Crazyninjaguy
Member
Battle/Map BGM Selector - By Crazyninjaguy
Heya!
Time for me to post my second ever script!
Basically, it allows you to choose your favourite Music and either play it on the map, or play it in battle.
It's that simple really. The script itself is plug'n'play so all you have to do is paste it in a new script section, but i reccomend downloading the demo.
Screenshots:
Script:
Demo:
http://www.mediafire.com/?sharekey=f38405da97d00006111096d429abd360e04e75f6e8ebb871
Hope you enjoy my second ever script! Please give credit if used as it took me a while to write this ( I'm still learning :D )
Heya!
Time for me to post my second ever script!
Basically, it allows you to choose your favourite Music and either play it on the map, or play it in battle.
It's that simple really. The script itself is plug'n'play so all you have to do is paste it in a new script section, but i reccomend downloading the demo.
Screenshots:
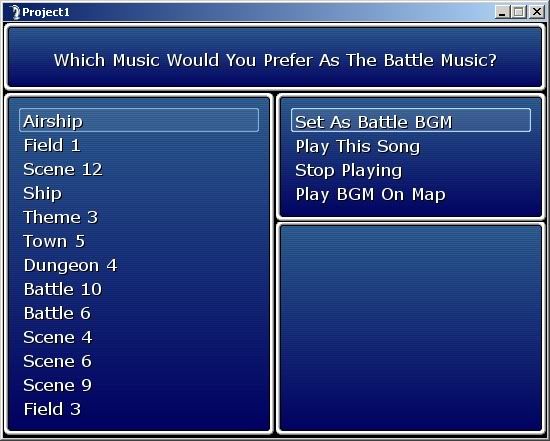
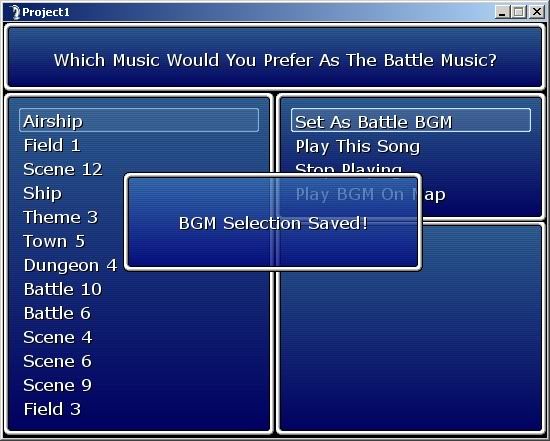
Script:
Code:
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
# * Battle BGM Selector by Crazyninjaguy
# http://www.planetdev.co.nr
# --------------------------------------------------------------------------
# ** Allows you to choose the battle music from the menu
# --------------------------------------------------------------------------
# *** Features
# -- Preview BGM before setting as battle bgm or map bgm
# -- Stop playing the BGM
# -- Set your favourite as the battle theme
# -- Play your favourite BGM on the map
# --------------------------------------------------------------------------
# *** Editable Fields
# -- Lines 87-99. The names of the BGM/Music. NOT THE FILENAME!
# -- Lines 174,182,190,198,206,214,222,230,238,246,254,262,270
# EXACT FILENAMES! CASE SENSITIVE!
# --------------------------------------------------------------------------
# *** Notes
# -- This is my second ever script so don't expect too much lol.
# -- Please give credit if you use this in your game.
# -- If anything goes wrong with the script then don't hesitate to PM
# me on my site [url=http://www.planetdev.co.nr]http://www.planetdev.co.nr[/url]
# -- Also if you have any ideas on how i could improve the script,
# please PM me on my site [url=http://www.planetdev.co.nr]http://www.planetdev.co.nr[/url]
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
module Music_Selection
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
# * Constants
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
MUSIC_LABEL = 'Music'
MENU_INDEX = 4
end
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
# * This window simply displays text
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
class Window_Text < Window_Base
def initialize
super(0, 0, 544, 70)
self.contents = Bitmap.new(width - 32, height - 32)
update
end
def update
self.contents.clear
self.contents.draw_text(-50, 0, 612, 42, "Which Music Would You Prefer As The Battle Music?", 1)
end
end
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
# * Pop-up window showing that BGM choice was saved
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
class Window_Saved < Window_Base
def initialize
super(120, 150, 300, 100)
self.contents = Bitmap.new(width - 32, height - 32)
update
end
def update
self.contents.clear
self.contents.draw_text(0, 0, 268, 68, "BGM Selection Saved!", 1)
end
end
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
# * Space filling window
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
class Window_Misc < Window_Base
def initialize
super(272, 198, 272, 216)
self.contents = Bitmap.new(width - 32, height - 32)
update
end
def update
self.contents.clear
self.contents.draw_text(0, 0, 544, 416, "Badger!", 1)
end
end
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
# * The options for the songs
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
class Window_Options < Window_Selectable
def initialize
super(272, 70, 272, 130)
@commands = ["Set As Battle BGM", "Play This Song", "Stop Playing", "Play BGM On Map"]
@item_max = 4
self.contents = Bitmap.new(width - 32, height - 32)
refresh
end
def refresh
self.contents.clear
for i in [email=0...@commands.size]0...@commands.size[/email]
x = 4
y = i * 24
self.contents.draw_text(x, y, 272, 27, @commands[i])
end
end
end
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
# * The main scene, nothing works without this!
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
class Scene_MusicSelect
def initialize(menu_index = 0)
@menu_index = menu_index
end
def main
# Make Misc Window
@window_misc = Window_Misc.new
# Make Command Window
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
# * Edit name of song (Not Filename)
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
s1 = "Airship"
s2 = "Field 1"
s3 = "Scene 12"
s4 = "Ship"
s5 = "Theme 3"
s6 = "Town 5"
s7 = "Dungeon 4"
s8 = "Battle 10"
s9 = "Battle 6"
s10 = "Scene 4"
s11 = "Scene 6"
s12 = "Scene 9"
s13 = "Field 3"
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
# * End Of Editing
#=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=
@command_window = Window_Command.new(272, [s1, s2, s3, s4, s5, s6, s7, s8, s9, s10, s11, s12, s13])
@command_window.index = @menu_index
@command_window.x = 0
@command_window.y = 70
# Make Text Window
@text_window = Window_Text.new
# Make Options Window
@options_window = Window_Options.new
# Execute transition
Graphics.transition
# Main loop
loop do
# Update game screen
Graphics.update
# Update input information
Input.update
# Frame update
update
# Abort loop if screen is changed
if $scene != self
break
end
end
# Prepare for transition
Graphics.freeze
# Dispose of windows
@command_window.dispose
@text_window.dispose
@options_window.dispose
@window_misc.dispose
end
def update
@command_window.update
@text_window.update
@options_window.update
@window_misc.update
# If command window is active: call update_command
if @command_window.active
update_command
return
end
# If options window is active: call update_options
if @options_window.active
update_options
return
end
end
end
def update_command
# If B button was pressed
if Input.trigger?(Input::B)
# Play cancel SE
Sound.play_cancel
# Stop playing music
Audio.bgm_stop
# Switch to menu screen
$scene = Scene_Map.new
return
end
# If C button was pressed
if Input.trigger?(Input::C)
# Branch by command window cursor position
case @command_window.index
when 0
# Play decision SE
Sound.play_decision
# Make options window active
@command_window.active = false
@options_window.active = true
@options_window.index = 0
@bgm = "Airship" # Filename, Case Sensitive
when 1
# Play decision SE
Sound.play_decision
# Make options window active
@command_window.active = false
@options_window.active = true
@options_window.index = 0
@bgm = "Field1" # Filename, Case Sensitive
when 2
# Play decision SE
Sound.play_decision
# Make options window active
@command_window.active = false
@options_window.active = true
@options_window.index = 0
@bgm = "Scene12" # Filename, Case Sensitive
when 3
# Play decision SE
Sound.play_decision
# Make options window active
@command_window.active = false
@options_window.active = true
@options_window.index = 0
@bgm = "Ship" # Filename, Case Sensitive
when 4
# Play decision SE
Sound.play_decision
# Make options window active
@command_window.active = false
@options_window.active = true
@options_window.index = 0
@bgm = "Theme3" # Filename, Case Sensitive
when 5
# Play decision SE
Sound.play_decision
# Make options window active
@command_window.active = false
@options_window.active = true
@options_window.index = 0
@bgm = "Town5" # Filename, Case Sensitive
when 6
# Play decision SE
Sound.play_decision
# Make options window active
@command_window.active = false
@options_window.active = true
@options_window.index = 0
@bgm = "Dungeon4" # Filename, Case Sensitive
when 7
# Play decision SE
Sound.play_decision
# Make options window active
@command_window.active = false
@options_window.active = true
@options_window.index = 0
@bgm = "Battle10" # Filename, Case Sensitive
when 8
# Play decision SE
Sound.play_decision
# Make options window active
@command_window.active = false
@options_window.active = true
@options_window.index = 0
@bgm = "Battle6" # Filename, Case Sensitive
when 9
# Play decision SE
Sound.play_decision
# Make options window active
@command_window.active = false
@options_window.active = true
@options_window.index = 0
@bgm = "Scene4" # Filename, Case Sensitive
when 10
# Play decision SE
Sound.play_decision
# Make options window active
@command_window.active = false
@options_window.active = true
@options_window.index = 0
@bgm = "Scene6" # Filename, Case Sensitive
when 11
# Play decision SE
Sound.play_decision
# Make options window active
@command_window.active = false
@options_window.active = true
@options_window.index = 0
@bgm = "Scene9" # Filename, Case Sensitive
when 12
# Play decision SE
Sound.play_decision
# Make options window active
@command_window.active = false
@options_window.active = true
@options_window.index = 0
@bgm = "Field3" # Filename, Case Sensitive
end
return
end
end
def update_options
# If B button was pressed
if Input.trigger?(Input::B)
# Play cancel SE
Sound.play_cancel
# Make command window active
@command_window.active = true
@options_window.active = false
@options_window.index = -1
return
end
# If C button was pressed
if Input.trigger?(Input::C)
# Branch by command window cursor position
case @options_window.index
when 0 # Set As Battle BGM
# Play decision SE
Sound.play_decision
$game_system.battle_bgm = RPG::BGM.new(@bgm)
Window_Saved.new
Graphics.wait(60)
Audio.bgm_stop
$scene = Scene_Map.new
when 1 # Play Song
# Play decision SE
Sound.play_decision
Audio.bgm_play("Audio/BGM/" + @bgm, 100, 100)
when 2 # Stop Playing
# Play decision SE
Sound.play_decision
Audio.bgm_stop
when 3 # Play On Map
# Play decision SE
Sound.play_decision
Audio.bgm_play("Audio/BGM/" + @bgm, 100, 100)
$scene = Scene_Map.new
end
return
end
end
#==============================================================================
# ** Scene_Menu
#~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
# Summary of Changes:
# aliased methods - initialize, create_command_window, update_command_selection
#==============================================================================
class Scene_Menu < Scene_Base
#~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
# * Object Initialization
# menu_index : command cursor's initial position
#~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
alias music_select initialize
def initialize(menu_index = 0)
music_select (menu_index)
if @menu_index == 'Music'
@menu_index = Music_Selection::MENU_INDEX
elsif @menu_index >= Music_Selection::MENU_INDEX
@menu_index += 1
end
end
#~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
# * Create Command Window
#~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
alias music_select_menu_cmmnd_win_create create_command_window
def create_command_window
music_select_menu_cmmnd_win_create
c = @command_window.commands
c.insert (Music_Selection::MENU_INDEX, Music_Selection::MUSIC_LABEL)
width = @command_window.width
@command_window.dispose
@command_window = Window_Command.new(width, c)
@command_window.index = @menu_index
end
#~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
# * Update Command Selection
#~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
alias music_select_menu_cmmnd_select_upd update_command_selection
def update_command_selection
if @command_window.index == Music_Selection::MENU_INDEX && Input.trigger? (Input::C)
# Open Music Window
Sound.play_decision
$scene = Scene_MusicSelect.new
end
return
end
#~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
# * Update Actor Selection
#~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
alias music_select_actor_selection_upd update_actor_selection
def update_actor_selection
changed = false
if @command_window.index > Music_Selection::MENU_INDEX
@command_window.index = (@command_window.index - 1) % @command_window.commands.size
changed = true
end
music_select_actor_selection_upd
@command_window.index = (@command_window.index + 1) % @command_window.commands.size if changed
end
end
Demo:
http://www.mediafire.com/?sharekey=f38405da97d00006111096d429abd360e04e75f6e8ebb871
Hope you enjoy my second ever script! Please give credit if used as it took me a while to write this ( I'm still learning :D )