version: 2.0
maker built for: RMXP
by: Brewmeister, Gubid, and Star
Description:
Day/Night system that goes from light to dark depending on the time of day. Clear lights that open up the darkness on the map instead of layering over darkness to make it look foggy, making it easier to place events as light sources. You are also able to use your own graphic and with further updates I will have different graphics for different lights. An onscreen clock appears and shows you the current time of day as well. Lag free with default scripts, tested with some but could cause some compatibility issues. Updated versions will include efforts to make compatible with most scripts.
Features:
- Gradual darkness and brightness change (won't even see it happen)
- Setting the speed for how long a day lasts (Normal time is set at 24 earth minutes = 24 game hours
- Switches to make screen dark for caves and screens darker for houses
- Setting the clock visible or invisible at start-up and by switch
- Setting the opacity of caves
- Setting the extra opacity of houses
- Starting hour and day
- Current hour and day recorded in variables
- Stopping the flow of time by starting and stopping the timer in event
- Restarting the timer at any time will start the timer at the time you left it off at.
- Lights are checked by putting light100 as a comment in an event
- Ability to change between standard and military time
- Onscreen images that show moon or sun
Screenshots:
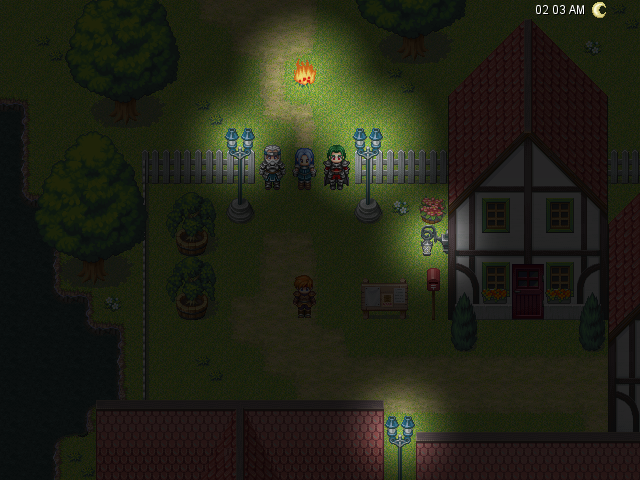
Demo:
Script:
Incase the demo is down.
Code:
=begin
---------------------------------------------
DAY_NIGHT_LIGHT_SYSTEM
---------------------------------------------
by
- Brewmeister
- Gubid
- Star
---------------------------------------------
=end
#-----------------------------------
# Editing of the Script
#-----------------------------------
# How many real time minutes does it take for a whole gameday to go by?
# Normal time would be 24 minutes so a minute in game goes by every second.
#-----------------------------------
GAME_DAY_IN_REAL_MINUTES = 24
# Current_Game_Day is the variable of the current day
#-----------------------------------
CURRENT_GAME_DAY = 15
# Day you want Game_Day to start at.
#-----------------------------------
INTIALIZE_GAME_DAY = 1
# If Flickercolon is true the : in the 00:00 flashes every second
#-----------------------------------
FLICKERCOLON = true
# Hour_variable is the variable of the current hour
#-----------------------------------
HOUR_VARIABLE = 9
# Inside_Switch is the switch set to make insides darker, not meant for caves
#-----------------------------------
INSIDE_SWITCH = 9
# Inside_Opacity is the opacity set to increase when entering insides
#-----------------------------------
INSIDE_OPACITY = 10
# Cave_Switch is the switch set meant for caves
#-----------------------------------
CAVE_SWITCH = 11
# Cave_Opacity is the total opacity you want when entering caves. Generally
# Should be set higher than the highest darkness.
#-----------------------------------
CAVE_OPACITY = 220
# if AM_AND_PM is true, everything is not in military time
#-----------------------------------
AM_AND_PM = true
# Starthour is the hour the game starts at
#-----------------------------------
STARTHOUR = 2
# New_hour is the variable that changes the current hour. You must use this in
# order to change the hour. It will not tell you the current hour, only
# Hour_variable can tell the current hour.
#-----------------------------------
NEW_HOUR = 10
# Set this switch to on in order to hide the clock
#-----------------------------------
INVISIBLE_TIMER_SWITCH = 10
#---------------------------------------------
#Bitmap
#---------------------------------------------
class Bitmap
def sub(ox,oy,light)
ox -= (light.width / 2)
oy -= (light.height / 2)
for x in 0...light.width
for y in 0...light.height
lt_color = light.get_pixel(x,y)
ds_color = self.get_pixel(x + ox,y + oy)
ds_color.alpha -= lt_color.alpha
self.set_pixel(x + ox,y + oy,ds_color)
end
end
end
end
#---------------------------------------------
#end Bitmap
#---------------------------------------------
#---------------------------------------------
#Game_System
#---------------------------------------------
class Game_System
alias init_gme_sys initialize
def initialize
init_gme_sys
@timer_working = true
@timer_remember = 0
@timer = STARTHOUR * 2400
end
def update
# reduce timer by 1
if @timer_working
if @timer > 57600
@timer = 0
$game_variables[CURRENT_GAME_DAY] += 1
else
if @timer_remember != 0 and @timer != @timer_remember
@timer = @timer_remember
@timer_remember = 0
else
@timer += (1 * (24/GAME_DAY_IN_REAL_MINUTES))
end
end
elsif @timer_remember != @timer and @timer_working == false
@timer_remember = @timer
end
end
end
#---------------------------------------------
#end Game_System
#---------------------------------------------
#---------------------------------------------
#Sprite_Timer
#---------------------------------------------
class Sprite_Timer < Sprite
def initialize
super
self.bitmap = Bitmap.new(120, 20)
self.bitmap.font.name = "Arial"
self.bitmap.font.size = 15
self.x = 620 - self.bitmap.width
self.y = 0
self.z = 2000
@flicker = true
if $game_variables[CURRENT_GAME_DAY] < INTIALIZE_GAME_DAY
$game_variables[CURRENT_GAME_DAY] = INTIALIZE_GAME_DAY
end
update
end
def update
super
# Set timer to visible if working
if $game_switches[INVISIBLE_TIMER_SWITCH] == true
self.visible = false
else
self.visible = true
end
if $game_variables[NEW_HOUR] != 0 and
$game_variables[NEW_HOUR] != $game_variables[HOUR_VARIABLE]
$game_system.timer = $game_variables[NEW_HOUR] * 2400
if $game_system.timer > 57600
$game_system.timer % 57600
$game_variables[CURRENT_GAME_DAY] += 1
end
$game_variables[NEW_HOUR] = 0
end
# If timer needs to be redrawn
if $game_system.timer / Graphics.frame_rate != @total_sec and $game_system.timer_working
# Clear window contents
self.bitmap.clear
# Calculate total number of seconds
@total_sec = $game_system.timer / Graphics.frame_rate
# Make a string for displaying the timer
case $game_variables[HOUR_VARIABLE]
when 0,1,2,3,4,5,18,19,20,21,22,23,24
moon_and_sun = RPG::Cache.picture("Moon")
cw = moon_and_sun.width
ch = moon_and_sun.height
rect = Rect.new(0, 0, cw, ch)
when 6,7,8,9,10,11,12,13,14,15,16,17
moon_and_sun = RPG::Cache.picture("Sun")
cw = moon_and_sun.width
ch = moon_and_sun.height
rect = Rect.new(0, 0, cw, ch)
end
if $game_variables[HOUR_VARIABLE] > 24
moon_and_sun = RPG::Cache.picture("Moon")
cw = moon_and_sun.width
ch = moon_and_sun.height
rect = Rect.new(0, 0, cw, ch)
end
if AM_AND_PM == true
if $game_variables[HOUR_VARIABLE] > 12
min = (@total_sec - 720) / 60
sec = @total_sec % 60
else
min = @total_sec / 60
sec = @total_sec % 60
end
if $game_variables[HOUR_VARIABLE] > 11 and $game_variables[HOUR_VARIABLE] != 24
setting = "PM"
else
setting = "AM"
end
if FLICKERCOLON == true and @flicker == false
text = sprintf("%02d %02d " + setting, min, sec)
@flicker = true
else
text = sprintf("%02d:%02d " + setting, min, sec)
if FLICKERCOLON == true
@flicker = false
end
end
else
min = @total_sec / 60
sec = @total_sec % 60
text = sprintf("%02d:%02d", min, sec)
end
if $game_variables[HOUR_VARIABLE] > 12 and AM_AND_PM == true
$game_variables[HOUR_VARIABLE] = min + 12
else
$game_variables[HOUR_VARIABLE] = min
end
$sec = sec
# Draw timer
rect2 = Rect.new(1, 1, 120, 20)
self.bitmap.font.color.set(0, 0, 0)
self.bitmap.draw_text(rect2, text, 1)
self.bitmap.font.color.set(255, 255, 255)
self.bitmap.draw_text(self.bitmap.rect, text, 1)
self.bitmap.blt(90, 0, moon_and_sun, rect)
end
end
end
#---------------------------------------------
#end Sprite_Timer
#---------------------------------------------
#---------------------------------------------
#Game_Event
#---------------------------------------------
class Game_Event < Game_Character
attr_reader :erased
attr_accessor :is_light
alias init_gm_evnt_lights initialize
def initialize(*args)
init_gm_evnt_lights(*args)
@is_light = false
end
alias refresh_gm_evnt_lights refresh
def refresh
tmp_page = @page
refresh_gm_evnt_lights
if tmp_page != @page
@is_light = false
end
end
end
#---------------------------------------------
#end Game_Event
#---------------------------------------------
#---------------------------------------------
#Sprite_Nighttime
#---------------------------------------------
class Nighttime < Sprite
def initialize(x,y)
super()
@width = $game_map.width * 32
@height = $game_map.height * 32
@lights = {}
self.x = x
self.y = y
self.bitmap = Bitmap.new(@width, @height)
self.bitmap.clear
fillnight
end
def refresh
self.bitmap.clear
create_lights
fillnight
end
def create_lights
for event in $game_map.events.values
unless event.erased or event.list == nil
for i in 0...event.list.size
if event.list[i].code == 108 #comment
if event.erased and event.list[i].parameters[0].include?("light")
@lights.delete(event)
next
elsif event.list[i].parameters[0].include?("light")
size = event.list[i].parameters[0].delete("light").to_i #convert text to interger
@lights[event] = Light.new(event, size) unless @lights.keys.include?(event)
event.is_light = true
next
end
end
end
#in the event page is changed and it is no longer a light.. remove it.
if @lights[event] != nil and event.is_light == false
@lights.delete(event)
end
end
end
end
def fillnight
if $sec == nil
$sec = 0
end
if $game_switches[INSIDE_SWITCH] == true
extralpha = INSIDE_OPACITY
else
extralpha = 0
end
case $game_variables[HOUR_VARIABLE]
when 0, 1, 23
startop = 210 - extralpha
when 2
startop = 210 - ($sec / 2) + extralpha
when 3
startop = 180 - ($sec / 2) + extralpha
when 4
startop = 150 - ($sec / 2) + extralpha
when 5
startop = 120 - ($sec / 2) + extralpha
when 6
startop = 90 - ($sec / 2) + extralpha
when 7
startop = 60 - ($sec / 2) + extralpha
when 8
startop = 30 - ($sec / 2) + extralpha
when 9, 10, 11, 12, 13, 14, 15
startop = 0 + extralpha
when 16
startop = 0 + ($sec / 2) + extralpha
when 17
startop = 30 + ($sec / 2) + extralpha
when 18
startop = 60 + ($sec / 2) + extralpha
when 19
startop = 90 + ($sec / 2) + extralpha
when 20
startop = 120 + ($sec / 2) + extralpha
when 21
startop = 150 + ($sec / 2) + extralpha
when 22
startop = 180 + ($sec / 2) + extralpha
end
if $game_switches[CAVE_SWITCH] == true
startop = CAVE_OPACITY
end
self.bitmap.fill_rect(0, 0, @width, @height, Color.new(0,0,0,255))
self.opacity = startop
# Now subtract out the lights
# Testing with a single light at tile 7,11
apply_lights
end
def apply_lights
applied_space = []
for light in @lights.values
image_sub = RPG::Cache.picture("LightSUB").clone
image_blt = RPG::Cache.picture("LightBLT").clone
base_rect = image_sub.rect
overlapLights = []
size = (light.size/100.0)
lwidth = base_rect.width*size
light_rect = Rect.new(light.x-(lwidth/2), light.y-(lwidth/2), lwidth, lwidth)
for applied_rect in applied_space
if light_rect.overlap?(applied_rect)
overlapLights << light_rect.area_in_overlap(applied_rect)
end
end
#now draw it
if overlapLights.size == 0 #no overlap detected.. then just BLT it into the image
self.bitmap.fill_rect(light_rect, Color.new(0,0,0,0))
self.bitmap.stretch_blt(light_rect, image_blt, base_rect)
else
self.bitmap.sub(light.x,light.y,image_sub)
end
applied_space << light_rect
image_sub.dispose
image_blt.dispose
end
end
def update
if $game_switches[INSIDE_SWITCH] == true
extralpha = INSIDE_OPACITY
else
extralpha = 0
end
case $game_variables[HOUR_VARIABLE]
when 0, 1, 23
self.opacity = 210 - extralpha
when 2
self.opacity = 210 - ($sec / 2) + extralpha
when 3
self.opacity = 180 - ($sec / 2) + extralpha
when 4
self.opacity = 150 - ($sec / 2) + extralpha
when 5
self.opacity = 120 - ($sec / 2) + extralpha
when 6
self.opacity = 90 - ($sec / 2) + extralpha
when 7
self.opacity = 60 - ($sec / 2) + extralpha
when 8
self.opacity = 30 - ($sec / 2) + extralpha
when 9, 10, 11, 12, 13, 14, 15
self.opacity = 0 + extralpha
when 16
self.opacity = 0 + ($sec / 2) + extralpha
when 17
self.opacity = 30 + ($sec / 2) + extralpha
when 18
self.opacity = 60 + ($sec / 2) + extralpha
when 19
self.opacity = 90 + ($sec / 2) + extralpha
when 20
self.opacity = 120 + ($sec / 2) + extralpha
when 21
self.opacity = 150 + ($sec / 2) + extralpha
when 22
self.opacity = 180 + ($sec / 2) + extralpha
end
if $game_switches[CAVE_SWITCH] == true
startop = CAVE_OPACITY
end
end
end
class Rect
def overlap?(rect)
corners = []
corners << [rect.x, rect.y] #UL
corners << [rect.x+rect.width, rect.y] #UR
corners << [rect.x+rect.width, rect.y+height] #LR
corners << [rect.x, rect.y+height] #LL
for corner in corners
if corner[0].between?(self.x, self.x+width) and corner[1].between?(self.y, self.y+height)
return true
end
end
return false
end
def area_in_overlap(rect)
return rect
end
end
class Light
def initialize(event, size)
@event = event
@size = size
end
def size
return @size
end
def x
return @event.real_x/4 + 16
end
def y
return @event.real_y/4 + 16
end
end
#---------------------------------------------
#end Sprite_Nighttime
#---------------------------------------------
#---------------------------------------------
#Spriteset_Map
#---------------------------------------------
class Spriteset_Map
alias init_spr_map_lights initialize
def initialize
# Make nighttime sprite
@nighttime = Nighttime.new(0,0)
@nighttime.z = 2000
init_spr_map_lights
@nighttime.refresh
@map_idd = $game_map.map_id
end
alias dns_dispose dispose
def dispose
dns_dispose
@nighttime.dispose
end
alias upd_spr_map_lights update
def update
upd_spr_map_lights
# Update nighttime sprite
@nighttime.ox = $game_map.display_x / 4
@nighttime.oy = $game_map.display_y / 4
if @hour_day != $game_variables[HOUR_VARIABLE]
$game_map.refresh
@hour_day = $game_variables[HOUR_VARIABLE]
end
if @map_idd != $game_map.map_id
@nighttime.refresh
@map_idd = $game_map.map_id
end
@nighttime.update
end
end
#---------------------------------------------
#end Spriteset_Map
#---------------------------------------------
Images:
Incase demo is down


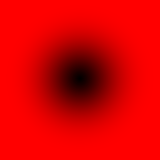
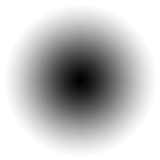
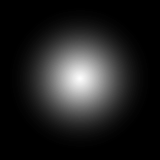
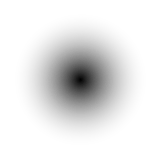
Instructions:
Place above main
in events with lights, make a comment that says "light" plus the size of the radius of the light. The normal size is 100 for bigger, use bigger numbers, smaller, smaller numbers. For example a normal light is "light100"
Terms and Conditions:
Free to use in any project, please give credit where it is due. Credit goes to Brewmeister, Gubid and Star