Drago del Fato
Member
RMVX - PointSpendSystem
Version: 1.05
By: Drago del Fato
Introduction
With this script you will increase the attributes by yourself inside the game. I've already made one for RMXP back in the day and I decided to make another one just for RMVX. You can set a limited number of points to be spent per each new level, and you can also customize it the way you want (set other text and stuff like that).
Features
Screenshots
Script
Instructions
Just place the script in the Materials, above Main.
FAQ
Q. I have a question... what do I do?
A. Post it.
Q. Can you decrease points to get stat?
A. Yes, but no more than original stats at that current level are.
Demo
VX Demo 351KB
Click Here to download
Compatibility
For now this is unknown. Please post feedback so I can fill this.
Author's Notes
There is a same version of this script made for RMXP but because RMXP scripts aren't compatible with RMVX scripts I made a completely new one just for RMVX. :P
Terms and Conditions
This script is FREE for personal and commercial use. If you use this script I only want my name in the credits, that's all.
Version: 1.05
By: Drago del Fato
Introduction
With this script you will increase the attributes by yourself inside the game. I've already made one for RMXP back in the day and I decided to make another one just for RMVX. You can set a limited number of points to be spent per each new level, and you can also customize it the way you want (set other text and stuff like that).
Features
- Diablo Style spending points per level.
- Customizeable
- Option to select wether will notify you until you have all the points spend or just until the next level.
Screenshots
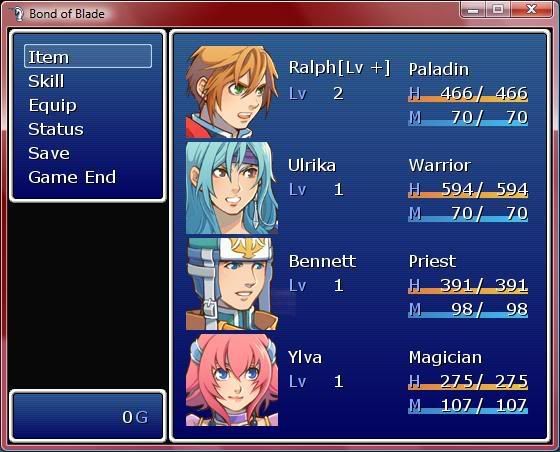
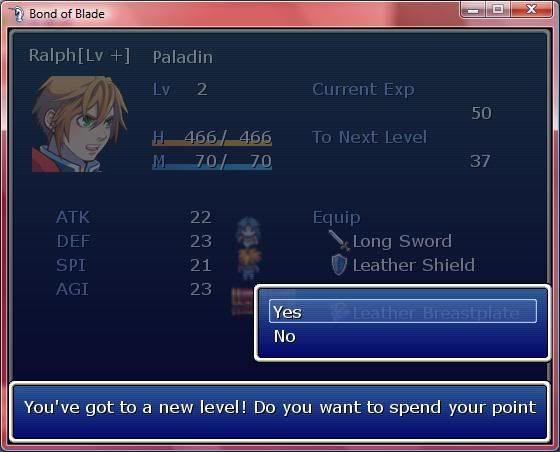
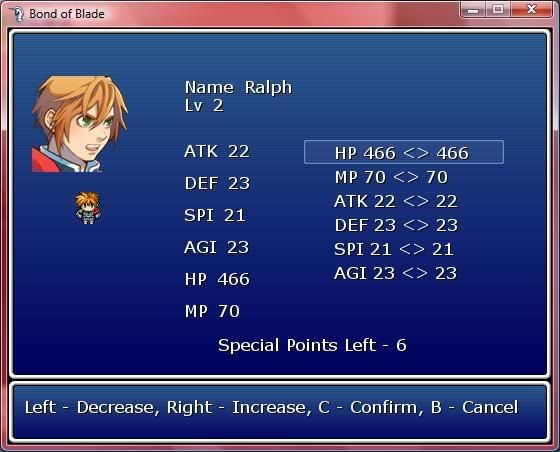
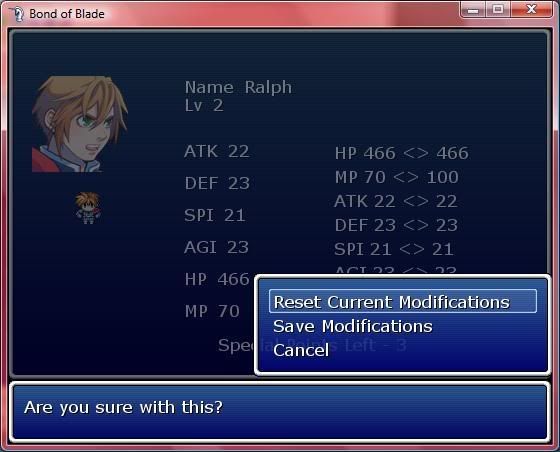
Script
Code:
#==============================================================================
# ** RMVX Drago del Fato - PointSpendSystem
# - Type: Custom Material
# - Author: Drago del Fato
# - Version: 1.05
# - RPG Maker Version: VX
# - Implementation: Put in Materials list.
#------------------------------------------------------------------------------
# When you gain a new level in your RPG your character attributes will need to
# be manually increased instead of automatically. You can set a specified number
# of points user can spend on each level as well as how much each attribute will
# be increased. If you use this script please give credits, thx ^^
#==============================================================================
#--------------------------------------------------------------------------
# * Phase 1 - Language and Other Resources
#--------------------------------------------------------------------------
#--- Main Text Resources
PSS_TEXT = "You've got to a new level! Do you want to spend your points now?"
PSS_ANSWERS = ["Yes","No"]
PSS_ACTOR_TEXT = "[Lv +]"
PSS_DESCRIPTION = "Left - Decrease, Right - Increase, C - Confirm, B - Cancel"
PSS_B1 = "Reset Current Modifications"
PSS_B2 = "Save Modifications"
PSS_B3 = "Cancel"
PSS_RES1 = "Special Points Left - "
PSS_RES2 = "Are you sure with this?"
PSS_SEP = " <> "
PSS_NAME = "Name"
#--- Other Resources
$PSS_POINTS = 6
PSS_HP = 10
PSS_MP = 10
PSS_ATK = 1
PSS_DEF = 2
PSS_SPI = 3
PSS_AGI = 4
#--- Set to True if you want to be notified until that character has points left
PSS_NOTIFY_POINTS = true
#--- Global Resources (do not modify if you arent skilled in RGSS)
$PSS_ACTOR_POINTS = []
$PSS_ACTOR_LVL_UP = []
$PSS_ACTOR_ATTR = [1,1,1,1,1,1]
$PSS_ACTOR_ADD = [1,1,1,1,1,1]
$PSS_RETURN_POINTS = 0
$PSS_POINTER = [PSS_HP, PSS_MP, PSS_ATK, PSS_DEF, PSS_SPI, PSS_AGI]
#--------------------------------------------------------------------------
# * Phase 2 - Class Modifications
#--------------------------------------------------------------------------
class Game_Actor < Game_Battler
#--------------------------------------------------------------------------
# * Change Experience
# exp : New experience
# show : Level up display flag
#--------------------------------------------------------------------------
def change_exp(exp, show)
last_level = @level
last_skills = skills
@exp = [[exp, 9999999].min, 0].max
while @exp >= @exp_list[@level+1] and @exp_list[@level+1] > 0
level_up
#-- Overwrite the new level parameters with past level one.
if !$BTEST
actor.parameters[0, @level] = actor.parameters[0, @level - 1]
actor.parameters[1, @level] = actor.parameters[1, @level - 1]
actor.parameters[2, @level] = actor.parameters[2, @level - 1]
actor.parameters[3, @level] = actor.parameters[3, @level - 1]
actor.parameters[4, @level] = actor.parameters[4, @level - 1]
actor.parameters[5, @level] = actor.parameters[5, @level - 1]
end
#-- Add Points to an actor when he levels up.
$PSS_ACTOR_LVL_UP[@actor_id] = true
$PSS_ACTOR_POINTS[@actor_id] += $PSS_POINTS
end
#-- Level down is deleted.
@hp = [@hp, maxhp].min
@mp = [@mp, maxmp].min
if show and @level > last_level
display_level_up(skills - last_skills)
end
end
def pss_change_actor_params(param_id, new_value)
actor.parameters[param_id, @level] = new_value
end
end
class Scene_Title < Scene_Base
#--------------------------------------------------------------------------
# * Create Game Objects
#--------------------------------------------------------------------------
def create_game_objects
$game_temp = Game_Temp.new
$game_message = Game_Message.new
$game_system = Game_System.new
$game_switches = Game_Switches.new
$game_variables = Game_Variables.new
$game_self_switches = Game_SelfSwitches.new
$game_actors = Game_Actors.new
$game_party = Game_Party.new
$game_troop = Game_Troop.new
$game_map = Game_Map.new
$game_player = Game_Player.new
for i in 0..$data_actors.size - 2
$PSS_ACTOR_POINTS.push(0)
$PSS_ACTOR_LVL_UP.push(false)
end
end
end
#-- Draw Level Up when a character goes to a new level, delete this class if
#-- you don't like this
class Window_Base < Window
def draw_actor_name(actor, x, y)
self.contents.font.color = hp_color(actor)
if $PSS_ACTOR_POINTS[actor.id] == 0
self.contents.draw_text(x, y, 108, WLH, actor.name)
else
ts = self.contents.text_size(actor.name + PSS_ACTOR_TEXT)
self.contents.draw_text(x, y, ts.width, ts.height, actor.name + PSS_ACTOR_TEXT)
end
end
end
#-- End of Level Up Character Name
class Scene_Status < Scene_Base
def start
#-- Original Status commands
super
create_menu_background
@actor = $game_party.members[@actor_index]
@status_window = Window_Status.new(@actor)
#-- End
if $PSS_ACTOR_LVL_UP[@actor_index + 1]
@pss_help = Window_PSS_Help.new
@pss_help.z = 9998
@pss_help.refresh_3
@status_window.opacity = 100
@status_window.contents_opacity = 100
@pss_confirm = Window_Command.new(300, [PSS_ANSWERS[0], PSS_ANSWERS[1]])
@pss_confirm.x = 245
@pss_confirm.y = 255
@pss_confirm.active = true
end
end
def update
if @pss_confirm != nil and @pss_confirm.active
@pss_confirm.update
if Input.trigger?(Input::C)
case @pss_confirm.index
when 0
@pss_confirm.visible = false
@pss_help.visible = false
$scene = Scene_PSS.new(@actor_index + 1)
when 1
@pss_confirm.visible = false
@pss_help.visible = false
@status_window.opacity = 160
@status_window.contents_opacity = 255
end
elsif Input.trigger?(Input::B)
@pss_confirm.visible = false
@pss_help.visible = false
@status_window.opacity = 160
@status_window.contents_opacity = 255
end
end
#-- Original Status commands
update_menu_background
@status_window.update
if Input.trigger?(Input::B)
Sound.play_cancel
return_scene
elsif Input.trigger?(Input::R)
Sound.play_cursor
next_actor
elsif Input.trigger?(Input::L)
Sound.play_cursor
prev_actor
end
super
#-- End
end
def terminate
#-- Original Status Commands
super
dispose_menu_background
@status_window.dispose
#-- End
@pss_confirm.dispose if @pss_confirm != nil
@pss_help.dispose if @pss_help != nil
end
end
#--------------------------------------------------------------------------
# * Phase 3 - PointSpendSystem Scene
#--------------------------------------------------------------------------
class Window_PSS_Help < Window_Base
def initialize
super(0,352,544,64)
refresh
end
def refresh
self.contents.clear
ts = self.contents.text_size(PSS_DESCRIPTION)
self.contents.draw_text(0,0,ts.width,ts.height, PSS_DESCRIPTION)
end
def refresh_2
self.contents.clear
ts = self.contents.text_size(PSS_RES2)
self.contents.draw_text(0,0,ts.width,ts.height, PSS_RES2)
end
def refresh_3
self.contents.clear
ts = self.contents.text_size(PSS_TEXT)
self.contents.draw_text(0,0,ts.width,ts.height, PSS_TEXT)
end
end
class Window_PSS_Main < Window_Selectable
def initialize(actor)
super(0, 0, 544, 352)
@actor = actor
refresh
self.index = 0
end
def refresh
self.contents.clear
#-- Draw Text Data
ts = self.contents.text_size(PSS_NAME)
self.contents.draw_text(160,32,ts.width,ts.height, PSS_NAME)
ts_a = self.contents.text_size(@actor.name)
self.contents.draw_text(170 + ts.width,32,ts_a.width,ts_a.height, @actor.name)
ts = self.contents.text_size(Vocab.level_a)
self.contents.draw_text(160,50,ts.width,ts.height,Vocab.level_a)
ts_a = self.contents.text_size(@actor.level)
self.contents.draw_text(170 + ts.width,50,ts_a.width,ts_a.height, @actor.level)
ts = self.contents.text_size(Vocab.atk)
self.contents.draw_text(160,96,ts.width,ts.height,Vocab.atk)
ts_a = self.contents.text_size(@actor.base_atk)
self.contents.draw_text(170 + ts.width,96,ts_a.width,ts_a.height, @actor.base_atk)
ts = self.contents.text_size(Vocab.def)
self.contents.draw_text(160,128,ts.width,ts.height,Vocab.def)
ts_a = self.contents.text_size(@actor.base_def)
self.contents.draw_text(170 + ts.width,128,ts_a.width,ts_a.height, @actor.base_def)
ts = self.contents.text_size(Vocab.spi)
self.contents.draw_text(160,160,ts.width,ts.height,Vocab.spi)
ts_a = self.contents.text_size(@actor.base_spi)
self.contents.draw_text(170 + ts.width,160,ts_a.width,ts_a.height, @actor.base_spi)
ts = self.contents.text_size(Vocab.agi)
self.contents.draw_text(160,192,ts.width,ts.height,Vocab.agi)
ts_a = self.contents.text_size(@actor.base_agi)
self.contents.draw_text(170 + ts.width,192,ts_a.width,ts_a.height, @actor.base_agi)
ts = self.contents.text_size(Vocab.hp)
self.contents.draw_text(160,224,ts.width,ts.height,Vocab.hp)
ts_a = self.contents.text_size(@actor.base_maxhp)
self.contents.draw_text(170 + ts.width,224,ts_a.width,ts_a.height, @actor.base_maxhp)
ts = self.contents.text_size(Vocab.mp)
self.contents.draw_text(160,256,ts.width,ts.height,Vocab.mp)
ts_a = self.contents.text_size(@actor.base_maxmp)
self.contents.draw_text(170 + ts.width,256,ts_a.width,ts_a.height, @actor.base_maxmp)
p_text = PSS_RES1 + $PSS_ACTOR_POINTS[@actor.id].to_s
ts_a = self.contents.text_size(p_text)
self.contents.draw_text(170 + ts.width,290,ts_a.width,ts_a.height, p_text)
#-- Draw Face
draw_actor_face(@actor, 8, 32)
draw_actor_graphic(@actor, 64, 180)
s1 = Vocab.hp + " " + $PSS_ACTOR_ATTR[0].to_s + PSS_SEP + $PSS_ACTOR_ADD[0].to_s
s2 = Vocab.mp + " " + $PSS_ACTOR_ATTR[1].to_s + PSS_SEP + $PSS_ACTOR_ADD[1].to_s
s3 = Vocab.atk + " " + $PSS_ACTOR_ATTR[2].to_s + PSS_SEP + $PSS_ACTOR_ADD[2].to_s
s4 = Vocab.def + " " + $PSS_ACTOR_ATTR[3].to_s + PSS_SEP + $PSS_ACTOR_ADD[3].to_s
s5 = Vocab.spi + " " + $PSS_ACTOR_ATTR[4].to_s + PSS_SEP + $PSS_ACTOR_ADD[4].to_s
s6 = Vocab.agi + " " + $PSS_ACTOR_ATTR[5].to_s + PSS_SEP + $PSS_ACTOR_ADD[5].to_s
@commands = [s1,s2,s3,s4,s5,s6]
for i in 0...6
draw_item(i)
end
@item_max = 6
@column_max = 1
end
def item_rect(index)
rect = Rect.new(0, 0, 0, 0)
rect.width = 200
rect.height = WLH
rect.x = 280 + (index % @column_max * (rect.width + @spacing))
rect.y = 96 + (index / @column_max * WLH)
return rect
end
def draw_item(index, enabled = true)
rect = item_rect(index)
rect.x += 30
self.contents.clear_rect(rect)
self.contents.font.color = normal_color
self.contents.font.color.alpha = enabled ? 255 : 128
self.contents.draw_text(rect, @commands[index])
end
def update
super
end
end
class Scene_PSS < Scene_Base
def initialize(actor_id)
@actor = $game_actors[actor_id]
end
def start
get_attributes
@pss_main = Window_PSS_Main.new(@actor)
@pss_help = Window_PSS_Help.new
@pss_confirm = Window_Command.new(300, [PSS_B1, PSS_B2, PSS_B3])
@pss_confirm.x = 245
@pss_confirm.y = 245
@pss_confirm.visible = false
end
def set_attributes
@actor.pss_change_actor_params(0,$PSS_ACTOR_ADD[0])
@actor.pss_change_actor_params(1,$PSS_ACTOR_ADD[1])
@actor.pss_change_actor_params(2,$PSS_ACTOR_ADD[2])
@actor.pss_change_actor_params(3,$PSS_ACTOR_ADD[3])
@actor.pss_change_actor_params(4,$PSS_ACTOR_ADD[4])
@actor.pss_change_actor_params(5,$PSS_ACTOR_ADD[5])
end
def get_attributes
$PSS_ACTOR_ATTR[0] = @actor.maxhp
$PSS_ACTOR_ATTR[1] = @actor.maxmp
$PSS_ACTOR_ATTR[2] = @actor.base_atk
$PSS_ACTOR_ATTR[3] = @actor.base_def
$PSS_ACTOR_ATTR[4] = @actor.base_spi
$PSS_ACTOR_ATTR[5] = @actor.base_agi
for i in 0..$PSS_ACTOR_ADD.size - 1
$PSS_ACTOR_ADD[i] = $PSS_ACTOR_ATTR[i]
end
$PSS_RETURN_POINTS = $PSS_ACTOR_POINTS[@actor.id]
end
def subtract_points
$PSS_ACTOR_ADD[@pss_main.index] -= $PSS_POINTER[@pss_main.index]
#-- When subtracted, add points back
$PSS_ACTOR_POINTS[@actor.id] += 1
#-- No subtraction if values is less than original
if $PSS_ACTOR_ADD[@pss_main.index] < $PSS_ACTOR_ATTR[@pss_main.index]
$PSS_ACTOR_ADD[@pss_main.index] = $PSS_ACTOR_ATTR[@pss_main.index]
$PSS_ACTOR_POINTS[@actor.id] -= 1
end
end
def add_points
if $PSS_ACTOR_POINTS[@actor.id] > 0
$PSS_ACTOR_ADD[@pss_main.index] += $PSS_POINTER[@pss_main.index]
$PSS_ACTOR_POINTS[@actor.id] -= 1
end
end
def update
@pss_main.update if @pss_main.active
@pss_confirm.update if @pss_confirm.active
if Input.trigger?(Input::LEFT)
if @pss_main.active
subtract_points
@pss_main.refresh
end
elsif Input.trigger?(Input::RIGHT)
if @pss_main.active
add_points
@pss_main.refresh
end
elsif Input.trigger?(Input::C)
if @pss_main.active
@pss_main.opacity = 100
@pss_main.contents_opacity = 100
@pss_help.refresh_2
@pss_confirm.visible = true
@pss_confirm.active = true
@pss_main.active = false
elsif @pss_confirm.active
case @pss_confirm.index
when 0
for i in 0..$PSS_ACTOR_ADD.size - 1
$PSS_ACTOR_ADD[i] = $PSS_ACTOR_ATTR[i]
end
$PSS_ACTOR_POINTS[@actor.id] = $PSS_RETURN_POINTS
@pss_main.refresh
pss_return_back
when 1
set_attributes
$PSS_ACTOR_LVL_UP[@actor.id] = false
if PSS_NOTIFY_POINTS
$PSS_ACTOR_LVL_UP[@actor.id] = true if $PSS_ACTOR_POINTS[@actor.id] > 0
end
$scene = Scene_Map.new
when 2
pss_return_back
end
end
elsif Input.trigger?(Input::B)
if @pss_main.active
$scene = Scene_Map.new
elsif @pss_confirm.active
pss_return_back
end
end
end
def pss_return_back
@pss_main.opacity = 255
@pss_main.contents_opacity = 255
@pss_help.refresh
@pss_confirm.visible = false
@pss_confirm.active = false
@pss_main.active = true
end
def pre_terminate
@pss_main.dispose
@pss_help.dispose
@pss_confirm.dispose
end
end
Instructions
Just place the script in the Materials, above Main.
FAQ
Q. I have a question... what do I do?
A. Post it.
Q. Can you decrease points to get stat?
A. Yes, but no more than original stats at that current level are.
Demo
VX Demo 351KB
Click Here to download
Compatibility
For now this is unknown. Please post feedback so I can fill this.
Author's Notes
There is a same version of this script made for RMXP but because RMXP scripts aren't compatible with RMVX scripts I made a completely new one just for RMVX. :P
Terms and Conditions
This script is FREE for personal and commercial use. If you use this script I only want my name in the credits, that's all.