ImmuneEntity
Sponsor
Every time I go through my game and test it, I eventually get the following error after a battle when the Battle Report is displayed.
Below is the code I am using for the Battle Report script. It is not the original; someone edited it for me at some point to allow me to select certain party members to not be listed in the Battle Report display. The line that crashes is line 339.
Note that to get this to work with my battle system, I did add a section of code. All I did was rename some class names. It certainly is probably not a good solution, but it's the only fix I could find at the time due to my lack of understanding of the RMXP scripts and Ruby itself. The code I added is just below.
Any help would be appreciated, for this is a very annoying random problem. I cannot see a pattern to when it happens, it just happens once in a very rare while and crashes the game. If for example I re-load my last save and fight the battle that made it crash over again, it does NOT crash the second time.
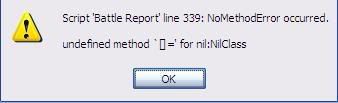
Below is the code I am using for the Battle Report script. It is not the original; someone edited it for me at some point to allow me to select certain party members to not be listed in the Battle Report display. The line that crashes is line 339.
Code:
#==============================================================================
# ** Battle Report v 1.6 by Illustrationism
# Â * Posted & Edited by Raziel
#==============================================================================
# Â A script that shows the battle result like in the Final Fantasy games.
#
# Â Features
# Â * Scrolling down Exp
#
# Â Edits:
# Â * Exp Bars filling up
# Â * Possible displaying of Facesets instead of Charactersets
# Â * Exp scrolling down faster, depending on how much exp you gain after battle.
# Â * Play a ME as long as you want in the result window.
#==============================================================================
Â
# EDIT
# Enlist here actor IDs who you do not wish to display on level-up window.
# Example as follows: (separate by commas)
TEMPORARY_ACTORS = [9, 10, 11]
Â
class Game_Actor < Game_Battler
 def exp=(exp)
  @exp = [[exp, 9999999].min, 0].max
  while @exp >= @exp_list[@level+1] and @exp_list[@level+1] > 0
   @level += 1
  Â
   # NEW - David
   $d_new_skill = nil
  Â
   for j in $data_classes[@class_id].learnings
    if j.level == @level
     learn_skill(j.skill_id)
  Â
     # NEW - David
     skill = $data_skills[j.skill_id]
     $d_new_skill = skill.name
  Â
    end
   end
  end
  while @exp < @exp_list[@level]
   @level -= 1
  end
  @hp = [@hp, self.maxhp].min
  @sp = [@sp, self.maxsp].min
 end
Â
 #--------------------------------------------------------------------------
 # * Get the current EXP
 #--------------------------------------------------------------------------
 def now_exp
  return @exp - @exp_list[@level]
 end
 #--------------------------------------------------------------------------
 # * Get the next level's EXP
 #--------------------------------------------------------------------------
 def next_exp
  return @exp_list[@level+1] > 0 ? @exp_list[@level+1] - @exp_list[@level] : 0
 end
Â
end
Â
class Window_LevelUp < Window_Base2
Â
 #----------------------------------------------------------------
 def initialize(actor, pos)
  #change this to false to show the actor's graphic
  @face = true
  @actor = actor
  y = (pos * 120)
  super(280, y, 360, 120)
  self.contents = Bitmap.new(width - 32, height - 32)
  self.back_opacity = 255  Â
  if $d_dum == false
   refresh
  end
 end
Â
 #----------------------------------------------------------------
 def dispose
  super
 end
Â
 #----------------------------------------------------------------
 def refresh
  return unless @actor.is_a?(Game_Actor)
  self.contents.clear
  self.contents.font.size = 18
  if @face == true
  draw_actor_face(@actor, 4, 0)
  else
  draw_actor_graphic(@actor, 50, 80)
  end
  draw_actor_name(@actor, 111, 0)
  draw_actor_level(@actor, 186, 0)
  show_next_exp = @actor.level == 99 ? "---" : "#{@actor.next_exp}"
  min_bar = @actor.level == 40 ? 1 : @actor.now_exp #EDIT by ImmuneEntity: replaced 99 with 40
  max_bar = @actor.level == 40 ? 1 : @actor.next_exp #EDIT by ImmuneEntity: replaced 99 with 40
  draw_slant_bar(115, 80, min_bar, max_bar, 190, 6, bar_color = Color.new(0, 100, 0, 255), end_color = Color.new(0, 255, 0, 255))
  self.contents.draw_text(115, 24, 300, 32, "Exp:#{@actor.now_exp}")
  self.contents.draw_text(115, 48, 300, 32, "Level Up:" + show_next_exp)
 end
Â
Â
 #----------------------------------------------------------------
 def level_up
  self.contents.font.color = system_color
  self.contents.draw_text(230, 48, 80, 32, "LEVEL UP!")
 end
 #-----------------------------------------------------------------
  def learn_skill(skill)
  self.contents.font.color = normal_color
  unless $d_new_skill == nil
   Audio.se_play("Audio/SE/105-Heal01")
   self.contents.draw_text(186, 24, 80, 32, "Learned:")
   self.contents.font.color = system_color
   self.contents.draw_text(261, 24, 90, 32, skill)
  end
 end
Â
Â
 #----------------------------------------------------------------
 def update
  super
 end
Â
end # of Window_LevelUp
Â
#=================================
#Window_EXP
# Written by: David Schooley
#=================================
Â
class Window_EXP < Window_Base2
Â
 #----------------------------------------------------------------
 def initialize(exp)
  super(0, 0, 280, 60)
  self.contents = Bitmap.new(width - 32, height - 32)
  self.back_opacity = 255
  refresh(exp)
 end
Â
 #----------------------------------------------------------------
 def dispose
  super
 end
Â
 #----------------------------------------------------------------
 def refresh(exp)
  self.contents.clear
  self.contents.font.color = system_color
  self.contents.draw_text(0, 0, 150, 32, "Exp Earned:")
  self.contents.font.color = normal_color
  self.contents.draw_text(180, 0, 54, 32, exp.to_s, 2)
 end
Â
 #----------------------------------------------------------------
 def update
  super
 end
Â
end # of Window_EXP
Â
#=================================
#Window_Money_Items
# Written by: David Schooley
#=================================
Â
class Window_Money_Items < Window_Base2
Â
 #----------------------------------------------------------------
 def initialize(money, treasures)
  @treasures = treasures
  super(0, 60, 280, 420)
  self.contents = Bitmap.new(width - 32, height - 32)
  self.back_opacity = 255
  refresh(money)
 end
Â
 #----------------------------------------------------------------
 def dispose
  super
 end
Â
 #----------------------------------------------------------------
 def refresh(money)
  @money = money
  self.contents.clear
 Â
  self.contents.font.color = system_color
  self.contents.draw_text(4, 4, 100, 32, "Items Found:")
  self.contents.font.color = normal_color
 Â
  y = 32
  for item in @treasures
   draw_item_name(item, 4, y)
   y += 32
  end
 Â
  cx = contents.text_size($data_system.words.gold).width
  self.contents.font.color = normal_color
  self.contents.draw_text(4, 340, 220-cx-2, 32, $game_party.gold.to_s, 2)
  self.contents.font.color = normal_color
  self.contents.draw_text(4, 300, 220-cx-2, 32, "+ " + @money.to_s, 2)
  self.contents.font.color = system_color
  self.contents.draw_text(124-cx, 340, cx + 100, 32, $data_system.words.gold, 2)
 end
Â
 def update
  super
 end
Â
end # of Window_Money_Items
Â
Â
class Scene_Battle
 alias raz_battle_report_main main
 alias raz_battle_report_be battle_end
Â
 def main
  # NEW - David
  #$battle_end = false
  @lvup_window = []
  @show_dummies = true # Show dummy windows or not?
  raz_battle_report_main
  # NEW - David
  @lvup_window = nil
  @level_up = nil
  @ch_stats = nil
  @ch_compare_stats = nil
  Audio.me_stop
 end
Â
 def battle_end(result)
  raz_battle_report_be(result)
  # NEW - David
  @status_window.visible = false
  @spriteset.dispose
Â
=begin  Â
  # Added for RTAB Patch version
  @actor_command_window.dispose
  @party_command_window.dispose
  @help_window.dispose
  @status_window.dispose
  @message_window.dispose
  # End of RTAB Patch Version
=end         Â
Â
  Graphics.transition
  if result == 0
   display_lv_up(@exp, @gold, @treasures)
   loop do
    Graphics.update
    Input.update
    if Input.trigger?(Input::C)
     break
    end
   end
   trash_lv_up
  end
Â
 end
 Â
 def start_phase5
  @phase = 5
  if $game_switches[12] == false #switch 12 is "don't play ME" (this line added by ImmuneEntity)
   $game_system.me_play($game_system.battle_end_me)
  end
  #$game_system.bgm_play($game_temp.map_bgm) commented out by ImmuneEntity
  exp = 0
  gold = 0
  treasures = []
  for enemy in $game_troop.enemies
   unless enemy.hidden
    exp += enemy.exp
    gold += enemy.gold
    if rand(100) < enemy.treasure_prob
     if enemy.item_id > 0
      treasures.push($data_items[enemy.item_id])
     end
     if enemy.weapon_id > 0
      treasures.push($data_weapons[enemy.weapon_id])
     end
     if enemy.armor_id > 0
      treasures.push($data_armors[enemy.armor_id])
     end
    end
   end
  end
  treasures = treasures[0..5]
Â
  # NEW - David
  @treasures = treasures
  @exp  = exp
  @gold = gold
Â
 Â
  for item in treasures
   case item
   when RPG::Item
    $game_party.gain_item(item.id, 1)
   when RPG::Weapon
    $game_party.gain_weapon(item.id, 1)
   when RPG::Armor
    $game_party.gain_armor(item.id, 1)
   end
  end
  @phase5_wait_count = 10
 end
Â
 def update_phase5
  if @phase5_wait_count > 0
   @phase5_wait_count -= 1
   if @phase5_wait_count == 0
   Â
    # NEW - David
    $game_temp.battle_main_phase = false    Â
   end
   return
  end
Â
  # NEW - David
   battle_end(0)
Â
 end
Â
 def display_lv_up(exp, gold, treasures)
 Â
  $d_dum = false
  d_extra = 0
  i = 0
  for actor in $game_party.actors
    # Fill up the Lv up windows
    # NEW - ONLY if s/he joins permanently
    next if TEMPORARY_ACTORS.include?(actor.id)
    [b]@lvup_window[i] = Window_LevelUp.new($game_party.actors[i], i)[/b]
    #@lvup_window[i] = Window_LevelUp.new(actor, i)
    i += 1
  end
 Â
 Â
  # Make Dummies
  if @show_dummies == true
   $d_dum = true
   for m in i..3
    @lvup_window[m] = Window_LevelUp.new(m, m)
   end
  end
 Â
  @exp_window = Window_EXP.new(exp)
  @m_i_window = Window_Money_Items.new(gold, treasures)
  @press_enter = nil
  gainedexp = exp
  @level_up = [0, 0, 0, 0]
  @d_new_skill = ["", "", "", ""]
  @d_breakout = false
  @m_i_window.refresh(gold)
  wait_for_OK
Â
  #@d_remember = $game_system.bgs_memorize (commented out by ImmuneEntity)
  Audio.bgs_play("Audio/SE/032-Switch01", 100, 300)
 Â
  # NEW - David
  max_exp = exp
  value = 28
  if exp < value
   value = exp
  end
  if value == 0
   value = 1
  end
  for n in 0..gainedexp - (max_exp / value)
   exp -= (max_exp / value)
   if @d_breakout == false
    Input.update
   end
  Â
   for i in 0...$game_party.actors.size
    actor = $game_party.actors[i]
    if actor.cant_get_exp? == false
     last_level = actor.level
     actor.exp += (max_exp / value)
     # Fill up the Lv up windows
     if @d_breakout == false
      @lvup_window[i].refresh unless @lvup_window[i] == nil
      @exp_window.refresh(exp)
     end
    Â
     if actor.level > last_level
      @level_up[i] = 5
      Audio.se_play("Audio/SE/056-Right02.ogg", 70, 150)
      if $d_new_skill
       @d_new_skill[i] = $d_new_skill
      end
     end
    Â
     if @level_up[i] == 0
      @d_new_skill[i] = ""
     end
    Â
     if @level_up[i] > 0
      @lvup_window[i].level_up
      if @d_new_skill[i] != ""
       @lvup_window[i].learn_skill(@d_new_skill[i])
      end
     end
    Â
     if Input.trigger?(Input::C) or exp <= 0
      @d_breakout = true
     end
    end
   Â
    if @d_breakout == false
     if @level_up[i] >0
      @level_up[i] -= 1
     end
     Graphics.update
    end
   end
  Â
   if @d_breakout == true
    for i in 0...$game_party.actors.size
     actor = $game_party.actors[i]
     if actor.cant_get_exp? == false
      actor.exp += exp
     end
    end
    exp = 0
    break
   end
  end
  Audio.bgs_stop
  #@d_remember = $game_system.bgs_restore (commented out by ImmuneEntity)
 Â
  for i in 0...$game_party.actors.size
   actor = $game_party.actors[i]
   @lvup_window[i].refresh unless @lvup_window[i] == nil
  end
  @exp_window.refresh(exp)
  Audio.se_play("Audio/SE/006-System06.ogg", 70, 150)
  $game_party.gain_gold(gold)
  @m_i_window.refresh(0)
  Graphics.update
 end
Â
 def trash_lv_up
  # NEW - David
  i=0
 Â
  for i in 0 ... 4
   @lvup_window[i].visible = false unless @lvup_window[i] == nil
  end
  @exp_window.visible = false
  @m_i_window.visible = false
  @lvup_window = nil
  @exp_window = nil
  @m_i_window = nil
 end
Â
 # Wait until OK key is pressed
 def wait_for_OK
  loop do
   Input.update
   Graphics.update
   if Input.trigger?(Input::C)
    break
   end
  end
 end
Â
end
Â
class Window_Base2 < Window
 def draw_actor_face(actor, x, y)
  bitmap = RPG::Cache.character("Faces/" + actor.character_name, actor.character_hue)
  self.contents.blt(x, y, bitmap, Rect.new(0,0,96,96))
 end Â
 #--------------------------------------------------------------------------
 # * Draw Slant Bar(by SephirothSpawn)
 #--------------------------------------------------------------------------
 def draw_slant_bar(x, y, min, max, width = 152, height = 6,
   bar_color = Color.new(150, 0, 0, 255), end_color = Color.new(255, 255, 60, 255))
  # Draw Border
  for i in 0..height
   self.contents.fill_rect(x + i, y + height - i, width + 1, 1, Color.new(50, 50, 50, 255))
  end
  # Draw Background
  for i in 1..(height - 1)
   r = 100 * (height - i) / height + 0 * i / height
   g = 100 * (height - i) / height + 0 * i / height
   b = 100 * (height - i) / height + 0 * i / height
   a = 255 * (height - i) / height + 255 * i / height
   self.contents.fill_rect(x + i, y + height - i, width, 1, Color.new(r, b, g, a))
  end
  # Draws Bar
  for i in 1..( (min / max.to_f) * width - 1)
   for j in 1..(height - 1)
    r = bar_color.red * (width - i) / width + end_color.red * i / width
    g = bar_color.green * (width - i) / width + end_color.green * i / width
    b = bar_color.blue * (width - i) / width + end_color.blue * i / width
    a = bar_color.alpha * (width - i) / width + end_color.alpha * i / width
    self.contents.fill_rect(x + i + j, y + height - j, 1, 1, Color.new(r, g, b, a))
   end
  end
 end
end
Â
Note that to get this to work with my battle system, I did add a section of code. All I did was rename some class names. It certainly is probably not a good solution, but it's the only fix I could find at the time due to my lack of understanding of the RMXP scripts and Ruby itself. The code I added is just below.
Code:
#This fixes the Battle Report so that the ATB does not interfere with the way it
#is displayed.
class Window_LevelUp < Window_Base2 #edit: 2 added by ImmuneEntity
 alias initialize_fix_buger1 initialize
 def initialize(actor, pos)
  initialize_fix_buger1(actor, pos)
  self.z=2000
 end
end
Â
class Window_EXP < Window_Base2 #edit: 2 added by ImmuneEntity
 alias initialize_fix_buger2 initialize
 def initialize(exp)
  initialize_fix_buger2(exp)
  self.z=2000
 end
end
Â
class Window_Money_Items < Window_Base2 #edit: 2 added by ImmuneEntity
 alias initialize_fix_buger3 initialize
 def initialize(money, treasures)
  initialize_fix_buger3(money, treasures)
  self.z=2000
 end
end
Any help would be appreciated, for this is a very annoying random problem. I cannot see a pattern to when it happens, it just happens once in a very rare while and crashes the game. If for example I re-load my last save and fight the battle that made it crash over again, it does NOT crash the second time.