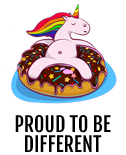
#--------------------------------------------------------------------------
# * Create Command Window
#--------------------------------------------------------------------------
def create_command_window
s1 = Vocab::item
s2 = Vocab::skill
s3 = Vocab::equip
s4 = Vocab::status
s5 = Vocab::save
s6 = Vocab::game_end
@command_window = Window_Command.new(160, [s1, s2, s3, s4, s5, s6])
@command_window.index = @menu_index
if $game_party.members.size == 0 # If number of party members is 0
@command_window.draw_item(0, false) # Disable item
@command_window.draw_item(1, false) # Disable skill
@command_window.draw_item(2, false) # Disable equipment
@command_window.draw_item(3, false) # Disable status
end
if $game_system.save_disabled # If save is forbidden
@command_window.draw_item(4, false) # Disable save
end
end
#--------------------------------------------------------------------------
# * Create Command Window
#--------------------------------------------------------------------------
def create_command_window
s1 = Vocab::item
s2 = Vocab::skill
s3 = Vocab::equip
s4 = Vocab::status
s5 = Vocab::save
s6 = Vocab::game_end
s7 = "Position"
@command_window = Window_Command.new(160, [s1, s2, s3, s4, s5, s6,s7])
@command_window.index = @menu_index
if $game_party.members.size == 0 # If number of party members is 0
@command_window.draw_item(0, false) # Disable item
@command_window.draw_item(1, false) # Disable skill
@command_window.draw_item(2, false) # Disable equipment
@command_window.draw_item(3, false) # Disable status
end
if $game_system.save_disabled # If save is forbidden
@command_window.draw_item(4, false) # Disable save
end
end
when 6
$scene = Scene_Position.new
case @command_window.index
when 0 # Item
$scene = Scene_Item.new
when 1,2,3 # Skill, equipment, status
start_actor_selection
when 4 # Save
$scene = Scene_File.new(true, false, false)
when 5 # End Game
$scene = Scene_End.new
class Scene_Position
def main
@spriteset = Spriteset_Map.new
make_command_window
make_position_window
@dummywindow = Window_Posdummy.new
Graphics.transition
loop do
Input.update
Graphics.update
update
if $scene != self
break
end
end
Graphics.freeze
@command_window.dispose
@position_window.dispose
@spriteset.dispose
@dummywindow.dispose
end
def update
if @command_window.active
update_command
end
if @position_window.active
update_number
end
end
def update_command
@command_window.update
if Input.trigger?(Input::C) || Input.trigger?(Input::RIGHT)
Sound.play_decision
@position_window.active = true
@actor = @command_window.index
@position_window.index = 4 - $game_party.members[@actor].odds
@command_window.active = false
@selection = true
end
if Input.trigger?(Input::B)
Sound.play_cancel
$scene = Scene_Menu.new(6)
end
end
def update_number
unless @selection
if Input.trigger?(Input::C)
Sound.play_decision
$game_party.members[@actor].odds = @position_window.index
@dummywindow.refresh
end
end
if Input.trigger?(Input::B) || Input.trigger?(Input::LEFT)
Sound.play_cancel
@position_window.active = false
@position_window.index = -1
@command_window.active = true
end
if @selection
@selection = false
end
@position_window.update
end
def make_command_window
@commands = [$game_actors[1].name, $game_actors[2].name, $game_actors[3].name,
$game_actors[4].name]
@command_window = Window_Command.new(160,@commands)
@command_window.x = 160
@command_window.y = 144
end
def make_position_window
@position_window = Window_Command.new(160,["Front","Middle","Rear"])
@position_window.index = -1
@position_window.x = 320
@position_window.y = 160
end
end
class Window_Posdummy < Window_Base
def initialize
super (0,0,640,480)
self.opacity = 0
refresh
end
def refresh
self.contents.clear
for actor in $game_party.members
position = 4 - actor.odds
draw_actor_face(actor, 40 - 20*position, actor.index * 96 + 2, 92)
end
end
end
#==============================================================================
# ** Game_Actor
#------------------------------------------------------------------------------
# This class handles actors. It's used within the Game_Actors class
# ($game_actors) and referenced by the Game_Party class ($game_party).
#==============================================================================
class Game_Actor < Game_Battler
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_accessor :odds
#--------------------------------------------------------------------------
# * Get Ease of Hitting
#--------------------------------------------------------------------------
def odds
if @odds == nil
@odds = 4 - self.class.position
end
return @odds
end
#--------------------------------------------------------------------------
# * Fake position counter
#--------------------------------------------------------------------------
def odds=(val)
@odds = 4 - val
end
end
class Scene_Position
def main
@spriteset = Spriteset_Map.new
make_command_window
make_position_window
@dummywindow = Window_Posdummy.new
Graphics.transition
loop do
Input.update
Graphics.update
update
if $scene != self
break
end
end
Graphics.freeze
@command_window.dispose
@position_window.dispose
@spriteset.dispose
@dummywindow.dispose
end
def update
if @command_window.active
update_command
end
if @position_window.active
update_number
end
end
def update_command
@command_window.update
if Input.trigger?(Input::C) || Input.trigger?(Input::RIGHT)
$game_system.se_play($data_system.decision_se)
@position_window.active = true
@actor = @command_window.index
@position_window.index = 4 - $game_party.actors[@actor].odds(@actor)
@command_window.active = false
@selection = true
end
if Input.trigger?(Input::B)
$game_system.se_play($data_system.cancel_se)
#change what's after "Scene_" to go to a different place upon exit
$scene = Scene_Map.new
end
end
def update_number
unless @selection
if Input.trigger?(Input::C)
$game_system.se_play($data_system.decision_se)
$game_party.actors[@actor].odds = @position_window.index
@dummywindow.refresh
end
end
if Input.trigger?(Input::B) || Input.trigger?(Input::LEFT)
$game_system.se_play($data_system.cancel_se)
@position_window.active = false
@position_window.index = -1
@command_window.active = true
end
if @selection
@selection = false
end
@position_window.update
end
def make_command_window
@commands = []
for actor in $game_party.actors
@commands.push(actor.name)
end
@command_window = Window_Command.new(160,@commands)
@command_window.x = 160
@command_window.y = 240 - (@command_window.height/2)
end
def make_position_window
@position_window = Window_Command.new(160,["Front","Middle","Rear"])
@position_window.index = -1
@position_window.x = 320
@position_window.y = 176
end
end
class Window_Posdummy < Window_Base
def initialize
super (0,0,640,480)
self.contents = Bitmap.new(width - 32, height - 32)
self.opacity = 0
refresh
end
def refresh
self.contents.clear
for actor in $game_party.actors
position = 4 - actor.odds(actor)
x = 60 - 20*position
y = actor.index * 50 + 180
bitmap = RPG::Cache.character(actor.character_name, actor.character_hue)
cw = bitmap.width / 4
ch = bitmap.height / 4
src_rect = Rect.new(0, 2*ch, cw, ch)
self.contents.blt(x - cw / 2, y - ch, bitmap, src_rect)
end
end
end
#==============================================================================
# ** Game_Actor
#------------------------------------------------------------------------------
# This class handles actors. It's used within the Game_Actors class
# ($game_actors) and referenced by the Game_Party class ($game_party).
#==============================================================================
class Game_Actor < Game_Battler
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_accessor :odds
#--------------------------------------------------------------------------
# * Get Ease of Hitting
#--------------------------------------------------------------------------
def odds(actor)
if @odds == nil
@odds = 4 - $data_classes[actor.class_id].position
end
return @odds
end
#--------------------------------------------------------------------------
# * Fake position counter
#--------------------------------------------------------------------------
def odds=(val)
@odds = 4 - val
end
end
n = 4 - position
n = self.actors[actor.index].odds(actor)
#==============================================================================
# ** Commands Manager
#------------------------------------------------------------------------------
# Author: Dargor
# Version 1.2
# 20/09/2007
#==============================================================================
# * Instructions
#------------------------------------------------------------------------------
# - To add a command to the command window use:
# @command_window.add_command("command")
# - To remove a command to the command window use:
# @command_window.remove_command("command")
# - To rearrange the commands in the command window use:
# @command_window.set_commands(["cmd1","cmd2","cmd3"...])
# - You can also rearrange the default menu commands using:
# $game_system.menu_commands = ["cmd1","cmd2","cmd3"...]
#------------------------------------------------------------------------------
# * Note
#------------------------------------------------------------------------------
# - This script rewrites the update_command and update_status methods
# in Scene_Menu.
#==============================================================================
#==============================================================================
# ** Game_System
#------------------------------------------------------------------------------
# This class handles data surrounding the system. Backround music, etc.
# is managed here as well. Refer to "$game_system" for the instance of
# this class.
#==============================================================================
class Game_System
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_accessor :default_commands # default commands
attr_accessor :menu_commands # menu commands
attr_accessor :commands_max # commands max
#--------------------------------------------------------------------------
# * Alias Listing
#--------------------------------------------------------------------------
alias dargor_menu_commands_initialize initialize
#--------------------------------------------------------------------------
# * Object Initialization
#--------------------------------------------------------------------------
def initialize
@default_commands = ["Item","Skill","Equip","Status","Save","End Game"]
@menu_commands = ["Item","Skill","Equip","Status","Save","End Game"]
@commands_max = 6
dargor_menu_commands_initialize
end
end
#==============================================================================
# ** Window_Command
#------------------------------------------------------------------------------
# This window deals with general command choices.
#==============================================================================
class Window_Command < Window_Selectable
#--------------------------------------------------------------------------
# * Public Instance Variables
#--------------------------------------------------------------------------
attr_accessor :commands # commands
#--------------------------------------------------------------------------
# * Alias Listing
#--------------------------------------------------------------------------
alias dargor_index_update update
alias dargor_disable_item disable_item
alias dargor_draw_item draw_item
#--------------------------------------------------------------------------
# * Add Command (command)
#--------------------------------------------------------------------------
def add_command(command)
return if @commands.include?(command)
@commands.push(command)
set_commands(@commands)
end
#--------------------------------------------------------------------------
# * Remove Command (command)
#--------------------------------------------------------------------------
def remove_command(command)
@commands.delete(command)
set_commands(@commands)
end
#--------------------------------------------------------------------------
# * Set Commands (commands)
#--------------------------------------------------------------------------
def set_commands(commands)
return if commands == [] or commands == nil
@commands = commands
@item_max = @commands.size
self.contents = Bitmap.new(width - 32, @item_max * 32)
refresh
self.height = 32 + (32 * $game_system.commands_max)
end
#--------------------------------------------------------------------------
# * Draw Item
# index : item number
# color : text color
#--------------------------------------------------------------------------
def draw_item(index, color)
return if index.nil?
dargor_draw_item(index, color)
end
#--------------------------------------------------------------------------
# * Frame Update
#--------------------------------------------------------------------------
def update
dargor_index_update
update_index
end
#--------------------------------------------------------------------------
# * Frame Update (index)
#--------------------------------------------------------------------------
def update_index
if self.index >= @commands.size
self.index = @commands.size-1
return
end
end
#--------------------------------------------------------------------------
# * Disable Item
# index : item number / item string
#--------------------------------------------------------------------------
def disable_item(index)
if index.is_a?(String)
new_index = @commands.index(index)
draw_item(new_index, disabled_color)
else
draw_item(index, disabled_color)
end
end
#--------------------------------------------------------------------------
# * Enable Item
# index : item number
#--------------------------------------------------------------------------
def enable_item(index)
draw_item(index, normal_color)
end
end
#==============================================================================
# ** Scene_Menu
#------------------------------------------------------------------------------
# This class performs menu screen processing.
#==============================================================================
class Scene_Menu
#--------------------------------------------------------------------------
# * Alias listing
#--------------------------------------------------------------------------
alias add_remove_command_main main
alias add_remove_command_update update
#--------------------------------------------------------------------------
# * Main Processing
#--------------------------------------------------------------------------
def main
# Default menu commands
@default_commands = $game_system.default_commands
# Create an empty array of command
@commands = []
# Call the original main method
add_remove_command_main
end
#--------------------------------------------------------------------------
# * Set Commands Access
#--------------------------------------------------------------------------
def set_commands_access
# Reinitialize menu commands
unless @command_window.commands == @commands
@command_window.set_commands($game_system.menu_commands)
end
# Se basic commands name
s1 = @default_commands[0]
s2 = @default_commands[1]
s3 = @default_commands[2]
s4 = @default_commands[3]
s5 = @default_commands[4]
# If number of party members is 0
if $game_party.actors.size == 0
# Disable items, skills, equipment, and status
@command_window.disable_item(s1)
@command_window.disable_item(s2)
@command_window.disable_item(s3)
@command_window.disable_item(s4)
end
# If save is forbidden
if $game_system.save_disabled
# Disable save
@command_window.disable_item(s5)
end
end
#--------------------------------------------------------------------------
# * Frame Update
#--------------------------------------------------------------------------
def update
# Set commands access
set_commands_access
# Set the array equal to the current commands
@commands = @command_window.commands
# Set commands access
# Call the original update method
add_remove_command_update
end
#--------------------------------------------------------------------------
# * Frame Update (when command window is active)
#--------------------------------------------------------------------------
def update_command
# Assign @command_window.index to its strings
command = @commands[@command_window.index]
# If B button was pressed
if Input.trigger?(Input::B)
# Play cancel SE
$game_system.se_play($data_system.cancel_se)
# Switch to map screen
$scene = Scene_Map.new
return
end
# If C button was pressed
if Input.trigger?(Input::C)
# If command other than save or end game, and party members = 0
if $game_party.actors.size == 0 and @command_window.index < 4
# Play buzzer SE
$game_system.se_play($data_system.buzzer_se)
return
end
# Branch by command window cursor position
case command
when @default_commands[0]
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to item screen
$scene = Scene_Item.new
when @default_commands[1]
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Make status window active
@command_window.active = false
@status_window.active = true
@status_window.index = 0
when @default_commands[2]
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Make status window active
@command_window.active = false
@status_window.active = true
@status_window.index = 0
when @default_commands[3]
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Make status window active
@command_window.active = false
@status_window.active = true
@status_window.index = 0
when @default_commands[4]
# If saving is forbidden
if $game_system.save_disabled
# Play buzzer SE
$game_system.se_play($data_system.buzzer_se)
return
end
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to save screen
$scene = Scene_Save.new
when @default_commands[5]
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to end game screen
$scene = Scene_End.new
end
return
end
end
#--------------------------------------------------------------------------
# * Frame Update (when status window is active)
#--------------------------------------------------------------------------
def update_status
# Assign @command_window.index to its strings
command = @commands[@command_window.index]
# If B button was pressed
if Input.trigger?(Input::B)
# Play cancel SE
$game_system.se_play($data_system.cancel_se)
# Make command window active
@command_window.active = true
@status_window.active = false
@status_window.index = -1
return
end
# If C button was pressed
if Input.trigger?(Input::C)
# Branch by command window cursor position
case command
when @default_commands[1]
# If this actor's action limit is 2 or more
if $game_party.actors[@status_window.index].restriction >= 2
# Play buzzer SE
$game_system.se_play($data_system.buzzer_se)
return
end
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to skill screen
$scene = Scene_Skill.new(@status_window.index)
when @default_commands[2]
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to equipment screen
$scene = Scene_Equip.new(@status_window.index)
when @default_commands[3]
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to status screen
$scene = Scene_Status.new(@status_window.index)
end
return
end
end
end
#==============================================================================
# ** Scene_Menu
#------------------------------------------------------------------------------
# This class performs menu screen processing.
#==============================================================================
class Scene_Menu
#--------------------------------------------------------------------------
# * Object Initialization
# menu_index : command cursor's initial position
#--------------------------------------------------------------------------
def initialize(menu_index = 0)
@menu_index = menu_index
end
#--------------------------------------------------------------------------
# * Main Processing
#--------------------------------------------------------------------------
def main
# Make command window
s1 = $data_system.words.item
s2 = $data_system.words.skill
s3 = $data_system.words.equip
s4 = "Status"
s5 = "Save"
s6 = "End Game"
@command_window = Window_Command.new(160, [s1, s2, s3, s4, s5, s6])
@command_window.index = @menu_index
# If number of party members is 0
if $game_party.actors.size == 0
# Disable items, skills, equipment, and status
@command_window.disable_item(0)
@command_window.disable_item(1)
@command_window.disable_item(2)
@command_window.disable_item(3)
end
# If save is forbidden
if $game_system.save_disabled
# Disable save
@command_window.disable_item(4)
end
# Make play time window
@playtime_window = Window_PlayTime.new
@playtime_window.x = 0
@playtime_window.y = 224
# Make steps window
@steps_window = Window_Steps.new
@steps_window.x = 0
@steps_window.y = 320
# Make gold window
@gold_window = Window_Gold.new
@gold_window.x = 0
@gold_window.y = 416
# Make status window
@status_window = Window_MenuStatus.new
@status_window.x = 160
@status_window.y = 0
# Execute transition
Graphics.transition
# Main loop
loop do
# Update game screen
Graphics.update
# Update input information
Input.update
# Frame update
update
# Abort loop if screen is changed
if $scene != self
break
end
end
# Prepare for transition
Graphics.freeze
# Dispose of windows
@command_window.dispose
@playtime_window.dispose
@steps_window.dispose
@gold_window.dispose
@status_window.dispose
end
#--------------------------------------------------------------------------
# * Frame Update
#--------------------------------------------------------------------------
def update
# Update windows
@command_window.update
@playtime_window.update
@steps_window.update
@gold_window.update
@status_window.update
# If command window is active: call update_command
if @command_window.active
update_command
return
end
# If status window is active: call update_status
if @status_window.active
update_status
return
end
end
#--------------------------------------------------------------------------
# * Frame Update (when command window is active)
#--------------------------------------------------------------------------
def update_command
# If B button was pressed
if Input.trigger?(Input::B)
# Play cancel SE
$game_system.se_play($data_system.cancel_se)
# Switch to map screen
$scene = Scene_Map.new
return
end
# If C button was pressed
if Input.trigger?(Input::C)
# If command other than save or end game, and party members = 0
if $game_party.actors.size == 0 and @command_window.index < 4
# Play buzzer SE
$game_system.se_play($data_system.buzzer_se)
return
end
# Branch by command window cursor position
case @command_window.index
when 0 # item
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to item screen
$scene = Scene_Item.new
when 1 # skill
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Make status window active
@command_window.active = false
@status_window.active = true
@status_window.index = 0
when 2 # equipment
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Make status window active
@command_window.active = false
@status_window.active = true
@status_window.index = 0
when 3 # status
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Make status window active
@command_window.active = false
@status_window.active = true
@status_window.index = 0
when 4 # save
# If saving is forbidden
if $game_system.save_disabled
# Play buzzer SE
$game_system.se_play($data_system.buzzer_se)
return
end
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to save screen
$scene = Scene_Save.new
when 5 # end game
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to end game screen
$scene = Scene_End.new
end
return
end
end
#--------------------------------------------------------------------------
# * Frame Update (when status window is active)
#--------------------------------------------------------------------------
def update_status
# If B button was pressed
if Input.trigger?(Input::B)
# Play cancel SE
$game_system.se_play($data_system.cancel_se)
# Make command window active
@command_window.active = true
@status_window.active = false
@status_window.index = -1
return
end
# If C button was pressed
if Input.trigger?(Input::C)
# Branch by command window cursor position
case @command_window.index
when 1 # skill
# If this actor's action limit is 2 or more
if $game_party.actors[@status_window.index].restriction >= 2
# Play buzzer SE
$game_system.se_play($data_system.buzzer_se)
return
end
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to skill screen
$scene = Scene_Skill.new(@status_window.index)
when 2 # equipment
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to equipment screen
$scene = Scene_Equip.new(@status_window.index)
when 3 # status
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to status screen
$scene = Scene_Status.new(@status_window.index)
end
return
end
end
end
# Make command window
s1 = $data_system.words.item
s2 = $data_system.words.skill
s3 = $data_system.words.equip
s4 = "Status"
s5 = "Save"
s6 = "End Game"
@command_window = Window_Command.new(160, [s1, s2, s3, s4, s5, s6])
# Make command window
s1 = $data_system.words.item
s2 = $data_system.words.skill
s3 = $data_system.words.equip
s4 = "Status"
s5 = "Save"
s6 = "End Game"
s7 = "Position"
@command_window = Window_Command.new(160, [s1, s2, s3, s4, s5, s6,s7])
when 5 # end game
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to end game screen
$scene = Scene_End.new
when 6 # Position menu
# Play decision SE
$game_system.se_play($data_system.decision_se)
# Switch to positioning scene
$scene = Scene_Position.new