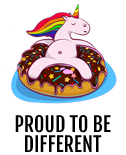
killerzkings":2hn1anis said:Use the search function next time when you request a script
There is a gameover to Inn script, so don't simply ask questions.
drerius":3irb00ab said:Revive on spot with penalty (lose item or whatever I chose idk how that works lol)