Emerald Lance
Member
Hi, this is my first post here and I hope I'm writing in the correct forum. My problem isn't exactly an error message, but rather an effect that should be coming from the script I'm using and isn't. I'm using Minkoff's Animated Battlers script. Everything works alright except for two things.
The enemy is in the middle (like how it is in the default BS) but I want it to mirror the player's actor position. It isn't simply the graphic thats displaced, but the entire enemy signature, in that the arrow is in the middle as well. Also, the sprite isn't being horizontally flipped to face the player actors despite "@mirror_enemies" being set to "true". I can hardly understand RGSS or the process of scripting, so I have no clue on how to fix these two problems myself. Also, I haven't been able to find the Minkoff script at all for a while, and ended up finding it in a youtube video; if I had to guess what the problem is, I would guess that the script was edited in some way (the video itself also had the enemy in the middle, but the image was reflected properly) and since I don't have the original source, I can't distinguish the edited parts. I could be wrong, but I thinks thats it.
If somebody could point me in the right direction then it would be greatly appreciated! Here is a copy of the script I'm using:
I appreciate the help, and again, thanks.
EDIT: I'm sorry, i fixed the positioning error, it was actually an error on my part: I didn't properly position the enemy in the "Troops" section, my bad. But there is still the problem with the direction the enemy is facing which is still technically a positioning error. I don't want to manually position the enemies because I intend to use many of them as allies, so if there is a way to fix this problem in the scripting then that would be wonderful.
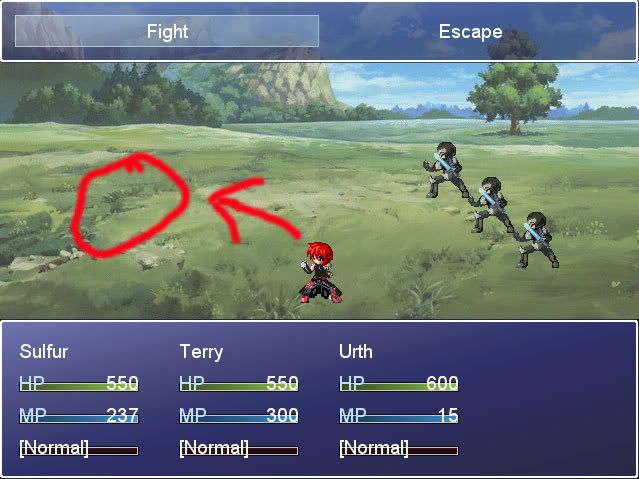
The enemy is in the middle (like how it is in the default BS) but I want it to mirror the player's actor position. It isn't simply the graphic thats displaced, but the entire enemy signature, in that the arrow is in the middle as well. Also, the sprite isn't being horizontally flipped to face the player actors despite "@mirror_enemies" being set to "true". I can hardly understand RGSS or the process of scripting, so I have no clue on how to fix these two problems myself. Also, I haven't been able to find the Minkoff script at all for a while, and ended up finding it in a youtube video; if I had to guess what the problem is, I would guess that the script was edited in some way (the video itself also had the enemy in the middle, but the image was reflected properly) and since I don't have the original source, I can't distinguish the edited parts. I could be wrong, but I thinks thats it.
If somebody could point me in the right direction then it would be greatly appreciated! Here is a copy of the script I'm using:
Code:
#==============================================================================
# ** Sprite_Battler
#------------------------------------------------------------------------------
# Â Animated Battlers by Minkoff, Updated by DerVVulfman
#==============================================================================
Â
class Sprite_Battler < RPG::Sprite
 #--------------------------------------------------------------------------
 # * Initialize
 #--------------------------------------------------------------------------
 alias cbs_initialize initialize
 def initialize(viewport, battler = nil)
Â
  # Configuration
  @speed        = 4    # Framerate speed of the battlers
  @frames       = 4    # Number of frames in each pose
  @poses        = 11   # Number of poses (stances) in the template
  @mirror_enemies   = true  # Enemy battlers use reversed image
  @stationary_enemies = false  # If the enemies don't move while attacking
  @stationary_actors  = false  # If the actors don't move while attacking
  @calculate_speed   = true  # System calculates a mean/average speed
  @phasing       = false  # Characters fade in/out while attacking
  @default_collapse  = false  # Restores the old 'red fade' effect to enemies
  # Array that holds the id # of weapons that forces the hero to be stationary
  @stationary_weapons = [] # (examples are bows & guns)
Â
  # DO NOT EDIT BELOW THIS LINE UNLESS YOU KNOW WHAT YOU'RE DOING
  @frame, @pose = 0, 0
  @last_time = 0
  @last_move_time = 0
  cbs_initialize(viewport, battler)
# Â Â self.mirror = !!battler and @mirror_enemies
  viewport.z = 99
 end
 #--------------------------------------------------------------------------
 # * Update
 #--------------------------------------------------------------------------
 alias cbs_update update
 def update
  return unless @battler
Â
  # Regular Update
  cbs_update
Â
  # Start Routine
  unless @started
   @pose = state
   @width = @width / @frames
   @height = @height / @poses
   @display_x = @battler.screen_x
   @display_y = @battler.screen_y
   @destination_x = @display_x
   @destination_y = @display_y
   @started = true
  end
Â
  # Cut Out Frame
  self.src_rect.set(@width * @frame, @height * @pose, @width, @height)
Â
  # Position Sprite
  self.x = @display_x
  self.y = @display_y
  self.z = @display_y
  self.ox = @width / 2
  self.oy = @height
Â
  # Setup Animation
  time = Graphics.frame_count / (Graphics.frame_rate / @speed)
  if @last_time < time
   @frame = (@frame + 1) % @frames
   if @frame == 0
    if @freeze
     @frame = @frames - 1
     return
    end
    @pose = state
   end
  end
  @last_time = time
Â
  # Move It
  move if moving
 end
 #--------------------------------------------------------------------------
 # * Current State
 #--------------------------------------------------------------------------
 def state
  # Damage State
  if [nil,{}].include?(@battler.damage)
   # Battler Fine
   @state = 0
   # Battler Wounded
   @state = 2 if @battler.hp < @battler.maxhp / 4
Â
   if @default_collapse
    # Battler Dead (Red-Out Collapse)
    if @battler.dead? and @battler.is_a?(Game_Actor)
     @state = 10
     # Fix Opacity
     self.opacity = 255
    end
   else
    # Battler Dead (Pose-Type Collapse)
    if @battler.dead?
     @state = 10
     # Fix Opacity
     self.opacity = 255
    end
   end
  end
  # Guarding State
  @state = 3 if @battler.guarding?
  # Moving State
  if moving
   # If enemy battler moving
   if @battler.is_a?(Game_Enemy)
    # Battler Moving Left
    @state = 5 if moving.eql?(0)
    # Battler Moving Right
    @state = 4 if moving.eql?(1)
   # Else actor battler moving
   else
    # Battler Moving Left
    @state = 4 if moving.eql?(0)
    # Battler Moving Right
    @state = 5 if moving.eql?(1)
   end
  end
  # Return State
  return @state
 end
 #--------------------------------------------------------------------------
 # * Move
 #--------------------------------------------------------------------------
 def move
  time = Graphics.frame_count / (Graphics.frame_rate.to_f / (@speed * 5))
  if @last_move_time < time
Â
   # Pause for Animation
   return if @pose != state
Â
   # Phasing
   if @phasing
    d1 = (@display_x - @original_x).abs
    d2 = (@display_y - @original_y).abs
    d3 = (@display_x - @destination_x).abs
    d4 = (@display_y - @destination_y).abs
    self.opacity = [255 - ([d1 + d2, d3 + d4].min * 1.75).to_i, 0].max
   end
Â
   # Calculate Difference
   difference_x = (@display_x - @destination_x).abs
   difference_y = (@display_y - @destination_y).abs
Â
   # Done? Reset, Stop
   if [difference_x, difference_y].max.between?(0, 8)
    @display_x = @destination_x
    @display_y = @destination_y
    @pose = state
    return
   end
Â
   # Calculate Movement Increments
   increment_x = increment_y = 1
   if difference_x < difference_y
    increment_x = 1.0 / (difference_y.to_f / difference_x)
   elsif difference_y < difference_x
    increment_y = 1.0 / (difference_x.to_f / difference_y)
   end
  Â
   # Calculate Movement Speed
   if @calculate_speed
    total = 0; $game_party.actors.each{ |actor| total += actor.agi }
    speed = @battler.agi.to_f / (total / $game_party.actors.size)
    increment_x *= speed
    increment_y *= speed
   end
  Â
   # Multiply and Move
   multiplier_x = (@destination_x - @display_x > 0 ? 8 : -8)
   multiplier_y = (@destination_y - @display_y > 0 ? 8 : -8)
   @display_x += (increment_x * multiplier_x).to_i
   @display_y += (increment_y * multiplier_y).to_i
  end
  @last_move_time = time
 end
 #--------------------------------------------------------------------------
 # * Set Movement
 #--------------------------------------------------------------------------
 def setmove(destination_x, destination_y)
  unless (@battler.is_a?(Game_Enemy) and @stationary_enemies) or
      (@battler.is_a?(Game_Actor) and @stationary_actors)
   unless @stationary_weapons.include?(@battler.weapon_id)
    @original_x = @display_x
    @original_y = @display_y
    @destination_x = destination_x
    @destination_y = destination_y
   end
  end
 end
 #--------------------------------------------------------------------------
 # * Movement Check
 #--------------------------------------------------------------------------
 def moving
  if (@display_x != @destination_x and @display_y != @destination_y and !@battler.dead?)
   return (@display_x > @destination_x ? 0 : 1)
  end
 end
 #--------------------------------------------------------------------------
 # * Set Pose
 #--------------------------------------------------------------------------
 def pose=(pose)
  @pose = pose
  @frame = 0
 end
 #--------------------------------------------------------------------------
 # * Freeze
 #--------------------------------------------------------------------------
 def freeze
  @freeze = true
 end
 #--------------------------------------------------------------------------
 # * Fallen Pose
 #--------------------------------------------------------------------------
 alias cbs_collapse collapse
 def collapse
  if @default_collapse
   cbs_collapse if @battler.is_a?(Game_Enemy)
   end
  end
 end
Â
#==============================================================================
# ** Game_Actor
#==============================================================================
Â
class Game_Actor
 #--------------------------------------------------------------------------
 # * Actor X Coordinate
 #--------------------------------------------------------------------------
 def screen_x
  if self.index != nil
     return self.index * 45 + 450
  else
   return 0
  end
 end
 #--------------------------------------------------------------------------
 # * Actor Y Coordinate
 #--------------------------------------------------------------------------
 def screen_y
  return self.index * 35 + 200
 end
 #--------------------------------------------------------------------------
 # * Actor Z Coordinate
 #--------------------------------------------------------------------------
 def screen_z
  return screen_y
 end
end
Â
#==============================================================================
# ** Scene_Battle
#==============================================================================
Â
class Scene_Battle
 #--------------------------------------------------------------------------
 # * Action Animation, Movement
 #--------------------------------------------------------------------------
 alias cbs_update_phase4_step3 update_phase4_step3
 def update_phase4_step3(battler = @active_battler)
  @rtab = !@target_battlers
  target = (@rtab ? battler.target : @target_battlers)[0]
  @moved = {} unless @moved
  return if @spriteset.battler(battler).moving
  case battler.current_action.kind
  when 0 # Attack
   if not (@moved[battler] or battler.guarding?)
    offset = (battler.is_a?(Game_Actor) ? 40 : -40)
    @spriteset.battler(battler).setmove(target.screen_x + offset, target.screen_y)
    @moved[battler] = true
    return
   elsif not battler.guarding?
    @spriteset.battler(battler).pose = 6 + rand(2)
    @spriteset.battler(battler).setmove(battler.screen_x, battler.screen_y)
   end
  when 1 # Skill
   @spriteset.battler(battler).pose = 8
  when 2 # Item
   @spriteset.battler(battler).pose = 8
  end
  @moved[battler] = false
  @rtab ? cbs_update_phase4_step3(battler) : cbs_update_phase4_step3
 end
 #--------------------------------------------------------------------------
 # * Hit Animation
 #--------------------------------------------------------------------------
 alias cbs_update_phase4_step4 update_phase4_step4
 def update_phase4_step4(battler = @active_battler)
  for target in (@rtab ? battler.target : @target_battlers)
   damage = (@rtab ? target.damage[battler] : target.damage)
   if damage.is_a?(Numeric) and damage > 0
    @spriteset.battler(target).pose = 1
   end
  end
  @rtab ? cbs_update_phase4_step4(battler) : cbs_update_phase4_step4
 end
 #--------------------------------------------------------------------------
 # * Victory Animation
 #--------------------------------------------------------------------------
 alias cbs_start_phase5 start_phase5
 def start_phase5
  for actor in $game_party.actors
   return if @spriteset.battler(actor).moving
  end
  for actor in $game_party.actors
   unless actor.dead?
    @spriteset.battler(actor).pose = 9
    @spriteset.battler(actor).freeze
   end
  end
  cbs_start_phase5
 end
 #--------------------------------------------------------------------------
 # * Change Arrow Viewport
 #--------------------------------------------------------------------------
 alias cbs_start_enemy_select start_enemy_select
 def start_enemy_select
  cbs_start_enemy_select
  @enemy_arrow.dispose
  @enemy_arrow = Arrow_Enemy.new(@spriteset.viewport2)
  @enemy_arrow.help_window = @help_window
 end
end
Â
#==============================================================================
# ** Spriteset_Battle
#==============================================================================
Â
class Spriteset_Battle
 #--------------------------------------------------------------------------
 # * Change Enemy Viewport
 #--------------------------------------------------------------------------
 alias cbs_initialize initialize
 def initialize
  cbs_initialize
  @enemy_sprites = []
  for enemy in $game_troop.enemies.reverse
   @enemy_sprites.push(Sprite_Battler.new(@viewport2, enemy))
  end
 end
 #--------------------------------------------------------------------------
 # * Find Sprite From Battler Handle
 #--------------------------------------------------------------------------
 def battler(handle)
  for sprite in @actor_sprites + @enemy_sprites
   return sprite if sprite.battler == handle
  end
 end
end
Â
#==============================================================================
# ** Arrow_Base
#==============================================================================
Â
class Arrow_Base < Sprite
 #--------------------------------------------------------------------------
 # * Reposition Arrows
 #--------------------------------------------------------------------------
 alias cbs_initialize initialize
 def initialize(viewport)
  cbs_initialize(viewport)
  self.ox = 14
  self.oy = 10
 end
end
EDIT: I'm sorry, i fixed the positioning error, it was actually an error on my part: I didn't properly position the enemy in the "Troops" section, my bad. But there is still the problem with the direction the enemy is facing which is still technically a positioning error. I don't want to manually position the enemies because I intend to use many of them as allies, so if there is a way to fix this problem in the scripting then that would be wonderful.