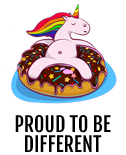
class Window_Location < Window_Base
def initialize
super(0, 0, 240, 60)
self.contents = Bitmap.new(width - 32, height - 32)
self.z = 9999
refresh
end
def refresh
self.contents.clear
text = $game_map.name
cx = contents.text_size(text).width
self.contents.font.color = shadow_color
self.contents.draw_text(1, 5, 120, 32, text, 2)
self.contents.font.color = normal_color
self.contents.draw_text(0, 4, 120, 32, text, 2)
end
def update
super
refresh
end
end
location_window = Window_Location.new
class My_Window < Window_Base
end
class My_Window < Window_Base
def initialize
super(50,100,520,192)
self.contents = Bitmap.new(width - 32, height - 32)
end
end
class My_Window < Window_Base
def initialize
super(50,100,520,192)
self.contents = Bitmap.new(width - 32, height - 32)
refresh
end
def refresh
self.contents.clear
text = 'my text'
self.contents.draw_text(0, 0, 250, 32, text)
end
end