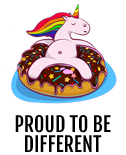
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize(width)
super(80, 336, 480, 32 * $game_temp.choice_text.size + 32 - 40) #336 #136 # 32 * $game_temp.choice_text.size + 32 - 40
self.contents = Bitmap.new(width - 32, height - 32)
self.contents.font.name = $fontface
self.contents.font.size = $fontsize
self.opacity = 0
self.z = 200
@item_max = $game_temp.choice_text.size
self.index = 0
@width = width
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
for i in 0...$game_temp.choice_text.size
x = 2
y = i * 32
self.contents.draw_text(x, y, @width, 32, $game_temp.choice_text[i])
end
end
#--------------------------------------------------------------------------
# * Update Cursor Rectangle
#--------------------------------------------------------------------------
def update_cursor_rect
if @index >= 0
if @width != nil
self.cursor_rect.set(0, @index * 32, @width - 43, 32)
end
else
self.cursor_rect.empty
end
end
end
alias :update_cursor_rect_original :update_cursor_rect
def update_cursor_rect
if @index >= 0
if @width != nil
update_cursor_rect_original
end
else
self.cursor_rect.empty
end
end
class Window_Choices < Window_Selectable
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize(width)
super(80, 336, 480, 160 - 32)
self.contents = Bitmap.new(width - 32, height - 32)
self.opacity = 0
self.z = 9999
@item_max = $game_temp.choice_text.size
self.index = 0
@width = width
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
for i in 0...$game_temp.choice_text.size
x = 8
y = i * 32
self.contents.draw_text(x, y, @width, 32, $game_temp.choice_text[i])
end
end
#--------------------------------------------------------------------------
# * Update Cursor Rectangle
#--------------------------------------------------------------------------
alias update_cursor_rect_original update_cursor_rect
def update_cursor_rect
if @index >= 0
if @width != nil
update_cursor_rect_original
self.cursor_rect.set(6, @index * 32, @width - 42, 32)
end
else
self.cursor_rect.empty
end
end
end