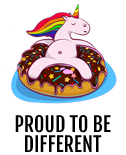
class Game_Map
Terrain_Tag_Sound_Effects = {}
# Don't Edit these lines
Terrain_Tag_Sound_Effects.default = {}
Terrain_Tag_Sound_Effects.default.default = nil
Terrain_Tag_Sound_Effects.values.each {|x| x.default = nil}
def terrain_tag_sound_effect(terrain_tag)
return Terrain_Tag_Sound_Effects[@map_id][terrain_tag]
end
end
class Game_Player
alias_method :seph_terraintileses_gmplyr_is, :increase_steps
def increase_steps
seph_terraintileses_gmplyr_is
if $game_map.terrain_tag_sound_effect(terrain_tag) != nil
n = $game_map.terrain_tag_sound_effect(terrain_tag)
$game_system.se_play(AudioFile.new(n))
end
end
end
Terrain_Tag_Sound_Effects[map_id] = {}
Terrain_Tag_Sound_Effects[map_id][terrain_tag] = 'SE Filename'
Terrain_Tag_Sound_Effects[3] = {}
Terrain_Tag_Sound_Effects[3][3] = 'Filename 1'
Terrain_Tag_Sound_Effects[3][5] = 'Filename 2'
class Game_Map
Terrain_Tag_Sound_Effects = {}
Terrain_Tag_Sound_Effects[@map_id] = {}
Terrain_Tag_Sound_Effects[@map_id][1] = '001-System01'
# Don't Edit these lines
Terrain_Tag_Sound_Effects.default = {}
Terrain_Tag_Sound_Effects.default.default = nil
Terrain_Tag_Sound_Effects.values.each {|x| x.default = nil}
def terrain_tag_sound_effect(terrain_tag)
return Terrain_Tag_Sound_Effects[@map_id][terrain_tag]
end
end
class Game_Player
alias_method :seph_terraintileses_gmplyr_is, :increase_steps
def increase_steps
seph_terraintileses_gmplyr_is
if $game_map.terrain_tag_sound_effect(terrain_tag) != nil
n = $game_map.terrain_tag_sound_effect(terrain_tag)
$game_system.se_play(AudioFile.new(n))
end
end
end
SephirothSpawn;315787 said:Try this:
Code:class Game_Map Terrain_Tag_Sound_Effects = {} # Don't Edit these lines Terrain_Tag_Sound_Effects.default = {} Terrain_Tag_Sound_Effects.default.default = nil Terrain_Tag_Sound_Effects.values.each {|x| x.default = nil} def terrain_tag_sound_effect(terrain_tag) return Terrain_Tag_Sound_Effects[@map_id][terrain_tag] end end class Game_Player alias_method :seph_terraintileses_gmplyr_is, :increase_steps def increase_steps seph_terraintileses_gmplyr_is if $game_map.terrain_tag_sound_effect(terrain_tag) != nil n = $game_map.terrain_tag_sound_effect(terrain_tag) $game_system.se_play(AudioFile.new(n)) end end end
Add lines below Terrain_Tag_Sound_Effects = {} like so:
Code:Terrain_Tag_Sound_Effects[map_id] = {} Terrain_Tag_Sound_Effects[map_id][terrain_tag] = 'SE Filename'
Start by adding the first line changing the map_id with the map id you want to change the terrain for.
Then add lines for each terrain you would like for your map with the second line.
For example:
Map 3: Terrain 3 - SE 'Filename 1', Terrain 5 - SE 'Filename 2'
Code:Terrain_Tag_Sound_Effects[3] = {} Terrain_Tag_Sound_Effects[3][3] = 'Filename 1' Terrain_Tag_Sound_Effects[3][5] = 'Filename 2'
Just let me know if that doesn't work or if you need any help.
class Game_Map
Terrain_Tag_Sound_Effects = {}
# Don't Edit these lines
Terrain_Tag_Sound_Effects.default = {}
Terrain_Tag_Sound_Effects.default.default = nil
Terrain_Tag_Sound_Effects.values.each {|x| x.default = nil}
def terrain_tag_sound_effect(terrain_tag)
return Terrain_Tag_Sound_Effects[@map_id][terrain_tag]
end
end
class Game_Player
alias_method :seph_terraintileses_gmplyr_is, :increase_steps
def increase_steps
seph_terraintileses_gmplyr_is
if $game_map.terrain_tag_sound_effect(terrain_tag) != nil
n = $game_map.terrain_tag_sound_effect(terrain_tag)
$game_system.se_play(AudioFile.new(*n))
end
end
end
Terrain_Tag_Sound_Effects[map_id][terrain_tag] = 'SE Filename'
# or
Terrain_Tag_Sound_Effects[map_id][terrain_tag] = 'SE Filename', volume
# or
Terrain_Tag_Sound_Effects[map_id][terrain_tag] = 'SE Filename', volume, pitch
SephirothSpawn;317732 said:Sure.
Code:class Game_Map Terrain_Tag_Sound_Effects = {} # Don't Edit these lines Terrain_Tag_Sound_Effects.default = {} Terrain_Tag_Sound_Effects.default.default = nil Terrain_Tag_Sound_Effects.values.each {|x| x.default = nil} def terrain_tag_sound_effect(terrain_tag) return Terrain_Tag_Sound_Effects[@map_id][terrain_tag] end end class Game_Player alias_method :seph_terraintileses_gmplyr_is, :increase_steps def increase_steps seph_terraintileses_gmplyr_is if $game_map.terrain_tag_sound_effect(terrain_tag) != nil n = $game_map.terrain_tag_sound_effect(terrain_tag) $game_system.se_play(AudioFile.new(*n)) end end end
Ok. So I only edited one line. Now, you can edit your constant like so:
Code:Terrain_Tag_Sound_Effects[map_id][terrain_tag] = 'SE Filename' # or Terrain_Tag_Sound_Effects[map_id][terrain_tag] = 'SE Filename', volume # or Terrain_Tag_Sound_Effects[map_id][terrain_tag] = 'SE Filename', volume, pitch
Let me know if you have any trouble.