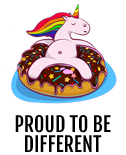
#==============================================================================
# ** Scene_Map
#------------------------------------------------------------------------------
# This class performs map screen processing.
#==============================================================================
class Scene_Map
#--------------------------------------------------------------------------
# * Main Processing
#--------------------------------------------------------------------------
def main
main_draw
# Main loop
loop do
main_loop
break if main_scenechange?
end
main_dispose
main_tiletrigger
end
#--------------------------------------------------------------------------
# * Main Draw
#--------------------------------------------------------------------------
def main_draw
# Make sprite set
@spriteset = Spriteset_Map.new
# Make message window
@message_window = Window_Message.new
if @need_update = 2 or @need_update = 0
#-----
else
@need_update = 0
end
@hud1 = Window_HudEquip.new
@hud2 = Window_HudPortrait.new
@hud3 = Window_HudInfo.new
@hud4 = Window_HudSkills.new
# Transition run
Graphics.transition
@window1 = Window_Quests.new
@window2 = Window_QuestRewards.new
@herowin = Window_HeroStats.new
@petwin = Window_PetStats.new
@help_window = Window_Info.new
@help_window.z = 9000
@help_window.set_text("")
@hud1.z = 200
@hud2.z = 200
@hud3.z = 200
@hud4.z = 200
@window1.z = 200
@window2.z = 200
@herowin.z = 200
@petwin.z = 200
@help_window.z = 200
# Selectable Menu
#s1 = "Player Info"
s2 = "Inventory"
s3 = "Spellbook"
s4 = "Equipment"
s5 = "Skills"
s6 = "Guilds"
s7 = "Pet menu"
s8 = "Log Off"
@list10008548 = Pre_Command.new(130,[s2,s3,s4,s5,s6,s7,s8,],16)
@list10008548.windowskin = RPG::Cache.windowskin('ws1')
@list10008548.z = 9999
@list10008548.x = 30
@list10008548.y = 182
# ----------
@menubutton = Window_MenuButton.new
@list10008548.visible = false
@list10008548.active = false
# Sprites and Images
@fogimage = Sprite.new
@fogimage.bitmap = RPG::Cache.picture('002-fog02.png')
@fogimage.z = 100
end
#--------------------------------------------------------------------------
# * Main Loop
#--------------------------------------------------------------------------
def main_loop
# Update game screen
Graphics.update
# Update input information
Input.update
# Frame update
update
end
#--------------------------------------------------------------------------
# * Main Scene Change
#--------------------------------------------------------------------------
def main_scenechange?
# Abort loop if screen is changed
if $scene != self
return true
end
return false
end
#--------------------------------------------------------------------------
# * Main Dispose
#--------------------------------------------------------------------------
def main_dispose
Graphics.freeze
@spriteset.dispose
automatic_dispose
end
#--------------------------------------------------------------------------
# * Main Tiletrigger
#--------------------------------------------------------------------------
def main_tiletrigger
# If switching to title screen
if $scene.is_a?(Scene_Title)
# Fade out screen
Graphics.transition
Graphics.freeze
end
end
#--------------------------------------------------------------------------
# * Frame Update
#--------------------------------------------------------------------------
def update
# Loop
loop do
update_systems
# Abort loop if player isn't place moving
unless $game_temp.player_transferring
break
end
# Run place move
transfer_player
# Abort loop if transition processing
if $game_temp.transition_processing
break
end
end
update_graphics
update_stuff
return if update_game_over? == true
return if update_to_title? == true
# If transition processing
if $game_temp.transition_processing
# Clear transition processing flag
$game_temp.transition_processing = false
# Execute transition
if $game_temp.transition_name == ""
Graphics.transition(20)
else
Graphics.transition(40, "Graphics/Transitions/" +
$game_temp.transition_name)
end
end
# If showing message window
if $game_temp.message_window_showing
return
end
# If encounter list isn't empty, and encounter count is 0
if $game_player.encounter_count == 0 and $game_map.encounter_list != []
# If event is running or encounter is not forbidden
unless $game_system.map_interpreter.running? or
$game_system.encounter_disabled
# Confirm troop
n = rand($game_map.encounter_list.size)
troop_id = $game_map.encounter_list[n]
# If troop is valid
if $data_troops[troop_id] != nil
# Set battle calling flag
$game_temp.battle_calling = true
$game_temp.battle_troop_id = troop_id
$game_temp.battle_can_escape = true
$game_temp.battle_can_lose = false
$game_temp.battle_proc = nil
end
end
end
update_chat
update_call_menu
update_call_debug
update_winds
check_mouseover
# If player is not moving
unless $game_player.moving?
update_scene
end
end
#--------------------------------------------------------------------------
# * Update Systems
#--------------------------------------------------------------------------
def update_systems
# Update map, interpreter, and player order
# (this update order is important for when conditions are fulfilled
# to run any event, and the player isn't provided the opportunity to
# move in an instant)
$game_map.update
$game_system.map_interpreter.update
$game_player.update
# Update system (timer), screen
$game_system.update
$game_screen.update
end
#--------------------------------------------------------------------------
# * Update
#--------------------------------------------------------------------------
def update_graphics
# Update sprite set
@spriteset.update
# Update message window
@message_window.update
if @list10008548.visible == true
if Input.trigger?(Input::C)
case @list10008548.index
#s1 = "Player Info"
#s2 = "Inventory"
#s3 = "Spellbook"
#s4 = "Equipment"
#s5 = "Skills"
#s6 = "Guilds"
#s7 = "Pet menu"
#s8 = "Log Off"
when 0
# -----
when 1
$game_system.se_play($data_system.decision_se)
$scene=Scene_Item.new
when 2
$game_system.se_play($data_system.decision_se)
$scene=Scene_Skill.new
when 3
$game_system.se_play($data_system.decision_se)
$scene=Scene_Equip.new
when 4
$game_system.se_play($data_system.decision_se)
$scene=Scene_SkillSystem.new
when 5
$game_system.se_play($data_system.decision_se)
$scene=Scene_Guilds.new
when 6
$game_system.se_play($data_system.decision_se)
$Scene=Scene_PetSystem.new
when 7
$game_system.se_play($data_system.decision_se)
$scene=Scene_End.new
# -----
end
return
end
end
if mouse_over?(@menubutton)
if Input.triggerd?(Input::Mouse_Left)
@list10008548.visible = true
@list10008548.active = true
end
if Input.triggerd?(Input::Mouse_Right)
@list10008548.visible = false
@list10008548.active = false
end
end
end
def update_stuff
# Update Hud Windows
@hud1.update
@hud2.update
@hud3.update
@hud4.update
@window1.update
@window2.update
@herowin.update
@petwin.update
@help_window.update
@list10008548.update
@menubutton.update
end
#--------------------------------------------------------------------------
# * Update windows
#--------------------------------------------------------------------------
def update_windows
@window1.update
@window2.update
end
#--------------------------------------------------------------------------
# * Update Game Over
#--------------------------------------------------------------------------
def update_game_over?
# If game over
if $game_temp.gameover
# Switch to game over screen
$scene = Scene_Gameover.new
return true
end
return false
end
#--------------------------------------------------------------------------
# * Update To Title
#--------------------------------------------------------------------------
def update_to_title?
# If returning to title screen
if $game_temp.to_title
# Change to title screen
$scene = Scene_Title.new
return true
end
return false
end
#--------------------------------------------------------------------------
# * Update Menu Call
#--------------------------------------------------------------------------
def update_call_menu
# If B button was pressed
if Input.trigger?(Input::B)
# If event is running, or menu is not forbidden
unless $game_system.map_interpreter.running? or
$game_system.menu_disabled
# Set menu calling flag or beep flag
$game_temp.menu_calling = true
$game_temp.menu_beep = true
end
end
end
#--------------------------------------------------------------------------
# * Update Debug Call
#--------------------------------------------------------------------------
def update_call_debug
# If debug mode is ON and F9 key was pressed
if $DEBUG and Input.press?(Input::F9)
# Set debug calling flag
$game_temp.debug_calling = true
end
end
#--------------------------------------------------------------------------
# * Update Scene
#--------------------------------------------------------------------------
def update_scene
# Run calling of each screen
if $game_temp.battle_calling
call_battle
elsif $game_temp.shop_calling
call_shop
elsif $game_temp.name_calling
call_name
elsif $game_temp.menu_calling
call_menu
elsif $game_temp.save_calling
call_save
elsif $game_temp.debug_calling
call_debug
end
end
#--------------------------------------------------------------------------
# * Update Chat
#--------------------------------------------------------------------------
def update_chat
end
def update_winds
#if Input.trigger?(Input::F8)
# bool |= @window1.visible
# @window1.visible = @window2.visible = bool
#return
#end
#if Input.trigger?(Input::F9)
# bool |= @herowin.visible
# @herowin.visible = @petwin.visible = bool
#end
if Input.pressed?(Input::Fkeys[8])
@window1.visible = true
@window2.visible = true
else
@window1.visible = false
@window2.visible = false
end
if Input.pressed?(Input::Fkeys[9])
@petwin.visible = true
@herowin.visible = true
else
@petwin.visible = false
@herowin.visible = false
end
end
#--------------------------------------------------------------------------
# Check Mouse over window?
#--------------------------------------------------------------------------
def mouse_over?(window)
if $mouse.x > window.x and $mouse.x < window.x + window.width and
$mouse.y > window.y and $mouse.y < window.y + window.height
return true
end
return false
end
def check_mouseover
if mouse_over?(@hud1)
@help_window.contents.draw_text(0, 0, 400, 22, "Line 1")
@help_window.contents.draw_text(0, 22, 400, 22, "Line 2")
@help_window.visible = true
else
if mouse_over?(@hud2)
@help_window.set_text("A window")
@help_window.visible = true
else
if mouse_over?(@hud3)
@help_window.set_text("A window")
@help_window.visible = true
else
if mouse_over?(@hud4)
@help_window.set_text("A window")
@help_window.visible = true
else
#@help_window.text = ""
@help_window.visible = false
end
end
end
end
end
end
class Window_Quests < Window_Base
#------------
# Initialize
#------------
def initialize
super(194, 11, 285, 353)
self.contents = Bitmap.new(width - 32, height - 32)
self.back_opacity = 160
refresh
end
#---------
# Refresh
#---------
def refresh
self.contents.clear
self.contents.font.color = normal_color
self.contents.font.size = 16
q_data
end
#------------
# Quest Data
#------------
def q_data
#LINES MUST BE NO MORE THAN 30 CHARACTERS
self.contents.draw_text(0, 0, 284, 25, "Quest Log")
#No_Quest
if $game_variables[1] == 0
self.contents.draw_text(0, 50, 284, 25, "You do not have any quests in")
self.contents.draw_text(0, 75, 284, 25, "your Quest Log. To start a new")
self.contents.draw_text(0, 100, 284, 25, "quest speak to an NPC and see")
self.contents.draw_text(0, 125, 284, 25, "if they have any quests for")
self.contents.draw_text(0, 150, 284, 25, "you to complete.")
end
#Quest 1
if $game_variables[1] == 1
self.contents.draw_text(0, 50, 284, 25, "SEARCHING FOR ZARMIN FLARE")
self.contents.draw_text(0, 100, 284, 25, "Priest Ahrin of Tiben Town has")
self.contents.draw_text(0, 125, 284, 25, "asked you to find his friend Zarmin")
self.contents.draw_text(0, 150, 284, 25, "Flare. Search the whole town, he could")
self.contents.draw_text(0, 175, 284, 25, "be anywhere... Make sure you talk to")
self.contents.draw_text(0, 200, 284, 25, "any NPC's along the way as they may help.")
end
if $game_variables[1] == 2
self.contents.draw_text(0, 50, 284, 25, "DARK MAGIC")
self.contents.draw_text(0, 100, 284, 25, "Necromancer Zeni of Moorlake Valley")
self.contents.draw_text(0, 125, 284, 25, "has a task for you. Find the caves of")
self.contents.draw_text(0, 150, 284, 25, "Xanar the Dark, and defeat six of his")
self.contents.draw_text(0, 175, 284, 25, "Dark Minions.")
end
if $game_variables[1] == 3
#Insert commands for quest [3]
end
if $game_variables[1] == 4
#Insert commands for quest [4]
end
end
end
class Window_QuestRewards < Window_Base
#------------
# Initialize
#------------
def initialize
super(194, 260, 285, 104)
self.contents = Bitmap.new(width - 32, height - 32)
self.back_opacity = 160
self.windowskin = RPG::Cache.windowskin("ws2.png")
refresh
end
#---------
# Refresh
#---------
def refresh
self.contents.clear
self.contents.font.color = normal_color
self.contents.font.size = 16
q_rewards
end
#---------------
# Quest Rewards
#---------------
def q_rewards
self.contents.draw_text(0, 0, 284, 25, "Quest Rewards")
# No_Quest
if $game_variables[1] == 0
self.contents.draw_text(0, 22, 284, 25, "There are no rewards for this")
self.contents.draw_text(0, 44, 284, 25, "quest. You are not currently")
self.contents.draw_text(0, 66, 284, 25, "in a quest.")
end
if $game_variables[1] == 1
@bitmap = RPG::Cache.picture("prayer.png")
self.contents.blt(0, 21, @bitmap, Rect.new(0, 0, @bitmap.width, @bitmap.height), 160)
self.contents.draw_text(55, 35, 284, 25, "PRAYER: Priest class skill")
end
if $game_variables[1] == 2
@bitmap = RPG::Cache.icon("046-skill03.png")
self.contents.blt(0, 21, @bitmap, Rect.new(0, 0, @bitmap.width, @bitmap.height), 160)
self.contents.draw_text(55, 35, 284, 25, "DARKNESS: Dark Mage class skill")
end
end
end
Or useRaziel said:I think you can put it in a method and then call the method, for example in Scene_Map you could have
Code:def window_update @window1.update end
then using a call script:
Code:Scene_Map.new.window_update
$scene.window_update