Authors: game_guy
Version: 1.34
Introduction
Okay well its a system that makes it so you can't use all of your skills at once while in battle. Instead you have to equip them. Now here's the catch. Every skill has its own "ap" so when its equipped it takes some of the actor's ap. That way you can't use all of your skills and it adds some uniqueness to the game.
Features
- Equip Skills Strategically
- Only Equip Limited Amount of Skills Using Ap
- Have Different Starting Ap for Each Actor
- Have Different Ap Gain for Each Actor per Level Up
- Have Different Ap for Each Skill
- Have an Exit Scene for When the Skill Equipment Closes
Screenshots
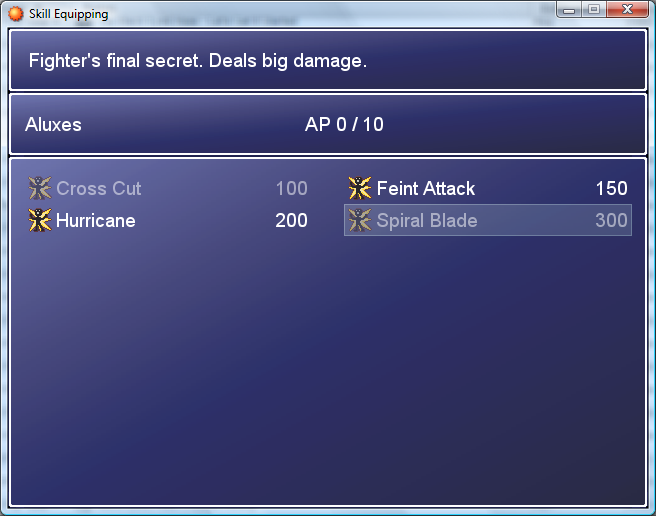
Demo
Updating Demo
Script
Code:
#===============================================================================
# Skill Equipment System
# Author game_guy
# Version 1.34
#-------------------------------------------------------------------------------
# Intro:
# Okay well its a system that makes it so you can't use all of your skills at
# once while in battle. Instead you have to equip them. Now here's the catch.
# Every skill has its own "ap" so when its equipped it takes some of the actor's
# ap. That way you can't use all of your skills and it adds some uniqueness to
# the game.
#
# Features:
# Equip Skills Strategically
# Only Equip Limited Amount of Skills Using Ap
# Have Different Starting Ap for Each Actor
# Have Different Ap Gain for Each Actor per Level Up
# Have Different Ap for Each Skill
# Have an Exit Scene for When the Skill Equipment Closes
#
# Instructions:
# Okay so these could be confusing but lets try and get through this.
# Okay they're not that confusing. Okay so go down where it says
# # Begin Config
# And do all of you're configuration there.
#
# Now theres some stuff you need to know.
#
# When adding a skill it does not automatically equip it. So to equip a
# skill use this
# $game_actors[actor_id].equip_skill(skill_id)
# To unequip a skill use this
# $game_actors[id].remove_skill(skill_id)
#
# Now it will not equip it unless there's enough ap left. So you can either do
# this $game_actors[id].add_map(amount) to add to max ap or you can do this
# $game_actors[id].clear_skills to unequip all skills and give all ap back.
# Just to make it a bit easier.
#
# You can always remove max ap to by doing this
# $game_actors[id].remove_map(amount) but note that doing that clears the
# equipped skills as well to avoid any problems.
#
# To open the skill equipment scene use this.
# $scene = $scene = Scene_SkillEquip.new(actor_id)
# To tell if a skill is equipped or not you'll notice the text is greyed out.
# That means it equipped. If its white it means it can be equipped.
# In the scene though to equip/unequip things just press the action button.
#
# Well thats about it.
# Credits:
# game_guy ~ for making it
# Ethan ~ for the idea of it
# Branden ~ beta testing
#
# Enjoy and give credits.
#===============================================================================
module GameGuy
#---------------------------------------------------------------------------
# ApWord = This is the word that displays when showing the ap the actor
# has.
#---------------------------------------------------------------------------
ApWord = "AP"
#---------------------------------------------------------------------------
# StartingAp = The starting ap for every actor. Different amounts can be
# defined below at # Config Starting Ap.
#---------------------------------------------------------------------------
StartingAp = 5
#---------------------------------------------------------------------------
# ApCost = The default ap cost for all skills. Different amounts can be
# defined below at # Config Ap Costs.
#---------------------------------------------------------------------------
ApCost = 5
#---------------------------------------------------------------------------
# ApGain = The max ap gained when an actor levels up. Different amounts
# can be defined below at # Config Sp Gain
#---------------------------------------------------------------------------
ApGain = 2
#---------------------------------------------------------------------------
# ExitScene = The scene it goes to when the Skill Equipment closes.
#
#---------------------------------------------------------------------------
ExitScene = Scene_Menu.new
def self.start_ap(id)
case id
#-------------------------------------------------------------------------
# Config Starting Ap
# Use this when configuring
# when actor_id then return starting_ap
#-------------------------------------------------------------------------
when 1 then return 10 # Actor 1 : Aluxes
when 2 then return 8 # Actor 2 : Basil
end
return StartingAp
end
def self.skill_ap(id)
case id
#-------------------------------------------------------------------------
# Config Ap Costs
# Use this when configuring
# when skill_id then return ap_cost
#-------------------------------------------------------------------------
when 1 then return 2 # Skill Id 1 : Heal
when 7 then return 3 # Skill Id 7 : Fire
end
return ApCost
end
def self.ap_gain(id)
case id
#-------------------------------------------------------------------------
# Config Ap gain
# Use this when configuring
# when actor_id then return ap_gain
#-------------------------------------------------------------------------
when 1 then return 4 # Actor 1 : Aluxes
end
return ApGain
end
end
#==============================================================================
# Game_Actor
#------------------------------------------------------------------------------
# Added stuff for Skill Equipping.
#==============================================================================
class Game_Actor
attr_accessor :eskills
attr_accessor :skills
attr_accessor :skillap
attr_accessor :skillmaxap
attr_accessor :eeskills
alias gg_add_stuff_lat_ap setup
def setup(actor_id)
@actor = $data_actors[actor_id]
@skillap = GameGuy.start_ap(actor_id)
@skillmaxap = GameGuy.start_ap(actor_id)
@eskills = []
@eeskills = []
return gg_add_stuff_lat_ap(actor_id)
end
def learn_skill(skill_id)
if skill_id > 0 and not skill_learn?(skill_id)
@eskills.push(skill_id)
@eskills.sort!
end
end
def forget_skill(skill_id)
@eskills.delete(skill_id)
end
def equip_skill(skill_id)
skill = $data_skills[skill_id]
if @skillap < GameGuy.skill_ap(skill.id)
return
end
@skillap -= GameGuy.skill_ap(skill.id)
if skill_id > 0 && @eskills.include?(skill_id)
@skills.push(skill_id)
@skills.sort!
@eeskills.push(skill_id)
end
end
def remove_skill(id)
@skillap += GameGuy.skill_ap(id)
@skills.delete(id)
@eeskills.delete(id)
end
def add_map(n)
@skillmaxap += n
clear_skills
@skillap = @skillmaxap
end
def remove_map(n)
@skillmaxap -= n
if @skillmaxap < 0
@skillmaxap = 0
end
if @skillap > @skillmaxap
@skillap = @skillmaxap
end
clear_skills
end
def exp=(exp)
@exp = [[exp, 9999999].min, 0].max
while @exp >= @exp_list[@level+1] and @exp_list[@level+1] > 0
@level += 1
@ap += GameGuy.ap_gain(@id)
for j in $data_classes[@class_id].learnings
if j.level == @level
learn_skill(j.skill_id)
end
end
end
while @exp < @exp_list[@level]
@level -= 1
end
@hp = [@hp, self.maxhp].min
@sp = [@sp, self.maxsp].min
end
def clear_skills
@skills = []
@eeskills = []
@ap = @maxap
end
end
#==============================================================================
# Window_GGAPSkill
#------------------------------------------------------------------------------
# Copy of Window_Skill but just slightly different.
#==============================================================================
class Window_GGAPSkill < Window_Selectable
def initialize(actor)
super(0, 128, 640, 352)
@actor = actor
@column_max = 2
refresh
self.index = 0
end
def skill
return @data[self.index]
end
def refresh
if self.contents != nil
self.contents.dispose
self.contents = nil
end
@data = []
for i in [email=0...@actor.eskills.size]0...@actor.eskills.size[/email]
skill = $data_skills[@actor.eskills[i]]
if skill != nil
@data.push(skill)
end
end
@item_max = @data.size
if @item_max > 0
self.contents = Bitmap.new(width - 32, row_max * 32)
for i in 0...@item_max
draw_item(i)
end
end
end
def draw_item(index)
skill = @data[index]
if @actor.skill_can_use?(skill.id)
self.contents.font.color = normal_color
else
self.contents.font.color = disabled_color
end
x = 4 + index % 2 * (288 + 32)
y = index / 2 * 32
rect = Rect.new(x, y, self.width / @column_max - 32, 32)
self.contents.fill_rect(rect, Color.new(0, 0, 0, 0))
bitmap = RPG::Cache.icon(skill.icon_name)
opacity = self.contents.font.color == normal_color ? 255 : 128
self.contents.blt(x, y + 4, bitmap, Rect.new(0, 0, 24, 24), opacity)
self.contents.draw_text(x + 28, y, 204, 32, skill.name, 0)
self.contents.draw_text(x + 232, y, 48, 32, skill.sp_cost.to_s, 2)
end
def update_help
@help_window.set_text(self.skill == nil ? "" : self.skill.description)
end
end
#==============================================================================
# Window_GGAPSkillEquip
#------------------------------------------------------------------------------
# Window uses for equipping skills.
#==============================================================================
class Window_GGAPSkillEquip < Window_Selectable
def initialize(actor)
super(0, 128, 640, 352)
@actor = actor
@column_max = 2
refresh
self.index = 0
end
def skill
return @data[self.index]
end
def refresh
if self.contents != nil
self.contents.dispose
self.contents = nil
end
@data = []
for i in [email=0...@actor.eskills.size]0...@actor.eskills.size[/email]
skill = $data_skills[@actor.eskills[i]]
if skill != nil
@data.push(skill)
end
end
@item_max = @data.size
if @item_max > 0
self.contents = Bitmap.new(width - 32, row_max * 32)
for i in 0...@item_max
draw_item(i)
end
end
end
def draw_item(index)
skill = @data[index]
if @actor.eeskills.include?(skill.id)
self.contents.font.color = normal_color
else
self.contents.font.color = disabled_color
end
x = 4 + index % 2 * (288 + 32)
y = index / 2 * 32
rect = Rect.new(x, y, self.width / @column_max - 32, 32)
self.contents.fill_rect(rect, Color.new(0, 0, 0, 0))
bitmap = RPG::Cache.icon(skill.icon_name)
opacity = self.contents.font.color == normal_color ? 255 : 128
self.contents.blt(x, y + 4, bitmap, Rect.new(0, 0, 24, 24), opacity)
self.contents.draw_text(x + 28, y, 204, 32, skill.name, 0)
self.contents.draw_text(x + 232, y, 48, 32, skill.sp_cost.to_s, 2)
end
def update_help
@help_window.set_text(self.skill == nil ? "" : self.skill.description)
end
end
#==============================================================================
# Window_GGActorAp
#------------------------------------------------------------------------------
# Window used to display AP and Actor name.
#==============================================================================
class Window_GGActorAp < Window_Base
def initialize(actor)
super(0,64,640,64)
self.contents = Bitmap.new(width-32,height-32)
@actor = $game_actors[actor]
refresh
end
def refresh
self.contents.clear
ap = GameGuy::ApWord
self.contents.draw_text(0, 0, 640, 32, "#{@actor.name}")
self.contents.draw_text(0, 0, 640, 32, "#{ap} #{@actor.skillap} / " +
"#{@actor.skillmaxap}", 2)
end
end
#==============================================================================
# Scene_Skill
#------------------------------------------------------------------------------
# Just slightly modded the main method.
#==============================================================================
#==============================================================================
# Scene_SkillEquip
#------------------------------------------------------------------------------
# The scene that deals with equipping skills.
#==============================================================================
class Scene_SkillEquip
def initialize(actor_id=1)
@id = actor_id
end
def main
@actor = $game_actors[@id]
@help_window = Window_Help.new
@skill_window = Window_GGAPSkillEquip.new(@actor)
@skill_window.help_window = @help_window
@status_window = Window_GGActorAp.new(@actor.id)
Graphics.transition
loop do
Graphics.update
Input.update
update
if $scene != self
break
end
end
@help_window.dispose
@skill_window.dispose
@status_window.dispose
end
def update
@help_window.update
@skill_window.update
@status_window.update
if @skill_window.active
update_skill
return
end
end
def update_skill
if Input.trigger?(Input::B)
$game_system.se_play($data_system.cancel_se)
$scene = GameGuy::ExitScene
return
end
if Input.trigger?(Input::C)
$game_system.se_play($data_system.decision_se)
skill = @skill_window.skill
if @actor.eeskills.include?(skill.id)
@actor.remove_skill(skill.id)
else
if @actor.skillap < GameGuy.skill_ap(skill.id)
$game_system.se_play($data_system.buzzer_se)
return
end
@actor.equip_skill(skill.id)
end
@status_window.refresh
@skill_window.refresh
return
end
end
end
Instructions
In the script.
Compatibility
Not tested with SDK.
Credits and Thanks
- game_guy ~ for making it
- Ethan (A Friend) ~ for making the idea of it
- Branden ~ beta testing
Author's Notes
Give credits and enjoy