Kain Nobel
Member
Introduction
This is a neat little extra to add to your game, it allows you to set cursors to any and all of your selectable windows. The customization is super simple, wether you want to use one constant cursor throughout all of your window scenes, or you want all of your windows to each have their own special cursor, its up to you! Since there are many cursors of all shapes and sizes, its easy to line up the coordinates just how you need them without EVER having to edit/hardcode it into the script! Lastly (although I chose to leave mine on) you can even decide if you'd like to hide the actual Cursor Rect from your windowskin while using this script.
Instructions
Place this script anywhere below SDK (XP if using) and above 'main' at the bottom of your scripts page. You can set which directory you would like to list your cursor images in the settings module (its "Graphics/Windowskins/Cursors/" by default). The settings module prettymuch explains itself, its the easiest thing in the world.
Sceenshots
Script
[spoiler='Window.CursorSprite' (XP w/ SDK)]
[/spoiler]
[spoiler='Window.CursorSprite (XP w/o SDK)]Its the same as above, just replace Scene_File in the SDK Version with...
...Then delete the SDK.log, SDK.enabled? test and the last 'end' in the script.[/spoiler]
[spoiler='Window.CursorSprite' (VX)]
[/spoiler]
http://i224.photobucket.com/albums/dd28 ... cursor.png[/img]
I believe credit goes to gRaJiVa for the finger?
Author's Notes
Free to use in commercial and non-commercial games, please remember to credit me and have fun :thumb:
This is a neat little extra to add to your game, it allows you to set cursors to any and all of your selectable windows. The customization is super simple, wether you want to use one constant cursor throughout all of your window scenes, or you want all of your windows to each have their own special cursor, its up to you! Since there are many cursors of all shapes and sizes, its easy to line up the coordinates just how you need them without EVER having to edit/hardcode it into the script! Lastly (although I chose to leave mine on) you can even decide if you'd like to hide the actual Cursor Rect from your windowskin while using this script.
Instructions
Place this script anywhere below SDK (XP if using) and above 'main' at the bottom of your scripts page. You can set which directory you would like to list your cursor images in the settings module (its "Graphics/Windowskins/Cursors/" by default). The settings module prettymuch explains itself, its the easiest thing in the world.
Sceenshots
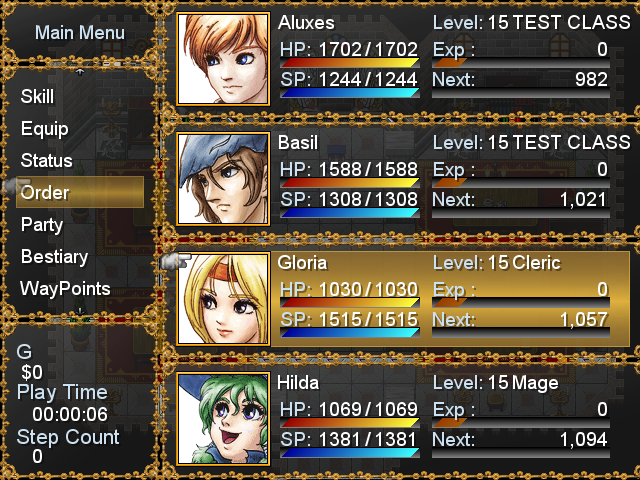
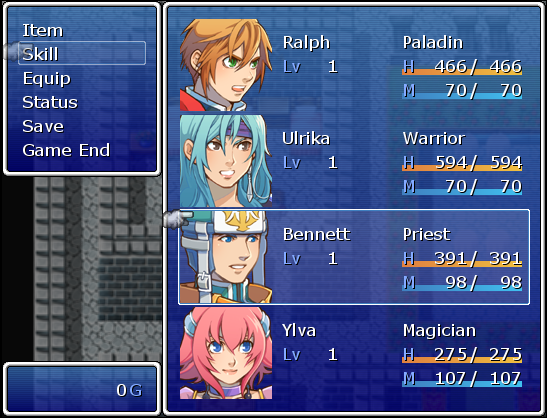
Script
[spoiler='Window.CursorSprite' (XP w/ SDK)]
Code:
#===============================================================================
# ** Window Cursor Sprite                    (by Kain Nobel)
#===============================================================================
#-------------------------------------------------------------------------------
# * SDK Log
#-------------------------------------------------------------------------------
SDK.log('Window.CursorSprite', 'Kain Nobel', 2.5, '12.02.2008')
#-------------------------------------------------------------------------------
# * SDK Enabled Test - BEGIN
#-------------------------------------------------------------------------------
if SDK.enabled?('Window.CursorSprite')
#===============================================================================
# ** Window::Cursor
#===============================================================================
module Window::Cursor
 #-----------------------------------------------------------------------------
 # * Directory = "Graphics/..."
 #-----------------------------------------------------------------------------
 Directory = "Graphics/Windowskins/Cursors"
 #-----------------------------------------------------------------------------
 # * Hide_Cursor_Rect
 #-----------------------------------------------------------------------------
 Hide_SRCRect = false
 #-----------------------------------------------------------------------------
 # * Enabled  = {"Window_???" => true/false}
 #-----------------------------------------------------------------------------
 Enabled = {}
 Enabled.default = true
 #-----------------------------------------------------------------------------
 # * Filenames = {"Window_???" => "filename"}
 #-----------------------------------------------------------------------------
 Filenames = {}
 Filenames.default = "Cursor"
 #-----------------------------------------------------------------------------
 # * Delay   = {"Window_???" => (Integer)} (*Note : Set as 1 or higher)
 #-----------------------------------------------------------------------------
 Delay = {}
 Delay.default = 5
 #-----------------------------------------------------------------------------
 # * OX  = {"Filename" => (Integer)}
 #-----------------------------------------------------------------------------
 OX = {}
 OX.default = 0
 #-----------------------------------------------------------------------------
 # * OY  = {"Filename" => (Integer)}
 #-----------------------------------------------------------------------------
 OY = {}
 OY.default = 16
 #-----------------------------------------------------------------------------
 # * Mirror = {"Filename" => true/false}
 #-----------------------------------------------------------------------------
 Mirror = {}
 Mirror.default = false
 #-----------------------------------------------------------------------------
 # * Angle = {"Filename" => (Numeric)} (*Note: Anything between 0 and 360)
 #-----------------------------------------------------------------------------
 Angle = {}
 Angle.default = 0
 #-----------------------------------------------------------------------------
 # * Opaq  = {"Filename" => (Integer)}
 #-----------------------------------------------------------------------------
 Opaq = {}
 Opaq.default = 200
 #-----------------------------------------------------------------------------
 # * MissingError = true/false
 #-----------------------------------------------------------------------------
 MissingError = true
end
#===============================================================================
# ** Window_Selectable
#===============================================================================
class Window_Selectable < Window_Base
 #-----------------------------------------------------------------------------
 # * Public Instance Variables
 #-----------------------------------------------------------------------------
 attr_accessor :cursor_image
 #-----------------------------------------------------------------------------
 # * Alias Listings
 #-----------------------------------------------------------------------------
 alias_method :cursor_winselect_initialize, :initialize
 alias_method :cursor_winselect_dispose,   :dispose
 alias_method :cursor_winselect_update,   :update
 #-----------------------------------------------------------------------------
 # * Object Initialization
 #-----------------------------------------------------------------------------
 def initialize(*arguments)
  cursor_winselect_initialize(*arguments)
  if cursor_sprite_enabled?
   @cursor_sprite = Sprite_CursorSelectable.new(self)
  end
  @cursor_image = cursor_sprite_image?
 end
 #-----------------------------------------------------------------------------
 # * X = (n)
 #-----------------------------------------------------------------------------
 def x=(n)
  super
  unless @cursor_sprite.nil? || @cursor_sprite.disposed?
   @cursor_sprite.x = cursor_sprite_x?
  end
 end
 #-----------------------------------------------------------------------------
 # * Y = (n)
 #-----------------------------------------------------------------------------
 def y=(n)
  super
  unless @cursor_sprite.nil? || @cursor_sprite.disposed?
   @cursor_sprite.y = cursor_sprite_y?
  end
 end
 #-----------------------------------------------------------------------------
 # * Dispose
 #-----------------------------------------------------------------------------
 def dispose
  unless @cursor_sprite.nil? || @cursor_sprite.disposed?
   @cursor_sprite.dispose
  end
  cursor_winselect_dispose
 end
 #-----------------------------------------------------------------------------
 # * Update
 #-----------------------------------------------------------------------------
 def update
  unless @cursor_sprite.nil? || @cursor_sprite.disposed?
   @cursor_sprite.update
  end
  cursor_winselect_update
 end
 #-----------------------------------------------------------------------------
 # * Cursor Sprite Enabled ?
 #-----------------------------------------------------------------------------
 def cursor_sprite_enabled?
  return Window::Cursor::Enabled[self.class.to_s]
 end
 #-----------------------------------------------------------------------------
 # * Cursor Sprite Image ?
 #-----------------------------------------------------------------------------
 def cursor_sprite_image?
  return Window::Cursor::Filenames[self.class.to_s]
 end
 #-----------------------------------------------------------------------------
 # * Cursor Sprite X ?
 #-----------------------------------------------------------------------------
 def cursor_sprite_x?
  return self.cursor_rect.x + self.x + Window::Cursor::OX[@cursor_image]
 end
 #-----------------------------------------------------------------------------
 # * Cursor Sprite Y ?
 #-----------------------------------------------------------------------------
 def cursor_sprite_y?
  return self.cursor_rect.y + self.y + Window::Cursor::OY[@cursor_image]
 end
 #-----------------------------------------------------------------------------
 # * Cursor Sprite Visible ?
 #-----------------------------------------------------------------------------
 def cursor_sprite_visible?
  return false unless self.index >= 0 && cursor_sprite_enabled?
  return (self.visible && self.opacity > 0)
 end
 #-----------------------------------------------------------------------------
 # * Cursor Sprite Opacity ?
 #-----------------------------------------------------------------------------
 def cursor_sprite_opacity?
  opacity = [Window::Cursor::Opaq[self.class.to_s], 1].max
  return (self.active && self.index >= 0 ? opacity : opacity / 2)
 end
 #-----------------------------------------------------------------------------
 # * Cursor Sprite Delay ?
 #-----------------------------------------------------------------------------
 def cursor_sprite_delay?
  return [Window::Cursor::Delay[self.class.to_s], 1].max
 end
end
#===============================================================================
# ** Sprite_CursorSelectable
#===============================================================================
class Sprite_CursorSelectable < Sprite
 #-----------------------------------------------------------------------------
 # * Object Initialization
 #-----------------------------------------------------------------------------
 def initialize(window)
  super()
  @window = window
  self.x = @window.cursor_sprite_x?
  self.y = @window.cursor_sprite_y?
  update
 end
 #-----------------------------------------------------------------------------
 # * Update Bitmap
 #-----------------------------------------------------------------------------
 def update_bitmap
  unless self.bitmap.nil? || self.bitmap.disposed?
   self.bitmap.dispose
   self.bitmap = nil
  end
  @cursor_image = @window.cursor_image
  self.bitmap = RPG::Cache.window_cursor(@cursor_image)
  self.mirror = Window::Cursor::Mirror[@cursor_image]
  self.angle = Window::Cursor::Angle[@cursor_image]
 end
 #-----------------------------------------------------------------------------
 # * Update : X
 #-----------------------------------------------------------------------------
 def update_x
  ox, delay = @window.cursor_sprite_x?, @window.cursor_sprite_delay?
  n = [(self.x < ox ? (ox - self.x) : (self.x - ox)) / delay, 1].max
  self.x += (self.x < ox ? n : -n)
 end
 #-----------------------------------------------------------------------------
 # * Update : Y
 #-----------------------------------------------------------------------------
 def update_y
  oy, delay = @window.cursor_sprite_y?, @window.cursor_sprite_delay?
  n = [(self.y < oy ? (oy - self.y) : (self.y - oy)) / delay, 1].max
  self.y += (self.y < oy ? n : -n)
 end
 #-----------------------------------------------------------------------------
 # * Update
 #-----------------------------------------------------------------------------
 def update
  super
  update_bitmap if @cursor_image != @window.cursor_image
  update_x   if self.x != @window.cursor_sprite_x?
  update_y   if self.y != @window.cursor_sprite_y?
  self.opacity = @window.cursor_sprite_opacity?
  self.visible = @window.cursor_sprite_visible?
  self.z = (@window.z + 5)
 end
end
#===============================================================================
# ** Scene_File
#===============================================================================
class Scene_File < SDK::Scene_Base
 #-----------------------------------------------------------------------------
 # * Alias Listings
 #-----------------------------------------------------------------------------
 alias_method :cursor_scnfile_mainsprite, :main_sprite
 alias_method :cursor_scnfile_update,   :update
 #-----------------------------------------------------------------------------
 # ** Main Sprite
 #-----------------------------------------------------------------------------
 def main_sprite
  cursor_scnfile_mainsprite
  if Window::Cursor::Enabled["Window_SaveFile"]
   @cursor_sprite = Sprite.new
   @cursor_image = Window::Cursor::Filenames["Window_SaveFile"]
   @cursor_sprite.bitmap = RPG::Cache.window_cursor(@cursor_image)
   @cursor_sprite.mirror = Window::Cursor::Mirror[@cursor_image]
   @cursor_sprite.angle = Window::Cursor::Angle[@cursor_image]
  end
 end
 #-----------------------------------------------------------------------------
 # * Selected Window?
 #-----------------------------------------------------------------------------
 def selected_window?
  for i in 0...@savefile_windows.size
   if @savefile_windows[i].selected == true
    return @savefile_windows[i]
   end
  end
 end
 #-----------------------------------------------------------------------------
 # * Update
 #-----------------------------------------------------------------------------
 def update
  cursor_scnfile_update
  w, c = selected_window?, @cursor_sprite
  oy = w.cursor_rect.y + w.y + Window::Cursor::OY[@cursor_image]
  c.x = w.cursor_rect.x + w.x + Window::Cursor::OX[@cursor_image]
  c.z = (w.z + 5)
  if c.y != oy
   @cs_delay ||= Window::Cursor::Delay["Window_SaveFile"]
   n = [(c.y < oy ? (oy - c.y) : (c.y - oy)) / @cs_delay, 1].max
   c.y += (c.y < oy ? n : -n)
  end
 end
end
#===============================================================================
# ** RPG::Cache
#===============================================================================
module RPG::Cache
 #-----------------------------------------------------------------------------
 # * Alias Listings
 #-----------------------------------------------------------------------------
 class << self
  alias_method :cursor_rpgcache_windowskin, :windowskin
 end
 #-----------------------------------------------------------------------------
 # * RPG::Cache.windowskin
 #-----------------------------------------------------------------------------
 def self.windowskin(filename)
  bitmap = cursor_rpgcache_windowskin(filename)
  if Window::Cursor::Hide_SRCRect
   bitmap.fill_rect(128, 64, 32, 32, Color.new(0,0,0,0))
  end
  return bitmap
 end
 #-----------------------------------------------------------------------------
 # * RPG::Cache.window_cursor(filename)
 #-----------------------------------------------------------------------------
 def self.window_cursor(filename)
  directory = Window::Cursor::Directory
  if directory.length > 0
   unless directory[-1] == "/"
    directory += "/"
   end
  end
  begin
   unless Dir.exists?(directory)
    Dir.mkdir(directory)
   end
   self.load_bitmap(directory, filename)
  rescue
   if Window::Cursor::MissingError
    self.load_bitmap(directory, filename)
   end
  end
 end
end
#-------------------------------------------------------------------------------
# * SDK Enabled Test - END
#-------------------------------------------------------------------------------
end
[spoiler='Window.CursorSprite (XP w/o SDK)]Its the same as above, just replace Scene_File in the SDK Version with...
Code:
#===============================================================================
# ** Scene_File
#===============================================================================
class Scene_File
 #-----------------------------------------------------------------------------
 # * Alias Listings
 #-----------------------------------------------------------------------------
 alias_method :cursor_scnfile_main,    :main
 alias_method :cursor_scnfile_update,   :update
 #-----------------------------------------------------------------------------
 # ** Main Sprite
 #-----------------------------------------------------------------------------
 def main
  if Window::Cursor::Enabled["Window_SaveFile"]
   @cursor_sprite = Sprite.new
   @cursor_image = Window::Cursor::Filenames["Window_SaveFile"]
   @cursor_sprite.bitmap = RPG::Cache.window_cursor(@cursor_image)
   @cursor_sprite.mirror = Window::Cursor::Mirror[@cursor_image]
   @cursor_sprite.angle = Window::Cursor::Angle[@cursor_image]
  end
  cursor_scnfile_main
 end
 #-----------------------------------------------------------------------------
 # * Selected Window?
 #-----------------------------------------------------------------------------
 def selected_window?
  for i in 0...@savefile_windows.size
   if @savefile_windows[i].selected == true
    return @savefile_windows[i]
   end
  end
 end
 #-----------------------------------------------------------------------------
 # * Update
 #-----------------------------------------------------------------------------
 def update
  cursor_scnfile_update
  w, c = selected_window?, @cursor_sprite
  oy = w.cursor_rect.y + w.y + Window::Cursor::OY[@cursor_image]
  c.x = w.cursor_rect.x + w.x + Window::Cursor::OX[@cursor_image]
  c.z = (w.z + 5)
  if c.y != oy
   @cs_delay ||= Window::Cursor::Delay["Window_SaveFile"]
   n = [(c.y < oy ? (oy - c.y) : (c.y - oy)) / @cs_delay, 1].max
   c.y += (c.y < oy ? n : -n)
  end
 end
end
...Then delete the SDK.log, SDK.enabled? test and the last 'end' in the script.[/spoiler]
[spoiler='Window.CursorSprite' (VX)]
Code:
#===============================================================================
# ** Window Cursor Sprite
#===============================================================================
# Written by : Kain Nobel
# Version   : 2.5
# Last Update : 12.02.2008
#===============================================================================
#===============================================================================
# ** Window::Cursor
#===============================================================================
module Window::Cursor
 #-----------------------------------------------------------------------------
 # * Directory = "Graphics/..."
 #-----------------------------------------------------------------------------
 Directory = "Graphics/System"
 #-----------------------------------------------------------------------------
 # * Enabled  = {"Window_???" => true/false}
 #-----------------------------------------------------------------------------
 Enabled = {}
 Enabled.default = true
 #-----------------------------------------------------------------------------
 # * Hide_Cursor_Rect
 #-----------------------------------------------------------------------------
 Hide_SRCRect = true
 #-----------------------------------------------------------------------------
 # * Filenames = {"Window_???" => "filename"}
 #-----------------------------------------------------------------------------
 Filenames = {}
 Filenames.default = "Cursor"
 #-----------------------------------------------------------------------------
 # * Delay   = {"Window_???" => (Integer)} (*Note : Set as 1 or higher)
 #-----------------------------------------------------------------------------
 Delay = {}
 Delay.default = 5
 #-----------------------------------------------------------------------------
 # * OX  = {"Filename" => (Integer)}
 #-----------------------------------------------------------------------------
 OX = {}
 OX.default = 0
 #-----------------------------------------------------------------------------
 # * OY  = {"Filename" => (Integer)}
 #-----------------------------------------------------------------------------
 OY = {}
 OY.default = 16
 #-----------------------------------------------------------------------------
 # * Mirror = {"Filename" => true/false}
 #-----------------------------------------------------------------------------
 Mirror = {}
 Mirror.default = false
 #-----------------------------------------------------------------------------
 # * Angle = {"Filename" => (Numeric)} (*Note: Anything between 0 and 360)
 #-----------------------------------------------------------------------------
 Angle = {}
 Angle.default = 0
 #-----------------------------------------------------------------------------
 # * Opaq  = {"Filename" => (Integer)}
 #-----------------------------------------------------------------------------
 Opaq = {}
 Opaq.default = 200
 #-----------------------------------------------------------------------------
 # * MissingError = true/false
 #-----------------------------------------------------------------------------
 MissingError = true
end
#===============================================================================
# ** Window_Selectable
#===============================================================================
class Window_Selectable < Window_Base
 #-----------------------------------------------------------------------------
 # * Public Instance Variables
 #-----------------------------------------------------------------------------
 attr_accessor :cursor_image
 #-----------------------------------------------------------------------------
 # * Alias Listings
 #-----------------------------------------------------------------------------
 alias_method :cursor_winselect_initialize, :initialize
 alias_method :cursor_winselect_dispose,   :dispose
 alias_method :cursor_winselect_update,   :update
 #-----------------------------------------------------------------------------
 # * Object Initialization
 #-----------------------------------------------------------------------------
 def initialize(*arguments)
  cursor_winselect_initialize(*arguments)
  if cursor_sprite_enabled?
   @cursor_sprite = Sprite_CursorSelectable.new(self)
  end
  @cursor_image = cursor_sprite_image?
 end
 #-----------------------------------------------------------------------------
 # * X = (n)
 #-----------------------------------------------------------------------------
 def x=(n)
  super
  unless @cursor_sprite.nil? || @cursor_sprite.disposed?
   @cursor_sprite.x = cursor_sprite_x?
  end
 end
 #-----------------------------------------------------------------------------
 # * Y = (n)
 #-----------------------------------------------------------------------------
 def y=(n)
  super
  unless @cursor_sprite.nil? || @cursor_sprite.disposed?
   @cursor_sprite.y = cursor_sprite_y?
  end
 end
 #-----------------------------------------------------------------------------
 # * Dispose
 #-----------------------------------------------------------------------------
 def dispose
  unless @cursor_sprite.nil? || @cursor_sprite.disposed?
   @cursor_sprite.dispose
  end
  cursor_winselect_dispose
 end
 #-----------------------------------------------------------------------------
 # * Update
 #-----------------------------------------------------------------------------
 def update
  unless @cursor_sprite.nil? || @cursor_sprite.disposed?
   @cursor_sprite.update
  end
  cursor_winselect_update
 end
 #-----------------------------------------------------------------------------
 # * Cursor Sprite Enabled ?
 #-----------------------------------------------------------------------------
 def cursor_sprite_enabled?
  return Window::Cursor::Enabled[self.class.to_s]
 end
 #-----------------------------------------------------------------------------
 # * Cursor Sprite Image ?
 #-----------------------------------------------------------------------------
 def cursor_sprite_image?
  return Window::Cursor::Filenames[self.class.to_s]
 end
 #-----------------------------------------------------------------------------
 # * Cursor Sprite X ?
 #-----------------------------------------------------------------------------
 def cursor_sprite_x?
  return self.cursor_rect.x + self.x + Window::Cursor::OX[@cursor_image]
 end
 #-----------------------------------------------------------------------------
 # * Cursor Sprite Y ?
 #-----------------------------------------------------------------------------
 def cursor_sprite_y?
  return self.cursor_rect.y + self.y + Window::Cursor::OY[@cursor_image]
 end
 #-----------------------------------------------------------------------------
 # * Cursor Sprite Visible ?
 #-----------------------------------------------------------------------------
 def cursor_sprite_visible?
  return false unless self.index >= 0 && cursor_sprite_enabled?
  return (self.visible && self.opacity > 0)
 end
 #-----------------------------------------------------------------------------
 # * Cursor Sprite Opacity ?
 #-----------------------------------------------------------------------------
 def cursor_sprite_opacity?
  opacity = [Window::Cursor::Opaq[self.class.to_s], 1].max
  return (self.active && self.index >= 0 ? opacity : opacity / 2)
 end
 #-----------------------------------------------------------------------------
 # * Cursor Sprite Delay ?
 #-----------------------------------------------------------------------------
 def cursor_sprite_delay?
  return [Window::Cursor::Delay[self.class.to_s], 1].max
 end
end
#===============================================================================
# ** Sprite_CursorSelectable
#===============================================================================
class Sprite_CursorSelectable < Sprite
 #-----------------------------------------------------------------------------
 # * Object Initialization
 #-----------------------------------------------------------------------------
 def initialize(window)
  super()
  @window = window
  self.x = @window.cursor_sprite_x?
  self.y = @window.cursor_sprite_y?
  update
 end
 #-----------------------------------------------------------------------------
 # * Update Bitmap
 #-----------------------------------------------------------------------------
 def update_bitmap
  unless self.bitmap.nil? || self.bitmap.disposed?
   self.bitmap.dispose
   self.bitmap = nil
  end
  @cursor_image = @window.cursor_image
  self.bitmap = Cache.window_cursor(@cursor_image)
  self.mirror = Window::Cursor::Mirror[@cursor_image]
  self.angle = Window::Cursor::Angle[@cursor_image]
 end
 #-----------------------------------------------------------------------------
 # * Update : X
 #-----------------------------------------------------------------------------
 def update_x
  ox, delay = @window.cursor_sprite_x?, @window.cursor_sprite_delay?
  n = [(self.x < ox ? (ox - self.x) : (self.x - ox)) / delay, 1].max
  self.x += (self.x < ox ? n : -n)
 end
 #-----------------------------------------------------------------------------
 # * Update : Y
 #-----------------------------------------------------------------------------
 def update_y
  oy, delay = @window.cursor_sprite_y?, @window.cursor_sprite_delay?
  n = [(self.y < oy ? (oy - self.y) : (self.y - oy)) / delay, 1].max
  self.y += (self.y < oy ? n : -n)
 end
 #-----------------------------------------------------------------------------
 # * Update
 #-----------------------------------------------------------------------------
 def update
  super
  update_bitmap if @cursor_image != @window.cursor_image
  update_x   if self.x != @window.cursor_sprite_x?
  update_y   if self.y != @window.cursor_sprite_y?
  self.opacity = @window.cursor_sprite_opacity?
  self.visible = @window.cursor_sprite_visible?
  self.z = (@window.z + 5)
 end
end
#===============================================================================
# ** Scene_File
#===============================================================================
class Scene_File < Scene_Base
 #-----------------------------------------------------------------------------
 # * Alias Listings
 #-----------------------------------------------------------------------------
 alias_method :cursor_scnfile_start,   :start
 alias_method :cursor_scnfile_terminate, :terminate
 alias_method :cursor_scnfile_update,   :update
 #-----------------------------------------------------------------------------
 # ** Main Sprite
 #-----------------------------------------------------------------------------
 def start
  cursor_scnfile_start
  if Window::Cursor::Enabled["Window_SaveFile"]
   @cursor_sprite = Sprite.new
   @cursor_image = Window::Cursor::Filenames["Window_SaveFile"]
   begin
    @cursor_sprite.bitmap = Cache.window_cursor(@cursor_image)
    @cursor_sprite.mirror = Window::Cursor::Mirror[@cursor_image]
    @cursor_sprite.angle = Window::Cursor::Angle[@cursor_image]
   rescue
    if Window::Cursor::MissingError
     @cursor_sprite.bitmap = Cache.window_cursor(@cursor_image)
    end
   end
  end
 end
 #-----------------------------------------------------------------------------
 # * Selected Window?
 #-----------------------------------------------------------------------------
 def selected_window?
  for i in 0...@savefile_windows.size
   if @savefile_windows[i].selected == true
    return @savefile_windows[i]
   end
  end
 end
 #-----------------------------------------------------------------------------
 # * Terminate
 #-----------------------------------------------------------------------------
 def terminate
  cursor_scnfile_terminate
  @cursor_sprite.dispose
 end
 #-----------------------------------------------------------------------------
 # * Update
 #-----------------------------------------------------------------------------
 def update
  cursor_scnfile_update
  w, c = selected_window?, @cursor_sprite
  oy = w.cursor_rect.y + w.y + Window::Cursor::OY[@cursor_image]
  c.x = w.cursor_rect.x + w.x + Window::Cursor::OX[@cursor_image]
  c.z = (w.z + 5)
  if c.y != oy
   @cs_delay ||= Window::Cursor::Delay["Window_SaveFile"]
   n = [(c.y < oy ? (oy - c.y) : (c.y - oy)) / @cs_delay, 1].max
   c.y += (c.y < oy ? n : -n)
  end
 end
end
#===============================================================================
# ** Cache
#===============================================================================
module Cache
 #-----------------------------------------------------------------------------
 # * Alias Listings
 #-----------------------------------------------------------------------------
 class << self
  alias_method :cursor_cache_system, :system
 end
 #-----------------------------------------------------------------------------
 # * Cache.system
 #-----------------------------------------------------------------------------
 def self.system(filename)
  bitmap = cursor_cache_system(filename)
  if Window::Cursor::Hide_SRCRect && filename == "Window"
   bitmap.clear_rect(64, 64, 32, 32)
  end
  return bitmap
 end
 #-----------------------------------------------------------------------------
 # * Cache.window_cursor(filename)
 #-----------------------------------------------------------------------------
 def self.window_cursor(filename)
  directory = Window::Cursor::Directory
  if directory.length > 0
   unless directory[-1] == "/"
    directory += "/"
   end
  end
  begin
   unless Dir.exists?(directory)
    Dir.mkdir(directory)
   end
   self.load_bitmap(directory, filename)
  rescue
   if Window::Cursor::MissingError
    self.load_bitmap(directory, filename)
   end
  end
 end
end
http://i224.photobucket.com/albums/dd28 ... cursor.png[/img]
I believe credit goes to gRaJiVa for the finger?
Author's Notes
Free to use in commercial and non-commercial games, please remember to credit me and have fun :thumb: