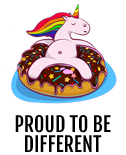
#==============================================================================
# ** Game_Party
#------------------------------------------------------------------------------
# This class handles the party. It includes information on amount of gold
# and items. Refer to "$game_party" for the instance of this class.
#==============================================================================
class Game_Party
#--------------------------------------------------------------------------
# * Constant Variables
#--------------------------------------------------------------------------
MAX_ITEMS = 255
MAX_ARMORS = 255
MAX_WEAPONS = 255
#--------------------------------------------------------------------------
# * Gain Items (or lose)
# item_id : item ID
# n : quantity
#--------------------------------------------------------------------------
def gain_item(item_id, n)
# Update quantity data in the hash.
if item_id > 0
@items[item_id] = [[item_number(item_id) + n, 0].max, MAX_ITEMS].min
end
end
#--------------------------------------------------------------------------
# * Gain Weapons (or lose)
# weapon_id : weapon ID
# n : quantity
#--------------------------------------------------------------------------
def gain_weapon(weapon_id, n)
# Update quantity data in the hash.
if weapon_id > 0
@weapons[weapon_id] = [[weapon_number(weapon_id) + n, 0].max, MAX_ARMORS].min
end
end
#--------------------------------------------------------------------------
# * Gain Armor (or lose)
# armor_id : armor ID
# n : quantity
#--------------------------------------------------------------------------
def gain_armor(armor_id, n)
# Update quantity data in the hash.
if armor_id > 0
@armors[armor_id] = [[armor_number(armor_id) + n, 0].max, MAX_WEAPONS].min
end
end
end
#==============================================================================
# ** Game_Party
#------------------------------------------------------------------------------
# This class handles the party. It includes information on amount of gold
# and items. The instance of this class is referenced by $game_party.
#==============================================================================
class Game_Party < Game_Unit
#--------------------------------------------------------------------------
# * Constant Variables
#--------------------------------------------------------------------------
MAX_ITEMS = 255
MAX_ARMORS = 255
MAX_WEAPONS = 255
#--------------------------------------------------------------------------
# * Gain Items (or lose)
# item : Item
# n : Number
# include_equip : Include equipped items
#--------------------------------------------------------------------------
def gain_item(item, n, include_equip = false)
number = item_number(item)
case item
when RPG::Item
@items[item.id] = [[number + n, 0].max, MAX_ITEMS].min
when RPG::Weapon
@weapons[item.id] = [[number + n, 0].max, MAX_WEAPONS].min
when RPG::Armor
@armors[item.id] = [[number + n, 0].max, MAX_ARMORS].min
end
n += number
if include_equip and n < 0
for actor in members
while n < 0 and actor.equips.include?(item)
actor.discard_equip(item)
n += 1
end
end
end
end
end
#==============================================================================
# ** Inventory_Limits
#------------------------------------------------------------------------------
# This class holds the values that dictate the maximum values of that the
# inventory can hold.
#==============================================================================
module Inventory_Limits
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
@max_items = 99
@max_armors = 99
@max_weapons = 99
#--------------------------------------------------------------------------
# * Max Items =
# max_items : the new maximum amount of items you can have.
#--------------------------------------------------------------------------
def self.max_items=(max_items)
return unless max_items.is_a?(Integer) || max_items < 0
@max_items = max_items
return if $game_party.nil?
$game_party.get_items.each do |id, amount|
$game_party.change_item(id, [amount, @max_items].min)
end
end
#--------------------------------------------------------------------------
# * Max Items
#--------------------------------------------------------------------------
def self.max_items
return @max_items
end
#--------------------------------------------------------------------------
# * Max Weapons =
# max_weapons : the new maximum amount of weapons you can have.
#--------------------------------------------------------------------------
def self.max_weapons=(max_weapons)
return unless max_weapons.is_a?(Integer) || max_weapons < 0
@max_weapons = max_weapons
return if $game_party.nil?
$game_party.weapons.each do |id, amount|
$game_party.weapons[id] = [amount, @max_weapons].min
end
end
#--------------------------------------------------------------------------
# * Max Weapons
#--------------------------------------------------------------------------
def self.max_weapons
return @max_weapons
end
#--------------------------------------------------------------------------
# * Max Armors =
# max_items : the new maximum amount of armors you can have.
#--------------------------------------------------------------------------
def self.max_armors=(max_armors)
return unless max_armors.is_a?(Integer) || max_armors < 0
@max_armors = max_armors
return if $game_party.nil?
$game_party.armors.each do |id, amount|
$game_party.armors[id] = [amount, @max_armors].min
end
end
#--------------------------------------------------------------------------
# * Max Armors
#--------------------------------------------------------------------------
def self.max_armors
return @max_armors
end
#--------------------------------------------------------------------------
# * Max All =
# max_all : the new max of items, weapons, and armors you can have.
#--------------------------------------------------------------------------
def self.max_all(max_all)
return unless max_all.is_a?(Integer) || max_all < 0
self.max_items = max_all
self.max_weapons = max_all
self.max_armors = max_all
end
end
#==============================================================================
# ** Game_Party
#------------------------------------------------------------------------------
# This class handles the party. It includes information on amount of gold
# and items. The instance of this class is referenced by $game_party.
#==============================================================================
class Game_Party < Game_Unit
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_accessor :armors, :weapons
#--------------------------------------------------------------------------
# * Gain Items (or lose) !OVERRIDE!
# item : Item
# n : Number
# include_equip : Include equipped items
#--------------------------------------------------------------------------
def gain_item(item, n, include_equip = false)
number = item_number(item)
case item
when RPG::Item
@items[item.id] = [[number + n, 0].max, Inventory_Limits.max_items].min
when RPG::Weapon
@weapons[item.id] = [[number + n, 0].max, Inventory_Limits.max_weapons].min
when RPG::Armor
@armors[item.id] = [[number + n, 0].max, Inventory_Limits.max_armors].min
end
n += number
if include_equip and n < 0
for actor in members
while n < 0 and actor.equips.include?(item)
actor.discard_equip(item)
n += 1
end
end
end
end
#--------------------------------------------------------------------------
# * Get The Items
#--------------------------------------------------------------------------
def get_items
return @items
end
#--------------------------------------------------------------------------
# * Change Item
# id : the id of the item you want to change the amount of.
# amount : the amount of the item.
#--------------------------------------------------------------------------
def change_item(id, amount)
return unless @items.has_key?(id)
@items[id] = amount
end
end
Inventory_Limits.max_items = n
Inventory_Limits.max_armors = n
Inventory_Limits.max_weapons = n
Inventory_Limits.max_all = n
Inventory_Limits.item_max(item_id, new_max)
Inventory_Limits.weapon_max(weapon_id, new_max)
Inventory_Limits.armor_max(armor_id, new_max)
#==============================================================================
# ** Inventory_Limits
#------------------------------------------------------------------------------
# This class holds the values that dictate the maximum values of that the
# inventory can hold.
#==============================================================================
module Inventory_Limits
#--------------------------------------------------------------------------
# * Constant Variables
#--------------------------------------------------------------------------
#------------------------------------------------------------------------
# - Items_Hash_Index
# The index in $game_variables where the items hash is stored.
#------------------------------------------------------------------------
Items_Hash_Index = 1
#------------------------------------------------------------------------
# - Weapons_Hash_Index
# The index in $game_variables where the weapons hash is stored.
#------------------------------------------------------------------------
Weapons_Hash_Index = 2
#------------------------------------------------------------------------
# - Armors_Hash_Index
# The index in $game_variables where the armors hash is stored.
#------------------------------------------------------------------------
Armors_Hash_Index = 3
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
@max_items = {
0 => 60,
1 => 100,
4 => 50,
}
# If the item is not mentioned in the max items hash.
@max_items.default = 99
@max_armors = {
0 => 255,
1 => 100,
4 => 70,
}
# If the item is not mentioned in the max armors hash.
@max_armors.default = 99
@max_weapons = {
0 => 2,
1 => 67,
4 => 123,
}
@max_weapons.default = 99
#--------------------------------------------------------------------------
# * Max Items =
# item_id : the id of the item you want to set the max for.
# max_items : the new maximum amount of items you can have.
#--------------------------------------------------------------------------
def self.max_items=(item_id, max_items)
return unless max_items.is_a?(Integer) || max_items < 0
@max_items[item_id] = max_items
return if $game_party.nil?
if $game_party.get_items.has_key?(item_id)
$game_party.change_item(item_id, [amount, @max_items[item_id]].min)
end
end
#--------------------------------------------------------------------------
# * Max Items
# item_id : the id of the item you are trying to retrieve the max for.
#--------------------------------------------------------------------------
def self.max_items(item_id = nil)
return @max_items[item_id]
end
#--------------------------------------------------------------------------
# * Max Weapons =
# weapon_id : the id of the weapon you are trying to set the max for.
# max_weapons : the new maximum amount of weapons you can have.
#--------------------------------------------------------------------------
def self.max_weapons=(weapon_id, max_weapons)
return unless max_weapons.is_a?(Integer) || max_weapons < 0
@max_weapons[weapon_id] = max_weapons
return if $game_party.nil?
if $game_party.weapons.has_key?(weapon_id)
$game_party.weapons[weapon_id] = [amount, @max_weapons[weapon_id]].min
end
end
#--------------------------------------------------------------------------
# * Max Weapons
# weapon_id : the id of the weapon you are trying to retrieve the max
# for.
#--------------------------------------------------------------------------
def self.max_weapons(weapon_id = nil)
return @max_weapons[weapon_id]
end
#--------------------------------------------------------------------------
# * Max Armors =
# armor_id : the id of the armor you are trying to set the max for.
# max_items : the new maximum amount of armors you can have.
#--------------------------------------------------------------------------
def self.max_armors=(armor_id, max_armors)
return unless max_armors.is_a?(Integer) || max_armors < 0
@max_armors[armor_id] = max_armors
return if $game_party.nil?
if $game_party.armors.has_key?(armor_id)
$game_party.armors[armor_id] = [amount, @max_armors[armor_id]].min
end
end
#--------------------------------------------------------------------------
# * Max Armors
# armor_id : the id of the armor you are trying to retrieve the max
# for.
#--------------------------------------------------------------------------
def self.max_armors(armor_id = nil)
return @max_armors[armor_id]
end
def self.get_items
return @max_items
end
def self.get_weapons
return @max_weapons
end
def self.get_armors
return @max_armors
end
def self.set_items=(items)
@max_items = items
end
def self.set_weapons=(weapons)
@max_weapons = weapons
end
def self.set_armors=(armors)
@max_armors = armors
end
end
#==============================================================================
# ** Scene_File
#------------------------------------------------------------------------------
# This class performs the save and load screen processing.
#==============================================================================
class Scene_File < Scene_Base
#--------------------------------------------------------------------------
# * Alias Methods
#--------------------------------------------------------------------------
alias_method :omon_item_max_scene_file_do_save, :do_save
alias_method :omon_item_max_scene_file_do_load, :do_load
#--------------------------------------------------------------------------
# * Execute Save
#--------------------------------------------------------------------------
def do_save
# Save the current items to the $game_variables.
$game_variables[Inventory_Limits::Items_Hash_Index] =
Inventory_Limits.get_items
$game_variables[Inventory_Limits::Weapons_Hash_Index] =
Inventory_Limits.get_weapons
$game_variables[Inventory_Limits::Armors_Hash_Index] =
Inventory_Limits.get_armors
omon_item_max_scene_file_do_save
end
#--------------------------------------------------------------------------
# * Execute Load
#--------------------------------------------------------------------------
def do_load
omon_item_max_scene_file_do_load
if $game_variables[Inventory_Limits::Items_Hash_Index].is_a?(Hash)
Inventory_Limits.set_items =
$game_variables[Inventory_Limits::Items_Hash_Index]
else
items = Hash.new
items.default = 99
Inventory_Limits.set_items = items
end
if $game_variables[Inventory_Limits::Weapons_Hash_Index].is_a?(Hash)
# Load the loaded items.
Inventory_Limits.set_weapons =
$game_variables[Inventory_Limits::Weapons_Hash_Index]
else
weapons = Hash.new
weapons.default = 99
Inventory_Limits.set_items = weapons
end
if $game_variables[Inventory_Limits::Armors_Hash_Index].is_a?(Hash)
# Load the loaded items.
Inventory_Limits.set_armors =
$game_variables[Inventory_Limits::Armors_Hash_Index]
else
armors = Hash.new
armors.default = 99
Inventory_Limits.set_items = armors
end
end
end
#==============================================================================
# ** Game_Party
#------------------------------------------------------------------------------
# This class handles the party. It includes information on amount of gold
# and items. The instance of this class is referenced by $game_party.
#==============================================================================
class Game_Party < Game_Unit
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_accessor :armors, :weapons
#--------------------------------------------------------------------------
# * Gain Items (or lose) !OVERRIDE!
# item : Item
# n : Number
# include_equip : Include equipped items
#--------------------------------------------------------------------------
def gain_item(item, n, include_equip = false)
number = item_number(item)
case item
when RPG::Item
@items[item.id] =
[[number + n, 0].max, Inventory_Limits.max_items(item.id)].min
when RPG::Weapon
@weapons[item.id] =
[[number + n, 0].max, Inventory_Limits.max_weapons(item.id)].min
when RPG::Armor
@armors[item.id] =
[[number + n, 0].max, Inventory_Limits.max_armors(item.id)].min
end
n += number
if include_equip and n < 0
for actor in members
while n < 0 and actor.equips.include?(item)
actor.discard_equip(item)
n += 1
end
end
end
end
#--------------------------------------------------------------------------
# * Get The Items
#--------------------------------------------------------------------------
def get_items
return @items
end
#--------------------------------------------------------------------------
# * Change Item
# id : the id of the item you want to change the amount of.
# amount : the amount of the item.
#--------------------------------------------------------------------------
def change_item(id, amount)
return unless @items.has_key?(id)
@items[id] = amount
end
end
khmp":2a3m4tfd said:... :dead: You should mention that clearly for the sake of my sanity. Right now its set for inventory overall, not item specific. *drags feet back to uncomfortable swivel chair* Let me get back to work.
[edit]
Ok. There are three more constants to be aware of at the top of Inventory_Limits. Change these numbers to the indexes of the $game_variables you will use to have the inventory limits too. Just because I didn't want to destroy any previous saves. To specify a item maximum now:
Code:Inventory_Limits.item_max(item_id, new_max) Inventory_Limits.weapon_max(weapon_id, new_max) Inventory_Limits.armor_max(armor_id, new_max)
I recommend saving a game first and not loading an existing save as it will reset the inventory limits to a default of 99.
Code:#============================================================================== # ** Inventory_Limits #------------------------------------------------------------------------------ # This class holds the values that dictate the maximum values of that the # inventory can hold. #============================================================================== module Inventory_Limits #-------------------------------------------------------------------------- # * Constant Variables #-------------------------------------------------------------------------- #------------------------------------------------------------------------ # - Items_Hash_Index # The index in $game_variables where the items hash is stored. #------------------------------------------------------------------------ Items_Hash_Index = 1 #------------------------------------------------------------------------ # - Weapons_Hash_Index # The index in $game_variables where the weapons hash is stored. #------------------------------------------------------------------------ Weapons_Hash_Index = 2 #------------------------------------------------------------------------ # - Armors_Hash_Index # The index in $game_variables where the armors hash is stored. #------------------------------------------------------------------------ Armors_Hash_Index = 3 #-------------------------------------------------------------------------- # * Public Instance Variables #-------------------------------------------------------------------------- @max_items = { 0 => 60, 1 => 100, 4 => 50, } # If the item is not mentioned in the max items hash. @max_items.default = 99 @max_armors = { 0 => 255, 1 => 100, 4 => 70, } # If the item is not mentioned in the max armors hash. @max_armors.default = 99 @max_weapons = { 0 => 2, 1 => 67, 4 => 123, } @max_weapons.default = 99 #-------------------------------------------------------------------------- # * Max Items = # item_id : the id of the item you want to set the max for. # max_items : the new maximum amount of items you can have. #-------------------------------------------------------------------------- def self.max_items=(item_id, max_items) return unless max_items.is_a?(Integer) || max_items < 0 @max_items[item_id] = max_items return if $game_party.nil? if $game_party.get_items.has_key?(item_id) $game_party.change_item(item_id, [amount, @max_items[item_id]].min) end end #-------------------------------------------------------------------------- # * Max Items # item_id : the id of the item you are trying to retrieve the max for. #-------------------------------------------------------------------------- def self.max_items(item_id = nil) return @max_items[item_id] end #-------------------------------------------------------------------------- # * Max Weapons = # weapon_id : the id of the weapon you are trying to set the max for. # max_weapons : the new maximum amount of weapons you can have. #-------------------------------------------------------------------------- def self.max_weapons=(weapon_id, max_weapons) return unless max_weapons.is_a?(Integer) || max_weapons < 0 @max_weapons[weapon_id] = max_weapons return if $game_party.nil? if $game_party.weapons.has_key?(weapon_id) $game_party.weapons[weapon_id] = [amount, @max_weapons[weapon_id]].min end end #-------------------------------------------------------------------------- # * Max Weapons # weapon_id : the id of the weapon you are trying to retrieve the max # for. #-------------------------------------------------------------------------- def self.max_weapons(weapon_id = nil) return @max_weapons[weapon_id] end #-------------------------------------------------------------------------- # * Max Armors = # armor_id : the id of the armor you are trying to set the max for. # max_items : the new maximum amount of armors you can have. #-------------------------------------------------------------------------- def self.max_armors=(armor_id, max_armors) return unless max_armors.is_a?(Integer) || max_armors < 0 @max_armors[armor_id] = max_armors return if $game_party.nil? if $game_party.armors.has_key?(armor_id) $game_party.armors[armor_id] = [amount, @max_armors[armor_id]].min end end #-------------------------------------------------------------------------- # * Max Armors # armor_id : the id of the armor you are trying to retrieve the max # for. #-------------------------------------------------------------------------- def self.max_armors(armor_id = nil) return @max_armors[armor_id] end def self.get_items return @max_items end def self.get_weapons return @max_weapons end def self.get_armors return @max_armors end def self.set_items=(items) @max_items = items end def self.set_weapons=(weapons) @max_weapons = weapons end def self.set_armors=(armors) @max_armors = armors end end #============================================================================== # ** Scene_File #------------------------------------------------------------------------------ # This class performs the save and load screen processing. #============================================================================== class Scene_File < Scene_Base #-------------------------------------------------------------------------- # * Alias Methods #-------------------------------------------------------------------------- alias_method :omon_item_max_scene_file_do_save, :do_save alias_method :omon_item_max_scene_file_do_load, :do_load #-------------------------------------------------------------------------- # * Execute Save #-------------------------------------------------------------------------- def do_save # Save the current items to the $game_variables. $game_variables[Inventory_Limits::Items_Hash_Index] = Inventory_Limits.get_items $game_variables[Inventory_Limits::Weapons_Hash_Index] = Inventory_Limits.get_weapons $game_variables[Inventory_Limits::Armors_Hash_Index] = Inventory_Limits.get_armors omon_item_max_scene_file_do_save end #-------------------------------------------------------------------------- # * Execute Load #-------------------------------------------------------------------------- def do_load omon_item_max_scene_file_do_load if $game_variables[Inventory_Limits::Items_Hash_Index].is_a?(Hash) Inventory_Limits.set_items = $game_variables[Inventory_Limits::Items_Hash_Index] else items = Hash.new items.default = 99 Inventory_Limits.set_items = items end if $game_variables[Inventory_Limits::Weapons_Hash_Index].is_a?(Hash) # Load the loaded items. Inventory_Limits.set_weapons = $game_variables[Inventory_Limits::Weapons_Hash_Index] else weapons = Hash.new weapons.default = 99 Inventory_Limits.set_items = weapons end if $game_variables[Inventory_Limits::Armors_Hash_Index].is_a?(Hash) # Load the loaded items. Inventory_Limits.set_armors = $game_variables[Inventory_Limits::Armors_Hash_Index] else armors = Hash.new armors.default = 99 Inventory_Limits.set_items = armors end end end #============================================================================== # ** Game_Party #------------------------------------------------------------------------------ # This class handles the party. It includes information on amount of gold # and items. The instance of this class is referenced by $game_party. #============================================================================== class Game_Party < Game_Unit #-------------------------------------------------------------------------- # * Public Instance Variables #-------------------------------------------------------------------------- attr_accessor :armors, :weapons #-------------------------------------------------------------------------- # * Gain Items (or lose) !OVERRIDE! # item : Item # n : Number # include_equip : Include equipped items #-------------------------------------------------------------------------- def gain_item(item, n, include_equip = false) number = item_number(item) case item when RPG::Item @items[item.id] = [[number + n, 0].max, Inventory_Limits.max_items(item.id)].min when RPG::Weapon @weapons[item.id] = [[number + n, 0].max, Inventory_Limits.max_weapons(item.id)].min when RPG::Armor @armors[item.id] = [[number + n, 0].max, Inventory_Limits.max_armors(item.id)].min end n += number if include_equip and n < 0 for actor in members while n < 0 and actor.equips.include?(item) actor.discard_equip(item) n += 1 end end end end #-------------------------------------------------------------------------- # * Get The Items #-------------------------------------------------------------------------- def get_items return @items end #-------------------------------------------------------------------------- # * Change Item # id : the id of the item you want to change the amount of. # amount : the amount of the item. #-------------------------------------------------------------------------- def change_item(id, amount) return unless @items.has_key?(id) @items[id] = amount end end