by Atoa

Scripts RGSS, Resources, Tutorials and Translations by Atoa is licensed under a
Creative Commons Atribuição-Uso Não-Comercial-Compartilhamento pela mesma Licença 2.5 Brasil License.
Permissions beyond the scope of this license may be available at Santuário RPG Maker
Introduction
This script has the objective of storing text bitmap objects in cache.
By deafult, the RPG Maker draw each character individually from scratch, even if the same character is repeated frequently.
This make bitmap.draw_text really slow.
This script store the character bitmap on cache at the first it's drawn, and then use an clone of that bitmap if it's needed again
The main objective of this script is reduce the lag caused by windows that are updated constantly or windows with really long text (like an item window with 400 items xD)
The script has one drawback: if the text is bigger than the width set for the draw_text, it won't be streched to fit the smaller space.
Screenshots
This is an screen of an "HUD" with more than 300 character being refreshed every frame, withou the script, take a look at the FPS:
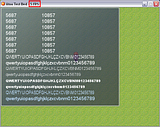
This is the same "HUD" refreshed every frame, the FBS is greatly rised:
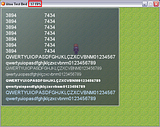
How to Use
Paste the code above main
You can clean the cache with an 'script call' and the code clear_text_cache
Script
Code:
#==============================================================================
# Text Cache
# by atoa
#==============================================================================
# This script has the objective of storing text bitmap objects in cache.
# By deafult, the RPG Maker draw each character individually from
# scratch, even if the same character is repeated frequently.
# This make bitmap.draw_text really slow.
#
# This script store the character bitmap on cache at the first it's drawn,
# and then use an clone of that bitmap if it's needed again
#
# The main objective of this script is reduce the lag caused by windows
# that are updated constantly or windows with really long text (like an
# item window with 400 items xD)
#
# The script has one drawback: if the text is bigger than the width set
# for the draw_text, it won't be streched to fit the smaller space.
#==============================================================================
#==============================================================================
# ■ Bitmap
#------------------------------------------------------------------------------
# Classe that handles bitmaps
#==============================================================================
class Bitmap
#------------------------------------------------------------------------------
# Draw text
# x : Text Coord X or text rect
# y : Text Coord y or text sting
# width : Text width or text align
# height : Text height
# str : Text string
# align : Text align
#------------------------------------------------------------------------------
alias text_cache_draw_text draw_text if !method_defined?(:text_cache_draw_text)
def draw_text(x, y, width = 0, height = 0, str = '', align = 0)
case x
when Numeric
adj = align == 0 ? 0 : align == 1 ? (width / 2) - (text_size(str).width / 2) : width - text_size(str).width
i = 0
for digit in str.to_s.split('')
bitmap = check_bitmap(digit)
blt(adj + x + i, y + 4, bitmap[0], bitmap[1])
i += bitmap[0].width - 2
end
when Rect
rect = x
str = y
align = width
adj = align == 0 ? 0 : align == 1 ? (rect.width / 2) - (text_size(str).width / 2) : rect.width - text_size(str).width
i = 0
for digit in str.to_s.split('')
bitmap = check_bitmap(digit)
blt(adj + rect.x + i, rect.y + 4, bitmap[0], bitmap[1])
i += bitmap[0].width - 2
end
end
end
#------------------------------------------------------------------------------
# Check if bitmap is areay in cache
# digit : digit to be drawn
#------------------------------------------------------------------------------
def check_bitmap(digit)
$text_cache ||= {}
color = [font.color.red, font.color.green, font.color.blue, font.color.alpha]
key = [digit, color, font.size, font.name]
if $text_cache[key].nil? or $text_cache[key][0].disposed?
width = text_size(digit).width + 2
height = text_size(digit).height + 2
b = Bitmap.new(width, height)
$text_cache[key] = [b, b.rect]
b.font.name = font.name
b.font.size = font.size
b.font.color.set(color[0], color[1], color[2], color[3])
b.text_cache_draw_text(0, 0, width, height, digit)
end
return $text_cache[key]
end
end
#==============================================================================
# ■ Object
#------------------------------------------------------------------------------
# All classes inherit methods from this class
#==============================================================================
class Object
#------------------------------------------------------------------------------
# clear text cache
#------------------------------------------------------------------------------
def clear_text_cache
$text_cache ||= {}
for key in $text_cache.key
$text_cache[key].dispose
end
$text_cache.clear
end
end
Credits and Thanks
- By Atoa