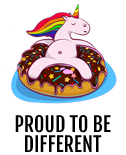
self.contents = Bitmap.new(width - 32, height - 32)
self.contents = Bitmap.new(width - 32, @item_max * 32)
def update_status
# If B button was pressed
if Input.trigger?(Input::B)
# Play cancel SE
$game_system.se_play($data_system.cancel_se)
# Make command window active
@player_menu.active = true
@status_window.active = false
@status_window.visible = false
return
end
# If C button was pressed
if Input.trigger?(Input::A) or Input.trigger?(Input::C)
# Branch by command window cursor position
case @player_menu.index
when 0 # status
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to status screen
$scene = Scene_Status.new(@status_window.index)
when 1 # equipment
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to equipment screen
$scene = Scene_Equip.new(@status_window.index)
when 2 # skill
# If this actor's action limit is 2 or more
if $game_party.actors[@status_window.index].restriction >= 2
# Play buzzer SE
$game_system.se_play($data_system.buzzer_se)
return
end
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to skill screen
$scene = Scene_Skill.new(@status_window.index)
end
end
if @status_window.index <= 2
@status_window.oy = 0
end
if @status_window.index > 2 and @status_window.index <=5
@status_window.oy = (120 * 3)
end
if @status_window.index > 5 and @status_window.index <=8
@status_window.oy = (120 * 6)
end
if @status_window.index > 8 and @status_window.index <=11
@status_window.oy = (120 * 9)
end
end
class Window_MenuStatus
def initialize
super(0, 0, 480, (12*120))
@item_max = $game_party.actors.size
self.contents = Bitmap.new(width - 32, (12 * 120))
refresh
self.active = false
self.index = -1
end
def update_cursor_rect
if @index < 0
self.cursor_rect.empty
else
if (@index * 116) < 348
self.cursor_rect.set(0, @index * 116, self.width - 32, 96)
else
self.cursor_rect.set(0, (@index - 3) * 116, self.width - 32, 96)
if (@index * 116) > 580
self.cursor_rect.set(0, (@index - 6) * 116, self.width - 32, 96)
if (@index * 116) > 928
self.cursor_rect.set(0, (@index - 9) * 116, self.width - 32, 96)
end
end
end
end
end
end
#----------------
def update_status
# If B button was pressed
if Input.trigger?(Input::B)
# Play cancel SE
$game_system.se_play($data_system.cancel_se)
# Make command window active
@player_menu.active = true
@status_window.active = false
@status_window.visible = false
return
end
# If C button was pressed
if Input.trigger?(Input::A) or Input.trigger?(Input::C)
# Branch by command window cursor position
case @player_menu.index
when 0 # status
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to status screen
$scene = Scene_Status.new(@status_window.index)
when 1 # equipment
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to equipment screen
$scene = Scene_Equip.new(@status_window.index)
when 2 # skill
# If this actor's action limit is 2 or more
if $game_party.actors[@status_window.index].restriction >= 2
# Play buzzer SE
$game_system.se_play($data_system.buzzer_se)
return
end
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to skill screen
$scene = Scene_Skill.new(@status_window.index)
end
end
if @status_window.index > 2
@status_window.oy = (116 * 3)
if @status_window.index > 5
@status_window.oy = (116 * 6)
if @status_window.index > 8
@status_window.oy = (116 * 9)
end
end
else
@status_window.oy = 0
end
end