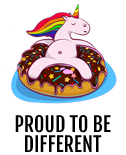
# Make actor command window
s1 = $data_system.words.attack
s2 = $data_system.words.skill
s3 = $data_system.words.guard
s4 = $data_system.words.item
@actor_command_window = Window_Command.new(160, [s1, s2, s3, s4])
@actor_command_window.y = 160
@actor_command_window.back_opacity = 160
@actor_command_window.active = false
@actor_command_window.visible = false
# Make actor command window
s1 = $data_system.words.attack
s2 = $data_system.words.skill
s3 = $data_system.words.guard
s4 = $data_system.words.item
# this is the line we're changing, don't display attack, and make the window shorter
@actor_command_window = Window_Command.new(128, [s2, s3, s4])
@actor_command_window.y = 160
@actor_command_window.back_opacity = 160
@actor_command_window.active = false
@actor_command_window.visible = false
# If C button was pressed
if Input.trigger?(Input::C)
y = @active_battler.battle_commands
x = y[@actor_command_windows[@actor_index].index]
case x
when 0 # attack
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Set action
@active_battler.current_action.kind = 0
@active_battler.current_action.basic = 0
# Start enemy selection
start_enemy_select
when 1 # skill
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Set action
@active_battler.current_action.kind = 1
# Start skill selection
start_skill_select
when 2 # guard
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Set action
@active_battler.current_action.kind = 0
@active_battler.current_action.basic = 1
# Go to command input for next actor
phase3_next_actor
when 3 # item
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Set action
@active_battler.current_action.kind = 2
# Start item selection
start_item_select
end
return
end
# If C button was pressed
if Input.trigger?(Input::C)
y = @active_battler.battle_commands
x = y[@actor_command_windows[@actor_index].index]
case x
when 0 # skill
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Set action
@active_battler.current_action.kind = 1
# Start skill selection
start_skill_select
when 1 # guard
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Set action
@active_battler.current_action.kind = 0
@active_battler.current_action.basic = 1
# Go to command input for next actor
phase3_next_actor
when 2 # item
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Set action
@active_battler.current_action.kind = 2
# Start item selection
start_item_select
end
return
end
class Scene_Battle
def main
# Initialize each kind of temporary battle data
$game_temp.in_battle = true
$game_temp.battle_turn = 0
$game_temp.battle_event_flags.clear
$game_temp.battle_abort = false
$game_temp.battle_main_phase = false
$game_temp.battleback_name = $game_map.battleback_name
$game_temp.forcing_battler = nil
# Initialize battle event interpreter
$game_system.battle_interpreter.setup(nil, 0)
# Prepare troop
@troop_id = $game_temp.battle_troop_id
$game_troop.setup(@troop_id)
# Make actor command window
s1 = $data_system.words.skill
s2 = $data_system.words.guard
s3 = $data_system.words.item
@actor_command_window = Window_Command.new(160, [s1, s2, s3])
@actor_command_window.y = 160
@actor_command_window.back_opacity = 160
@actor_command_window.active = false
@actor_command_window.visible = false
# Make other windows
@party_command_window = Window_PartyCommand.new
@help_window = Window_Help.new
@help_window.back_opacity = 160
@help_window.visible = false
@status_window = Window_BattleStatus.new
@message_window = Window_Message.new
# Make sprite set
@spriteset = Spriteset_Battle.new
# Initialize wait count
@wait_count = 0
# Execute transition
if $data_system.battle_transition == ""
Graphics.transition(20)
else
Graphics.transition(40, "Graphics/Transitions/" +
$data_system.battle_transition)
end
# Start pre-battle phase
start_phase1
# Main loop
loop do
# Update game screen
Graphics.update
# Update input information
Input.update
# Frame update
update
# Abort loop if screen is changed
if $scene != self
break
end
end
# Refresh map
$game_map.refresh
# Prepare for transition
Graphics.freeze
# Dispose of windows
@actor_command_window.dispose
@party_command_window.dispose
@help_window.dispose
@status_window.dispose
@message_window.dispose
if @skill_window != nil
@skill_window.dispose
end
if @item_window != nil
@item_window.dispose
end
if @result_window != nil
@result_window.dispose
end
# Dispose of sprite set
@spriteset.dispose
# If switching to title screen
if $scene.is_a?(Scene_Title)
# Fade out screen
Graphics.transition
Graphics.freeze
end
# If switching from battle test to any screen other than game over screen
if $BTEST and not $scene.is_a?(Scene_Gameover)
$scene = nil
end
end
def update_phase3_basic_command
# If B button was pressed
if Input.trigger?(Input::B)
# Play cancel SE
$game_system.se_play($data_system.cancel_se)
# Go to command input for previous actor
phase3_prior_actor
return
end
# If C button was pressed
if Input.trigger?(Input::C)
# Branch by actor command window cursor position
case @actor_command_window.index
when 0 # skill
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Set action
@active_battler.current_action.kind = 1
# Start skill selection
start_skill_select
when 1 # guard
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Set action
@active_battler.current_action.kind = 0
@active_battler.current_action.basic = 1
# Go to command input for next actor
phase3_next_actor
when 2 # item
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Set action
@active_battler.current_action.kind = 2
# Start item selection
start_item_select
end
return
end
end
end
Myonosken said:s1 = $data_system.words.attack
I don't know why Ccoa left this as this is what displays attack.