------------------------------
# ● Actor Wait/Idle Animation
#--------------------------------------------------------------------------
def normal
case @actor_id
when 1
return "WAIT"
end
# Default action sequence for all unassigned Actor IDs.
return "WAIT"
end
#--------------------------------------------------------------------------
# ● Actor Critical (1/4th HP) Animation
#--------------------------------------------------------------------------
def pinch
case @actor_id
when 1
return "WAIT-CRITICAL"
end
# Default action sequence for all unassigned Actor IDs.
return "WAIT-CRITICAL"
end
#--------------------------------------------------------------------------
# ● Actor Guarding Animation
#--------------------------------------------------------------------------
def defence
case @actor_id
when 1
return "GUARD_ATTACK"
end
# Default action sequence for all unassigned Actor IDs.
return "GUARD_ATTACK"
end
#--------------------------------------------------------------------------
# ● Actor Damage Taken Animation
#--------------------------------------------------------------------------
def damage_hit
case @actor_id
when 1
return "DAMAGE"
end
# Default action sequence for all unassigned Actor IDs.
return "DAMAGE"
end
#--------------------------------------------------------------------------
# ● Actor Evasion Animation
#--------------------------------------------------------------------------
def evasion
case @actor_id
when 1
return "EVADE_ATTACK"
end
# Default action sequence for all unassigned Actor IDs.
return "EVADE_ATTACK"
end
#--------------------------------------------------------------------------
# ● Actor Command Input Animation
#--------------------------------------------------------------------------
def command_b
case @actor_id
when 1
return "COMMAND_INPUT"
end
# Default action sequence for all unassigned Actor IDs.
return "COMMAND_INPUT"
end
#--------------------------------------------------------------------------
# ● Actor Command Selected Animation
#--------------------------------------------------------------------------
def command_a
case @actor_id
when 1
return "COMMAND_SELECT"
end
# Default action sequence for all unassigned Actor IDs.
return "COMMAND_SELECT"
end
#--------------------------------------------------------------------------
# ● Actor Flee Success Animation
#--------------------------------------------------------------------------
def run_success
case @actor_id
when 1
return "FLEE"
end
# Default action sequence for all unassigned Actor IDs.
return "FLEE"
end
#--------------------------------------------------------------------------
# ● Actor Flee Failure Animation
#--------------------------------------------------------------------------
def run_ng
case @actor_id
when 1
return "FLEE_FAIL"
end
# Default action sequence for all unassigned Actor IDs.
return "FLEE_FAIL"
end
#--------------------------------------------------------------------------
# ● Actor Victory Animation
#--------------------------------------------------------------------------
def win
case @actor_id
when 1
return "VICTORY"
end
# Default action sequence for all unassigned Actor IDs.
return "VICTORY"
end
#--------------------------------------------------------------------------
# ● Actor Battle Start Animation
#--------------------------------------------------------------------------
def first_action
case @actor_id
when 1
return "BATTLE_START"
end
# Default action sequence for all unassigned Actor IDs.
return "BATTLE_START"
end
#--------------------------------------------------------------------------
# ● Actor Return Action when actions are interuptted/canceled
#--------------------------------------------------------------------------
def recover_action
case @actor_id
when 1
return "RESET_POSITION"
end
# Default action sequence for all unassigned Actor IDs.
return "RESET_POSITION"
end
#--------------------------------------------------------------------------
# ● Actor Shadow
#--------------------------------------------------------------------------
# return "shadow01" <- Image file name in .Graphics\Characters
# return "" <- No shadow used.
def shadow
case @actor_id
when 1
return "shadow00"
end
# Default shadow for all unassigned Actor IDs.
return "shadow00"
end
#--------------------------------------------------------------------------
# ● Actor Shadow Adjustment
#--------------------------------------------------------------------------
# return [ X-Coordinate, Y-Coordinate]
def shadow_plus
case @actor_id
when 1
return [ 0, 4]
end
# Default shadow positioning for all unassigned Actor IDs.
return [ 0, 4]
end
end
#==============================================================================
# ■ Game_Enemy
#------------------------------------------------------------------------------
# Enemy Basic Action Settings
#==============================================================================
class Game_Enemy < Game_Battler
#--------------------------------------------------------------------------
# ● Enemy Unarmed Attack Animation Sequence
#--------------------------------------------------------------------------
# when 1 <- EnemyID#
# return "ENEMY_UNARMED_ATK" <- Corresponding action sequence name.
def base_action
case @enemy_id
when 1
return "ENEMY_UNARMED_ATK"
end
# Default action sequence for all unassigned Enemy IDs.
return "ENEMY_UNARMED_ATK"
end
#--------------------------------------------------------------------------
# ● Enemy Wait/Idle Animation
#--------------------------------------------------------------------------
def normal
case @enemy_id
when 1
return "WAIT"
end
# Default action sequence for all unassigned Enemy IDs.
return "WAIT"
end
#--------------------------------------------------------------------------
# ● Enemy Critical (1/4th HP) Animation
#--------------------------------------------------------------------------
def pinch
case @enemy_id
when 1
return "WAIT"
end
# Default action sequence for all unassigned Enemy IDs.
return "WAIT"
end
#--------------------------------------------------------------------------
# ● Enemy Guarding Animation
#--------------------------------------------------------------------------
def defence
case @enemy_id
when 1
return "GUARD_ATTACK"
end
# Default action sequence for all unassigned Enemy IDs.
return "GUARD_ATTACK"
end
#--------------------------------------------------------------------------
# ● Enemy Damage Taken Animation
#--------------------------------------------------------------------------
def damage_hit
case @enemy_id
when 1
return "DAMAGE"
end
# Default action sequence for all unassigned Enemy IDs.
return "DAMAGE"
end
#--------------------------------------------------------------------------
# ● Enemy Evasion Animation
#--------------------------------------------------------------------------
def evasion
case @enemy_id
when 1
return "ENEMY_EVADE_ATTACK"
end
# Default action sequence for all unassigned Enemy IDs.
return "ENEMY_EVADE_ATTACK"
end
#--------------------------------------------------------------------------
# ● Enemy Flee Animation
#--------------------------------------------------------------------------
def run_success
case @enemy_id
when 1
return "ENEMY_FLEE"
end
# Default action sequence for all unassigned Enemy IDs.
return "ENEMY_FLEE"
end
#--------------------------------------------------------------------------
# ● Enemy Battle Start Animation
#--------------------------------------------------------------------------
def first_action
case @enemy_id
when 1
return "BATTLE_START"
end
# Default action sequence for all unassigned Enemy IDs.
return "BATTLE_START"
end
#--------------------------------------------------------------------------
# ● Enemy Return Action when action is interuptted/discontinued
#--------------------------------------------------------------------------
def recover_action
case @enemy_id
when 1
return "RESET_POSITION"
end
# Default action sequence for all unassigned Enemy IDs.
return "RESET_POSITION"
end
#--------------------------------------------------------------------------
# ● Enemy Shadow
#--------------------------------------------------------------------------
# return "shadow01" <- Image file name in .Graphics\Characters
# return "" <- No shadow used.
def shadow
case @enemy_id
when 1
return "shadow01"
when 30
return ""
end
# Default shadow for all unassigned Enemy IDs.
return "shadow01"
end
#--------------------------------------------------------------------------
# ● Enemy Shadow Adjustment
#--------------------------------------------------------------------------
# return [ X-Coordinate, Y-Coordinate]
def shadow_plus
case @enemy_id
when 1
return [ 0, -8]
end
# Default shadow positioning for all unassigned Enemy IDs.
return [ 0, 0]
end
#--------------------------------------------------------------------------
# ● Enemy Equipped Weapon
#--------------------------------------------------------------------------
# return 0 (Unarmed/No weapon equipped.)
# return 1 (Weapon ID number. (1~999))
def weapon
case @enemy_id
when 1 # Enemy ID
return 0 # Weapon ID
end
# Default weapon for all unassigned Enemy IDs.
return 0
end
#--------------------------------------------------------------------------
# ● Enemy Screen Positioning Adjustment
#--------------------------------------------------------------------------
# return [ 0, 0] <- [X-coordinate、Y-coordinate]
def position_plus
case @enemy_id
when 1
return [0, 0]
end
# Default positioning for all unassigned Enemy IDs.
return [ 0, 0]
end
#--------------------------------------------------------------------------
# ● Enemy Collapse Animation Settings
#--------------------------------------------------------------------------
# return 1 (Enemy sprite stays on screen after death.)
# return 2 (Enemy disappears from the battle like normal.)
# return 3 (Special collapse animation.) <- Good for bosses.
def collapse_type
case @enemy_id
when 1
return 2
when 30
return 3
end
# Default collapse for all unassigned Enemy IDs.
return 2
end
#--------------------------------------------------------------------------
# ● Enemy Multiple Action Settings
#--------------------------------------------------------------------------
# Maximum Actions, Probability, Speed Adjustment
# return [ 2, 100, 100]
#
# Maximum Actions - Maximum number of actions enemy may execute in a turn.
# Probability - % value. Chance for a successive action.
# Speed Adjustment - % value that decreases enemy's speed after
# each successive action.
def action_time
case @enemy_id
when 1
return [ 1, 100, 100]
end
# Default action for all unassigned Enemy IDs.
return [ 1, 100, 100]
end
#--------------------------------------------------------------------------
# ● Enemy Animated Battler Settings
#--------------------------------------------------------------------------
# return true - Enemy battler uses same animation frames as actors.
# return false - Default enemy battler.
# [Settings]
# 1.Enemy animated battler file must be in .Graphics\Characters folder.
# 2.Enemy battler file names must match between .Graphics\Characters and
# .Graphics/Battlers folders.
def anime_on
case @enemy_id
when 1
return false
end
# Default setting for all unassigned Enemy IDs.
return false
end
#--------------------------------------------------------------------------
# ● Enemy Invert Settings
#--------------------------------------------------------------------------
# return false <- Normal
# return true <- Inverts enemy image
def action_mirror
case @enemy_id
when 1
return false
end
# Default setting for all unassigned Enemy IDs.
return false
end
end
#==============================================================================
# ■ module RPG
#------------------------------------------------------------------------------
# State Action Settings
#==============================================================================
class RPG::State
#--------------------------------------------------------------------------
# ● State Affliction Wait Animation Settings
#--------------------------------------------------------------------------
# when 1 <- State ID number
# return "DEAD" <- Action sequence when afflicted by specified state.
def base_action
case @id
when 1 # Incapacitated(HP0). Has the highest priority.
return "DEAD"
when 2,3,4,5,7
return "WAIT-NORMAL"
when 6
return "WAIT-SLEEP"
when 17
return "WAIT-FLOAT"
end
# Default action sequence for all unassigned State IDs.
return "WAIT"
end
#--------------------------------------------------------------------------
# ● State Enhancement Extension Settings
#--------------------------------------------------------------------------
# Note about REFLECT and NULL states:
# An item/skill is considered physical if "Physical Attack" is
# checked under "Options" in your Database. Otherwise, it is magical.
#
# "AUTOLIFE/50" - Automatically revives when Incapacitated.
# Value after "/" is % of MAXHP restored when revived.
# "MAGREFLECT/39" - Reflects magical skills to the original caster.
# Value after "/" is Animation ID when triggered.
# "MAGNULL/39" - Nullify magical skills and effects.
# Value after "/" is Animation ID when triggered.
# "PHYREFLECT/39" - Reflects physical skills to the original caster.
# Value after "/" is Animation ID when triggered.
# "PHYNULL/39" - Nullify physical skills and effects.
# Value after "/" is Animation ID when triggered.
# "COSTABSORB" - Absorbs the MP (or HP) cost of an incoming skill when
# affected. This will not appear as POP Damage. This
# function is similar to Celes' "Runic" from FF6.
# "ZEROTURNLIFT" - State is lifted at the end of turn regardless.
# "EXCEPTENEMY" - Enemies will not use animation sequence assigned
# under State Affliction Wait Animation Settings when
# afflicted. (Actors still will.)
# "NOPOP" - State name will not appear as POP Damage.
# "HIDEICON" - State icon will not appear in the BattleStatus Window.
# "NOSTATEANIME" - State's caster and enemies will not use animation
# sequence assigned under State Affliction Wait Animation
# Settings when afflicted.
# "SLIPDAMAGE" - Apply slip damage. Assign values under Slip Damage Settings.
# "REGENERATION" - Apply regeneration. Assign values under Slip Damage Settings.
# "NONE" - No extension. Used as a default.
def extension
case @id
when 1 # Incapacitated State. Has highest priority.
return ["NOPOP","EXCEPTENEMY"]
when 2 # Poison
return ["SLIPDAMAGE"]
when 18 # 2-Man Tech
return ["ZEROTURNLIFT","HIDEICON"]
when 19 # 4-Man Tech
return ["ZEROTURNLIFT","HIDEICON"]
when 20 # Cat Transformation
return ["HIDEICON","NOSTATEANIME"]
end
# Default extension for unassigned State IDs.
return ["NONE"]
end
#--------------------------------------------------------------------------
# ● Slip Damage Settings
#--------------------------------------------------------------------------
# Also includes regeneration options.
#
# when 1 <- State ID. Slip Damage only applies if "SLIPDAMAGE" is assigned above.
# Multiple settings may be applied. Ex)[["hp",0,5,true],["mp",0,5,true]]
#
# Type, Constant, %, POP?, Allow Death
# return [["hp", 0, 10, true, true]]
#
# Type – "hp" or "mp".
# Constant – Set a constant value to apply each turn.
# Positive values are damage. Negative values are recovery.
# % - Set a percentage value to apply each turn based on MAX HP/MP.
# Positive values are damage. Negative values are recovery.
# POP? - Determines whether or not you want slip damage value to
# appear as POP Damage.
# Allow Death - true: Slip damage can kill.
# false: Slip damage will not kill. (Battler will be left at 1 HP)
def slip_extension
case @id
when 2 # Poison
return [["hp", 0, 10, true, true]]
end
return []
end
end
#==============================================================================
# ■ module RPG
#------------------------------------------------------------------------------
# Weapon Action Settings
#==============================================================================
class RPG::Weapon
#--------------------------------------------------------------------------
# ● Weapon Animation Sequence Settings
#--------------------------------------------------------------------------
# Assigns a specific animation sequence when using a weapon.
#
# when 1 <- Weapon ID number
# return "NORMAL_ATTACK" <- Action sequence for assigned Weapon ID.
def base_action
case @id
when 1
return "NORMAL_ATTACK"
end
# Default action sequence for unassigned Weapon IDs.
return "NORMAL_ATTACK"
end
#--------------------------------------------------------------------------
# ● Weapon Graphic Assignment Settings
#--------------------------------------------------------------------------
# Allows use of a seperate weapon graphic besides the one assigned
# from Iconset.png
#
# return "001-Weapon01" <- Weapon image file name. If "", none is used.
# File must be in the .Graphics\Characters folder
# of your project.
def graphic
case @id
when 1
return ""
end
# Default weapon graphic for unassigned Weapon IDs.
return ""
end
#--------------------------------------------------------------------------
# ● Throwing Weapon Graphic Settings
#--------------------------------------------------------------------------
# Allows use of a seperate throwing weapon graphic besides the one assigned
# from Iconset.png. This is useful for arrows when you don't want the bow
# to be thrown.
#
# return "001-Weapon01" <- Weapon image file name. If "", none is used.
# File must be in the .Graphics\Characters folder
# of your project.
def flying_graphic
case @id
when 1
return ""
end
# Default throwing weapon graphic for unassigned Weapon IDs.
return ""
end
end
#==============================================================================
# ■ module RPG
#------------------------------------------------------------------------------
# Skill Action Settings
#==============================================================================
class RPG::Skill
#--------------------------------------------------------------------------
# ● Skill ID Sequence Assignments
#--------------------------------------------------------------------------
# Assign a skill ID from the Database to execute a defined action sequence.
# Only action sequence names can be assigned. Single-action names cannot
# be directly assigned here.
def base_action
case @id
when 84
return "THROW_WEAPON"
when 85
return "MULTI_ATTACK"
when 86
return "RAPID_MULTI_ATTACK"
when 87
return "MULTI_SHOCK"
when 88
return "SHOCK_WAVE"
when 89
return "MULTI_ATTACK_RAND"
when 90
return "SKILL_90_SEQUENCE"
when 91
return "SKILL_91_SEQUENCE"
when 92
return "NORMAL_ATTACK"
when 93
return "CUT_IN"
when 94
return "STOMP"
when 95
return "ALL_ATTACK_1"
when 96
return "SKILL_ALL"
when 97
return "TRANSFORM_CAT"
when 98
return "2-MAN_ATTACK"
when 99
return "2-MAN_ATTACK_ASSIST"
when 100
return "4-MAN_ATTACK"
when 101
return "4-MAN_ATTACK_ASSIST"
when 102
return "4-MAN_ATTACK_ASSIST"
when 103
return "4-MAN_ATTACK_ASSIST"
when 104
return "THROW_FRIEND"
end
# Default action sequence for unassigned Skill IDs.
return "NORMAL_ATTACK" if self.atk_f > 0
return "SKILL_USE"
end
#--------------------------------------------------------------------------
# ● Skill Enhancement Extension Settings
#--------------------------------------------------------------------------
# Multiple extensions may be applied to a skill ID.
# If "CONSUMEHP" is applied along with any other extensions that deal with
# MP in a forumla, it will be HP instead.
# This script WILL have compatibility issues with KGC_MPCostAlter.
#
# "NOEVADE" -Cannot be evaded regardless.
# "CONSUMEHP" -Consumes HP instead of MP.
# "%COSTMAX" -Consumes % of MAXMP. Example: Actor MAXMP500,
# 10 set in Database, MP50 cost.
# "%COSTNOW" -Consumes % of current MP.
# "IGNOREREFLECT" -Ignores damage reflection states.
# "%DAMAGEMAX/30" -Changes damage formula of skill to:
# damage = ENEMY MAX HP * [Integer] / 100
# [Integer] is the number you apply after "/".
# "%DAMAGENOW/30" -Changes damage formula of skill to:
# damage = ENEMY CURRENT HP * [Integer] / 100
# [Integer] is the number you apply after "/".
# "COSTPOWER" -Changes damage formula of skill to:
# damage = base damage * cost / MAX MP
# The more the skill costs, the more damage it will do.
# "HPNOWPOWER" -Changes damage formula of skill to:
# damage = base damage * CURRENT HP / MAX HP
# The less current HP you have, the less damage.
# "MPNOWPOWER" -Changes damage formula of skill to:
# damage = base damage * CURRENT MP / MAX MP
# The less current MP you have, the less damage.
# "NOHALFMPCOST" -"Half MP Cost" from armor options will not apply.
# "HELPHIDE" -Help window when casting will not appear.
# "TARGETALL" -Will affect all enemies and allies simultaneously.
# "RANDOMTARGET" -Target is chosen at random.
# "OTHERS" -Skill will not affect caster.
# "NOOVERKILL" -Damage will not be applied after the target reaches zero HP.
# "NOFLASH" -Battler will not flash when taking action.
# "FAST" -Battler will be the first to take action in the turn
# "SLOW" -Battler will be the last to take action in the turn
# "SPDAMAGE" -Damage is dealt to the target's SP instead of HP.
# "%DMGABSORB/50" -Part of the damage is converted into HP/SP to the user
# of the skill. Recovered % is the number after "/".
# "NONE" -No extension. Used as a default.
def extension
case @id
when 81
return ["SPDAMAGE"]
when 86
return ["NOOVERKILL"]
when 89
return ["RANDOMTARGET"]
when 94
return ["NOOVERKILL"]
when 96
return ["TARGETALL"]
when 98
return ["NOOVERKILL"]
when 99
return ["HELPHIDE","NOFLASH","FAST"]
when 100
return ["NOOVERKILL"]
when 101
return ["HELPHIDE","NOFLASH","FAST"]
when 102
return ["HELPHIDE","NOFLASH","FAST"]
when 103
return ["HELPHIDE","NOFLASH","FAST"]
end
# Default extensions for unassigned Skill IDs.
return ["NONE"]
end
#--------------------------------------------------------------------------
# ● Skill Throwing Weapon Graphic Settings
#--------------------------------------------------------------------------
# - Allows use of a seperate throwing weapon graphic besides the one assigned
# from Iconset.png. This section is specifically for skills.
#
# return "001-Weapon01" <- Weapon image file name. If "", none is used.
# File must be in the .Graphics\Characters folder
# of your project.
def flying_graphic
case @id
when 1
return ""
end
# Default throwing skill graphic for unassigned Weapon IDs.
return ""
end
end
#==============================================================================
# ■ module RPG
#------------------------------------------------------------------------------
# Item Action Settings
#==============================================================================
class RPG::Item
#--------------------------------------------------------------------------
# ● Item ID Sequence Assignment
#--------------------------------------------------------------------------
def base_action
case @id
when 1
return "ITEM_USE"
end
# Default action sequence for unassigned Item IDs.
return "ITEM_USE"
end
#--------------------------------------------------------------------------
# ● Item Enhancement Extension Settings
#--------------------------------------------------------------------------
# "NOEVADE" -Cannot be evaded regardless.
# "IGNOREREFLECT" -Ignores damage reflection states.
# "HELPHIDE" -Help window when casting will not appear.
# "TARGETALL" -Will affect all enemies and allies simultaneously.
# "RANDOMTARGET" -Target is chosen at random.
# "OTHERS" -Item will not affect caster.
# "NOOVERKILL" -Damage will not be applied after the target reaches zero HP.
# "NOFLASH" -Battler will not flash when taking action.
# "FAST" -Battler will be the first to take action in the turn
# "SLOW" -Battler will be the last to take action in the turn
# "NONE" -No extension. Used as a default.
def extension
case @id
when 1
return ["NONE"]
end
# Default extensions for unassigned Item IDs.
return ["NONE"]
end
end
Code:
#==============================================================================
# Sideview Battle System XP Configurations Version 2.2xp
#==============================================================================
# These configurations are exclusive for the RPG Maker XP
# These are new configuration constants used so the script can work on RMXP
# These are essential for the script to work well and smoothly
# These were separated from the previous configuration to allow you
# to use the whole configurations already settled for RMVX.
# To avoid conflicts with the script's basic configuration,
# it is recomended to place this script below the other configuration
# Some requested functions present in other script were added here
# as basic functions of the script, to avoid incompatibility.
#==============================================================================
module N01
# Character Animation Repeat
NEW_PATTERN_REPEAT = true
# true = XP style, the frames will follow this sequence: 1, 2, 3, 4, 1, 2, 3...
# false = VX style, the frames will follow this sequence: 1, 2, 3, 2, 1, 2, 3...
# Choose the Damage Alogarithm
DAMAGE_ALGORITHM_TYPE = 1
# 0 = Default XP Style, no changes
# 1 = Default XP Modified, def/mdef reduces damage in % ( 10 def = 1% )
# 2 = Default VX, Vitality replaces Dexterity, the status Attack, P.Def and
# M.Def are totally ignored. (Edit the menus script to remove these status)
# 3 = Customized, an mix of XP e VX alogarithm, Vitality replaces Dexterity
# Names of the status Evasion and Vitality (Vitality is only used if
# DAMAGE_ALGORITHM_TYPE > 1
STAT_EVA = "Evasion"
STAT_VIT = "Vitality"
# Define here the character's attack power when unarmed.
UNARMED_ATTACK = 10
# Define here the character's attack animation when unarmed.
UNARMED_ANIM = 4
# No animated battler, remaining the same way as the enemies.
# Only let it as true if you want a battle system with completely not animated
# allies.
NO_ANIM_BATTLER = false
# Show status effects balloons on the battles?
BALLOON_ANIM = true
# Show effects' animations on the battles?
STATE_ANIM = true
# EXP division by the number of members on party
EXP_SHARE = true
# Cursor position on the target
# 0 = customizable
# 1 = below the target
# 2 = above the target (adjusts self to the target's height)
CURSOR_TYPE = 1
# Readjust the cursor's position.
CURSOR_POSITION = [ 0, 0]
# Command Window Position
COMMAND_WINDOW_POSITION = [440, 128]
# Show Battler Name window?
BATTLER_NAME_WINDOW = false
# Effects' icons configuration
# Icons must have the same name of effect plus "_st"
# Ex.: Status Venom, must have an icon named "Venom_st"
Icon_max = 5 # Maximum amount of showed icons
Icon_X = 24 # X size of the icon (width)
Icon_Y = 24 # Y size of the icon (height)
X_Adjust = 0 # Readjustment of the X position
Y_Adjust = 0 # Readjustment of the Y position
# State Cycle times
STATE_CYCLE_TIME = 4
# Damage Exhibition configuration
# Red Green Blue
HP_DMG_COLOR = [255, 255, 255] # HP damage color
HP_REC_COLOR = [176, 255, 144] # HP cure color
SP_DMG_COLOR = [144, 96, 255] # SP damage color
SP_REC_COLOR = [255, 144, 255] # SP cure color
CRT_DMG_COLOR = [255, 144, 96] # Critical damage color
CRT_TXT_COLOR = [255, 96, 0] # Critical damage text color
DAMAGE_FONT = "Arial Black" # Damage exhibition font
DMG_F_SIZE = 32 # Size of the damage exhibition font
DMG_DURATION = 40 # Duration, in frames, that the damage stays on screen
CRITIC_TEXT = true # Show text when critical damage is delt?
CRITIC_FLASH = false # Flash effect when critical damage is dealt?
MULTI_POP = false # Style in which the damage is shown true = normal / false = FF styled
POP_MOVE = false # Moviment for damage exhibition?
DMG_SPACE = 12 # Space between the damage digits
DMG_X_MOVE = 2 # X movement of the damage (only if POP_MOVE = true)
DMG_Y_MOVE = 6 # Y movement of the damage
DMG_GRAVITY = 0.98 # Gravity effect, affects on the heeight the damage "jumps"
# Configurations of the Battle Window
STATUS_OPACITY = 160 # Opacity of the Battle Window
MENU_OPACITY = 160 # Opacity of the Item/Skills window
HELP_OPACITY = 160 # Opacity of the Help Window
COMMAND_OPACITY = 160 # Opacity of the Commands Window
HIDE_WINDOW = true # Hide status window when selecting items/skills?
# Name of the sound file used when a dodge occurs.
# This file must be on the Audio/SE folder of your project
EVASION_EFFECT = "015-Jump01"
# Message shown when a flee attempt succeeds
ESCAPE_SUCCESS = "Ran away!!"
# Message shown when a flee attempt fails
ESCAPE_FAIL = "They're too fast!"
# Allow Ambushes to occur?
BACK_ATTACK = true
# Define here the Ambush occurance rate
BACK_ATTACK_RATE = 10
# Define the message shown when an Ambush occurs
BACK_ATTACK_ALERT = "They got us!"
# Invert the character's position when an ambush occurs?
BACK_ATTACK_MIRROR = false
# Invert the battle background when an Ambush occurs?
BACK_ATTACK_BATTLE_BACK_MIRROR = false
# Here you can configurate the system (itens, skills, switchs) to protect
# the character from Ambushes. The item must be equiped, the skill must be
# learned, and switches must be ON so the Ambush protection works.
# Only one of the 3 need to match the requirements to work.
# In other words, the item can be equiped, but the skill not learned and the
# switch OFF for the item's effect to take place.
# For one item/skill/switch only: = [1]
# For multiple: = [1,2]
# Weapons' ID's
NON_BACK_ATTACK_WEAPONS = []
# Shields' ID's
NON_BACK_ATTACK_ARMOR1 = []
# Helmets' ID's
NON_BACK_ATTACK_ARMOR2 = []
# Armors' ID's
NON_BACK_ATTACK_ARMOR3 = []
# Accesories' ID's
NON_BACK_ATTACK_ARMOR4 = []
# Skills' ID's
NON_BACK_ATTACK_SKILLS = []
# Number of the Switch - when ON, the chance for Ambushes is zero
NO_BACK_ATTACK_SWITCH = []
# Number of the Switch - when ON, the chance for Ambushes is 100%
BACK_ATTACK_SWITCH = []
# Allow Preemptive Attacks to occur?
PREEMPTIVE = true
# Define here the occurance rate of Preemptive Attacks
PREEMPTIVE_RATE = 10
# Define here the message shown when a Preemptive Attack occurs
PREEMPTIVE_ALERT = "Surprised the enemy!"
# Here you can configurate the system (itens, skills, switchs) to increase
# the occurance of Preemptive Attacks. The item must be equiped, the skill must
# be learned, and switches must be ON so the Ambush protection works.
# Only one of the 3 need to match the requirements to work.
# In other words, the item can be equiped, but the skill not learned and the
# switch OFF for the item's effect to take place.
# For one item/skill/switch only: = [1]
# For multiple: = [1,2]
# Weapons' ID's
PREEMPTIVE_WEAPONS = []
# Shields' ID's
PREEMPTIVE_ARMOR1 = []
# Helmets' ID's
PREEMPTIVE_ARMOR2 = []
# Armors' ID's
PREEMPTIVE_ARMOR3 = []
# Accesories' ID's
PREEMPTIVE_ARMOR4 = []
# Skills' ID's
PREEMPTIVE_SKILLS = []
# Number of the Switch - when ON, the chance for Preemptive Attacks is zero
NO_PREEMPTIVE_SWITCH = []
# Number of the Switch - when ON, the chance for Preemptive Attacks is 100%
PREEMPTIVE_SWITCH = []
end
Code:
#==============================================================================
# Sideview Battle System Version 2.2xp
#==============================================================================
#==============================================================================
# ■ Sprite_Battler
#==============================================================================
class Sprite_Battler < RPG::Sprite
#--------------------------------------------------------------------------
APPEAR = 3
DISAPPEAR = 4
COLLAPSE = 5
#--------------------------------------------------------------------------
include N01
#--------------------------------------------------------------------------
def initialize(viewport, battler = nil)
super(viewport)
@battler = battler
@battler_visible = false
@effect_type = 0
@effect_duration = 0
@move_x = 0
@move_y = 0
@move_z = 0
@distanse_x = 0
@distanse_y = 0
@moving_x = 0
@moving_y = 0
@move_speed_x = 0
@move_speed_y = 0
@move_speed_plus_x = 0
@move_speed_plus_y = 0
@move_boost_x = 0
@move_boost_y = 0
@jump_time = 0
@jump_time_plus = 0
@jump_up = 0
@jump_down = 0
@jump_size = 0
@float_time = 0
@float_up = 0
@jump_plus = 0
@angle = 0
@angling = 0
@angle_time = 0
@angle_reset = 0
@zoom_x = 0
@zoom_y = 0
@zooming_x = 0
@zooming_y = 0
@zoom_time = 0
@zoom_reset = 0
@target_battler = []
@now_targets = []
@pattern = 0
@pattern_back = false
@wait = 0
@unloop_wait = 0
@action = []
@anime_kind = 0
@anime_speed = 0
@frame = 0
@anime_loop = 0
@anime_end = false
@anime_freeze = false
@anime_freeze_kind = false
@anime_moving = false
@base_width = ANIME_PATTERN
@base_height = ANIME_KIND
@join = false
@width = 0
@height = 0
@picture_time = 0
@individual_targets = []
@balloon_duration = 65
@reverse = false
return @battler_visible = false if @battler == nil
@anime_flug = true if @battler.actor? && !NO_ANIM_BATTLER
@anime_flug = true if !@battler.actor? && @battler.anime_on
@weapon_R = Sprite_Weapon.new(viewport,@battler) if @anime_flug
make_battler
end
#--------------------------------------------------------------------------
def make_battler
@battler.base_position
@battler_hue = @battler.battler_hue
if @anime_flug
@battler_name = @battler.battler_name if !@battler.actor?
@battler_name = @battler.character_name if @battler.actor?
@battler_hue = @battler.character_hue if @battler.actor?
self.mirror = true if !@battler.actor? && @battler.action_mirror
self.mirror = false if !@battler.actor? && @battler.action_mirror and $back_attack
self.bitmap = RPG::Cache.character(@battler_name, @battler_hue) if WALK_ANIME
begin
self.bitmap = RPG::Cache.character(@battler_name + "_1", @battler_hue) unless WALK_ANIME
rescue
self.bitmap = RPG::Cache.character(@battler_name, @battler_hue) unless WALK_ANIME
end
@width = self.bitmap.width / @base_width
@height = self.bitmap.height / @base_height
@sx = @pattern * @width
@sy = @anime_kind * @height
self.src_rect.set(@sx, @sy, @width, @height)
else
@battler_name = @battler.battler_name
self.bitmap = RPG::Cache.battler(@battler_name, @battler_hue)
@width = bitmap.width
@height = bitmap.height
end
unless @back_attack_flug
if self.mirror && $back_attack
self.mirror = false
elsif $back_attack
self.mirror = true
end
@back_attack_flug = true
end
@battler.reset_coordinate
self.ox = @width / 2
self.oy = @height * 2 / 3
update_move
@move_anime = Sprite_MoveAnime.new(viewport,battler)
@picture = Sprite.new
make_shadow if SHADOW
end
#--------------------------------------------------------------------------
def update_battler_graphic
return if @graphic_change or !WALK_ANIME or @anime_freeze or @battler.dead?
if @battler.actor? and @anime_flug
@battler.base_position
@battler_name = @battler.character_name if @battler.actor?
@battler_hue = @battler.character_hue if @battler.actor?
self.bitmap = RPG::Cache.character(@battler_name, @battler_hue)if WALK_ANIME
begin
self.bitmap = RPG::Cache.character(@battler_name + "_1", @battler_hue) unless WALK_ANIME
rescue
self.bitmap = RPG::Cache.character(@battler_name, @battler_hue) unless WALK_ANIME
end
@width = self.bitmap.width / @base_width
@height = self.bitmap.height / @base_height
@sx = @pattern * @width
@sy = @anime_kind * @height
self.src_rect.set(@sx, @sy, @width, @height)
self.ox = @width / 2
self.oy = @height * 2 / 3
end
unless @back_attack_flug
if self.mirror && $back_attack
self.mirror = false
elsif $back_attack
self.mirror = true
end
@back_attack_flug = true
end
end
#--------------------------------------------------------------------------
def make_shadow
@shadow.dispose if @shadow != nil
@battler_hue = @battler.battler_hue
@shadow = Sprite.new(viewport)
@shadow.z = 200
@shadow.visible = false
@shadow.bitmap = RPG::Cache.character(@battler.shadow, @battler_hue)
@shadow_height = @shadow.bitmap.height
@shadow_plus_x = @battler.shadow_plus[0] - @width / 2
@shadow_plus_y = @battler.shadow_plus[1]
@shadow.zoom_x = @width * 1.0 / @shadow.bitmap.width
update_shadow
@skip_shadow = true
end
#--------------------------------------------------------------------------
def dispose
self.bitmap.dispose if self.bitmap != nil
@weapon_R.dispose if @weapon_R != nil
@move_anime.dispose if @move_anime != nil
@picture.dispose if @picture != nil
@shadow.dispose if @shadow != nil
@balloon.dispose if @balloon != nil
mirage_off
super
end
#--------------------------------------------------------------------------
def damage_action(action)
if action[0] == "absorb"
action[0] = nil
now_hp = @battler.hp
now_sp = @battler.sp
@battler.hp += action[3] if action[2] == "hp"
@battler.sp += action[3] if action[2] == "sp"
@battler.damage = now_hp - @battler.hp if action[2] == "hp"
@battler.damage = now_sp - @battler.sp if action[2] == "sp"
@battler.sp_damage = true if @battler.damage != 0 && action[2] == "sp"
action[2] = false
end
unless @battler.evaded or @battler.missed or action[0] == nil
@battler.animation_id = action[0]
@battler.animation_hit = true
@battler.anime_mirror = action[1]
end
dmg = @battler.damage
dmg = 0 unless dmg.is_a?(Numeric)
start_action(@battler.damage_hit) if dmg > 0 && action[2]
if @battler.evaded or @battler.missed
start_action(@battler.evasion) if action[2]
Audio.se_play("Audio/SE/" + EVASION_EFFECT) if action[2]
end
damage_pop
end
#--------------------------------------------------------------------------
def damage_pop
damage(@battler.damage, @battler.critical, @battler.sp_damage)
@battler.damage = nil
@battler.sp_damage = false
@battler.critical = false
@battler.damage_pop = false
end
#--------------------------------------------------------------------------
def first_action
action = @battler.first_action unless @battler.restriction == 4
action = $data_states[@battler.state_id].base_action if @battler.states[0] != nil && @battler.restriction == 4
start_action(action)
@skip_shadow = false
end
#--------------------------------------------------------------------------
def start_action(kind)
reset
stand_by
@action = ACTION[kind].dup
active = @action.shift
@action.push("End")
@active_action = ANIME[active]
@wait = active.to_i if @active_action == nil
action
end
#--------------------------------------------------------------------------
def start_one_action(kind,back)
reset
stand_by
@action = [back]
@action.push("End")
@active_action = ANIME[kind]
action
end
#--------------------------------------------------------------------------
def next_action
return @wait -= 1 if @wait > 0
return if @anime_end == false
return @unloop_wait -= 1 if @unloop_wait > 0
active = @action.shift
@active_action = ANIME[active]
@wait = active.to_i if @active_action == nil
action
end
#--------------------------------------------------------------------------
def stand_by
@repeat_action = @battler.normal
@repeat_action = @battler.pinch if @battler.hp <= @battler.maxhp / 4
@repeat_action = @battler.defence if @battler.guarding?
unless @battler.state_id == nil
for state in @battler.battler_states.reverse
next if state.extension.include?("NOSTATEANIME")
next if @battler.is_a?(Game_Enemy) && state.extension.include?("EXCEPTENEMY")
@repeat_action = state.base_action
end
end
end
#--------------------------------------------------------------------------
def push_stand_by
action = @battler.normal
action = @battler.pinch if @battler.hp <= @battler.maxhp / 4
action = @battler.defence if @battler.guarding?
for state in @battler.battler_states.reverse
next if state.extension.include?("NOSTATEANIME")
next if @battler.is_a?(Game_Enemy) && state.extension.include?("EXCEPTENEMY")
action = state.base_action
end
@repeat_action = action
@action.delete("End")
act = ACTION[action].dup
for i in 0...act.size
@action.push(act[i])
end
@action.push("End")
@anime_end = true
@angle = self.angle = 0
end
#--------------------------------------------------------------------------
def reset
self.zoom_x = self.zoom_y = 1
self.oy = @height * 2 / 3
@angle = self.angle = 0
@anime_end = true
@non_repeat = false
@anime_freeze = false
@unloop_wait = 0
end
#--------------------------------------------------------------------------
def jump_reset
@battler.jump = @jump_time = @jump_time_plus = @jump_up = @jump_down = 0
@jump_size = @jump_plus = @float_time = @float_up = 0
end
#--------------------------------------------------------------------------
def get_target(target)
return if @battler.individual
@target_battler = target
end
#--------------------------------------------------------------------------
def send_action(action)
@battler.play = 0
@battler.play = action if @battler.active
end
#--------------------------------------------------------------------------
def battler_join
if @battler.exist? && !@battler_visible
return if !@battler.exist? and @battler.is_a?(Game_Enemy)
if @battler.revival && @anime_flug
return @battler.revival = false
elsif @battler.revival && !@anime_flug
@battler.revival = false
return self.visible = true
end
@anime_flug = true if @battler.actor? && !NO_ANIM_BATTLER
@anime_flug = true if !@battler.actor? && @battler.anime_on
make_battler
first_action if !@battler.actor?
end
first_action if @battler.actor? and !@battler_visible
end
#--------------------------------------------------------------------------
def update
super
return self.bitmap = nil && loop_animation(nil) if @battler == nil
battler_join
next_action
update_battler_graphic
update_anime_pattern
update_target
update_force_action
update_move
update_shadow if @shadow != nil
@weapon_R.update if @weapon_action
update_float if @float_time > 0
update_angle if @angle_time > 0
update_zoom if @zoom_time > 0
update_mirage if @mirage_flug
update_picture if @picture_time > 0
update_move_anime if @anime_moving
update_balloon if @balloon_duration <= 64
damage_pop if @battler.damage_pop
setup_new_effect
update_effect
update_battler_bitmap
end
#--------------------------------------------------------------------------
def update_anime_pattern
return @frame -= 1 if @frame != 0
@weapon_R.action if @weapon_action && @weapon_R != nil
if NEW_PATTERN_REPEAT
if @pattern_back
if @anime_loop == 0
if @reverse
@pattern -= 1
@pattern = (@pattern < 0 ? @base_width - 1 : @pattern)
if @pattern == -1
@pattern_back = false
@anime_end = true
end
else
@pattern += 1
@pattern = (@pattern > @base_width - 1 ? 0 : @pattern)
if @pattern == @base_width
@pattern_back = false
@anime_end = true
end
end
else
@anime_end = true
if @anime_loop == 1
@pattern = 0 if !@reverse
@pattern = @base_width - 1 if @reverse
@pattern_back = false
end
end
else
if @reverse
@pattern -= 1
@pattern = (@pattern < 0 ? @base_width - 1 : @pattern)
@pattern_back = true if @pattern == 0
else
@pattern += 1
@pattern = (@pattern > @base_width - 1 ? 0 : @pattern)
@pattern_back = true if @pattern == @base_width - 1
end
end
else
if @pattern_back
if @anime_loop == 0
if @reverse
@pattern += 1
if @pattern == @base_width - 1
@pattern_back = false
@anime_end = true
end
else
@pattern -= 1
if @pattern == 0
@pattern_back = false
@anime_end = true
end
end
else
@anime_end = true
if @anime_loop == 1
@pattern = 0 if !@reverse
@pattern = @base_width - 1 if @reverse
@pattern_back = false
end
end
else
if @reverse
@pattern -= 1
@pattern_back = true if @pattern == 0
else
@pattern += 1
@pattern_back = true if @pattern == @base_width - 1
end
end
end
@frame = @anime_speed
return if @anime_freeze
return unless @anime_flug
@sx = @pattern * @width
@sy = @anime_kind * @height
self.src_rect.set(@sx, @sy, @width, @height)
end
#--------------------------------------------------------------------------
def update_target
return if @battler.force_target == 0
return if @battler.individual
@target_battler = @battler.force_target[1]
@battler.force_target = 0
end
#--------------------------------------------------------------------------
def update_force_action
action = @battler.force_action
return if action == 0
@battler.force_action = 0
return if @battler.active
return collapse_action if action[0] == "N01collapse"
return start_one_action(action[2],action[1]) if action[0] == "SINGLE"
start_action(action[2])
return if action[1] == ""
@action.delete("End")
@action.push(action[1])
@action.push("End")
end
#--------------------------------------------------------------------------
def update_move
if @move_speed_plus_x > 0
@move_x += @moving_x
@battler.move_x = @move_x
@move_speed_plus_x -= 1
elsif @move_speed_x > 0
if @move_boost_x != 0
@moving_x += @move_boost_x
end
@move_x += @moving_x
@battler.move_x = @move_x
@move_speed_x -= 1
end
if @move_speed_plus_y > 0
@move_y += @moving_y
@battler.move_y = @move_y
@move_speed_plus_y -= 1
elsif @move_speed_y > 0
if @move_boost_y != 0
@moving_y += @move_boost_y
end
@move_y += @moving_y
@battler.move_y = @move_y
@move_speed_y -= 1
end
if @jump_up != 0
@jump_plus += @jump_up
@battler.jump = @jump_plus
@jump_up = @jump_up / 2
@jump_time -= 1
if @jump_time == 0 or @jump_up == @jump_sign
@jump_down = @jump_up * 2 * @jump_sign * @jump_sign2
@jump_time_plus += @jump_time * 2
@jump_up = 0
return
end
end
if @jump_down != 0
if @jump_time_plus != 0
@jump_time_plus -= 1
elsif @jump_down != @jump_size
@jump_plus += @jump_down
@battler.jump = @jump_plus
@jump_down = @jump_down * 2
if @jump_down == @jump_size
if @jump_flug
@jump_flug = false
else
@jump_plus += @jump_down
@battler.jump = @jump_plus
@jump_down = @jump_size = 0
end
end
end
end
self.x = @battler.position_x
self.y = @battler.position_y
self.z = @battler.position_z
end
#--------------------------------------------------------------------------
def update_shadow
@shadow.opacity = self.opacity
@shadow.x = self.x + @shadow_plus_x
@shadow.y = self.y + @shadow_plus_y - @jump_plus
end
#--------------------------------------------------------------------------
def update_float
@float_time -= 1
@jump_plus += @float_up
@battler.jump = @jump_plus
end
#--------------------------------------------------------------------------
def update_angle
@angle += @angling
self.angle = @angle
@angle_time -= 1
return @angle = 0 if @angle_time == 0
self.angle = 0 if @angle_reset
end
#--------------------------------------------------------------------------
def update_zoom
@zoom_x += @zooming_x
@zoom_y += @zooming_y
self.zoom_x = @zoom_x
self.zoom_y = @zoom_y
@zoom_time -= 1
return if @zoom_time != 0
@zoom_x = @zoom_y = 0
self.oy = @height
self.zoom_x = self.zoom_y = 1 if @zoom_reset
end
#--------------------------------------------------------------------------
def update_mirage
mirage(@mirage0) if @mirage_count == 1
mirage(@mirage1) if @mirage_count == 3
mirage(@mirage2) if @mirage_count == 5
@mirage_count += 1
@mirage_count = 0 if @mirage_count == 6
end
#--------------------------------------------------------------------------
def update_picture
@picture_time -= 1
@picture.x += @moving_pic_x
@picture.y += @moving_pic_y
end
#--------------------------------------------------------------------------
def update_move_anime
@move_anime.update
@anime_moving = false if @move_anime.finish?
@move_anime.action_reset if @move_anime.finish?
end
#--------------------------------------------------------------------------
def setup_new_effect
if @battler.blink
blink_on
else
blink_off
end
if @battler.white_flash
whiten
@battler.white_flash = false
end
effects_update
if not @battler_visible and @battler.exist?
@effect_type = APPEAR
@effect_duration = 16
@battler_visible = true
end
if @battler_visible and @battler.hidden
@effect_type = DISAPPEAR
@effect_duration = 32
@battler_visible = false
end
if @battler.collapse
@effect_type = COLLAPSE
@effect_duration = 48
@battler.collapse = false
@battler_visible = false
end
if @battler_visible && @battler.animation_id != 0
animation = $data_animations[@battler.animation_id]
@battler.animation_hit = true unless @battler.evaded or @battler.missed
animation(animation, @battler.animation_hit)
if @active_battler.is_a?(Game_Enemy)
if @battler.actor? and @battler.anime_mirror
@battler.anime_mirror = false
elsif @battler.actor? and !@battler.anime_mirror
@battler.anime_mirror = true
end
end
animation_mirror(@battler.anime_mirror)
@battler.animation_id = 0
@battler.anime_mirror = false
end
end
#--------------------------------------------------------------------------
def effects_update
if @battler.damage == nil and @battler.state_animation_id != @state_animation_id and STATE_ANIM and @battler_visible
@state_animation_id = @battler.state_animation_id == nil ? 0 : @battler.state_animation_id
loop_animation($data_animations[@state_animation_id])
end
end
#--------------------------------------------------------------------------
def update_effect
if @effect_duration > 0
@effect_duration -= 1
case @effect_type
when APPEAR
update_appear
when DISAPPEAR
update_disappear
when COLLAPSE
update_collapse
end
end
end
#--------------------------------------------------------------------------
def update_whiten
self.blend_type = 0
self.color.set(255, 255, 255, 128)
self.opacity = 255
self.color.alpha = 128 - (16 - @effect_duration) * 10
end
#--------------------------------------------------------------------------
def update_appear
self.blend_type = 0
self.color.set(0, 0, 0, 0)
self.opacity = (16 - @effect_duration) * 16
end
#--------------------------------------------------------------------------
def update_disappear
self.blend_type = 0
self.color.set(0, 0, 0, 0)
self.opacity = 256 - (32 - @effect_duration) * 10
end
#--------------------------------------------------------------------------
def update_collapse
normal_collapse if @collapse_type == 2
boss_collapse if @collapse_type == 3
end
#--------------------------------------------------------------------------
def update_balloon
@balloon_duration -= 1 if @balloon_duration > 0 && !@balloon_back
@balloon_duration += 1 if @balloon_back
if @balloon_duration == 64
@balloon_back = false
@balloon.visible = false
elsif @balloon_duration == 0
@balloon.visible = false if @balloon_loop == 0
@balloon_back = true if @balloon_loop == 1
end
@balloon.x = self.x
@balloon.y = self.y
@balloon.z = self.y
@balloon.opacity = self.opacity
sx = 7 * 32 if @balloon_duration < 12
sx = (7 - (@balloon_duration - 12) / 8) * 32 unless @balloon_duration < 12
@balloon.src_rect.set(sx, @balloon_id * 32, 32, 32)
@balloon.visible = false if @battler.dead?
end
#--------------------------------------------------------------------------
def update_battler_bitmap
return if @graphic_change
return if @battler.actor?
if @battler.battler_name != @battler_name or @battler.battler_hue != @battler_hue
@battler_name = @battler.battler_name
@battler_hue = @battler.battler_hue
make_battler
self.opacity = 0 if @battler.dead? or @battler.hidden
end
end
#--------------------------------------------------------------------------
def action
return if @active_action == nil
action = @active_action[0]
return mirroring if action == "Invert"
return angling if action == "angle"
return zooming if action == "zoom"
return mirage_on if action == "Afterimage ON"
return mirage_off if action == "Afterimage OFF"
return picture if action == "pic"
return @picture.visible = false && @picture_time = 0 if action == "Clear image"
return graphics_change if action == "change"
return battle_anime if action == "anime"
return balloon_anime if action == "balloon"
return sound if action == "sound"
return $game_switches[@active_action[1]] = @active_action[2] if action == "switch"
return variable if action == "variable"
return two_swords if action == "Two Wpn Only"
return non_two_swords if action == "One Wpn Only"
return necessary if action == "nece"
return derivating if action == "der"
return individual_action if action == "Process Skill"
return individual_action_end if action == "Process Skill End"
return non_repeat if action == "Don't Wait"
return @battler.change_base_position(self.x, self.y) if action == "Start Pos Change"
return @battler.base_position if action == "Start Pos Return"
return change_target if action == "target"
return send_action(action) if action == "Can Collapse"
return send_action(action) if action == "Cancel Action"
return state_on if action == "sta+"
return state_off if action == "sta-"
return Graphics.frame_rate = @active_action[1] if action == "fps"
return floating if action == "float"
return eval(@active_action[1]) if action == "script"
return force_action if @active_action.size == 4
return reseting if @active_action.size == 5
return moving if @active_action.size == 7
return battler_anime if @active_action.size == 9
return moving_anime if @active_action.size == 11
return anime_finish if action == "End"
end
#--------------------------------------------------------------------------
def mirroring
if self.mirror
self.mirror = false
@weapon_R.mirroring if @anime_flug
else
self.mirror = true
@weapon_R.mirroring if @anime_flug
end
end
#--------------------------------------------------------------------------
def angling
jump_reset
@angle_time = @active_action[1]
start_angle = @active_action[2]
end_angle = @active_action[3]
@angle_reset = @active_action[4]
start_angle *= -1 if $back_attack
end_angle *= -1 if $back_attack
start_angle *= -1 if @battler.is_a?(Game_Enemy)
end_angle *= -1 if @battler.is_a?(Game_Enemy)
if @angle_time <= 0
self.angle = end_angle
return @angle_time = 0
end
@angling = (end_angle - start_angle) / @angle_time
@angle = (end_angle - start_angle) % @angle_time + start_angle
end
#--------------------------------------------------------------------------
def zooming
jump_reset
@zoom_time = @active_action[1]
zoom_x = @active_action[2] - 1
zoom_y = @active_action[3] - 1
@zoom_reset = @active_action[4]
@zoom_x = @zoom_y = 1
return @zoom_time = 0 if @zoom_time <= 0
@zooming_x = zoom_x / @zoom_time
@zooming_y = zoom_y / @zoom_time
end
#--------------------------------------------------------------------------
def mirage_on
return if @battler.dead?
@mirage0 = Sprite.new(self.viewport)
@mirage1 = Sprite.new(self.viewport)
@mirage2 = Sprite.new(self.viewport)
@mirage_flug = true
@mirage_count = 0
end
#--------------------------------------------------------------------------
def mirage(body)
body.bitmap = self.bitmap.dup
body.x = self.x
body.y = self.y
body.ox = self.ox
body.oy = self.oy
body.z = self.z
body.mirror = self.mirror
body.angle = @angle
body.opacity = 160
body.zoom_x = self.zoom_x
body.zoom_y = self.zoom_y
body.src_rect.set(@sx, @sy, @width, @height) if @anime_flug
body.src_rect.set(0, 0, @width, @height) unless @anime_flug
end
#--------------------------------------------------------------------------
def mirage_off
@mirage_flug = false
@mirage0.dispose if @mirage0 != nil
@mirage1.dispose if @mirage1 != nil
@mirage2.dispose if @mirage2 != nil
end
#--------------------------------------------------------------------------
def picture
pic_x = @active_action[1]
pic_y = @active_action[2]
pic_end_x = @active_action[3]
pic_end_y = @active_action[4]
@picture_time = @active_action[5]
@moving_pic_x = (pic_end_x - pic_x)/ @picture_time
@moving_pic_y = (pic_end_y - pic_y)/ @picture_time
plus_x = (pic_end_x - pic_x)% @picture_time
plus_y = (pic_end_y - pic_y)% @picture_time
@picture.bitmap = RPG::Cache.picture(@active_action[7])
@picture.x = pic_x + plus_x
@picture.y = pic_y + plus_y
@picture.z = 1
@picture.z = 1900
@picture.z = 3000 if @active_action[6]
@picture.visible = true
end
#--------------------------------------------------------------------------
def graphics_change
return if @battler.is_a?(Game_Enemy)
@battler_name = @active_action[2]
@bitmap = RPG::Cache.character(@battler_name , @battler_hue) if WALK_ANIME
@bitmap = RPG::Cache.character(@battler_name + "_1", @battler_hue) unless WALK_ANIME
@width = @bitmap.width / @base_width
@height = @bitmap.height / @base_height
@battler.graphic_change(@active_action[2]) unless @active_action[1]
@graphic_change = true
end
#--------------------------------------------------------------------------
def battle_anime
return if @active_action[5] && !@battler.actor?
return if @active_action[5] && @battler.weapons[1] == nil
if @battler.actor?
return if !@active_action[5] && @battler.weapons[0] == nil && @battler.weapons[1] != nil
end
anime_id = @active_action[1]
if $back_attack
mirror = true if @active_action[3] == false
mirror = false if @active_action[3]
end
if anime_id < 0
if @battler.current_action.kind == 1 && anime_id != -2
anime_id = $data_skills[@battler.current_action.skill_id].animation2_id
elsif @battler.current_action.kind == 2 && anime_id != -2
anime_id = $data_items[@battler.current_action.item_id].animation2_id
else
anime_id = NO_WEAPON
if @battler.actor?
weapon_id = @battler.weapon_id
anime_id = UNARMED_ANIM
anime_id = battler.weapons[0].animation2_id if battler.weapons[0] != nil
anime_id = battler.weapons[1].animation2_id if @active_action[5]
else
weapon_id = @battler.weapon
anime_id = $data_weapons[weapon_id].animation2_id if weapon_id != 0
end
end
@wait = $data_animations[anime_id].frame_max if $data_animations[anime_id] != nil && @active_action[4]
waitflug = true
damage_action = [anime_id, mirror, true]
return @battler.play = ["OBJ_ANIM",damage_action] if @battler.active
end
if @active_action[2] == 0 && $data_animations[anime_id] != nil
@battler.animation_id = anime_id
@battler.animation_hit = true
@battler.anime_mirror = mirror
elsif $data_animations[anime_id] != nil
for target in @target_battler
target.animation_id = anime_id
target.anime_mirror = mirror
end
end
@wait = $data_animations[anime_id].frame_max if $data_animations[anime_id] != nil && @active_action[4] && !waitflug
end
#--------------------------------------------------------------------------
def sound
pitch = @active_action[2]
vol = @active_action[3]
name = @active_action[4]
case @active_action[1]
when "se"
Audio.se_play("Audio/SE/" + name, vol, pitch)
when "bgm"
if @active_action[4] == ""
now_bgm = RPG::BGM.last
name = now_bgm.name
end
Audio.bgm_play("Audio/BGM/" + name, vol, pitch)
when "bgs"
if @active_action[4] == ""
now_bgs = RPG::BGS.last
name = now_bgs.name
end
Audio.bgs_play("Audio/BGS/" + name, vol, pitch)
end
end
#--------------------------------------------------------------------------
def balloon_anime
return if self.opacity == 0
if @balloon == nil
@balloon = Sprite.new
@balloon.bitmap = RPG::Cache.picture("Balloon")
@balloon.ox = @width / 16
@balloon.oy = 320 / 10 + @height / 3
end
@balloon_id = @active_action[1]
@balloon_loop = @active_action[2]
@balloon_duration = 64
@balloon_back = false
update_balloon
@balloon.visible = true
@balloon.visible = false unless BALLOON_ANIM
end
#--------------------------------------------------------------------------
def variable
operand = @active_action[3]
case @active_action[2]
when 0
$game_variables[@active_action[1]] = operand
when 1
$game_variables[@active_action[1]] += operand
when 2
$game_variables[@active_action[1]] -= operand
when 3
$game_variables[@active_action[1]] *= operand
when 4
$game_variables[@active_action[1]] /= operand
when 5
$game_variables[@active_action[1]] %= operand
end
end
#--------------------------------------------------------------------------
def two_swords
return @action.shift unless @battler.actor?
return @action.shift if @battler.weapons[1] == nil
active = @action.shift
@active_action = ANIME[active]
@wait = active.to_i if @active_action == nil
action
end
#--------------------------------------------------------------------------
def non_two_swords
return unless @battler.actor?
return @action.shift if @battler.weapons[1] != nil
active = @action.shift
@active_action = ANIME[active]
@wait = active.to_i if @active_action == nil
action
end
#--------------------------------------------------------------------------
def necessary
nece1 = @active_action[3]
nece2 = @active_action[4]
case @active_action[1]
when 0
target = [$game_party.actors[@battler.index]] if @battler.actor?
target = [$game_troop.enemies[@battler.index]] if @battler.is_a?(Game_Enemy)
when 1
target = @target_battler
when 2
target = $game_troop.enemies
when 3
target = $game_party.actors
end
return start_action(@battler.recover_action) if target.size == 0
case @active_action[2]
when 0
state_on = true if nece2 > 0
state_member = nece2.abs
if nece2 == 0
state_member = $game_party.actors.size if @battler.actor?
state_member = $game_troop.enemies.size if @battler.is_a?(Game_Enemy)
end
for member in target
state_member -= 1 if member.state?(nece1)
end
if state_member == 0 && state_on
return
elsif state_member == nece2.abs
return if state_on == nil
end
when 1
num_over = true if nece2 > 0
num = 0
for member in target
case nece1
when 0
num += member.hp
when 1
num += member.mp
when 2
num += member.atk
when 3
num += member.dex
when 4
num += member.agi
when 5
num += member.int
end
end
num = num / target.size
if num > nece2.abs && num_over
return
elsif num < nece2.abs
return if num_over == nil
end
when 2
if $game_switches[nece1]
return if nece2
else
return unless nece2
end
when 3
if nece2 > 0
return if $game_variables[nece1] > nece2
else
return unless $game_variables[nece1] > nece2.abs
end
when 4
skill_member = nece2.abs
for member in target
skill_member -= 1 if member.skill_learn?(nece1)
return if skill_member == 0
end
end
return @action = ["End"] if @non_repeat
action = @battler.recover_action
action = @battler.defence if @battler.guarding?
return start_action(action)
end
#--------------------------------------------------------------------------
def derivating
return unless @active_action[2] && !@battler.skill_learn?(@active_action[3])
return if rand(100) > @active_action[1]
@battler.derivation = @active_action[3]
@action = ["End"]
end
#--------------------------------------------------------------------------
def individual_action
@battler.individual = true
@individual_act = @action.dup
send_action(["Individual"])
@individual_targets = @target_battler.dup
@target_battler = [@individual_targets.shift]
end
#--------------------------------------------------------------------------
def individual_action_end
return @battler.individual = false if @individual_targets.size == 0
@action = @individual_act.dup
@target_battler = [@individual_targets.shift]
end
#--------------------------------------------------------------------------
def non_repeat
@repeat_action = []
@non_repeat = true
anime_finish
end
#--------------------------------------------------------------------------
def change_target
return @target_battler = @now_targets.dup if @active_action[2] == 3
target = [@battler] if @active_action[2] == 0
target = @target_battler.dup if @active_action[2] != 0
if @active_action[2] == 2
@now_targets = @target_battler.dup
@target_battler = []
end
if @active_action[1] >= 1000
members = $game_party.actors if @battler.actor?
members = $game_troop.enemies unless @battler.actor?
index = @active_action[1] - 1000
if index < members.size
if members[index].exist? && @battler.index != index
members[index].force_target = ["N01target_change", target]
@target_battler = [members[index]] if @active_action[2] == 2
change = true
else
for member in members
next if @battler.index == member.index
next unless member.exist?
member.force_target = ["N01target_change", target]
@target_battler = [member] if @active_action[2] == 2
break change = true
end
end
end
elsif @active_action[1] > 0
for member in $game_party.actors + $game_troop.enemies
if member.state?(@active_action[1])
member.force_target = ["N01target_change", target]
@target_battler.push(member) if @active_action[2] == 2
change = true
end
end
elsif @active_action[1] < 0
skill_id = @active_action[1].abs
for actor in $game_party.actors
if actor.skill_learn?(skill_id)
actor.force_target = ["N01target_change", target]
@target_battler.push(target) if @active_action[2] == 2
change = true
end
end
else
for member in @target_battler
member.force_target = ["N01target_change", target]
@target_battler.push(member) if @active_action[2] == 2
change = true
end
end
return if change
return @action = ["End"] if @non_repeat
return start_action(@battler.recover_action)
end
#--------------------------------------------------------------------------
def state_on
state_id = @active_action[2]
case @active_action[1]
when 0
@battler.add_state(state_id)
when 1
if @target_battler != nil
for target in @target_battler
target.add_state(state_id)
end
end
when 2
for target in $game_troop.enemies
target.add_state(state_id)
end
when 3
for target in $game_party.actors
target.add_state(state_id)
end
when 4
for target in $game_party.actors
if target.index != @battler.index
target.add_state(state_id)
end
end
end
start_action(@battler.recover_action) unless @battler.movable?
end
#--------------------------------------------------------------------------
def state_off
state_id = @active_action[2]
case @active_action[1]
when 0
@battler.remove_state(state_id)
when 1
if @target_battler != nil
for target in @target_battler
target.remove_state(state_id)
end
end
when 2
for target in $game_troop.enemies
target.remove_state(state_id)
end
when 3
for target in $game_party.actors
target.remove_state(state_id)
end
when 4
for target in $game_party.actors
if target.index != @battler.index
target.remove_state(state_id)
end
end
end
end
#--------------------------------------------------------------------------
def floating
jump_reset
@jump_plus = @active_action[1]
float_end = @active_action[2]
@float_time = @active_action[3]
@float_up = (float_end - @jump_plus)/ @float_time
@wait = @float_time
if @anime_flug
move_anime = ANIME[@active_action[4]]
if move_anime != nil
@active_action = move_anime
battler_anime
@anime_end = true
end
end
@battler.jump = @jump_plus
end
#--------------------------------------------------------------------------
def force_action
kind = @active_action[0]
rebirth = @active_action[2]
play = @active_action[3]
action = [kind,rebirth,play]
if @active_action[1] >= 1000
members = $game_party.actors if @battler.actor?
members = $game_troop.enemies unless @battler.actor?
index = @active_action[1] - 1000
if index < members.size
if members[index].exist? && @battler.index != index
return members[index].force_action = action
else
for target in members
next if @battler.index == target.index
next unless target.exist?
force = true
break target.force_action = action
end
end
end
return if force
return @action = ["End"] if @non_repeat
return start_action(@battler.recover_action)
elsif @active_action[1] == 0
for target in @target_battler
target.force_action = action if target != nil
end
elsif @active_action[1] > 0
for target in $game_party.actors + $game_troop.enemies
target.force_action = action if target.state?(@active_action[1])
end
elsif @active_action[1] < 0
return if @battler.is_a?(Game_Enemy)
for actor in $game_party.actors
unless actor.id == @battler.id
actor.force_action = action if actor.skill_id_learn?(@active_action[1].abs)
end
end
end
end
#--------------------------------------------------------------------------
def reseting
jump_reset
self.angle = 0
@distanse_x = @move_x * -1
@distanse_y = @move_y * -1
@move_speed_x = @active_action[1]
@move_speed_y = @move_speed_x
@move_boost_x = @active_action[2]
@move_boost_y = @move_boost_x
@jump = @active_action[3]
move_distance
if @anime_flug
move_anime = ANIME[@active_action[4]]
if move_anime != nil
@active_action = move_anime
battler_anime
end
@anime_end = true
end
end
#--------------------------------------------------------------------------
def moving
jump_reset
xx = @active_action[1]
xx *= -1 if $back_attack
case @active_action[0]
when 0
@distanse_x = xx
@distanse_y = @active_action[2]
when 1
if @target_battler == nil
@distanse_x = xx
@distanse_y = @active_action[2]
else
target_x = 0
target_y = 0
time = 0
for i in 0...@target_battler.size
if @target_battler[i] != nil
time += 1
target_x += @target_battler[i].position_x
target_y += @target_battler[i].position_y
end
end
if time == 0
@distanse_x = xx
@distanse_y = @active_action[2]
else
target_x = target_x / time
target_y = target_y / time
@distanse_y = target_y - self.y + @active_action[2]
if @battler.actor?
@distanse_x = target_x - self.x + xx
else
@distanse_x = self.x - target_x + xx
end
end
end
when 2
if @battler.actor?
@distanse_x = xx - self.x
@distanse_x = 640 + xx - self.x if $back_attack
else
@distanse_x = self.x - xx
@distanse_x = self.x - (Graphics.width + xx) if $back_attack
end
@distanse_y = @active_action[2] - self.y
when 3
if @battler.actor?
@distanse_x = xx + @battler.base_position_x - self.x
else
@distanse_x = xx + self.x - @battler.base_position_x
end
@distanse_y = @active_action[2] + @battler.base_position_y - @battler.position_y
end
@move_speed_x = @active_action[3]
@move_speed_y = @active_action[3]
@move_boost_x = @active_action[4]
@move_boost_y = @active_action[4]
@jump = @active_action[5]
@jump_plus = 0
move_distance
if @anime_flug
move_anime = ANIME[@active_action[6]]
if move_anime != nil
@active_action = move_anime
battler_anime
end
@anime_end = true
end
end
#--------------------------------------------------------------------------
def move_distance
if @move_speed_x == 0
@moving_x = 0
@moving_y = 0
else
@moving_x = @distanse_x / @move_speed_x
@moving_y = @distanse_y / @move_speed_y
over_x = @distanse_x % @move_speed_x
over_y = @distanse_y % @move_speed_y
@move_x += over_x
@move_y += over_y
@battler.move_x = @move_x
@battler.move_y = @move_y
@distanse_x -= over_x
@distanse_y -= over_y
end
if @distanse_x == 0
@move_speed_x = 0
end
if @distanse_y == 0
@move_speed_y = 0
end
boost_x = @moving_x
move_x = 0
if @move_boost_x > 0 && @distanse_x != 0
if @distanse_x == 0
@move_boost_x = 0
elsif @distanse_x < 0
@move_boost_x *= -1
end
for i in 0...@move_speed_x
boost_x += @move_boost_x
move_x += boost_x
over_distance = @distanse_x - move_x
if @distanse_x > 0 && over_distance < 0
@move_speed_x = i
break
elsif @distanse_x < 0 && over_distance > 0
@move_speed_x = i
break
end
end
before = over_distance + boost_x
@move_speed_plus_x = (before / @moving_x).abs
@move_x += before % @moving_x
@battler.move_x = @move_x
elsif @move_boost_x < 0 && @distanse_x != 0
if @distanse_x == 0
@move_boost_x = 0
elsif @distanse_x < 0
@move_boost_x *= -1
end
for i in 0...@move_speed_x
boost_x += @move_boost_x
move_x += boost_x
lost_distance = @distanse_x - move_x
before = lost_distance
if @distanse_x > 0 && boost_x < 0
@move_speed_x = i - 1
before = lost_distance + boost_x
break
elsif @distanse_x < 0 && boost_x > 0
@move_speed_x= i - 1
before = lost_distance + boost_x
break
end
end
plus = before / @moving_x
@move_speed_plus_x = plus.abs
@move_x += before % @moving_x
@battler.move_x = @move_x
end
boost_y = @moving_y
move_y = 0
if @move_boost_y > 0 && @distanse_y != 0
if @distanse_y == 0
@move_boost_y = 0
elsif @distanse_y < 0
@move_boost_y *= -1
end
for i in 0...@move_speed_y
boost_y += @move_boost_y
move_y += boost_y
over_distance = @distanse_y - move_y
if @distanse_y > 0 && over_distance < 0
@move_speed_y = i
break
elsif @distanse_y < 0 && over_distance > 0
@move_speed_y = i
break
end
end
before = over_distance + boost_y
@move_speed_plus_y = (before / @moving_y).abs
@move_y += before % @moving_y
@battler.move_y = @move_y
elsif @move_boost_y < 0 && @distanse_y != 0
if @distanse_y == 0
@move_boost_y = 0
elsif @distanse_y < 0
@move_boost_y *= -1
end
for i in 0...@move_speed_y
boost_y += @move_boost_y
move_y += boost_y
lost_distance = @distanse_y - move_y
before = lost_distance
if @distanse_y > 0 && boost_y < 0
@move_speed_y = i
before = lost_distance + boost_y
break
elsif @distanse_y < 0 && boost_y > 0
@move_speed_y = i
before = lost_distance + boost_y
break
end
end
plus = before / @moving_y
@move_speed_plus_y = plus.abs
@move_y += before % @moving_y
@battler.move_y = @move_y
end
x = @move_speed_plus_x + @move_speed_x
y = @move_speed_plus_y + @move_speed_y
if x > y
end_time = x
else
end_time = y
end
@wait = end_time
if @jump != 0
if @wait == 0
@wait = @active_action[3]
end
@jump_time = @wait / 2
@jump_time_plus = @wait % 2
@jump_sign = 0
@jump_sign2 = 0
if @jump < 0
@jump_sign = -1
@jump_sign2 = 1
@jump = @jump * -1
else
@jump_sign = 1
@jump_sign2 = -1
end
@jump_up = 2 ** @jump * @jump_sign
if @jump_time == 0
@jump_up = 0
elsif @jump_time != 1
@jump_size = @jump_up * @jump_sign * @jump_sign2
else
@jump_size = @jump_up * 2 * @jump_sign * @jump_sign2
@jump_flug = true
end
end
end
#--------------------------------------------------------------------------
def battler_anime
@anime_kind = @active_action[1]
@anime_speed = @active_action[2]
@anime_loop = @active_action[3]
@unloop_wait = @active_action[4]
@anime_end = true
@reverse = false
if @weapon_R != nil && @active_action[8] != ""
weapon_kind = ANIME[@active_action[8]]
two_swords_flug = weapon_kind[11]
return if two_swords_flug && !@battler.actor?
return if two_swords_flug && @battler.weapons[1] == nil && @battler.actor?
if @battler.actor? && @battler.weapons[0] == nil && !two_swords_flug
@weapon_R.action_reset
elsif @battler.actor? && @battler.weapons[1] == nil && two_swords_flug
@weapon_R.action_reset
elsif !@battler.actor? && @battler.weapon == 0
@weapon_R.action_reset
else
@weapon_R.action_reset
if @active_action[5] != -1
@weapon_R.freeze(@active_action[5])
end
@weapon_R.weapon_graphics unless two_swords_flug
@weapon_R.weapon_graphics(true) if two_swords_flug
@weapon_R.weapon_action(@active_action[8],@anime_loop)
@weapon_action = true
@weapon_R.action
end
elsif @weapon_R != nil
@weapon_R.action_reset
end
@anime_end = false
if @active_action[5] != -1 && @active_action[5] != -2
@anime_freeze = true
@anime_end = true
elsif @active_action[5] == -2
@anime_freeze = false
@reverse = true
@pattern = @base_width - 1
if @weapon_action && @weapon_R != nil
@weapon_R.action
@weapon_R.update
end
else
@anime_freeze = false
@pattern = 0
if @weapon_action && @weapon_R != nil
@weapon_R.action
@weapon_R.update
end
end
@pattern_back = false
@frame = @anime_speed
@battler.move_z = @active_action[6]
if @shadow != nil
@shadow.visible = true if @active_action[7]
@shadow.visible = false unless @active_action[7]
@shadow.visible = false if @skip_shadow
end
file_name = ""
unless @active_action[0] == 0
file_name = "_" + @active_action[0].to_s
end
return unless @anime_flug
begin
self.bitmap = RPG::Cache.character(@battler_name + file_name, @battler_hue)
rescue
self.bitmap = RPG::Cache.character(@battler_name, @battler_hue)
end
@sx = @pattern * @width
@sy = @anime_kind * @height
@sx = @active_action[5] * @width if @anime_freeze
self.src_rect.set(@sx, @sy, @width, @height)
end
#--------------------------------------------------------------------------
def moving_anime
@move_anime.action_reset if @anime_moving
@anime_moving = true
mirror = false
mirror = true if $back_attack
id = @active_action[1]
target = @active_action[2]
x = y = mem = 0
if target == 0
if @target_battler == nil
x = self.x
y = self.y
else
if @target_battler[0] == nil
x = self.x
y = self.y
else
x = @target_battler[0].position_x
y = @target_battler[0].position_y
end
end
elsif target == 1
if @battler.actor?
for target in $game_troop.enemies
x += target.position_x
y += target.position_y
mem += 1
end
x = x / mem
y = y / mem
else
for target in $game_party.actors
x += target.position_x
y += target.position_y
mem += 1
end
x = x / mem
y = y / mem
end
elsif target == 2
if @battler.actor?
for target in $game_party.actors
x += target.position_x
y += target.position_y
mem += 1
end
x = x / mem
y = y / mem
else
for target in $game_troop.enemies
x += target.position_x
y += target.position_y
mem += 1
end
x = x / mem
y = y / mem
end
else
x = self.x
y = self.y
end
plus_x = @active_action[6]
plus_y = @active_action[7]
plus_x *= -1 if @battler.is_a?(Game_Enemy)
distanse_x = x - self.x - plus_x
distanse_y = y - self.y - plus_y
type = @active_action[3]
speed = @active_action[4]
orbit = @active_action[5]
if @active_action[8] == 0
@move_anime.base_x = self.x + plus_x
@move_anime.base_y = self.y + plus_y
elsif @active_action[8] == 1
@move_anime.base_x = x + plus_x
@move_anime.base_y = y + plus_y
distanse_y = distanse_y * -1
distanse_x = distanse_x * -1
else
@move_anime.base_x = x
@move_anime.base_y = y
distanse_x = distanse_y = 0
end
if @active_action[10] == ""
weapon = ""
elsif @anime_flug != true
weapon = ""
else
if @battler.actor?
battler = $game_party.actors[@battler.index]
weapon_id = battler.weapon_id
else
battler = $game_troop.enemies[@battler.index]
weapon_id = battler.weapon
end
weapon_act = ANIME[@active_action[10]].dup if @active_action[10] != ""
if weapon_id != 0 && weapon_act.size == 3
weapon_file = $data_weapons[weapon_id].flying_graphic
if weapon_file == ""
weapon_name = $data_weapons[weapon_id].graphic
icon_weapon = false
if weapon_name == ""
weapon_name = $data_weapons[weapon_id].icon_name
icon_weapon = true
end
else
icon_weapon = false
weapon_name = weapon_file
end
weapon = @active_action[10]
elsif weapon_act.size == 3
weapon = ""
elsif weapon_act != nil && $data_skills[@active_battler.current_action.skill_id] != nil
icon_weapon = false
weapon_name = $data_skills[@battler.current_action.skill.id].flying_graphic
weapon = @active_action[10]
end
end
@move_anime.z = 1
@move_anime.z = 1000 if @active_action[9]
@move_anime.anime_action(id,mirror,distanse_x,distanse_y,type,speed,orbit,weapon,weapon_name,icon_weapon)
end
#--------------------------------------------------------------------------
def anime_finish
return individual_action_end if @individual_targets.size != 0
send_action(@active_action[0]) if @battler.active
mirage_off if @mirage_flug
start_action(@repeat_action) unless @non_repeat
end
#--------------------------------------------------------------------------
def collapse_action
@non_repeat = true
@effect_type = COLLAPSE
@collapse_type = @battler.collapse_type unless @battler.actor?
@battler_visible = false unless @battler.actor?
@effect_duration = COLLAPSE_WAIT + 32 if @collapse_type == 2
@effect_duration = 360 if @collapse_type == 3
end
#--------------------------------------------------------------------------
def normal_collapse
if @effect_duration == 31
$game_system.se_play($data_system.enemy_collapse_se)
self.blend_type = 1
self.color.set(255, 64, 64, 255)
end
self.opacity = 256 - (48 - @effect_duration) * 6 if @effect_duration <= 31
end
#--------------------------------------------------------------------------
def boss_collapse
if @effect_duration == 320
Audio.se_play("Audio/SE/124-Thunder02", 100, 80)
self.flash(Color.new(255, 255, 255), 60)
viewport.flash(Color.new(255, 255, 255), 20)
end
if @effect_duration == 290
Audio.se_play("Audio/SE/124-Thunder02", 100, 80)
self.flash(Color.new(255, 255, 255), 60)
viewport.flash(Color.new(255, 255, 255), 20)
end
if @effect_duration == 250
Audio.se_play("Audio/SE/049-Explosion02",100, 50)
reset
self.blend_type = 1
self.color.set(255, 128, 128, 128)
end
if @effect_duration < 250
self.src_rect.set(0, @effect_duration - 250, @width, @height - @shadow.bitmap.height / 2)
self.x += 10 if @effect_duration % 2 == 0
self.opacity = @effect_duration - 20
return if @effect_duration < 100
Audio.se_play("Audio/SE/049-Explosion02",100, 50) if @effect_duration % 80 == 0
end
end
end
Code:
#==============================================================================
# Sideview Battle System Version 2.2xp
#==============================================================================
#==============================================================================
# ■ Atoa Module
#==============================================================================
$atoa_script = {} if $atoa_script.nil?
$atoa_script["SBS Tankentai"] = true
#==============================================================================
# ■ Scene_Battle
#==============================================================================
class Scene_Battle
#--------------------------------------------------------------------------
include N01
#--------------------------------------------------------------------------
attr_accessor :spriteset
#--------------------------------------------------------------------------
def main
fix_weapon_init
start
create_viewport
process_transition
update_battle
terminate
fix_weapon_end
end
#--------------------------------------------------------------------------
def start
@battle_start = true
$game_temp.in_battle = true
$game_temp.battle_turn = 0
$game_temp.battle_event_flags.clear
$game_temp.battle_abort = false
$game_temp.battle_main_phase = false
$game_temp.battleback_name = $game_map.battleback_name
$game_temp.forcing_battler = nil
$game_system.battle_interpreter.setup(nil, 0)
@troop_id = $game_temp.battle_troop_id
$game_troop.setup(@troop_id)
for enemy in $game_troop.enemies
enemy.true_immortal = enemy.immortal
end
end
#--------------------------------------------------------------------------
def create_viewport
s1 = $data_system.words.attack
s2 = $data_system.words.skill
s3 = $data_system.words.guard
s4 = $data_system.words.item
@actor_command_window = Window_Command.new(160, [s1, s2, s3, s4])
@actor_command_window.y = 160
@actor_command_window.back_opacity = 160
@actor_command_window.active = false
@actor_command_window.visible = false
@party_command_window = Window_PartyCommand.new
@help_window = Window_Help.new
@help_window.back_opacity = 160
@help_window.visible = false
@active_battler_window = Window_NameCommand.new(@active_battler, 240, 64)
@active_battler_window.visible = false
@status_window = Window_BattleStatus.new
@message_window = Window_Message.new
@spriteset = Spriteset_Battle.new
@wait_count, @escape_ratio = 0, 50
end
#--------------------------------------------------------------------------
def process_transition
if $data_system.battle_transition == ""
Graphics.transition(20)
else
Graphics.transition(40, "Graphics/Transitions/" + $data_system.battle_transition)
end
start_phase1
end
#--------------------------------------------------------------------------
def update_battle
loop do
Graphics.update
Input.update
update
break if $scene != self
end
end
#--------------------------------------------------------------------------
def terminate
$game_map.refresh
Graphics.freeze
@actor_command_window.dispose
@party_command_window.dispose
@help_window.dispose
@status_window.dispose
@message_window.dispose
@skill_window.dispose if @skill_window != nil
@item_window.dispose if @item_window != nil
@result_window.dispose if @result_window != nil
@spriteset.dispose
if $scene.is_a?(Scene_Title)
Graphics.transition
Graphics.freeze
end
$scene = nil if $BTEST and not $scene.is_a?(Scene_Gameover)
end
#--------------------------------------------------------------------------
def fix_weapon_init
for member in $game_party.actors
if member.weapons[0] == nil and member.weapons[1] != nil
weapon_to_equip = member.armor1_id
member.equip(1, 0)
member.equip(0, weapon_to_equip)
member.two_swords_change = true
end
end
end
#--------------------------------------------------------------------------
def fix_weapon_end
for member in $game_party.actors
if member.two_swords_change
weapon_to_re_equip = member.weapon_id
member.equip(0, 0)
member.equip(1, weapon_to_re_equip)
member.two_swords_change = false
end
end
end
#--------------------------------------------------------------------------
def update_basic
Graphics.update
Input.update
$game_system.update
$game_screen.update
@spriteset.update
end
#--------------------------------------------------------------------------
def update_effects
for battler in $game_party.actors + $game_troop.enemies
if battler.exist?
battler_sprite = @spriteset.actor_sprites[battler.index] if battler.actor?
battler_sprite = @spriteset.enemy_sprites[battler.index] if battler.is_a?(Game_Enemy)
battler_sprite.effects_update
end
end
end
#--------------------------------------------------------------------------
def wait(duration)
for i in 0...duration
update_basic
end
end
#--------------------------------------------------------------------------
def pop_help(obj)
@help_window.set_text(obj, 1)
loop do
update_basic
break @help_window.visible = false if Input.trigger?(Input::C)
end
end
#--------------------------------------------------------------------------
alias start_phase1_n01 start_phase1
def start_phase1
for member in $game_party.actors + $game_troop.enemies
member.dead_anim = member.dead? ? true : false
@spriteset.set_stand_by_action(member.actor?, member.index) unless member.dead_anim
end
start_phase1_n01
$clear_enemies_actions = false
if $preemptive
pop_help(PREEMPTIVE_ALERT)
$clear_enemies_actions = true
end
@battle_start = false unless $back_attack
return unless $back_attack
pop_help(BACK_ATTACK_ALERT)
@battle_start = false
$game_party.clear_actions
start_phase4
end
#--------------------------------------------------------------------------
alias start_phase4_n01 start_phase4
def start_phase4
start_phase4_n01
@active_battler_window.visible = false
if $clear_enemies_actions
$clear_enemies_actions = false
$game_troop.clear_actions
end
end
#--------------------------------------------------------------------------
def judge
if $game_party.all_dead? or $game_party.actors.size == 0
if $game_temp.battle_can_lose
$game_system.bgm_play($game_temp.map_bgm)
battle_end(2)
return true
end
$game_temp.gameover = true
return true
end
for enemy in $game_troop.enemies
return false if enemy.exist?
end
process_victory
return true
end
#--------------------------------------------------------------------------
def update_phase2_escape
enemies_agi = enemies_number = 0
for enemy in $game_troop.enemies
if enemy.exist?
enemies_agi += enemy.agi
enemies_number += 1
end
end
enemies_agi /= [enemies_number, 1].max
actors_agi = actors_number = 0
for actor in $game_party.actors
if actor.exist?
actors_agi += actor.agi
actors_number += 1
end
end
actors_agi /= [actors_number, 1].max
@success = rand(100) < @escape_ratio * actors_agi / enemies_agi
@party_command_window.visible = false
@party_command_window.active = false
wait(2)
if @success
$game_system.se_play($data_system.escape_se)
for actor in $game_party.actors
unless actor.dead?
@spriteset.set_action(true, actor.index, actor.run_success)
end
end
pop_help(ESCAPE_SUCCESS)
$game_system.bgm_play($game_temp.map_bgm)
battle_end(1)
else
@escape_ratio += 5
$game_party.clear_actions
$game_system.se_play($data_system.escape_se)
for actor in $game_party.actors
unless actor.dead?
@spriteset.set_action(true, actor.index,actor.run_ng)
end
end
pop_help(ESCAPE_FAIL)
start_phase4
end
end
#--------------------------------------------------------------------------
def process_victory
for enemy in $game_troop.enemies
break boss_wait = true if enemy.collapse_type == 3
end
wait(440) if boss_wait
wait(WIN_WAIT) unless boss_wait
for actor in $game_party.actors
unless actor.restriction == 4
@spriteset.set_action(true, actor.index,actor.win)
end
end
start_phase5
end
#--------------------------------------------------------------------------
def start_phase5
@phase = 5
$game_system.me_play($game_system.battle_end_me)
$game_system.bgm_play($game_temp.map_bgm)
treasures = []
for enemy in $game_troop.enemies
gold = gold.nil? ? enemy.gold : gold + enemy.gold
treasures << treasure_drop(enemy) unless enemy.hidden
end
exp = gain_exp
treasures = treasures.compact
$game_party.gain_gold(gold)
for item in treasures
case item
when RPG::Item
$game_party.gain_item(item.id, 1)
when RPG::Weapon
$game_party.gain_weapon(item.id, 1)
when RPG::Armor
$game_party.gain_armor(item.id, 1)
end
end
@result_window = Window_BattleResult.new(exp, gold, treasures)
@result_window.add_multi_drops if $atoa_script['Multi Drop']
@phase5_wait_count = 100
end
#--------------------------------------------------------------------------
def treasure_drop(enemy)
if rand(100) < enemy.treasure_prob
treasure = $data_items[enemy.item_id] if enemy.item_id > 0
treasure = $data_weapons[enemy.weapon_id] if enemy.weapon_id > 0
treasure = $data_armors[enemy.armor_id] if enemy.armor_id > 0
end
return treasure
end
#--------------------------------------------------------------------------
def gain_exp
exp = exp_gained
for i in 0...$game_party.actors.size
actor = $game_party.actors[i]
if actor.cant_get_exp? == false
last_level = actor.level
actor.exp += exp
if actor.level > last_level
@status_window.level_up(i)
end
end
end
return exp
end
#--------------------------------------------------------------------------
def exp_gained
for enemy in $game_troop.enemies
exp = exp.nil? ? enemy.exp : exp + enemy.exp
end
if EXP_SHARE
actor_number = 0
for actor in $game_party.actors
actor_number += 1 unless actor.cant_get_exp?
end
exp = exp / [actor_number, 1].max
end
return exp
end
#--------------------------------------------------------------------------
alias acbs_update_phase5 update_phase5
def update_phase5
@result_window.update
acbs_update_phase5
end
#--------------------------------------------------------------------------
alias phase3_next_actor_n01 phase3_next_actor
def phase3_next_actor
if @active_battler != nil && @active_battler.inputable?
@spriteset.set_action(true, @actor_index, @active_battler.command_a)
end
@wait_count = 32 if @actor_index == $game_party.actors.size-1
phase3_next_actor_n01
if @active_battler != nil && @active_battler.inputable?
@spriteset.set_action(true, @actor_index,@active_battler.command_b)
end
end
#--------------------------------------------------------------------------
alias phase3_prior_actor_n01 phase3_prior_actor
def phase3_prior_actor
if @active_battler != nil && @active_battler.inputable?
@active_battler.current_action.clear
@spriteset.set_action(true, @actor_index,@active_battler.command_a)
end
phase3_prior_actor_n01
if @active_battler != nil && @active_battler.inputable?
@active_battler.current_action.clear
@spriteset.set_action(true, @actor_index,@active_battler.command_b)
end
end
#--------------------------------------------------------------------------
alias start_phase2_n01 start_phase2
def start_phase2
@active_battler_window.visible = false
start_phase2_n01
end
#--------------------------------------------------------------------------
alias phase3_setup_command_window_n01 phase3_setup_command_window
def phase3_setup_command_window
phase3_setup_command_window_n01
@actor_command_window.x = COMMAND_WINDOW_POSITION[0]
@actor_command_window.y = COMMAND_WINDOW_POSITION[1]
@actor_command_window.z = 2000
@actor_command_window.index = 0
@actor_command_window.back_opacity = COMMAND_OPACITY
@active_battler_window.refresh(@active_battler)
@active_battler_window.visible = true if BATTLER_NAME_WINDOW
end
#--------------------------------------------------------------------------
alias acbs_update_phase3_basic_command_scenebattle update_phase3_basic_command
def update_phase3_basic_command
if Input.trigger?(Input::C)
case @actor_command_window.commands[@actor_command_window.index]
when $data_system.words.attack
$game_system.se_play($data_system.decision_se)
@active_battler.current_action.kind = 0
@active_battler.current_action.basic = 0
@actor_command_window.active = false
@actor_command_window.visible = false
start_enemy_select
return
when $data_system.words.item
$game_system.se_play($data_system.decision_se)
@active_battler.current_action.kind = 2
start_item_select
return
when $data_system.words.guard
$game_system.se_play($data_system.decision_se)
@active_battler.current_action.kind = 0
@active_battler.current_action.basic = 1
phase3_next_actor
return
end
end
acbs_update_phase3_basic_command_scenebattle
end
#--------------------------------------------------------------------------
def now_action(battler = @active_battler)
return if battler.nil?
@now_action = nil
case battler.current_action.kind
when 0
@now_action = $data_weapons[battler.weapon_id] if battler.current_action.basic == 0
when 1
@now_action = $data_skills[battler.current_action.skill_id]
when 2
@now_action = $data_items[battler.current_action.item_id]
end
end
#--------------------------------------------------------------------------
def start_enemy_select
now_action
@enemy_arrow = Arrow_Enemy.new(@spriteset.viewport2)
@enemy_arrow.help_window = @help_window
@actor_command_window.active = false
@actor_command_window.visible = false
@active_battler_window.visible = false
@status_window.visible = true
end
#--------------------------------------------------------------------------
alias start_actor_select_n01 start_actor_select
def start_actor_select
now_action
start_actor_select_n01
@status_window.visible = true
@active_battler_window.visible = false
@actor_arrow.input_right if @now_action.extension.include?("OTHERS")
end
#--------------------------------------------------------------------------
alias update_phase3_actor_select_n01 update_phase3_actor_select
def update_phase3_actor_select
@actor_arrow.input_update_target if @now_action.extension.include?("OTHERS") and @actor_arrow.index == @active_battler.index
update_phase3_actor_select_n01
end
#--------------------------------------------------------------------------
alias update_phase3_n01 update_phase3
def update_phase3
if @enemy_arrow_all != nil
update_phase3_select_all_enemies
return
elsif @actor_arrow_all != nil
update_phase3_select_all_actors
return
elsif @battler_arrow_all != nil
update_phase3_select_all_battlers
return
end
update_phase3_n01
end
#--------------------------------------------------------------------------
alias update_phase3_skill_select_n01 update_phase3_skill_select
def update_phase3_skill_select
@status_window.visible = false if HIDE_WINDOW
if Input.trigger?(Input::C)
@skill = @skill_window.skill
if @skill == nil or not @active_battler.skill_can_use?(@skill.id)
$game_system.se_play($data_system.buzzer_se)
return
end
@active_battler.current_action.skill_id = @skill.id
@skill_window.visible = false
if @skill.extension.include?("TARGETALL")
$game_system.se_play($data_system.decision_se)
start_select_all_battlers
return
end
if (@skill.extension.include?("RANDOMTARGET") and @skill.scope <= 2) or @skill.scope == 2
$game_system.se_play($data_system.decision_se)
start_select_all_enemies
return
end
if (@skill.extension.include?("RANDOMTARGET") and @skill.scope > 2) or @skill.scope == 4
$game_system.se_play($data_system.decision_se)
start_select_all_actors
return
end
end
update_phase3_skill_select_n01
end
#--------------------------------------------------------------------------
alias update_phase3_item_select_n01 update_phase3_item_select
def update_phase3_item_select
@status_window.visible = false if HIDE_WINDOW
if Input.trigger?(Input::C)
@item = @item_window.item
if @item == nil or not $game_party.item_can_use?(@item.id)
$game_system.se_play($data_system.buzzer_se)
return
end
@active_battler.current_action.item_id = @item.id
@item_window.visible = false
if @item.extension.include?("TARGETALL")
$game_system.se_play($data_system.decision_se)
start_select_all_battlers
return
end
if @item.extension.include?("RANDOMTARGET") and @item.scope <= 2 or @item.scope == 2
$game_system.se_play($data_system.decision_se)
start_select_all_enemies
return
end
if (@item.extension.include?("RANDOMTARGET") and @item.scope > 2) or @item.scope == 4
$game_system.se_play($data_system.decision_se)
start_select_all_actors
return
end
end
update_phase3_item_select_n01
end
#--------------------------------------------------------------------------
def update_phase3_select_all_enemies
@enemy_arrow_all.update_multi_arrow
if Input.trigger?(Input::B)
$game_system.se_play($data_system.cancel_se)
end_select_all_enemies
return
end
if Input.trigger?(Input::C)
$game_system.se_play($data_system.decision_se)
if @skill_window != nil
end_skill_select
end
if @item_window != nil
end_item_select
end
end_select_all_enemies
phase3_next_actor
return
end
end
#--------------------------------------------------------------------------
def update_phase3_select_all_actors
@actor_arrow_all.update_multi_arrow
if Input.trigger?(Input::B)
$game_system.se_play($data_system.cancel_se)
end_select_all_actors
return
end
if Input.trigger?(Input::C)
$game_system.se_play($data_system.decision_se)
if @skill_window != nil
end_skill_select
end
if @item_window != nil
end_item_select
end
end_select_all_actors
phase3_next_actor
return
end
end
#--------------------------------------------------------------------------
def update_phase3_select_all_battlers
@battler_arrow_all.update_multi_arrow
if Input.trigger?(Input::B)
$game_system.se_play($data_system.cancel_se)
end_select_all_battlers
return
end
if Input.trigger?(Input::C)
$game_system.se_play($data_system.decision_se)
if @skill_window != nil
end_skill_select
end
if @item_window != nil
end_item_select
end
end_select_all_battlers
phase3_next_actor
return
end
end
#--------------------------------------------------------------------------
def start_select_all_enemies
now_action
@status_window.visible = true
@active_battler_window.visible = false
@enemy_arrow_all = Arrow_Enemy_All.new(@spriteset.viewport2)
end
#--------------------------------------------------------------------------
def start_select_all_actors
now_action
@status_window.visible = true
@active_battler_window.visible = false
@actor_arrow_all = Arrow_Actor_All.new(@spriteset.viewport2)
end
#--------------------------------------------------------------------------
def start_select_all_battlers
now_action
@status_window.visible = true
@active_battler_window.visible = false
@battler_arrow_all = Arrow_Battler_All.new(@spriteset.viewport2)
end
#--------------------------------------------------------------------------
def end_select_all_actors
@actor_arrow_all.dispose_multi_arrow
@actor_arrow_all = nil
@active_battler_window.visible = true if @actor_command_window.index == 0 and BATTLER_NAME_WINDOW
end
#--------------------------------------------------------------------------
def end_select_all_enemies
@enemy_arrow_all.dispose_multi_arrow
@enemy_arrow_all = nil
@active_battler_window.visible = true if @actor_command_window.index == 0 and BATTLER_NAME_WINDOW
end
#--------------------------------------------------------------------------
def end_select_all_battlers
@battler_arrow_all.dispose_multi_arrow
@battler_arrow_all = nil
@active_battler_window.visible = true if @actor_command_window.index == 0 and BATTLER_NAME_WINDOW
end
#--------------------------------------------------------------------------
alias end_enemy_select_n01 end_enemy_select
def end_enemy_select
end_enemy_select_n01
@active_battler_window.visible = true if @actor_command_window.index == 0 and BATTLER_NAME_WINDOW
end
#--------------------------------------------------------------------------
alias start_skill_select_n01 start_skill_select
def start_skill_select
start_skill_select_n01
@status_window.visible = false if HIDE_WINDOW
@active_battler_window.visible = false
end
#--------------------------------------------------------------------------
alias end_skill_select_n01 end_skill_select
def end_skill_select
end_skill_select_n01
@status_window.visible = true
@active_battler_window.visible = true if BATTLER_NAME_WINDOW
end
#--------------------------------------------------------------------------
alias start_item_select_n01 start_item_select
def start_item_select
start_item_select_n01
@status_window.visible = false if HIDE_WINDOW
@active_battler_window.visible = false
end
#--------------------------------------------------------------------------
alias end_item_select_n01 end_item_select
def end_item_select
end_item_select_n01
@status_window.visible = true
@active_battler_window.visible = true if BATTLER_NAME_WINDOW
end
#--------------------------------------------------------------------------
alias make_action_orders_n01 make_action_orders
def make_action_orders
make_action_orders_n01
for battler in @action_battlers
skill_id = battler.current_action.skill_id
item_id = battler.current_action.item_id
next if battler.current_action.kind == 0
extension = $data_skills[skill_id].extension if skill_id != 0
extension = $data_items[item_id].extension if item_id != 0
battler.current_action.speed = 9999 if extension.include?("FAST")
battler.current_action.speed = -1 if extension.include?("SLOW")
end
@action_battlers.sort! {|a,b|
b.current_action.speed - a.current_action.speed }
for enemy in $game_troop.enemies
if enemy.action_time[0] != 1
action_time = 0
for i in 1...enemy.action_time[0]
action_time += 1 if rand(100) < enemy.action_time[1]
end
enemy.act_time = action_time
action_time.times do
enemy_order_time(enemy)
action_time -= 1
break if action_time == 0
end
enemy.adj_speed = nil
end
end
end
#--------------------------------------------------------------------------
def enemy_order_time(enemy)
enemy.make_action_speed2(enemy.action_time[2])
select_time = 0
for member in @action_battlers
select_time += 1
break @action_battlers.push(enemy) if member.current_action.speed < enemy.adj_speed
break @action_battlers.push(enemy) if select_time == @action_battlers.size
end
end
#--------------------------------------------------------------------------
def update_phase4_step1
return if @spriteset.effect?
return @wait_count -= 1 if @wait_count > 0
@help_window.visible = false
return if judge
if $game_temp.forcing_battler == nil
setup_battle_event
return if $game_system.battle_interpreter.running?
end
if $game_temp.forcing_battler != nil
@action_battlers.delete($game_temp.forcing_battler)
@action_battlers.unshift($game_temp.forcing_battler)
end
if @action_battlers.size == 0
turn_ending
start_phase2
return
end
@animation1_id = 0
@animation2_id = 0
@common_event_id = 0
@active_battler = @action_battlers.shift
return if @active_battler.index == nil
@active_battler.remove_states_auto
@status_window.refresh
@phase4_step = 2
end
#--------------------------------------------------------------------------
def turn_ending
for member in $game_party.actors + $game_troop.enemies
member.current_action.clear
next unless member.exist?
member.slip_damage = false
actor = member.actor?
for state in member.battler_states
member.remove_state(state.id) if state.extension.include?("ZEROTURNLIFT")
end
damage = 0
for state in member.battler_states
next unless state.extension.include?("SLIPDAMAGE")
for ext in state.slip_extension
if ext[0] == "hp"
base_damage = ext[1] + member.maxhp * ext[2] / 100
damage += base_damage + base_damage * (rand(5) - rand(5)) / 100
slip_pop = ext[3]
slip_dead = ext[4]
slip_damage_flug = true
member.slip_damage = true
end
end
end
if member.slip_damage && member.exist? && !slip_damage_flug
damage += member.apply_variance(member.maxhp / 10, 10)
slip_dead = false
slip_pop = true
slip_damage_flug = true
member.slip_damage = true
end
damage = member.hp - 1 if damage >= member.hp && slip_dead = false
member.hp -= damage
member.damage = damage if damage > 0
member.perform_collapse if member.dead? && member.slip_damage
@spriteset.set_damage_pop(actor, member.index, damage) if slip_pop
@spriteset.set_stand_by_action(actor, member.index) if member.hp <= 0 and not member.dead_anim
member.dead_anim = member.dead? ? true : false
end
@status_window.refresh
wait(DMG_DURATION / 2) if slip_damage_flug
slip_damage_flug = false
for member in $game_party.actors + $game_troop.enemies
next unless member.exist?
actor = member.actor?
damage = 0
for state in member.battler_states
next unless state.extension.include?("SLIPDAMAGE")
for ext in state.slip_extension
if ext[0] == "mp"
base_damage = ext[1] + member.maxsp * ext[2] / 100
damage += base_damage + base_damage * (rand(5) - rand(5)) / 100
slip_pop = ext[3]
slip_damage_flug = true
end
end
member.sp_damage = true
member.sp -= damage
member.damage = damage if damage > 0
@spriteset.set_damage_pop(actor, member.index, damage) if slip_pop
end
end
@status_window.refresh
wait(DMG_DURATION / 2) if slip_damage_flug
for member in $game_party.actors + $game_troop.enemies
next unless member.exist?
actor = member.actor?
damage = 0
for state in member.battler_states
next unless state.extension.include?("REGENERATION")
for ext in state.slip_extension
if ext[0] == "hp"
base_damage = ext[1] + member.maxhp * ext[2] / 100
damage += base_damage + base_damage * (rand(5) - rand(5)) / 100
slip_pop = ext[3]
slip_damage_flug = true
end
end
member.hp -= damage
member.damage = damage if damage < 0
@spriteset.set_damage_pop(actor, member.index, damage) if slip_pop
end
end
@status_window.refresh
wait(DMG_DURATION / 2) if slip_damage_flug
for member in $game_party.actors + $game_troop.enemies
next unless member.exist?
actor = member.actor?
damage = 0
for state in member.battler_states
next unless state.extension.include?("REGENERATION")
for ext in state.slip_extension
if ext[0] == "mp"
base_damage = ext[1] + member.maxhp * ext[2] / 100
damage += base_damage + base_damage * (rand(5) - rand(5)) / 100
slip_pop = ext[3]
slip_damage_flug = true
end
end
member.sp_damage = true
member.sp -= damage
member.damage = damage if damage < 0
@spriteset.set_damage_pop(actor, member.index, damage) if slip_pop
end
end
@status_window.refresh
wait(DMG_DURATION / 2) if slip_damage_flug
end
#--------------------------------------------------------------------------
alias update_phase4_step2_n01 update_phase4_step2
def update_phase4_step2
for member in $game_party.actors + $game_troop.enemies
member.dead_anim = member.dead? ? true : false
end
if @active_battler.current_action.kind != 0
obj = $data_skills[@active_battler.current_action.skill_id] if @active_battler.current_action.kind == 1
obj = $data_items[@active_battler.current_action.item_id] if @active_battler.current_action.kind == 2
@active_battler.white_flash = false if obj != nil &&obj.extension.include?("NOFLASH")
end
@active_battler.active = true
update_phase4_step2_n01
if @active_battler != nil && @active_battler.derivation != 0
@active_battler.current_action.kind = 1
@active_battler.current_action.skill_id = @active_battler.derivation
@action_battlers.unshift(@active_battler)
end
if @active_battler != nil && !@active_battler.actor? && @active_battler.act_time != 0
@active_battler.make_action
@active_battler.act_time -= 1
end
update_phase4_step6
end
#--------------------------------------------------------------------------
alias update_phase4_step6_n01 update_phase4_step6
def update_phase4_step6
update_phase4_step6_n01
@active_battler.active = false if @active_battler != nil
end
#--------------------------------------------------------------------------
def make_basic_action_result
if @active_battler.current_action.basic == 0
execute_action_attack
return
end
if @active_battler.current_action.basic == 1
@help_window.set_text("#{@active_battler.name} defends", 1)
@help_window.visible = true
@active_battler.active = false
@active_battler.defense_pose = true
@spriteset.set_stand_by_action(@active_battler.actor?, @active_battler.index)
wait(45)
@help_window.visible = false
return
end
if @active_battler.is_a?(Game_Enemy) and @active_battler.current_action.basic == 2
@spriteset.set_action(false, @active_battler.index, @active_battler.run_success)
$game_system.se_play($data_system.escape_se)
@active_battler.escape
pop_help("#{@active_battler.name} escaped...")
return
end
if @active_battler.current_action.basic == 3
@active_battler.active = false
$game_temp.forcing_battler = nil
@phase4_step = 1
return
end
end
#--------------------------------------------------------------------------
def execute_action_attack
if @active_battler.actor?
if @active_battler.weapon_id == 0
action = @active_battler.non_weapon
immortaling
else
action = $data_weapons[@active_battler.weapon_id].base_action
if $data_weapons[@active_battler.weapon_id].plus_state_set.include?(1)
for member in $game_party.actors + $game_troop.enemies
next if member.immortal
next if member.dead?
member.dying = true
end
else
immortaling
end
end
else
if @active_battler.weapon == 0
action = @active_battler.base_action
immortaling
else
action = $data_weapons[@active_battler.weapon].base_action
if $data_weapons[@active_battler.weapon].plus_state_set.include?(1)
for member in $game_party.actors + $game_troop.enemies
next if member.immortal
next if member.dead?
member.dying = true
end
else
immortaling
end
end
end
target_decision
@spriteset.set_action(@active_battler.actor?, @active_battler.index, action)
playing_action
end
#--------------------------------------------------------------------------
def make_attack_targets
@target_battlers = []
if @active_battler.is_a?(Game_Enemy)
if @active_battler.restriction == 3
target = $game_troop.random_target_enemy
elsif @active_battler.restriction == 2
target = $game_party.random_target_actor
else
index = @active_battler.current_action.target_index
target = $game_party.smooth_target_actor(index)
end
end
if @active_battler.actor?
if @active_battler.restriction == 3
target = $game_party.random_target_actor
elsif @active_battler.restriction == 2
target = $game_troop.random_target_enemy
else
index = @active_battler.current_action.target_index
target = $game_troop.smooth_target_enemy(index)
end
end
@target_battlers = [target]
return @target_battlers
end
#--------------------------------------------------------------------------
def make_skill_action_result
skill = $data_skills[@active_battler.current_action.skill_id]
if skill.plus_state_set.include?(1)
for member in $game_party.actors + $game_troop.enemies
next if member.immortal
next if member.dead?
member.dying = true
end
else
immortaling
end
return unless @active_battler.skill_can_use?(skill.id)
target_decision(skill)
@active_battler.consum_skill_cost(skill)
@status_window.refresh
@spriteset.set_action(@active_battler.actor?, @active_battler.index, skill.base_action)
@help_window.set_text(skill.name, 1) unless skill.extension.include?("HELPHIDE")
playing_action
@common_event_id = skill.common_event_id
end
#--------------------------------------------------------------------------
def make_item_action_result
item = $data_items[@active_battler.current_action.item_id]
unless $game_party.item_can_use?(item.id)
@phase4_step = 1
return
end
if @item.consumable
$game_party.lose_item(item.id, 1)
end
immortaling
target_decision(item)
@spriteset.set_action(@active_battler.actor?, @active_battler.index, item.base_action)
@help_window.set_text(item.name, 1) unless item.extension.include?("HELPHIDE")
playing_action
@common_event_id = item.common_event_id
end
#--------------------------------------------------------------------------
def target_decision(obj = nil)
if obj != nil
set_target_battlers(obj.scope)
if obj.extension.include?("TARGETALL")
@target_battlers = []
if obj.scope != 5 or obj.scope != 6
for target in $game_troop.enemies + $game_party.actors
@target_battlers.push(target) if target.exist?
end
else
for target in $game_troop.enemies + $game_party.actors
@target_battlers.push(target) if target != nil && target.hp0?
end
end
end
@target_battlers.delete(@active_battler) if obj.extension.include?("OTHERS")
if obj.extension.include?("RANDOMTARGET")
randum_targets = @target_battlers.dup
@target_battlers = [randum_targets[rand(randum_targets.size)]]
end
else
@target_battlers = make_attack_targets
end
if @target_battlers.size == 0
action = @active_battler.recover_action
@spriteset.set_action(@active_battler.actor?, @active_battler.index, action)
end
@spriteset.set_target(@active_battler.actor?, @active_battler.index, @target_battlers)
end
#--------------------------------------------------------------------------
def playing_action
loop do
update_basic
update_effects
action = @active_battler.play
next if action == 0
@active_battler.play = 0
if action[0] == "Individual"
individual
elsif action == "Can Collapse"
unimmortaling
elsif action == "Cancel Action"
break action_end
elsif action == "End"
break action_end
elsif action[0] == "OBJ_ANIM"
damage_action(action[1])
end
end
end
#--------------------------------------------------------------------------
def individual
@individual_target = @target_battlers
@stand_by_target = @target_battlers.dup
end
#--------------------------------------------------------------------------
def immortaling
for member in $game_party.actors + $game_troop.enemies
member.immortal = true unless member.dead?
end
end
#--------------------------------------------------------------------------
def unimmortaling
return if @active_battler.individual
for member in $game_party.actors + $game_troop.enemies
member.immortal = false
member.add_state(1) if member.dead?
if member.dead? and not member.dead_anim
member.perform_collapse
@spriteset.set_stand_by_action(member.actor?, member.index)
end
member.dead_anim = member.dead? ? true : false
next unless member.dead?
resurrection(member)
end
update_basic
@status_window.refresh
end
#--------------------------------------------------------------------------
def resurrection(target)
for state in target.battler_states
for ext in state.extension
name = ext.split('')
next unless name[0] == "A"
wait(25)
name = name.join
name.slice!("AUTOLIFE/")
target.hp = target.maxhp * name.to_i / 100
target.remove_state(1)
target.remove_state(state.id)
target.animation_id = RESURRECTION
target.animation_hit = true
target.anime_mirror = true if $back_attack
@status_window.refresh
wait($data_animations[RESURRECTION].frame_max * 2)
end
end
end
#--------------------------------------------------------------------------
def magic_reflection(target, obj)
return if obj != nil and $data_skills[@active_battler.current_action.skill_id].int_f == 0
for state in target.battler_states
for ext in state.extension
name = ext.split('')
next unless name[0] == "M"
if name[3] == "R"
name = name.join
name.slice!("MAGREFLECT/")
target.animation_id = name.to_i
target.animation_hit = true
target.anime_mirror = true if $back_attack
@reflection = true
else
name = name.join
name.slice!("MAGNULL/")
target.animation_id = name.to_i
target.animation_hit = true
target.anime_mirror = true if $back_attack
@invalid = true
end
end
end
end
#--------------------------------------------------------------------------
def physics_reflection(target, obj)
return if obj != nil && $data_skills[@active_battler.current_action.skill_id].str_f == 0
for state in target.battler_states
for ext in state.extension
name = ext.split('')
next unless name[0] == "P"
if name[3] == "R"
name = name.join
name.slice!("PHYREFLECT/")
target.animation_id = name.to_i
target.animation_hit = true
target.anime_mirror = true if $back_attack
@reflection = true
else
name = name.join
name.slice!("PHYNULL/")
target.animation_id = name.to_i
target.animation_hit = true
target.anime_mirror = true if $back_attack
@invalid = true
end
end
end
end
#--------------------------------------------------------------------------
def absorb_cost(target, obj)
for state in target.battler_states
if state.extension.include?("COSTABSORB")
cost = @active_battler.calc_sp_cost(@active_battler, obj)
return target.hp += cost if obj.extension.include?("HPCONSUME")
return target.mp += cost
end
end
end
#--------------------------------------------------------------------------
def absorb_attack(obj, target, index, actor)
for ext in obj.extension
return if target.evaded or target.missed or target.damage == 0 or target.damage == nil
name = ext.split('')
next unless name[3] == "G" and name[1] == "D"
name = name.join
name.slice!("%DMGABSORB/")
kind = "hp" unless target.sp_damage
kind = "sp" if target.sp_damage
absorb = target.damage * name.to_i / 100
@wide_attack = true if obj.scope == 2 or obj.scope == 4 or obj.scope == 6 or obj.extension.include?("TARGETALL")
if @wide_attack && @absorb == nil && @target_battlers.size != 1
@absorb = absorb
@absorb_target_size = @target_battlers.size - 2
elsif @absorb != nil && @absorb_target_size > 0
@absorb += absorb
@absorb_target_size -= 1
elsif @absorb != nil
@absorb += absorb
absorb_action = ["absorb", nil, kind, @absorb]
@spriteset.set_damage_action(actor, index, absorb_action)
@absorb = nil
@absorb_target_size = nil
@active_battler.perform_collapse
else
absorb_action = ["absorb", nil, kind, absorb]
@spriteset.set_damage_action(actor, index, absorb_action)
@active_battler.perform_collapse
end
end
end
#--------------------------------------------------------------------------
def action_end
@individual_target = nil
@help_window.visible = false if @help_window != nil && @help_window.visible
@active_battler.active = false
unimmortaling
for member in $game_troop.enemies
member.non_dead = false if member.non_dead
end
if @active_battler.reflex != nil
if @active_battler.current_action.kind == 1
obj = $data_skills[@active_battler.current_action.skill_id]
@active_battler.perfect_skill_effect(@active_battler, obj)
elsif @active_battler.current_action.kind == 2
obj = $data_items[@active_battler.current_action.item_id]
@active_battler.item_effect(@active_battler, obj)
else
@active_battler.perfect_attack_effect(@active_battler)
end
pop_damage(@active_battler, obj, @active_battler.reflex)
@active_battler.perform_collapse
@active_battler.reflex = nil
wait(COLLAPSE_WAIT)
end
if @active_battler.derivation != 0
@active_battler.current_action.skill_id = @active_battler.derivation
@active_battler.current_action.kind = 1
@active_battler.derivation = 0
@action_battlers.unshift(@active_battler)
else
@spriteset.set_stand_by_action(@active_battler.actor?, @active_battler.index)
wait(ACTION_WAIT + 20)
end
end
#--------------------------------------------------------------------------
def damage_action(action)
@target_battlers = [@individual_target.shift] if @active_battler.individual
if @active_battler.current_action.kind == 1
obj = $data_skills[@active_battler.current_action.skill_id]
for target in @target_battlers
return if target == nil
if obj.scope == 5 or obj.scope == 6
return unless target.dead?
else
return if target.dead?
end
if target.hp == 0 && obj.scope != 5 && obj.scope != 6
target.perfect_skill_effect(@active_battler, obj)
elsif obj.extension.include?("NOEVADE")
target.perfect_skill_effect(@active_battler, obj)
else
magic_reflection(target, obj) unless obj.extension.include?("IGNOREREFLECT")
physics_reflection(target, obj) unless obj.extension.include?("IGNOREREFLECT")
target.skill_effect(@active_battler, obj) unless @reflection or @invalid
end
pop_damage(target, obj, action) unless @reflection or @invalid
absorb_cost(target, obj)
@active_battler.reflex = action if @reflection
@reflection = false
@invalid = false
end
elsif @active_battler.current_action.kind == 2
obj = $data_items[@active_battler.current_action.item_id]
for target in @target_battlers
return if target == nil
if obj.scope == 5 or obj.scope == 6
return unless target.dead?
else
return if target.dead?
end
target.revival = true if obj.scope == 5 or obj.scope == 6
target.item_effect(obj)
pop_damage(target, obj, action)
end
else
for target in @target_battlers
return if target == nil or target.dead?
physics_reflection(target, nil)
target.perfect_attack_effect(@active_battler) if target.hp <= 0
target.attack_effect(@active_battler) unless target.hp <= 0 unless @reflection or @invalid
pop_damage(target, obj, action) unless @reflection or @invalid
@active_battler.reflex = action if @reflection
@reflection = false
@invalid = false
end
end
return if obj == nil
target_decision(obj) if obj.extension.include?("RANDOMTARGET")
end
#--------------------------------------------------------------------------
def pop_damage(target, obj, action)
index = @active_battler.index
actor = @active_battler.actor?
if obj != nil && obj.extension.size != 0
absorb_attack(obj, target, index, actor)
action[2] = false if obj.extension.include?("NOOVERKILL")
end
@spriteset.set_damage_action(target.actor?, target.index, action)
@status_window.refresh
end
end
#==============================================================================
# ■ Scene_Map
#==============================================================================
class Scene_Map
#--------------------------------------------------------------------------
include N01
#--------------------------------------------------------------------------
alias call_battle_n01 call_battle
#--------------------------------------------------------------------------
def call_battle
$back_attack = $preemptive = false
preemptive_or_back_attack
call_battle_n01
end
#--------------------------------------------------------------------------
def preemptive_or_back_attack
enemies_agi = 0
for enemy in $game_troop.enemies
enemies_agi += enemy.agi
end
enemies_agi /= [$game_troop.enemies.size, 1].max
actors_agi = 0
for actor in $game_party.actors
actors_agi += actor.agi
end
actors_agi /= [$game_party.actors.size, 1].max
preemptive_plus
if actors_agi >= enemies_agi
percent_preemptive = PREEMPTIVE_RATE * ($preemptive_plus ? 3 : 1)
percent_back_attack = BACK_ATTACK_RATE / 2
else
percent_preemptive = (PREEMPTIVE_RATE / 2) * ($preemptive_plus ? 3 : 1)
percent_back_attack = BACK_ATTACK_RATE
end
if rand(100) < percent_preemptive
$preemptive = true
elsif rand(100) < percent_back_attack
$back_attack = true
end
special_back_attack_conditions
special_preemptive_conditions
$preemptive = false if $back_attack or !PREEMPTIVE
$back_attack = false if !BACK_ATTACK
end
#--------------------------------------------------------------------------
def special_back_attack_conditions
for i in 0...BACK_ATTACK_SWITCH.size
return $back_attack = true if $game_switches[BACK_ATTACK_SWITCH[i]]
end
for i in 0...NO_BACK_ATTACK_SWITCH.size
return $back_attack = false if $game_switches[NON_BACK_ATTACK_SWITCH[i]]
end
for actor in $game_party.actors
return $back_attack = false if NON_BACK_ATTACK_WEAPONS.include?(actor.weapon_id)
return $back_attack = false if NON_BACK_ATTACK_ARMOR1.include?(actor.armor1_id)
return $back_attack = false if NON_BACK_ATTACK_ARMOR2.include?(actor.armor2_id)
return $back_attack = false if NON_BACK_ATTACK_ARMOR3.include?(actor.armor3_id)
return $back_attack = false if NON_BACK_ATTACK_ARMOR4.include?(actor.armor4_id)
for i in 0...NON_BACK_ATTACK_SKILLS.size
return $back_attack = false if actor.skill_id_learn?(NON_BACK_ATTACK_SKILLS[i])
end
end
end
#--------------------------------------------------------------------------
def special_preemptive_conditions
for i in 0...PREEMPTIVE_SWITCH.size
return $preemptive = true if $game_switches[PREEMPTIVE_SWITCH[i]]
end
for i in 0...NO_PREEMPTIVE_SWITCH.size
return $preemptive = false if $game_switches[NON_PREEMPTIVE_SWITCH[i]]
end
end
#--------------------------------------------------------------------------
def preemptive_plus
$preemptive_plus = false
for actor in $game_party.actors
return $preemptive_plus = true if PREEMPTIVE_WEAPONS.include?(actor.weapon_id)
return $preemptive_plus = true if PREEMPTIVE_ARMOR1.include?(actor.armor1_id)
return $preemptive_plus = true if PREEMPTIVE_ARMOR2.include?(actor.armor2_id)
return $preemptive_plus = true if PREEMPTIVE_ARMOR3.include?(actor.armor3_id)
return $preemptive_plus = true if PREEMPTIVE_ARMOR4.include?(actor.armor4_id)
for i in 0...PREEMPTIVE_SKILLS.size
return $preemptive_plus = true if actor.skill_id_learn?(PREEMPTIVE_SKILLS[i])
end
end
end
end
#==============================================================================
# ■ Spriteset_Battle
#==============================================================================
class Spriteset_Battle
#--------------------------------------------------------------------------
attr_reader :viewport1
attr_reader :viewport2
attr_accessor :actor_sprites
attr_accessor :enemy_sprites
#--------------------------------------------------------------------------
include N01
#--------------------------------------------------------------------------
def initialize
@viewport1 = Viewport.new(0, 0, 640, 480)
@viewport2 = Viewport.new(0, 0, 640, 480)
@viewport3 = Viewport.new(0, 0, 640, 480)
@viewport4 = Viewport.new(0, 0, 640, 480)
@viewport2.z = 101
@viewport3.z = 200
@viewport4.z = 5000
@battleback_sprite = Sprite.new(@viewport1)
@battleback_sprite.mirror = true if $back_attack && BACK_ATTACK_BATTLE_BACK_MIRROR
@enemy_sprites = []
for enemy in $game_troop.enemies
@enemy_sprites.push(Sprite_Battler.new(@viewport2, enemy))
end
@actor_sprites = []
for i in 0...$game_party.actors.size
@actor_sprites.push(Sprite_Battler.new(@viewport2, $game_party.actors[i]))
end
@weather = RPG::Weather.new(@viewport1)
@picture_sprites = []
for i in 51..100
@picture_sprites.push(Sprite_Picture.new(@viewport3, $game_screen.pictures[i]))
end
@timer_sprite = Sprite_Timer.new
update
end
#--------------------------------------------------------------------------
def update
if $game_party.actors.size > @actor_sprites.size
for i in @actor_sprites.size...$game_party.actors.size
@actor_sprites.push(Sprite_Battler.new(@viewport2, $game_party.actors[i]))
end
elsif @actor_sprites.size > $game_party.actors.size
for i in 0...@actor_sprites.size
@actor_sprites[i].dispose
end
@actor_sprites = []
for i in 0...$game_party.actors.size
@actor_sprites.push(Sprite_Battler.new(@viewport2, $game_party.actors[i]))
end
end
for i in 0...$game_party.actors.size
@actor_sprites[i].battler = $game_party.actors[i]
end
if @battleback_name != $game_temp.battleback_name
@battleback_name = $game_temp.battleback_name
if @battleback_sprite.bitmap != nil
@battleback_sprite.bitmap.dispose
end
@battleback_sprite.bitmap = RPG::Cache.battleback(@battleback_name)
@battleback_sprite.src_rect.set(0, 0, 640, 480)
end
for sprite in @enemy_sprites + @actor_sprites
sprite.update
end
@weather.type = $game_screen.weather_type
@weather.max = $game_screen.weather_max
@weather.update
for sprite in @picture_sprites
sprite.update
end
@timer_sprite.update
@viewport1.tone = $game_screen.tone
@viewport1.ox = $game_screen.shake
@viewport2.tone = $game_screen.tone
@viewport2.ox = $game_screen.shake
@viewport4.color = $game_screen.flash_color
@viewport1.update
@viewport2.update
@viewport4.update
end
#--------------------------------------------------------------------------
def set_damage_action(actor, index, action)
return if index.nil?
@actor_sprites[index].damage_action(action) if actor
@enemy_sprites[index].damage_action(action) unless actor
end
#--------------------------------------------------------------------------
def set_damage_pop(actor, index, damage)
return if index.nil?
@actor_sprites[index].damage_pop if actor
@enemy_sprites[index].damage_pop unless actor
end
#--------------------------------------------------------------------------
def set_target(actor, index, target)
return if index.nil?
@actor_sprites[index].get_target(target) if actor
@enemy_sprites[index].get_target(target) unless actor
end
#--------------------------------------------------------------------------
def set_action(actor, index, kind)
return if index.nil?
@actor_sprites[index].start_action(kind) if actor
@enemy_sprites[index].start_action(kind) unless actor
end
#--------------------------------------------------------------------------
def set_stand_by_action(actor, index)
return if index.nil?
@actor_sprites[index].push_stand_by if actor
@enemy_sprites[index].push_stand_by unless actor
end
end
#==============================================================================
# ■ Sprite_MoveAnime
#==============================================================================
class Sprite_MoveAnime < RPG::Sprite
#--------------------------------------------------------------------------
attr_accessor :battler
attr_accessor :base_x
attr_accessor :base_y
#--------------------------------------------------------------------------
def initialize(viewport,battler = nil)
super(viewport)
@battler = battler
self.visible = false
@base_x = 0
@base_y = 0
@move_x = 0
@move_y = 0
@moving_x = 0
@moving_y = 0
@orbit = 0
@orbit_plus = 0
@orbit_time = 0
@through = false
@finish = false
@time = 0
@angle = 0
@angling = 0
end
#--------------------------------------------------------------------------
def anime_action(id,mirror,distanse_x,distanse_y,type,speed,orbit,weapon,icon_index,icon_weapon)
@time = speed
@moving_x = distanse_x / speed
@moving_y = distanse_y / speed
@through = true if type == 1
@orbit_plus = orbit
@orbit_time = @time
if weapon != ""
action = ANIME[weapon].dup
@angle = action[0]
end_angle = action[1]
time = action[2]
@angling = (end_angle - @angle)/ time
self.angle = @angle
self.mirror = mirror
if icon_weapon
self.bitmap = RPG::Cache.icon(icon_index)
self.ox = 12
self.oy = 12
else
self.bitmap = RPG::Cache.character(icon_index, 0)
self.ox = self.bitmap.width / 2
self.oy = self.bitmap.height / 2
end
self.visible = true
self.z = 1000
end
self.x = @base_x + @move_x
self.y = @base_y + @move_y + @orbit
if id != 0 && !icon_weapon
animation($data_animations[id],true)
elsif id != 0 && icon_weapon
loop_animation($data_animations[id])
end
end
#--------------------------------------------------------------------------
def action_reset
@moving_x = @moving_y = @move_x = @move_y = @base_x = @base_y = @orbit = 0
@orbit_time = @angling = @angle = 0
@through = self.visible = @finish = false
dispose_animation
end
#--------------------------------------------------------------------------
def finish?
return @finish
end
#--------------------------------------------------------------------------
def update
super
@time -= 1
if @time >= 0
@move_x += @moving_x
@move_y += @moving_y
if @time < @orbit_time / 2
@orbit_plus = @orbit_plus * 5 / 4
elsif @time == @orbit_time / 2
@orbit_plus *= -1
else
@orbit_plus = @orbit_plus * 2 / 3
end
@orbit += @orbit_plus
end
@time = 100 if @time < 0 && @through
@finish = true if @time < 0 && !@through
self.x = @base_x + @move_x
self.y = @base_y + @move_y + @orbit
if self.x < -200 or self.x > 840 or self.y < -200 or self.y > 680
@finish = true
end
if self.visible
@angle += @angling
self.angle = @angle
end
end
end
#==============================================================================
# ■ Sprite_Weapon
#==============================================================================
class Sprite_Weapon < RPG::Sprite
#--------------------------------------------------------------------------
include N01
#--------------------------------------------------------------------------
attr_accessor :battler
#--------------------------------------------------------------------------
def initialize(viewport,battler = nil)
super(viewport)
@battler = battler
@action = []
@move_x = 0
@move_y = 0
@move_z = 0
@plus_x = 0
@plus_y = 0
@angle = 0
@zoom_x = 1
@zoom_y = 1
@moving_x = 0
@moving_y = 0
@angling = 0
@zooming_x = 1
@zooming_y = 1
@freeze = -1
@mirroring = false
@time = ANIME_PATTERN + 1
weapon_graphics
end
#--------------------------------------------------------------------------
def dispose
self.bitmap.dispose if self.bitmap != nil
super
end
#--------------------------------------------------------------------------
def weapon_graphics(left = false)
if @battler.actor?
weapon = @battler.weapons[0] unless left
weapon = @battler.weapons[1] if left
else
weapon = $data_weapons[@battler.weapon]
@mirroring = true if @battler.action_mirror
end
return if weapon == nil
if weapon.graphic == ""
self.bitmap = RPG::Cache.icon(weapon.icon_name)
@weapon_width = @weapon_height = 24
else
self.bitmap = RPG::Cache.icon(weapon.graphic)
@weapon_width = self.bitmap.width
@weapon_height = self.bitmap.height
end
end
#--------------------------------------------------------------------------
def freeze(action)
@freeze = action
end
#--------------------------------------------------------------------------
def weapon_action(action,loop)
if action == ""
self.visible = false
elsif @weapon_id == 0
self.visible = false
else
@action = ANIME[action]
act0 = @action[0]
act1 = @action[1]
act2 = @action[2]
act3 = @action[3]
act4 = @action[4]
act5 = @action[5]
act6 = @action[6]
act7 = @action[7]
act8 = @action[8]
act9 = @action[9]
act10 = @action[10]
if @mirroring
act0 *= -1
act3 *= -1
act4 *= -1
act9 *= -1
end
if $back_attack && BACK_ATTACK
act0 *= -1
act3 *= -1
act4 *= -1
act9 *= -1
end
time = ANIME_PATTERN
if act2
self.z = @battler.position_z + 1
else
self.z = @battler.position_z - 1
end
if act6
if self.mirror
self.mirror = false
else
self.mirror = true
end
end
if @mirroring
if self.mirror
self.mirror = false
else
self.mirror = true
end
end
if $back_attack && BACK_ATTACK
if self.mirror
self.mirror = false
else
self.mirror = true
end
end
@moving_x = act0 / time
@moving_y = act1 / time
@angle = act3
self.angle = @angle
@angling = (act4 - act3)/ time
@angle += (act4 - act3) % time
@zooming_x = (1 - act7) / time
@zooming_y = (1 - act8) / time
if self.mirror
case act5
when 1
act5 = 2
when 2
act5 = 1
when 3
act5 = 4
when 4
act5 = 3
end
end
case act5
when 0
self.ox = @weapon_width / 2
self.oy = @weapon_height / 2
when 1
self.ox = 0
self.oy = 0
when 2
self.ox = @weapon_width
self.oy = 0
when 3
self.ox = 0
self.oy = @weapon_height
when 4
self.ox = @weapon_width
self.oy = @weapon_height
end
@plus_x = act9
@plus_y = act10
@loop = true if loop == 0
@angle -= @angling
@zoom_x -= @zooming_x
@zoom_y -= @zooming_y
@move_x -= @moving_x
@move_y -= @moving_y
@move_z = 1000 if act2
if @freeze != -1
for i in 0..@freeze + 1
@angle += @angling
@zoom_x += @zooming_x
@zoom_y += @zooming_y
@move_x += @moving_x
@move_y += @moving_y
end
@angling = 0
@zooming_x = 0
@zooming_y = 0
@moving_x = 0
@moving_y = 0
end
self.visible = true
end
end
#--------------------------------------------------------------------------
def action_reset
@moving_x = @moving_y = @move_x = @move_y = @plus_x = @plus_y = 0
@angling = @zooming_x = @zooming_y = @angle = self.angle = @move_z = 0
@zoom_x = @zoom_y = self.zoom_x = self.zoom_y = 1
self.mirror = self.visible = @loop = false
@freeze = -1
@action = []
@time = ANIME_PATTERN + 1
end
#--------------------------------------------------------------------------
def action_loop
@angling *= -1
@zooming_x *= -1
@zooming_y *= -1
@moving_x *= -1
@moving_y *= -1
end
#--------------------------------------------------------------------------
def mirroring
return @mirroring = false if @mirroring
@mirroring = true
end
#--------------------------------------------------------------------------
def action
return if @time <= 0
@time -= 1
@angle += @angling
@zoom_x += @zooming_x
@zoom_y += @zooming_y
@move_x += @moving_x
@move_y += @moving_y
if @loop && @time == 0
@time = ANIME_PATTERN + 1
action_loop
end
end
#--------------------------------------------------------------------------
def update
super
self.angle = @angle
self.zoom_x = @zoom_x
self.zoom_y = @zoom_y
self.x = @battler.position_x + @move_x + @plus_x
self.y = @battler.position_y + @move_y + @plus_y
self.z = @battler.position_z + @move_z - 1
end
end
#==============================================================================
# Game_Party
#==============================================================================
class Game_Party
#--------------------------------------------------------------------------
include N01
#--------------------------------------------------------------------------
def add_actor(actor_id)
actor = $game_actors[actor_id]
if @actors.size < MAX_MEMBER and not @actors.include?(actor)
@actors.push(actor)
$game_player.refresh
end
end
end
#==============================================================================
# ■ RPG::Weapon
#==============================================================================
class RPG::Weapon
#--------------------------------------------------------------------------
def magic?
return false
end
end
#==============================================================================
# ■ RPG::Skill
#==============================================================================
class RPG::Skill
#--------------------------------------------------------------------------
def magic?
return @atk_f == 0 ? true : false
end
end
#==============================================================================
# ■ RPG::Item
#==============================================================================
class RPG::Item
#--------------------------------------------------------------------------
def magic?
return false
end
end
#==============================================================================
# ■ RPG::Cache
#==============================================================================
module RPG::Cache
def self.faces(filename, hue = 0)
self.load_bitmap('Graphics/Faces/', filename, hue)
end
end
#==============================================================================
# ■ Game_Battler
#==============================================================================
class Game_Battler
#--------------------------------------------------------------------------
include N01
#--------------------------------------------------------------------------
attr_accessor :sp_damage
attr_accessor :collapse
attr_accessor :move_x
attr_accessor :move_y
attr_accessor :move_z
attr_accessor :jump
attr_accessor :active
attr_accessor :non_dead
attr_accessor :slip_damage
attr_accessor :derivation
attr_accessor :individual
attr_accessor :play
attr_accessor :force_action
attr_accessor :force_target
attr_accessor :revival
attr_accessor :reflex
attr_accessor :absorb
attr_accessor :anime_mirror
attr_accessor :dying
attr_accessor :state_animation_id
attr_accessor :dead_anim
attr_accessor :missed
attr_accessor :evaded
attr_accessor :true_immortal
attr_accessor :defense_pose
attr_reader :base_position_x
attr_reader :base_position_y
attr_reader :base_height
#--------------------------------------------------------------------------
alias initialize_n01 initialize
def initialize
initialize_n01
@move_x = @move_y = @move_z = @plus_y = @jump = @derivation = @act_time = 0
@force_action = @force_target = @base_position_x = @base_position_y = 0
@absorb = @play = @now_state = @state_frame = @state_animation_id = 0
@active = @non_dead = @individual = @slip_damage = @revival = false
@collapse = @sp_damage = @anime_mirror = @dying = false
@evaded = @missed = false
@anim_states = []
end
#--------------------------------------------------------------------------
def state_id
return @states[@states.size - 1]
end
#--------------------------------------------------------------------------
def in_danger
return @hp <= self.maxhp / 4
end
#--------------------------------------------------------------------------
def battler_states
bat_states = []
for i in self.states
bat_states.push($data_states[i])
end
return bat_states
end
#--------------------------------------------------------------------------
def apply_variance(damage, variance)
if damage != 0
amp = [damage.abs * variance / 100, 0].max
damage += rand(amp+1) + rand(amp+1) - amp
end
return damage
end
#--------------------------------------------------------------------------
def state_animation_id
return 0 if @states.empty?
return 0 if @states.include?(1)
@state_frame -= 1 if @state_frame > 0
return @state_animation_id if @state_frame > 0
if @anim_states.empty?
for state in @states
@anim_states << state if $data_states[state].animation_id > 0
end
end
now_state = @anim_states.shift
return 0 if now_state.nil?
@state_animation_id = $data_states[now_state].animation_id
return 0 if $data_animations[@state_animation_id].nil?
@state_frame = $data_animations[@state_animation_id].frame_max * STATE_CYCLE_TIME * 2
return @state_animation_id
end
#--------------------------------------------------------------------------
def skill_can_use?(skill_id)
skill = $data_skills[skill_id]
if skill.extension.include?("CONSUMEHP")
return false if calc_sp_cost(self, skill) >= self.hp
else
return false if calc_sp_cost(self, skill) > self.sp
end
return false if dead?
return false if skill.atk_f == 0 and self.restriction == 1
occasion = skill.occasion
return (occasion == 0 or occasion == 1) if $game_temp.in_battle
return (occasion == 0 or occasion == 2)
end
#--------------------------------------------------------------------------
def calc_sp_cost(user, skill)
cost = skill.sp_cost
if skill.extension.include?("%COSTMAX")
return user.maxhp * cost / 100 if skill.extension.include?("CONSUMEHP")
return user.maxsp * cost / 100
elsif skill.extension.include?("%COSTNOW")
return user.hp * cost / 100 if skill.extension.include?("CONSUMEHP")
return user.sp * cost / 100
end
return cost
end
#--------------------------------------------------------------------------
def consum_skill_cost(skill)
return false unless skill_can_use?(skill.id)
cost = calc_sp_cost(self, skill)
return self.hp -= cost if skill.extension.include?("CONSUMEHP")
return self.sp -= cost
end
#--------------------------------------------------------------------------
def attack_effect(attacker)
self.critical = @evaded = @missed = false
hit_result = (rand(100) < attacker.hit)
set_attack_result(attacker) if hit_result
weapon = attacker.actor? ? $data_weapons[attacker.weapon_id] : nil
if hit_result
set_attack_state_change(attacker)
else
self.critical = false
@missed = true
end
self.damage = POP_EVA if @evaded
self.damage = POP_MISS if @missed
return true
end
#--------------------------------------------------------------------------
def perfect_attack_effect(attacker)
self.critical = @evaded = @missed = false
set_attack_result(attacker)
weapon = attacker.actor? ? $data_weapons[attacker.weapon_id] : nil
set_attack_state_change(attacker)
return true
end
#--------------------------------------------------------------------------
def set_attack_result(attacker)
set_attack_damage_value(attacker)
if self.damage > 0
self.damage /= 2 if self.guarding?
set_attack_critical(attacker)
set_critical_damage(attacker) if self.critical
end
apply_variance(15) if self.damage.abs > 0
set_attack_hit_value(attacker)
end
#--------------------------------------------------------------------------
def set_attack_damage_value(attacker)
case DAMAGE_ALGORITHM_TYPE
when 0
atk = [attacker.atk - (self.pdef / 2), 0].max
str = [20 + attacker.str, 0].max
when 1
atk = [attacker.atk - ((attacker.atk * self.pdef) / 1000), 0].max
str = [20 + attacker.str, 0].max
when 2
atk = 20
str = [(attacker.str * 4) - (self.dex * 2) , 0].max
when 3
atk = [(10 + attacker.atk) - (self.pdef / 2), 0].max
str = [(20 + attacker.str) - (self.dex / 2), 0].max
end
self.damage = atk * str / 20
self.damage = 1 if self.damage == 0 and (rand(100) > 40)
self.damage *= elements_correct(attacker.element_set)
self.damage /= 100
end
#--------------------------------------------------------------------------
def apply_variance(variance)
amp = [self.damage.abs * variance / 100, 1].max
self.damage += rand(amp + 1) + rand(amp + 1) - amp
end
#--------------------------------------------------------------------------
def set_attack_hit_value(attacker)
atk_hit = DAMAGE_ALGORITHM_TYPE > 1 ? attacker.agi : attacker.dex
eva = (8 * self.agi / atk_hit) + self.eva
hit = self.damage < 0 ? 100 : 100 - eva
hit = self.cant_evade? ? 100 : hit
hit_result = (rand(100) < hit)
@evaded = true unless hit_result
end
#--------------------------------------------------------------------------
def set_attack_critical(attacker)
atk_crt = DAMAGE_ALGORITHM_TYPE > 1 ? attacker.agi : attacker.dex
self.critical = rand(100) < 5 * atk_crt / self.agi
end
#--------------------------------------------------------------------------
def set_critical_damage(attacker)
self.damage += self.damage
end
#--------------------------------------------------------------------------
def set_attack_state_change(attacker)
remove_states_shock
effective = apply_damage(attacker)
@state_changed = false
states_plus(attacker.plus_state_set)
states_minus(attacker.minus_state_set)
return effective
end
#--------------------------------------------------------------------------
def skill_effect(user, skill)
self.critical = @evaded = @missed = false
if ((skill.scope == 3 or skill.scope == 4) and self.hp == 0) or
((skill.scope == 5 or skill.scope == 6) and self.hp >= 1)
return false
end
effective = false
effective |= skill.common_event_id > 0
hit = skill.hit
hit *= set_skill_hit(user, skill)
hit_result = (rand(100) < hit)
effective |= hit < 100
effective |= set_skill_result(user, skill, effective) if hit_result
if hit_result
effective |= set_skill_state_change(user, skill, effective)
else
@missed = true unless @evaded
end
self.damage = nil unless $game_temp.in_battle
self.damage = POP_EVA if @evaded
self.damage = POP_MISS if @missed
return effective
end
#--------------------------------------------------------------------------
def perfect_skill_effect(user, skill)
self.critical = @evaded = @missed = false
if ((skill.scope == 3 or skill.scope == 4) and self.hp == 0) or
((skill.scope == 5 or skill.scope == 6) and self.hp >= 1)
return false
end
effective = false
effective |= skill.common_event_id > 0
effective |= set_skill_result(user, skill, effective)
effective |= set_skill_state_change(user, skill, effective)
self.damage = nil unless $game_temp.in_battle
return effective
end
#--------------------------------------------------------------------------
def set_skill_hit(user, skill)
if skill.magic?
return 1
else
return user.hit / 100
end
end
#--------------------------------------------------------------------------
def set_skill_result(user, skill, effective)
set_skill_damage_value(user, skill)
if self.damage > 0
self.damage /= 2 if self.guarding?
end
apply_variance(skill.variance) if skill.variance > 0 and self.damage.abs > 0
effective |= set_skill_hit_value(user, skill, effective)
return effective
end
#--------------------------------------------------------------------------
def set_skill_damage_value(user, skill)
power = set_skill_power(user, skill)
if power > 0
case DAMAGE_ALGORITHM_TYPE
when 0,3
power -= (self.pdef * skill.pdef_f) / 200
power -= (self.mdef * skill.mdef_f) / 200
when 1
power -= (power * (self.pdef * skill.pdef_f)) / 100000
power -= (power * (self.mdef * skill.mdef_f)) / 100000
when 2
power -= ((self.dex * 2 * skill.pdef_f) / 100)
power -= ((self.int * 1 * skill.mdef_f) / 100)
end
power = [power, 0].max
end
rate = set_skill_rate(user, skill) unless DAMAGE_ALGORITHM_TYPE == 2
rate = [rate, 0].max
self.damage = power * rate / 20
self.damage *= elements_correct(skill.element_set)
self.damage /= 100
end
#--------------------------------------------------------------------------
def set_skill_power(user, skill)
case DAMAGE_ALGORITHM_TYPE
when 0,1,3
power = skill.power + ((user.atk * skill.atk_f) / 100)
when 2
user_str = (user.str * 4 * skill.str_f / 100)
user_int = (user.int * 2 * skill.int_f / 100)
if skill.power > 0
power = skill.power + user_str + user_int
else
power = skill.power - user_str - user_int
end
end
return power
end
#--------------------------------------------------------------------------
def set_skill_rate(user, skill)
case DAMAGE_ALGORITHM_TYPE
when 0,1,2
rate = 20
when 3
rate = 40
rate -= (self.dex / 2 * skill.pdef_f / 200)
rate -= ((self.dex + self.int)/ 4 * skill.mdef_f / 200)
end
rate += (user.str * skill.str_f / 100)
rate += (user.dex * skill.dex_f / 100)
rate += (user.agi * skill.agi_f / 100)
rate += (user.int * skill.int_f / 100)
return rate
end
#--------------------------------------------------------------------------
def set_skill_hit_value(user, skill, effective)
atk_hit = DAMAGE_ALGORITHM_TYPE > 1 ? user.agi : user.dex
eva = 8 * self.agi / atk_hit + self.eva
hit = self.damage < 0 ? 100 : 100 - eva * skill.eva_f / 100
hit = self.cant_evade? ? 100 : hit
hit_result = (rand(100) < hit)
@evaded = true unless hit_result
effective |= hit < 100
return effective
end
#--------------------------------------------------------------------------
def set_skill_state_change(user, skill, effective = false)
if skill.power != 0 and not skill.magic?
remove_states_shock
effective = true
end
effective |= apply_damage(user)
@state_changed = false
effective |= states_plus(skill.plus_state_set)
effective |= states_minus(skill.minus_state_set)
if skill.power == 0
self.damage = ""
@missed = true unless @state_changed
end
return effective
end
#--------------------------------------------------------------------------
def apply_damage(user)
return true if @evaded or @missed or not self.damage.is_a?(Numeric)
if self.sp_damage
last_sp = self.sp
self.sp -= self.damage
effective = self.sp != last_sp
else
last_hp = self.hp
self.hp -= self.damage
effective = self.hp != last_hp
end
return effective
end
#--------------------------------------------------------------------------
def item_effect(item)
self.critical = @evaded = @missed = false
if ((item.scope == 3 or item.scope == 4) and self.hp == 0) or
((item.scope == 5 or item.scope == 6) and self.hp >= 1)
return false
end
effective = false
effective |= item.common_event_id > 0
hit_result = (rand(100) < item.hit)
@missed = true unless hit_result
effective |= item.hit < 100
if hit_result == true
effective |= make_item_damage_value(item)
else
@missed = true
end
self.damage = nil unless $game_temp.in_battle
self.damage = POP_MISS if @missed
return effective
end
#--------------------------------------------------------------------------
def perfect_item_effect(item)
self.critical = @evaded = @missed = false
if ((item.scope == 3 or item.scope == 4) and self.hp == 0) or
((item.scope == 5 or item.scope == 6) and self.hp >= 1)
return false
end
effective = false
effective |= item.common_event_id > 0
effective |= item.hit < 100
effective |= make_item_damage_value(item)
self.damage = nil unless $game_temp.in_battle
self.damage = POP_MISS if @missed
return effective
end
#--------------------------------------------------------------------------
def make_item_damage_value(item)
recover_hp = maxhp * item.recover_hp_rate / 100 + item.recover_hp
recover_sp = maxsp * item.recover_sp_rate / 100 + item.recover_sp
if recover_hp < 0
recover_hp += self.pdef * item.pdef_f / 20
recover_hp += self.mdef * item.mdef_f / 20
recover_hp = [recover_hp, 0].min
end
recover_hp *= elements_correct(item.element_set)
recover_hp /= 100
recover_sp *= elements_correct(item.element_set)
recover_sp /= 100
if item.variance > 0 and recover_hp.abs > 0
amp = [recover_hp.abs * item.variance / 100, 1].max
recover_hp += rand(amp+1) + rand(amp+1) - amp
end
if item.variance > 0 and recover_sp.abs > 0
amp = [recover_sp.abs * item.variance / 100, 1].max
recover_sp += rand(amp+1) + rand(amp+1) - amp
end
recover_hp /= 2 if recover_hp < 0 and self.guarding?
self.damage = -recover_hp
last_hp = self.hp
last_sp = self.sp
self.hp += recover_hp
self.sp += recover_sp
effective |= self.hp != last_hp
effective |= self.sp != last_sp
@state_changed = false
effective |= states_plus(item.plus_state_set)
effective |= states_minus(item.minus_state_set)
if item.parameter_type > 0 and item.parameter_points != 0
case item.parameter_type
when 1
@maxhp_plus += item.parameter_points
when 2
@maxsp_plus += item.parameter_points
when 3
@str_plus += item.parameter_points
when 4
@dex_plus += item.parameter_points
when 5
@agi_plus += item.parameter_points
when 6
@int_plus += item.parameter_points
end
effective = true
end
if item.recover_hp_rate == 0 and item.recover_hp == 0
self.damage = ""
if item.recover_sp_rate == 0 and item.recover_sp == 0 and
(item.parameter_type == 0 or item.parameter_points == 0)
unless @state_changed
@missed = true
end
end
end
return effective
end
#--------------------------------------------------------------------------
alias skill_effect_n01 skill_effect
def skill_effect(user, skill)
now_hp = self.hp
if ((skill.scope == 3 or skill.scope == 4) and self.hp == 0) or
((skill.scope == 5 or skill.scope == 6) and self.hp >= 1)
return false
end
effective = skill_effect_n01(user, skill)
return if effective == false
check_extension(skill)
if @ratio_maxdamage != nil
self.damage = self.maxhp * @ratio_maxdamage / 100 unless @sp_damage
self.damage = self.maxsp * @ratio_maxdamage / 100 if @sp_damage
end
if @ratio_nowdamage != nil
self.damage = self.hp * @ratio_nowdamage / 100 unless @sp_damage
self.damage = self.sp * @ratio_nowdamage / 100 if @sp_damage
end
if @cost_damage
cost = calc_sp_cost(user, skill)
if skill.extension.include?("CONSUMEHP")
self.damage = self.damage * cost / user.maxhp
else
self.damage = self.damage * cost / user.maxsp
end
end
self.damage = self.damage * user.hp / user.maxhp if @nowhp_damage
self.damage = self.damage * user.sp / user.maxsp if @nowsp_damage
if @sp_damage
self.hp = now_hp
self.sp -= self.damage
elsif @extension
self.hp = now_hp
self.hp -= self.damage
end
return true
end
#--------------------------------------------------------------------------
alias perfect_skill_effect_n01 perfect_skill_effect
def perfect_skill_effect(user, skill)
now_hp = self.hp
if ((skill.scope == 3 or skill.scope == 4) and self.hp == 0) or
((skill.scope == 5 or skill.scope == 6) and self.hp >= 1)
return false
end
effective = perfect_skill_effect_n01(user, skill)
return if effective == false
check_extension(skill)
if @ratio_maxdamage != nil
self.damage = self.maxhp * @ratio_maxdamage / 100 unless @sp_damage
self.damage = self.maxsp * @ratio_maxdamage / 100 if @sp_damage
end
if @ratio_nowdamage != nil
self.damage = self.hp * @ratio_nowdamage / 100 unless @sp_damage
self.damage = self.sp * @ratio_nowdamage / 100 if @sp_damage
end
if @cost_damage
cost = calc_sp_cost(user, skill)
if skill.extension.include?("CONSUMEHP")
self.damage = self.damage * cost / user.maxhp
else
self.damage = self.damage * cost / user.maxsp
end
end
self.damage = self.damage * user.hp / user.maxhp if @nowhp_damage
self.damage = self.damage * user.sp / user.maxsp if @nowsp_damage
if @sp_damage
self.hp = now_hp
self.sp -= self.damage
elsif @extension
self.hp = now_hp
self.hp -= self.damage
end
return true
end
#--------------------------------------------------------------------------
def check_extension(skill)
@extension = false
@sp_damage = false
@cost_damage = false
@nowhp_damage = false
@nowsp_damage = false
@ratio_maxdamage = nil
@ratio_nowdamage = nil
for ext in skill.extension
break if self.damage == "Errou!" or self.damage == "" or self.damage == 0
if ext == "SPDAMAGE"
next @sp_damage = true
elsif ext == "COSTPOWER"
@extension = true
next @cost_damage = true
elsif ext == "HPNOWPOWER"
@extension = true
next @nowhp_damage = true
elsif ext == "MPNOWPOWER"
@extension = true
next @nowsp_damage = true
else
name = ext.split('')
if name[7] == "M"
name = name.join
name.slice!("%DAMAGEMAX/")
@extension = true
next @ratio_maxdamage = name.to_i
elsif name[7] == "N"
name = name.join
name.slice!("%DAMAGENOW/")
@extension = true
next @ratio_nowdamage = name.to_i
end
end
end
end
#--------------------------------------------------------------------------
def change_base_position(x, y)
@base_position_x = x
@base_position_y = y
end
#--------------------------------------------------------------------------
def reset_coordinate
@move_x = @move_y = @move_z = @jump = @derivation = 0
@active = @non_dead = @individual = false
end
end
#==============================================================================
# ■ Game_Actor
#==============================================================================
class Game_Actor < Game_Battler
#--------------------------------------------------------------------------
attr_reader :armor5_id
attr_reader :armor6_id
attr_reader :armor7_id
attr_accessor :actor_height
attr_accessor :two_swords_change
#--------------------------------------------------------------------------
include N01
#--------------------------------------------------------------------------
def actor?
return true
end
#--------------------------------------------------------------------------
def weapons
return [$data_weapons[@weapon_id]]
end
#--------------------------------------------------------------------------
def armors
result = []
result << $data_armors[@armor1_id]
result << $data_armors[@armor2_id]
result << $data_armors[@armor3_id]
result << $data_armors[@armor4_id]
return result
end
#--------------------------------------------------------------------------
def equips
return weapons + armors
end
#--------------------------------------------------------------------------
def skill_id_learn?(skill_id)
return @skills.include?(skill_id)
end
#--------------------------------------------------------------------------
def exp=(exp)
@exp = [exp, 0].max
while @exp >= @exp_list[@level+1] and @exp_list[@level+1] > 0
@level += 1
for j in $data_classes[@class_id].learnings
if j.level == @level
learn_skill(j.skill_id)
end
end
end
while @exp < @exp_list[@level]
@level -= 1
end
@hp = [@hp, self.maxhp].min
@sp = [@sp, self.maxsp].min
end
#--------------------------------------------------------------------------
def base_atk
n = 0
for item in weapons.compact do n += item.atk end
n = UNARMED_ATTACK if weapons[0] == nil and weapons[1] == nil
return n
end
#--------------------------------------------------------------------------
def base_pdef
n = 0
for item in equips.compact do n += item.pdef end
return n
end
#--------------------------------------------------------------------------
def base_mdef
n = 0
for item in equips.compact do n += item.mdef end
return n
end
#--------------------------------------------------------------------------
def base_eva
n = 0
for item in armors.compact do n += item.eva end
return n
end
#--------------------------------------------------------------------------
def graphic_change(character_name)
@character_name = character_name
end
#--------------------------------------------------------------------------
def perform_collapse
$game_system.se_play($data_system.actor_collapse_se) if $game_temp.in_battle and dead?
end
#--------------------------------------------------------------------------
def base_position
base = ACTOR_POSITION[self.index]
@base_position_x = base[0]
@base_position_y = base[1]
@base_position_x = 640 - base[0] if $back_attack and BACK_ATTACK_MIRROR
end
#--------------------------------------------------------------------------
def actor_height
@base_height = 0 if CURSOR_TYPE != 1
@base_height = 72 if CURSOR_TYPE == 1
return @base_height
end
#--------------------------------------------------------------------------
def position_x
return 0 if self.index == nil
return @base_position_x + @move_x
end
#--------------------------------------------------------------------------
def position_y
return 0 if self.index == nil
return @base_position_y + @move_y + @jump
end
#--------------------------------------------------------------------------
def position_z
return 0 if self.index == nil
return position_y + @move_z - @jump + 200
end
end
#==============================================================================
# ■ Game_Enemy
#==============================================================================
class Game_Enemy < Game_Battler
#--------------------------------------------------------------------------
include N01
#--------------------------------------------------------------------------
attr_accessor :adj_speed
attr_accessor :act_time
#--------------------------------------------------------------------------
def actor?
return false
end
#--------------------------------------------------------------------------
def make_action_speed2(adj)
@adj_speed = @current_action.speed if @adj_speed == nil
@adj_speed = @adj_speed * adj / 100
end
#--------------------------------------------------------------------------
def perform_collapse
@force_action = ["N01collapse"] if $game_temp.in_battle and dead?
end
#--------------------------------------------------------------------------
def element_set2
return []
end
#--------------------------------------------------------------------------
def base_position
return if self.index == nil
bitmap = Bitmap.new("Graphics/Battlers/" + @battler_name) if !self.anime_on
bitmap = Bitmap.new("Graphics/Characters/" + @battler_name) if self.anime_on && WALK_ANIME
bitmap = Bitmap.new("Graphics/Characters/" + @battler_name + "_1") if self.anime_on && !WALK_ANIME
height = bitmap.height
@base_position_x = self.screen_x + self.position_plus[0]
@base_position_y = self.screen_y + self.position_plus[1] - height / 3 + 32
@base_position_x = 640 - self.screen_x - self.position_plus[0] if $back_attack and BACK_ATTACK_MIRROR
@base_height = 0 if CURSOR_TYPE == 0
@base_height = height / 3 + 64 if CURSOR_TYPE == 1 if !self.anime_on
@base_height = height /12 + 64 if CURSOR_TYPE == 1 if self.anime_on
@base_height = -(height / 3) if CURSOR_TYPE == 2 if !self.anime_on
@base_height = -(height /12) if CURSOR_TYPE == 2 if self.anime_on
bitmap.dispose
end
#--------------------------------------------------------------------------
def enemy_height
return @base_height
end
#--------------------------------------------------------------------------
def position_x
return @base_position_x - @move_x
end
#--------------------------------------------------------------------------
def position_y
return @base_position_y + @move_y + @jump
end
#--------------------------------------------------------------------------
def position_z
return position_y + @move_z - @jump + 200
end
end
#==============================================================================
# Arrow_Base
#==============================================================================
class Arrow_Base < Sprite
#--------------------------------------------------------------------------
include N01
#--------------------------------------------------------------------------
def update_multi_arrow
return if @arrows == nil or @arrows == []
for i in [email=0...@arrows.size]0...@arrows.size[/email]
@blink_count = (@blink_count + 1) % 40
if @blink_count < 20
@arrows[i].src_rect.set(128, 96, 32, 32) if @arrows[i] != nil
else
@arrows[i].src_rect.set(160, 96, 32, 32) if @arrows[i] != nil
end
end
end
#--------------------------------------------------------------------------
def dispose_multi_arrow
for i in [email=0...@arrows.size]0...@arrows.size[/email]
@arrows[i].dispose if @arrows[i] != nil
end
end
end
#==============================================================================
# Arrow_Enemy_All
#==============================================================================
class Arrow_Enemy_All < Arrow_Base
#--------------------------------------------------------------------------
def initialize(viewport)
super(viewport)
@arrows = []
for battler in $game_troop.enemies
if battler.exist?
@arrows[battler.index] = Arrow_Enemy.new(viewport)
@arrows[battler.index].index = battler.index
end
end
end
#--------------------------------------------------------------------------
def update_multi_arrow
super
for i in [email=0...@arrows.size]0...@arrows.size[/email]
enemy = $game_troop.enemies[i]
if enemy != nil && @arrows[i] != nil && @arrows[i].enemy != nil
@arrows[i].x = @arrows[i].enemy.position_x + CURSOR_POSITION[0]
@arrows[i].y = @arrows[i].enemy.position_y + CURSOR_POSITION[1] + enemy.enemy_height
end
end
end
end
#==============================================================================
# Arrow_Actor_All
#==============================================================================
class Arrow_Actor_All < Arrow_Base
#--------------------------------------------------------------------------
def initialize(viewport)
super(viewport)
@arrows = []
for battler in $game_party.actors
if battler.exist?
@arrows[battler.index] = Arrow_Actor.new(viewport)
@arrows[battler.index].index = battler.index
end
end
end
#--------------------------------------------------------------------------
def update_multi_arrow
super
for i in [email=0...@arrows.size]0...@arrows.size[/email]
actor = $game_party.actors[i]
if actor != nil && @arrows[i] != nil && @arrows[i].actor != nil
@arrows[i].x = @arrows[i].actor.position_x + CURSOR_POSITION[0]
@arrows[i].y = @arrows[i].actor.position_y + CURSOR_POSITION[1] + actor.actor_height
end
end
end
end
#==============================================================================
# Arrow_Battler_All
#==============================================================================
class Arrow_Battler_All < Arrow_Base
#--------------------------------------------------------------------------
def initialize(viewport)
super(viewport)
@arrows = []
s = 0
for battler in $game_party.actors + $game_troop.enemies
@arrows[s] = Arrow_Actor.new(viewport) if battler.actor?
@arrows[s] = Arrow_Enemy.new(viewport) if battler.is_a?(Game_Enemy)
@arrows[s].index = battler.index
s += 1
end
end
#--------------------------------------------------------------------------
def update_multi_arrow
super
s = 0
for i in [email=0...@arrows.size]0...@arrows.size[/email]
if @arrows[i].is_a?(Arrow_Actor)
actor = $game_party.actors[i]
if @arrows[i].actor != nil
@arrows[i].x = @arrows[i].actor.position_x + CURSOR_POSITION[0]
@arrows[i].y = @arrows[i].actor.position_y + CURSOR_POSITION[1] + actor.actor_height
s += 1
end
elsif @arrows[i].is_a?(Arrow_Enemy)
enemy = $game_troop.enemies[i - s]
if @arrows[i].enemy != nil or @arrows[i] != nil
@arrows[i].x = @arrows[i].enemy.position_x + CURSOR_POSITION[0]
@arrows[i].y = @arrows[i].enemy.position_y + CURSOR_POSITION[1] + enemy.enemy_height
end
end
end
end
end
#==============================================================================
# ■ Arrow_Enemy
#==============================================================================
class Arrow_Enemy < Arrow_Base
#--------------------------------------------------------------------------
def update
super
$game_troop.enemies.size.times do
break if self.enemy.exist?
@index += 1
@index %= $game_troop.enemies.size
end
if Input.repeat?(Input::RIGHT)
cursor_up if $back_attack
cursor_down unless $back_attack
end
if Input.repeat?(Input::LEFT)
cursor_up unless $back_attack
cursor_down if $back_attack
end
if Input.repeat?(Input::UP)
cursor_down
end
if Input.repeat?(Input::DOWN)
cursor_up
end
if self.enemy != nil
self.x = self.enemy.position_x + CURSOR_POSITION[0]
self.y = self.enemy.position_y + CURSOR_POSITION[1] + enemy.enemy_height
end
end
#--------------------------------------------------------------------------
def cursor_up
$game_system.se_play($data_system.cursor_se)
$game_troop.enemies.size.times do
@index += $game_troop.enemies.size - 1
@index %= $game_troop.enemies.size
break if self.enemy.exist?
end
end
#--------------------------------------------------------------------------
def cursor_down
$game_system.se_play($data_system.cursor_se)
$game_troop.enemies.size.times do
@index += 1
@index %= $game_troop.enemies.size
break if self.enemy.exist?
end
end
end
#==============================================================================
# ■ Arrow_Actor
#==============================================================================
class Arrow_Actor < Arrow_Base
#--------------------------------------------------------------------------
def update
super
if Input.repeat?(Input::RIGHT)
cursor_up if $back_attack
cursor_down unless $back_attack
end
if Input.repeat?(Input::LEFT)
cursor_up unless $back_attack
cursor_down if $back_attack
end
if Input.repeat?(Input::UP)
cursor_up
end
if Input.repeat?(Input::DOWN)
cursor_down
end
if self.actor != nil
self.x = self.actor.position_x + CURSOR_POSITION[0]
self.y = self.actor.position_y + CURSOR_POSITION[1] + actor.actor_height
end
end
#--------------------------------------------------------------------------
def cursor_up
$game_system.se_play($data_system.cursor_se)
@index += $game_party.actors.size - 1
@index %= $game_party.actors.size
end
#--------------------------------------------------------------------------
def cursor_down
$game_system.se_play($data_system.cursor_se)
@index += 1
@index %= $game_party.actors.size
end
#--------------------------------------------------------------------------
def input_right
@index += 1
@index %= $game_party.actors.size
end
#--------------------------------------------------------------------------
def input_update_target
if Input.repeat?(Input::RIGHT)
if @index == self.actor.index
cursor_up if $back_attack
cursor_down unless $back_attack
end
end
if Input.repeat?(Input::LEFT)
if @index == self.actor.index
cursor_up unless $back_attack
cursor_down if $back_attack
end
end
if Input.repeat?(Input::UP)
cursor_up if @index == self.actor.index
end
if Input.repeat?(Input::DOWN)
cursor_down if @index == self.actor.index
end
if self.actor != nil
self.x = self.actor.screen_x
self.y = self.actor.screen_y
end
end
end
#==============================================================================
# ■ Game_Troop
#==============================================================================
class Game_Troop
def clear_actions
for enemies in @enemies
enemies.current_action.clear
end
end
end
#==============================================================================
# ■ Window_Command
#==============================================================================
class Window_Command < Window_Selectable
#--------------------------------------------------------------------------
attr_accessor :commands
end
#==============================================================================
# ■ Window_Base
#==============================================================================
class Window_Base < Window
#--------------------------------------------------------------------------
include N01
#--------------------------------------------------------------------------
def draw_actor_state(actor, x, y, width = 0)
status_icon = []
for i in actor.states
if $data_states[i].rating >= 1
begin
status_icon.push(RPG::Cache.icon($data_states[i].name + "_st"))
break if status_icon.size > (Icon_max - 1)
rescue
end
end
end
for icon in status_icon
self.contents.blt(x + X_Adjust, y + Y_Adjust + 4, icon, Rect.new(0, 0, Icon_X, Icon_Y), 255)
x += Icon_X + 2
end
end
#--------------------------------------------------------------------------
def draw_actor_parameter(actor, x, y, type)
case type
when 0
parameter_name = $data_system.words.atk
parameter_value = actor.atk
when 1
parameter_name = $data_system.words.pdef
parameter_value = actor.pdef
when 2
parameter_name = $data_system.words.mdef
parameter_value = actor.mdef
when 3
parameter_name = $data_system.words.str
parameter_value = actor.str
when 4
parameter_name = (DAMAGE_ALGORITHM_TYPE == 0 ? STAT_VIT : $data_system.words.dex)
parameter_value = actor.dex
when 5
parameter_name = $data_system.words.agi
parameter_value = actor.agi
when 6
parameter_name = $data_system.words.int
parameter_value = actor.int
when 7
parameter_name = STAT_EVA
parameter_value = actor.eva
end
self.contents.font.color = system_color
self.contents.draw_text(x, y, 120, 32, parameter_name)
self.contents.font.color = normal_color
self.contents.draw_text(x + 120, y, 36, 32, parameter_value.to_s, 2)
end
end
#==============================================================================
# ■ Window_Help
#==============================================================================
class Window_Help < Window_Base
#--------------------------------------------------------------------------
def initialize
super(0, 0, 640, 64)
self.contents = Bitmap.new(width - 32, height - 32)
self.z = 2000 if $game_temp.in_battle
self.back_opacity = HELP_OPACITY if $game_temp.in_battle
end
#--------------------------------------------------------------------------
def set_text_update(text, align = 0)
self.contents.clear
self.contents.font.color = normal_color
self.contents.draw_text(4, 0, self.width - 40, 32, text, align)
@text = text
@align = align
@actor = nil
self.visible = true
end
#--------------------------------------------------------------------------
def set_enemy(enemy)
text = enemy.name
set_text_update(text, 1)
text_width = self.contents.text_size(text).width
x = (text_width + self.width)/2
status_icon = []
for i in enemy.states
if $data_states[i].rating >= 1
begin
status_icon.push(RPG::Cache.icon($data_states[i].name + "_st"))
break if status_icon.size > (Icon_max - 1)
rescue
end
end
end
for icon in status_icon
self.contents.blt(x + X_Adjust, y + Y_Adjust + 4, icon, Rect.new(0, 0, Icon_X, Icon_Y), 255)
x += Icon_X + 2
end
end
end
#==============================================================================
# ■ Window_Skill
#==============================================================================
class Window_Skill < Window_Selectable
#--------------------------------------------------------------------------
def initialize(actor)
super(0, 128, 640, 352)
@actor = actor
@column_max = 2
refresh
self.index = 0
if $game_temp.in_battle
self.y = 320
self.height = 160
self.z = 2100
self.back_opacity = MENU_OPACITY
end
end
#--------------------------------------------------------------------------
def draw_item(index)
skill = @data[index]
if @actor.skill_can_use?(skill.id)
self.contents.font.color = normal_color
else
self.contents.font.color = disabled_color
end
x = 4 + index % 2 * (288 + 32)
y = index / 2 * 32
rect = Rect.new(x, y, self.width / @column_max - 32, 32)
self.contents.fill_rect(rect, Color.new(0, 0, 0, 0))
bitmap = RPG::Cache.icon(skill.icon_name)
opacity = self.contents.font.color == normal_color ? 255 : 128
self.contents.blt(x, y + 4, bitmap, Rect.new(0, 0, 24, 24), opacity)
self.contents.draw_text(x + 28, y, 204, 32, skill.name, 0)
cost = @actor.calc_sp_cost(@actor, skill)
self.contents.draw_text(x + 232, y, 48, 32, cost.to_s, 2)
end
end
#==============================================================================
# ■ Window_Item
#==============================================================================
class Window_Item < Window_Selectable
#--------------------------------------------------------------------------
def initialize
super(0, 64, 640, 416)
@column_max = 2
refresh
self.index = 0
if $game_temp.in_battle
self.y = 320
self.height = 160
self.z = 2100
self.back_opacity = MENU_OPACITY
end
end
end
#==============================================================================
# ■ Window_PartyCommand
#==============================================================================
class Window_PartyCommand < Window_Selectable
#--------------------------------------------------------------------------
alias initialize_n01 initialize
#--------------------------------------------------------------------------
def initialize
initialize_n01
self.z = 2000
end
end
#==============================================================================
# ■ Window_BattleStatus
#==============================================================================
class Window_BattleStatus < Window_Base
#--------------------------------------------------------------------------
def initialize
super(0, 320, 640, 160)
self.contents = Bitmap.new(width - 32, height - 32)
@level_up_flags = []
for i in 0...$game_party.actors.size
@level_up_flags.push(false)
end
self.z = 2000
self.opacity = STATUS_OPACITY
refresh
end
#--------------------------------------------------------------------------
def update
super
end
end
#==============================================================================
# ■ Window_NameCommand
#==============================================================================
class Window_BattleResult < Window_Base
#--------------------------------------------------------------------------
def initialize(exp, gold, treasures)
@exp = exp
@gold = gold
@treasures = treasures
super(160, 0, 320, @treasures.size * 32 + 64)
self.contents = Bitmap.new(width - 32, height - 32)
self.y = 160 - height / 2
self.back_opacity = 160
self.z = 2000
self.visible = false
refresh
end
end
#==============================================================================
# ■ Window_NameCommand
#==============================================================================
class Window_NameCommand < Window_Base
#--------------------------------------------------------------------------
def initialize(actor, x, y)
super(x, y, 160, 64)
self.contents = Bitmap.new(width - 32, height - 32)
self.contents.font.name = Font.default_name
self.z = 2000
self.back_opacity = COMMAND_OPACITY
refresh(actor)
end
#--------------------------------------------------------------------------
def refresh(actor)
self.contents.clear
self.contents.font.color = normal_color
self.contents.draw_text(0, 0, 128, 32, actor.name, 1) if actor != nil
end
end
#==============================================================================
# ■ RPG::Sprite
#==============================================================================
class RPG::Sprite < ::Sprite
#--------------------------------------------------------------------------
include N01
#--------------------------------------------------------------------------
def initialize(viewport = nil)
super(viewport)
@_whiten_duration = 0
@_appear_duration = 0
@_escape_duration = 0
@_collapse_duration = 0
@_damage_duration = 0
@_animation_duration = 0
@_blink = false
@_damage_durations = []
@time = 0
end
#--------------------------------------------------------------------------
def appear
end
#--------------------------------------------------------------------------
def escape
end
#--------------------------------------------------------------------------
def collapse
end
#--------------------------------------------------------------------------
def damage(value, critical, sp_damage = nil)
dispose_damage(0...@_damage_durations.size)
@_damage_sprites = []
if value.is_a?(Numeric)
damage_string = value.abs.to_s
else
damage_string = value.to_s
end
@damage_size = 1 if !MULTI_POP
@damage_size = damage_string.size if MULTI_POP
for i in 0...@damage_size
letter = damage_string[i..i] if MULTI_POP
letter = damage_string if !MULTI_POP
bitmap = Bitmap.new(160, 48)
bitmap.font.name = DAMAGE_FONT
bitmap.font.size = DMG_F_SIZE
bitmap.font.color.set(0, 0, 0)
bitmap.draw_text(-1, 12-1,160, 36, letter, 1)
bitmap.draw_text(+1, 12-1,160, 36, letter, 1)
bitmap.draw_text(-1, 12+1,160, 36, letter, 1)
bitmap.draw_text(+1, 12+1,160, 36, letter, 1)
if value.is_a?(Numeric) and value < 0
bitmap.font.color.set(HP_REC_COLOR[0],HP_REC_COLOR[1],HP_REC_COLOR[2]) if !sp_damage
bitmap.font.color.set(SP_REC_COLOR[0],SP_REC_COLOR[1],SP_REC_COLOR[2]) if sp_damage
else
bitmap.font.color.set(HP_DMG_COLOR[0],HP_DMG_COLOR[1],HP_DMG_COLOR[2]) if !sp_damage
bitmap.font.color.set(SP_DMG_COLOR[0],SP_DMG_COLOR[1],SP_DMG_COLOR[2]) if sp_damage
bitmap.font.color.set(CRT_DMG_COLOR[0],CRT_DMG_COLOR[1],CRT_DMG_COLOR[2]) if critical
end
bitmap.draw_text(0, 12,160, 36, letter, 1)
if critical and CRITIC_TEXT and i == 0
x_pop = (MULTI_POP ? (damage_string.size - 1) * (DMG_SPACE / 2) : 0)
bitmap.font.size = ((DMG_F_SIZE * 2) / 3).to_i
bitmap.font.color.set(0, 0, 0)
bitmap.draw_text(-1 + x_pop, -1, 160, 20, POP_CRI, 1)
bitmap.draw_text(+1 + x_pop, -1, 160, 20, POP_CRI, 1)
bitmap.draw_text(-1 + x_pop, +1, 160, 20, POP_CRI, 1)
bitmap.draw_text(+1 + x_pop, +1, 160, 20, POP_CRI, 1)
bitmap.font.color.set(CRT_TXT_COLOR[0],CRT_TXT_COLOR[1],CRT_TXT_COLOR[2]) if critical
bitmap.draw_text(0 + x_pop, 0, 160, 20, POP_CRI, 1)
end
if critical and CRITIC_FLASH
$game_screen.start_flash(Color.new(255, 255, 255, 255),10)
end
@_damage_sprites[i] = ::Sprite.new(self.viewport)
@_damage_sprites[i].bitmap = bitmap
@_damage_sprites[i].ox = 80
@_damage_sprites[i].oy = 20
@_damage_sprites[i].x = self.x + i * DMG_SPACE
@_damage_sprites[i].y = self.y - self.oy / 2
@_damage_sprites[i].z = DMG_DURATION + 3000 + i * 2
end
end
#--------------------------------------------------------------------------
def dispose
dispose_damage(0...@_damage_durations.size)
dispose_animation
dispose_loop_animation
if @damage_sprites != nil
for damage_sprite in @damage_sprites
damage_sprite.dispose
end
end
super
end
#--------------------------------------------------------------------------
def update
super
@damage_sprites = [] if @damage_sprites.nil?
@damage_durations = [] if @damage_durations.nil?
if @_damage_sprites != nil
for sprite in @_damage_sprites
if sprite != nil and sprite.visible
x = DMG_X_MOVE
y = DMG_Y_MOVE
d = sprite.z - 3000
m = self.mirror
@damage_sprites.push(Sprite_Damage.new(sprite, x, y, d, m))
sprite.visible = false
end
end
end
for damage_sprite in @damage_sprites
damage_sprite.update
end
for i in 0...@damage_sprites.size
@damage_sprites[i] = nil if @damage_sprites[i].disposed?
end
@damage_sprites.compact!
if @_whiten_duration > 0
@_whiten_duration -= 1
self.color.alpha = 128 - (16 - @_whiten_duration) * 10
end
if @_animation != nil and (Graphics.frame_count % 2 == 0)
@_animation_duration -= 1
update_animation
end
if @_loop_animation != nil and (Graphics.frame_count % 2 == 0)
update_loop_animation
@_loop_animation_index += 1
@_loop_animation_index %= @_loop_animation.frame_max
end
if @_blink
@_blink_count = (@_blink_count + 1) % 32
if @_blink_count < 16
alpha = (16 - @_blink_count) * 6
else
alpha = (@_blink_count - 16) * 6
end
self.color.set(255, 255, 255, alpha)
end
@@_animations.clear
end
#--------------------------------------------------------------------------
def dispose_damage(index)
return if @_damage_sprites == nil
if @_damage_sprites[index].is_a?(::Sprite) and @_damage_sprites[index].bitmap != nil
@_damage_sprites[index].bitmap.dispose
@_damage_sprites[index].dispose
@_damage_durations[index] = 0
end
end
#--------------------------------------------------------------------------
def dispose_animation
if @_animation_sprites != nil
sprite = @_animation_sprites[0]
if sprite != nil
@@_reference_count[sprite.bitmap] -= 1
if @@_reference_count[sprite.bitmap] == 0
sprite.bitmap.dispose
end
end
for sprite in @_animation_sprites
sprite.dispose
end
@_animation_sprites = nil
@_animation = nil
end
@mirror = false
end
#--------------------------------------------------------------------------
def animation_mirror(mirror_effect)
@mirror = mirror_effect
end
#--------------------------------------------------------------------------
def animation_set_sprites(sprites, cell_data, position)
for i in 0..15
sprite = sprites[i]
pattern = cell_data[i, 0]
if sprite == nil or pattern == nil or pattern == -1
sprite.visible = false if sprite != nil
next
end
sprite.visible = true
sprite.src_rect.set(pattern % 5 * 192, pattern / 5 * 192, 192, 192)
if position == 3
if self.viewport != nil
sprite.x = self.viewport.rect.width / 2
sprite.y = self.viewport.rect.height - 160
else
sprite.x = 320
sprite.y = 240
end
else
sprite.x = self.x - self.ox + self.src_rect.width / 2
sprite.y = self.y - self.oy + self.src_rect.height / 2
sprite.y -= self.src_rect.height / 4 if position == 0
sprite.y += self.src_rect.height / 4 if position == 2
end
sprite.x += cell_data[i, 1]
sprite.y += cell_data[i, 2]
sprite.z = 2000
if @mirror
sprite.ox = 88
else
sprite.ox = 104
end
sprite.oy = 96
sprite.zoom_x = cell_data[i, 3] / 100.0
sprite.zoom_y = cell_data[i, 3] / 100.0
sprite.angle = cell_data[i, 4]
sprite.mirror = (cell_data[i, 5] == 1)
if @mirror
if sprite.mirror
sprite.mirror = false
else
sprite.mirror = true
end
end
sprite.opacity = cell_data[i, 6] * self.opacity / 255.0
sprite.blend_type = cell_data[i, 7]
end
end
end
#==============================================================================
# ■ Sprite_Damage
#==============================================================================
class Sprite_Damage < Sprite
#--------------------------------------------------------------------------
include N01
#--------------------------------------------------------------------------
def initialize(sprite, init_x_speed, init_y_speed, duration, mirror)
super(nil)
self.bitmap = sprite.bitmap.dup unless sprite.bitmap.nil?
self.opacity = 255
self.x = sprite.x
self.y = sprite.y
self.z = 3000
self.ox = sprite.ox
self.oy = sprite.oy
@damage_mirror = mirror
@now_x_speed = init_x_speed
@now_y_speed = init_y_speed
@potential_x_energy = 0.0
@potential_y_energy = 0.0
@duration = duration
end
#--------------------------------------------------------------------------
def update
@duration -= 1
return unless @duration <= DMG_DURATION
super
n = self.oy + @now_y_speed
if n <= 0
@now_y_speed *= -1
@now_y_speed /= 2
@now_x_speed /= 2
end
self.oy = [n, 0].max
@potential_y_energy += DMG_GRAVITY
speed = @potential_y_energy.floor
@now_y_speed -= speed
@potential_y_energy -= speed
@potential_x_energy += @now_x_speed if @damage_mirror if POP_MOVE
@potential_x_energy -= @now_x_speed if !@damage_mirror if POP_MOVE
speed = @potential_x_energy.floor
self.ox += speed
@potential_x_energy -= speed
case @duration
when 1..10
self.opacity -= 25
when 0
self.dispose
end
end
end
This is the error message I'm getting
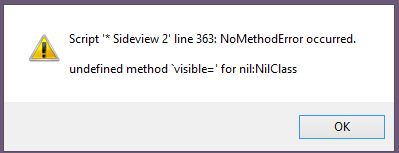
Looking for a fix, I found out someone else had a similar issue years ago, but it was left unresolved.
I'd be willing to switch for another message system entirely...
I actually attempted using another one, Custom Message Script by Hodgeelmf
Code:
#==============================================================================
# ** Custom Message Script by Hodgeelmf **
#
# Version 1.4
#
# Version History
# 1.0.0 - Basic functionality
# 1.1.0 - Fixed issue with bubble size and bolded text
# Modified gold window
# Added /q function
# 1.2.0 - Added Icon display
# Added Face Graphics
# Fixed Gold command iteration error (This is what formerly broke
# the script)
# Added use of Windowskin Graphic
# Fixed sub window termination to be more natural
# 1.3.0 - Added thought indicater option
# Added emotion colors
# 1.4.0 - Fixed glitch when switching from Windowskin to Bubble mode
# Added function to disable bubble bouncing
# 1.4.1 - Fixed text drawing issue when choices displayed without
# preceding message text
# Created \nc command
#
# **************UPDATE NOTE*******************
# This script displays all choices immediately after the text
# which precedes them. I have added a command to prevent this
# should you elect to do so:
#
# \nc
#
# I see no reason to turn this into a toggle currently unless
# there were significant push for it
#
#
# Necessary updates
#
# -Add other various functions like display hp, quest, etc.
#
#==============================================================================
=begin
Welcome to the Custom Message script!
Installation instructions:
1. Install this script above Main, preferrably directly above
2. Download attached graphics (message_bubble and pointer2)
and place in Graphics/Pictures
3. That's it!
Message commands:
\e[x] Display message over a map event
By default, bubble messages display over the player's sprite. Values
outside of the map events range will display over the player as well.
\c[x] Change message text color (0-8)
\n[x] Insert actor name
\v[x] Insert variable value
\b Toggle bold text
\w[x] Wait control (0-999)
Wait values are determined by frame. 40 FPS is the script rate.
\p Pause control
Forces the player to press enter before continuing.
\q Forced ending
Forces the message winddow to close once the character is encountered.
Typically best to use directly after a wait timer.
\f Force position
Forces a specific position for the message bubble.
0 - Upper right of the sprite
1 - Upper left of the sprite
2 - Lower right of the sprite
3 - Lower left of the sprite
\g Displays gold window
Fades in the gold window to the bottom right corner of the screen. To keep
it displayed after current message, add \g into the next master message.
\i[x] Display icon
Displays an icon from the "Icons" folder. A constant array, Icons, is
compiled at runtime to store imported icon names in addition to RTP.
\z[x] Display face graphic (found in Graphics/Faces - you may have to create this
folder)
Displays a face graphic on the left of the message. Currently this script
only supports images with one face (as opposed to a whole faceset).
\t Thought Bubble
Uses thought indicater (ie two dots instead of mouth opening style)
\m[x] Mood Coloring
Changes bubble color to indicate speakers/thinkers mood (0-4)
\nc Do not Display Choices
Keeps choices from being appended to the text preceding them
\h[x] Display Hp
Not functioning at this point
\s[x] Sub window controls (explained below)
Master - the message which controls sub windows
Sub - any message that displays as a part of a master
1. The master message always has a higher z value than any sub messages
2. Sub message must immediately follow the master message and be in
consecutive order (ex. sub 0 must be the next event command after
the master message, sub 1 must be the next event command after
sub 0, etc.)
3. Sub messagge numbers do not reset after a master message. For
example, if the first master messsage contains two sub messages,
the second master message with sub messages must begin with sub
message number 3, and so on.
4. Use \s[x] where x is the number of the sub message to indicate that
a particular message is a sub message
5. Call sub messages in a master message with \s[x] where x is the
number of the sub message.
6. There is no limit to the number of sub messages, but since text is
drawn one letter at a time, too many messages may cause lag.
***NOTE***
If any of these commands seem bulky, it is because there is a glitch in
the run script command in the event editor which does not allow for
single lines changing global variables to function properly.
Script commands (from the Event Editor):
1. Choose message style (bubble, windowskin or invisible)
***** If you do not have the correct images in Graphics/Pictures
***** the script will automatically change to WINDOWSKIN mode and
***** change the default text color to white
$game_system.message_text_mode = x
TEXT_MODE_BUBBLE
TEXT_MODE_INVISIBLE
TEXT_MODE_WINDOWSKIN
2. Enable or disable bubble bouncing (only applies when in TEXT_MODE_BUBBLE)
$game_system.message_bubble_bounce = x
BUBBLE_BOUNCE_ON
BUBBLE_BOUNCE_OFF
2. Change position of invisible text
$game_system.invisible_position = x
INVISIBLE_POSITION_TOP
INVISIBLE_POSITION_MIDDLE
INVISIBLE_POSITION_BOTTOM
INVISIBLE_POSITION_BOOK(arbitrary value from one of my projects)
3. Change outline text options
$game_system.message_text_outline = x
TEXT_NORMAL
TEXT_OUTLINE
4. Change text speed
$game_system.message_text_speed = x
TEXT_SPEED_SLOW
TEXT_SPEED_MEDIUM
TEXT_SPEED_FAST
5. Change text skip rate (one letter per frame up to all at once)
$game_system.message_text_skip = x
TEXT_SKIP_NORMAL (1)
TEXT_SKIP_DOUBLE (2)
TEXT_SKIP_TRIPLE (3)
TEXT_SKIP_FULL
5. Change text size
$game_system.message_text_size = x
TEXT_SIZE_SMALL
TEXT_SIZE_MEDIUM
TEXT_SIZE_LARGE
TEXT_SIZE_JUMBO
6. Change text font
$game_system.message_text_font = x
TEXT_FONT_DEFAULT
TEXT_FONT_CORSIVA
7. Color Constants
TEXT_COLOR_BLACK
TEXT_COLOR_WHITE
8. Face Graphic Functions
$game_system.face_graphic_type = x
9. Face Graphic Constants
FACE_GRAPHIC_FULL - typically a bust which looks better offset to the left
and extending abovve the bubble
FACE_GRAPHIC_BOX - traditional boxed in face graphics which need to be
encased by the bubble to look correct
10. Global Methods (for ease of access)
message(string) - place in script box in event processing
***** Only place this command once per Event Script Box *****
You may wish to have dialogue or entries that are longer than three
lines. This global method allows you to supply a string to
$game_temp.message_text manually. The best way to do so is to store
a message in a variable and pass it to the method. In this example
script there is a Game_Messages class which has public methods that
assign a message to a variable. For full games, it would be better
to create a text file to load from. The best formatter to use is
%q (ex @message = %q(lots of text...can be multiple lines). The
%q formatter mimicks the event dialogue box text editing format
so you can use single "\" characters instead of using "\\" or not
being able to use special characters at all.
default_color(x) - set message default color
Input either a number 0 through 8 or a Color object.
face(string) - set default face graphic
Use this method to supply a default face graphic for messages. This graphic
has secondary priority to one explicitly declared in a message, so is useful
for largely unbroken monologues.
clear_face - clears the default face graphic
small_text
medium_text - Shortcut methods to change $game_system.message_text_size
large_text
jumbo_text
slow_text
fast_text - Shortcut methods to change $game_system.messge_text_speed
Enjoy! And remember to credit!
=end
#========================================================================
# Game_Messages
# You can easily create messages to display of any length using this
# Class or a similar one of your creation.
#========================================================================
class Game_Messages
# This message is obviously far too long for a bubble message, but it looks
# quite good with a darkened screen tone
# When loaded into a global variable, these class methods can be called to
# the event editor with two lines of code. For your own projects, you will
# probably want a more sophisticated heirarchy.
def message_1
@message = %q(\c[0]\bMessage commands:\b
\c[6]\b\\e[x]\c[0] Display message over a map event\b
By default, bubble messages display over the player's sprite. Values
outside of the map events range will display over the player as well.
\c[6]\b\\c[x]\c[0] Change message text color (0-8)\b
\c[6]\b\\n[x]\c[0] Insert actor name\b
\c[6]\b\\v[x]\c[0] Insert variable value\b
\c[6]\b\\\\b\c[0] Toggle bold text\b
\c[6]\b\\w[x]\c[0] Wait control (0-999)\b
Wait values are determined by frame. 40 FPS is the script rate.
\c[6]\b\\\\p\c[0] Pause control\b
Forces the player to press enter before continuing.
\c[6]\b\\\\q\c[0] Forced ending\b
Forces the message winddow to close once the character is encountered.
Typically best to use directly after a wait timer.
\c[6]\b\\\\f[x]\c[0] Force position\b
Forces a specific position for the message bubble.
\b0\b - Upper right of the sprite
\b1\b - Upper left of the sprite
\b2\b - Lower right of the sprite
\b3\b - Lower left of the sprite
\c[6]\b\\\\g\c[0] Displays gold window\b
Fades in the gold window to the bottom right corner of the screen. To keep
it displayed after current message, add \\\\g into the next master message.
\c[6]\b\\\\i[x]\c[0] Displays an icon from Graphics/Icons\b
\c[6]\b\\\\z[x]\c[0] Displays a face graphic from Graphics/Faces\b
References an array compiled at runtime from Graphics/Faces. You will have
to create this folder and manually install files.
\c[6]\b\\\\s[x]\c[0] Sub window controls\b (see documentation for full instructions))
end
def message_2
@message = %q( \c[6]\b$game_system Properties\b\c[0]
Change these properties in the Event Editor by using the Script command. Begin with
$game_system, then add a "." followed by the property you wish to alter. In the
Script command you will need to use two lines. For example:
$game_system.message_text_speed =\
TEXT_SPEED_FAST
The properties are listed below with available values to the right. For full
documentation, refer to the script instructions.
\c[6]\bmessage_text_mode\b\c[0] TEXT_MODE_INVISIBLE,TEXT_MODE_BUBBLE,TEXT_MODE_WINDOWSKIN
\c[6]\binvisible_position\b\c[0] INVISIBLE_POSITION_TOP,INVISIBLE_POSITION_MIDDLE,
INVISIBLE_POSITION_BOTTOM
\c[6]\bmessage_text_outline\b\c[0] TEXT_NORMAL,TEXT_OUTLINE
\c[6]\bmessage_text_speed\b\c[0] TEXT_SPEED_SLOW,TEXT_SPEED_MEDIUM,TEXT_SPEED_FAST
\c[6]\bmessage_text_size\b\c[0] TEXT_SIZE_SMALL,TEXT_SIZE_MEDIUM,TEXT_SIZE_LARGE,
TEXT_SIZE_JUMBO
\c[6]\bmessage_text_font\b\c[0] TEXT_FONT_DEFAULT, whatever constant you would
like to create
\c[6]\bmessage_text_skip\b\c[0] TEXT_SKIP_NORMAL,TEXT_SKIP_DOUBLE,TEXT_SKIP_TRIPLE,
TEXT_SKIP_QUAD,
TEXT_SKIP_QUIN,TEXT_SKIP_FULL
\c[6]\bmessage_skip_disabled\b\c[0] true,false
\c[6]\bface_graphic_type\b\c[0] FACE_GRAPHIC_BOX,FACE_GRAPHIC_FULL
\c[6]\bface_graphic_name\b\c[0] file name of graphic - without folders
\c[6]\bdefault_color\b\c[0] any Color object
\c[6]\bmessage_text_outline_color\b\c[0] any Color object)
end
end
#========================================================================
# Global Variables - simplest way to create communication between
# Interpreter and Window_Message
#========================================================================
$separate_choices = false
#========================================================================
# Constant Declaration
#========================================================================
# Stores the class methods for quick custom message access
$game_messages = Game_Messages.new
BUBBLE_BOUNCE_ON = 1
BUBBLE_BOUNCE_OFF = 0
BUBBLE_UPPER_LEFT = Rect.new(0,0,13,10)
BUBBLE_LOWER_LEFT = Rect.new(0,10,13,9)
BUBBLE_UPPER_RIGHT = Rect.new(13,0,12,10)
BUBBLE_LOWER_RIGHT = Rect.new(13,10,12,9)
SKIN_UPPER_LEFT = Rect.new(128,0,10,10)
SKIN_LOWER_LEFT = Rect.new(128,54,10,10)
SKIN_UPPER_RIGHT = Rect.new(182,0,10,10)
SKIN_LOWER_RIGHT = Rect.new(182,54,10,10)
TEXT_MODE_BUBBLE = 0
TEXT_MODE_INVISIBLE = 1
TEXT_MODE_WINDOWSKIN = 2
INVISIBLE_POSITION_TOP = [320,0]
INVISIBLE_POSITION_MIDDLE = [320,240]
INVISIBLE_POSITION_BOTTOM = [320,480]
INVISIBLE_POSITION_BOOK = [460,35]
TEXT_NORMAL = 0
TEXT_OUTLINE = 1
TEXT_SPEED_SLOW = 2
TEXT_SPEED_MEDIUM = 1
TEXT_SPEED_FAST = 0
TEXT_SKIP_NORMAL = 1
TEXT_SKIP_DOUBLE = 2
TEXT_SKIP_TRIPLE = 3
TEXT_SKIP_QUAD = 4
TEXT_SKIP_QUIN = 5
TEXT_SKIP_FULL = 6
TEXT_SIZE_SMALL = 14
TEXT_SIZE_MEDIUM = 16
TEXT_SIZE_LARGE = 18
TEXT_SIZE_JUMBO = 28
# Remember to set a common default or fallback font since some users won't have
# them all
TEXT_FONT_DEFAULT = "Arial"
TEXT_FONT_CORSIVA = ["Monotype Corsiva", "Arial"]
TEXT_FONT_BOLI = ["MV Boli", "Arial"]
TEXT_FONT_COMICSANS = ["Comic Sans MS", "Arial"]
TEXT_COLOR_BLACK = Color.new(0,0,0)
TEXT_COLOR_WHITE = Color.new(250,250,250)
FACE_GRAPHIC_FULL = 0
FACE_GRAPHIC_BOX = 1
# Compile Icon array
# RTP icons are best stored manually
Icons = ['001-Weapon01','002-Weapon02','003-Weapon03','004-Weapon04'\
,'005-Weapon05','006-Weapon06','007-Weapon07','008-Weapon08'\
,'009-Shield01','010-Head01','011-Head02','012-Head03','013-Body01'\
,'014-Body02','015-Body03','016-Accessory01','017-Accessory02'\
,'018-Accessory03','019-Accessory04','020-Accessory05'\
,'021-Potion01','022-Potion02','023-Potion03','024-Potion04'\
,'025-Herb01','026-Herb02','027-Herb03','028-Herb04'\
,'029-Key01','030-Key02','031-Key03','032-Item01'\
,'033-Item02','034-Item03','035-Item04','036-Item05'\
,'037-Item06','038-Item07','039-Item08','040-Item09'\
,'041-Item10','042-Item11','043-Item12','044-Skill01'\
,'045-Skill02','046-Skill03','047-Skill04','048-Skill05'\
,'049-Skill06','050-Skill07']
# All icons in the icon folder are dynamically added at runtime
ICON_FOLDER_PATH = Dir::getwd + "/Graphics/Icons/"
dir = Dir.new(ICON_FOLDER_PATH)
dir.each { |file|
if file != "." && file != ".."
Icons.push file
end
}
# Compile Face array
if File.directory? Dir::getwd + "/Graphics/Faces/"
Faces = []
FACE_FOLDER_PATH = Dir::getwd + "/Graphics/Faces/"
dir = Dir.new(FACE_FOLDER_PATH)
dir.each { |file|
if file != "." && file != ".."
Faces.push file
end
}
end
#========================================================================
# Global Methods
# Use these methods to quickly modify basic settings or set text
# to $game_temp.message_text manually
#========================================================================
def message(text)
$game_system.map_interpreter.message_waiting = true
$game_temp.message_proc = Proc.new { @message_waiting = false }
$game_temp.message_text = text
end
def default_color(color)
# If an integer passed to the method
if color.class == Fixnum
case color
when 0
$game_system.default_color = TEXT_COLOR_BLACK
when 1
$game_system.default_color = Color.new(128, 128, 255, 255)
when 2
$game_system.default_color = Color.new(255, 128, 128, 255)
when 3
$game_system.default_color = Color.new(128, 255, 128, 255)
when 4
$game_system.default_color = Color.new(128, 255, 255, 255)
when 5
$game_system.default_color = Color.new(255, 128, 255, 255)
when 6
$game_system.default_color = Color.new(255, 255, 128, 255)
when 7
$game_system.default_color = Color.new(192, 192, 192, 255)
when 8
$game_system.default_color = TEXT_COLOR_WHITE
else
$game_system.default_color = TEXT_COLOR_BLACK
end
# If a Color class value is passed to the method
elsif color.class == Color
$game_sytem.default_color = color
end
return true
end
def mood_color(color)
case color
when 0
return Color.new(70,130,180,190)
when 1
return Color.new(178,34,34,190)
when 2
return Color.new(238,174,238,190)
when 3
return Color.new(139,136,120,180)
when 4
return Color.new(255,215,0,190)
end
end
def face(name)
$game_system.face_graphic_name = name
end
def clear_face
$game_system.face_graphic_name = nil
end
def small_text
$game_system.message_text_size == TEXT_SIZE_SMALL
end
def medium_text
$game_system.message_text_size == TEXT_SIZE_MEDIUM
end
def large_text
$game_system.message_text_size == TEXT_SIZE_LARGE
end
def jumbo_text
$game_system.message_text_size == TEXT_SIZE_JUMBO
end
def slow_text
$game_system.message_text_speed == TEXT_SPEED_SLOW
end
def fast_text
$game_system.message_text_speed == TEXT_SPEED_FAST
end
#========================================================================
# Game Temp
#
# @current_event - tracks the current game event for ease of access
#========================================================================
class Game_Temp
attr_accessor :current_event
attr_accessor :consecutive_gold_window
alias old_initialize initialize
def initialize
old_initialize
@current_event = nil
@consecutive_gold_window = false
end
end
#========================================================================
# Game System
#
# Stores message text options
# Use $game_system to view and change values
#========================================================================
class Game_System
attr_accessor :message_text_mode
attr_accessor :message_bubble_bounce
attr_accessor :invisible_position
attr_accessor :message_text_outline
attr_accessor :message_text_speed
attr_accessor :message_text_size
attr_accessor :message_text_font
attr_accessor :message_skip_disabled
attr_accessor :face_graphic_type
attr_accessor :face_graphic_name
attr_accessor :message_text_skip
attr_accessor :default_color
attr_accessor :message_text_outline_color
alias old_initialize initialize
def initialize
old_initialize
@message_text_mode = TEXT_MODE_BUBBLE
@message_bubble_bounce = BUBBLE_BOUNCE_ON
@invisible_position = INVISIBLE_POSITION_TOP
@message_text_outline = TEXT_NORMAL
@message_text_speed = TEXT_SPEED_FAST
@message_text_size = TEXT_SIZE_SMALL
@message_text_font = TEXT_FONT_DEFAULT
@message_text_skip = TEXT_SKIP_NORMAL
@message_skip_disabled = false
@face_graphic_type = FACE_GRAPHIC_BOX
@face_graphic_name = nil
@default_color = TEXT_COLOR_BLACK
@message_text_outline_color = TEXT_COLOR_WHITE
end
end
#========================================================================
# Game Player
#
# The only change is in the condition --if Input.trigger?(Input::C)--
# The change allows for $game_temp.message text to be manually
# altered and still function properly
#========================================================================
class Game_Player
#--------------------------------------------------------------------------
# * Frame Update
#--------------------------------------------------------------------------
def update
# Remember whether or not moving in local variables
last_moving = moving?
# If moving, event running, move route forcing, and message window
# display are all not occurring
unless moving? or $game_system.map_interpreter.running? or
@move_route_forcing or $game_temp.message_window_showing
# Move player in the direction the directional button is being pressed
case Input.dir4
when 2
move_down
when 4
move_left
when 6
move_right
when 8
move_up
end
end
# Remember coordinates in local variables
last_real_x = @real_x
last_real_y = @real_y
super
# If character moves down and is positioned lower than the center
# of the screen
if @real_y > last_real_y and @real_y - $game_map.display_y > CENTER_Y
# Scroll map down
$game_map.scroll_down(@real_y - last_real_y)
end
# If character moves left and is positioned more let on-screen than
# center
if @real_x < last_real_x and @real_x - $game_map.display_x < CENTER_X
# Scroll map left
$game_map.scroll_left(last_real_x - @real_x)
end
# If character moves right and is positioned more right on-screen than
# center
if @real_x > last_real_x and @real_x - $game_map.display_x > CENTER_X
# Scroll map right
$game_map.scroll_right(@real_x - last_real_x)
end
# If character moves up and is positioned higher than the center
# of the screen
if @real_y < last_real_y and @real_y - $game_map.display_y < CENTER_Y
# Scroll map up
$game_map.scroll_up(last_real_y - @real_y)
end
# If not moving
unless moving?
# If player was moving last time
if last_moving
# Event determinant is via touch of same position event
result = check_event_trigger_here([1,2])
# If event which started does not exist
if result == false
# Disregard if debug mode is ON and ctrl key was pressed
unless $DEBUG and Input.press?(Input::CTRL)
# Encounter countdown
if @encounter_count > 0
@encounter_count -= 1
end
end
end
end
#-----------------------------------------------------------------
# Allows messages to display without interruption when directly
# assigned to $game_temp.message_text
#-----------------------------------------------------------------
# If C button was pressed
if Input.trigger?(Input::C) && $game_temp.message_text == nil
# Same position and front event determinant
check_event_trigger_here([0])
check_event_trigger_there([0,1,2])
end
end
end
end
#========================================================================
# Interpreter
#
# Edit forces the interpreter to wait to update until all messages
# currently on screen have finished writing
#========================================================================
class Interpreter
attr_accessor :list
attr_accessor :index
attr_accessor :message_waiting
alias old_update update
def update
#if $scene.message_window != nil && $scene.message_window.finished != nil
if $game_temp.message_text != nil
return
else
old_update
$game_temp.current_event = @event_id
end
end
def command_101
# If other text has been set to message_text
if $game_temp.message_text != nil
# End
return false
end
# Check for consecutive gold window calls
temp_index = @index+1
loop do
case @list[temp_index].code
when 401
temp_index += 1
when 101
break
else
break
end
end
# Search for consecutive message window with /g formatter
text = nil
# Iterate through max possible message size
for i in 0...3
# Check for existence of list element before checking code
if @list[temp_index+i] != nil
# Search for /g
if @list[temp_index+i].code == 101 or @list[temp_index+i].code == 401
text = @list[temp_index+i].parameters[0].slice(/\\[Gg]/)
if text != nil
# Allows for gold window to continue after first message
$game_temp.consecutive_gold_window = true
break
end
end
end
end
# Turns consecutive flag off if need be
if $game_temp.consecutive_gold_window == true && text == nil
$game_temp.consecutive_gold_window = false
end
# Set message end waiting flag and callback
@message_waiting = true
$game_temp.message_proc = Proc.new { @message_waiting = false }
# Set message text on first line
$game_temp.message_text = @list[@index].parameters[0] + "\n"
line_count = 1
# Loop
loop do
# If next event command text is on the second line or after
if @list[@index+1].code == 401
# Add the second line or after to message_text
$game_temp.message_text += @list[@index+1].parameters[0] + "\n"
line_count += 1
# If event command is not on the second line or after
else
# If next event command is show choices
if @list[@index+1].code == 102
# Make sure choice appellation is allowed
for choice in @list[@index+1].parameters[0]
if choice.slice(/\\[Nn][Cc]/)
$separate_choices = true
break
end
end
if $separate_choices == true
return
end
# Advance index
@index += 1
# Choices setup
$game_temp.choice_start = line_count
#Insert 5 spaces for indenting choices
for i in 0...@list[@index].parameters[0].size
if @list[@index].parameters[0][i].slice(0,5) != ' '
@list[@index].parameters[0][i].insert(0,' ')
end
end
setup_choices(@list[@index].parameters)
# If next event command is input number
elsif @list[@index+1].code == 103
# If number input window fits on screen
if line_count < 4
# Advance index
@index += 1
# Number input setup
$game_temp.num_input_start = line_count
$game_temp.num_input_variable_id = @list[@index].parameters[0]
$game_temp.num_input_digits_max = @list[@index].parameters[1]
end
end
# Continue
return true
end
# Advance index
@index += 1
end
end
end
#========================================================================
# Spriteset Map
#
# Edit allows access to the character_sprites variable
# Use $game_map.character_sprites to access
#========================================================================
class Spriteset_Map
attr_accessor :character_sprites
end
class Scene_Map
attr_accessor :spriteset
attr_accessor :message_window
end
#========================================================================
# Window Base
#
# Edit allows for color 8 (black)
#========================================================================
class Window_Base < Window
#--------------------------------------------------------------------------
# * Get Text Color
# n : text color number (0-7)
#--------------------------------------------------------------------------
def text_color(n)
case n
when 0
return Color.new(250, 250, 250, 255)
when 1
return Color.new(45, 45, 255, 255)
when 2
return Color.new(255, 45, 45, 255)
when 3
return Color.new(45, 255, 45, 255)
when 4
return Color.new(45, 215, 215, 255)
when 5
return Color.new(255, 45, 255, 255)
when 6
return Color.new(230, 230, 15, 255)
when 7
return Color.new(125, 125, 125, 255)
when 8
return Color.new(0, 0, 0)
else
normal_color
end
end
end
#==============================================================================
# ** Window_Gold
#------------------------------------------------------------------------------
# The gold window has been modified to use an icon and some outlined text
#==============================================================================
class Window_Gold_New < Window_Base
def initialize
@current_gold = $game_party.gold
@icon = Sprite.new
@icon.opacity = 0
@icon.bitmap = Bitmap.new('Graphics/Icons/032-Item01')
@icon.ox = @icon.bitmap.width/2
@icon.oy = @icon.bitmap.height/2
@icon.x,@icon.y = 624,460
draw_gold
end
def update
refresh
@icon.opacity += 12 if @icon.opacity < 192
@gold.opacity += 12 if @gold.opacity < 192
end
def refresh
if @current_gold != $game_party.gold
@current_gold = $game_party.gold
@gold.bitmap.clear
draw_gold
end
end
def draw_gold
@gold = Sprite.new
@gold.opacity = 0
@gold.bitmap = Bitmap.new(100,32)
@gold.ox,@gold.oy = @gold.bitmap.width/2,@gold.bitmap.height/2
@gold.x = 560
@gold.y = 465
@gold.z = 50
@gold.bitmap.font.size = 18
@gold.bitmap.font.bold = true
@gold.bitmap.font.color = text_color(8)
@gold.bitmap.draw_text(22,0,75,30,$game_party.gold.to_s,2)
@gold.bitmap.draw_text(22,2,75,30,$game_party.gold.to_s,2)
@gold.bitmap.draw_text(21,1,75,30,$game_party.gold.to_s,2)
@gold.bitmap.draw_text(23,1,75,30,$game_party.gold.to_s,2)
@gold.bitmap.font.color = text_color(6)
@gold.bitmap.draw_text(22,1,75,30,@current_gold.to_s,2)
end
def fade_out
@icon.opacity -= 48
@gold.opacity -= 48
end
def dispose
@icon.bitmap.dispose
@icon.dispose
@gold.bitmap.dispose
@gold.dispose
end
end
#==============================================================================
# ** Window_InputNumber
#------------------------------------------------------------------------------
# This window is for inputting numbers, and is used within the
# message window.
#==============================================================================
class Window_InputNumber < Window_Base
attr_accessor :x
attr_accessor :y
#--------------------------------------------------------------------------
# * Object Initialization
# digits_max : digit count
#--------------------------------------------------------------------------
def initialize(digits_max,x=0,y=0,indent=0)
@digits_max = digits_max
@number = 0
@x = x
@y = y
@indent = indent
@count = 0
@move_right = true
# Calculate character width for drawing and tracking cursor movement
dummy_bitmap = Bitmap.new(32, 32)
dummy_bitmap.font.size = $game_system.message_text_size
dummy_bitmap.font.name = $game_system.message_text_font
@width = (dummy_bitmap.text_size('0').width\
+ dummy_bitmap.text_size(' ').width)
@x = @x - (@width*@digits_max)
dummy_bitmap.dispose
@index = 0
# Create number display bitmap
@number_window = Sprite.new
@number_window.x = @x
@number_window.y = @y
@number_window.z = 15
@number_window.bitmap = Bitmap.new(@width*@digits_max, 40)
@number_window.bitmap.font.color = TEXT_COLOR_BLACK
@number_window.bitmap.font.size = $game_system.message_text_size
@number_window.bitmap.font.name = $game_system.message_text_font
# Create the cursor to track input position
create_cursor
end
#--------------------------------------------------------------------------
# * Get Number
#--------------------------------------------------------------------------
def number
return @number
end
#--------------------------------------------------------------------------
# * Set Number
# number : new number
#--------------------------------------------------------------------------
def number=(number)
@number = [[number, 0].max, 10 ** @digits_max - 1].min
refresh
end
#--------------------------------------------------------------------------
# * Create Cursor
#--------------------------------------------------------------------------
def create_cursor
@cursor = Sprite.new
@cursor.bitmap = Bitmap.new(32,5)
@cursor.x = @number_window.x
@cursor.y = @number_window.y\
+ @number_window.bitmap.text_size('0').height + 3
@cursor.z = 16
@cursor.opacity = 185
width = @number_window.bitmap.text_size('0').width + 2
@cursor.bitmap.fill_rect(0,0,width,2,text_color(1))
end
#--------------------------------------------------------------------------
# * Cursor Update
#--------------------------------------------------------------------------
def update_cursor
@count += 1
if @count % 10 == 0
if @move_right == true
@cursor.x += 1
else
@cursor.x -= 1
end
if @count == 20
if @move_right == true
@move_right = false
else
@move_right = true
end
@count = 0
end
end
end
#--------------------------------------------------------------------------
# * Frame Update
#--------------------------------------------------------------------------
def update
update_position
# If up or down directional button was pressed
if Input.repeat?(Input::UP) or Input.repeat?(Input::DOWN)
$game_system.se_play($data_system.cursor_se)
# Get current place number and change it to 0
place = 10 ** (@digits_max - 1 - @index)
n = @number / place % 10
@number -= n * place
# If up add 1, if down substract 1
n = (n + 1) % 10 if Input.repeat?(Input::UP)
n = (n + 9) % 10 if Input.repeat?(Input::DOWN)
# Reset current place number
@number += n * place
refresh
end
# Cursor right
if Input.repeat?(Input::RIGHT)
if @digits_max >= 2
$game_system.se_play($data_system.cursor_se)
@index = (@index + 1) % @digits_max
@cursor.x += @width
if @index == 0
@cursor.x = @number_window.x
end
end
end
# Cursor left
if Input.repeat?(Input::LEFT)
if @digits_max >= 2
$game_system.se_play($data_system.cursor_se)
@index = (@index + @digits_max - 1) % @digits_max
@cursor.x -= @width
if @index == @digits_max - 1
@cursor.x += @width * @digits_max
end
end
end
update_cursor
end
#--------------------------------------------------------------------------
# * Dispose
#--------------------------------------------------------------------------
def dispose
@number_window.bitmap.clear
@number_window = nil
@cursor.bitmap.clear
@cursor = nil
end
#--------------------------------------------------------------------------
# * Update Position for Bubble Movement
#--------------------------------------------------------------------------
def update_position
if @number_window.x != @x
@number_window.x = @x
@cursor.x = @number_window.x + (@index * @width)
end
if @number_window.y != @y
@number_window.y = @y
@cursor.y = @number_window.y\
+ @number_window.bitmap.text_size('0').height + 3
end
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
@number_window.bitmap.clear
s = sprintf("%0*d", @digits_max, @number)
for i in 0...@digits_max
@number_window.bitmap.draw_text(i * @width, 0, 32, 32, s[i,1])
end
end
end
#========================================================================
# Window Message
#
# Controls message displayed from the show text event command
#
# This modified version also allows for a message to be displayed
# from a script. Simply change $game_temp.message_text to
# whatever text you want to display. Remember that outside
# of the message entry box in the event commands, you will have
# to manuall insert the newline character "\n".)
#========================================================================
class Window_Message < Window_Base
attr_accessor :contents_showing
attr_accessor :finished
attr_accessor :fade_in
attr_accessor :fade_out
attr_accessor :message
attr_accessor :enter_pressed
#--------------------------------------------------------------------------
# * Object Initialization
#
# Prepare message variables, then wait for re_initialize
#--------------------------------------------------------------------------
def initialize(sub=false, text=nil)
# Bubble message variables
@enter_pressed = false
@text_height = 0
@fade_in = false
@event = 0
@contents_showing = false
@finished = nil
@position = ''
@active = false
@lines = 0
@up = 0
@count = 0
@cursor_count = 0
@cursor_move_right = nil
@wait = 0
@wait_add = 0
@pause = false
@index = 0
@sub = sub
@face = nil
@face_extension_x = 0
@face_extension_y = 0
@thought = false
@bubble_color = nil
@hp = nil
if @sub == false
@sub_number = nil
@sub_windows = []
end
@indent = false
@text = text
end
#--------------------------------------------------------------------------
# * Re_Initialize
#
# Call when actual text processing occurs
#--------------------------------------------------------------------------
def re_initialize
if $game_temp.message_text != nil
# Prevent improper cursor indention on choices
@indent = false
if $game_system.face_graphic_name != nil
@face = $game_system.face_graphic_name
else
@face = nil
end
@face_extension_x = 0
@face_extension_y = 0
temp = Sprite.new
temp = Bitmap.new(32,32)
# Set @text to whatever text will display
# For sub messages this will already be set
if @text == nil
@text = $game_temp.message_text
end
# Change \ escaped characters to character codes for processing
prep_text
# Determine number of lines in the message
@lines = 0
@text.each_line {|line|
@lines += 1
}
temp_text = @text.clone
# Get rid of any non displayed elements to determine the
# appropriate message box size
def get_digits(integer)
if integer < 10
return 1
elsif integer >= 10
return 2
elsif integer >= 100
return 3
end
end
while temp_text.slice(/\001\[([0-9]+)\]/)
temp_text.slice!(/\001\[([0-9]+)\]/)
end
while temp_text.slice(/\002/)
temp_text.slice!(/\002/)
end
while temp_text.slice(/\004\[([0-9]+)\]/)
temp_text.slice!(/\004\[([0-9]+)\]/)
end
while temp_text.slice(/\005\[([0-9]+)\]/) != nil
temp_text.slice!(/\005\[([0-9]+)\]/)
end
while temp_text.slice(/\006/)
temp_text.slice!(/\006/)
end
while temp_text.slice(/\007\[([0-9]+)\]/)
temp_text.slice!(/\007\[([0-9]+)\]/)
end
while temp_text.slice(/\011/) != nil
temp_text.slice!(/\011/)
end
while temp_text.slice(/\015\[([0-9]+)\]/)
temp_text.slice!(/\015\[([0-9]+)\]/)
@face = Faces[$1.to_i]
end
# Make sure @thought is false unless specified true
@thought = false
while temp_text.slice(/\017/)
temp_text.slice!(/\017/)
@thought = true
@text.slice!(/\017/)
end
# Change @bubble_color to keep default color unless specified other color
@bubble_color = nil
while temp_text.slice(/\018\[([0-9]+)\]/)
temp_text.slice!(/\018\[([0-9]+)\]/)
@bubble_color = mood_color($1.to_i)
@text.slice!(/\018\[([0-9]+)\]/)
end
# Determine message bubble size
bitmap = Sprite.new
bitmap.bitmap = Bitmap.new(32,32)
bitmap.bitmap.font.name = $game_system.message_text_font
bitmap.bitmap.font.size = $game_system.message_text_size
@text_rect = Rect.new(0,0,0,0)
@line_width = []
i = 0
temp_text.each_line {|line|
temp = bitmap.bitmap.text_size(line)
@line_width[i] = temp.width
if temp.width > @text_rect.width
@text_rect.width = temp.width
end
@text_height = temp.height
i += 1
}
# Add space for number input if necessary
if $game_temp.num_input_variable_id > 0 && @sub == false
@lines += 1
end
@text_rect.height = @text_height * @lines
total_size = 0
longest_line = 0
current_line_height = @text_height
@line_height_correction = [0,0,0,0]
line = 0
skip = 0
for i in 0...temp_text.size
# Check for bold toggling and icon insertion
if temp_text[i] == 3
bitmap.bitmap.font.bold == false ? bitmap.bitmap.font.bold = true : bitmap.bitmap.font.bold = false
end
# Check for icon insertion and add 24 pixels to this line's width
if temp_text[i] == 12
# Add sufficient width for an icon to be inserted in the text
total_size += 24
# Adjust the height of the text box if necessary
if current_line_height < 24
current_line_height = 24
@text_rect.height += (24 - @text_height)
@line_height_correction[line] = (24 - @text_height)
end
# Set variable to skip over icon text in sizing operations
skip = 4
end
# Check for face graphic
if temp_text[i] == 13
temp_text.slice(/\015\[([0-9]+)\]/)
skip = 4
@face = Faces[$1.to_i]
end
# Check for new line character
if temp_text[i] == 10
total_size = 0
current_line_height = @text_height
line += 1
end
# Do not size characters relating to an icon
if skip == 0
total_size += bitmap.bitmap.text_size(temp_text.slice(i,1)).width
else
skip -= 1
end
# Make sure longest line sets the bubble width value
longest_line = total_size if total_size > longest_line
end
# Insert facce graphic name if none explicit in message and
# one is stored in $game_system
if $game_system.face_graphic_name != nil && temp_text.slice(/\015\[([0-9]+)\]/) == nil
@face = $game_system.face_graphic_name
end
# Account for face graphic in message box graphic
if @face != nil
determine_face_extension
else
@face_extension_x = 0
@face_extension_y = 0
end
if $game_system.face_graphic_type == FACE_GRAPHIC_FULL
@text_rect.width = longest_line
else
@text_rect.width = longest_line + @face_extension_x
end
# Set start values for text drawing
@x=@y=0
# Determine message position and create the message bubble
#(only if $game_system.message_text_mode == TEXT_MODE_BUBBLE
if $game_system.message_text_mode != TEXT_MODE_INVISIBLE
# Check for existence of necessary Bubble files if mode is set to Bubble
if $game_system.message_text_mode == TEXT_MODE_BUBBLE
# Correct text mode if files do not exist
if File.exist?("Graphics/Pictures/Message_Bubble.png") == false || \
File.exist?("Graphics/Pictures/Pointer2.png") == false
$game_system.message_text_mode = TEXT_MODE_WINDOWSKIN
default_color(8)
end
end
determine_position
create_bubble
else
@bubble_correction_y = 0
@message_box = Sprite.new
@message_box.bitmap = Bitmap.new(@text_rect.width+25, @text_rect.height+26)
@message_box.ox = @message_box.bitmap.width/2
@message_box.oy = @message_box.bitmap.height/2
@message_box.x = $game_system.invisible_position[0]
@message_box.y = $game_system.invisible_position[1]
if @message_box.y - @message_box.bitmap.height/2 < 0
@message_box.y += @message_box.bitmap.height/2
elsif @message_box.y + @message_box.bitmap.height/2 > 480
@message_box.y -= @message_box.bitmap.height/2
end
end
# Create message sprite for drawing actual text
@message = Sprite.new
@message.x = @message_box.x
@message.y = @message_box.y
if @sub == false
@message.z += 1
end
@message.ox = @message_box.ox
@message.oy = @message_box.oy
@message.bitmap = \
Bitmap.new(@message_box.bitmap.width,@message_box.bitmap.height)
@message.bitmap.font.size = $game_system.message_text_size
@message.bitmap.font.color = TEXT_COLOR_BLACK
@speed = $game_system.message_text_speed
@text_color = $game_system.default_color
end
end
#--------------------------------------------------------------------------
# * Dispose
#--------------------------------------------------------------------------
def dispose
terminate_message
$game_temp.message_window_showing = false
if @input_number_window != nil
@input_number_window.dispose
end
end
#--------------------------------------------------------------------------
# * Subs Finished?
#
# Checks to see if all sub messages have finished writing
#--------------------------------------------------------------------------
def subs_finished?
abort_flag = true
count = 0
for sub in @sub_windows
count += 1 if (sub.finished == true && sub.enter_pressed == false)
if sub.finished && sub.enter_pressed
sub.enter_pressed = false
end
end
abort_flag = false if count == @sub_windows.size
if abort_flag == true
return false
else
return true
end
end
#--------------------------------------------------------------------------
# * Erase Subs
#
# Erases any existing subb messages
#--------------------------------------------------------------------------
def erase_subs
if @sub_windows != nil
for sub in @sub_windows
sub.fade_out = true
end
end
end
#--------------------------------------------------------------------------
# * Terminate Message
#--------------------------------------------------------------------------
def terminate_message
# Call message callback
if @message != nil
@message_box.bitmap.clear
@message.z -= 1
@message.bitmap.clear
end
if @cursor != nil
@cursor.bitmap.clear
@cursor.dispose
@cursor = nil
end
if $game_temp.message_proc != nil
$game_temp.message_proc.call
end
# Clear variables related to text, choices, and number input
$game_temp.message_text = nil
$game_temp.message_proc = nil
$game_temp.choice_start = 99
$game_temp.choice_max = 0
$game_temp.choice_cancel_type = 0
$game_temp.choice_proc = nil
$game_temp.num_input_start = 99
$game_temp.num_input_variable_id = 0
$game_temp.num_input_digits_max = 0
# Dispose of gold window
if @gold_window != nil && $game_temp.consecutive_gold_window == false
@gold_window.dispose
@gold_window = nil
end
@contents_showing = false
@finished = nil
@active = false
$game_temp.message_window_showing = false
@text = nil
if @sub == false
erase_subs
temp = subs_finished?
if temp == true
for i in 0...@sub_windows.size
@sub_windows[i] = nil
end
@sub_windows = []
end
end
@sub_number = nil
end
#--------------------------------------------------------------------------
# * Draw_Message
#--------------------------------------------------------------------------
def draw_message
if @pause == true
return
end
if @text == nil
return
end
# Indent if choice and following message text
index = $game_system.map_interpreter.index - 2
code = $game_system.map_interpreter.list[index].code
# Check for choice following a line of text
if @y == $game_temp.choice_start && (code == 101 || code == 401) \
&& @indent == false && $separate_choices == false
@indent = true
#@x = 16 + @face_extension_x
end
if @wait == 0
# Set correction for icon insertion
y_correction = 0
for i in 0..@y
if @line_height_correction[i] != nil
y_correction += @line_height_correction[i]
end
end
# Set offset value dependant on text mode
if $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
offset = 10
elsif $game_system.message_text_mode == TEXT_MODE_BUBBLE
offset = 13
else
offset = 0
end
y = @text_y + (@text_height * @y) + @bubble_correction_y + y_correction
# Insert face graphic if included
if @text.slice!(/\015\[([0-9]+)\]/) || @face != nil
draw_face
end
# Check for formatting options character from text
@c = @text[0,1].slice(/./m)
case @c
# If \\
when "\000"
# Return to original text
@c = "\\"
@text.sub!("\000") {"\\"}
return
# If \C[n]
when "\001"
# Change text color
@text.sub!(/\[([0-9]+)\]/, "")
color = $1.to_i
if color >= 0 and color <= 8
@message.bitmap.font.color = text_color(color)
@text_color = text_color(color)
end
@text.slice!("\001")
# go to next text
return
# If \G
when "\002"
# Make gold window
if @gold_window == nil
@gold_window = Window_Gold_New.new
#@gold_window.x = 560 - @gold_window.width
if $game_temp.in_battle
# @gold_window.y = 192
else
# @gold_window.y = 0
end
#@gold_window.opacity = @message.opacity
#@gold_window.back_opacity = 200
end
@text.slice!("\002")
# go to next text
return
# If \B
when "\003"
if @message.bitmap.font.bold == false
@message.bitmap.font.bold = true
else
@message.bitmap.font.bold = false
end
@text.slice!("\003")
return
# If sub window is being called
when "\004"
@text.slice!("\004")
@text.sub!(/\[([0-9]+)\]/, "")
if @sub == false
@sub_number = $1
event_key = $game_temp.current_event
# Find the cooresponding slave window
# Must immediately follow master window and be
# in consecutive order
num = 0
index = 0
if $game_system.map_interpreter.list[$game_system.map_interpreter.index].code == 101 \
&& num != @sub_number.to_i
for i in $game_system.map_interpreter.index\
...$game_system.map_interpreter.list.size
if $game_system.map_interpreter.list[i].code == 101
num = $game_system.map_interpreter.list[i].parameters[0].slice(/\\[Ss]\[([0-9]+)\]/)
num = num.slice(/([0-9]+)/).to_i if num!= nil
if num != nil
if num != @sub_number.to_i
num += 1
end
index = i
end
if num == @sub_number.to_i
break
end
end
end
else
index = $game_system.map_interpreter.index
end
$game_system.map_interpreter.index = index
# Save original index value for later
original_index = $game_system.map_interpreter.index
lines = 1
if $game_system.map_interpreter.list[index].code == 101
# Loop until the cooresponding \s[x] character is found
loop do
# Break if there is nothing more to sort through
if $game_system.map_interpreter.list[index] == nil
break
end
# Check the \s[x] for a match with the initial match in master message
temp = $game_system.map_interpreter.list[index].parameters.clone
number = temp[0].slice(/\\[Ss]\[([0-9]+)\]/)
if number != nil
number = number.slice(/[0-9]+/)
end
# If the proper match is found, create sub window
if number == @sub_number
# Create message text for sub message
# Make sure sub message begins at first line
while $game_system.map_interpreter.list[index].code != 101
index -= 1
end
text = $game_system.map_interpreter.list[index].parameters[0].clone
if $game_system.map_interpreter.list[index+1].code == 401
text = text + "\n"
lines += 1
end
# Continue to add text if there is something to be added
if $game_system.map_interpreter.list[index+1].code == 401
# Loop until all sub message text is added
loop do
index += 1
text = text + $game_system.map_interpreter.list[index].parameters[0].clone
if $game_system.map_interpreter.list[index+1].code == 401
text = text + "\n"
lines += 1
else
break
end
end
end
# If last sub window, set event index
if @text.slice("\004") == nil
$game_system.map_interpreter.index = index + 1
end
# Create actual sub window object and place it into
# @sub_windows array for update and disposal
sub = Window_Message.new(true,text)
@sub_windows.push sub
break
else
index += 1
end
end # end loop
return
end # end if code == 101
end
# When \w[x]
when "\005"
@text.slice!(/\005\[([0-9]+)\]/)
@wait_add = $1.to_i
@wait += @wait_add
@wait_add = 0
return
# When \p
when "\006"
@text.slice!("\006")
if @enter_pressed == false
@pause = true
end
return
# When \q
when "\011"
terminate_message
return
# When \i[x]
when "\014"
@text.slice!(/\014\[([0-9]+)\]/)
icon = Sprite.new
icon.opacity = 0
icon.bitmap = Bitmap.new("Graphics/Icons/#{Icons[$1.to_i]}")
true_y = @text_y + (@y * @text_height) + y_correction #+ y + @face_extension_y - (@text_rect.height + 25)
@message.bitmap.blt(offset+@x+@face_extension_x,true_y,icon.bitmap,Rect.new(0,0,24,24))
@x += 24
# When \h[x]
when "\016"
@text.slice!(/\016\[([0-9]+)\]/)
@hp = $game_party.actors[$1.to_i].hp
end
# Get 1 character from text
@c = @text.slice!(/./m)
if @c == nil
@finished = true
if $game_temp.choice_max > 0
@cursor_move_right = true
@index = 0
create_cursor
$separate_choices = false
elsif $game_temp.num_input_variable_id > 0 && @sub == false
digits_max = $game_temp.num_input_digits_max
number = $game_variables[$game_temp.num_input_variable_id]
x = @message.x + @message.bitmap.width/2 - 13
y = @message.y - @message.bitmap.height/2\
+ @text_height * (@y) + @bubble_correction_y
@input_number_window = Window_InputNumber.new(digits_max,x,y,@face_extension_x)
@input_number_window.number = number
end
return
end
# If new line text
if @c == "\n"
# Add 1 to y
@y += 1
@x = 0
# Indent if choice
if @y >= $game_temp.choice_start
@x = 0
end
# go to next text
return
end
# Draw text
@message.bitmap.font.name = $game_system.message_text_font
# Set exact y value for drawing
if @face_extension_y != 0
true_y = y + @face_extension_y - (@text_rect.height + 25)
else
true_y = y
end
true_y = @text_y + @y*@text_height + y_correction
if $game_system.message_text_outline == TEXT_OUTLINE
if $game_system.message_text_outline == TEXT_OUTLINE
@message.bitmap.font.color = $game_system.message_text_outline_color
elsif @message.bitmap.font.color != $game_system.default_color
@message.bitmap.font.color = $game_system.default_color
end
# Correct character writing position for lower case "f"
# due to Monotype Corsiva text writing glitch
temp = @x
if @c == 'f' && $game_system.message_text_font == TEXT_FONT_CORSIVA
@x -= 2
end
@message.bitmap.draw_text(offset+@x+@face_extension_x+1,true_y,40,32,@c)
@message.bitmap.draw_text(offset+@x+@face_extension_x-1,true_y,40,32,@c)
@message.bitmap.draw_text(offset+@x+@face_extension_x,true_y+1,40,32,@c)
@message.bitmap.draw_text(offset+@x+@face_extension_x,true_y-1,40,32,@c)
# Correct any change made to @x so that text is written
# in the proper place
if @x != temp
@x = temp
end
end
@message.bitmap.font.color = @text_color
# Correct character writing position for lower case "f"
# due to Monotype Corsiva text writing glitch
temp = @x
if @c == 'f' && $game_system.message_text_font == TEXT_FONT_CORSIVA
@x -= 2
end
@message.bitmap.draw_text(offset+@x+@face_extension_x,true_y,40,32,@c)
# Correct any change made to @x so that text is written
# in the proper place
if @x != temp && $game_system.message_text_font == TEXT_FONT_CORSIVA
@x = temp
end
# Add x to drawn text width
@x += @message.bitmap.text_size(@c).width
@wait = $game_system.message_text_speed
@wait_add = 0
else
@wait -= 1
end
end
#--------------------------------------------------------------------------
# * Determine Face Extension
# This method determines how much distance needs to be added to the
# face graphic
#--------------------------------------------------------------------------
def determine_face_extension
sprite = Sprite.new
sprite.opacity = 0
sprite.bitmap = Bitmap.new("Graphics/Faces/#{@face}")
# Set extension value in horizontal direction
if @face != nil
@face_extension_x = sprite.bitmap.width
if $game_system.face_graphic_type == FACE_GRAPHIC_BOX
@face_extension_x += 5
end
if sprite.bitmap.height > @text_rect.height
@face_extension_y = sprite.bitmap.height - @text_rect.height
end
end
sprite.bitmap.dispose
sprite = nil
end
#--------------------------------------------------------------------------
# * Draw Face Graphics
#--------------------------------------------------------------------------
def draw_face
sprite = Sprite.new
sprite.opacity = 0
sprite.bitmap = Bitmap.new("Graphics/Faces/#{@face}")
rect = Rect.new(0,0,sprite.bitmap.width,sprite.bitmap.height)
if $game_system.face_graphic_type == FACE_GRAPHIC_FULL
@message.bitmap.blt(0,0,sprite.bitmap,rect)
else
y = @text_y + 10
@message.bitmap.blt(13,y,sprite.bitmap,rect)
end
sprite.bitmap.dispose
sprite = nil
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
if @pause == false
if Input.trigger?(Input::C) && $game_system.message_skip_disabled == false
@enter_pressed = true
until @c == nil || @text == nil
draw_message
end
@enter_pressed = false if @sub == false
else
if $game_system.message_text_skip != TEXT_SKIP_FULL
count = $game_system.message_text_skip
for i in 0...count
draw_message if (@c != nil || @text != nil)
end
else
if @c == nil && @text != nil
draw_message
end
until @c == nil || @text == nil
draw_message
end
end
end
else
if Input.trigger?(Input::C)
temp = subs_finished?
if temp == true
erase_subs
@pause = false
end
end
draw_message
end
end
#--------------------------------------------------------------------------
# * Frame Update
#--------------------------------------------------------------------------
def update
# Update sub messages if they exist
if @sub_windows != nil
for i in 0...@sub_windows.size
@sub_windows[i].update
end
end
# Update gold window if it exists
if @gold_window != nil
@gold_window.update
end
# Update bubble graphic
if @active == true && ($game_system.message_text_mode == TEXT_MODE_BUBBLE \
|| $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN)
update_bubble
end
# If fade in
if @fade_in
if $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
max_opacity = 192
else
max_opacity = 255
end
@message_box.opacity += 24
if @input_number_window != nil
@input_number_window.contents_opacity += 24
end
if @message_box.opacity == max_opacity
@fade_in = false
end
return
end
# If fade out
if @fade_out
@message_box.opacity -= 48
@message.opacity -= 48
if @cursor != nil
@cursor.opacity -= 48
end
if @input_number_window != nil
@input_number_window.contents_opacity -= 24
end
if @gold_window != nil && $game_temp.consecutive_gold_window == false
@gold_window.fade_out
end
if @message_box.opacity == 0 && @message.opacity == 0
@fade_out = false
terminate_message if @sub == false
end
return
end
# If inputting number
if @input_number_window != nil
@input_number_window.update
# Confirm
if Input.trigger?(Input::C)
$game_system.se_play($data_system.decision_se)
$game_variables[$game_temp.num_input_variable_id] =
@input_number_window.number
$game_map.need_refresh = true
# Dispose of number input window
@input_number_window.dispose
@input_number_window = nil
if @sub_windows != nil
temp = subs_finished?
if temp == true
@fade_out = true
erase_subs
end
end
end
return
end
# If message is being displayed
if @contents_showing && @finished == true
# If choice isn't being displayed, show pause sign
if $game_temp.choice_max == 0
@active = true
else
update_cursor
end
# Cancel
if Input.trigger?(Input::B)
if $game_temp.choice_max > 0 and $game_temp.choice_cancel_type > 0
$game_system.se_play($data_system.cancel_se)
$game_temp.choice_proc.call($game_temp.choice_cancel_type - 1)
terminate_message
end
end
# Confirm
if Input.trigger?(Input::C)
if $game_temp.choice_max > 0
$game_system.se_play($data_system.decision_se)
$game_temp.choice_proc.call(@index)
end
if @sub_windows != nil
temp = subs_finished?
if temp == true
@fade_out = true
erase_subs
end
end
end
return
end
# If display wait message or choice exists when not fading out
if $game_temp.message_text != nil && @finished == nil
@contents_showing = true
$game_temp.message_window_showing = true
@finished = false
re_initialize
Graphics.frame_reset
if @input_number_window != nil
@input_number_window.contents_opacity = 0
end
@fade_in = true
return
elsif @finished == false
refresh
return
end
end
#--------------------------------------------------------------------------
# Create Message Bubble
#--------------------------------------------------------------------------
# Remember! The bubble will currently display based on the src_rect of
# the $character_sprite bitmap...need to work on this...
def create_bubble # Text can be multiple lines
sprites = $scene.spriteset.character_sprites
@event_x = sprites[@event].x
@event_y = sprites[@event].y
# Draw bubble bitmap to blt from
bubble = Sprite.new
if @position.include?('Flipped_Down')
@bubble_correction_y = 7
else
@bubble_correction_y = 0
end
# Set basic x and y values for bubble drawing
# If a face graphic will be drawn
if @face != nil
# If face graphic drawn outside bubble
if $game_system.face_graphic_type == FACE_GRAPHIC_FULL
base_y = (@face_extension_y + @text_rect.height) - (@text_rect.height + 20)
# If bubble is flipped horizontally
if @position.include?('Mirror')
base_x = 0
else
base_x = @face_extension_x
end
# If bubble is not flipped vertically
if not @position.include?('Flipped_Down')
base_y -= 6
end
base_height = @text_rect.height
height_extension = @face_extension_y
if $game_system.message_text_mode == TEXT_MODE_BUBBLE
width_extension = @face_extension_x + 25
elsif $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
width_extension = @face_extension_x + 20
end
# If face graphic drawn inside bubble
else
base_x = 0
base_y = @bubble_correction_y
base_height = @face_extension_y + @text_rect.height
if $game_system.message_text_mode == TEXT_MODE_BUBBLE
width_extension = 25
height_extension = 20 + 6
elsif $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
width_extension = 20
height_extension = 20
end
end
else
base_x = 0
base_y = @bubble_correction_y
base_height = @text_rect.height
if $game_system.message_text_mode == TEXT_MODE_BUBBLE
height_extension = 20 + 7
width_extension = 25
elsif $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
height_extension = 20
width_extension = 20
end
end
base_width = @text_rect.width
# Set text drawing starting coordinates
@text_y = base_y
if $game_system.message_text_mode == TEXT_MODE_BUBBLE
bubble.bitmap = Bitmap.new("Graphics/Pictures/Message_Bubble")
elsif $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
bubble.bitmap = Bitmap.new("Graphics/Windowskins/"+$data_system.windowskin_name)
end
# Make the bubble bitmap the appropriate size
@message_box = Sprite.new
true_width = base_width + width_extension
true_height = base_height + height_extension
@message_box.bitmap = Bitmap.new(true_width,true_height)
@message_box.ox = @message_box.bitmap.width/2
@message_box.oy = @message_box.bitmap.height/2
@message_box.x = sprites[@event].x\
+ @message_box.bitmap.width/2\
+ sprites[@event].src_rect.width/2 - 15
if $game_system.face_graphic_type == FACE_GRAPHIC_FULL
@message_box.x -= @face_extension_x
end
@message_box.y = sprites[@event].y\
- ((sprites[@event].src_rect.height/3)*2)\
- @message_box.oy
if $game_system.face_graphic_type == FACE_GRAPHIC_FULL \
&& @position.include?('Flipped_Down')
@message_box.y -= @face_extension_y
end
if @sub == false
@message_box.z += 1
end
# Set rectangles for graphic block transfers
if $game_system.message_text_mode == TEXT_MODE_BUBBLE
upper_left = BUBBLE_UPPER_LEFT
lower_left = BUBBLE_LOWER_LEFT
upper_right = BUBBLE_UPPER_RIGHT
lower_right = BUBBLE_LOWER_RIGHT
elsif $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
background = Rect.new(base_x+1,base_y+1,base_width+20-2,base_height+20-2)
source_rect = Rect.new(0,0,128,128)
@message_box.bitmap.stretch_blt(background,bubble.bitmap,source_rect)
upper_left = SKIN_UPPER_LEFT
lower_left = SKIN_LOWER_LEFT
upper_right = SKIN_UPPER_RIGHT
lower_right = SKIN_LOWER_RIGHT
end
# Blt the upper left quadrant of the bubble
rect = Rect.new(0,0,13,10)
@message_box.bitmap.blt(base_x,base_y,bubble.bitmap,upper_left)
# Blt the lower left quadrant of the bubble
rect.set(0,10,13,9)
y = base_y + base_height + lower_left.height
@message_box.bitmap.blt(base_x,y,bubble.bitmap,lower_left)
# Blt the upper right quadrant of the bubble
rect.set(13,0,12,10)
x = base_x + base_width + upper_right.width
@message_box.bitmap.blt(x,base_y,bubble.bitmap,upper_right)
# Blt the lower right quadrant of the bubble
rect.set(13,10,12,9)
x = base_x + base_width + lower_right.width
y = base_y + base_height + upper_right.height
@message_box.bitmap.blt(x,y,bubble.bitmap,lower_right)
if $game_system.message_text_mode == TEXT_MODE_BUBBLE
# Fill in the left side gap
rect.set(0,8,13,1)
x = base_x
y = base_y + upper_left.height
rect2 = Rect.new(x,y,13,base_height)
@message_box.bitmap.stretch_blt(rect2,bubble.bitmap,rect)
# Fill in the right side gap
rect.set(13,8,12,1)
x = base_x + base_width + upper_right.width
y = base_y + upper_right.height
rect2 = Rect.new(x,y,13,base_height)
@message_box.bitmap.stretch_blt(rect2,bubble.bitmap,rect)
# Fill in the middle gap
x = base_x + upper_left.width - 1
y = base_y
width = base_width
height = base_height + upper_left.height + lower_left.height
rect.set(x,y,1,height)
rect2.set(x,y,base_width,height)
@message_box.bitmap.stretch_blt(rect2,@message_box.bitmap,rect)
# Place character pointer either below or above bubble
if @position.include?('Flipped_Down')
if @thought == false
rect.set(15,19,10,8)
else
rect.set(25,8,10,8)
end
x = base_x + 9
y = base_y - @bubble_correction_y
@message_box.bitmap.blt(x,y,bubble.bitmap,rect)
@message_box.y = sprites[@event].y\
- ((sprites[@event].src_rect.height/3)*2)\
+ @message_box.oy - @face_extension_y#- (offset_y-@text_rect.height-20)
else
if @thought == false
rect.set(0,19,10,8)
else
rect.set(25,0,10,8)
end
x = base_x + 9
y = base_y + base_height + 17
@message_box.bitmap.blt(x,y,bubble.bitmap,rect)
end
if @position.include?('Mirror')
@message_box.mirror = true
@message_box.x = sprites[@event].x\
- sprites[@event].src_rect.width/2\
- @message_box.bitmap.width/2 + 15 + base_x #+offset_x
end
# Fill in rim of a windowskin box
elsif $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
# Top rim
dest_rect = Rect.new(base_x+10,base_y,base_width,10)
source_rect = Rect.new(148,0,24,10)
@message_box.bitmap.stretch_blt(dest_rect,bubble.bitmap,source_rect)
# Bottom rim
dest_rect = Rect.new(base_x+10,base_y+base_height+20-10,base_width,10)
source_rect = Rect.new(148,54,24,10)
@message_box.bitmap.stretch_blt(dest_rect,bubble.bitmap,source_rect)
# Left rim
dest_rect = Rect.new(base_x,base_y+10,10,base_height)
source_rect = Rect.new(128,10,10,44)
@message_box.bitmap.stretch_blt(dest_rect,bubble.bitmap,source_rect)
# Right rim
dest_rect = Rect.new(base_x+base_width+20-10,base_y+10,10,base_height)
source_rect = Rect.new(182,10,10,44)
@message_box.bitmap.stretch_blt(dest_rect,bubble.bitmap,source_rect)
end
# Prepare bubble for up/down animation
@up = true
@message_box.opacity = 0
@fade_in = true
# Dispose of the blted bubble
bubble.bitmap.dispose
bubble = nil
@contents_showing = true
@active = true
if @bubble_color.class == Color
@message_box.color = @bubble_color
end
end
#--------------------------------------------------------------------------
# * Determine Bubble Position
#--------------------------------------------------------------------------
def determine_position(no_text=false)
sprites = $scene.spriteset.character_sprites
@position = ''
# Check upper screen boundary
temp = @text_rect.height + 25 + sprites[@event].src_rect.height
if sprites[@event].y - temp < 0
@position += 'Flipped_Down'
end
temp = @text_rect.width + 45
if sprites[@event].x\
+ sprites[@event].src_rect.width/2 + temp > 640
@position += 'Mirror'
end
if no_text == false
if @text.slice(/\007\[([0-9]+)\]/)
#c = @text.slice(/[0-9]+/).to_i
@text.slice!(/\007\[([0-9]+)\]/)
c = $1.to_i
@position = ''
if c == 0
@position = ''
elsif c == 1
@position = 'Flipped_Down'
elsif c == 2
@position = 'Mirror'
elsif c == 3
@position = 'Flipped_Down Mirror'
end
end
end
end
#--------------------------------------------------------------------------
# * Bubble Graphic Update
#--------------------------------------------------------------------------
def update_bubble
# Create an easier to use access point to the screen sprites
sprites = $scene.spriteset.character_sprites
# Bounce bubble
if $game_system.message_text_mode == TEXT_MODE_BUBBLE \
&& $game_system.message_bubble_bounce == BUBBLE_BOUNCE_ON
@count += 1
if @count % 10 == 0 && @up == true
@message_box.y -= 1
if @input_number_window != nil
@input_number_window.y -= 1
end
if @cursor != nil
@cursor.y -= 1
end
if @count == 20
@count = 0
@up = false
end
elsif @count % 10 == 0 && @up == false
@message_box.y += 1
if @input_number_window != nil
@input_number_window.y += 1
end
if @cursor != nil
@cursor.y += 1
end
if @count == 20
@count = 0
@up = true
end
end
end
x = @event_x
y = @event_y
@event_x = sprites[@event].x
@event_y = sprites[@event].y
if @event_x != x || @event_y != y
if @event_x != x
@message_box.x = @message_box.x + (@event_x - x)
if @input_number_window != nil
@input_number_window.x = @input_number_window.x + (@event_x-x)
end
if @cursor != nil
@cursor.x = @cursor.x + (@event_x - x)
end
end
if @event_y - @face_extension_y != y
@message_box.y = @message_box.y + (@event_y - y)
if @input_number_window != nil
@input_number_window.y = @input_number_window.y + (@event_y-y)
end
if @cursor != nil
@cursor.y = @cursor.y + (@event_y - y)
end
end
change = false
# Check upper screen boundary
temp = @message_box.y - @message_box.bitmap.height/2
if temp < 0 && (@position.include?('Flipped_Down') == false)
change = true
end
# Check lower screen boundary
temp = @message_box.y + @message_box.bitmap.height/2
if temp > 480
change = true
end
# Check right screen boundary
temp = @message_box.x + @message_box.bitmap.width/2
if temp > 640
change = true
end
# Check left screen boundary
temp = @message_box.x - @message_box.bitmap.width/2
if temp < 0
change = true
end
if change == true
@message_box.bitmap.clear
determine_position(true)
create_bubble
if @cursor != nil
reset_cursor
end
if @position.include?('Flipped_Down')
get_text
end
@message_box.z -= 1
end
end
@message.y = @message_box.y
@message.x = @message_box.x
end
#--------------------------------------------------------------------------
# * Get Message Text
#--------------------------------------------------------------------------
def get_text
index = $game_system.map_interpreter.index - 1
until $game_system.map_interpreter.list[index].code == 101
index -= 1
end
old_text = @text.clone
@text = ""
@lines.times do
if $game_system.map_interpreter.list[index].code == 102 \
|| $game_system.map_interpreter.list[index].code == 402
for i in 0...$game_system.map_interpreter.list[index].parameters.size
if $game_system.map_interpreter.list[index].parameters[0][i].class == String
@text = @text + $game_system.map_interpreter.list[index].parameters[0][i].clone + "\n"
end
end
else
@text = @text + $game_system.map_interpreter.list[index].parameters[0].clone + "\n"
end
index += 1
end
prep_text
@message.bitmap.clear
@x = 0
@x += 10 if $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
@y = 0
begin
draw_message
end until @text == old_text
end
#--------------------------------------------------------------------------
# * Prepare Text for processing
#--------------------------------------------------------------------------
def prep_text
# Determine what event the message will be over
if @text.slice!(/\\[Ee]\[([0-9]+)\]/) == nil
@event = -1 # Default to over player
else
@event = $1.to_i - 1
end
# Control text processing
begin
@last_text = @text.clone
@text.gsub!(/\\[Vv]\[([0-9]+)\]/) { $game_variables[$1.to_i] }
end until @text == @last_text
@text.gsub!(/\\[Nn]\[([0-9]+)\]/) do
$game_actors[$1.to_i] != nil ? "#{$game_actors[$1.to_i].name}" : ""
end
# Change "\\\\" to "\000" for convenience
@text.gsub!(/\\\\/) { "\000" }
# Change "\\C" to "\001" and "\\G" to "\002"
# and "\\B" to "\003" and "\\S" to "\004"
# and "\\W" to "\005" and "\\p" to "\006"
# and "\\F" to "\007" and "\\q" to "\011"
# and "\\I" to "\014 and "\\z" to "\015"
# and "\\T" to "\017 and "\\m" to "\018"
while @text.slice(/\\[Cc]\[([0-9]+)\]/) != nil
@text.gsub!(/\\[Cc]\[([0-9]+)\]/) { "\001[#{$1}]" }
end
@text.gsub!(/\\[Gg]/) { "\002" }
@text.gsub!(/\\[Bb]/) { "\003" }
@text.gsub!(/\\[Ss]/) { "\004" }
while @text.slice(/\\[Ww]\[([0-9]+)\]/) != nil
@text.gsub!(/\\[Ww]\[([0-9]+)\]/) { "\005[#{$1}]" }
end
while @text.slice(/\\[Pp]/) != nil
@text.gsub!(/\\[Pp]/) { "\006" }
end
while @text.slice(/\\[Ii]/) != nil
@text.gsub!(/\\[Ii]\[([0-9]+)\]/) { "\014[#{$1}]" }
end
while @text.slice(/\\[Zz]/) != nil
# Check for face directory existence
if File.directory? Dir::getwd + "/Graphics/Faces/"
@text.slice(/\\[Zz]\[([0-9]+)\]/)
# Check for file existence
if Faces[$1.to_i] != nil
@text.gsub!(/\\[Zz]\[([0-9]+)\]/) { "\015[#{$1}]" }
else
@text.gsub!(/\\[Zz]\[([0-9]+)\]/) { "" }
end
else
@text.gsub!(/\\[Zz]\[([0-9]+)\]/) { "" }
end
end
while @text.slice(/\\[Hh]/) != nil
@text.gsub!(/\\[Hh]\[([0-9]+)\]/) { "\016[#{$1}]" }
end
@text.gsub!(/\\[Ff]/) { "\007" }
@text.gsub!(/\\[Qq]/) { "\011" }
@text.gsub!(/\\[Tt]/) { "\017" }
while @text.slice(/\\[Mm]\[([0-9]+)]/)
@text.gsub!(/\\[Mm]\[([0-9]+)]/) { "\018[#{$1}]" }
end
@text.slice!(/\\[Nn][Cc]/)
end
#--------------------------------------------------------------------------
# * Create cursor graphic
#--------------------------------------------------------------------------
def create_cursor
if $game_system.message_text_mode == TEXT_MODE_BUBBLE
@cursor = Sprite.new
@cursor.bitmap = Bitmap.new("Graphics/Pictures/Pointer2")
@cursor.ox = @cursor.bitmap.width
if $separate_choices == false
indent = @message.bitmap.text_size(" ").width
else
indent = @message.bitmap.text_size(" ").width
end
elsif $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
template = Sprite.new
template.opacity = 0
template.bitmap = Bitmap.new("Graphics/Windowskins/"+$data_system.windowskin_name)
source_rect = Rect.new(128,64,32,32)
# Set width of the cursor rectangle
width = @line_width[@lines-$game_temp.choice_max+@index]
# Correct for added spaces in choices following main text
width -= @message.bitmap.text_size(' ').width if @indent == true
dest_rect = Rect.new(0,0,width,@text_height)
if @cursor == nil
@cursor = Sprite.new
else
@cursor.bitmap.clear
@cursor.bitmap.dispose
end
@cursor.opacity = 255
@cursor.bitmap = Bitmap.new(width,@text_height)
# Upper left
source_rect = Rect.new(128,64,3,3)
@cursor.bitmap.blt(0,0,template.bitmap,source_rect)
# Upper right
source_rect = Rect.new(157,64,3,3)
@cursor.bitmap.blt(width-3,0,template.bitmap,source_rect)
# Lower left
source_rect = Rect.new(128,93,3,3)
@cursor.bitmap.blt(0,@text_height-3,template.bitmap,source_rect)
# Lower right
source_rect = Rect.new(157,93,3,3)
@cursor.bitmap.blt(width-3,@text_height-3,template.bitmap,source_rect)
# Top fill in
source_rect = Rect.new(131,64,26,3)
dest_rect = Rect.new(3,0,width-6,3)
@cursor.bitmap.stretch_blt(dest_rect,template.bitmap,source_rect)
# Bottom fill in
source_rect = Rect.new(131,93,26,3)
dest_rect = Rect.new(3,@text_height-3,width-6,3)
@cursor.bitmap.stretch_blt(dest_rect,template.bitmap,source_rect)
# Left fill in
source_rect = Rect.new(128,67,3,@text_height-6)
dest_rect = Rect.new(0,3,3,@text_height-6)
@cursor.bitmap.stretch_blt(dest_rect,template.bitmap,source_rect)
# Right fill in
source_rect = Rect.new(157,67,3,@text_height-6)
dest_rect = Rect.new(width-3,3,3,@text_height-6)
@cursor.bitmap.stretch_blt(dest_rect,template.bitmap,source_rect)
# Center fill in
source_rect = Rect.new(131,67,26,26)
dest_rect = Rect.new(3,3,width-6,@text_height-6)
@cursor.bitmap.stretch_blt(dest_rect,template.bitmap,source_rect)
@cursor.ox = 0
template.bitmap.clear
template = nil
indent = @message.bitmap.text_size(" ").width
end
@cursor.oy = @cursor.bitmap.height/2
if @indent == true
@cursor.x = @message.x - @message.ox + 14 + @face_extension_x + indent
else
@cursor.x = @message.x - @message.ox + @face_extension_x + indent
end
y_correction = 0
@line_height_correction.each { |number|
y_correction += number
}
@cursor.y = @message.y - @message.oy + @bubble_correction_y \
+ 10 + @text_height/2 + ((@lines-$game_temp.choice_max+@index)*@text_height)\
+ y_correction
@cursor.z += 5
# More accurately place windowskin rectangle
if $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
@cursor.y -= 1
end
# Correct for bad sizing of bitmap draw_text method
if $game_system.message_text_size == TEXT_SIZE_LARGE
@cursor.y -= 2
elsif
$game_system.message_text_size == TEXT_SIZE_JUMBO
@cursor.y -= 6
end
end
#--------------------------------------------------------------------------
# * Reset cursor graphic
#--------------------------------------------------------------------------
def reset_cursor
@cursor.x = @message_box.x - @message_box.ox + 16
@cursor.y = @message_box.y - @message_box.oy\
+ ((@lines-$game_temp.choice_max + 1)*@text_height)\
+ @bubble_correction_y
end
#--------------------------------------------------------------------------
# * Update cursor graphic
#--------------------------------------------------------------------------
def update_cursor
if $game_system.message_text_mode == TEXT_MODE_BUBBLE
modulus = 10
elsif $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
modulus = 3
end
@cursor_count += 1
if @cursor_count % modulus == 0 && @cursor_move_right == true
if $game_system.message_text_mode == TEXT_MODE_BUBBLE
@cursor.x += 1
elsif $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
@cursor.opacity -= 10
end
if @cursor_count == modulus * 5
@cursor_count = 0
@cursor_move_right = false
end
elsif @cursor_count % modulus == 0 && @cursor_move_right == false
if $game_system.message_text_mode == TEXT_MODE_BUBBLE
@cursor.x -= 1
elsif $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
@cursor.opacity += 10
end
if @cursor_count == modulus * 5
@cursor_count = 0
@cursor_move_right = true
end
end
if Input.trigger?(Input::UP)
if @index != 0
@cursor.y -= @text_height
@index -= 1
else
@cursor.y += @text_height * ($game_temp.choice_max-1)
@index = $game_temp.choice_max-1
end
if $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
create_cursor
end
elsif Input.trigger?(Input::DOWN)
if @index != $game_temp.choice_max-1
@cursor.y += @text_height
@index += 1
else
@cursor.y -= @text_height * ($game_temp.choice_max-1)
@index = 0
end
if $game_system.message_text_mode == TEXT_MODE_WINDOWSKIN
create_cursor
end
end
end
end
I thought of maybe figuring a way to temporarily disable it in gameplay
So, deactivate it before a fight that requires speech, using boring-default-speech, and then reactivate it
But my no-scripting-skills self couldn't figure how to do that without breaking anything.
I'm assuming the issues stem from the fact SBS uses non-default spritesets?... I'm not exactly a scripter, so I just stumble in the dark here. I figured maybe someone else could know how to actually fix this.
Is anyone able to help make this work?
Thank you for your patience.