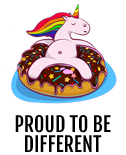
#==============================================================================
# ** Scene_Title
#------------------------------------------------------------------------------
# This class performs title screen processing.
#==============================================================================
class Scene_Title
#--------------------------------------------------------------------------
# * Main Processing
#--------------------------------------------------------------------------
def main
# If battle test
if $BTEST
battle_test
return
end
# Load database
$data_actors = load_data("Data/Actors.rxdata")
$data_classes = load_data("Data/Classes.rxdata")
$data_skills = load_data("Data/Skills.rxdata")
$data_items = load_data("Data/Items.rxdata")
$data_weapons = load_data("Data/Weapons.rxdata")
$data_armors = load_data("Data/Armors.rxdata")
$data_enemies = load_data("Data/Enemies.rxdata")
$data_troops = load_data("Data/Troops.rxdata")
$data_states = load_data("Data/States.rxdata")
$data_animations = load_data("Data/Animations.rxdata")
$data_tilesets = load_data("Data/Tilesets.rxdata")
$data_common_events = load_data("Data/CommonEvents.rxdata")
$data_system = load_data("Data/System.rxdata")
# Make system object
$game_system = Game_System.new
# Make title graphic
@sprite = Sprite.new
@sprite.bitmap = RPG::Cache.title($data_system.title_name)
# Make command window
s1 = "New Game"
s2 = "Continue"
s3 = "Play Online"
s4 = "Credits"
s5 = "Shutdown"
@command_window = Window_Command.new(192, [s1, s2, s3, s4, s5])
@command_window.back_opacity = 160
@command_window.x = 320 - @command_window.width / 2
@command_window.y = 288
# Continue enabled determinant
# Check if at least one save file exists
# If enabled, make @continue_enabled true; if disabled, make it false
@continue_enabled = false
for i in 0..4
if FileTest.exist?("Save#{i+1}.rxdata")
@continue_enabled = true
end
end
# If continue is enabled, move cursor to "Continue"
# If disabled, display "Continue" text in gray
if @continue_enabled
# The 5 stands for the options you can chose from.
# If you have the command window go to s8 put an 8 where the 5 is.
@command_window.height = 5 * 32 + 32
@command_window.index = 1
else
@command_window.disable_item(1)
end
# Play title BGM
$game_system.bgm_play($data_system.title_bgm)
# Stop playing ME and BGS
Audio.me_stop
Audio.bgs_stop
# Execute transition
Graphics.transition
# Main loop
loop do
# Update game screen
Graphics.update
# Update input information
Input.update
# Frame update
update
# Abort loop if screen is changed
if $scene != self
break
end
end
# Prepare for transition
Graphics.freeze
# Dispose of command window
@command_window.dispose
# Dispose of title graphic
@sprite.bitmap.dispose
@sprite.dispose
end
#--------------------------------------------------------------------------
# * Frame Update
#--------------------------------------------------------------------------
def update
# Update command window
@command_window.update
# If C button was pressed
if Input.trigger?(Input::C)
# Branch by command window cursor position
case @command_window.index
when 0 # New game
command_new_game
when 1 # Continue
command_continue
when 2 # Play online
command_online
when 3 # Credits
command_credits
when 4 # Shutdown
command_shutdown
end
end
end
#--------------------------------------------------------------------------
# * Command: New Game
#--------------------------------------------------------------------------
def command_new_game
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Stop BGM
Audio.bgm_stop
# Reset frame count for measuring play time
Graphics.frame_count = 0
# Make each type of game object
$game_temp = Game_Temp.new
$game_system = Game_System.new
$game_switches = Game_Switches.new
$game_variables = Game_Variables.new
$game_self_switches = Game_SelfSwitches.new
$game_screen = Game_Screen.new
$game_actors = Game_Actors.new
$game_party = Game_Party.new
$game_troop = Game_Troop.new
$game_map = Game_Map.new
$game_player = Game_Player.new
# Set up initial party
$game_party.setup_starting_members
# Set up initial map position
$game_map.setup($data_system.start_map_id)
# Move player to initial position
$game_player.moveto($data_system.start_x, $data_system.start_y)
# Refresh player
$game_player.refresh
# Run automatic change for BGM and BGS set with map
$game_map.autoplay
# Update map (run parallel process event)
$game_map.update
# Switch to map screen
$scene = Scene_Map.new
end
#--------------------------------------------------------------------------
# * Command: Continue
#--------------------------------------------------------------------------
def command_continue
# If continue is disabled
unless @continue_enabled
# Play buzzer SE
$game_system.se_play($data_system.buzzer_se)
return
end
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to load screen
$scene = Scene_Load.new
end
#--------------------------------------------------------------------------
# * Command: Play Online
#--------------------------------------------------------------------------
def command_online
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Stop BGM
Audio.bgm_stop
# Set x to the id of the switch used in the nrmxp online script.
$game_switches[x] = true
end
#--------------------------------------------------------------------------
# * Command: Credits
#--------------------------------------------------------------------------
def command_credits
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Stop BGM
Audio.bgm_stop
# Switch to credit screen
$scene = Scene_Credits.new # Change this to your credits screen.
end
#--------------------------------------------------------------------------
# * Command: Shutdown
#--------------------------------------------------------------------------
def command_shutdown
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Fade out BGM, BGS, and ME
Audio.bgm_fade(800)
Audio.bgs_fade(800)
Audio.me_fade(800)
# Shutdown
$scene = nil
end
#--------------------------------------------------------------------------
# * Battle Test
#--------------------------------------------------------------------------
def battle_test
# Load database (for battle test)
$data_actors = load_data("Data/BT_Actors.rxdata")
$data_classes = load_data("Data/BT_Classes.rxdata")
$data_skills = load_data("Data/BT_Skills.rxdata")
$data_items = load_data("Data/BT_Items.rxdata")
$data_weapons = load_data("Data/BT_Weapons.rxdata")
$data_armors = load_data("Data/BT_Armors.rxdata")
$data_enemies = load_data("Data/BT_Enemies.rxdata")
$data_troops = load_data("Data/BT_Troops.rxdata")
$data_states = load_data("Data/BT_States.rxdata")
$data_animations = load_data("Data/BT_Animations.rxdata")
$data_tilesets = load_data("Data/BT_Tilesets.rxdata")
$data_common_events = load_data("Data/BT_CommonEvents.rxdata")
$data_system = load_data("Data/BT_System.rxdata")
# Reset frame count for measuring play time
Graphics.frame_count = 0
# Make each game object
$game_temp = Game_Temp.new
$game_system = Game_System.new
$game_switches = Game_Switches.new
$game_variables = Game_Variables.new
$game_self_switches = Game_SelfSwitches.new
$game_screen = Game_Screen.new
$game_actors = Game_Actors.new
$game_party = Game_Party.new
$game_troop = Game_Troop.new
$game_map = Game_Map.new
$game_player = Game_Player.new
# Set up party for battle test
$game_party.setup_battle_test_members
# Set troop ID, can escape flag, and battleback
$game_temp.battle_troop_id = $data_system.test_troop_id
$game_temp.battle_can_escape = true
$game_map.battleback_name = $data_system.battleback_name
# Play battle start SE
$game_system.se_play($data_system.battle_start_se)
# Play battle BGM
$game_system.bgm_play($game_system.battle_bgm)
# Switch to battle screen
$scene = Scene_Battle.new
end
end
if $game_switches[x] = true
(your nrmxp online script here)
end
$scene = scene_credits.new
razendnomar":2s5k0zck said:Can some good spriter overlook this for any errors?
class Scene_Credits
def main
$scene = Scene_Title.new
end
end
class Scene_Title
def main
#razendnomar's override code here.
end
def update
#razendnomar's override code here.
end
def command_credits
#razendnomar's code here.
end
def command_online
#razendnomar's code here.
end
end
Thanks by the way im not using net play im using nrmxp 2.1.6 and what ever is the best, easy to use would be what im looking for thanks, sorry for double psot if u want me to edit my first i willkhmp":2w70yuls said:Well that error about Scene_Credits not existing is because you probably don't have it defined.
That can just be a filler for someone who doesn't have the credits scene.Code:class Scene_Credits def main $scene = Scene_Title.new end end
Uh next big thing. Alias or override what you need. No need to replace an entire class with the same definition with altered functionality. The only methods you changed from the default ones are main and update you can just make another class definition of Scene_Title to append to it. You don't need command_newgame, command_continue, command_shutdown, or battle_test.
Code:class Scene_Title def main #razendnomar's override code here. end def update #razendnomar's override code here. end def command_credits #razendnomar's code here. end def command_online #razendnomar's code here. end end
Next thing. I wouldn't use a $game_variables or $game_switch in this case to dictate whether you are using online or offline. I would create a new global boolean for something so important. $online = true/false, you would need to add some additional saving and loading code to make sure the variable is saved with a save game as well as loaded with a continue. Although I'm not sure how you want to handle that exactly. This depends upon design. You can do the usual and have the same character for online as you do offline or have two separate characters and it gets rather messy thinking about it. Anyway back to the reason we shouldn't use $game_variables/$game_switches. The reason why is because they are initialized in a new game so anything you save to them even if you create the array yourself it will be lost the moment they select New Game. Also when loading a game through Continue these arrays are overwritten with the save data. I'm know next to nothing about Netplay's code so I have no idea how to present the user with a login interface or any of the fancy things you see with a ORPG. In any case.
Good luck with it Brody! :thumb:
def command_online
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Stop BGM
Audio.bgm_stop
# Online is the boolean used to determen you use nrmxp or not.
$online = true
end
if $online = true
(your nrmxp online script here)
end
Kain Nobel":2qw7ur4u said:razendnomar":2qw7ur4u said:Can some good spriter overlook this for any errors?
*Cough*scripter*cough*
Code:def update #razendnomar's override code here. end
=begin=======================================================================
This is my first script. Insert it above main. Thanks to Khmp to let me use a
boolean for the play online.
One extra note: don't forget to add the following to your online script:
if $online = true
Put your online script here!!
end
I've tested it and it doesn't have any errors in it. I hope this helped.
=============================================================================
=end
class Scene_Title
def main
# If battle test
if $BTEST
battle_test
return
end
# Load database
$data_actors = load_data("Data/Actors.rxdata")
$data_classes = load_data("Data/Classes.rxdata")
$data_skills = load_data("Data/Skills.rxdata")
$data_items = load_data("Data/Items.rxdata")
$data_weapons = load_data("Data/Weapons.rxdata")
$data_armors = load_data("Data/Armors.rxdata")
$data_enemies = load_data("Data/Enemies.rxdata")
$data_troops = load_data("Data/Troops.rxdata")
$data_states = load_data("Data/States.rxdata")
$data_animations = load_data("Data/Animations.rxdata")
$data_tilesets = load_data("Data/Tilesets.rxdata")
$data_common_events = load_data("Data/CommonEvents.rxdata")
$data_system = load_data("Data/System.rxdata")
# Make system object
$game_system = Game_System.new
# Make title graphic
@sprite = Sprite.new
@sprite.bitmap = RPG::Cache.title($data_system.title_name)
# Make command window
s1 = "New Game"
s2 = "Continue"
s3 = "Play Online"
s4 = "Credits"
s5 = "Shutdown"
@command_window = Window_Command.new(192, [s1, s2, s3, s4, s5])
@command_window.back_opacity = 160
@command_window.x = 320 - @command_window.width / 2
@command_window.y = 288
# Continue enabled determinant
# Check if at least one save file exists
# If enabled, make @continue_enabled true; if disabled, make it false
@continue_enabled = false
for i in 0..4
if FileTest.exist?("Save#{i+1}.rxdata")
@continue_enabled = true
end
end
# If continue is enabled, move cursor to "Continue"
# If disabled, display "Continue" text in gray
if @continue_enabled
# The 5 stands for the options you can chose from.
# If you have the command window go to s8 put an 8 where the 5 is.
@command_window.height = 5 * 32 + 32
@command_window.index = 1
else
@command_window.disable_item(1)
end
# Play title BGM
$game_system.bgm_play($data_system.title_bgm)
# Stop playing ME and BGS
Audio.me_stop
Audio.bgs_stop
# Execute transition
Graphics.transition
# Main loop
loop do
# Update game screen
Graphics.update
# Update input information
Input.update
# Frame update
update
# Abort loop if screen is changed
if $scene != self
break
end
end
# Prepare for transition
Graphics.freeze
# Dispose of command window
@command_window.dispose
# Dispose of title graphic
@sprite.bitmap.dispose
@sprite.dispose
end
def update
# Update command window
@command_window.update
# If C button was pressed
if Input.trigger?(Input::C)
# Branch by command window cursor position
case @command_window.index
when 0 # New game
command_new_game
when 1 # Continue
command_continue
when 2 # Play online
command_online
when 3 # Credits
command_credits
when 4 # Shutdown
command_shutdown
end
end
end
def command_credits
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Stop BGM
Audio.bgm_stop
# Switch to credit screen
$scene = Scene_Credits.new # Change this to your credits screen.
end
def command_online
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Stop BGM
Audio.bgm_stop
=begin Don't forget to add the following to your online script.
-------------------------------------------------------------------------
if $online = true
your online script here
end
-------------------------------------------------------------------------
=end
$online = true
end
end
=begin
=============================================================================
Made by: Ramon Hoefnagels a.k.a. Razendnomar a.k.a. nomar
I prefer if u use my own name for credits, because there more users who use
nomar / razendnomar (or any variations of it).
=============================================================================
=============================================================================
Version: 1.1
=============================================================================
1.0: Just edited the Scene_Title.
1.1: Made it as an seperate script.
Also use a boolean for instead of an switch
(Thanks to Khmp to showing me those things)
-----------------------------------------------------------------------------
This is my first script. Insert it above main. Thanks to Khmp to let me use a
boolean for the play online.
One extra note: don't forget to add the following to your online script:
-----------------------------------------------------------------------------
if $online = true
Put your online script here!!
end
-----------------------------------------------------------------------------
-----------------------------------------------------------------------------
If you're using Nrmxp (I made this to be used with it) put that around all the
scenelogin [SC] parts,It's at the end of the scripts list.
Look for ---------- Scene Login -----------. The scripts I mean are below it.
Don't forget to put it around the subparts!
Also put it around all the Network [NET] scripts.
-----------------------------------------------------------------------------
It's now in a testing phase.
At the moment the Credits part is tested and it has no errors in it.
If you have any errors, Pm to razendnomar at rmxp.org, and discribe them,
I'll try to fix them
=============================================================================
=end
class Scene_Title
def main
# If battle test
if $BTEST
battle_test
return
end
# Load database
$data_actors = load_data("Data/Actors.rxdata")
$data_classes = load_data("Data/Classes.rxdata")
$data_skills = load_data("Data/Skills.rxdata")
$data_items = load_data("Data/Items.rxdata")
$data_weapons = load_data("Data/Weapons.rxdata")
$data_armors = load_data("Data/Armors.rxdata")
$data_enemies = load_data("Data/Enemies.rxdata")
$data_troops = load_data("Data/Troops.rxdata")
$data_states = load_data("Data/States.rxdata")
$data_animations = load_data("Data/Animations.rxdata")
$data_tilesets = load_data("Data/Tilesets.rxdata")
$data_common_events = load_data("Data/CommonEvents.rxdata")
$data_system = load_data("Data/System.rxdata")
# Make system object
$game_system = Game_System.new
# Make title graphic
@sprite = Sprite.new
@sprite.bitmap = RPG::Cache.title($data_system.title_name)
# Make command window
s1 = "New Game"
s2 = "Continue"
s3 = "Play Online"
s4 = "Credits"
s5 = "Shutdown"
@command_window = Window_Command.new(192, [s1, s2, s3, s4, s5])
@command_window.back_opacity = 160
@command_window.x = 320 - @command_window.width / 2
@command_window.y = 288
# Continue enabled determinant
# Check if at least one save file exists
# If enabled, make @continue_enabled true; if disabled, make it false
@continue_enabled = false
for i in 0..4
if FileTest.exist?("Save#{i+1}.rxdata")
@continue_enabled = true
end
end
# If continue is enabled, move cursor to "Continue"
# If disabled, display "Continue" text in gray
if @continue_enabled
# The 5 stands for the options you can chose from.
# If you have the command window go to s8 put an 8 where the 5 is.
@command_window.height = 5 * 32 + 32
@command_window.index = 1
else
@command_window.disable_item(1)
end
# Play title BGM
$game_system.bgm_play($data_system.title_bgm)
# Stop playing ME and BGS
Audio.me_stop
Audio.bgs_stop
# Execute transition
Graphics.transition
# Main loop
loop do
# Update game screen
Graphics.update
# Update input information
Input.update
# Frame update
update
# Abort loop if screen is changed
if $scene != self
break
end
end
# Prepare for transition
Graphics.freeze
# Dispose of command window
@command_window.dispose
# Dispose of title graphic
@sprite.bitmap.dispose
@sprite.dispose
end
def update
# Update command window
@command_window.update
# If C button was pressed
if Input.trigger?(Input::C)
# Branch by command window cursor position
case @command_window.index
when 0 # New game
command_new_game
when 1 # Continue
command_continue
when 2 # Play online
command_online
when 3 # Credits
command_credits
when 4 # Shutdown
command_shutdown
end
end
end
def command_credits
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Stop BGM
Audio.bgm_stop
# Switch to credit screen
$scene = Scene_Credits.new # Change this to your credits screen.
end
def command_online
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Stop BGM
Audio.bgm_stop
#Don't forget to add the following to your online script.
#------------------------------------------------------------------------
#if $online = true
# your online script here
# end
#------------------------------------------------------------------------
$online = true
end
end
#===============================================================================
# ** Scene_Login
# By Vengeance
#===============================================================================
if $online = true
class Scene_Login < Scene_Base
#--------------------------------------------------------------------------
# Initialiation
#--------------------------------------------------------------------------
def initialize
# Loads data (If not loaded)
$data_actors ||= load_data("Data/Actors.rxdata")
$data_classes ||= load_data("Data/Classes.rxdata")
$data_skills ||= load_data("Data/Skills.rxdata")
$data_items ||= load_data("Data/Items.rxdata")
$data_weapons ||= load_data("Data/Weapons.rxdata")
$data_armors ||= load_data("Data/Armors.rxdata")
$data_enemies ||= load_data("Data/Enemies.rxdata")
$data_troops ||= load_data("Data/Troops.rxdata")
$data_states ||= load_data("Data/States.rxdata")
$data_animations ||= load_data("Data/Animations.rxdata")
$data_tilesets ||= load_data("Data/Tilesets.rxdata")
$data_common_events ||= load_data("Data/CommonEvents.rxdata")
# Load system Data
$data_system = load_data("Data/System.rxdata")
# Make System Object
$game_system = Game_System.new
# Make Temporary Object
$game_temp = Game_Temp.new
end
def main
# Make title graphic
@sprite = Sprite.new
@sprite.bitmap = RPG::Cache.title($data_system.title_name)
$Mouse.reset
main_windows
main_data
# Execute transition
Graphics.transition
Network::Main.retrieve_version
Network::Main.retrieve_mod if $game_temp.motd.to_s == "" or $game_temp.motd.to_s == $game_language.read_lang( 0,"NOMOTD")
# Srarts LOOP Update.
loop do
# Update
Network::Main.update if Network::Main.socket != nil
main_loop
break if main_scenechange?
end
# Prepare for transition
$Mouse.reset
Graphics.freeze
@cmd_login.dispose
@cmd_reg.dispose if User_Edit::REGISTRATION_BY_CLIENT
@ckh_box.dispose
@icn_motd.dispose
main_dispose
end
#--------------------------------------------------------------------------
def main_windows
@mainlogin = Window_MainLogin.new
@motd = Window_Motd.new
@motd.x = @mainlogin.x + @mainlogin.width + 8
@motd.y = @mainlogin.y
@motd.width = 260
@motd.height = 180
# Buttons
@cmd_login = Button.new(@mainlogin,120,64 + 12,$game_language.read_lang( 0,"SCLOG_ENTER")) {login}
@cmd_reg = Button.new(@mainlogin,120,96 + 12,$game_language.read_lang( 0,"SCLOG_REGISTER")) {registra} if User_Edit::REGISTRATION_BY_CLIENT
# Check Box
@ckh_box = Check_Box.new(@mainlogin,16,96-24)
# Icon
@icn_motd = Icon.new(@mainlogin,"033-Item02",$game_language.read_lang( 0,"SCLOG_NEWS"),16,96,[$game_language.read_lang( 0,"SCLOG_SHOWWINDOW"),$game_language.read_lang( 0,"SCLOG_LASTNEWS")],false)
end
#--------------------------------------------------------------------------
def main_data
if FileTest.exist?("Account.rxdata")
@dati = []
file = File.open("Account.rxdata", "rb")
@dati = Marshal.load(file)
file.close
@ckh_box.value = true
@mainlogin.set_username = @dati[0]
@mainlogin.set_password = @dati[1]
end
end
#--------------------------------------------------------------------------
def update
@mainlogin.update
@motd.update
@motd.refresh if @motd.active and @motd.visible
@icn_motd.update
# Se premuta l'icona MOTD
if @icn_motd.active == true
@motd.x = @mainlogin.x + @mainlogin.width + 8
@motd.y = @mainlogin.y
@motd.width = 260
@motd.height = 180
if @motd.visible == false
@motd.visible = true
end
@icn_motd.active = false
end
end
def main_dispose
# Dispose of windows
@motd.dispose
@mainlogin.dispose
@icn_motd.dispose
# automatic_dispose
end
#--------------------------------------------------------------------------
def main_scenechange?
# Abort loop if screen is changed
if $scene != self
if @ckh_box.checked
@dati = []
@dati[0] = @mainlogin.username
@dati[1] = @mainlogin.password
file = File.open("Account.rxdata", "wb")
Marshal.dump(@dati,file)
file.close
end
return true
end
return false
end
#--------------------------------------------------------------------------
def set_status(status)
@mainlogin.text = status
@mainlogin.refresh
end
#--------------------------------------------------------------------------
def login
$game_system.se_play($data_system.decision_se)
set_status $game_language.read_lang( 0,"REGISTER_STATUS")
if @mainlogin.username != "" && @mainlogin.password != ""
Network::Main.send_login(@mainlogin.username,@mainlogin.password)
else
set_status $game_language.read_lang( 0,"REGISTER_ERROR")
$game_system.se_play($data_system.cancel_se)
end
end
#--------------------------------------------------------------------------
def registra
$game_system.se_play($data_system.decision_se)
set_status $game_language.read_lang( 0,"REGISTER_STATUS")
if @mainlogin.username != "" && @mainlogin.password != ""
Network::Main.send_register(@mainlogin.username,@mainlogin.password)
else
set_status $game_language.read_lang( 0,"REGISTER_ERROR")
$game_system.se_play($data_system.cancel_se)
end
end
#--------------------------------------------------------------------------
def update_motd
@motd.testo = $game_temp.motd
@motd.refresh
@motd.visible = true
end
def username
return @mainlogin.username
end
end
end
#===============================================================================
# ** Window_MainLogin
# By Vengeance
#===============================================================================
if $online = true
class Window_MainLogin < Window_Base
attr_accessor :text
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
super(80, 48, 240, 180)
@dragable = true
@scalable = false
@clickable = true
@closable = false
@text = $game_language.read_lang( 0,"WMAINLOG_WELCOMEON") + "#{User_Edit::GAME_NAME}"
self.contents = Bitmap.new(width - 32, height - 32)
self.contents.font.size = 16
self.contents.font.color = normal_color
# Username Text Box
self.contents.draw_text(0, -8, self.width-64, 32,$game_language.read_lang( 0,"WMAINLOG_NAME"))
@txt_username = Text_Box.new(self,96,16,100,12,1)
@txt_username.extended
# Password Text Box
self.contents.draw_text(0, -8+24, self.width-64, 32,$game_language.read_lang( 0,"WMAINLOG_PASSWORD"))
@txt_password = Text_Box.new(self,96,42,100,12,1)
@txt_password.extended
self.contents.draw_text(96, -8+42+4, self.width-64, 32,$game_language.read_lang( 0,"WMAINLOG_REGISTER"))
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
self.contents.draw_text(0, -8, self.width-64, 32,$game_language.read_lang( 0,"WMAINLOG_NAME"))
self.contents.draw_text(0, -8+24, self.width-64, 32,$game_language.read_lang( 0,"WMAINLOG_PASSWORD"))
self.contents.draw_text(24, -8+42+12, self.width-64, 32,$game_language.read_lang( 0,"WMAINLOG_REGISTER"))
self.contents.draw_text(0, 120, self.width-4, 32,"#{text}")
end
#--------------------------------------------------------------------------
def username
return @txt_username.text.to_s
end
#--------------------------------------------------------------------------
def password
return @txt_password.text.to_s
end
#--------------------------------------------------------------------------
#--------------------------------------------------------------------------
def set_username=(s)
return @txt_username.text = s
end
#--------------------------------------------------------------------------
def set_password=(s)
return @txt_password.text = s
end
#--------------------------------------------------------------------------
end
end
#===============================================================================
# ** Window_Motd
# By Vengeance
#===============================================================================
if $online = true
class Window_Motd < Window_Base
attr_accessor :testo
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
super(320, 32, 260, 180)
self.visible = false
@dragable = true
@scalable = true
@clickable = true
@closable = true
self.contents = Bitmap.new(width - 32, height - 32)
self.contents.font.size = 16
self.contents.font.color = normal_color
@testo = $game_temp.motd
refresh
end
#--------------------------------------------------------------------------
# * Refresh
#--------------------------------------------------------------------------
def refresh
self.contents.clear
self.contents = Bitmap.new(width - 32, height - 32)
self.contents.font.color = normal_color
self.contents.font.size = 16
if @testo == ""
@testo = $game_language.read_lang( 0,"NOMOTD")
end
@to_draw = @testo.split("<->")
for i in 0...@to_draw.size
self.contents.draw_text(2, (i*24), self.width * 2, 32,@to_draw[i].to_s)
end
end
end
end
#===============================================================================
# ** Module Win32 - Handles numerical based data.
#-------------------------------------------------------------------------------
# Author Ruby
# Version 1.8.1
#===============================================================================
if $onine = true
SDK.log("Win32", "Ruby", "1.8.1", "Unknown")
#-------------------------------------------------------------------------------
# Begin SDK Enabled Check
#-------------------------------------------------------------------------------
if SDK.state('Win32') == true
module Win32
#--------------------------------------------------------------------------
# â—
#===============================================================================
# ** Network - Manages network data.
#-------------------------------------------------------------------------------
# Author Meâ„¢ and Shark_Tooth
# Version 1.0
# Date 11-04-06
#==============================================================================
if $online = true
SDK.log("Network", "Shark_Tooth and Meâ„¢", "1.0", " 11-04-06")
p "TCPSocket script not found (class Network)" if not SDK.state('TCPSocket')
#-------------------------------------------------------------------------------
# Begin SDK Enabled Check
#-------------------------------------------------------------------------------
if SDK.state('TCPSocket') == true and SDK.state('Network')
module Network
class Main
#--------------------------------------------------------------------------
# * Attributes
#--------------------------------------------------------------------------
attr_accessor :socket
attr_accessor :pm
# getpm, pmname, writepm
# AUTHENFICATION = 0 => Authenficates
# ID_REQUEST = 1 => Returns ID
# NAME_REQUEST = 2 => Returns UserName
# GROUP_REQUEST = 3 => Returns Group
# CLOSE = 4 => Close, Stop Connection
# NET_PLAYER = 5 => Netplayers Data
# MAP_PLAYER = 6 => Mapplayers Data
# KICK = 7 => Kick a Player
# KICK ALL = 8 => Kick all Players
# REMOVE = 9 => Remove Player (CLOSE SYNONYM)
# SYSTEM = 10 => System (Var's and Switches)
# MOD_CHANGE = 11 => Message of Day Change
# TRADE = 12 => Trade
# PRIVATE_CHAT = 13 => Private Chat
# = 14
# POKE = 15 => Poke Player
# SLAP = 16 => Decrease HP or SP PLayer
# SLAP ALL = 17 => Decrease Hp or SP ALL
# ADM - MOD = 18 => Admin/Mod Command Returns
# = 19
# TEST STOP = 20 => Connection Test End
#--------------------------------------------------------------------------
# * Initialiation
#--------------------------------------------------------------------------
def self.initialize
@data_to_do = [""]
@mapheader = false
@players = {}
@mapplayers = {}
@netactors = {}
@pm = {}
@pm_lines = []
@user_test = false
@user_exist = false
@socket = nil
@nooprec = 0
@id = -1
@name = ""
@group = ""
@status = ""
@oldx = -1
@oldy = -1
@oldd = -1
@oldp = -1
@oldid = -1
@login = false
@pchat_conf = false
@send_conf = false
@trade_conf = false
@servername = ""
@pm_getting = false
@self_key1 = nil
@self_key2 = nil
@self_key3 = nil
@self_value = nil
@trade_compelete = false
@trading = false
@trade_id = -1
end
def self.mapheader
return @mapheader
end
#--------------------------------------------------------------------------
# * Returns Servername
#--------------------------------------------------------------------------
def self.servername
return @servername.to_s
end
#--------------------------------------------------------------------------
# * Returns Socket
#--------------------------------------------------------------------------
def self.socket
return @socket
end
def self.socket_supporto
return @socket_supporto
end
def self.socket_event
return @socket_event
end
#--------------------------------------------------------------------------
# * Returns UserID
#--------------------------------------------------------------------------
def self.id
return @id
end
#--------------------------------------------------------------------------
# * Returns UserName
#--------------------------------------------------------------------------
def self.name
return @name
end
#--------------------------------------------------------------------------
# * Returns current Status
#--------------------------------------------------------------------------
def self.status
return "" if @status == nil or @status == []
return @status
end
#--------------------------------------------------------------------------
# * Returns Group
#--------------------------------------------------------------------------
def self.group
# CHANGE
if @group.downcase.include?("adm")
group = "admin"
elsif @group.downcase.include?("mod")
group = "mod"
else
group = "standard"
end
return group
end
#--------------------------------------------------------------------------
# * Returns Mapplayers
#--------------------------------------------------------------------------
def self.mapplayers
if @mapplayers == nil
return {}
end
return @mapplayers
end
#--------------------------------------------------------------------------
# * Returns NetActors
#--------------------------------------------------------------------------
def self.netactors
if @netactors == nil
return {}
end
return @netactors
end
#--------------------------------------------------------------------------
# * Returns Players
#--------------------------------------------------------------------------
def self.players
if @players == nil
return {}
end
return @players
end
def self.leave_map(id)
@mapplayers[id.to_s] = "" rescue nil
for player in @mapplayers
if player[0].to_i == id.to_i
@mapplayers.delete(id.to_s)
end
end
if $scene.is_a?(Scene_Map)
begin
$scene.spriteset[id].dispose unless $scene.spriteset[id].disposed?
rescue
nil
end
end
end
def self.destroy(id)
if @players[id.to_s] != nil
@players[id.to_s].on_disconnect
if $game_temp.party_name != "" and @players[id.to_s].party == $game_temp.party_name
$game_netparty.actors.delete(@players[id.to_s]) if $game_netparty.actors.include?(@players[id.to_s])
end
end
@players[id.to_s] = nil rescue nil
@mapplayers[id.to_s] = "" rescue nil
for player in @mapplayers
if player[0].to_i == id.to_i
@mapplayers.delete(id.to_s)
end
end
for player in @players
if player[0].to_i == id.to_i
@players.delete(id.to_s)
end
end
if $scene.is_a?(Scene_Map)
begin
$scene.spriteset[id].dispose unless $scene.spriteset[id].disposed?
rescue
nil
end
end
end
#--------------------------------------------------------------------------
# * Create A socket
#--------------------------------------------------------------------------
def self.start_connection(host, port)
@socket = nil
@socket_supporto = nil
@socket_event = nil
@socket = TCPSocket.new(host, port)
# @socket_supporto = TCPSocket.new(host, port+1)
# @socket_event = TCPSocket.new(host, port+2)
end
#--------------------------------------------------------------------------
# * Asks for Id
#--------------------------------------------------------------------------
def self.get_id
@socket.send("1|<->")
end
#--------------------------------------------------------------------------
# * Asks for name
#--------------------------------------------------------------------------
def self.get_name
@socket.send("2|<->")
end
#--------------------------------------------------------------------------
# * Asks for Group
#--------------------------------------------------------------------------
def self.get_group
#@socket.send("<3>'req'</3>\n")
@group = "standard"
@socket.send("CHECK|#{self.name}|<->")
end
#--------------------------------------------------------------------------
# * Registers (Attempt to)
#--------------------------------------------------------------------------
def self.send_register(user,pass)
# Register with User as name, and Pass as password
@socket.send("REG|#{user}|#{pass}|email@nonvalida|<->")
# Start Loop for Retrival
loop = 0
loop do
loop += 1
self.update
# Break If Registration Succeeded
break if @registered
# Break if Loop reached 10000
break if loop == 10000
end
end
#--------------------------------------------------------------------------
# * Asks for Network Version Number
#--------------------------------------------------------------------------
def self.retrieve_version
@socket.send("VER|#{User_Edit::VERSION}|<->")
end
#--------------------------------------------------------------------------
# * Asks for Message of the day
#--------------------------------------------------------------------------
def self.retrieve_mod
@socket.send("MOD|<->")
#@socket_supporto.send("<successo>#{self.id}</successo>\n")
end
#--------------------------------------------------------------------------
# * Asks for Login (and confirmation)
#--------------------------------------------------------------------------
def self.send_login(user,pass)
@socket.send("LOG|#{user}|#{pass}|<->")
end
#--------------------------------------------------------------------------
# * Send Errors
#--------------------------------------------------------------------------
def self.send_error(lines)
if lines.is_a?(Array)
for line in lines
@socket.send("ERR|#{line}|<->")
end
elsif lines.is_a?(String)
@socket.send("ERR|#{lines}|<->")
end
end
#--------------------------------------------------------------------------
# * Authenfication
#--------------------------------------------------------------------------
def self.amnet_auth
# Send Authenfication
@socket.send("0|#{User_Edit::GAME_CODE}|<->")
@auth = false
# Set Try to 0, then start Loop
try = 0
loop do
# Add 1 to Try
try += 1
loop = 0
# Start Loop for Retrieval
loop do
loop += 1
self.update
# Break if Authenficated
break if @auth
# Break if loop reaches 20000
break if loop == 60000
end
# If the loop was breaked because it reached 10000, display message
p $game_language.read_lang( 0,"NOTAUTH") + ", Tentativo #{try} su #{User_Edit::CONNFAILTRY}" if loop == 60000
# Stop everything if try mets the maximum try's
break if try == User_Edit::CONNFAILTRY
# Break loop if Authenficated
break if @auth
end
# Go to Scene Login if Authenficated
$scene = Scene_Login.new if @auth
end
#--------------------------------------------------------------------------
# * Inventario
#--------------------------------------------------------------------------
def self.send_personal_data
return if $game_party == nil
send = ""
arrayID = ""
arrayQN = ""
for i in 1...$data_items.size
if $game_party.item_number(i) > 0
arrayID += "#{$data_items[i].id},"
arrayQN += "#{$game_party.item_number(i)},"
end
end
send += "ODTITEMSARRAY|#{arrayID}|#{arrayQN}|<->"
arrayID = ""
arrayQN = ""
for i in 1...$data_weapons.size
if $game_party.weapon_number(i) > 0
arrayID += "#{$data_weapons[i].id},"
arrayQN += "#{$game_party.weapon_number(i)},"
end
end
send += "ODTWPNARRAY|#{arrayID}|#{arrayQN}|<->"
arrayID = ""
arrayQN = ""
for i in 1...$data_armors.size
if $game_party.armor_number(i) > 0
arrayID += "#{$data_armors[i].id},"
arrayQN += "#{$game_party.armor_number(i)},"
end
end
send += "ODTARMARRAY|#{arrayID}|#{arrayQN}|<->"
arrayID = ""
arrayQN = ""
for i in 1...$data_skills.size
if $game_party.actors[0].skill_learn?(i)
arrayID += "#{i},"
end
end
send += "ODTSKLLARRAY|#{arrayID}|<->"
send += "DTGUILD|#{$game_temp.guild_name}|<->"
@socket.send("#{send}")
end
#--------------------------------------------------------------------------
# * Send Start Data
#--------------------------------------------------------------------------
def self.send_start
send = ""
# Send Nome Personaggio
send += "DTNOME|#{$game_party.actors[0].name}|<->"
send += "DTCLASSE|#{$game_party.actors[0].class_id}|<->"
# Send Username And character's Graphic Name
send += "DTUSERNAME|#{self.name}|<->DTCHR|#{$game_player.character_name}|<->DTHUE|#{$game_player.character_hue}|<->"
# Sends Map ID, X and Y positions
# Sends Map ID, X and Y positions
send += "DTMAP|#{$game_map.map_id}|<->DTX|#{$game_player.x}|<->DTY|#{$game_player.y}|<->"
# Sends Name
if User_Edit::Bandwith >= 1
send += "DTUSERNAME|#{self.name}|<->"
end
# Sends Direction
if User_Edit::Bandwith >= 2
send += "DTDIR|#{$game_player.direction}|<->"
end
# Sends Move Speed
if User_Edit::Bandwith >= 3
send += "DTMOVESPEED|#{$game_player.move_speed}|<->"
end
# Send Visual equipment if active
# if SDK.state('Visual Equipment') == true
send += "DTEQU|0|#{$game_party.actors[0].weapon_id}|<->"
send += "DTEQU|1|#{$game_party.actors[0].armor1_id}|<->"
send += "DTEQU|2|#{$game_party.actors[0].armor2_id}|<->"
send += "DTEQU|3|#{$game_party.actors[0].armor3_id}|<->"
send += "DTEQU|4|#{$game_party.actors[0].armor4_id}|<->"
# end
send += "DTGUILD|#{$game_temp.guild_name}|<->"
# Sends Requesting start
# send+= "5|start(#{self.id})|<->"
send += "RETREVIE|<->"
send += "ADDTOLIST|<->"
@socket.send("#{send}")
self.send_newstats
#send_actor_start
end
def self.save_server_pg
return if $game_temp.chr_in_use == 0
send = ""
# Send Nome Personaggio
send += "ODTNOME|#{$game_party.actors[0].name}|<->"
send += "ODTCLASSE|#{$game_party.actors[0].class_id}|<->"
# Send Username And character's Graphic Name
send += "ODTUSERNAME|#{self.name}|<->ODTCHR|#{$game_player.character_name}|<->"
# Sends Map ID, X and Y positions
send += "ODTMAP|#{$game_map.map_id}|<->ODTX|#{$game_player.x}|<->ODTY|#{$game_player.y}|<->"
# Sends Name
if User_Edit::Bandwith >= 1
send += "ODTUSERNAME|#{self.name}|<->"
end
# Sends Direction
if User_Edit::Bandwith >= 2
send += "ODTDIR|#{$game_player.direction}|<->"
end
# Sends Move Speed
if User_Edit::Bandwith >= 3
send += "ODTMOVESPEED|#{$game_player.move_speed}|<->"
end
send += "DTEQU|0|#{$game_party.actors[0].weapon_id}|<->"
send += "DTEQU|1|#{$game_party.actors[0].armor1_id}|<->"
send += "DTEQU|2|#{$game_party.actors[0].armor2_id}|<->"
send += "DTEQU|3|#{$game_party.actors[0].armor3_id}|<->"
send += "DTEQU|4|#{$game_party.actors[0].armor4_id}|<->"
send_personal_data
send += "DTGUILD|#{$game_temp.guild_name}|<->"
# Sends Requesting start
@socket.send("#{send}")
hp = $game_party.actors[0].hp
sp = $game_party.actors[0].sp
maxhp = $game_party.actors[0].maxhp
maxsp = $game_party.actors[0].maxsp
agi = $game_party.actors[0].agi
eva = $game_party.actors[0].base_eva
pdef = $game_party.actors[0].pdef
mdef = $game_party.actors[0].mdef
level = $game_party.actors[0].level
a = $game_party.actors[0]
c = "["
m = 1
for i in a.states
next if $data_states[i] == nil
c += i.to_s
c += ", " if m != a.states.size
m += 1
end
c += "]"
stats = "ODTHP|#{hp}|#{maxhp}|<->ODTSP|#{sp}|#{maxsp}|<->ODTAGI|#{agi}|<->ODTEVA|#{eva}|<->ODTPDEF|#{pdef}|<->ODTMDEF|#{mdef}|<->ODTLVL|#{level}|<->"
str = $game_party.actors[0].str
int = $game_party.actors[0].int
dex = $game_party.actors[0].dex
stats += "ODTSTR|#{str}|<->"
stats += "ODTINT|#{int}|<->"
stats += "ODTDEX|#{dex}|<->"
@socket.send("#{stats}")
@socket.send("SAVEPG|<->")
#send_actor_start
end
#def self.send_actor_start
# return
# send =""
# send += "@name=#{$game_party.actors[0].name};"
# send += "@class_id=#{$game_party.actors[0].class_id};"
# send += "@weapon_id=#{$game_party.actors[0].weapon_id};"
# send += "@armor1_id=#{$game_party.actors[0].armor1_id};"
# send += "@armor2_id=#{$game_party.actors[0].armor2_id};"
# send += "@armor3_id=#{$game_party.actors[0].armor3_id};"
# send += "@armor4_id=#{$game_party.actors[0].armor4_id};"
# send += "@level=#{$game_party.actors[0].level};"
# send += "@skills=#{$game_party.actors[0].skills};"
# @socket.send("<netact>data=#{send} id=#{$game_party.actors[0].actor_id}</netact>\n")
#end
#--------------------------------------------------------------------------
# * Return PMs
#-------------------------------------------------------------------------
def self.pm
return @pm
end
#--------------------------------------------------------------------------
# * Get PMs
#--------------------------------------------------------------------------
def self.get_pms
@pm_getting = true
@socket.send("<22a>'Get';</22a>\n")
end
#--------------------------------------------------------------------------
# * Update PMs
#--------------------------------------------------------------------------
def self.update_pms(message)
#Starts all the variables
@pm = {}
@current_Pm = nil
@index = -1
@message = false
#Makes a loop to look thru the message
for line in message
#If the line is a new PM
if line == "$NEWPM"
@index+=1
@pm[@index] = Game_PM.new(@index)
@message = false
#if the word has $to_from
elsif line == "$to_from" and @message == false
to_from = true
#if the word has $subject
elsif line == "$Subject" and @message == false
subject = true
#if the word has $message
elsif line == "$Message" and @message == false
@message = true
elsif @message
@pm[@index].message += line+"\n"
elsif to_from
@pm[@index].to_from = line
to_from = false
elsif subject
@pm[@index].subject = line
subject = false
end
end
@pm_getting = false
end
#--------------------------------------------------------------------------
# * Checks if the PM is still not get.
#--------------------------------------------------------------------------
def self.pm_getting
return @pm_getting
end
#--------------------------------------------------------------------------
# * Write PMs
#--------------------------------------------------------------------------
def self.write_pms(message)
send = message
@socket.send("<22d>#{send}</22d>\n")
end
#--------------------------------------------------------------------------
# * Delete All PMs
#--------------------------------------------------------------------------
def self.delete_all_pms
@socket.send("<22e>'delete'</22e>\n")
@pm = {}
end
#--------------------------------------------------------------------------
# * Delete pm(id)
#--------------------------------------------------------------------------
def self.delete_pm(id)
@pm.delete(id)
end
#--------------------------------------------------------------------------
# * Send Requested Data
#--------------------------------------------------------------------------
def self.send_start_request(id)
send = ""
# Send Nome Personaggio
send += "DTNOME|#{$game_party.actors[0].name}|<->"
send += "DTCLASSE|#{$game_party.actors[0].class_id}|<->"
# Send Username And character's Graphic Name
send += "DTUSERNAME|#{self.name}|<->DTCHR|#{$game_player.character_name}|<->DTHUE|#{$game_player.character_hue}|<->"
# Sends Map ID, X and Y positions
send += "DTMAP|#{$game_map.map_id}|<->DTX|#{$game_player.x}|<->DTY|#{$game_player.y}|<->"
# Sends Name
if User_Edit::Bandwith >= 1
send += "DTUSERNAME|#{self.name}|<->"
end
# Sends Direction
if User_Edit::Bandwith >= 2
send += "DTDIR|#{$game_player.direction}|<->"
end
# Sends Move Speed
if User_Edit::Bandwith >= 3
send += "DTMOVESPEED|#{$game_player.move_speed}|<->"
end
@socket.send("#{send}")
self.send_newstats
#self.send_map
#self.send_actor_start
end
#--------------------------------------------------------------------------
# * Send Map Id data
#--------------------------------------------------------------------------
def self.send_map
send = ""
# Send Nome Personaggio
send += "DTNOME|#{$game_party.actors[0].name}|<->"
send += "DTCLASSE|#{$game_party.actors[0].class_id}|<->"
# Send Username And character's Graphic Name
send += "DTUSERNAME|#{self.name}|<->DTCHR|#{$game_player.character_name}|<->DTHUE|#{$game_player.character_hue}|<->"
# Sends Map ID, X and Y positions
send += "DTMAP|#{$game_map.map_id}|<->DTX|#{$game_player.x}|<->DTY|#{$game_player.y}|<->"
# Sends Name
if User_Edit::Bandwith >= 1
send += "DTUSERNAME|#{self.name}|<->"
end
# Sends Direction
if User_Edit::Bandwith >= 2
send += "DTDIR|#{$game_player.direction}|<->"
end
# Sends Move Speed
if User_Edit::Bandwith >= 3
send += "DTMOVESPEED|#{$game_player.move_speed}|<->"
end
# Send Visual equipment if active
# if SDK.state('Visual Equipment') == true
send += "DTEQU|0|#{$game_party.actors[0].weapon_id}|<->"
send += "DTEQU|1|#{$game_party.actors[0].armor1_id}|<->"
send += "DTEQU|2|#{$game_party.actors[0].armor2_id}|<->"
send += "DTEQU|3|#{$game_party.actors[0].armor3_id}|<->"
send += "DTEQU|4|#{$game_party.actors[0].armor4_id}|<->"
#end
send_personal_data
# Sends Status
@socket.send("DTOST|Online|<->")
@socket.send("#{send}")
@socket.send("REQUESTMAPDATA|#{$game_map.map_id}|<->")
self.send_newstats
for player in @players.values
next if player.netid == -1
# If the Player is on the same map...
if player.map_id == $game_map.map_id
# Update Map Players
self.update_map_player(player.netid, nil)
elsif @mapplayers[player.netid.to_s] != nil
# Remove from Map Players
self.update_map_player(player.netid, nil, true)
end
end
end
def self.send_newstat
return self.send_newstats
end
def self.send_newstats
return if $game_party == nil
hp = $game_party.actors[0].hp
sp = $game_party.actors[0].sp
maxhp = $game_party.actors[0].maxhp
maxsp = $game_party.actors[0].maxsp
agi = $game_party.actors[0].agi
eva = $game_party.actors[0].base_eva
pdef = $game_party.actors[0].pdef
mdef = $game_party.actors[0].mdef
level = $game_party.actors[0].level
exp = $game_party.actors[0].exp
now_exp = $game_party.actors[0].now_exp
next_exp = $game_party.actors[0].next_exp
a = $game_party.actors[0]
c = "["
m = 1
for i in a.states
next if $data_states[i] == nil
c += i.to_s
c += ", " if m != a.states.size
m += 1
end
c += "]"
stats = "DTHP|#{hp}|#{maxhp}|<->DTSP|#{sp}|#{maxsp}|<->DTAGI|#{agi}|<->DTEVA|#{eva}|<->DTPDEF|#{pdef}|<->DTMDEF|#{mdef}|<->DTLVL|#{level}|<->DTEXP|#{exp}|#{now_exp}|#{next_exp}<->"
str = $game_party.actors[0].str
int = $game_party.actors[0].int
dex = $game_party.actors[0].dex
stats += "ODTSTR|#{str}|<->"
stats += "ODTINT|#{int}|<->"
stats += "ODTDEX|#{dex}|<->"
@socket.send("#{stats}")
end
def self.send_btstats
hp = $game_party.actors[0].hp
sp = $game_party.actors[0].sp
maxhp = $game_party.actors[0].maxhp
maxsp = $game_party.actors[0].maxsp
stats = "DTBTS|#{hp}|#{maxhp}|#{sp}|#{maxsp}|<->"
@socket.send("#{stats}")
end
#--------------------------------------------------------------------------
# * Send Move Update
#--------------------------------------------------------------------------
def self.send_move_change
send = ""
if @mapplayers == {}
# Increase Steps if the oldx or the oldy do not match the new ones
if User_Edit::Bandwith >= 1
if @oldx != $game_player.x or @oldy != $game_player.y
send += "5|ic|<->"
end
end
# New x if x does not mathc the old one
if @oldx != $game_player.x
send += "ODTX|#{$game_player.x}|<->"
@oldx = $game_player.x
end
# New y if y does not match the old one
if @oldy != $game_player.y
send += "ODTY|#{$game_player.y}|<->"
@oldy = $game_player.y
end
# Send the Direction if it is different then before
if User_Edit::Bandwith >= 2
if @oldd != $game_player.direction
send += "ODTDIR|#{$game_player.direction}|<->"
@oldd = $game_player.direction
end
end
else
# Increase Steps if the oldx or the oldy do not match the new ones
if User_Edit::Bandwith >= 1
if @oldx != $game_player.x or @oldy != $game_player.y
send += "5|ic|<->"
end
end
# New x if x does not mathc the old one
if @oldx != $game_player.x
send += "DTX|#{$game_player.x}|<->"
@oldx = $game_player.x
end
# New y if y does not match the old one
if @oldy != $game_player.y
send += "DTY|#{$game_player.y}|<->"
@oldy = $game_player.y
end
# Send the Direction if it is different then before
if User_Edit::Bandwith >= 2
if @oldd != $game_player.direction
send += "DTDIR|#{$game_player.direction}|<->"
@oldd = $game_player.direction
end
end
end
# Send everything that needs to be sended
@socket.send("#{send}") if send != ""
end
#--------------------------------------------------------------------------
# * Send HP
#--------------------------------------------------------------------------
def self.send_newhp
@socket.send("DTHP|#{$game_party.actors[0].hp}|#{$game_party.actors[0].maxhp}<->")
end
#--------------------------------------------------------------------------
# * Close Socket
#--------------------------------------------------------------------------
def self.close_socket
return if @socket == nil
# send_newstats
# send_personal_data
@socket.send("CLOSE|#{self.id}|<->")
@socket.close
@socket = nil
end
#--------------------------------------------------------------------------
# * Change Messgae of the Day
#--------------------------------------------------------------------------
def self.change_mod(newmsg)
@socket.send("<11>#{newmsg}</11>\n")
end
#--------------------------------------------------------------------------
# * Private Chat Send
#--------------------------------------------------------------------------
def self.pchat(id,mesg)
@pchat_conf = false
# Send the Private Chat Id (without close!!! \<13a>)
@socket.send("13a|#{id}|<->")
# Send Priavte Chat Message
@socket.send("13|#{mesg}|<->") #if @pchat_conf
#p "could not send #{mesg} to #{id}..." if not @pchat_conf
end
def self.parse(data,sep="|")
return data.split("|")
end
#--------------------------------------------------------------------------
# * Private Chat Send
#--------------------------------------------------------------------------
def self.mchat(id,mesg)
@mchat_conf = false
# Send the Chat Id (without close!!! \<21a>)
@socket.send("21a|#{id}|<->")
# Send Chat Message
@socket.send("21|#{mesg}|<->")
end
#--------------------------------------------------------------------------
# * Check if the user exists on the network..
#--------------------------------------------------------------------------
def self.user_exist?(username)
# Enable the test
# @user_test = true
# @user_exist = false
# Send the data for the test
# self.send_login(username.to_s,"*test*")
# Check for how to long to wait for data (Dependent on username size)
# if username.size <= 8
# Wait 1.5 seconds if username is less then 8
# for frame in 0..(1.5*40)
# self.update
# end
# elsif username.size > 8 and username.size <= 15
# Wait 2.3 seconds if username is less then 15
# for frame in 0..(2.3*40)
# self.update
# end
# elsif username.size > 15
# Wait 3 seconds if username is more then 15
# for frame in 0..(3*40)
# self.update
# end
# end
# Start Retreival Loop
# loop = 0
# loop do
# loop += 1
# self.update
## # Break if User Test was Finished
# break if @user_test == false
# # Break if loop meets 10000
# break if loop == 10000
# end
# If it failed, display message
# p User_Edit::USERTFAIL if loop == 10000
# # Return User exists if failed, or if it exists
# return true if @user_exist or loop == 10000
return false
end
def self.login_spc(username,pass)
# Enable the test
@user_test = false
@user_exist = false
# Send the data for the test
self.send_login(username.to_s,pass.to_s)
=begin
# Check for how to long to wait for data (Dependent on username size)
if username.length <= 8
# Wait 1.5 seconds if username is less then 8
for frame in 0..(1.5*40)
self.update
end
elsif username.length > 8 and username.size <= 15
# Wait 2.3 seconds if username is less then 15
for frame in 0..(2.3*40)
self.update
end
elsif username.length > 15
# Wait 3 seconds if username is more then 15
for frame in 0..(3*40)
self.update
end
end
=end
# Start Retreival Loop
loop = 0
loop do
loop += 1
self.update
# Break if User Test was Finished
break if @user_test == false
# Break if loop meets 10000
break if loop == 10000
end
# If it failed, display message
p $game_language.read_lang( 0,"USERTFAIL") if loop == 10000
# Return User exists if failed, or if it exists
return true if @user_exist or loop == 10000
return false
end
#--------------------------------------------------------------------------
# * Trade
#--------------------------------------------------------------------------
def self.trade(id,type,item,num=1,todo='gain')
# Gain is used for normal trade,
# Lose is used as Admin Command
# Check if you got item
case type
when 0
got = $game_party.item_number(item)
when 1
got = $game_party.weapon_number(item)
when 2
got = $game_party.armor_number(item)
end
# Print if not, return
p $game_language.read_lang( 0,"DONTGOTTRADE") + "(trade: kind = #{type} id = #{item})" if got == 0 and todo == 'gain'
return if got == 0 and todo == 'gain'
p $game_language.read_lang( 0,"NOTENOUGHTRADE") + "(trade: kind = #{type} id = #{item})" if got < num and todo == 'gain'
return if got < num and todo == 'gain'
@pchat_conf = false
# Send the Trade Id (without close!!! \<12a>)
@socket.send("12a|#{id}|<->")
@socket.send("12|type=#{type} item=#{item} num=#{num} todo=#{todo}|<->")
case type
when 0
$game_party.lose_item(item,num) if todo == 'gain'
when 1
$game_party.lose_weapon(item, num) if todo == 'gain'
when 2
$game_party.lose_armor(item, num) if todo == 'gain'
end
#else
# p "could not trade..."
#end
end
def self.send_result(id)
@socket.send("RSID|#{id}|<->")
@socket.send("RSEF|#{self.id}|<->")
end
def self.send_dead
send_newstats
send_personal_data
@socket.send("9|#{self.id}|<->")
#@socket.send("<5>@map_id = -1;</5>\n")
#@mapplayers = {}
end
#--------------------------------------------------------------------------
# * Update Net Players
#--------------------------------------------------------------------------
def self.update_net_player(id, data)
return if id.to_i == self.id.to_i
$game_temp.spriteset_refresh = true
# Turn Id in Hash into Netplayer (if not yet)
@players[id] ||= Game_NetPlayer.new
# Set the Global NetId if it is not Set yet
@players[id].do_id(id) if @players[id].netid == -1
# Refresh -> Change data to new data
@players[id].refresh(data)
# Return if the Id is Yourself
return if id.to_i == self.id.to_i
# Return if you are not yet on a Map
return if $game_map == nil
# If the Player is on the same map...
if @players[id].map_id == $game_map.map_id
# Update Map Players
self.update_map_player(id, data)
elsif @mapplayers[id] != nil
# Remove from Map Players
self.update_map_player(id, data, true)
end
end
#--------------------------------------------------------------------------
# * Update Map Players
#--------------------------------------------------------------------------
def self.update_map_player(id, data, kill=false)
# Return if the Id is Yourself
return if id.to_i == self.id.to_i
# If Kill (remove) is true...
if kill and @mapplayers[id] != nil
# Delete Map Player
@mapplayers.delete(id.to_i) rescue nil
if $scene.is_a?(Scene_Map)
$scene.spriteset.delete(id.to_i) rescue nil
end
$game_temp.spriteset_refresh = true
return
end
@mapplayers[id] ||= @players[id] if @players[id] != nil
# Turn Id in Hash into Netplayer (if not yet)
@mapplayers[id] ||= Game_NetPlayer.new
# Set the Global NetId if it is not Set yet
@mapplayers[id].netid = id if @mapplayers[id].netid == -1
# Refresh -> Change data to new data
@mapplayers[id].refresh(data)
self.send_newstats if @mapplayers[id] == nil
end
#--------------------------------------------------------------------------
# * Update Net Actors
#--------------------------------------------------------------------------
def self.update_net_actor(id,data,actor_id)
return
return if id.to_i == self.id.to_i
# Turn Id in Hash into Netplayer (if not yet)
@netactors[id] ||= Game_NetActor.new(actor_id)
# Set the Global NetId if it is not Set yet
@netactors[id].netid = id if @netactors[id].netid == -1
# Refresh -> Change data to new data
@netactors[id].refresh(data)
end
#--------------------------------------------------------------------------
# * Update
#--------------------------------------------------------------------------
def self.update
# self.update_socket_event
# self.update_supporto
# If Socket got lines, continue
return unless @socket.ready?
for line in @socket.recv(0xfff).split("<|>")
line.gsub!("\000") {""}
self.controllo_stringa(line) if line != ""
end
end
def self.do_work
return if @data_to_do.empty?
for line in 1...@data_to_do.size
@nooprec += 1 if @data_to_do[line].include?("\000\000\000\000")
return if @data_to_do[line].include?("\000\000\000\000")
if not @data_to_do[line].include?("ENDENDLINE")
p "#{@data_to_do[line]}" unless @data_to_do[line].include?("<5>") or @data_to_do[line].include?("<6>")or not $DEBUG or not User_Edit::PRINTLINES
# Set Used line to false
updatebool = false
# Update Ingame Protocol, if LogedIn and Map loaded
updatebool = self.update_ingame(@data_to_do[line]) if @login and $game_map != nil
# Update System Protocol, if command is not Ingame
updatebool = self.update_system(@data_to_do[line]) if updatebool == false
# Update Admin/Mod Protocol, if command is not System
updatebool = self.update_admmod(@data_to_do[line]) if updatebool == false
# Update Outgame Protocol, if command is not Admin/Mod
updatebool = self.update_outgame(@data_to_do[line]) if updatebool == false
if updatebool == false and $DEBUG
#p "Unused Line Recieved:"
#p line
end
if updatebool == true
@data_to_do[line] = "ENDENDLINE"
end
end
end
end
def self.controllo_stringa(str)
return if str.include?("\000")
# Set Used line to false
updatebool = false
# Update Ingame Protocol, if LogedIn and Map loaded
updatebool = self.update_ingame(str) if @login and $game_map != nil
# Update System Protocol, if command is not Ingame
updatebool = self.update_system(str) if updatebool == false
# Update Admin/Mod Protocol, if command is not System
updatebool = self.update_admmod(str) if updatebool == false
# Update Outgame Protocol, if command is not Admin/Mod
updatebool = self.update_outgame(str) if updatebool == false
if updatebool == false and $DEBUG
end
end
#--------------------------------------------------------------------------
# * Update Admin and Mod Command Recievals -> 18
#--------------------------------------------------------------------------
def self.update_admmod(line)
@stringa = self.parse(line)
case @stringa[0].to_s
# Admin Command Recieval
when "018" #/<18>(.*)<\/18>/
# Kick All Command
if @stringa[1].to_s == "kick_all"
p $game_language.read_lang( 0,"ADMKICKALL")
self.close_socket
$scene = nil
return true
# Kick Command
elsif @stringa[1].to_s == "kicked"
p $game_language.read_lang( 0,"ADMKICK")
self.close_socket
$scene = nil
return true
end
return false
end
return false
end
#--------------------------------------------------------------------------
# * Update all Not-Ingame Protocol Parts -> 0, login, reges
#--------------------------------------------------------------------------
def self.update_outgame(line)
@stringa = self.parse(line)
case @stringa[0].to_s
when "PGD"# /<pgd>(.*);(.*)<\/pgd>/
case @stringa[1]
when "CHR"
$game_party.actors[0].set_chara(@stringa[2].to_s)
$game_player.character_name = @stringa[2].to_s
when "HP"
$game_party.actors[0].hp = @stringa[2].to_i
when "SP"
$game_party.actors[0].sp = @stringa[2].to_i
when "LV"
$game_party.actors[0].level = @stringa[2].to_i
when "MVS"
$game_player.move_speed = @stringa[2].to_i
when "NM"
$game_party.actors[0].name = @stringa[2].to_s
when "DIR"
$game_player.direction = @stringa[2].to_i
end
$game_player.refresh
return true
when "PGP"#/<pgpos>(.*);(.*);(.*)<\/pgpos>/
if $scene.is_a?(Scene_Map)
$game_temp.player_new_map_id = @stringa[1].to_i
$game_temp.player_new_x = @stringa[2].to_i
$game_temp.player_new_y = @stringa[3].to_i
$game_temp.player_new_direction = $game_player.direction
$scene.transfer_player
end
return true
# Server Authenfication
when "000" #/<0 (.*)>(.*) n=(.*)<\/0>/
a = self.authenficate(@stringa[1],@stringa[2])
@servername = @stringa[3].to_s
return true if a
# Registration Conformation
when "REG"#/<reges>(.*)<\/reges>/
@user_test = false
if @stringa[1] == "'OK'"
@registered = true
@status = $game_language.read_lang( 0,"REGISTERED")
text = [@status]
$scene.set_status(@status) if $scene.is_a?(Scene_Login)
else
@registered = false
@status = $game_language.read_lang( 0,"REGISTER_DENIED")
text = [@status]
$scene.set_status(@status) if $scene.is_a?(Scene_Login)
end
return true
# Login, With User Test
when "LOG" #/<login>(.*)<\/login>/
@user_test = false
if @user_test == false
# When Log in Succeeded, and not User Test...
if @stringa[1] == "allow" and not @user_test
# Login and Status to Logged-in
@login = true
@status = $game_language.read_lang( 0,"NETWORK_LOGOK")
text = [@status]
$scene.set_status(@status) if $scene.is_a?(Scene_Login)
# Get Name (By Server)
self.get_name
# Start Loop for Retrieval
loop = 0
loop do
self.update
loop += 1
# Break if loop reaches 10000
break if loop == 10000
# Break if Name and ID are recieved
break if self.name != "" and self.name != nil and self.id != -1
end
self.get_group
# Goto Scene Title
@name = $scene.username if $scene.is_a?(Scene_Login)
$scene = Scene_Character.new
return true
# When Wrong Username and not User Test
elsif @stringa[1] == "wu" and not @user_test
# Set status to Incorrect Username
$scene.set_status($game_language.read_lang( 0,"NETWORK_USERNAMEERROR")) if $scene.is_a?(Scene_Login)
return true
# When Wrong Password and not User Test
elsif @stringa[1] == "wp" and not @user_test
# Set status to Incorrect Passowrd
$scene.set_status($game_language.read_lang( 0,"NETWORK_PASSERROR}")) if $scene.is_a?(Scene_Login)
return true
# When Other Command, and Not User test
elsif not @user_test
# ERROR!
p $game_language.read_lang( 0,"NETWORK_ERRORUNkNOWN1")
return true
end
# If it IS a user test...
else
@user_exist = false
#...and wrong user -> does not exist
if @stringa[1] == "wu"
# User does not Exist
@user_exist = false
@user_test = false
#...and wrong pass -> does exist
elsif @stringa[1] == "wp"
# User Does Exist
@user_exist = true
@user_test = false
end
return true
end
return false
end
return false
end
def self.partyrequest(netid)
@socket.send("PARTYREQUEST|#{netid}|<->")
end
def self.guildrequest(netid)
@socket.send("GUILDREQUEST|#{netid}|<->")
end
def self.guildrequestbyname(name)
@socket.send("GLDRN|#{name}|<->")
end
#--------------------------------------------------------------------------
# * Update System Protocol Parts -> ver, mod, 1, 2, 3, 10
#--------------------------------------------------------------------------
def self.update_system(line)
@stringa = self.parse(line)
case @stringa[0].to_s
when "IPB" #/<ipbanned>(.*)<\/ipbanned>/
p $game_language.read_lang( 0,"NETWORK_BANIP")
return true
# Version Recieval
when "VER" #/<ver>(.*)<\/ver>/
$game_temp.msg = $game_language.read_lang( 0,"NETWORK_ERRORVERSIONUNKNOWN") if @stringa[1].to_s == nil
if "#{User_Edit::VERSION}" != "#{@stringa[1]}"
p $game_language.read_lang( 0,"NETWORK_ERRORVERSIONUNKNOWN")
begin
# Close Connection
@socket.close rescue @socket = nil
end
exit
else
end
@version = @stringa[1].to_s
return true
# Message Of the Day Recieval
when "MOD" #/<mod>(.*)<\/mod>/
$game_temp.motd = @stringa[1].to_s
if $scene.is_a?(Scene_Login)
$scene.update_motd
end
return true
# User ID Recieval (Session Based)
when "001"#/<1>(.*)<\/1>/
@id = @stringa[1].to_s
return true
# User Name Recieval
when "002"#/<2>(.*)<\/2>/
@name = @stringa[1].to_s
return true
# Group Recieval
when "003"#/<3>(.*)<\/3>/
case @stringa[1].to_s
when "0"
@group = "standard"
when "1"
@group = "moderator"
when "2"
@group = "admin"
end
return true
when "CHK"#/<check>(.*)<\/check>/
case @stringa[1].to_s
when "0"
@group = "standard"
when "1"
@group = "moderator"
when "2"
@group = "admin"
end
return true
when "0SW" #/<SW>(.*);(.*)<\/SW>/
if @stringa[2].to_i == 0
$game_switches[@stringa[1].to_i] = false
end
if @stringa[2].to_i == 1
$game_switches[@stringa[1].to_i] = true
end
return true
when "0VR" #/<VR>(.*);(.*)<\/VR>/
$game_variables[@stringa[1].to_i] = @stringa[2].to_i
$game_map.need_refresh = true
return true
when "MP0"#/<mapheader>(.*)<\/mapheader>/
if @stringa[1].to_s == "ON"
@mapheader = true
end
return true
# Respawn Enemy
when "RSP"#/<respawn>(.*);(.*)<\/respawn>/
self.respawn_event(@stringa[1].to_i,@stringa[2].to_i)
return true
# Kill Enemy
when "KLE" #/<killev>(.*)<\/killev>/
$game_map.need_refresh = true
$game_map.update
self.uccidi_event($game_map.map_id,@stringa[1].to_i)
return true
# New Mail
when "MLE"#/<mail>(.*),(.*),(.*)<\/mail>/
self.mail(@stringa[2].to_s,@stringa[1].to_s,@stringa[3])
return true
# Weather Update
when "WHT"#/<wht>(.*);(.*);(.*)<\/wht>/
$game_screen.weather(@stringa[1].to_i,@stringa[2].to_i,@stringa[3].to_i)
return true
# System Update
when "010"#/<10>(.*)<\/10>/
eval(@stringa[1].to_s)
$game_map.need_refresh = true
$scene.update if not $scene.is_a?(Scene_Reinit)
$game_map.update
return true
# Player information Processing
when "PG1"#/<PGD>(.*)<\/PGD>/
eval(@stringa[1])
$game_player.refresh
return true
# Party Info
when "PY0"#/<addtoparty>(.*);(.*)<\/addtoparty>/
return true if @players[@stringa[2]] == nil
if $game_temp.party_name != "" and @players[@stringa[2]].netid != -1
if @players[@stringa[2]].netid != @id
@message_party = $game_language.read_lang( 0,"NETWORK_SERVER") + "#{@stringa[1]}" + $game_language.read_lang( 0,"NETWORK_SERVER_INPARTY}")
$game_netparty.actors.push(@players[@stringa[2]]) if !$game_netparty.actors.include?(@players[@stringa[2]])
$game_temp.chat_log.push(@message_party)
$game_temp.map_chat_log.push(@message_party)
$game_temp.party_chat_log.push(@message_party)
$game_temp.gilda_chat_log.push(@message_party)
end
end
return true
when "PY1"#/<party>(.*)<\/party>/
@already = 0
@accept = 1
@decline = 2
@take_out = 3
case @stringa[1].to_i
when @already
@message_party = $game_language.read_lang( 0,"NETWORK_SERVER_ALREADYINPARTY")
when @accept
@message_party = $game_language.read_lang( 0,"NETWORK_SERVER_ACCEPTED")
when @decline
@message_party = $game_language.read_lang( 0,"NETWORK_SERVER_NOTACCEPTED")
when @take_out
@message_party = $game_language.read_lang( 0,"NETWORK_SERVER_QUITPARTY")
end
$game_temp.chat_log.push(@message_party)
$game_temp.map_chat_log.push(@message_party)
$game_temp.party_chat_log.push(@message_party)
$game_temp.gilda_chat_log.push(@message_party)
return true
when "PY2"#/<partyexp>(.*)<\/partyexp>/
actor = $game_party.actors[0]
if actor.cant_get_exp? == false
last_level = actor.level
actor.exp += @stringa[1].to_i
$game_player.show_demage("#{@stringa[1]}" + $game_language.read_lang( 0,"NETWORK_PARTYEXP"),false)
$game_player.animation_id = 105
if actor.level > last_level
actor.hp = actor.maxhp
actor.sp = actor.maxsp
end
end
Network::Main.send_newstat
return true
when "PY3"#/<partyrequest>(.*);(.*)<\/partyrequest>/
if $scene.is_a?(Scene_Map)
if @stringa[2] != ""
$scene.call_pop(1,"#{@stringa[1]}" + $game_language.read_lang( 0,"NETWORK_WANTINPARTYGO1") + "#{@stringa[2]}")
$party_name_r = "#{@stringa[2]}"
else
$scene.call_pop(1,"#{@stringa[1]}" + $game_language.read_lang( 0,"NETWORK_WANTINPARTYGO2"))
end
end
return true
# Guild Info
when "GL0"#/<guild>(.*)<\/guild>/
@already = 0
@accept = 1
@decline = 2
@take_out = 3
case @stringa[1].to_i
when @already
@message_party = $game_language.read_lang( 0,"NETWORK_SERVER_ALREADYINGUILD")
when @accept
@message_party = $game_language.read_lang( 0,"NETWORK_SERVER_ACCEPTGUILD")
when @decline
@message_party = $game_language.read_lang( 0,"NETWORK_SERVER_NOTACCEPTEDGUILD")
when @take_out
@message_party = $game_language.read_lang( 0,"NETWORK_SERVER_QUITGUILD")
end
$game_temp.chat_log.push(@message_party)
$game_temp.map_chat_log.push(@message_party)
$game_temp.party_chat_log.push(@message_party)
$game_temp.gilda_chat_log.push(@message_party)
return true
when "GL1"#/<guildrequest>(.*);(.*)<\/guildrequest>/
if $scene.is_a?(Scene_Map)
if @stringa[2] != ""
$scene.call_pop(2,"#{@stringa[1]}" + $game_language.read_lang( 0,"NETWORK_WANTINGUILDGO1") + "#{@stringa[2]}")
$guild_name_r = "#{@stringa[2]}"
else
$scene.call_pop(2,"#{@stringa[1]}" + $game_language.read_lang( 0,"NETWORK_WANTINGUILDGO2}"))
end
end
return true
when "EHT"#/<ehit>(.*);(.*);(.*);(.*);(.*);<\/ehit>/
if $scene.is_a?(Scene_Map) and $ABS != nil
$ABS.hit_enemy_bynet(@stringa[1].to_i,@stringa[2].to_i,@stringa[3].to_i,@stringa[4].to_i) if @stringa[5].to_i != self.id.to_i
end
return true
# Server Msg
when "SVR"#/<servermsg>(.*)<\/servermsg>/
$game_temp.chat_log.push($game_language.read_lang( 0,"NETWORK_SERVER") + @stringa[1].to_s)
$game_temp.map_chat_log.push($game_language.read_lang( 0,"NETWORK_SERVER") + @stringa[1].to_s)
$game_temp.party_chat_log.push($game_language.read_lang( 0,"NETWORK_SERVER") + @stringa[1].to_s)
$game_temp.gilda_chat_log.push($game_language.read_lang( 0,"NETWORK_SERVER") + @stringa[1].to_s)
return true
# Account From Server
when "NC0"#/<charexist>/
p $game_language.read_lang( 0,"NETWORK_NAMEALREADYUSED")
return true
# CHANGE
when "NC1"#/<nch>(.*)<\/nch>/
@id_a = @stringa[1].to_i
$game_system.save_data = [
"???", # Nome 0
0, # HP 1
0, # SP 2
0, # EXP 3
0, # LEVEL 4
0, # CLASS 5
0, # MAP 6
0, # WPN 7
0, # ARMOR 1 8
0, # ARMOR 2 9
0, # ARMOR 3 10
0, # ARMOR 4 11
0, # X 12
0, # Y 13
0, # DIR 14
"", # CHARACTER_NAME 15
0, # CHARACTER_HUE 16
"", # GUILD_NAME 17
0, # STR 18
0, # INT 19
0, # DEX 20
0, # AGI 21
]
if @id_a.to_i == 1
$scene.create_iconA(1,20,50 + 50,$game_system.save_data)
elsif @id_a.to_i == 2
$scene.create_iconB(2,20,130 + 50,$game_system.save_data)
elsif @id_a.to_i == 3
$scene.create_iconC(3,20,210 + 50,$game_system.save_data)
end
return true
# CHANGE
when "AC0"
$game_system.save_data = [
@stringa[1].to_s, # Nome 0
@stringa[2].to_i, # HP 1
@stringa[3].to_i, # SP 2
@stringa[4].to_i, # EXP 3
@stringa[5].to_i, # LEVEL 4
@stringa[6].to_i, # CLASS 5
@stringa[7].to_i, # MAP 6
@stringa[8].to_i, # WPN 7
@stringa[9].to_i, # ARMOR 1 8
@stringa[10].to_i, # ARMOR 2 9
@stringa[11].to_i, # ARMOR 3 10
@stringa[12].to_i, # ARMOR 4 11
@stringa[13].to_i, # X 12
@stringa[14].to_i, # Y 13
@stringa[15].to_i, # DIR 14
@stringa[16].to_s, # CHARACTER_NAME 15
@stringa[17].to_i, # CHARACTER_HUE 16
@stringa[19].to_s, # GUILD_NAME 17
@stringa[20].to_i, # STR 18
@stringa[21].to_i, # INT 19
@stringa[22].to_i, # DEX 20
@stringa[23].to_s, # AGI 21
]
@id_a = @stringa[18].to_i
@pmdata = @stringa[24].split("<NR>")
@pm_from = @pmdata[0]
@pm_to = @pmdata[1]
@pm_msg = @pmdata[2]
$game_system.save_data.push(@pm_from,@pm_to,@pm_msg)
if @id_a.to_i == 1
$scene.create_iconA(1,20,50 + 50,$game_system.save_data)
elsif @id_a.to_i == 2
$scene.create_iconB(2,20,130 + 50,$game_system.save_data)
elsif @id_a.to_i == 3
$scene.create_iconC(3,20,210 + 50,$game_system.save_data)
end
return true
# ------------------------------------------------------------------------------------------
# Account Skills/Gold/Items/Armors/Weapons
# ------------------------------------------------------------------------------------------
when "AC1"#/<account_skill>(.*)<\/account_skill>/
@wpn_ids = []
for id in @stringa[1].split(",")
@wpn_ids.push(id.to_i) if id != ""
end
if @wpn_ids.size > 0 then
for i in 0...@wpn_ids.size
$game_party.actors[0].learn_skill(@wpn_ids[i])
end
end
when "AC2"#/<account_gold>(.*)<\/account_gold>/
$game_party.gain_gold(@stringa[1].to_i)
return true
when "AC3"#/<account_wpn>(.*)\/(.*)<\/account_wpn>/
@wpn_ids = []
@wpn_qns = []
@qnt = @stringa[2].to_s
for id in @stringa[1].split(",")
@wpn_ids.push(id.to_i) if id != ""
end
for qn in @qnt.split(",")
@wpn_qns.push(qn.to_i) if qn != ""
end
if @wpn_qns.size > 0 then
for i in 0...@wpn_qns.size
$game_party.gain_weapon(@wpn_ids[i],@wpn_qns[i])
end
end
return true
when "AC4"#/<account_items>(.*)\/(.*)<\/account_items>/
@wpn_ids = []
@wpn_qns = []
@qnt = @stringa[2].to_s
for id in @stringa[1].split(",")
@wpn_ids.push(id.to_i) if id != ""
end
for qn in @qnt.split(",")
@wpn_qns.push(qn.to_i) if qn != ""
end
if @wpn_qns.size > 0 then
for i in 0...@wpn_qns.size
$game_party.gain_item(@wpn_ids[i],@wpn_qns[i])
end
end
return true
when "AC5" #/<account_armors>(.*)\/(.*)<\/account_armors>/
@wpn_ids = []
@wpn_qns = []
@qnt = @stringa[2].to_s
for id in @stringa[1].split(",")
@wpn_ids.push(id.to_i) if id != ""
end
for qn in @qnt.split(",")
@wpn_qns.push(qn.to_i) if qn != ""
end
if @wpn_qns.size > 0 then
for i in 0...@wpn_qns.size
$game_party.gain_armor(@wpn_ids[i],@wpn_qns[i])
end
end
return true
# ------------------------------------------------------------------------------------------
# Event Move
when "0EV"#/<ev (.*)>(.*)<\/ev>/
if $game_map != nil
if $game_map.map_id == @stringa[1].to_i
eval(@stringa[2])
end
end
return true
# Event Move Find
when "1EV" #/<ev2 (.*)>(.*);(.*);(.*)<\/ev>/
if $game_map != nil
if $game_map.map_id == @stringa[1].to_i
$game_map.events[@stringa[2].to_i].find_path_online(@stringa[3].to_i,@stringa[4].to_i)
end
end
return true
# Event Posisition
when "3EV"#/<evdata>(.*);(.*);(.*);(.*);(.*)<\/evdata>/
if $game_map != nil
if @stringa[5].to_i == 1
uccidi_event(@stringa[1].to_i,@stringa[2].to_i)
end
if $game_map.map_id == @stringa[1].to_i
$game_map.events[@stringa[2].to_i].moveto(@stringa[3].to_i,@stringa[4].to_i)
end
end
return true
when "023"#/<23>(.*)<\/23>/
eval(@stringa[1])
key = []
key.push(@self_key1)
key.push(@self_key2)
key.push(@self_key3)
$game_self_switches[key] = @self_value
@self_key1 = nil
@self_key2 = nil
@self_key3 = nil
@self_value = nil
$game_map.need_refresh = true
key = []
return true
when "ST0"#/<state>(.*)<\/state>/
$game_party.actors[0].refresh_states(@stringa[1])
return true
# Attacked!
when "AE0"#/<attack_effect>dam=(.*) ani=(.*) id=(.*) target=(.*)<\/attack_effect>/
@to_erase = @stringa[1].to_i
$game_party.actors[0].hp -= @to_erase
self.send_newstats
if $game_party.actors[0].hp <= 0 or $game_party.actors[0].dead?
$game_party.actors[0].hp = $game_party.actors[0].maxhp
self.send_result(@stringa[3].to_i)
self.send_dead
$scene = Scene_Gameover.new
end
return true
# Killed
when "RSE"#/<result_effect>(.*)<\/result_effect>/
$game_player.show_demage("Vittoria!",false)
return true
when "SHW"#/<show_message>(.*);(.*)<\/show_message>/
if @stringa[1].to_i == @id.to_i
$game_player.show_text(@stringa[2].to_s,$game_player.chat_color.to_i)
else
if @players[@stringa[1]].map_id == $game_map.map_id
@players[@stringa[1]].show_text(@stringa[2].to_s,@players[@stringa[1]].chat_color.to_i)
end
end
return true
when "SHD"#/<show_damage>(.*);(.*)<\/show_damage>/
if @stringa[1].to_i == @id.to_i
$game_player.show_demage(@stringa[2].to_s,false)
else
if @players[@stringa[1]] != nil and @players[@stringa[1]].map_id == $game_map.map_id
@players[@stringa[1]].show_demage(@stringa[2].to_s,false)
end
end
return true
when "SHA"#/<show_anim>(.*);(.*)<\/show_anim>/
if @stringa[1].to_i == @id.to_i
$game_player.animation_id = @stringa[2].to_i
else
if @players[@stringa[1]] != nil and @players[@stringa[1]].map_id == $game_map.map_id
@players[@stringa[1]].animation_id = @stringa[2].to_i
end
end
return true
when "DMG" #/<dmg_pg>(.*);(.*)<\/dmg_pg>/
if @stringa[1].to_i == @id.to_i
$game_player.show_demage(@stringa[2],false)
$game_party.actors[0].hp -= @stringa[2].to_i
self.send_newstats
if $game_party.actors[0].hp <= 0 or $game_party.actors[0].dead?
$game_party.actors[0].hp = $game_party.actors[0].maxhp
self.send_result(@stringa[3].to_i)
self.send_dead
$scene = Scene_Gameover.new
end
else
if @players[@stringa[1]].map_id == $game_map.map_id
@players[@stringa[1]].show_demage(@stringa[2],false)
end
end
return true
end
return false
end
#--------------------------------------------------------------------------
# * Update Ingame Parts -> player, 5, 6, 9, 12, 13
#--------------------------------------------------------------------------
def self.update_ingame(line)
@stringa = self.parse(line)
case @stringa[0].to_s
# Player information Processing
when "PID" #/<player id=(.*)>(.*)<\/player>/
self.update_net_player(@stringa[1], @stringa[2])
return true
# Player Processing
when "005"#/<5 (.*)>(.*)<\/5>/
# Update Player
self.update_net_player(@stringa[1], @stringa[2])
# If it is first time connected...
if @stringa[2].include?("start")
# ... and it is not yourself ...
if @stringa[1].to_i != self.id.to_i
# ... and it is on the same map...
if @players[@stringa[1]].map_id == $game_map.map_id
# ... Return the Requested Information
self.send_start_request(@stringa[1].to_i)
$game_temp.spriteset_refresh = true
end
end
end
return true
# Map PLayer Processing
when "006"#/<6 (.*)>(.*)<\/6>/
# Return if it is yourself
return true if @stringa[1].to_i == self.id.to_i
# Update Map Player
#self.update_map_player(@stringa[1], @stringa[2])
self.update_net_player(@stringa[1], @stringa[2])
# If it is first time connected...
if @stringa[2].include?("start") or @stringa[2].include?("map")
# ... and it is not yourself ...
if @stringa[1].to_i != self.id.to_i
# ... and it is on the same map...
if @players[@stringa[1]].map_id == $game_map.map_id
# ... Return the Requested Information
self.send_start_request(@stringa[1].to_i)
$game_temp.spriteset_refresh = true
end
end
end
return true
when "06A"#/<6a>(.*)<\/6a>/
@send_conf = true
return true if @stringa[1].to_s == "'Confirmed'"
# Chat Recieval
when "CH0"#/<chat>(.*)<\/chat>/
$game_temp.chat_log.push(@stringa[1].to_s)
$game_temp.chat_refresh = true
return true
when "CH1"#/<pchat>(.*)<\/pchat>/
$game_temp.party_chat_log.push(@stringa[1].to_s)
$game_temp.chat_refresh = true
return true
when "CH2"#/<gchat>(.*)<\/gchat>/
$game_temp.guild_chat_log.push(@stringa[1].to_s)
$game_temp.chat_refresh = true
return true
# Remove Player ( Disconnected )
when "009"#/<9>(.*)<\/9>/
# Destroy Netplayer and MapPlayer things
self.destroy(@stringa[1].to_i)
# Redraw Mapplayer Sprites
$game_temp.spriteset_refresh = true
$game_temp.spriteset_renew = true
return true
when "LVM"#/<lvm>(.*)<\/lvm>/
# Destroy MapPlayer things
return if @stringa[1].to_i == self.id
self.leave_map(@stringa[1].to_i)
# Redraw Mapplayer Sprites
$game_temp.spriteset_refresh = true
$game_temp.spriteset_renew = true
return true
when "012"#/<12>type=(.*) item=(.*) num=(.*) todo=(.*)<\/12>/
case @stringa[1].to_i
when 0
$game_party.gain_item(@stringa[2].to_i, @stringa[3].to_i) if @stringa[4] == 'gain'
$game_party.lose_item(@stringa[2].to_i, @stringa[3].to_i) if @stringa[4] == 'lose'
when 1
$game_party.gain_weapon(@stringa[2].to_i, @stringa[3].to_i) if @stringa[4] == 'gain'
$game_party.lose_weapon(@stringa[2].to_i, @stringa[3].to_i) if @stringa[4] == 'lose'
when 2
$game_party.gain_armor(@stringa[2].to_i, @stringa[3].to_i) if @stringa[4] == 'gain'
$game_party.lose_weapon(@stringa[2].to_i, @stringa[3].to_i) if @stringa[4] == 'lose'
end
$game_temp.refresh_itemtrade = true
return true
when "A12"#/<12a>(.*)<\/12a>/
@trade_conf = true
return true if @stringa[1].to_s == "'Confirmed'"
# Private Chat Incomming Message
when "013"#/<13 (.*)>(.*)<\/13>/
got = false
id = false
for chat in 0..$game_temp.pchat_log.size
if $game_temp.lastpchatid[chat].to_i == @stringa[1].to_i
got = true
id = chat
end
end
if got
$game_temp.pchat_log[id].push(@stringa[2])
$game_temp.lastpchatid[id] = @stringa[1].to_i
$game_temp.pchat_refresh[id] = true
else
id = $game_temp.pchat_log.size
$game_temp.pchat_log[id] = []
$game_temp.pchat_log[id].push(@stringa[2])
$game_temp.lastpchatid[id] = -1
$game_temp.lastpchatid[id] = @stringa[1].to_i
$game_temp.pchat_refresh[id] = false
$game_temp.pchat_refresh[id] = true
end
return true
# Private Chat ID confirmation
when "A13"#/<13a>(.*)<\/13a>/
@pchat_conf = true
return true if @stringa[1].to_s == "'Confirmed'"
# Private Chat ID confirmation
when "21A"#/<21a>(.*)<\/21a>/
@mchat_conf = true
return true if @stringa[1].to_s == "'Confirmed'"
#Map Chat Recieval
when "021"#/<21>(.*)<\/21>/
$game_temp.map_chat_log.push(@stringa[1].to_s)
$game_temp.chat_refresh = true
return true
when "A22"#/<22a>(.*)<\/22a>/
if @stringa[1] != "Compelete"
@pm_lines.push("#{@stringa[1]}")
elsif @stringa[1] == "Compelete"
update_pms(@pm_lines)
@pm_lines = []
end
return true
when "A24"#/<24a>(.*)<\/24a>/
@trading = true
return true
when "C24"#/<24c>(.*)<\/24c>/
eval(@stringa[1])
$scene = Scene_Trade.new(@trade_id)
self.trade_add(-1,-1,@trade_id)
@trade_id = -1
return true
when "D24"#/<24d>(.*)<\/24d>/
trade_exit = -1
eval(@stringa[1])
$game_temp.trade_window.dispose
$game_temp.trade_window = nil
trade_exit = -1
return true
when "A25"#/<25a>(.*)<\/25a>/
i_kind = -1
i_id = -1
id = -1
eval(@stringa[1])
# p id#$game_temp.items2[id], $game_temp.weapons2[id], $game_temp.armors2[id]
if i_id < 0 and i_kind < 0
$game_temp.trade_window.dispose if $game_temp.trade_window != nil
$game_temp.trade_window = nil
$game_temp.trade_window = Trade_List.new(id)
$game_temp.trade_window.refresh
@trade_compelete = true
@trading = false
end
$game_temp.items2[id] = {} if $game_temp.items2[id] == nil
$game_temp.weapons2[id] = {} if $game_temp.weapons2[id] == nil
$game_temp.armors2[id] = {} if $game_temp.armors2[id] == nil
return if i_id < 0
case i_kind
when 0
if $game_temp.items2[id][i_id] == nil
$game_temp.items2[id][i_id] = 1
else
$game_temp.items2[id][i_id] += 1
end
when 1
if $game_temp.weapons2[id][i_id] == nil
$game_temp.weapons2[id][i_id] = 1
else
$game_temp.weapons2[id][i_id] += 1
end
when 2
if $game_temp.armors2[id][i_id] == nil
$game_temp.armors2[id][i_id] = 1
else
$game_temp.armors2[id][i_id] += 1
end
end
$game_temp.trade_refresh = true
return true
when "B25"#/<25b>(.*)<\/25b>/
i_kind = -1
i_id = -1
id = -1
eval(@stringa[1])
$game_temp.items2[id] = {} if $game_temp.items2[id] == nil
$game_temp.weapons2[id] = {} if $game_temp.weapons2[id] == nil
$game_temp.armors2[id] = {} if $game_temp.armors2[id] == nil
case i_kind
when 0
if $game_temp.items2[id][i_id] > 1
$game_temp.items2[id][i_id] -= 1
else
$game_temp.items2[id].delete(i_id)
end
when 1
if $game_temp.weapons2[id][i_id] > 1
$game_temp.weapons2[id][i_id] -= 1
else
$game_temp.weapons2[id].delete(i_id)
end
when 2
if $game_temp.armors2[id][i_id] > 1
$game_temp.armors2[id][i_id] -= 1
else
$game_temp.armors2[id].delete(i_id)
end
end
$game_temp.trade_refresh = true
return true
when "D25"#/<25d>(.*)<\/25d>/
$game_temp.trade_accepting = true
return true
when "E25"#/<25e>(.*)<\/25e>/ #Accept
$game_temp.trade_accepting = false
$game_temp.start_trade = false
$game_temp.trade_now = true
return true
when "F25"#/<25f>(.*)<\/25f>/ #Decline
$game_temp.trade_accepting = false
$game_temp.start_trade = false
return true
end
return false
end
#--------------------------------------------------------------------------
# * Authenficate <0>
#--------------------------------------------------------------------------
def self.authenficate(id,echo)
if echo == "'e'"
# If Echo was returned, Authenficated
@auth = true
@id = id
return true
end
if echo == "'n'"
p $game_language.read_lang( 0,"NETWORK_ERRORAUTHERROR")
return false
end
return false
end
#--------------------------------------------------------------------------
# * Calls a player to trade
#--------------------------------------------------------------------------
def self.trade_call(id)
@trade_compelete = false
@trading = true
@socket.send("24|#{id}|<->")
ids = self.id
@socket.send("24c|@trade_id = #{ids}|<->")
end
#--------------------------------------------------------------------------
# * Exit trade.
#--------------------------------------------------------------------------
def self.trade_exit(id)
@socket.send("24|#{id}|<->")
ids = self.id
@socket.send("24d|trade_exit = #{ids}|<->")
end
#--------------------------------------------------------------------------
# * Checks if the trade is compelete
#--------------------------------------------------------------------------
def self.trade_compelete
return @trade_compelete
end
#--------------------------------------------------------------------------
# * Checks the trade result
#--------------------------------------------------------------------------
def self.trading
return @trading
end
#--------------------------------------------------------------------------
# * Send Move Update
#--------------------------------------------------------------------------
def self.trade_add(kind,item_id,netid)
@socket.send("25|#{netid}|<->")
me = self.id
@socket.send("25a|i_kind = #{kind}; i_id = #{item_id}; id = #{me}|<->")
end
#--------------------------------------------------------------------------
# * Send Move Update
#--------------------------------------------------------------------------
def self.trade_add(kind,item_id,netid)
@socket.send("25|#{netid}|<->")
me = self.id
@socket.send("25a|i_kind = #{kind}; i_id = #{item_id}; id = #{me}|<->")
end
#--------------------------------------------------------------------------
# * Send Move Update
#--------------------------------------------------------------------------
def self.trade_remove(kind,item_id,netid)
@socket.send("25|#{netid}|<->")
me = self.id
@socket.send("25b|i_kind = #{kind}; i_id = #{item_id}; id = #{me}|<->")
end
#--------------------------------------------------------------------------
# * Send Trade REquest
#--------------------------------------------------------------------------
def self.trade_request(netid)
@socket.send("25|#{netid}|<->")
me = self.id
@socket.send("25d|id = #{me}|<->")
end
#--------------------------------------------------------------------------
# * Send accept trade
#--------------------------------------------------------------------------
def self.trade_accept(netid)
@socket.send("25|#{netid}|<->")
me = self.id
@socket.send("25e|id = #{me};|<->")
end
#--------------------------------------------------------------------------
# * Send cancel trade
#--------------------------------------------------------------------------
def self.trade_cancel(netid)
@socket.send("25|#{netid}|<->")
me = self.id
@socket.send("25f|id = #{me};|<->")
end
def self.save_pg
return self.save_server_pg
end
end
#-------------------------------------------------------------------------------
# End Class
#-------------------------------------------------------------------------------
class Base
#--------------------------------------------------------------------------
# * Updates Default Classes
#--------------------------------------------------------------------------
def self.update
# Update Input
Input.update
# Update Graphics
Graphics.update
# Update Mouse
$mouse.update
# Update Main (if Connected)
Main.update if Main.socket != nil
end
end
#-------------------------------------------------------------------------------
# End Class
#-------------------------------------------------------------------------------
class Test
attr_accessor :socket
#--------------------------------------------------------------------------
# * Returns Testing Status
#--------------------------------------------------------------------------
def self.testing
return true if @testing
return false
end
#--------------------------------------------------------------------------
# * Tests Connection
#--------------------------------------------------------------------------
def self.test_connection(host, port)
# We are Testing, not Complted the Test
@testing = true
@complete = false
# Start Connection
@socket = TCPSocket.new(host, port)
if not @complete
# If the Test Succeeded (did not encounter errors...)
self.test_result(false)
@socket.send("20|'Test Completed'|<->")
begin
# Close Connection
@socket.close rescue @socket = nil
end
end
end
#--------------------------------------------------------------------------
# * Set Test Result
#--------------------------------------------------------------------------
def self.test_result(value)
# Set Result to value, and Complete Test
@result = value
@complete = true
end
#--------------------------------------------------------------------------
# * Returns Test Completed Status
#--------------------------------------------------------------------------
def self.testcompleted
return @complete
end
#--------------------------------------------------------------------------
# * Resets Test
#--------------------------------------------------------------------------
def self.testreset
# Reset all Values
@complete = false
@socket = nil
@result = nil
@testing = false
end
#--------------------------------------------------------------------------
# * Returns Result
#--------------------------------------------------------------------------
def self.result
return @result
end
end
#-------------------------------------------------------------------------------
# End Class
#-------------------------------------------------------------------------------
#--------------------------------------------------------------------------
# * Module Update
#--------------------------------------------------------------------------
def self.update
end
end
#-------------------------------------------------------------------------------
# End Module
#-------------------------------------------------------------------------------
end
#-------------------------------------------------------------------------------
# End SDK Enabled Test
#-------------------------------------------------------------------------------
end